Are you preparing for a Spring Boot interview with 10 years of experience? It requires a deep understanding of the framework’s advanced features and best practices. Below is a curated list of 20 Spring Boot interview questions tailored for seasoned/experienced professionals.
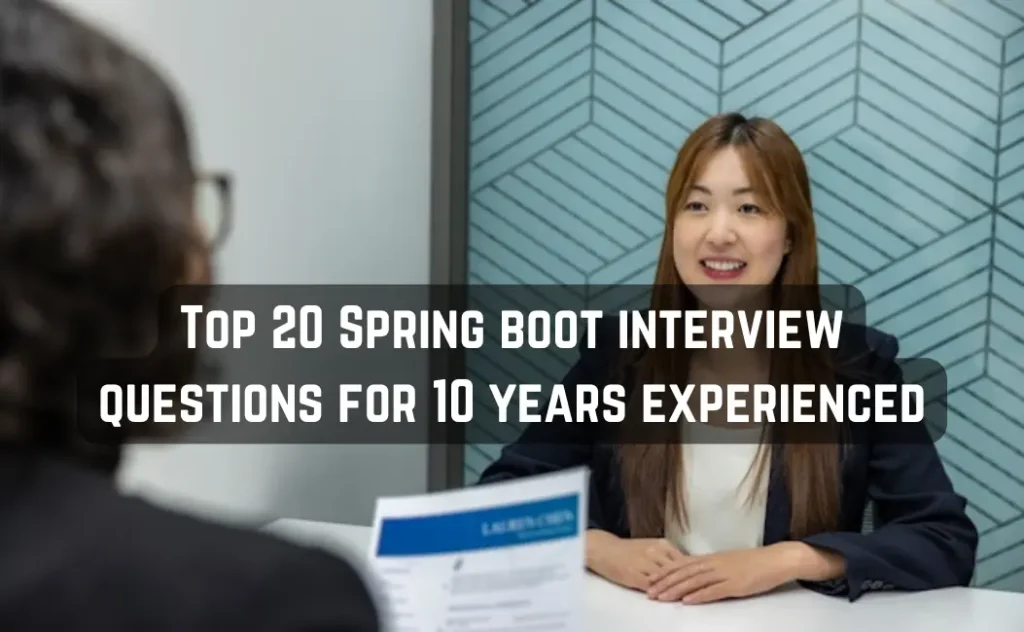
Spring boot interview questions for 10 years experienced
- What is Spring Boot, and how does it differ from the Spring Framework?
- Explain the key features of Spring Boot.
- How does Spring Boot’s auto-configuration mechanism work?
- What are Spring Boot Starters, and how do they simplify project setup?
- Describe the purpose and benefits of Spring Boot Actuator.
- How can you customize the embedded server in a Spring Boot application?
- What strategies can you employ to handle exceptions globally in Spring Boot?
- Discuss the role of @SpringBootApplication annotation.
- How do you manage externalized configuration in Spring Boot?
- Explain the concept of profiles in Spring Boot and their practical applications.
- What are the different ways to deploy a Spring Boot application?
- How does Spring Boot support microservices architecture?
- What is Spring Boot DevTools, and how does it enhance the development process?
- How can you secure a Spring Boot application?
- Describe the process of integrating a database using Spring Data JPA in Spring Boot.
- What is the significance of the application.properties and application.yml files?
- How do you implement caching in a Spring Boot application?
- What are the best practices for logging in Spring Boot applications?
- Discuss the role of Spring Boot CLI and its use cases.
1. What is Spring Boot, and how does it differ from the Spring Framework?
Answer: Spring Boot is an opinionated framework built on top of the traditional Spring Framework. It simplifies the development of stand-alone, production-grade Spring applications by offering defaults for configuration and providing embedded servers like Tomcat or Jetty. Unlike the traditional Spring Framework, which requires extensive XML configuration and setup, Spring Boot reduces boilerplate code and eliminates the need for manual configuration by leveraging auto-configuration and convention over configuration principles.
