Are you preparing for a Shell Scripting interview? Shell scripting is an essential skill for DevOps professionals, enabling the automation of tasks and seamless system management. To help you out, we have compiled the top 30+ shell scripting interview questions and detailed answers to prepare effectively. These questions will provide valuable insights and enhance your scripting skills.
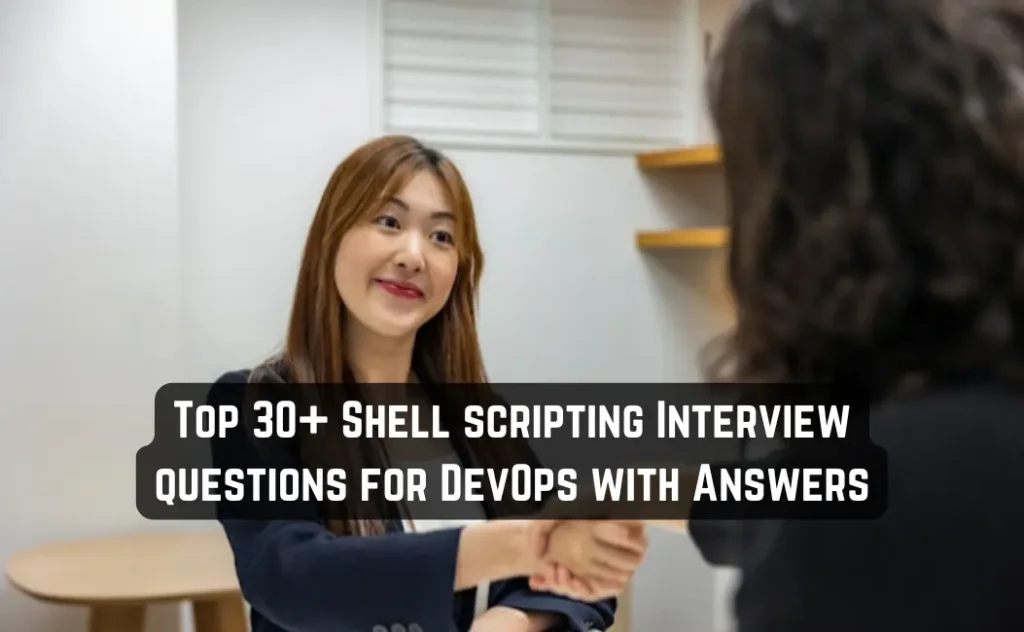
Top 30+ Shell scripting Interview questions for DevOps with Answers
- What is a Shell?
- What is Shell Scripting?
- How do you execute a Shell Script?
- What is the significance of the Shebang (#!/bin/bash) in a Shell Script?
- How do you pass and access arguments in a Shell Script?
- How do you check if a file exists in a Shell Script?
- How do you compare two numbers in a Shell Script?
- What is the difference between $* and $@ in Shell Scripting?
- How do you create a function in a Shell Script?
- How do you schedule a task to run at a specific time in Unix/Linux?
- How can you redirect both standard output and standard error to the same file?
- What is the purpose of the exec command in a shell script?
- How do you find the length of a string in Bash?
- How can you append text to a file in a shell script?
- How do you check if a variable is empty in a shell script?
- How do you perform arithmetic operations in a shell script?
- How do you read input from the user in a shell script?
- How can you create an array in Bash and iterate over its elements?
- How do you handle signals in a shell script?
- What is the difference between = and == in shell scripting?
- How do you declare and use a variable in a shell script?
- How can you make a script executable?
- What is the purpose of the shift command in shell scripting?
- How do you comment out multiple lines in a shell script?
- How can you check if a command was successful in a shell script?
- How do you perform a case-insensitive comparison of strings in a shell script?
- How do you check if a string contains a substring in a shell script?
- How do you remove leading and trailing whitespace from a string in a shell script?
- How can you generate a random number within a specific range in a shell script?
- How do you create a symbolic link in Unix/Linux?
- How do you find the process ID (PID) of a running program in Unix/Linux?
1. What is a Shell?
A shell is a command-line interpreter that provides a user interface for accessing an operating system’s services. It allows users to execute commands, run programs, and manage system resources. In Unix-like systems, common shells include Bourne Shell (sh), C Shell (csh), Korn Shell (ksh), and Bourne Again Shell (bash).
