Are you preparing for a Web API interview? This comprehensive guide is designed to help you crack the interview with a practical understanding and problem-solving skills in real-world API design and maintenance questions. Whether you’re an experienced developer or a fresher, this article delves deeply into Web API concepts- questions and answers.
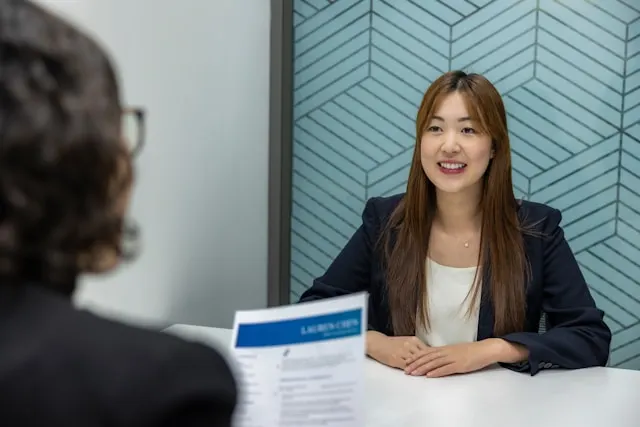
Web API Interview Questions and Answers
1. What is a Web API, and how does it differ from a web service?
2. What are the main HTTP methods in Web APIs, and how are they typically used?
3. What is REST, and what are its key constraints?
4. Explain the concept of RESTful API and how it differs from SOAP-based APIs.
5. What are API endpoints, and how are they structured?
6. What is CORS, and why is it critical in API security?
7. What is the purpose of HTTP status codes in API responses?
8. How does OAuth 2.0 differ from OAuth 1.0a?
9. What is JWT (JSON Web Token), and why is it commonly used for API authentication?
10. What is rate limiting, and how does it protect an API?
11. Explain the concept of versioning in APIs and common strategies for implementing it.
12. What are HATEOAS and its role in RESTful APIs?
13. What is GraphQL, and how does it differ from REST?
14. Describe API idempotency and its importance.
15. What is content negotiation, and how is it handled in APIs?
16. How does an API gateway function in an API architecture?
17. What are webhooks, and how do they differ from polling?
18. Explain the difference between synchronous and asynchronous API calls.
19. What are the benefits and drawbacks of using OpenAPI Specification for API documentation?
20. How do you ensure backward compatibility in APIs?
21. What is a Throttling mechanism, and how does it work in Web APIs?
22. How do you implement pagination in Web APIs?
23. What are the common security threats for Web APIs, and how can you mitigate them?
24. What is the Richardson Maturity Model for RESTful APIs?
25. How do you handle large file uploads in APIs efficiently?
26. Explain circuit breaking and its role in resilient API design.
27. How can caching improve API performance, and what caching strategies are common?
28. What is the role of API testing, and what tools are commonly used?
29. How do you handle errors and exceptions in API responses?
30. What is an API contract, and why is it critical?
31. How does gRPC differ from RESTful APIs?
32. What is a reverse proxy, and why is it used in API architecture?
1. What is a Web API, and how does it differ from a web service?
Answer:
A Web API is an interface that allows interaction between different software applications over the web. Unlike traditional web services (e.g., SOAP), Web APIs often follow RESTful principles and work directly over HTTP without the need for additional messaging protocols.
2. What are the main HTTP methods in Web APIs, and how are they typically used?
Answer:
Key HTTP methods include GET (retrieves data), POST (creates new resources), PUT (updates or replaces resources), DELETE (removes resources), and PATCH (updates specific fields of resources).
3. What is REST, and what are its key constraints?
Answer:
REST (Representational State Transfer) is an architectural style with constraints like statelessness, client-server architecture, cacheability, uniform interface, layered system, and optionally, code on demand.
4. Explain the concept of RESTful API and how it differs from SOAP-based APIs.
Answer:
RESTful APIs follow REST principles and are lightweight and flexible, using JSON over HTTP. SOAP APIs are protocol-based, more rigid, and rely on XML for messaging.
5. What are API endpoints, and how are they structured?
Answer:
API endpoints are specific paths in an API where resources are exposed, structured using a base URL and paths representing resource hierarchies (e.g., /api/users/{id}
).
6. What is CORS, and why is it critical in API security?
Answer:
CORS (Cross-Origin Resource Sharing) controls which origins are allowed to access API resources to prevent unauthorized access and cross-site attacks.
7. What is the purpose of HTTP status codes in API responses?
Answer:
HTTP status codes indicate the result of an API request, helping clients understand if the request succeeded (e.g., 200 OK), failed (e.g., 400 Bad Request), or requires further action (e.g., 401 Unauthorized).
8. How does OAuth 2.0 differ from OAuth 1.0a?
Answer:
OAuth 2.0 provides token-based authorization with a simpler flow, offering enhanced security and scalability, while OAuth 1.0a uses signatures, making it more complex but potentially more secure for certain use cases.
