Homework Help: Questions and Answers: The following algorithms were given for computing the nth power of a positive integer a. Use induction to prove that each of these algorithms is correct. (b) Hint: you need cases due to last two if-statements.
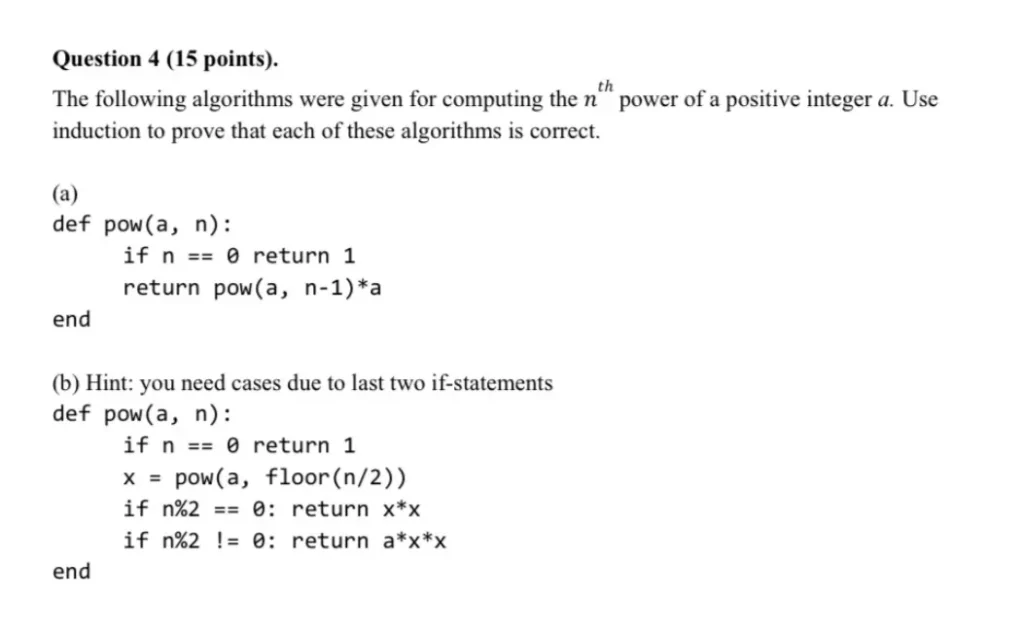
Solution: Step by Step Answering
To prove the correctness of the given algorithms using induction, we need to follow these steps:
- Base Case: Show that the algorithm works for the smallest input value, typically n = 0.
- Inductive Step: Assume the algorithm works for some arbitrary value k, and then show it works for k + 1.
Part (a)
Algorithm:
def pow(a, n):
if n == 0:
return 1
return pow(a, n-1) * a
Base Case
For n = 0:
pow (a, 0) = 1
This is correct because a0 = 1 for any positive integer a.
Inductive Step
Assume the algorithm works for n = k, i.e., assume:
pow (a, k) = ak
We need to show that the algorithm works for n = k + 1:
pow (a, k + 1) = pow (a, (k+1) – 1) . a = pow (a, k). a
By the induction hypothesis:
pow (a, k) = ak
Therefore:
pow (a, k + 1) = ak . a = ak + 1
Since both the base case and the inductive step are proven, by mathematical induction, the algorithm correctly computes an or all non-negative integers n.
Part (b)
Algorithm:
def pow(a, n):
if n == 0:
return 1
x = pow(a, floor(n/2))
if n % 2 == 0:
return x * x
if n % 2 != 0:
return a * x * x
Base Case
For n = 0:
pow (a, 0) = 1
This is correct because a0=1 for any positive integer a.
Inductive Step
Assume the algorithm works for n = k, i.e., assume:
pow (a, k) = ak
We need to show that the algorithm works for n = k + 1:
- If k + 1 is even:
Let k + 1 = 2m for some integer m
pow (a, 2m) = pow (a, m) . pow (a, m)
By the induction hypothesis:
pow (a, m) = am
Therefore:
pow (a, 2m) = am . am = a2m - If k + 1 is odd:
Let k + 1 = 2m + 1 for some integer m.
pow (a, 2m + 1) = a. pow (a, m). pow (a, m)
By the induction hypothesis:
pow (a, m) = am
Therefore:
pow (a, 2m + 1) = a . am . am = a . a2m = a2m + 1
Since both the base case and the inductive step are proven, by mathematical induction, the algorithm correctly computes an for all non-negative integers n.
This completes the proof for both algorithms using mathematical induction.
Learn More: Homework Help
Q. In cryptography what does the term “secret” refer to?
Q. What is a top data security challenge?
Q. What is important to understand about how generative Al models work?
Q. Which prompt would be used to generate an image of a “cute kitten in a basket”?