Node.js enables developers to create scalable and high-performance applications using JavaScript, a language traditionally confined to the client-side. With companies increasingly relying on Node.js for back-end services, proficiency in this technology is now a sought-after skill in the job market.
This article offers an extensive list of Nodejs interview questions and answers that will help you succeed in your next job interview.
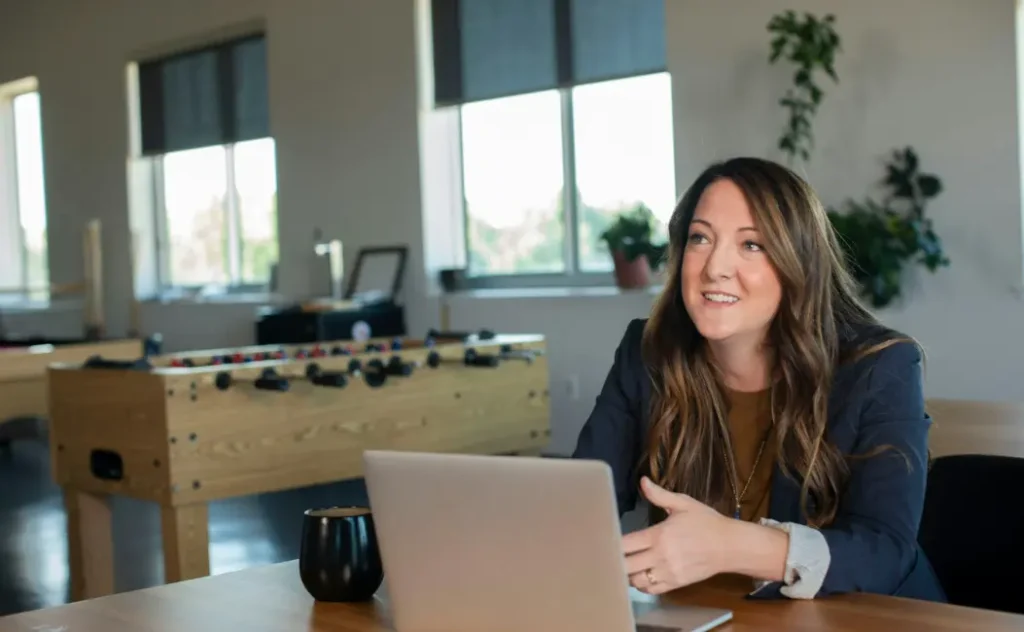
Nodejs interview questions and answers
1. What is Node.js?
2. What is the main difference between Node.js and JavaScript?
3. What are the main advantages of using Node.js?
4. What is the event-driven architecture in Node.js?
5. Explain the concept of the Event Loop in Node.js.
6. What is the difference between process.nextTick() and setImmediate()?
7. What are Streams in Node.js?
8. Explain the difference between fs.readFile() and fs.createReadStream().
9. What is the purpose of require() in Node.js?
10. What is the purpose of module.exports?
11. What are the core modules in Node.js?
12. Explain the purpose of middleware in Node.js.
13. What is the difference between synchronous and asynchronous methods in Node.js?
14. What is a callback function in Node.js?
15. What are promises in Node.js?
16. Explain async/await in Node.js.
17. What is clustering in Node.js?
18. What is the purpose of the child_process module in Node.js?
19. What is the difference between spawn() and exec() in the child_process module?
20. What is the purpose of the buffer class in Node.js?
21. What is Express.js?
22. What is the difference between PUT and PATCH methods in REST APIs?
23. How do you create a basic HTTP server in Node.js?
24. What is Express.js and why is it commonly used in Node.js applications?
25. How do you handle routing in a Node.js application using Express.js?
26. How do you connect to a database (e.g., MongoDB) from a Node.js application?
27. What are some common database design patterns for Node.js applications?
28. What techniques can you use to improve the performance of a Node.js application?
29. How does Node.js handle concurrency and scalability?
30. What tools and techniques do you use for testing Node.js applications?
31. How do you debug Node.js applications effectively?
32. What security best practices should be followed in Node.js applications?
33. What is WebSockets and how can you use them in Node.js applications?
34. What is GraphQL and how does it differ from REST APIs?
35. How do you implement server-side rendering (SSR) in a Node.js application?
36. What are some common design patterns used in Node.js applications?
37. How do you handle errors and exceptions in Node.js applications?
38. What is the difference between Node.js and Deno?
39. What are some of the latest trends and innovations in the Node.js ecosystem?
40. How would you handle error handling in Node.js?
41. What is CORS in Node.js?
42. What is JWT (JSON Web Token) and how does it work in Node.js?
43. What is the difference between npm and npx?
44. How do you manage environments in Node.js applications?
1. What is Node.js?
Answer:
Node.js is an open-source, cross-platform runtime environment that allows developers to execute JavaScript on the server side. It uses the V8 JavaScript engine to compile and run JavaScript code outside of a browser.
