When preparing for a Selenium interview, especially with five years of experience, it’s crucial to demonstrate not just theoretical knowledge but also practical expertise. Below are 40 commonly asked selenium interview questions for 5 years experience that reflect the depth of understanding expected from an experienced automation tester.
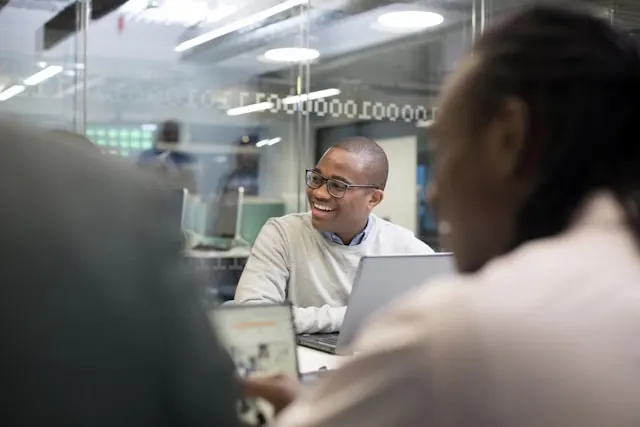
Selenium Interview Questions for Candidates with 5 Years of Experience
- What is Selenium and what are its components?
- Can you explain the difference between assert and verify commands in Selenium?
- How do you handle synchronization issues in Selenium?
- What are the different ways to locate elements in Selenium?
- Explain how you can handle alerts in Selenium WebDriver.
- What is Page Object Model (POM) and why is it useful?
- Describe how you can take screenshots using Selenium WebDriver.
- What are Desired Capabilities in Selenium?
- How do you handle file uploads in Selenium?
- Explain how you can perform drag-and-drop actions using Selenium WebDriver.
- What are some common exceptions encountered while using Selenium?
- How do you perform cross-browser testing with Selenium?
- Can you explain what JavaScriptExecutor is and its use cases?
- What is Fluent Wait and how does it differ from other waits?
- How do you manage dependencies in your automation framework?
- What are some best practices for writing maintainable Selenium tests?
- How do you integrate Selenium with TestNG or JUnit?
- How do you handle dynamic web elements in Selenium?
- Can you explain how you would set up a CI/CD pipeline for automated testing?
- What challenges have you faced while working with Selenium and how did you overcome them?
- How do you handle SSL certificate errors in Selenium WebDriver?
- What is Selenium Grid, and how do you set it up?
- Explain how to handle multiple windows or tabs in Selenium WebDriver.
- How can you handle browser cookies in Selenium?
- What are WebDriverWait and ExpectedConditions, and how are they used in Selenium?
- How can you scroll a web page using Selenium WebDriver?
- How would you handle AJAX calls in Selenium WebDriver?
- Explain the concept of Browser Profiles in Selenium.
- What is the Stale Element Reference Exception, and how do you resolve it?
- How would you validate the presence of an element without causing test failure?
- What is the purpose of the
Action
class in Selenium? - How can you manage browser notifications in Selenium WebDriver?
- Explain how to manage iframes in Selenium.
- How would you manage pop-ups in Selenium WebDriver?
- How do you handle mouse hover actions in Selenium?
- What strategies do you use for debugging Selenium tests?
- How would you handle CAPTCHA in Selenium?
- What is a headless browser, and how do you use it in Selenium?
- How would you verify text color or other CSS properties in Selenium?
- How do you handle file downloads in Selenium WebDriver?
1. What is Selenium and what are its components?
Answer:
Selenium is an open-source automation testing tool primarily used for automating web applications across various browsers and platforms. It consists of several components:
- Selenium IDE: A record-and-playback tool for creating tests without programming knowledge. It is primarily used for simple test creation.
- Selenium WebDriver: A more powerful tool that provides a programming interface to create and execute test scripts in various programming languages like Java, C#, Python, etc. It interacts directly with the browser without any intermediary.
- Selenium Grid: Allows for parallel execution of tests across multiple machines and browsers, enabling efficient testing in different environments simultaneously.
