Are you preparing for a Selenium interview? To assist you, we’ve compiled the top 30 scenario-based Selenium and Cucumber interview questions and answers. This comprehensive guide covers a wide range of real-world scenarios, from handling dynamic web elements to managing test execution order, equipping you for your next job interview.
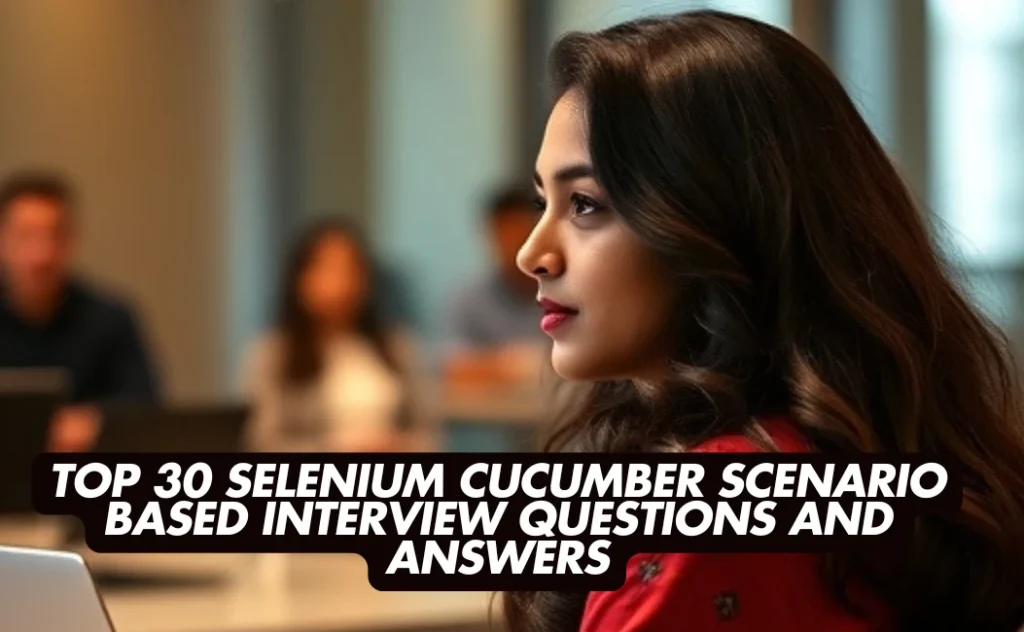
Top 30 Selenium Cucumber scenario based Interview Questions and Answers
- How would you handle dynamic web elements that have changing IDs in Selenium?
- Describe a scenario where you had to manage multiple browser windows or tabs using Selenium. How did you handle it?
- In a situation where a web page has multiple iframes, how would you interact with elements inside a specific iframe using Selenium?
- Explain how you would implement a data-driven test in Cucumber to validate a login functionality with multiple sets of credentials.
- How do you handle AJAX-based content loading in Selenium to ensure elements are available for interaction?
- Describe a scenario where you used Cucumber hooks to set up preconditions and postconditions for your tests.
- How would you manage test execution order in Cucumber when certain scenarios depend on the outcomes of others?
- Explain how you can capture screenshots in Selenium when a test scenario fails and integrate this into your Cucumber reports.
- In a scenario where you need to test a multilingual application, how would you structure your Cucumber feature files?
- Describe how you would handle file uploads in Selenium when automating a form submission.
- How do you manage browser cookies in Selenium to simulate different user sessions during testing?
- Explain a situation where you had to use JavaScript execution in Selenium to interact with a web element.
- How would you implement parallel test execution in a Selenium-Cucumber framework to reduce test execution time?
- Describe a scenario where you used Cucumber tags to organize and selectively run test cases.
- How do you handle dropdowns in Selenium, both static and dynamic, during test automation?
- Explain how you would validate tooltips or hover-over text in a web application using Selenium.
- In a situation where you need to test an application’s responsiveness, how would you use Selenium to validate different screen resolutions?
- Describe how you would handle browser alerts and pop-ups in Selenium during automated testing.
- How do you manage test data in Cucumber for scenarios that require complex data setups?
- Explain a scenario where you had to wait for an element to be clickable in Selenium. What wait strategies did you use?
- How would you handle CAPTCHA or reCAPTCHA in a web application during Selenium automation?
- Describe a situation where you integrated Selenium tests with a Continuous Integration (CI) pipeline. What challenges did you face?
- How do you verify PDF content that is generated as part of a web application using Selenium?
- Explain how you would test a web application’s performance using Selenium scripts.
