Preparing for a Data Structures and Algorithms (DSA) interview is crucial for software developers aiming to showcase their problem-solving skills and technical knowledge. Understanding key concepts such as arrays, linked lists, stacks, queues, trees, and graphs is essential for tackling complex coding challenges. This guide features over 30 top DSA interview questions and answers to help you grasp both the fundamentals and advanced aspects of data structures and algorithms.
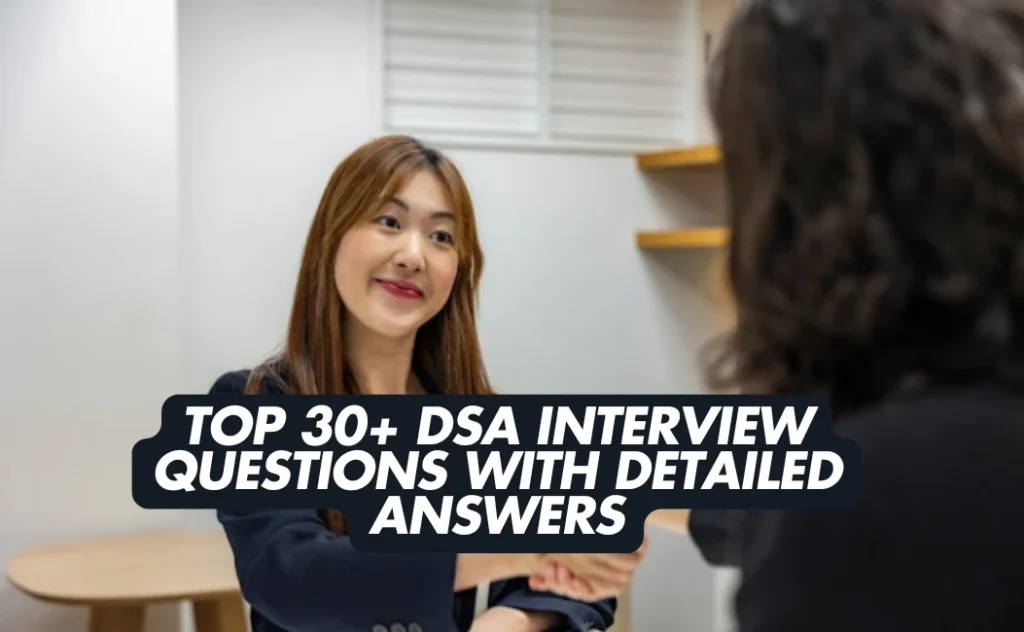
Top 30+ DSA Interview Questions and Answers
- What is a Data Structure?
- How does a Linked List differ from an Array?
- What is a Stack, and how does it function?
- Can you explain what a Queue is?
- What is a Binary Search Tree (BST)?
- How does a Hash Table work?
- What is a Heap, and where is it used?
- Can you explain what a Graph is in data structures?
- What is Dynamic Programming?
- How does the Quick Sort algorithm work?
- What is Merge Sort, and how does it function?
- What is a Red-Black Tree?
- What is Depth-First Search (DFS) in a Graph?
- What is Breadth-First Search (BFS) in a Graph?
- What is the difference between BFS and DFS?
- What is a Hash Table, and how does it work?
- What is Dynamic Programming, and how is it applied?
- What is a Segment Tree, and where is it applied?
- What is a Fenwick Tree (Binary Indexed Tree)?
- What is a B-Tree?
- What is a B+ Tree?
- What is a Graph, and how is it represented?
- What is Topological Sorting in a Directed Acyclic Graph (DAG)?
- What is Dijkstra’s Algorithm?
- What is the Bellman-Ford Algorithm?
- What is the Floyd-Warshall Algorithm?
- What is a Minimum Spanning Tree (MST)?
- What is Kruskal’s Algorithm?
- What is Prim’s Algorithm?
- What is the Difference Between Prim’s and Kruskal’s Algorithms?
- What is a Trie?
1. What is a Data Structure?
A data structure is a specialized format for organizing, processing, retrieving, and storing data. It enables efficient access and modification of data. Common data structures include arrays, linked lists, stacks, queues, trees, and graphs. Choosing the appropriate data structure is vital for optimizing algorithms and ensuring efficient data handling.
2. How does a Linked List differ from an Array?
Arrays and linked lists are both linear data structures but differ in memory allocation and operations:
- Memory Allocation: Arrays use contiguous memory locations, while linked lists consist of nodes that may be scattered throughout memory, connected via pointers.