9. What is JWT (JSON Web Token), and why is it commonly used for API authentication?
Answer:
JWT is a compact, self-contained token format used for secure data transmission. It contains claims that verify user identity, allowing stateless authentication.
10. What is rate limiting, and how does it protect an API?
Answer:
Rate limiting controls the number of requests a client can make within a time frame to prevent abuse and ensure fair usage of API resources.
11. Explain the concept of versioning in APIs and common strategies for implementing it.
Answer:
Versioning allows APIs to evolve without breaking existing clients. Common strategies include URL versioning (e.g., /v1/resource
), header versioning, and query parameter versioning.
12. What are HATEOAS and its role in RESTful APIs?
Answer:
HATEOAS (Hypermedia as the Engine of Application State) means that resources provide links to related actions, enabling clients to navigate the API dynamically.
13. What is GraphQL, and how does it differ from REST?
Answer:
GraphQL is a query language for APIs allowing clients to specify the exact data they need, minimizing over-fetching or under-fetching, whereas REST provides fixed endpoints.
14. Describe API idempotency and its importance.
Answer:
Idempotency ensures that multiple identical requests have the same effect, crucial for methods like PUT and DELETE to prevent unintended actions.
15. What is content negotiation, and how is it handled in APIs?
Answer:
Content negotiation enables clients to specify response formats (e.g., JSON, XML) via headers (e.g., Accept
header) for flexible content delivery.
16. How does an API gateway function in an API architecture?
Answer:
An API gateway serves as a single entry point for API requests, handling tasks like load balancing, rate limiting, and security.
17. What are webhooks, and how do they differ from polling?
Answer:
Webhooks allow servers to push data to clients in real-time when specific events occur, unlike polling, where clients repeatedly request updates.
18. Explain the difference between synchronous and asynchronous API calls.
Answer:
Synchronous calls block until a response is received, while asynchronous calls return immediately, allowing other tasks to proceed.
19. What are the benefits and drawbacks of using OpenAPI Specification for API documentation?
Answer:
OpenAPI provides standard, structured documentation, aiding development and testing, though it requires upfront planning to maintain accuracy.
20. How do you ensure backward compatibility in APIs?
Answer:
Backward compatibility is achieved through versioning, deprecation warnings, and avoiding breaking changes in responses or request structures.
21. What is a Throttling mechanism, and how does it work in Web APIs?
Answer:
Throttling limits request rates from clients, typically implemented to manage load, prevent abuse, and ensure fair resource distribution.
22. How do you implement pagination in Web APIs?
Answer:
Pagination uses parameters like limit
and offset
or page
and size
to break down large responses into manageable parts.
23. What are the common security threats for Web APIs, and how can you mitigate them?
Answer:
Common threats include SQL injection, XSS, CSRF, and brute-force attacks, mitigated by input validation, strong authentication, and secure headers.
24. What is the Richardson Maturity Model for RESTful APIs?
Answer:
This model categorizes REST APIs into levels (0 to 3), assessing their RESTfulness based on criteria like URI usage, resources, and hypermedia controls.
25. How do you handle large file uploads in APIs efficiently?
Answer:
Chunking uploads, setting size limits, and using multipart forms are ways to handle large file uploads while optimizing server performance.
26. Explain circuit breaking and its role in resilient API design.
Answer:
Circuit breaking temporarily halts requests to failing services, improving stability and allowing time for recovery, essential in microservice architectures.
27. How can caching improve API performance, and what caching strategies are common?
Answer:
Caching reduces server load and response time by storing frequently accessed data. Common strategies include client-side, server-side, and CDN caching.
28. What is the role of API testing, and what tools are commonly used?
Answer:
API testing ensures functionality, reliability, and security. Tools like Postman, SoapUI, and JMeter are commonly used.
29. How do you handle errors and exceptions in API responses?
Answer:
Use standard status codes, error messages, and structured error responses (e.g., JSON error objects) to help clients understand and resolve issues.
30. What is an API contract, and why is it critical?
Answer:
An API contract defines the expected request/response format, enabling consistent integration and reducing integration errors.
31. How does gRPC differ from RESTful APIs?
Answer:
gRPC is a high-performance RPC framework that uses HTTP/2 and Protocol Buffers for serialization, offering better speed and efficiency compared to JSON over HTTP used in REST.
32. What is a reverse proxy, and why is it used in API architecture?
Answer:
A reverse proxy routes client requests to servers, enhancing security, load distribution, and performance in API deployments.
Learn More: Carrer Guidance [Web API interview questions and answers]
57 Functional testing interview questions and answers
Spring MVC interview questions and answers
Laravel Interview Questions and Answers- Basic to Advanced
Data analyst interview questions and answers