Spring Boot is a widely-used framework in Java development, especially for building microservices and web applications. Below are some of the most common interview questions for freshers, along with detailed answers to help you prepare effectively. Below are 40+ essential spring boot interview questions and answers for freshers to help you prepare for your next interview.
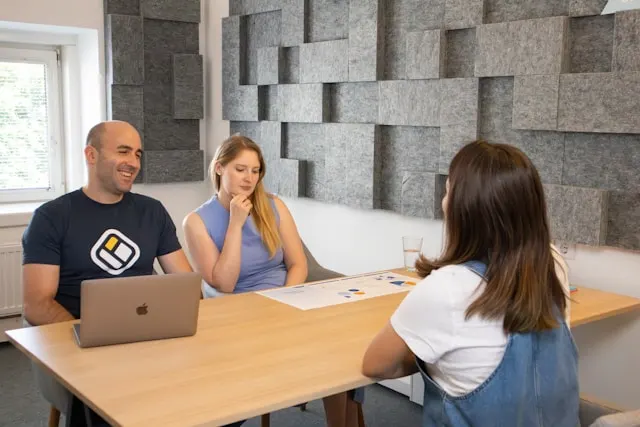
Spring Boot Interview Questions and Answers for Freshers
1. What is Spring Boot?
2. What are the advantages of using Spring Boot?
3. What are the key components of Spring Boot?
4. How do you create a Spring Boot application?
5. What is Spring Boot Actuator?
6. Explain the concept of auto-configuration in Spring Boot.
7. What are Spring Boot Starters?
8. How do you handle exceptions in a Spring Boot application?
9. What is Thymeleaf?
10. How do you connect a Spring Boot application to a database using JPA?
11. What is @RestController annotation?
12. Explain how dependency management works in Spring Boot.
13. What is the purpose of the application.properties file?
14. How do you secure a REST API in Spring Boot?
15. What are some common annotations used in Spring Boot?
16. How do you test a Spring Boot application?
17. What is the difference between @Component, @Service, @Repository, and @Controller annotations?
18. How do you deploy a Spring Boot application?
19. Explain how caching works in Spring Boot.
20. What is the role of profiles in Spring Boot?
21. What is Spring Boot Initializr, and how does it help in creating Spring Boot applications?
22. What is the @SpringBootApplication annotation, and why is it used?
23. Explain the difference between Spring Boot and Spring MVC.
24. How do you configure a custom banner in Spring Boot?
25. What is the purpose of @EnableAutoConfiguration?
26. How does Spring Boot manage embedded servers, and what are the supported server types?
27. How can you create custom properties in Spring Boot?
28. What is Spring Boot DevTools, and how does it enhance development?
29. How do you implement pagination in a Spring Boot application?
30. What is a CommandLineRunner in Spring Boot?
31. How can you enable asynchronous processing in Spring Boot?
32. What is the @PathVariable annotation used for in Spring Boot?
33. How does Spring Boot handle JSON serialization and deserialization?
34. How do you customize the embedded server configuration in Spring Boot?
35. What are health indicators in Spring Boot Actuator?
36. How do you configure an external configuration file in Spring Boot?
37. What is the role of the @Profile annotation?
38. How does Spring Boot handle scheduling?
39. What are Spring Boot Starters, and how do you create a custom starter?
40. How do you perform exception handling in REST APIs in Spring Boot?
1. What is Spring Boot?
Answer:
Spring Boot is an open-source framework that simplifies the process of building stand-alone, production-grade Spring applications. It is built on top of the Spring framework and offers a range of features that streamline configuration and deployment. The main goal of Spring Boot is to reduce the amount of boilerplate code and configuration required to set up a Spring application.
Benefits:
- Rapid Development: It allows developers to create applications quickly with minimal setup.
- Embedded Servers: Spring Boot applications can run on embedded servers like Tomcat or Jetty, eliminating the need for external server installations.
- Auto-Configuration: Automatically configures your application based on the libraries present in your project.
2. What are the advantages of using Spring Boot?
Answer: The advantages of using Spring Boot include:
- Reduced Development Time: It minimizes the need for complex configurations, allowing developers to focus on business logic.
- Convention over Configuration: Provides default configurations that can be overridden as needed, reducing the setup time for new projects.
- Microservices Ready: Designed to support microservices architecture, making it easier to build scalable applications.
- Built-in Features: Includes features like health checks, metrics, and monitoring through Actuator.
3. What are the key components of Spring Boot?
Answer: Key components of Spring Boot include:
- Spring Boot Auto-Configuration: Automatically configures your application based on the dependencies present in your project.