- Size Flexibility: Arrays have a fixed size determined at creation, whereas linked lists can dynamically grow or shrink during execution.
- Insertion and Deletion: Inserting or deleting elements in an array can be time-consuming due to shifting elements. In linked lists, these operations are more efficient as they involve updating pointers.
3. What is a Stack, and how does it function?
A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle. Operations include:
- Push: Add an element to the top.
- Pop: Remove the top element.
- Peek/Top: View the top element without removing it.
Stacks are used in scenarios like expression evaluation, backtracking algorithms, and managing function calls.
4. Can you explain what a Queue is?
A queue is a linear data structure that operates on the First-In-First-Out (FIFO) principle. Operations include:
- Enqueue: Add an element to the rear.
- Dequeue: Remove an element from the front.
Queues are utilized in scenarios like scheduling processes in operating systems, handling requests in web servers, and breadth-first search algorithms.
5. What is a Binary Search Tree (BST)?
A BST is a binary tree where each node has at most two children, and for each node:
- The left subtree contains nodes with keys less than the node’s key.
- The right subtree contains nodes with keys greater than the node’s key.
This property allows efficient searching, insertion, and deletion operations, typically with O(log n) time complexity if the tree is balanced.
6. How does a Hash Table work?
A hash table stores key-value pairs and uses a hash function to compute an index into an array of buckets or slots, from which the desired value can be found. In case of collisions (when multiple keys hash to the same index), methods like chaining (linking entries in a list) or open addressing (finding the next available slot) are used. Hash tables provide average-case constant time complexity, O(1), for search, insert, and delete operations.
7. What is a Heap, and where is it used?
A heap is a specialized binary tree-based data structure that satisfies the heap property:
- Max-Heap: Each parent node is greater than or equal to its child nodes.
- Min-Heap: Each parent node is less than or equal to its child nodes.
Heaps are commonly used in implementing priority queues, heap sort algorithms, and algorithms like Dijkstra’s shortest path.
8. Can you explain what a Graph is in data structures?
A graph is a collection of nodes (vertices) connected by edges. Graphs can be:
- Directed or Undirected: Edges have a direction or not.
- Weighted or Unweighted: Edges have weights or not.
Graphs are used to represent networks like social connections, communication systems, and transportation routes.
9. What is Dynamic Programming?
Dynamic programming is an algorithmic technique for solving complex problems by breaking them down into simpler subproblems. It involves storing the results of subproblems to avoid redundant computations, thus optimizing performance. It’s particularly useful in problems exhibiting overlapping subproblems and optimal substructure properties, such as the Fibonacci sequence, shortest path algorithms, and knapsack problems.
10. How does the Quick Sort algorithm work?
Quick Sort is a divide-and-conquer algorithm that:
- Partitioning: Selects a ‘pivot’ element and partitions the array into two sub-arrays: elements less than the pivot and elements greater than the pivot.
- Recursion: Recursively applies the above steps to the sub-arrays.
This results in a sorted array. Quick Sort has an average-case time complexity of O(n log n) but can degrade to O(n²) if the pivot selection is poor.
11. What is Merge Sort, and how does it function?
Merge Sort is a divide-and-conquer algorithm that:
- Divide: Splits the array into two halves.
- Conquer: Recursively sorts each half.
- Merge: Combines the two sorted halves into a single sorted array.
Merge Sort consistently performs with a time complexity of O(n log n) and is stable, but it requires additional space for merging.
12. What is a Red-Black Tree?
A Red-Black Tree is a self-balancing binary search tree (BST) that ensures operations like search, insert, and delete can be performed in O(log n) time. Each node in a Red-Black Tree contains an extra bit for storing color, which can be either red or black. This coloring helps maintain the tree’s balance during insertions and deletions.
Properties of Red-Black Trees:
- Coloring: Every node is either red or black.
- Root Property: The root node is black.
- Leaf Nodes: All leaves (null pointers) are considered black.
- Red Node Rule: A red node cannot have red children (i.e., no two red nodes can be adjacent).
- Black Depth: Every path from a node to its descendant leaves contains the same number of black nodes.
These properties ensure that the longest path from the root to a leaf is no more than twice as long as the shortest path, guaranteeing the tree remains approximately balanced.
Operations:
- Insertion: New nodes are inserted similarly to a standard BST and are initially colored red. After insertion, the tree is adjusted through rotations and recoloring to maintain Red-Black properties.
- Deletion: Nodes are removed similarly to a standard BST. After deletion, the tree undergoes rotations and recoloring to restore Red-Black properties.
Red-Black Trees are widely used in computer science, including in implementations of associative arrays and in the Linux kernel’s process scheduling.