- In a scenario where you need to validate email confirmations sent by the application, how would you automate this using Selenium?
- Describe how you would handle time zone differences in date and time validations during Selenium testing.
- How do you manage and validate web application logs during Selenium test execution?
- Explain a scenario where you had to mock backend services or APIs during Selenium testing.
- How would you handle browser-specific quirks and inconsistencies in Selenium test scripts?
- Describe a situation where you had to debug a failing Selenium-Cucumber test. What steps did you take to identify and resolve the issue?
1. How would you handle dynamic web elements that have changing IDs in Selenium?
- Identify stable attributes (like
name
,class
,data-*
attributes, or partial text) instead of relying on dynamic IDs. - Use CSS selectors or XPath with functions (e.g.,
contains()
orstarts-with()
) to locate elements. - Leverage wait conditions (Explicit Waits) to ensure the element is present before interacting with it.
Example (using XPath with contains):
WebElement dynamicElement = driver.findElement(By.xpath("//*[contains(@id, 'partialIdValue')]"));
2. Describe a scenario where you had to manage multiple browser windows or tabs using Selenium. How did you handle it?
- Switch between window handles provided by Selenium’s
getWindowHandles()
method. - Store the original window handle.
- After performing an action that opens a new tab or window, iterate through
getWindowHandles()
and switch to the new handle. - Perform actions on the new window and then switch back to the original if needed.
Example:
String originalWindow = driver.getWindowHandle();
for (String handle : driver.getWindowHandles()) {
if (!handle.equals(originalWindow)) {
driver.switchTo().window(handle);
break;
}
}
3. In a situation where a web page has multiple iframes, how would you interact with elements inside a specific iframe using Selenium?
- First, identify the iframe using a locator (e.g.,
By.id
,By.name
). - Use
driver.switchTo().frame()
to switch the context to that iframe. - Perform actions on elements inside the iframe.
- Switch back to the main content using
driver.switchTo().defaultContent()
ordriver.switchTo().parentFrame()
once done.
Example:
WebElement iframeElement = driver.findElement(By.id("frameId"));
driver.switchTo().frame(iframeElement);
// Interact with elements inside the iframe
driver.switchTo().defaultContent();
4. Explain how you would implement a data-driven test in Cucumber to validate a login functionality with multiple sets of credentials.
- Use a Scenario Outline in the feature file to define placeholders for username and password.
- Provide multiple data sets in the Examples section.
- In the step definitions, retrieve the parameters and perform login steps using these values.
- This approach allows executing the scenario once for each set of input data.
Example (Scenario Outline in feature file):
Scenario Outline: Validate login with multiple credentials
Given I navigate to the login page
When I enter "<username>" and "<password>"
And I click on the login button
Then I should see the homepage
Examples:
| username | password |
| user1 | pass1 |
| user2 | pass2 |
5. How do you handle AJAX-based content loading in Selenium to ensure elements are available for interaction?
- Use Explicit Waits (WebDriverWait) to wait until an element is visible, clickable, or until a certain condition is met (like presence of an element).
- Avoid hard-coded Thread.sleep() as it’s not reliable.
- Wait for conditions like
ExpectedConditions.elementToBeClickable()
orExpectedConditions.visibilityOfElementLocated()
before interacting.
Example (Explicit Wait):
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("dynamicElementId")));
6. Describe a scenario where you used Cucumber hooks to set up preconditions and postconditions for your tests.
- Before running scenarios that require a logged-in state, use a
@Before
hook to launch the browser, navigate to the login page, and perform a successful login. - After test completion, use an
@After
hook to close the browser or perform cleanup actions. - This ensures each scenario starts and ends in a known state, improving test reliability and maintainability.
Example (Hook in step definition file):
@Before
public void setUp() {
// Code to launch browser and navigate to the application
}
@After
public void tearDown() {
// Code to close browser
}
7. How would you manage test execution order in Cucumber when certain scenarios depend on the outcomes of others?
- Ideally, scenarios should be independent and not depend on others, but if needed, you can use tags and control execution order through the Cucumber options in the runner class.
- Group dependent scenarios under certain tags and run them in a sequence by configuring multiple runner classes or specifying tags in a particular order.
- Alternatively, use Hooks to achieve a known state rather than relying on previous scenario outcomes.
8. Explain how you can capture screenshots in Selenium when a test scenario fails and integrate this into your Cucumber reports.
- Use an
@After
hook that checks the scenario’s status. If it fails, capture a screenshot using Selenium’sgetScreenshotAs()
method. - Embed the screenshot in the Cucumber report so that failures can be visualized directly in the report.