2. What is Shell Scripting?
Shell scripting involves writing a series of commands in a text file, which the shell can execute sequentially. This approach automates repetitive tasks, manages system operations, and facilitates complex command executions. Shell scripts are widely used for system administration, application deployment, and data processing.
3. How do you execute a Shell Script?
To execute a shell script, follow these steps:
Make the script executable: Use the chmod
command to grant execute permissions.
chmod +x scriptname.sh
Run the script: Precede the script name with ./
to execute it.
./scriptname.sh
Alternatively, you can execute the script by specifying the interpreter explicitly:
bash scriptname.sh
4. What is the significance of the Shebang (#!/bin/bash
) in a Shell Script?
The shebang (#!
) at the beginning of a script specifies the path to the interpreter that should execute the script. For example, #!/bin/bash
indicates that the script should be run using the Bash shell. This ensures that the correct interpreter processes the script, regardless of the current shell environment.
5. How do you pass and access arguments in a Shell Script?
Arguments can be passed to a shell script by specifying them after the script name:
./scriptname.sh arg1 arg2
Within the script, these arguments are accessed using positional parameters:
$0
: The name of the script.$1
: The first argument.$2
: The second argument.$#
: The number of arguments passed.$@
or$*
: All arguments passed.
For example:
#!/bin/bash
echo "Script name: $0"
echo "First argument: $1"
echo "Second argument: $2"
echo "Total number of arguments: $#"
6. How do you check if a file exists in a Shell Script?
To check if a file exists, use the -e
option within an if
statement:
#!/bin/bash
if [ -e filename ]; then
echo "File exists."
else
echo "File does not exist."
fi
Other options include:
-f
: Check if it’s a regular file.-d
: Check if it’s a directory.
7. How do you compare two numbers in a Shell Script?
Use comparison operators within square brackets:
-eq
: Equal to-ne
: Not equal to-lt
: Less than-le
: Less than or equal to-gt
: Greater than-ge
: Greater than or equal to
Example:
#!/bin/bash
if [ "$1" -gt "$2" ]; then
echo "$1 is greater than $2"
else
echo "$1 is not greater than $2"
fi
8. What is the difference between $*
and $@
in Shell Scripting?
Both $*
and $@
represent all the arguments passed to a script, but they handle quoting differently:
$*
: Treats all arguments as a single word.$@
: Treats each argument as a separate word.
When quoted:
"$*"
: All arguments are combined into a single string."$@"
: Each argument is treated individually.
Example:
#!/bin/bash
for arg in "$*"; do
echo "$arg"
done
This will print all arguments as a single string.
#!/bin/bash
for arg in "$@"; do
echo "$arg"
done
This will print each argument on a new line.
9. How do you create a function in a Shell Script?
Define a function using the following syntax:
function_name() {
# Commands
}
Or:
function function_name {
# Commands
}
Example:
#!/bin/bash
greet() {
echo "Hello, $1!"
}
greet "World"
This script defines a function greet
that takes one argument and prints a greeting message.
10. How do you schedule a task to run at a specific time in Unix/Linux?
To schedule tasks in Unix/Linux, you can use the cron
daemon with the crontab
command. The crontab
file allows you to specify commands to run at scheduled intervals. Each line in a crontab
file follows this format:
* * * * * command_to_execute
- - - - -
| | | | |
| | | | +----- Day of the week (0 - 7) (Sunday=0 or 7)
| | | +------- Month (1 - 12)
| | +--------- Day of the month (1 - 31)
| +----------- Hour (0 - 23)
+------------- Minute (0 - 59)
For example, to run a script every day at 3:30 PM:
30 15 * * * /path/to/script.sh
To edit the crontab file, use:
crontab -e
After editing, save and exit to apply the changes.
11. How can you redirect both standard output and standard error to the same file?
In shell scripting, you can redirect both standard output (stdout) and standard error (stderr) to the same file using the following syntax:
command > output.log 2>&1
Here, >
redirects stdout to output.log
, and 2>&1
redirects stderr (file descriptor 2) to the same location as stdout (file descriptor 1). Alternatively, you can use:
command > output.log 2>&1
This syntax is supported in Bash and redirects both stdout and stderr to output.log
.