Key Differences:
- Auto-Configuration: Spring Boot automatically configures your application based on the dependencies present in the classpath.
- Embedded Servers: It provides embedded servlet containers, eliminating the need for deploying WAR files to external servers.
- Starter Dependencies: Spring Boot offers starter POMs to simplify dependency management.
- Production-Ready Features: Includes metrics, health checks, and externalized configuration out of the box.
2. Explain the key features of Spring Boot.
Answer: Key Features of Spring Boot:
- Auto-Configuration: Automatically configures Spring applications based on the dependencies and beans available in the classpath.
- Starter Dependencies: Simplifies Maven or Gradle configuration by providing a set of convenient dependency descriptors.
- Embedded Server Support: Comes with embedded Tomcat, Jetty, or Undertow servers to run applications without deploying to an external server.
- Actuator: Provides production-ready features like health checks, metrics, and monitoring.
- Externalized Configuration: Supports external configuration properties, making it easy to manage different environments.
- CLI Tool: Offers a command-line interface for quick prototyping with Groovy scripts.
- Spring Initializer: A web tool to quickly bootstrap new projects with the necessary dependencies.
3. How does Spring Boot’s auto-configuration mechanism work?
Answer: Spring Boot’s auto-configuration works by scanning the classpath and applying default configurations based on the found dependencies. It uses @EnableAutoConfiguration
and various @Conditional
annotations to conditionally configure beans. The mechanism checks for specific classes and properties, and if certain criteria are met, it auto-configures the necessary components.
How It Works:
- Classpath Scanning: Detects dependencies and beans available in the application.
- Conditional Configuration: Uses
@ConditionalOnClass
,@ConditionalOnMissingBean
, etc., to apply configurations conditionally. - Meta-Annotations:
@SpringBootApplication
includes@EnableAutoConfiguration
, which triggers the auto-configuration process. - Auto-Configuration Classes: Located under
META-INF/spring.factories
, these classes define the auto-configuration logic.
Developers can exclude or customize auto-configuration using @EnableAutoConfiguration(exclude = { ... })
or by defining custom configuration.
4. What are Spring Boot Starters, and how do they simplify project setup?
Answer: Spring Boot Starters are dependency descriptors that aggregate common libraries and configurations for a particular functionality. They simplify project setup by providing a set of convenient Maven or Gradle dependencies that include all the necessary transitive dependencies for a specific feature.
Benefits:
- Simplified Dependency Management: Reduce the need to specify multiple individual dependencies.
- Consistency: Ensure that compatible versions of dependencies are used together.
- Ease of Use: Quick inclusion of desired features like web, data, or security without manual configuration.
Example:
spring-boot-starter-web
: Includes dependencies for building web applications using Spring MVC, along with an embedded Tomcat server.
5. Describe the purpose and benefits of Spring Boot Actuator.
Purpose: Spring Boot Actuator provides production-ready features that help monitor and manage applications. It exposes operational information about the running application, such as health, metrics, info, environment, and more.
Benefits:
- Monitoring and Management: Offers endpoints to monitor application health, track metrics, and manage configurations.
- Customizable Endpoints: Allows developers to add custom endpoints or customize existing ones.
- Integration with Tools: Compatible with monitoring tools like Prometheus, Grafana, and Spring Boot Admin.
- Security: Actuator endpoints can be secured using Spring Security to restrict access to sensitive information.
Common Endpoints:
/actuator/health
: Displays application health status./actuator/metrics
: Shows various application metrics./actuator/env
: Exposes current environment properties.
6. How can you customize the embedded server in a Spring Boot application?
Answer: You can customize the embedded server in Spring Boot by configuring properties in application.properties
or application.yml
, or by programmatically customizing the server settings.