- Spring Boot Starters: A set of pre-defined dependencies that simplify dependency management (e.g.,
spring-boot-starter-web
for web applications). - Spring Boot CLI: A command-line tool that allows you to run and test Spring applications quickly using Groovy scripts.
- Spring Boot Actuator: Provides production-ready features such as health checks and metrics monitoring.
4. How do you create a Spring Boot application?
Answer: You can create a Spring Boot application using several methods:
- Using Spring Initializr:
- Go to the Spring Initializr website.
- Select project metadata (Group, Artifact, Name).
- Choose dependencies (like Web, JPA).
- Generate the project and download it as a ZIP file.
- Using Maven/Gradle:
- Create a new Maven or Gradle project.
- Add the necessary dependencies in
pom.xml
orbuild.gradle
. - Write your application code starting with a main class annotated with
@SpringBootApplication
.
5. What is Spring Boot Actuator?
Answer:
Spring Boot Actuator is a module that provides built-in endpoints to monitor and manage your application. It exposes various endpoints that provide insights into the application’s health, metrics, environment properties, and more.Common Endpoints:
/actuator/health
: Displays the health status of the application./actuator/metrics
: Provides various metrics about your application (e.g., memory usage).
6. Explain the concept of auto-configuration in Spring Boot.
Answer:
Auto-configuration in Spring Boot automatically configures your application based on the libraries present in your classpath. For example, if you have spring-webmvc
in your dependencies, Spring Boot will automatically configure necessary beans like DispatcherServlet
, without requiring explicit configuration from you.This feature significantly reduces boilerplate code and configuration files, allowing developers to get started quickly.
7. What are Spring Boot Starters?
Answer:
Spring Boot Starters are a set of convenient dependency descriptors that simplify adding dependencies to your project. Instead of specifying individual libraries and their versions, you can include a starter dependency that pulls in all required libraries.For example:
spring-boot-starter-web
: Includes everything needed to build web applications (Tomcat, Jackson).spring-boot-starter-data-jpa
: Adds support for JPA and Hibernate.
8. How do you handle exceptions in a Spring Boot application?
Answer: In Spring Boot, exceptions can be handled using @ControllerAdvice
and @ExceptionHandler
annotations:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<?>
handleResourceNotFound(ResourceNotFoundException ex) {
return
ResponseEntity.status(HttpStatus.NOT_FOUND).body(ex.getMessage());
}
}
This approach allows you to centralize exception handling across all controllers.
9. What is Thymeleaf?
Answer:
Thymeleaf is a modern server-side Java template engine used for rendering web pages in Spring applications. It allows developers to create dynamic HTML pages by embedding expressions within HTML tags.
Features:
- Natural templating: Thymeleaf templates can be correctly displayed in browsers without being processed by the server.
- Integration with Spring MVC: Easily integrates with Spring MVC for data binding and form handling.
10. How do you connect a Spring Boot application to a database using JPA?
Answer: To connect a Spring Boot application to a database using JPA:
1. Add dependencies for JPA and your database driver (e.g., H2, MySQL) in pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
2. Configure database properties in application.properties
:
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driver-class-name=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=password
spring.jpa.hibernate.ddl-auto=create
3. Create an entity class annotated with @Entity
and define repository interfaces extending JpaRepository
.
11. What is @RestController annotation?
Answer:
The @RestController
annotation is a specialized version of @Controller
used in RESTful web services. It combines both @Controller
and @ResponseBody
, which means that it handles HTTP requests and automatically serializes responses into JSON or XML format.
Example:
@RestController
@RequestMapping("/api")
public class UserController {
@GetMapping("/users")
public List<User> getAllUsers() {
return userService.findAll();
}
}
12. Explain how dependency management works in Spring Boot.
Answer:
Spring Boot manages dependencies through its starter POMs, which define common libraries used together. When you include a starter dependency, all required libraries are included without needing to specify versions manually. Additionally, when upgrading Spring Boot versions, all dependencies are updated automatically according to compatibility rules defined by the framework.
13. What is the purpose of the application.properties file?
Answer:
The application.properties
file is used to configure various settings for a Spring Boot application. It allows developers to define properties such as database connection details, server port numbers, logging levels, etc.
Example properties:
server.port=8080
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
logging.level.org.springframework=DEBUG
14. How do you secure a REST API in Spring Boot?
Answer:
To secure a REST API in Spring Boot, you can use Spring Security along with OAuth2 or JWT (JSON Web Tokens). Here’s how you can implement basic security:
- Add dependencies for Spring Security.
- Create a security configuration class extending
WebSecurityConfigurerAdapter
. - Override methods like
configure(HttpSecurity http)
to define security rules (e.g., which endpoints require authentication).
