Game development is a complex field that demands careful consideration when selecting the right programming language. Performance, control over hardware, and efficient resource management are critical factors influencing this decision. So, why C++ is the best choice in the game development industry? In this article, we’ll explore the reasons behind its popularity, deep delve into technical comparisons with other languages, and provide code examples to illustrate how C++ empowers developers to create high-performance games.
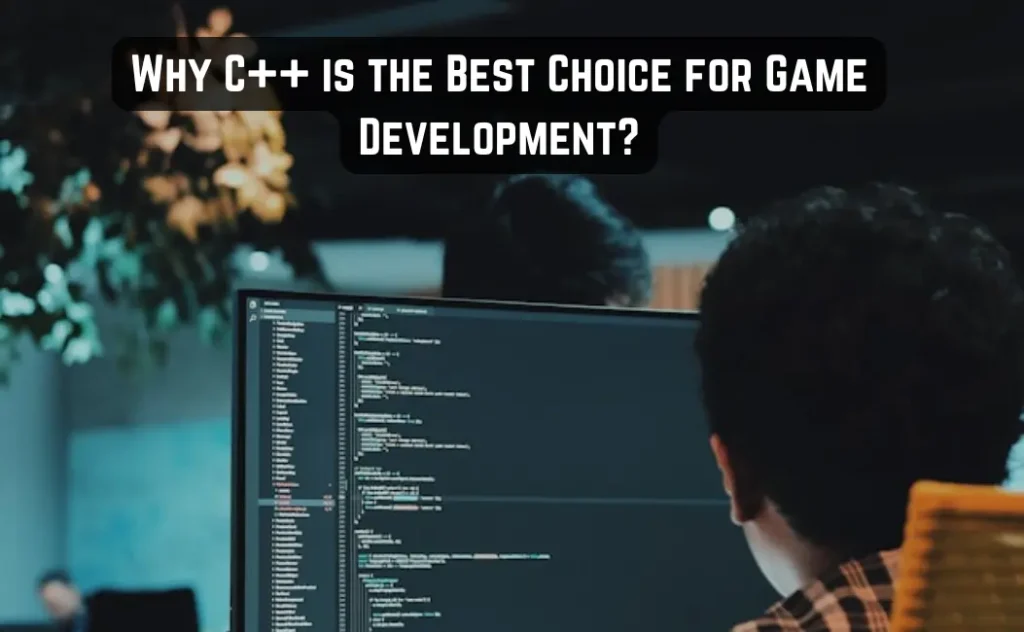
Table of Contents
- The Importance of Performance in Game Development
- Comparing Programming Languages for Game Development
- Deep Dive into C++
- Object-Oriented Programming and Code Reusability
- Platform Dependency and Cross-Platform Development
- Community and Industry Support
- Challenges of Using C++
- Conclusion
Performance in Game Development
In game development, performance is important. Games require real-time processing of graphics, physics simulations, artificial intelligence, and user input. Any lag or stutter can significantly impact the user experience. Therefore, developers need a language that can handle intensive computations efficiently.
C++ is renowned for its speed and flexibility. Being a compiled language, it translates code directly into machine language, enabling faster execution compared to interpreted languages. This efficiency is crucial for maintaining high frame rates and responsive gameplay.
2. Comparing Programming Languages for Game Development
Let’s see how C++ stacks up against other popular programming languages used in game development.
C++
- Performance: High, due to compiled code and manual memory management.
- Control: Offers fine-grained control over system resources.
- Portability: Highly portable with the right compilers.
- Use Cases: Both 2D and 3D games, AAA titles, and performance-critical applications.
C#
- Performance: Good, but slightly slower than C++ due to the .NET runtime.
- Control: Automatic memory management via garbage collection.
- Portability: Primarily Windows-focused but can be cross-platform with tools like Mono.
- Use Cases: Widely used with Unity Engine for both 2D and 3D games.
Key Differences Between C++ and C#:
- Memory Management:
- C++: Manual memory management.
- C#: Automatic garbage collection.
- Platform Dependency:
- C++: Platform-independent with appropriate compilers.
- C#: Historically Windows-centric, but cross-platform support is improving.
- Pointers:
- C++: Full support for pointers.
- C#: Limited use of pointers, only in unsafe contexts.
Java
- Performance: Decent, but may suffer due to the Java Virtual Machine (JVM) overhead.
- Control: Automatic memory management.
- Portability: Highly portable via the JVM.
- Use Cases: Mobile games (Android), indie games.
Key Differences Between C++ and Java:
- Memory Management:
- C++: Manual.
- Java: Automatic garbage collection.
- Platform Dependency:
- C++: Platform-independent with compilers.
- Java: Platform-independent via the JVM.
- Pointers:
- C++: Full pointer arithmetic.
- Java: Limited, only references; no pointer arithmetic.
JavaScript, Python, and Lua
- Performance: Generally slower than compiled languages.
- Control: High-level languages with automatic memory management.
- Portability: Highly portable.
- Use Cases: Web-based games (JavaScript), scripting in game engines (Lua), educational and indie games (Python).
3. Deep Dive into C++
C++ provides several features that make it ideal for game development, especially when performance and control are priorities.
Manual Memory Management
In C++, developers have direct control over memory allocation and deallocation. While this requires more responsibility, it allows for fine-tuned optimizations that can significantly improve game performance.
Pros:
- Optimized resource usage.
- No unexpected garbage collection pauses.
Cons:
- Increased complexity.
- Risk of memory leaks if not managed properly.
Pointers
Pointers are variables that store memory addresses. They are powerful tools in C++ that enable efficient manipulation of data structures and memory.