Methods:
- Properties Configuration:
- Port Change:
server.port=8081
- Context Path:
server.servlet.context-path=/myapp
- Port Change:
- Programmatic Configuration:
- Implementing a
WebServerFactoryCustomizer
:
- Implementing a
@Component
public class ServerCustomization implements WebServerFactoryCustomizer<ConfigurableWebServerFactory> {
@Override
public void customize(ConfigurableWebServerFactory factory) {
factory.setPort(8081);
factory.setContextPath("/myapp");
}
}
Customizing SSL, Compression, and Other Features:
- SSL Configuration:
server.ssl.enabled=true
server.ssl.key-store=classpath:keystore.p12
server.ssl.key-store-password=changeit
server.ssl.key-store-type=PKCS12
7. What strategies can you employ to handle exceptions globally in Spring Boot?
Answer: Strategies for Global Exception Handling:
- Controller Advice with
@RestControllerAdvice
or@ControllerAdvice
:- Example:
@RestControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<String> handleResourceNotFound(ResourceNotFoundException ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.NOT_FOUND);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleException(Exception ex) {
return new ResponseEntity<>("Internal Server Error", HttpStatus.INTERNAL_SERVER_ERROR);
}
}
- Extending
ResponseEntityExceptionHandler
:- Provides default implementations for common exceptions which can be overridden.
- Using
@ExceptionHandler
at Controller Level:- Handles exceptions within a specific controller.
- Implementing
HandlerExceptionResolver
:- For advanced exception handling scenarios.
Benefits:
- Centralizes exception handling logic.
- Improves code readability and maintainability.
- Provides consistent error responses to clients.
8. Discuss the role of @SpringBootApplication
annotation.
Answer: The @SpringBootApplication
annotation is a composite annotation that combines several important annotations to simplify the configuration of Spring Boot applications.
It Combines:
@SpringBootConfiguration
: A specialization of@Configuration
, indicating that the class provides Spring Boot application configuration.@EnableAutoConfiguration
: Enables Spring Boot’s auto-configuration mechanism.@ComponentScan
: Enables component scanning, allowing Spring to detect and register beans in the application context.
Role and Benefits:
- Entry Point: Marks the main class of a Spring Boot application.
- Simplification: Reduces the need to declare multiple annotations.
- Customization: You can customize the component scan by specifying base packages.
@SpringBootApplication(scanBasePackages = "com.example.app")
9. How do you manage externalized configuration in Spring Boot?
Answer: Spring Boot allows externalized configuration to enable different configurations for various environments without changing the codebase.
Methods:
- Properties and YAML Files:
application.properties
orapplication.yml
in the classpath.- Environment-specific files like
application-dev.properties
.
- Environment Variables and Command-Line Arguments:
- Override properties using
-Dproperty=value
or environment variables.
- Override properties using
- Profiles:
- Activate profiles to load specific configurations.
- Configuration Classes with
@ConfigurationProperties
:- Bind external properties to Java objects.
@ConfigurationProperties(prefix = "app") public class AppProperties { private String name; // getters and setters }
- Bind external properties to Java objects.
- Spring Cloud Config:
- Centralized configuration management across multiple services.
- Placeholders in Annotations:
- Use
${property}
placeholders in annotations like@Value
.
- Use
Benefits:
- Flexibility: Easily switch configurations for different environments.
- Security: Externalize sensitive data like passwords.
- Maintainability: Centralize configuration management.
10. Explain the concept of profiles in Spring Boot and their practical applications.
Concept:
Profiles in Spring Boot allow you to segregate parts of your application configuration and make it only available in certain environments.
Usage:
- Defining Profiles:
- Annotate beans with
@Profile("dev")
to include them only when the ‘dev’ profile is active.
- Annotate beans with
- Activating Profiles:
- Via properties file:
spring.profiles.active=dev
- Command-line arguments:
java -jar app.jar --spring.profiles.active=prod
- Via properties file:
Practical Applications:
- Environment-Specific Beans:
- Define beans that should only exist in certain environments, like mock implementations for testing.
- Configuration Files:
- Use
application-{profile}.properties
to define profile-specific configurations.
- Use
- Conditional Loading:
- Load different data sources or message brokers based on the active profile.
Benefits:
- Flexibility: Easily switch between environments.