13. What is Depth-First Search (DFS) in a Graph?
Depth-First Search (DFS) is a graph traversal algorithm that explores as far along each branch as possible before backtracking.
Algorithm:
- Start: Begin at the chosen starting vertex.
- Explore: Visit an adjacent unvisited vertex, mark it as visited, and push it onto the stack.
- Backtrack: If no adjacent unvisited vertex is found, pop a vertex from the stack.
- Continue: Repeat steps 2 and 3 until the stack is empty.
Implementation:
- Recursive: Utilizes the system call stack to manage backtracking.
- Iterative: Explicitly uses a stack data structure to manage traversal.
Applications:
- Pathfinding: Finding a path between two nodes.
- Cycle Detection: Determining if a graph contains cycles.
- Topological Sorting: Ordering vertices in a Directed Acyclic Graph (DAG).
DFS is particularly useful for problems that require exploring all possible paths or configurations, such as puzzle solving and maze navigation.
14. What is Breadth-First Search (BFS) in a Graph?
Breadth-First Search (BFS) is a graph traversal algorithm that explores all vertices at the present depth level before moving on to vertices at the next depth level. It utilizes a queue data structure to keep track of the next vertex to visit, ensuring that vertices are explored in the order they are discovered.
Algorithm Steps:
- Initialization: Start by enqueuing the source vertex and marking it as visited.
- Traversal:
- Dequeue a vertex from the queue.
- For each adjacent unvisited vertex, mark it as visited and enqueue it.
- Completion: Repeat the traversal steps until the queue is empty.
Pseudocode:
BFS(graph, start_vertex):
create a queue Q
mark start_vertex as visited
enqueue start_vertex into Q
while Q is not empty:
vertex = dequeue Q
for each neighbor of vertex:
if neighbor is not visited:
mark neighbor as visited
enqueue neighbor into Q
Applications:
- Shortest Path in Unweighted Graphs: BFS can be used to find the shortest path between two vertices in an unweighted graph, as it explores all vertices at the present depth level before moving on to the next level.
- Level Order Traversal in Trees: BFS is used to traverse each level of a tree, making it ideal for level order traversal.
- Cycle Detection: BFS can detect cycles in undirected graphs by checking for back edges.
- Connectivity Testing: BFS helps in determining if all vertices are reachable from a given vertex, thus testing the connectivity of the graph.
Time Complexity: O(V + E), where V is the number of vertices and E is the number of edges.
Space Complexity: O(V), due to the storage required for the queue and the visited list.
BFS is particularly useful in scenarios where the shortest path or minimal number of transitions is desired, such as in routing and navigation systems.
15. What is the difference between BFS and DFS?
Breadth-First Search (BFS) and Depth-First Search (DFS) are fundamental graph traversal algorithms, each with distinct characteristics and applications.
Traversal Order:
- BFS: Explores all vertices at the current depth level before moving on to the next level, utilizing a queue to manage the traversal process.
- DFS: Explores as far along a branch as possible before backtracking, utilizing a stack (either explicitly or via recursion) to manage the traversal process.
Data Structures Used:
- BFS: Employs a queue to keep track of the next vertex to visit.
- DFS: Employs a stack or recursion to keep track of the next vertex to visit.
Applications:
- BFS:
- Finding the shortest path in unweighted graphs.
- Level order traversal of trees.
- Cycle detection in undirected graphs.
- DFS:
- Topological sorting.
- Cycle detection in directed graphs.
- Solving puzzles with only one solution (e.g., mazes).
Time Complexity: Both BFS and DFS have a time complexity of O(V + E), where V is the number of vertices and E is the number of edges.
Space Complexity:
- BFS: O(V), due to the storage required for the queue and the visited list.
- DFS: O(V), due to the storage required for the stack and the visited list.
In summary, BFS is optimal for finding the shortest path in unweighted graphs and exploring nodes level by level, while DFS is more memory-efficient and better suited for scenarios requiring exhaustive path exploration.
16. What is a Hash Table, and how does it work?
A hash table is a data structure that implements an associative array, allowing for efficient insertion, deletion, and lookup operations. It uses a hash function to map keys to specific indices in an array, where the corresponding values are stored.
Key Components:
- Hash Function: Transforms a key into an index within the array.
- Buckets: Array slots where values are stored; each bucket can hold multiple entries in case of collisions.
Operations:
- Insertion:
- Compute the hash of the key to determine the index.
- Place the key-value pair in the corresponding bucket.
- Handle collisions if necessary.
