A NullReferenceException
in Unity is like a red flag telling you, “Hey, you’re trying to use something that doesn’t exist yet!” This pesky runtime error happens when your code attempts to access a variable or component that hasn’t been initialized. Whether you’re working on Unity 2022 or the latest Unity 6, this error is still one of the most common—and fixable—issues developers face.
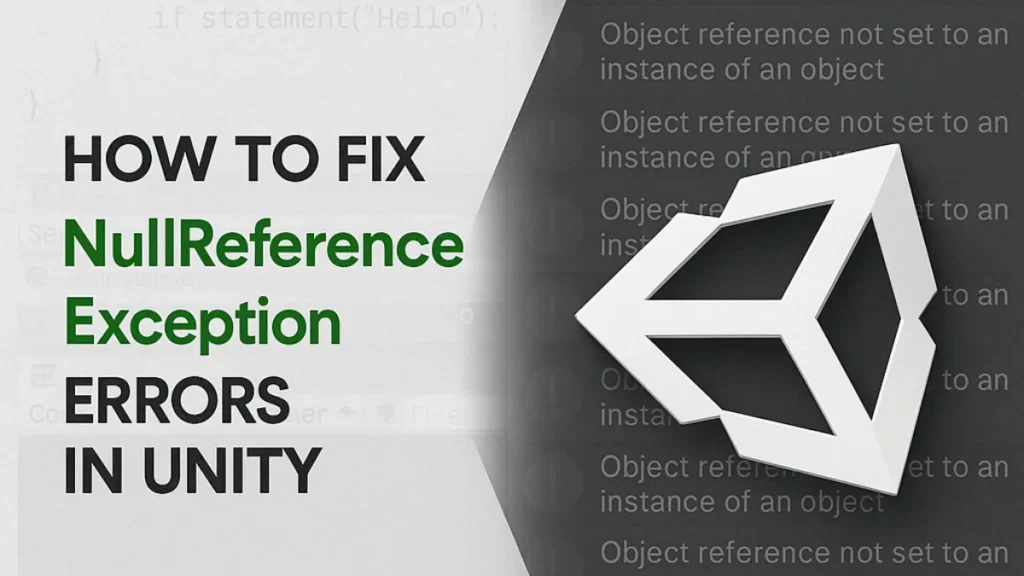
Let’s break down what it is, why it happens, and the five proven ways to fix it in 2025, based on real user cases and the official Unity guide.
What Causes a NullReferenceException?
Before jumping to fixes, understand the core issue:
You get a NullReferenceException
when you try to access members of an object that hasn’t been assigned. For example:
Rigidbody rb;
rb.velocity = new Vector3(0, 10, 0); // Error! rb is still null
Common Scenarios:
- Accessing a public field that wasn’t set in the Inspector.
- Calling methods on a component that wasn’t found by
GetComponent
. - Referring to a destroyed object.
- Forgetting to assign references in
Awake()
,Start()
, or scripts.
1. Double-Check Object Assignments in the Inspector
Why This Matters: Unity won’t throw a compile-time error if a public or [SerializeField]
variable isn’t assigned— but it will throw a NullReferenceException
at runtime.
How to Fix:
- Select the GameObject using the script.
- Look in the Inspector for missing fields.
- Assign the proper references (e.g., drag the required GameObject or Component).
Tip: Use
[SerializeField] private
instead ofpublic
to reduce accidental mismanagement.
2. Use Safe GetComponent<>()
with Checks
Why This Happens: When you use GetComponent<T>()
and the component doesn’t exist on that object, Unity returns null
.
How to Fix:
Renderer rend = GetComponent<Renderer>();
if (rend != null)
{
rend.enabled = true;
}
else
{
Debug.LogWarning("Renderer not found on this GameObject.");
}
Real-World Case:
In one issue, the Renderer visible;
field in the Fullblock and Emptyblock visibility scripts wasn’t assigned. Since visible
was never set via GetComponent
, the if (Block.Isempty)
check led to a NullReferenceException
.
3. Initialize Variables in Start()
or Awake()
Why It Works: Unity’s lifecycle functions ensure objects are initialized properly. If you depend on runtime assignments, use Start()
or Awake()
to set up references.
Example Fix:
void Awake()
{
visible = GetComponent<Renderer>(); // Initialize here
}
Avoid using variables before they’re initialized. Many errors arise because initialization happens after access.
4. Implement Null Checks Before Use
Defensive Coding Works:
Checking for null
before using an object prevents crashes and provides cleaner debug messages.
Sample:
if (myObject != null)
{
myObject.DoSomething();
}
else
{
Debug.LogError("myObject is null. Check your Inspector or initialization.");
}
This is especially useful when objects might be destroyed mid-game or removed dynamically.
5. Update Unity Packages (Especially Version Control)
Why This Is Crucial: In Unity 2022 and Unity 6, many NullReferenceException
errors come from bugs in Editor tools or outdated packages (e.g., SpriteLibraryEditorWindow, NavigationOverlay).
Fix Steps:
- Open Window → Package Manager.
- Find the Version Control or problematic package.
- Click Update to latest version.
- Clear the Console and test again.
A user experienced persistent
NullReferenceException
inSpriteLibraryEditorWindow.OnDestroy()
. Updating the 2D Animation package fixed the issue immediately.
Bonus: What Cannot Cause NullReferenceException?
- Value types like
int
,float
,bool
, and structs. - These types hold their value directly and don’t reference objects—so you’ll never get a null error from them.
Summary: 5-Step Checklist
Step | Fix | When to Use |
---|---|---|
1 | Check Inspector Assignments | Public/Serialized fields |
2 | Use GetComponent + Null Checks | Dynamically linked components |
3 | Initialize in Start() or Awake() | Script-controlled references |
4 | Use defensive if (x != null) checks | Runtime object conditions |
5 | Update Unity packages | Editor/runtime version issues |
Final Thoughts
A NullReferenceException
might seem frustrating, but it’s a helpful warning—telling you something is missing in your logic or setup. Whether you’re a beginner or building large Unity projects in 2025, mastering these five steps will keep your console clean and gameplay smooth.
Need help with a specific error log? Drop your script or message, and I’ll debug it with you.