- Selenium RC (Remote Control): An older component that allows the execution of Selenium tests in a browser by injecting JavaScript code into the browser. This has largely been replaced by WebDriver.
2. Can you explain the difference between assert and verify commands in Selenium?
Answer:
Both assert and verify commands are used to check conditions during test execution, but they behave differently:
- Assert: If an assertion fails, the test execution stops immediately. This is useful for critical checks where subsequent steps depend on the success of the assertion. For example:
Assert.assertEquals(expectedValue, actualValue);
- Verify: Unlike assert, if a verification fails, the test continues to execute. This allows for gathering more information about failures without halting the entire test. For example:
SoftAssert softAssert = new SoftAssert();
softAssert.assertEquals(expectedValue, actualValue);
softAssert.assertAll(); // To report all assertions at once
This distinction is crucial in determining how to handle failures based on the context of your tests.
3. How do you handle synchronization issues in Selenium?
Answer:
Synchronization issues arise when there’s a mismatch between the speed of your test execution and the speed at which web elements load on a page. There are several strategies to handle this:
- Implicit Wait: Sets a default waiting time for all elements to be found before throwing an exception.
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
- Explicit Wait: Allows you to wait for a specific condition to occur before proceeding further in the code. This is more flexible than implicit waits.
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementId")));
- Fluent Wait: A type of explicit wait that allows polling at regular intervals until a certain condition is met or a timeout occurs.
Wait<WebDriver> wait = new FluentWait<WebDriver>(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(5))
.ignoring(NoSuchElementException.class);
WebElement element = wait.until(driver -> driver.findElement(By.id("elementId")));
Using these methods effectively can help mitigate timing issues in your tests.
4. What are the different ways to locate elements in Selenium?
Answer:
Locating elements accurately is essential for effective automation testing. Here are various strategies:
- By ID: Most reliable method if IDs are unique.
driver.findElement(By.id("uniqueId"));
- By Name: Useful when elements have unique names.
driver.findElement(By.name("elementName"));
- By Class Name: Can be used when multiple elements share a class.
driver.findElement(By.className("className"));
- By Tag Name: Useful for locating elements by their HTML tag.
driver.findElement(By.tagName("input"));
- By Link Text/Partial Link Text: Useful for hyperlinks.
driver.findElement(By.linkText("Full Link Text"));
driver.findElement(By.partialLinkText("Partial Text"));
- By CSS Selector: Powerful and flexible method using CSS syntax.
driver.findElement(By.cssSelector("div.classname > input[type='text']"));
- By XPath: Can navigate through complex structures.
driver.findElement(By.xpath("//div[@class='classname']/input"));
Choosing the right locator strategy is pivotal based on the structure and attributes of the web page.
5. Explain how you can handle alerts in Selenium WebDriver.
Answer:
Handling alerts is crucial as they can interrupt the flow of automation scripts. Selenium provides methods to manage alerts:
- Switch to Alert: Use
switchTo()
method to focus on the alert.
Alert alert = driver.switchTo().alert();
- Accept or Dismiss Alerts:
- To accept (click OK):
java alert.accept();
- To dismiss (click Cancel):
java alert.dismiss();
- Get Alert Text:
- To retrieve text from an alert:
java String alertMessage = alert.getText();
- Send Keys to Alerts (for prompts):
- If an alert requires input:
java alert.sendKeys("Your Input"); alert.accept();
Proper handling of alerts ensures that your tests can proceed smoothly even when unexpected pop-ups occur.
6. What is Page Object Model (POM) and why is it useful?
Answer:
The Page Object Model (POM) is a design pattern that enhances test maintenance and reduces code duplication by representing each page of an application as a separate class:
- Each class contains methods that correspond to actions that can be performed on that page (e.g., filling out forms, clicking buttons).
- The main benefits include:
- Improved readability and maintainability of tests.
- Easier updates when UI changes occur; only corresponding page classes need modification.
- Encourages reusability of code across different tests.