2. What is the main difference between Node.js and JavaScript?
Answer:
JavaScript is a programming language that runs in the browser, whereas Node.js is a runtime environment for running JavaScript outside of the browser, mainly for server-side applications.
3. What are the main advantages of using Node.js?
Answer:
- Fast execution with the V8 engine
- Non-blocking I/O model
- Cross-platform compatibility
- Suitable for real-time applications
- Scalable and lightweight
4. What is the event-driven architecture in Node.js?
Answer:
In Node.js, operations are non-blocking and event-driven. When an operation (like file reading) is triggered, Node.js executes a callback function when the event (such as completing the operation) occurs.
5. Explain the concept of the Event Loop in Node.js.
Answer:
The event loop is the core mechanism in Node.js that handles asynchronous operations. It continuously checks for pending callbacks and executes them once an event completes, allowing non-blocking I/O operations.
6. What is the difference between process.nextTick() and setImmediate()?
Answer:
process.nextTick()
: Executes the callback function immediately after the current operation completes, before any I/O events.setImmediate()
: Executes the callback in the next iteration of the event loop, after I/O events.
7. What are Streams in Node.js?
Answer:
Streams are instances of EventEmitter and allow working with continuous data. They are used for handling large volumes of data, such as file streams or network communication. Types of streams include readable, writable, duplex, and transform streams.
8. Explain the difference between fs.readFile()
and fs.createReadStream()
.
Answer:
fs.readFile()
: Reads the entire file into memory before returning it, which is inefficient for large files.fs.createReadStream()
: Streams the file content, allowing the file to be read in chunks, thus improving performance for large files.
9. What is the purpose of require()
in Node.js?
Answer:
require()
is used to import modules, JSON, or local files into a Node.js file. It’s the primary way to include and reuse code across files and modules.
10. What is the purpose of module.exports?
Answer:
module.exports
is used to expose functions, objects, or variables from a module to be used in other files.
11. What are the core modules in Node.js?
Answer: Some core modules are:
http
: For creating HTTP serversfs
: File system module for handling file operationspath
: For handling file and directory pathsos
: Provides operating system-related utility functionsevents
: For handling events
12. Explain the purpose of middleware in Node.js.
Answer:
Middleware functions in Node.js are functions that have access to the request object, response object, and the next middleware function. They are used in frameworks like Express.js to handle request processing, logging, authentication, and error handling.
13. What is the difference between synchronous and asynchronous methods in Node.js?
Answer:
- Synchronous methods block the execution of subsequent code until the current operation completes.
- Asynchronous methods do not block the execution, allowing other operations to continue while waiting for the current task to complete.
14. What is a callback function in Node.js?
Answer:
A callback function is a function passed as an argument to another function, which will be called once the asynchronous operation completes.
15. What are promises in Node.js?
Answer:
Promises are objects representing the eventual completion or failure of an asynchronous operation. They provide a cleaner way to handle asynchronous operations compared to callbacks by using then()
, catch()
, and finally()
.
16. Explain async/await in Node.js.
Answer:
async
and await
are syntactic sugar for working with promises. async
makes a function return a promise, while await
pauses the execution of the function until the promise is resolved or rejected.
17. What is clustering in Node.js?
Answer:
Clustering allows Node.js to create multiple instances of the application (workers) to take advantage of multi-core systems, improving the app’s performance by handling more requests concurrently.
18. What is the purpose of the child_process
module in Node.js?
Answer:
The child_process
module is used to spawn new processes and execute commands. It provides different methods to create child processes such as exec()
, spawn()
, and fork()
.
19. What is the difference between spawn()
and exec()
in the child_process
module?
Answer:
spawn()
: Launches a new process and streams the output in real-time, suitable for large outputs.exec()
: Buffers the output and returns it in a callback after the process finishes, suitable for smaller outputs.
20. What is the purpose of the buffer
class in Node.js?
Answer:
The Buffer
class is used to handle binary data in Node.js, especially when dealing with file I/O, network packets, or other types of streams.