- Separation of Concerns: Keep environment-specific configurations isolated.
- Simplified Deployment: Deploy the same codebase with different configurations.
11. What are the different ways to deploy a Spring Boot application?
Deployment Methods:
- Executable JAR:
- Build a fat JAR using
spring-boot-maven-plugin
and run it usingjava -jar app.jar
.
- Build a fat JAR using
- Traditional WAR Deployment:
- Package the application as a WAR and deploy it to an external servlet container like Tomcat.
- Modify the main application class to extend
SpringBootServletInitializer
.
- Containerization with Docker:
- Create a Docker image of the application and run it in a container.
- Example:
FROM openjdk:17-jdk-alpine
COPY target/app.jar app.jar
ENTRYPOINT ["java","-jar","/app.jar"]
- Cloud Deployment:
- Deploy to cloud platforms like AWS Elastic Beanstalk, Google Cloud Run, or Azure App Service.
- Use platform-specific deployment methods.
- Kubernetes:
- Deploy the Dockerized application to a Kubernetes cluster for orchestration.
- Using Buildpacks:
- Use tools like Paketo or Cloud Native Buildpacks to create OCI-compliant images.
Considerations:
- Environment Variables: Use externalized configurations.
- Scaling: Choose deployment methods that support scaling if necessary.
- Monitoring: Ensure monitoring tools are integrated post-deployment.
12. How does Spring Boot support microservices architecture?
Support for Microservices:
- Embedded Servers:
- Each service can run independently with its own embedded server.
- RESTful Services:
- Simplifies the creation of REST APIs using
@RestController
.
- Simplifies the creation of REST APIs using
- Integration with Spring Cloud:
- Service Discovery: Use Eureka or Consul for service registry.
- Circuit Breakers: Implement resilience patterns with Resilience4j or Hystrix.
- API Gateway: Route requests through a gateway like Spring Cloud Gateway.
- Configuration Management: Use Spring Cloud Config for centralized configuration.
- Messaging Support:
- Integrate with messaging systems like RabbitMQ or Kafka for asynchronous communication.
- Security:
- Secure microservices using OAuth2 and JWT with Spring Security.
- Monitoring and Tracing:
- Integrate Actuator with distributed tracing systems like Zipkin or Sleuth.
Benefits:
- Scalability: Services can be scaled independently.
- Isolation: Fault isolation between services.
- Technology Diversity: Different services can use different technologies if needed.
13. What is Spring Boot DevTools, and how does it enhance the development process?
Spring Boot DevTools:
Spring Boot DevTools is a set of tools that enhance the development experience by providing features like automatic restarts, live reloads, and property defaults for development environments.
Features:
- Automatic Restart:
- Monitors classpath resources and restarts the application when changes are detected.
- LiveReload Integration:
- Automatically refreshes the browser when resources change.
- Property Defaults:
- Adjusts properties to optimize for development, such as caching templates.
- Remote Debugging:
- Supports remote update and restarts over HTTP.
Enhancements:
- Faster Development Cycle: Reduces the time spent on manual restarts.
- Immediate Feedback: See changes in real-time without restarting the server.
- Convenience: Simplifies the development setup.
Usage:
- Include the dependency in
pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<optional>true</optional>
</dependency>
Note: DevTools is automatically disabled in production environments.
14. How can you secure a Spring Boot application?
Methods to Secure a Spring Boot Application:
- Spring Security Integration:
- Use Spring Security to handle authentication and authorization.
- Add the dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
- Define Security Configuration:
- Extend
WebSecurityConfigurerAdapter
or useSecurityFilterChain
bean.
- Extend
@Configuration
public class SecurityConfig {
@Bean
public SecurityFilterChain filterChain(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin();
return http.build();
}
}
- Authentication Mechanisms:
- In-Memory Authentication: For simple cases or testing.
- Database Authentication: Use JDBC or JPA to authenticate against a database.
- LDAP Authentication: Integrate with LDAP servers.
- OAuth2/OpenID Connect: Implement SSO with OAuth2 providers.