Example structure:
public class LoginPage {
WebDriver driver;
@FindBy(id = "username")
WebElement username;
@FindBy(id = "password")
WebElement password;
@FindBy(id = "loginBtn")
WebElement loginButton;
public LoginPage(WebDriver driver) {
this.driver = driver;
PageFactory.initElements(driver, this);
}
public void login(String user, String pass) {
username.sendKeys(user);
password.sendKeys(pass);
loginButton.click();
}
}
Using POM leads to cleaner code and easier collaboration among team members.
7. Describe how you can take screenshots using Selenium WebDriver.
Answer:
Taking screenshots during test execution can help in debugging failures by providing visual evidence of application state:
- Use
TakesScreenshot
interface provided by Selenium. - Implement it as follows:
import org.openqa.selenium.OutputType;
import org.openqa.selenium.TakesScreenshot;
import org.openqa.selenium.WebDriver;
import org.apache.commons.io.FileUtils;
import java.io.File;
public void takeScreenshot(WebDriver driver) {
File screenshotFile = ((TakesScreenshot)driver).getScreenshotAs(OutputType.FILE);
try {
FileUtils.copyFile(screenshotFile, new File("path/to/screenshot.png"));
} catch (IOException e) {
e.printStackTrace();
}
}
This method captures a screenshot of the current browser window and saves it to a specified location.
8. What are Desired Capabilities in Selenium?
Answer:
Desired Capabilities are used in Selenium to configure browser settings before initiating a session:
- They allow you to set properties such as browser name, version, platform, and other options like enabling/disabling notifications or setting proxy settings.
Example usage:
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability("browserName", "chrome");
capabilities.setCapability("version", "89");
capabilities.setCapability("platform", "WINDOWS");
WebDriver driver = new RemoteWebDriver(new URL("http://localhost:4444/wd/hub"), capabilities);
This configuration is particularly useful when running tests on different environments or using cloud services.
9. How do you handle file uploads in Selenium?
Answer:
To handle file uploads in web applications using Selenium, you typically interact with <input type="file">
elements directly rather than simulating user interactions with file dialogs:
WebElement uploadElement = driver.findElement(By.id("uploadFile"));
uploadElement.sendKeys("/path/to/file.txt");
This command sends the file path directly to the input field designated for file uploads, bypassing any dialog boxes.
10. Explain how you can perform drag-and-drop actions using Selenium WebDriver.
Answer:
Drag-and-drop actions can be performed using either JavaScript or built-in methods provided by Selenium’s Actions class:
Using Actions class:
Actions actions = new Actions(driver);
WebElement source = driver.findElement(By.id("sourceElement"));
WebElement target = driver.findElement(By.id("targetElement"));
actions.dragAndDrop(source, target).perform();
This method simulates mouse actions effectively within web applications.
11. What are some common exceptions encountered while using Selenium?
Answer:
Common exceptions include:
- NoSuchElementException: Thrown when an element cannot be found using specified locators.
- TimeoutException: Occurs when a command does not complete within the specified time limit.
- StaleElementReferenceException: Happens when an element becomes stale after being located; usually occurs if the DOM changes after locating an element.
Handling these exceptions gracefully ensures that your tests can recover from errors or provide meaningful error messages.
12. How do you perform cross-browser testing with Selenium?
Answer:
Cross-browser testing ensures that your application behaves consistently across different browsers and versions:
- Use Selenium Grid to run tests on multiple browsers simultaneously.
- Configure Desired Capabilities for each browser instance as needed.
- Use tools like BrowserStack or Sauce Labs for cloud-based cross-browser testing solutions.
Example setup with Grid:
DesiredCapabilities capabilities = DesiredCapabilities.chrome(); // or firefox(), etc.
WebDriver driver = new RemoteWebDriver(new URL("http://localhost:4444/wd/hub"), capabilities);
This approach helps identify browser-specific issues early in development.
13. Can you explain what JavaScriptExecutor is and its use cases?
Answer:
JavaScriptExecutor is an interface provided by Selenium that allows executing JavaScript code directly within the context of the currently loaded page:
Use cases include:
- Manipulating DOM elements that may not be accessible through standard WebDriver methods.