Example: Using Pointers in C++
#include <iostream>
void pointerExample() {
int var = 42;
// Declare a pointer variable
int* ptr;
// Assign the address of var to ptr
ptr = &var;
// Output the value of var using the pointer
std::cout << "Value of var: " << var << std::endl;
std::cout << "Value at *ptr: " << *ptr << std::endl;
// Modify var using the pointer
*ptr = 100;
std::cout << "New value of var: " << var << std::endl;
}
int main() {
pointerExample();
return 0;
}
Output:
Value of var: 42
Value at *ptr: 42
New value of var: 100
Explanation:
int* ptr;
declares a pointer to an integer.ptr = &var;
assigns the address ofvar
toptr
.*ptr
accesses the value at the memory address stored inptr
.- Modifying
*ptr
changes the value ofvar
.
Therefore, pointers provide a way to directly manipulate variables in memory, which is essential for optimizing game performance.
Dynamic Memory Allocation
Dynamic memory allocation allows the program to request memory at runtime. This is essential for creating flexible data structures like dynamic arrays or linked lists.
Example: Dynamic Memory Allocation in C++
#include <iostream>
int main() {
int n;
std::cout << "Enter the number of elements: ";
std::cin >> n;
// Dynamically allocate memory for an array of n integers
int* array = new int[n];
// Check if memory has been successfully allocated
if (array == nullptr) {
std::cout << "Memory allocation failed!" << std::endl;
return 1;
}
// Input elements
for (int i = 0; i < n; ++i) {
std::cout << "Enter element " << i + 1 << ": ";
std::cin >> array[i];
}
// Display elements
std::cout << "You entered: ";
for (int i = 0; i < n; ++i) {
std::cout << array[i] << " ";
}
std::cout << std::endl;
// Deallocate memory
delete[] array;
return 0;
}
Explanation:
new int[n];
allocates memory forn
integers.- Always check if the allocation was successful.
- Use
delete[] array;
to deallocate the memory and prevent memory leaks.
Thus, dynamic memory allocation gives developers the flexibility to manage memory efficiently based on the game’s needs.
Avoiding Memory Leaks
Memory leaks occur when dynamically allocated memory is not deallocated. In long-running applications like games, memory leaks can cause increased memory usage and eventually crash the program.
Best Practices to Avoid Memory Leaks:
- Always pair
new
withdelete
andnew[]
withdelete[]
. - Use smart pointers (
std::unique_ptr
,std::shared_ptr
) from C++11 onwards. - Regularly test and profile your code for memory leaks.
Example: Using Smart Pointers
#include <iostream>
#include <memory>
int main() {
int n;
std::cout << "Enter the number of elements: ";
std::cin >> n;
// Use smart pointer for automatic memory management
std::unique_ptr<int[]> array(new int[n]);
// Input elements
for (int i = 0; i < n; ++i) {
std::cout << "Enter element " << i + 1 << ": ";
std::cin >> array[i];
}
// Display elements
std::cout << "You entered: ";
for (int i = 0; i < n; ++i) {
std::cout << array[i] << " ";
}
std::cout << std::endl;
// Memory is automatically deallocated when unique_ptr goes out of scope
return 0;
}
Explanation:
std::unique_ptr
automatically deallocates memory when it goes out of scope.- Helps prevent memory leaks without manual
delete[]
calls.
Consequently, smart pointers can significantly reduce the risk of memory leaks in C++ programs.
4. Object-Oriented Programming and Code Reusability
C++ is an object-oriented programming (OOP) language, which is advantageous in game development due to:
- Code Reusability: Classes and objects allow developers to reuse code efficiently.
- Modularity: Helps in organizing complex projects.
- Inheritance and Polymorphism: Enable flexible and maintainable code structures.
Example: Using Classes in C++
#include <iostream>
class Enemy {
public:
Enemy(int h) : health(h) {}
void takeDamage(int damage) {
health -= damage;
std::cout << "Enemy takes " << damage << " damage. Health now: " << health << std::endl;
}
private:
int health;
};
int main() {
Enemy goblin(100);
goblin.takeDamage(30);
goblin.takeDamage(50);
return 0;
}
Output:
Enemy takes 30 damage. Health now: 70
Enemy takes 50 damage. Health now: 20
Therefore, OOP in C++ facilitates the creation of complex game entities with reusable and maintainable code.
5. Platform Dependency and Cross-Platform Development
C++ can be compiled on various platforms, making it a versatile choice for cross-platform game development.
- C++: Platform-independent code with platform-specific compilers.
- C#: Historically tied to Windows, but cross-platform support via .NET Core and Mono.
- Java: Runs on any platform with the JVM, but JVM overhead can affect performance.
Thus, C++ offers flexibility in targeting multiple platforms without significant changes to the codebase.
6. Community and Industry Support
C++ has robust community and industry support, which includes:
- Game Engines: Major engines like Unreal Engine and CryEngine are built with C++.
- Libraries and Frameworks: Extensive libraries for graphics (OpenGL, DirectX), physics (Bullet, Box2D), and more.
- Community: A vast community with decades of accumulated knowledge, tutorials, and documentation.
Therefore, developers have access to a wealth of resources and tools when using C++ for game development.
7. Challenges of Using C++
While C++ offers numerous advantages, it also presents challenges:
- Steep Learning Curve: Complex syntax and concepts like pointers and manual memory management.
- Risk of Memory Leaks: Requires diligent memory management practices.
- Longer Development Time: More code and debugging needed compared to higher-level languages.
However, with proper education and practice, these challenges can be mitigated.
8. Conclusion
C++ remains a top choice for game development due to its unmatched performance, control over system resources, and support for object-oriented programming. While it demands a higher level of responsibility from developers, the benefits in terms of game performance and flexibility are significant.
Choosing the right programming language depends on the project’s specific needs. For developers aiming to create high-performance, resource-intensive games that require fine-tuned optimization, C++ is an excellent choice.