12. What is the purpose of the exec
command in a shell script?
The exec
command in a shell script is used to replace the current shell process with a specified command. When exec
is invoked, it does not create a new process; instead, it replaces the current shell with the command specified. This is useful for:
- Replacing the shell: To replace the current shell with another program.
- Redirecting file descriptors: To redirect input/output streams for the current shell.
For example, to redirect all output of a script to a file:
#!/bin/bash
exec > output.log 2>&1
# All subsequent commands' output will go to output.log
In this script, all output after the exec
command is redirected to output.log
.
13. How do you find the length of a string in Bash?
To find the length of a string in Bash, you can use parameter expansion:
#!/bin/bash
string="Hello, World!"
length=${#string}
echo "Length of the string is $length"
This script will output:
Length of the string is 13
Here, ${#string}
evaluates to the length of the string stored in the variable string
.
14. How can you append text to a file in a shell script?
To append text to a file in a shell script, use the >>
operator:
#!/bin/bash
echo "This is a new line" >> filename.txt
This command adds “This is a new line” to the end of filename.txt
. If the file does not exist, it will be created.
15. How do you check if a variable is empty in a shell script?
To check if a variable is empty in a shell script, you can use an if
statement with the -z
option:
#!/bin/bash
if [ -z "$variable" ]; then
echo "Variable is empty"
else
echo "Variable is not empty"
fi
The -z
option returns true if the length of the string is zero, indicating that the variable is empty.
16. How do you perform arithmetic operations in a shell script?
In shell scripting, you can perform arithmetic operations using:
expr
command:
#!/bin/bash
result=$(expr 5 + 3)
echo "Result: $result"
Double parentheses:
#!/bin/bash
result=$((5 + 3))
echo "Result: $result"
bc
command:
#!/bin/bash
result=$(echo "scale=2; 5 / 3" | bc)
echo "Result: $result"
These methods allow you to perform arithmetic operations within shell scripts.
17. How do you read input from the user in a shell script?
To read input from the user in a shell script, use the read
command:
#!/bin/bash
echo "Enter your name:"
read name
echo "Hello, $name!"
This script prompts the user to enter their name and then greets them using the input provided.
18. How can you create an array in Bash and iterate over its elements?
In Bash, arrays are versatile data structures that can store multiple values. You can create indexed arrays, where elements are accessed via numerical indices, or associative arrays, where elements are accessed using string keys.
Creating an Indexed Array:
You can define an indexed array using parentheses, with elements separated by spaces:
#!/bin/bash
# Define an indexed array
fruits=("Apple" "Banana" "Cherry")
Alternatively, you can declare an empty array and assign values to specific indices:
#!/bin/bash
# Declare an empty indexed array
declare -a fruits
# Assign values to specific indices
fruits[0]="Apple"
fruits[1]="Banana"
fruits[2]="Cherry"
Iterating Over an Indexed Array:
To loop through the elements of an indexed array, you can use a for
loop:
#!/bin/bash
# Define an indexed array
fruits=("Apple" "Banana" "Cherry")
# Iterate over array elements
for fruit in "${fruits[@]}"; do
echo "I like $fruit"
done
This script will output:
I like Apple
I like Banana
I like Cherry
In this loop, ${fruits[@]}
expands to all elements of the fruits
array, and each element is assigned to the variable fruit
in each iteration.
Creating an Associative Array:
Associative arrays allow you to use string keys. To declare an associative array, use the declare
command with the -A
option:
#!/bin/bash
# Declare an associative array
declare -A colors
# Assign key-value pairs
colors[Apple]="Red"
colors[Banana]="Yellow"
colors[Cherry]="Red"
Iterating Over an Associative Array:
To iterate over key-value pairs in an associative array:
#!/bin/bash
# Declare an associative array
declare -A colors
colors[Apple]="Red"
colors[Banana]="Yellow"
colors[Cherry]="Red"
# Iterate over keys
for fruit in "${!colors[@]}"; do
echo "The color of $fruit is ${colors[$fruit]}"
done
This script will output:
The color of Apple is Red
The color of Banana is Yellow
The color of Cherry is Red
Here, ${!colors[@]}
expands to all keys in the colors
array, allowing you to access each key and its corresponding value.