- Search:
- Compute the hash of the key to locate the bucket.
- Search within the bucket for the key.
- Deletion:
- Compute the hash to find the bucket.
- Remove the key-value pair from the bucket.
Collision Resolution Techniques:
- Chaining: Each bucket contains a list of entries that hash to the same index.
- Open Addressing: Find the next available slot through probing sequences like linear or quadratic probing.
Resizing (Rehashing):
To maintain efficiency, hash tables resize when the load factor (ratio of the number of entries to the number of buckets) exceeds a certain threshold. Resizing involves creating a new, larger array and rehashing all existing entries into this new array. This process ensures that the load factor remains optimal, thereby maintaining the average-case time complexity of O(1) for operations.
Applications:
- Databases: Implementing indexes for quick data retrieval.
- Caches: Storing frequently accessed data for rapid access.
- Symbol Tables: Used in compilers to manage scope and binding information.
Hash tables are fundamental in computer science due to their efficiency and versatility in various applications.
17. What is Dynamic Programming, and how is it applied?
Dynamic Programming (DP) is an algorithmic technique used to solve problems by breaking them down into simpler subproblems, solving each subproblem just once, and storing their solutions. This approach avoids redundant computations, making it particularly useful for optimization problems.
Key Concepts:
- Overlapping Subproblems: Problems that can be broken down into subproblems which are reused multiple times.
- Optimal Substructure: An optimal solution to the problem can be constructed from optimal solutions of its subproblems.
Approaches:
- Top-Down (Memoization):
- Start solving the problem by breaking it down recursively.
- Store the results of solved subproblems to avoid redundant computations.
- Bottom-Up (Tabulation):
- Solve smaller subproblems first and use their solutions to build up solutions to larger problems.
- Typically involves filling up a table based on the solutions of subproblems.
Applications:
- Fibonacci Sequence: Calculating the nth Fibonacci number efficiently.
- Knapsack Problem: Determining the most valuable combination of items to carry without exceeding weight capacity.
- Shortest Path Algorithms: Algorithms like Bellman-Ford for finding the shortest path in graphs.
Example: Fibonacci Sequence Using DP
Top-Down Approach (Memoization):
def fib(n, memo={}):
if n in memo:
return memo[n]
if n <= 2:
return 1
memo[n] = fib(n-1, memo) + fib(n-2, memo)
return memo[n]
Bottom-Up Approach (Tabulation):
def fib(n):
if n <= 2:
return 1
dp = [0] * (n + 1)
dp[1], dp[2] = 1, 1
for i in range(3, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
Dynamic Programming is a powerful technique for solving complex problems by breaking them down into simpler subproblems and building up solutions from these subproblems.
18. What is a Segment Tree, and where is it applied?
A Segment Tree is a binary tree used for storing intervals or segments. It allows querying which of the stored segments contain a given point efficiently.
Structure:
- Leaf Nodes: Represent individual elements of the array.
- Internal Nodes: Each internal node represents a segment that is the union of its child segments.
Operations:
- Build:
- Recursively divide the array into two halves until each segment contains a single element.
- Combine segments to build the tree from the bottom up.
- Query:
- To find the sum or minimum/maximum in a range, traverse the tree, combining results from relevant segments.
- Update:
- Modify the value of an element and update all segments that include this element.
Applications:
- Range Queries: Efficiently answer queries about sums, minimums, or maximums over a range of an array.
- Dynamic Arrays: Handle arrays where elements change frequently, as updates are efficient.
Advantages:
- Both query and update operations can be performed in O(log n) time.
Disadvantages:
- Requires O(n) space, which can be significant for large datasets.
Segment Trees are particularly useful in scenarios where multiple queries and updates are performed on array intervals, such as in competitive programming and real-time data analysis.
19. What is a Fenwick Tree (Binary Indexed Tree)?
A Fenwick Tree, also known as a Binary Indexed Tree (BIT), is a data structure that provides efficient methods for cumulative frequency tables. It allows querying prefix sums and updating elements in logarithmic time.
Structure:
- Implemented as an array where each element at index i contains the sum of a specific range of the input array elements.
Operations:
- Initialization:
- Construct the tree by processing each element and updating the relevant nodes.
- Update:
- To add a value to an element, update all relevant nodes that include this element in their range.
- Query:
- To get the sum of the first k elements, traverse nodes that contribute to this sum.
Applications:
- Prefix Sum Queries: Efficiently compute the sum of elements from the start of the array to a given index.
- Inversion Count: Determine the number of inversions in an array, which is useful in sorting algorithms.