- JWT Tokens:
- Use JSON Web Tokens for stateless authentication in RESTful services.
- Method-Level Security:
- Use annotations like
@PreAuthorize
and@Secured
for fine-grained control.
- Use annotations like
- Encrypt Sensitive Data:
- Secure passwords and sensitive information using encryption.
- CSRF Protection:
- Enable CSRF protection for state-changing requests.
- HTTPS Enforcement:
- Configure SSL to encrypt data in transit.
Best Practices:
- Principle of Least Privilege: Grant minimal necessary permissions.
- Password Management: Enforce strong password policies and hashing algorithms.
- Security Headers: Use HTTP security headers like
Content-Security-Policy
.
15. Describe the process of integrating a database using Spring Data JPA in Spring Boot.
Integration Steps:
- Add Dependencies:
- Include Spring Data JPA and a database driver in
pom.xml
.
- Include Spring Data JPA and a database driver in
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
- Configure Data Source:
- Define database connection properties in
application.properties
orapplication.yml
.
- Define database connection properties in
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.username=sa
spring.datasource.password=
spring.jpa.hibernate.ddl-auto=update
- Define Entity Classes:
- Annotate domain classes with
@Entity
and define mappings.
- Annotate domain classes with
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// getters and setters
}
- Create Repository Interfaces:
- Extend
JpaRepository
orCrudRepository
.
- Extend
public interface UserRepository extends JpaRepository<User, Long> {
List<User> findByName(String name);
}
- Enable JPA Repositories:
- Use
@EnableJpaRepositories
if your repositories are in a different package.
- Use
- Use Repositories in Services:
- Inject the repository and use CRUD methods.
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public User saveUser(User user) {
return userRepository.save(user);
}
}
Benefits:
- Simplifies Data Access Layer: Reduces boilerplate code.
- Query Methods: Allows the creation of queries by simply defining method names.
- Pagination and Sorting: Built-in support for paginated and sorted queries.
16. What is the significance of the application.properties
and application.yml
files?
Significance:
- Configuration Management: These files are used to define application configuration properties.
- Externalized Configuration: Allows properties to be externalized from the codebase, supporting different environments.
Usage:
- Default Configuration Files:
- Spring Boot automatically loads
application.properties
orapplication.yml
from the classpath.
- Spring Boot automatically loads
- Defining Properties:
- Set up database configurations, server ports, logging levels, etc.
server.port=8081
spring.jpa.show-sql=true
- Set up database configurations, server ports, logging levels, etc.
- Profile-Specific Configurations:
- Use
application-{profile}.properties
for environment-specific settings.
- Use
- Hierarchical Configuration with YAML:
application.yml
supports hierarchical data, making complex configurations more readable.
server:
port: 8081
spring:
jpa:
show-sql: true
Benefits:
- Flexibility: Easily change configurations without modifying the code.
- Clarity: Keep configuration separate from business logic.
- Environment Management: Simplify deployment to different environments.
17. How do you implement caching in a Spring Boot application?
Implementation Steps:
- Add Cache Dependency (if necessary):
- For concurrent maps:
server:
port: 8081
spring:
jpa:
show-sql: true
- For Ehcache, Redis, etc., include the relevant dependency.
Enable Caching:
Add @EnableCaching
to a configuration class.
@Configuration
@EnableCaching
public class CacheConfig {
// Cache manager beans if needed
}
Configure Cache Manager:
Optional, Spring Boot auto-configures a suitable CacheManager.
Use Caching Annotations:
- @Cacheable: Caches the result of a method.
@Cacheable("users")
public User getUser(Long id) {
// Method implementation
}
- @CachePut: Updates the cache without interfering with the method execution.
- @CacheEvict: Removes entries from the cache.
- @Caching: Combines multiple cache annotations.
Configure Cache Properties:
- Set cache properties in
application.properties
if needed.
External Cache Stores:
- For Redis or Ehcache, configure the cache store accordingly.