19. How do you handle signals in a shell script?
In Unix-like systems, signals are used to notify processes of events. Handling signals in a shell script allows you to define custom responses to various events, such as termination requests or interruptions. This is achieved using the trap
command.
Using the trap
Command:
The trap
command captures specified signals and executes a designated command or function when the signal is received. The general syntax is:
trap 'commands_to_execute' SIGNAL
commands_to_execute
: The commands or function to execute when the signal is received.SIGNAL
: The name or number of the signal to catch.
Common Signals:
SIGINT
(Interrupt): Signal 2, typically sent when the user pressesCtrl+C
.SIGTERM
(Terminate): Signal 15, the default signal for termination requests.SIGHUP
(Hang Up): Signal 1, sent when the terminal is closed.
Example: Handling SIGINT
(Ctrl+C):
#!/bin/bash
# Function to execute upon receiving SIGINT
cleanup() {
echo "Script interrupted. Performing cleanup..."
# Add cleanup commands here
exit 1
}
# Set up trap to catch SIGINT and call cleanup
trap cleanup SIGINT
# Main script logic
echo "Script is running. Press Ctrl+C to interrupt."
while true; do
# Simulate work
sleep 1
done
In this script:
- The
cleanup
function defines actions to take when the script is interrupted. trap cleanup SIGINT
sets up a trap that calls thecleanup
function upon receiving theSIGINT
signal.- The script enters an infinite loop, simulating ongoing work. Pressing
Ctrl+C
will trigger thecleanup
function.
Ignoring a Signal:
To ignore a specific signal, use the trap
command with an empty string:
#!/bin/bash
# Ignore SIGINT
trap '' SIGINT
echo "Try pressing Ctrl+C. It will be ignored."
while true; do
sleep 1
done
In this script, pressing Ctrl+C
will have no effect because SIGINT
is being ignored.
Resetting a Trap:
To reset a trap to its default behavior, use the trap
command with a hyphen (-
):
#!/bin/bash
# Custom handler for SIGINT
trap 'echo "Custom handler for SIGINT"' SIGINT
echo "Press Ctrl+C to see the custom handler."
sleep 5
# Reset SIGINT to default behavior
trap - SIGINT
echo "Press Ctrl+C again to terminate the script."
sleep 5
In this script:
- Initially, pressing
Ctrl+C
will trigger the custom handler. - After resetting the trap, pressing
Ctrl+C
will terminate the script as per the default behavior.
20. What is the difference between =
and ==
in shell scripting?
In shell scripting, particularly in the Bourne shell (sh
), the single equals sign =
is used for string comparison within [ ]
(test command). For example:
if [ "$a" = "$b" ]; then
echo "Strings are equal."
fi
In contrast, in the Bash shell (bash
), both =
and ==
can be used for string comparisons within [ ]
. However, when using the [[ ]]
test construct, ==
is specifically used for pattern matching. For example:
if [[ "$a" == "$b" ]]; then
echo "Strings are equal."
fi
It’s important to note that ==
is not universally supported in all shells, so using =
is generally more portable.
21. How do you declare and use a variable in a shell script?
In shell scripting, variables are declared by assigning a value without any spaces around the equals sign. For example:
#!/bin/bash
name="John Doe"
To access the value of a variable, prefix its name with a dollar sign $
:
echo "Hello, $name!"
This will output:
Hello, John Doe!
Remember not to include spaces around the =
during assignment, as this can lead to errors.
22. How can you make a script executable?
To make a shell script executable, use the chmod
command to modify its permissions:
chmod +x scriptname.sh
After setting the executable permission, you can run the script by specifying its path:
./scriptname.sh
This approach ensures that the script has the necessary permissions to be executed directly from the command line.
23. What is the purpose of the shift
command in shell scripting?
The shift
command in shell scripting is used to shift the positional parameters to the left. This means that $2
becomes $1
, $3
becomes $2
, and so on. The original $1
is discarded. This is particularly useful when processing multiple command-line arguments in a loop.
For example:
#!/bin/bash
while [ "$#" -gt 0 ]; then
echo "Processing parameter: $1"
shift
done
In this script, "$#"
represents the number of positional parameters, and $1
is the current parameter being processed. The shift
command moves to the next parameter in each iteration.