Advantages:
- Both update and query operations have a time complexity of O(log n).
- Requires O(n) space, making it space-efficient.
Disadvantages:
- Less intuitive than simpler data structures like arrays or linked lists.
Fenwick Trees are particularly useful in scenarios where frequent updates and prefix sum queries are required, such as in computational geometry and financial analysis.
20. What is a B-Tree?
A B-Tree is a self-balancing tree data structure that maintains sorted data, enabling efficient insertion, deletion, and search operations. It is especially useful for systems that handle large blocks of data, such as databases and file systems.
Key Properties:
- Node Structure: Each node holds multiple keys and child pointers.
- Balanced Height: All leaf nodes are at the same depth, ensuring the tree remains balanced.
- Order (m): Specifies the maximum number of children per node. Nodes have at most m children and at least ⌈m/2⌉ children (except the root).
Operations:
- Search: Uses binary search within nodes and follows child pointers recursively.
- Insertion: Adds keys in order, splitting nodes and promoting the middle key if a node exceeds capacity.
- Deletion: Removes keys and merges or redistributes nodes to maintain B-Tree properties.
Applications:
- Databases: For efficient indexing and data retrieval.
- File Systems: To manage directories and file blocks.
- Multilevel Indexing: Handling large indexes that don’t fit into memory.
Advantages:
- Maintains balanced structure with O(log n) time complexity for operations.
- Minimizes disk I/O, making it suitable for storage systems.
Disadvantages:
- More complex to implement compared to simpler structures like binary search trees.
B-Trees are essential for managing large datasets efficiently, providing a reliable method for maintaining sorted data with dynamic insertions and deletions.
21. What is a B+ Tree?
A B+ Tree is an extension of the B-Tree data structure, commonly used in database and file systems to store large amounts of data. It enhances the B-Tree by providing efficient insertion, deletion, and search operations, with all actual data stored in the leaf nodes.
Key Characteristics:
- Node Structure:
- Internal nodes contain only keys and act as guides to the leaf nodes.
- Leaf nodes contain keys and associated data pointers, linked sequentially to facilitate range queries.
- Balanced Height: All leaf nodes are at the same depth, ensuring consistent operation times.
Differences from B-Tree:
- Data Storage:
- In B+ Trees, all data records are stored in the leaf nodes, while internal nodes serve as indexing levels.
- In B-Trees, data can be stored in both internal and leaf nodes.
- Leaf Node Linking: Leaf nodes in B+ Trees are linked, allowing for efficient range queries and ordered traversals.
Operations:
- Search:
- Traverse from the root to the appropriate leaf node using internal keys.
- Retrieve the data from the leaf node.
- Insertion:
- Insert the key in the appropriate leaf node.
- If the leaf node overflows, split it and propagate the split to the parent node if necessary.
- Deletion:
- Remove the key from the leaf node.
- If the leaf node underflows, merge it with a sibling or redistribute keys, adjusting parent nodes as needed.
Applications:
- Database Indexing: Efficiently manage and access large datasets.
- File Systems: Organize files and directories for quick retrieval.
Advantages:
- Supports efficient range queries due to linked leaf nodes.
- Ensures consistent and predictable operation times with balanced height.
Disadvantages:
- More complex to implement compared to simpler data structures.
B+ Trees are widely used in database systems and file systems where efficient data retrieval and range queries are essential.
22. What is a Graph, and how is it represented?
A graph is a fundamental data structure that models pairwise relations between objects. It consists of:
- Vertices (Nodes): Fundamental units that represent entities.
- Edges (Links): Connections between pairs of vertices, indicating relationships.
Types of Graphs:
- Directed Graph (Digraph): Edges have a direction, indicating a one-way relationship from one vertex to another.
- Undirected Graph: Edges have no direction, indicating a bidirectional relationship.
- Weighted Graph: Edges carry weights, representing costs, distances, or capacities.
- Unweighted Graph: Edges do not carry weights.
Graph Representations:
- Adjacency Matrix:
- A 2D array where the cell at row i and column j indicates the presence (and possibly the weight) of an edge between vertex i and vertex j.
- Space Complexity: O(V²), where V is the number of vertices.
- Advantages: Efficient edge look-up; beneficial for dense graphs.
- Disadvantages: Consumes more space, especially for sparse graphs.
- Adjacency List:
- An array of lists; the i-th element contains a list of vertices adjacent to vertex i.
- Space Complexity: O(V + E), where E is the number of edges.
- Advantages: Space-efficient for sparse graphs; faster iteration over neighbors.