- Triggering events such as clicks or form submissions programmatically.
Example usage:
JavascriptExecutor js = (JavascriptExecutor)driver;
js.executeScript("arguments[0].click();", element); // Clicks an element via JavaScript
This capability enhances flexibility when interacting with complex web applications.
14. What is Fluent Wait and how does it differ from other waits?
Answer:
Fluent Wait is an advanced form of explicit wait that allows defining both timeout and polling intervals while ignoring specific exceptions during polling:
Wait<WebDriver> wait = new FluentWait<WebDriver>(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(5))
.ignoring(NoSuchElementException.class);
WebElement element = wait.until(driver -> driver.findElement(By.id("elementId")));
Unlike standard explicit waits, Fluent Wait can be customized extensively based on specific needs during test execution.
15. How do you manage dependencies in your automation framework?
Answer:
Managing dependencies effectively ensures smooth execution of your automation scripts:
- Use build tools like Maven or Gradle to manage project dependencies automatically through
pom.xml
orbuild.gradle
files respectively. - Organize libraries into appropriate directories (e.g.,
libs
,drivers
) within your project structure for easy access during runtime. - Keep dependencies updated regularly to leverage improvements and security patches.
This structured approach minimizes conflicts and enhances maintainability over time.
16. What are some best practices for writing maintainable Selenium tests?
Answer:
Best practices include:
- Implementing Page Object Model (POM) for better organization.
- Using meaningful naming conventions for test methods and classes.
- Keeping tests independent; avoid dependencies between them to ensure one failing test doesn’t affect others.
- Regularly refactoring code to eliminate redundancy and improve clarity.
- Logging important actions and results during test execution for easier debugging later on.
Following these practices contributes significantly to long-term project success.
17. How do you integrate Selenium with TestNG or JUnit?
Answer:
Integrating with TestNG or JUnit allows structured test execution along with reporting features:
For TestNG:
- Annotate your test methods with
@Test
. - Create an XML suite file if needed for running groups of tests together.
Example TestNG setup:
import org.testng.annotations.Test;
public class SampleTest {
@Test
public void sampleTestMethod() {
// Your test code here
}
}
For JUnit:
- Annotate your test methods with
@Test
.
Example JUnit setup:
import org.junit.Test;
public class SampleTest {
@Test
public void sampleTestMethod() {
// Your test code here
}
}
Both frameworks provide rich features like parallel execution, dependency management, and advanced reporting capabilities.
18. How do you handle dynamic web elements in Selenium?
Answer:
Dynamic web elements change their properties during runtime (e.g., IDs generated dynamically). Strategies include:
- Using more stable locators such as CSS selectors or XPath expressions that don’t rely on dynamic attributes.
- Implementing waits (implicit/explicit) to ensure elements are present before interacting with them.
- Using parent-child relationships in XPath expressions to locate elements based on their context rather than fixed attributes.
Example XPath handling dynamic IDs:
//div[contains(@id,'dynamicPart')]/span[text()='StaticText']
These techniques enhance reliability when dealing with unpredictable web applications.
19. Can you explain how you would set up a CI/CD pipeline for automated testing?
Answer:
Setting up a CI/CD pipeline involves integrating automated testing into your development workflow effectively:
- Choose CI/CD tools like Jenkins, GitLab CI/CD, or CircleCI.
- Configure jobs that trigger automated tests upon code commits or pull requests.
- Ensure environment setup scripts are included so tests run consistently across different stages (development, staging).
- Generate reports after each run; tools like Allure can provide visual feedback on test results over time.
- Monitor results continuously; integrate notifications via email or chat systems like Slack for immediate feedback on failures.
This approach fosters rapid development cycles while maintaining high-quality standards through automated validation processes.
20. What challenges have you faced while working with Selenium and how did you overcome them?
Answer:
Common challenges include handling flaky tests due to timing issues or unstable network conditions, managing browser compatibility issues, and dealing with complex user interactions like drag-and-drop or file uploads.