24. How do you comment out multiple lines in a shell script?
In shell scripting, single-line comments are created using the #
symbol. To comment out multiple lines, you can prefix each line with #
:
# This is a comment
# spanning multiple
# lines.
Alternatively, you can use a here document to create a block comment:
: << 'END'
This is a comment
spanning multiple
lines.
END
In this approach, :
is a no-op (does nothing), and the here document (<< 'END'
) treats everything until END
as input, effectively ignoring it.
25. How can you check if a command was successful in a shell script?
After executing a command in a shell script, you can check its exit status using the special variable $?
. An exit status of 0
indicates success, while any non-zero value indicates failure.
For example:
#!/bin/bash
command
if [ "$?" -eq 0 ]; then
echo "Command succeeded."
else
echo "Command failed."
fi
This script executes command
and then checks its exit status to determine if it was successful.
26. What is the difference between >
and >>
in shell scripting?
In shell scripting, both >
and >>
are used for redirection:
>
: Redirects standard output to a file, overwriting the file if it already exists.>>
: Redirects standard output to a file, appending to the file if it already exists.
For example:
echo "This will overwrite the file" > filename.txt
echo "This will append to the file" >> filename.txt
Use >
when you want to replace the file’s contents and >>
when you want to add to the existing contents.
27. How do you perform a case-insensitive comparison of strings in a shell script?
In shell scripting, string comparisons are case-sensitive by default. To perform a case-insensitive comparison, you can:
Convert both strings to the same case (either lowercase or uppercase) before comparing:
#!/bin/bash
str1="Hello"
str2="hello"
# Convert both strings to lowercase and compare
if [ "$(echo "$str1" | tr '[:upper:]' '[:lower:]')" = "$(echo "$str2" | tr '[:upper:]' '[:lower:]')" ]; then
echo "Strings are equal (case-insensitive)."
else
echo "Strings are not equal."
fi
In this example, tr '[:upper:]' '[:lower:]'
converts the strings to lowercase, allowing for a case-insensitive comparison.
Use the shopt
command to enable case-insensitive matching (Bash-specific):
#!/bin/bash
shopt -s nocasematch # Enable case-insensitive matching
str1="Hello"
str2="hello"
if [[ "$str1" == "$str2" ]]; then
echo "Strings are equal (case-insensitive)."
else
echo "Strings are not equal."
fi
shopt -u nocasematch # Disable case-insensitive matching
Here, shopt -s nocasematch
enables case-insensitive pattern matching within [[ ]]
constructs. Remember to disable it afterward using shopt -u nocasematch
to revert to default behavior.
28. How do you check if a string contains a substring in a shell script?
To check if a string contains a specific substring in a shell script, you can use:
Using *
wildcard within [[ ]]
:
#!/bin/bash
string="Hello, World!"
substring="World"
if [[ "$string" == *"$substring"* ]]; then
echo "Substring found."
else
echo "Substring not found."
fi
In this example, *
is a wildcard that matches any sequence of characters, allowing the condition to check if $substring
exists within $string
.
Using the grep
command:
#!/bin/bash
string="Hello, World!"
substring="World"
if echo "$string" | grep -q "$substring"; then
echo "Substring found."
else
echo "Substring not found."
fi
Here, grep -q
searches for $substring
within $string
and returns a success status if found.
29. How do you remove leading and trailing whitespace from a string in a shell script?
To remove leading and trailing whitespace from a string in a shell script, you can use:
Using sed
:
#!/bin/bash
string=" Hello, World! "
trimmed=$(echo "$string" | sed 's/^[ \t]*//;s/[ \t]*$//')
echo ">$trimmed<"
This command removes leading (^[ \t]*
) and trailing ([ \t]*$
) spaces and tabs.
Using awk
:
#!/bin/bash
string=" Hello, World! "
trimmed=$(echo "$string" | awk '{$1=$1};1')
echo ">$trimmed<"
Here, awk
trims the string by resetting $1
and reassembling the line.
30. How can you generate a random number within a specific range in a shell script?
To generate a random number within a specific range in a shell script, you can use:
Using $RANDOM
:
#!/bin/bash
min=1
max=100
random_number=$((RANDOM % (max - min + 1) + min))
echo "Random number between $min and $max: $random_number"
generates a random number between 0 and 32767. The expression scales it to the desired range.