- Disadvantages: Edge look-up can be slower compared to adjacency matrices.
Choosing the Right Representation:
- Adjacency Matrix: Suitable for dense graphs where edge existence queries are frequent.
- Adjacency List: Ideal for sparse graphs with fewer edges, optimizing space usage.
Understanding these representations is crucial for implementing graph algorithms effectively, as the choice impacts both time and space complexities.
23. What is Topological Sorting in a Directed Acyclic Graph (DAG)?
Topological sorting is the linear ordering of vertices in a Directed Acyclic Graph (DAG) such that for every directed edge u → v, vertex u appears before vertex v in the ordering.
Key Characteristics:
- Applicability: Only possible for DAGs, as cycles would violate the required ordering.
- Uniqueness: A DAG can have multiple valid topological orderings.
Algorithms for Topological Sorting:
- Depth-First Search (DFS) Based Algorithm:
- Perform a DFS traversal of the graph.
- On finishing the exploration of a vertex, push it onto a stack.
- After completing the DFS for all vertices, pop vertices from the stack to get the topological order.
- Kahn’s Algorithm (BFS Based):
- Compute in-degrees of all vertices.
- Initialize a queue with vertices having zero in-degree.
- While the queue is not empty:
- Dequeue a vertex, add it to the topological order.
- Decrease the in-degree of its neighbors by one.
- If a neighbor’s in-degree becomes zero, enqueue it.
Applications:
- Task Scheduling: Ordering tasks with dependencies.
- Course Prerequisites: Determining the sequence of course completion.
- Compilation Order: Sequencing compilation tasks in programming.
Example:
Consider a graph representing tasks with dependencies:
A → B
A → C
B → D
C → D
A possible topological order is: A, B, C, D.
Topological sorting is essential for scenarios where certain tasks must precede others, ensuring a feasible execution sequence.
24. What is Dijkstra’s Algorithm?
Dijkstra’s Algorithm is a graph search algorithm that finds the shortest path from a source vertex to all other vertices in a graph with non-negative edge weights.
Algorithm Steps:
- Initialization:
- Set the distance to the source vertex as 0 and to all other vertices as infinity.
- Mark all vertices as unvisited and set the source vertex as the current node.
- Visit Neighbors:
- For the current node, consider all its unvisited neighbors.
- Calculate tentative distances through the current node and update if they are smaller than the previously known distances.
- Mark as Visited:
- After considering all neighbors, mark the current node as visited.
- Select the unvisited vertex with the smallest tentative distance as the new current node.
- Repeat:
- Repeat steps 2 and 3 until all vertices are visited or the smallest tentative distance among unvisited vertices is infinity.
Time Complexity:
- Using Min-Heap (Priority Queue): O((V + E) log V), where V is the number of vertices and E is the number of edges.
Applications:
- GPS Navigation Systems: Finding the shortest route.
- Network Routing Protocols: Determining efficient data transfer paths.
- Robotics: Pathfinding for autonomous movement.
Limitations:
- Not suitable for graphs with negative edge weights; algorithms like Bellman-Ford are preferred in such cases.
Dijkstra’s Algorithm is fundamental in fields requiring efficient shortest path computations, ensuring optimal routing and navigation solutions.
25. What is the Bellman-Ford Algorithm?
The Bellman-Ford Algorithm computes the shortest paths from a single source vertex to all other vertices in a weighted graph, accommodating graphs with negative weight edges.
Algorithm Steps:
- Initialization:
- Set the distance to the source vertex as 0 and to all other vertices as infinity.
- Relaxation:
- For each edge (u, v) with weight w, if the distance to v through u is less than the current distance to v, update the distance to v.
- Repeat:
- Repeat the relaxation step for V-1 iterations, where V is the number of vertices.
- Check for Negative-Weight Cycles:
- Perform one more iteration over all edges; if any distance can still be updated, a negative-weight cycle exists.
Time Complexity:
- O(V * E), where V is the number of vertices and E is the number of edges.
Applications:
- Currency Arbitrage Detection: Identifying opportunities for profit in currency exchange by detecting negative weight cycles.
- Network Routing Protocols: Determining the most efficient paths in networks, especially when dealing with varying link costs.
- Graph Analysis: Detecting negative weight cycles in graphs, which can indicate issues like arbitrage opportunities or system instabilities.
Advantages:
- Handles graphs with negative weight edges.
- Detects negative weight cycles.
Disadvantages:
- Slower compared to algorithms like Dijkstra’s for graphs without negative weight edges.
The Bellman-Ford Algorithm is versatile, especially in scenarios where negative weights are present, providing both shortest path solutions and cycle detection capabilities.