Example (Capturing screenshot in an After hook):
@After
public void afterScenario(Scenario scenario) {
if (scenario.isFailed()) {
final byte[] screenshot = ((TakesScreenshot) driver).getScreenshotAs(OutputType.BYTES);
scenario.attach(screenshot, "image/png", "Failure Screenshot");
}
}
9. In a scenario where you need to test a multilingual application, how would you structure your Cucumber feature files?
- Create separate feature files for each language, or parameterize language strings through Scenario Outlines or external resource files.
- Ensure the step definitions are language-agnostic and focus on element interactions rather than language-specific text.
- Use tags to differentiate language-based features for separate executions.
Example structure:
features/
en/
login.feature
fr/
login.feature
10. Describe how you would handle file uploads in Selenium when automating a form submission.
- Use
sendKeys()
on the file input element to set the file path directly, provided the element is of typefile
. - No need for manual interaction with the file dialog. Just ensure the file path is correct and accessible.
Example:
WebElement fileInput = driver.findElement(By.id("uploadInput"));
fileInput.sendKeys("C:\\path\\to\\file.txt");
11. How do you manage browser cookies in Selenium to simulate different user sessions during testing?
- Use Selenium’s
manage().getCookies()
,manage().addCookie()
, andmanage().deleteCookie()
methods to manipulate browser cookies. - By adding or removing cookies, you can simulate different user states and sessions. For instance, adding a session cookie might bypass the login step for a returning user scenario.
Example:
Cookie newCookie = new Cookie("session_id", "12345");
driver.manage().addCookie(newCookie);
driver.navigate().refresh();
12. Explain a situation where you had to use JavaScript execution in Selenium to interact with a web element.
- When a standard Selenium click fails due to overlays or custom JavaScript behavior, using
JavascriptExecutor
can help. - For example, clicking on a hidden button or scrolling the element into view before interaction.
Example:
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("arguments[0].click();", element);
13. How would you implement parallel test execution in a Selenium-Cucumber framework to reduce test execution time?
- Use a test runner like TestNG or JUnit with Cucumber and configure it to run multiple threads.
- Ensure that each thread uses its own WebDriver instance.
- Tag scenarios that can safely run in parallel, and execute them through Maven or Gradle with parallel execution parameters.
14. Describe a scenario where you used Cucumber tags to organize and selectively run test cases.
- Tag high-priority scenarios with
@Smoke
and run only those first. - Exclude slow-running scenarios by tagging them
@Slow
and using the Cucumber options to skip these tags during certain test runs. - This approach streamlines selective execution and focuses on critical paths or quick checks.
15. How do you handle dropdowns in Selenium, both static and dynamic, during test automation?
- For static dropdowns using
<select>
tags, use theSelect
class and its methods likeselectByVisibleText()
,selectByValue()
, orselectByIndex()
. - For dynamic dropdowns (often custom-made), locate and click the dropdown element, then select from the resulting list items using normal WebElement interactions.
Example (Select class):
Select dropdown = new Select(driver.findElement(By.id("staticDropdown")));
dropdown.selectByVisibleText("Option 1");
16. Explain how you would validate tooltips or hover-over text in a web application using Selenium.
- Use
Actions
class to move the mouse over the element that triggers the tooltip. - After hovering, locate the tooltip element and verify its text.
- Alternatively, if tooltips are implemented as a title attribute, retrieve the
getAttribute("title")
and compare expected vs. actual text.
Example:
Actions actions = new Actions(driver);
actions.moveToElement(elementWithTooltip).perform();
String tooltipText = elementWithTooltip.getAttribute("title");
17. In a situation where you need to test an application’s responsiveness, how would you use Selenium to validate different screen resolutions?
- Resize the browser window using
driver.manage().window().setSize(new Dimension(width, height))
before testing. - Validate element positions, visibility, or layout changes at different sizes.
- Combine this with conditional checks or visual validation tools to ensure the UI responds correctly.
18. Describe how you would handle browser alerts and pop-ups in Selenium during automated testing.
- Use
driver.switchTo().alert()
to handle JavaScript alerts. - Use
accept()
,dismiss()
,sendKeys()
on the returned Alert object to interact with it. - For pop-ups not handled by the browser’s Alert interface, switch to the pop-up window handle if it’s a separate window.
Example:
Alert alert = driver.switchTo().alert();
alert.accept();
19. How do you manage test data in Cucumber for scenarios that require complex data setups?
- Store test data in external files (e.g., Excel, CSV, JSON, or databases) and load them in the
@Before
hook or step definitions. - Use dependency injection frameworks like PicoContainer or manage data through global variables accessible across steps.