Using /dev/urandom
:
#!/bin/bash
min=1
max=100
random_number=$(od -An -N2 -i /dev/urandom | awk -v min="$min" -v max="$max" '{print int(min + $1 % (max - min + 1))}')
echo "Random number between $min and $max: $random_number"
This approach reads random data from /dev/urandom
and scales it to the desired range.
31. How do you create a symbolic link in Unix/Linux?
In Unix/Linux systems, a symbolic link (also known as a symlink or soft link) is a special type of file that points to another file or directory. To create a symbolic link, you use the ln
command with the -s
option. The general syntax is:
ln -s [target_file_or_directory] [link_name]
target_file_or_directory
: The path to the existing file or directory you want to link to.link_name
: The name of the symbolic link you want to create.
Example: Creating a Symbolic Link to a File
Suppose you have a file located at /home/user/document.txt
and you want to create a symbolic link to this file in your current directory:
ln -s /home/user/document.txt ./doc_link.txt
This command creates a symbolic link named doc_link.txt
in the current directory, pointing to /home/user/document.txt
.
Example: Creating a Symbolic Link to a Directory
If you have a directory at /home/user/projects
and want to create a symbolic link to this directory in /var/www/html
:
ln -s /home/user/projects /var/www/html/projects_link
This command creates a symbolic link named projects_link
in /var/www/html
, pointing to /home/user/projects
.
Verifying the Symbolic Link
To verify that the symbolic link has been created and points to the correct target, use the ls -l
command:
ls -l [link_name]
For example:
ls -l doc_link.txt
The output will display something like:
lrwxrwxrwx 1 user user 20 Dec 2 19:03 doc_link.txt -> /home/user/document.txt
The ->
indicates that doc_link.txt
is a symbolic link pointing to /home/user/document.txt
.
Removing a Symbolic Link
To remove a symbolic link, use the rm
command followed by the name of the link:
rm [link_name]
For example:
rm doc_link.txt
This command removes the symbolic link doc_link.txt
without affecting the original file.
Note:
- Creating a symbolic link to a non-existent target is possible, but the link will be broken until the target is created.
- Symbolic links can point to files or directories located on different filesystems or partitions.
32. How do you find the process ID (PID) of a running program in Unix/Linux?
To find the process ID (PID) of a running program in Unix/Linux, you can use several commands:
1. Using the ps
Command with grep
:
The ps
command displays information about active processes. By combining it with grep
, you can filter the output to find the PID of a specific program.
ps aux | grep [program_name]
ps aux
: Displays all running processes with detailed information.grep [program_name]
: Filters the list to show only lines containing[program_name]
.
Example:
To find the PID of the nginx
process:
ps aux | grep nginx
The output might look like:
root 1234 0.0 0.1 12345 6789 ? Ss 12:00 0:00 nginx: master process /usr/sbin/nginx
www-data 1235 0.0 0.1 12345 6789 ? S 12:00 0:00 nginx: worker process
user 1236 0.0 0.0 6076 892 pts/0 S+ 12:01 0:00 grep --color=auto nginx
Here, the PID of the nginx
master process is 1234
.
2. Using the pgrep
Command:
The pgrep
command searches for processes by name and returns their PIDs.
pgrep [program_name]
Example:
To find the PID of the nginx
process:
pgrep nginx
The output will display the PID(s) of processes matching the name nginx
.
3. Using the pidof
Command:
The pidof
command returns the PID of a running program.
pidof [program_name]
Example:
To find the PID of the nginx
process:
pidof nginx
The output will display the PID(s) of the nginx
process.
Note:
- Ensure you have the necessary permissions to view the processes owned by other users.
- Some processes may have multiple instances running; these commands will list all matching PIDs.
Learn More: Carrer Guidance | Hiring Now!
Top 34 SSIS Interview Questions and Answers- Basic to Advanced
HSBC Interview Questions with Sample Answers
AutoCAD Interview Questions and Answers for Freshers
Java 8 Coding Interview Questions and Answers- Basic to Advanced
Chipotle Interview Questions and Answers
Batch Apex Interview Questions for Freshers with Answers
Mphasis interview Questions and Answers- Basic to Advanced
Redux Interview Questions and Answers: From Basics to Advanced Concepts