26. What is the Floyd-Warshall Algorithm?
The Floyd-Warshall Algorithm is a dynamic programming technique used to find the shortest paths between all pairs of vertices in a weighted graph.
Algorithm Steps:
- Initialization:
- Create a distance matrix where the cell (i, j) holds the weight of the edge from vertex i to vertex j; if no edge exists, set it to infinity.
- Iterative Update:
- For each vertex k, update the distance matrix by considering paths that go through k:
- For each pair of vertices (i, j), set distance[i][j] = min(distance[i][j], distance[i][k] + distance[k][j]).
- For each vertex k, update the distance matrix by considering paths that go through k:
Time Complexity:
- O(V³), where V is the number of vertices.
Applications:
- Shortest Path Computation: Finding shortest paths between all pairs of nodes in dense graphs.
- Transitive Closure: Determining reachability of nodes.
- Network Analysis: Evaluating all-pairs shortest paths in communication networks.
Advantages:
- Handles both positive and negative weight edges (without negative weight cycles).
- Provides shortest paths between all pairs of vertices.
Disadvantages:
- Inefficient for large, sparse graphs due to its cubic time complexity.
The Floyd-Warshall Algorithm is particularly useful in dense graphs where shortest paths between all pairs of vertices are required.
27. What is a Minimum Spanning Tree (MST)?
A Minimum Spanning Tree (MST) of a weighted, connected, undirected graph is a subset of the edges that connects all vertices together without any cycles and with the minimum possible total edge weight.
Key Characteristics:
- Spanning Tree: A subgraph that includes all vertices with the minimum number of edges required to connect them.
- Minimum Weight: Among all possible spanning trees, the MST has the least total edge weight.
Algorithms to Find MST:
- Kruskal’s Algorithm:
- Sort all edges in ascending order of their weights.
- Add edges one by one to the MST, ensuring no cycles are formed, until all vertices are connected.
- Prim’s Algorithm:
- Start with an arbitrary vertex and grow the MST by adding the smallest edge that connects a vertex in the MST to a vertex outside it.
Applications:
- Network Design: Designing least-cost networks like electrical grids, computer networks, and road networks.
- Approximation Algorithms: Serving as a basis for algorithms that approximate solutions to complex problems.
- Cluster Analysis: Identifying natural groupings in data.
Properties:
- A graph can have multiple MSTs with the same total weight.
- If all edge weights are distinct, the MST is unique.
Understanding MSTs is crucial in fields like network design and optimization, where connecting components efficiently is essential.
28. What is Kruskal’s Algorithm?
Kruskal’s Algorithm is a greedy method for finding the Minimum Spanning Tree (MST) of a connected, undirected graph. It works by sorting all edges in ascending order of their weights and adding them one by one to the MST, ensuring that no cycles are formed. This process continues until the MST includes all vertices with exactly V − 1 edges, where V is the number of vertices.
Key Steps:
- Sort Edges: Arrange all edges by increasing weight.
- Initialize Subsets: Use a disjoint-set (union-find) structure to manage connected components.
- Add Edges: Iteratively add the smallest edge that doesn’t create a cycle, using the union operation to merge subsets.
Time Complexity: O (E log E), primarily due to edge sorting.
Applications:
- Network Design: Optimizing the layout of telecommunications or computer networks.
- Cluster Analysis: Identifying natural groupings in data.
- Approximation Algorithms: Serving as a base for more complex network design solutions.
Advantages:
- Efficient for sparse graphs.
- Simple to implement with union-find structures.
Disadvantages:
- Sorting all edges can be time-consuming for graphs with many edges.
- Managing the union-find structure adds implementation complexity.
Kruskal’s Algorithm is essential in computer science for optimizing network structures and solving various graph-related problems efficiently.
29. What is Prim’s Algorithm?
Prim’s Algorithm is a greedy algorithm that finds the Minimum Spanning Tree (MST) of a connected, undirected graph by building the tree incrementally, starting from an arbitrary vertex and adding the smallest edge that expands the growing MST.
Algorithm Steps:
- Initialization:
- Start with an arbitrary vertex and add it to the MST.
- Initialize a priority queue (min-heap) to keep track of the edges connecting the MST to the remaining vertices, prioritized by edge weight.
- Expand MST:
- While the MST does not include all vertices:
- Extract the edge with the minimum weight from the priority queue.
- If the edge connects a vertex not yet in the MST, add the edge to the MST and include the new vertex.
- Update the priority queue with the edges connecting the newly added vertex to the remaining vertices, ensuring no cycles are formed.