Benefits:
- Performance Improvement: Reduces method execution time for resource-intensive operations.
- Scalability: Improves application scalability by reducing load on backend systems.
18. Explain the use of @RestController
and how it differs from @Controller
.
@RestController
:
- A specialized version of
@Controller
that combines@Controller
and@ResponseBody
. - Automatically serializes return objects to JSON/XML and writes it to the HTTP response.
Usage:
@RestController
public class UserController {
@GetMapping("/users")
public List<User> getUsers() {
return userService.findAll();
}
}
Differences from @Controller
:
- View Resolution:
@Controller
: Returns a view name, and a view resolver resolves it to a template.@RestController
: Returns the object itself; the response is converted usingHttpMessageConverter
.
- Response Handling:
@Controller
: Requires@ResponseBody
on each method to return data directly.@RestController
: Eliminates the need for@ResponseBody
on each method.
When to Use:
@RestController
:- For RESTful web services where the response is data (JSON/XML).
@Controller
:- For web applications that return views (HTML templates).
19. What are the best practices for logging in Spring Boot applications?
Best Practices:
- Use SLF4J with Logback:
- Spring Boot uses SLF4J with Logback by default.
- Allows for consistent logging API with pluggable backends.
- Define Logging Levels Appropriately:
- Use
TRACE
,DEBUG
,INFO
,WARN
,ERROR
levels appropriately. - Avoid logging sensitive information.
- Use
- Externalize Logging Configuration:
- Use
application.properties
to configure logging levels. logging.level.com.example=DEBUG
- Alternatively, use
logback-spring.xml
for advanced configurations.
- Use
- Structured Logging:
- Use log formats that are easily parseable by log management tools.
- Include contextual information like request IDs.
- Avoid Logging Sensitive Data:
- Be cautious not to log passwords, tokens, or personal data.
- Asynchronous Logging:
- Configure asynchronous logging for high-throughput applications.
- Centralized Logging:
- Integrate with log aggregation tools like ELK stack (Elasticsearch, Logstash, Kibana).
- Exception Logging:
- Log exceptions with stack traces at appropriate levels.
- Use Placeholders:
- Use
{}
placeholders instead of string concatenation for performance. logger.debug("User {} logged in", username);
- Use
- Monitor Log Size:
- Implement log rotation policies to prevent disk space issues.
20. Discuss the role of Spring Boot CLI and its use cases.
Answer: Spring Boot CLI (Command Line Interface) is a command-line tool that allows developers to quickly prototype Spring applications using Groovy scripts. It simplifies the development process by reducing boilerplate code and leveraging Spring Boot’s auto-configuration capabilities.
Key Features:
- Rapid Prototyping:
- Enables developers to write applications with minimal code.
- Ideal for experimenting with new ideas or creating quick demos.
- Groovy Integration:
- Supports Groovy, a dynamic language for the Java platform.
- Allows for concise and expressive code.
- Automatic Dependency Resolution:
- Automatically adds necessary dependencies based on the code.
- Simplifies dependency management.
Use Cases:
- Microservices Development:
- Quickly spin up lightweight services.
- Learning and Testing:
- Experiment with Spring Boot features without full project setup.
- Scripting:
- Automate tasks or write small utilities.
Example Usage:
Create a simple web application in a file named app.groovy
:
@RestController
class HelloController {
@GetMapping("/")
String home() {
"Hello, Spring Boot CLI!"
}
}
Run the application:
spring run app.groovy
Advantages:
- Speed: Reduces setup time for new applications.
- Simplicity: Minimal configuration required.
- Flexibility: Easily modify and run scripts.
Learn More: Carrer Guidance
Top 30 Entity Framework Interview Questions and Answers for Freshers
Full Stack Developer Interview Questions and Answers
Avasoft Interview Questions and answers- Basic to Advanced
Deloitte NLA Interview Questions and Answers
ADF Interview Questions Scenario based Questions with detailed Answers
Generative AI System Design Interview Questions and Answers- Basic to Advanced
Business Development Executive Interview Questions and Answers