Strategies employed include:
- Implementing robust synchronization techniques (explicit waits).
- Regularly updating browser drivers and ensuring compatibility across versions.
- Utilizing Action classes or JavaScriptExecutor for complex interactions instead of relying solely on standard WebDriver methods.
Sharing experiences about overcoming these challenges demonstrates problem-solving skills essential for automation roles.
21. How do you handle SSL certificate errors in Selenium WebDriver?
Answer:
SSL certificate errors can be bypassed by setting the desired capabilities in Selenium WebDriver. For instance, in Chrome, you can use:
ChromeOptions options = new ChromeOptions();
options.setAcceptInsecureCerts(true);
WebDriver driver = new ChromeDriver(options);
This method is useful when testing applications in staging environments with self-signed certificates.
22. What is Selenium Grid, and how do you set it up?
Answer:
Selenium Grid allows parallel test execution across multiple machines or environments, which improves test efficiency. It uses a Hub-Node architecture, where the Hub manages the tests, and Nodes execute them.
Setup: Install Selenium Server, start it as a Hub, and register multiple nodes specifying browser and OS configurations.
Selenium Grid is ideal for cross-browser and cross-platform testing.
23. Explain how to handle multiple windows or tabs in Selenium WebDriver.
Answer:
To switch between multiple windows or tabs, Selenium WebDriver provides getWindowHandles()
and switchTo().window(windowHandle)
.
String mainWindow = driver.getWindowHandle();
Set<String> allWindows = driver.getWindowHandles();
for (String window : allWindows) {
driver.switchTo().window(window);
if (!window.equals(mainWindow)) {
// Perform actions in the new window
driver.close();
}
}
driver.switchTo().window(mainWindow);
This approach helps in managing scenarios with pop-ups or redirects to new tabs.
24. How can you handle browser cookies in Selenium?
Answer: Selenium WebDriver provides methods to add, delete, and retrieve cookies.
driver.manage().addCookie(new Cookie("sessionKey", "xyz123"));
Cookie cookie = driver.manage().getCookieNamed("sessionKey");
driver.manage().deleteAllCookies();
Managing cookies is useful for session management or preserving login state across tests.
25. What are WebDriverWait and ExpectedConditions, and how are they used in Selenium?
Answer: WebDriverWait
and ExpectedConditions
provide explicit waits, allowing scripts to wait for certain conditions.
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementId")));
Explicit waits prevent tests from failing due to timeouts or dynamic loading delays.
26. How can you scroll a web page using Selenium WebDriver?
Answer: Scrolling can be achieved using JavaScriptExecutor, especially for pages with lazy-loading content.
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("window.scrollBy(0,1000)");
Scrolling helps in dealing with elements that are not initially visible on the screen.
27. How would you handle AJAX calls in Selenium WebDriver?
Answer:
AJAX calls load elements dynamically, so WebDriverWait
with specific ExpectedConditions
like presenceOfElementLocated
or custom waits can be used to handle AJAX requests.
new WebDriverWait(driver, Duration.ofSeconds(10))
.until(ExpectedConditions.visibilityOfElementLocated(By.id("ajaxElementId")));
This ensures that Selenium waits until the AJAX content is fully loaded.
28. Explain the concept of Browser Profiles in Selenium.
Answer:
Browser Profiles allow customizing browser settings (e.g., disabling extensions or setting preferences).
FirefoxProfile profile = new FirefoxProfile();
profile.setPreference("intl.accept_languages", "en-US");
WebDriver driver = new FirefoxDriver(new FirefoxOptions().setProfile(profile));
Profiles are useful when testing specific configurations or user settings.
29. What is the Stale Element Reference Exception, and how do you resolve it?
Answer:
This exception occurs when an element is no longer attached to the DOM. To resolve it, refetch the element after any page update.
WebElement element = driver.findElement(By.id("dynamicElement"));
element.click(); // If page reloads, re-locate the element.
Refetching ensures that WebDriver interacts with the most current element reference.