Example:
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/api/public/**").permitAll()
.anyRequest().authenticated()
.and()
.httpBasic();
}
}
15. What are some common annotations used in Spring Boot?
Answer: Some commonly used annotations include:
- @SpringBootApplication: Indicates the main class of a Spring Boot application.
- @Autowired: Automatically injects beans into components.
- @ComponentScan: Specifies packages to scan for components.
- @Value: Injects values from properties files into fields.
16. How do you test a Spring Boot application?
Answer:
Testing in Spring Boot can be done using JUnit along with Mockito for mocking dependencies. You can write unit tests for service classes and integration tests for controllers.
Example test case:
@RunWith(SpringRunner.class)
@SpringBootTest
public class UserServiceTest {
@Autowired
private UserService userService;
@MockBean
private UserRepository userRepository;
@Test
public void testFindAllUsers() {
when(userRepository.findAll()).thenReturn(new ArrayList<>());
List<User> users = userService.findAll();
assertTrue(users.isEmpty());
}
}
17. What is the difference between @Component, @Service, @Repository, and @Controller annotations?
Answer: These annotations are used for defining beans in different layers of an application:
Annotation | Purpose |
---|---|
@Component | General-purpose stereotype for any bean |
@Service | Indicates service layer components |
@Repository | Indicates persistence layer components |
@Controller | Indicates MVC controller components |
Using these specific annotations helps with better organization and clarity within your codebase.
18. How do you deploy a Spring Boot application?
Answer: To deploy a Spring Boot application:
- Package your application as an executable JAR file using Maven or Gradle:
mvn clean package
- Run the JAR file on any server with Java installed:
java -jar target/myapp.jar
- Optionally deploy it on cloud platforms like AWS or Heroku by following their specific deployment guidelines.
19. Explain how caching works in Spring Boot.
Answer:
Caching can be easily implemented in Spring Boot using annotations like @Cacheable
, @CachePut
, and @CacheEvict
. You need to enable caching by annotating your main class with @EnableCaching
.
Example usage:
@Service
public class UserService {
@Cacheable("users")
public User findUserById(Long id) {
// Simulate slow service call
return userRepository.findById(id).orElse(null);
}
}
This caches results from the method so subsequent calls with the same parameters will return cached data instead of hitting the database again.
20. What is the role of profiles in Spring Boot?
Answer:
Profiles allow you to define different configurations for different environments (e.g., development, testing, production). You can specify active profiles using properties files or command-line arguments.
Example usage:
# In application-dev.properties
spring.datasource.url=jdbc:h2:mem:testdb
# In application-prod.properties
spring.datasource.url=jdbc:mysql://prod-db-url/mydb
# Activate profile via command line:
java -jar myapp.jar --spring.profiles.active=prod
This helps maintain clean configurations tailored for specific deployment scenarios while keeping shared settings centralized.
21. What is Spring Boot Initializr, and how does it help in creating Spring Boot applications?
Answer:
Spring Boot Initializr is an online tool provided by Spring to bootstrap new Spring Boot projects with predefined configurations, dependencies, and frameworks. It generates a ready-to-run Spring Boot project with the specified dependencies.
You can specify the project type (Maven/Gradle), Java version, and add dependencies like Spring Web, JPA, and MySQL. It saves time, minimizes setup, and ensures compatibility across dependencies.
22. What is the @SpringBootApplication annotation, and why is it used?
Answer:
The @SpringBootApplication
annotation is a convenience annotation that combines @Configuration
, @EnableAutoConfiguration
, and @ComponentScan
. This enables auto-configuration of the Spring Boot application and component scanning for the package and sub-packages. It is used on the main class to enable these features by default, making it simpler to set up and run the application.
23. Explain the difference between Spring Boot and Spring MVC.
Answer:
Spring Boot is a framework for quickly developing standalone applications with minimal configuration. It includes embedded servers and auto-configuration. Spring MVC is a module within the larger Spring Framework focused on web-based applications, especially those using the Model-View-Controller (MVC) design pattern. Spring Boot can be used to run Spring MVC applications without external server configuration.
24. How do you configure a custom banner in Spring Boot?
Answer:
Custom banners can be displayed at the application startup by placing a banner.txt
file in the src/main/resources
directory. Spring Boot reads this file and displays its contents in the console. ASCII art and text can be added to personalize the application start-up.
25. What is the purpose of @EnableAutoConfiguration?
Answer:
The @EnableAutoConfiguration
annotation enables Spring Boot’s auto-configuration feature. It automatically configures application beans based on the project’s classpath settings, other beans, and property configurations. For instance, if spring-boot-starter-web
is present, Spring Boot will automatically configure an embedded Tomcat server.