21. What is Express.js?
Answer:
Express.js is a fast, minimalist web framework for Node.js, used to create web applications and APIs. It simplifies handling routes, requests, responses, middleware, and more.
22. What is the difference between PUT and PATCH methods in REST APIs?
Answer:
- PUT: Replaces the entire resource with the provided data.
- PATCH: Partially updates the resource with the provided data.
23. How do you create a basic HTTP server in Node.js?
Answer:
You can use the built-in http
module to create a basic HTTP server. This involves creating a server instance and listening for incoming requests.
24. What is Express.js and why is it commonly used in Node.js applications?
Answer:
Express.js is a popular web framework for Node.js that provides a robust set of features for building web applications. It simplifies common tasks like routing, middleware, and template rendering.
25. How do you handle routing in a Node.js application using Express.js?
Answer:
Routing in Express.js involves defining routes that map HTTP methods (GET, POST, PUT, DELETE) to specific handlers.
26. How do you connect to a database (e.g., MongoDB) from a Node.js application?
Answer:
You can use a database driver like mongoose
to connect to MongoDB from Node.js.
27. What are some common database design patterns for Node.js applications?
Answer:
Common database design patterns include schema design, indexing, and query optimization.
28. What techniques can you use to improve the performance of a Node.js application?
Answer:
Techniques include caching, code optimization, and using asynchronous operations effectively.
Answer:
29. How does Node.js handle concurrency and scalability?
Node.js uses a non-blocking, event-driven architecture that allows it to handle a large number of concurrent connections efficiently.
30. What tools and techniques do you use for testing Node.js applications?
Answer:
Popular testing tools include Jest, Mocha, and Chai.
31. How do you debug Node.js applications effectively?
Answer:
Debugging techniques include using a debugger, logging, and inspecting error messages.
32. What security best practices should be followed in Node.js applications?
Answer:
Security best practices include input validation, output encoding, using secure libraries, and preventing cross-site scripting (XSS) and SQL injection.
33. What is WebSockets and how can you use them in Node.js applications?
Answer:
WebSockets provide a full-duplex communication channel between a client and a server. They are useful for building real-time applications.
34. What is GraphQL and how does it differ from REST APIs?
Answer:
GraphQL is a query language for APIs that provides a more flexible and efficient way to fetch data compared to traditional REST APIs.
35. How do you implement server-side rendering (SSR) in a Node.js application?
Answer:
SSR involves rendering the initial HTML of a web page on the server side, which can improve SEO and performance.
36. What are some common design patterns used in Node.js applications?
Answer:
Common design patterns include the Observer pattern, the Module pattern, and the Singleton pattern.
37. How do you handle errors and exceptions in Node.js applications?
Answer:
Error handling involves using try...catch
blocks, error objects, and custom error classes.
38. What is the difference between Node.js and Deno?
Answer:
Deno is a newer runtime for JavaScript and TypeScript that aims to address some of the shortcomings of Node.js. It has a different module system and security model.
39. What are some of the latest trends and innovations in the Node.js ecosystem?
Answer:
Keep up-to-date with the latest trends like TypeScript integration, serverless functions, and emerging frameworks.
40. How would you handle error handling in Node.js?
Answer: Error handling in Node.js can be done using:
- Try-catch blocks (for synchronous code)
- Handling promise rejections with
.catch()
- Handling errors in callback functions (by passing the error as the first argument)
- Using middleware in Express.js for centralized error handling.
41. What is CORS in Node.js?
Answer:
CORS (Cross-Origin Resource Sharing) is a mechanism that allows restricted resources (like APIs) on a web page to be requested from another domain outside the domain from which the resource originated. CORS can be enabled in Node.js using middleware like cors
in Express.js.
42. What is JWT (JSON Web Token) and how does it work in Node.js?
Answer:
JWT is a compact, URL-safe token that is used to securely transmit information between parties as a JSON object. It is often used for authentication purposes in Node.js applications. It consists of three parts: header, payload, and signature.
43. What is the difference between npm and npx?
Answer:
- npm: The package manager for Node.js, used to install packages globally or locally.
- npx: Executes npm packages without globally installing them, used for running one-off commands.
44. How do you manage environments in Node.js applications?
Answer:
Environments in Node.js can be managed by using environment variables (process.env
) and tools like dotenv
to load environment variables from a .env
file for local development and different deployment environments.
Learn More: Carrer Guidance
1. Data Analyst Interview Questions for fresher
2. Data Analyst Interview Questions for Experienced
3. Redux Interview Question and Answers for experienced
4. Spring Boot Interview Questions and Answers