- While the MST does not include all vertices:
Time Complexity:
- The time complexity of Prim’s Algorithm is O(E log V), where E is the number of edges and V is the number of vertices. This efficiency is achieved using a priority queue to select the minimum weight edge.
Applications:
- Network Design: Prim’s Algorithm is used in designing networks like electrical grids and computer networks, ensuring minimal connection costs.
- Approximation Algorithms: It serves as a basis for algorithms that approximate solutions to complex optimization problems.
- Cluster Analysis: Prim’s Algorithm helps in identifying clusters within data by connecting points with minimal distances.
Advantages:
- Efficient for dense graphs, where the number of edges is large.
- Provides a straightforward approach to building the MST by expanding from a single vertex.
Disadvantages:
- Requires efficient data structures like priority queues for optimal performance, which can add implementation complexity.
Prim’s Algorithm is a fundamental technique in graph theory, offering an effective method for constructing minimum spanning trees, essential in optimizing various network-related problems.
30. What is the Difference Between Prim’s and Kruskal’s Algorithms?
Both Prim’s and Kruskal’s algorithms find the Minimum Spanning Tree (MST) of a graph but differ in their approaches and optimal use cases.
- Approach:
- Prim’s Algorithm:
- Vertex-based: Starts from an arbitrary vertex and grows the MST by adding the smallest edge that connects a new vertex.
- Kruskal’s Algorithm:
- Edge-based: Sorts all edges by weight and adds the smallest edge to the MST, ensuring no cycles are formed.
- Prim’s Algorithm:
- Data Structures:
- Prim’s: Utilizes a priority queue (min-heap) to select the next smallest edge.
- Kruskal’s: Uses a disjoint-set (union-find) structure to detect and prevent cycles.
- Efficiency:
- Prim’s: More efficient for dense graphs with many edges.
- Time Complexity: O(E + VlogV)
- Kruskal’s: More efficient for sparse graphs with fewer edges.
- Time Complexity: O(E log E) or O(E log V)
- Prim’s: More efficient for dense graphs with many edges.
- Graph Suitability:
- Prim’s: Best for connected, dense graphs.
- Kruskal’s: Can handle disconnected graphs by forming a minimum spanning forest.
- Cycle Handling:
- Prim’s: Avoids cycles by always connecting to the nearest unvisited vertex.
- Kruskal’s: Explicitly checks for cycles using the union-find structure before adding an edge.
Summary:
- Prim’s Algorithm is ideal for dense, connected graphs and builds the MST by expanding from a starting vertex.
- Kruskal’s Algorithm is suited for sparse graphs and constructs the MST by selecting the smallest available edges without forming cycles.
Both algorithms achieve the same goal but are optimized for different types of graph structures.
31. What is a Trie?
A Trie is a tree-like data structure used to store a dynamic set of strings, such as a dictionary. Each node represents a character, and paths from the root to a node form a word.
Key Features:
- Hierarchical Structure: Each level represents a character position in the strings.
- Prefix Sharing: Common prefixes are stored once, optimizing space.
- End-of-Word Indicators: Nodes mark the completion of words.
Operations:
- Insertion: Add characters sequentially, creating nodes as needed and marking the end of the word.
- Search: Traverse nodes based on characters; a word exists if the end marker is reached.
- Deletion: Remove the end marker and prune nodes if they are no longer part of other words.
Applications:
- Autocomplete Systems: Suggest word completions based on prefixes.
- Spell Checkers: Validate and suggest correct words.
- IP Routing: Manage routing tables in networks.
- Genome Analysis: Store and search DNA sequences.
Advantages:
- Efficient Search: O(L) time complexity, where L is the length of the string.
- Supports Prefix Queries: Ideal for autocomplete and similar features.
Disadvantages:
- High Memory Usage: Can consume a lot of space, especially with many unique prefixes.
- Complex Implementation: More intricate than simpler structures like hash tables.
Tries are powerful for applications requiring fast and efficient string retrieval and prefix-based operations, making them essential in areas like autocomplete, spell checking, and network routing.
Learn More: Carrer Guidance | Hiring Now!
Top 35+ Angular Interview Questions and Answers for Developers with 5 Years of Experience
Android Interview Questions for Senior Developer with Detailed Answers
SQL Query Interview Questions for Freshers with Answers
GCP (Google Cloud Platform) Interview Questions and Answers
Selenium Coding Interview Questions and Answers
Power Automate Interview Questions for Freshers with Answers
Mobile Testing Interview Questions and Answers- Basic to Advanced