26. How does Spring Boot manage embedded servers, and what are the supported server types?
Answer:
Spring Boot includes support for embedded servers like Tomcat, Jetty, and Undertow, allowing developers to package applications as self-contained JAR files. By default, Spring Boot applications are embedded with Tomcat, but this can be switched in the pom.xml
or build.gradle
file by excluding Tomcat and adding dependencies for Jetty or Undertow.
27. How can you create custom properties in Spring Boot?
Answer:
Custom properties can be added to the application.properties
or application.yml
file. You can define custom properties such as app.title=My Application
and access them in your application using the @Value
annotation or Environment
object.
28. What is Spring Boot DevTools, and how does it enhance development?
Answer:
Spring Boot DevTools provides features like automatic restarts, live reload, and configurations for a faster development experience. When changes are made to the codebase, DevTools restarts only the modified parts, making development more efficient by reducing the time needed for manual restarts.
29. How do you implement pagination in a Spring Boot application?
Answer:
Spring Boot supports pagination via Spring Data JPA’s Pageable
interface, which can be passed as a parameter in repository methods. PageRequest.of(page, size)
helps define the page number and page size. This can be utilized in repository methods to automatically retrieve paginated data from the database.
30. What is a CommandLineRunner in Spring Boot?
Answer:
CommandLineRunner
is a functional interface in Spring Boot used to execute specific code after the application context is loaded. It runs on application startup and can be implemented for initialization logic, such as loading data or performing configuration checks.
31. How can you enable asynchronous processing in Spring Boot?
Answer:
Asynchronous processing is enabled by adding the @EnableAsync
annotation to a configuration class and using the @Async
annotation on methods. This allows the methods to run in a separate thread, facilitating non-blocking operations.
32. What is the @PathVariable annotation used for in Spring Boot?
Answer:
The @PathVariable
annotation is used to bind URI template variables to method parameters in controller classes. It enables RESTful handling by allowing dynamic URLs in Spring Boot applications.
33. How does Spring Boot handle JSON serialization and deserialization?
Answer:
Spring Boot uses Jackson as the default library for JSON serialization and deserialization. It automatically converts Java objects to JSON format when returning data in REST APIs. Custom serializers and deserializers can be defined if specific formatting is required.
34. How do you customize the embedded server configuration in Spring Boot?
Answer:
The embedded server can be customized in the application.properties
file. Properties like server.port
, server.servlet.context-path
, and server.tomcat.max-threads
allow adjustments to the server configuration without altering code.
35.What are health indicators in Spring Boot Actuator?
Answer:
Health indicators are part of Spring Boot Actuator that report the health status of various application components like databases, caches, and custom components. These indicators provide real-time health checks through the /actuator/health
endpoint, helping in monitoring and maintenance.
36. How do you configure an external configuration file in Spring Boot?
Answer:
External configuration files can be provided by specifying the location of the application.properties
file in the command line or environment variable. This enables environment-specific configurations without changing the application source code.
37. What is the role of the @Profile annotation?
Answer:
The @Profile
annotation is used to activate specific beans based on the application’s active profile (e.g., dev
, prod
). By setting active profiles, Spring Boot selectively loads beans, configurations, or properties for different environments.
38. How does Spring Boot handle scheduling?
Answer:
Spring Boot allows scheduling with the @EnableScheduling
annotation. The @Scheduled
annotation can be applied to methods to execute tasks at specific intervals, defined using cron expressions or fixed delays.
39. What are Spring Boot Starters, and how do you create a custom starter?
Answer:
Spring Boot Starters are dependency descriptors that simplify adding necessary dependencies to a project. A custom starter can be created by defining dependencies, configurations, and properties in a new project and packaging it as a JAR file, which can then be reused in other applications.
40. How do you perform exception handling in REST APIs in Spring Boot?
Answer:
Spring Boot uses @ExceptionHandler
, @ControllerAdvice
, and @ResponseStatus
to handle exceptions in REST APIs. @ControllerAdvice
allows centralized exception handling across the entire application, enabling the application to return meaningful error messages instead of stack traces.
These questions cover fundamental concepts related to Spring Boot that freshers should understand before attending interviews. Preparing answers based on these topics will help boost confidence during discussions with employers.
Learn More: Carrer Guidance
Cypress Interview Questions with Detailed Answers
PySpark interview questions and answers
Salesforce admin interview questions and answers for experienced
Salesforce admin interview questions and answers for freshers
EPAM Systems Senior Java Developer Interview questions with answers
Flutter Interview Questions and Answers for all levels
Most common data structures and algorithms asked in Optum interviews