30. How would you validate the presence of an element without causing test failure?
Answer:
Use a try-catch block or conditional check to verify the presence of an element without failing the test.
boolean isPresent = driver.findElements(By.id("optionalElement")).size() > 0;
if (isPresent) {
System.out.println("Element is present");
} else {
System.out.println("Element is not present");
}
This approach is useful when dealing with optional elements.
31. What is the purpose of the Action
class in Selenium?
Answer:
The Actions
class provides methods to perform complex interactions like double-click, right-click, drag-and-drop, and hovering.
Actions actions = new Actions(driver);
actions.moveToElement(driver.findElement(By.id("hoverElement"))).perform();
Actions
enables simulating user interactions not possible through basic WebDriver commands.
32. How can you manage browser notifications in Selenium WebDriver?
Answer: Browser notifications can be handled by disabling them in browser capabilities. For Chrome:
ChromeOptions options = new ChromeOptions();
options.addArguments("--disable-notifications");
WebDriver driver = new ChromeDriver(options);
Disabling notifications prevents them from interrupting test executions.
33. Explain how to manage iframes in Selenium.
Answer: Selenium provides methods to switch to iframes using switchTo().frame()
with id, name, or index.
driver.switchTo().frame("frameName");
driver.switchTo().defaultContent(); // Switch back to the main document
This is essential for interacting with elements within embedded frames.
34. How would you manage pop-ups in Selenium WebDriver?
Answer: Alert pop-ups can be managed using the Alert
interface.
Alert alert = driver.switchTo().alert();
alert.accept();
This interface handles JavaScript alerts, confirmations, and prompts.
35. How do you handle mouse hover actions in Selenium?
Answer: The Actions
class is used for hover actions.
Actions actions = new Actions(driver);
actions.moveToElement(driver.findElement(By.id("hoverElement"))).perform();
Hover actions are commonly used to reveal hidden menus or tooltips.
36. What strategies do you use for debugging Selenium tests?
Answer: Common strategies include:
- Using breakpoints in IDEs.
- Adding logging statements.
- Taking screenshots on failure.
- Using try-catch blocks to catch and log exceptions.
These practices make debugging and troubleshooting tests easier.
37. How would you handle CAPTCHA in Selenium?
Answer: CAPTCHAs are designed to block automated scripts. Techniques to handle them include:
- Disabling CAPTCHA in test environments.
- Using third-party CAPTCHA-solving services.
- Requesting developer assistance to use test-friendly CAPTCHAs.
While Selenium doesn’t directly solve CAPTCHAs, these workarounds help maintain test continuity.
38. What is a headless browser, and how do you use it in Selenium?
Answer:
A headless browser runs tests without a graphical interface, speeding up execution. For instance, Chrome can run headless by setting the --headless
argument.
ChromeOptions options = new ChromeOptions();
options.addArguments("--headless");
WebDriver driver = new ChromeDriver(options);
Headless browsers are ideal for CI/CD pipelines and environments without display requirements.
39. How would you verify text color or other CSS properties in Selenium?
Answer: Selenium can retrieve CSS properties using the getCssValue
method.
String color = driver.findElement(By.id("textElement")).getCssValue("color");
System.out.println("Text color: " + color);
This approach is valuable for UI validation checks in tests.
40. How do you handle file downloads in Selenium WebDriver?
Answer:
File downloads can be managed by setting custom download paths using browser capabilities. For example, in Chrome:
HashMap<String, Object> chromePrefs = new HashMap<String, Object>();
chromePrefs.put("download.default_directory", "/path/to/download/folder");
ChromeOptions options = new ChromeOptions();
options.setExperimentalOption("prefs", chromePrefs);
WebDriver driver = new ChromeDriver(options);
Setting download directories ensures tests have access to downloaded files for validation purposes.
Learn More: Carrer Guidance
TypeScript Interview Questions and Answers
Operating System Interview Questions and Answers
Power BI Interview Questions with Detailed Answers
Java Microservices Interview Questions with Detailed Answers
OOP Interview Questions with Detailed Answers
React JS Interview Questions with Detailed Answers for Freshers