- Scenario Outlines and Examples tables can handle simpler data sets, while external sources handle complex data.
20. Explain a scenario where you had to wait for an element to be clickable in Selenium. What wait strategies did you use?
- When a button appears after an AJAX call, use
WebDriverWait
withExpectedConditions.elementToBeClickable()
. - This approach ensures the script only attempts to click when the element is both present and interactable, reducing flaky failures.
Example:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement clickableElement = wait.until(ExpectedConditions.elementToBeClickable(By.id("clickableButton")));
clickableElement.click();
21. How would you handle CAPTCHA or reCAPTCHA in a web application during Selenium automation?
- Ideally, avoid automating CAPTCHAs directly, as they’re designed to prevent automation.
- For testing, disable CAPTCHA in a test environment or use a testing key that auto-verifies.
- If unavoidable, integrate a third-party CAPTCHA solving service via API, but this is generally discouraged for genuine automation tests.
22. Describe a situation where you integrated Selenium tests with a Continuous Integration (CI) pipeline. What challenges did you face?
- Configured Selenium tests to run headless in a CI environment (e.g., Jenkins, GitLab CI) using a headless browser driver or Dockerized Selenium Grid.
- Faced challenges with environment setup, missing dependencies, and timing issues due to slower CI servers.
- Addressed challenges by adding explicit waits, robust environment configuration scripts, and ensuring stable test data availability.
23. How do you verify PDF content that is generated as part of a web application using Selenium?
- Selenium alone can’t directly verify PDF content.
- After downloading the PDF using Selenium interactions, use a separate library (e.g., Apache PDFBox) to parse and validate the PDF’s text or metadata.
- Compare the extracted text against expected values.
24. Explain how you would test a web application’s performance using Selenium scripts.
- Selenium is not primarily a performance testing tool.
- However, basic performance checks can be done by measuring page load times using
Navigation Timing API
via JavaScript orWebDriver
timing logs. - For more in-depth performance testing, integrate with dedicated tools like JMeter, Gatling, or Lighthouse, while Selenium focuses on user-flow validation.
25. In a scenario where you need to validate email confirmations sent by the application, how would you automate this using Selenium?
- Use a test email account and access it through a webmail interface or via API/IMAP protocols.
- If webmail: automate the login and check for the received email content with Selenium.
- If API/IMAP: retrieve emails programmatically, parse the content, and then validate against expected results outside of Selenium.
26. Describe how you would handle time zone differences in date and time validations during Selenium testing.
- Use a common, consistent time zone in your test environment and application under test.
- Convert displayed times to a standard format (like UTC) before validation.
- Compare actual results against expected times converted to the same zone, ensuring consistent and accurate validation.
27. How do you manage and validate web application logs during Selenium test execution?
- Use browser logs or server-side logging.
- Retrieve browser console logs using Selenium’s
manage().logs().get("browser")
. Check for errors or warnings during test steps. - For server logs, integrate API calls or remote access to log files and validate entries pre- or post-test execution.
28. Explain a scenario where you had to mock backend services or APIs during Selenium testing.
- When the backend is unstable or unavailable, use a tool like WireMock or a proxy server to mock API responses.
- Run the Selenium tests against a controlled and predictable API environment, ensuring consistent test results even if real services fluctuate.
29. How would you handle browser-specific quirks and inconsistencies in Selenium test scripts?
- Implement browser-specific logic or use separate locators if certain browsers render elements differently.
- Use conditions in the code to identify the browser at runtime and apply browser-appropriate actions.
- Regularly cross-check test results on multiple browsers and maintain separate driver configurations if needed.
30. Describe a situation where you had to debug a failing Selenium-Cucumber test. What steps did you take to identify and resolve the issue?
- Reviewed the failing scenario’s steps and checked the application state at failure.
- Captured screenshots, reviewed console logs, and added temporary logging statements in step definitions.
- Narrowed down if it was a locator issue, timing problem, or environment configuration error. Once identified, adjusted waits, updated locators, or fixed test data to resolve the issue.
Learn More: Carrer Guidance | Hiring Now!
Top 20 Tableau Scenario based Interview Questions and Answers
Unix Interview Questions and Answers
RabbitMQ Interview Questions and Answers
Kotlin Interview Questions and Answers for Developers
Mocha Interview Questions and Answers for JavaScript Developers
Java Multi Threading Interview Questions and Answers
Tosca Real-Time Scenario Questions and Answers
Advanced TOSCA Test Automation Engineer Interview Questions and Answers with 5+ Years of Experience
Tosca Interview Questions for Freshers with detailed Answers