Are you preparing for a Redux interview? Redux, a powerful state management library, has become a cornerstone for developers working with React and other JavaScript frameworks. To help you out, we have compiled the most commonly asked and top 40 Redux Interview Questions and Answers. This guide covers the core principles of Redux, including its architecture, actions, reducers, and middleware.
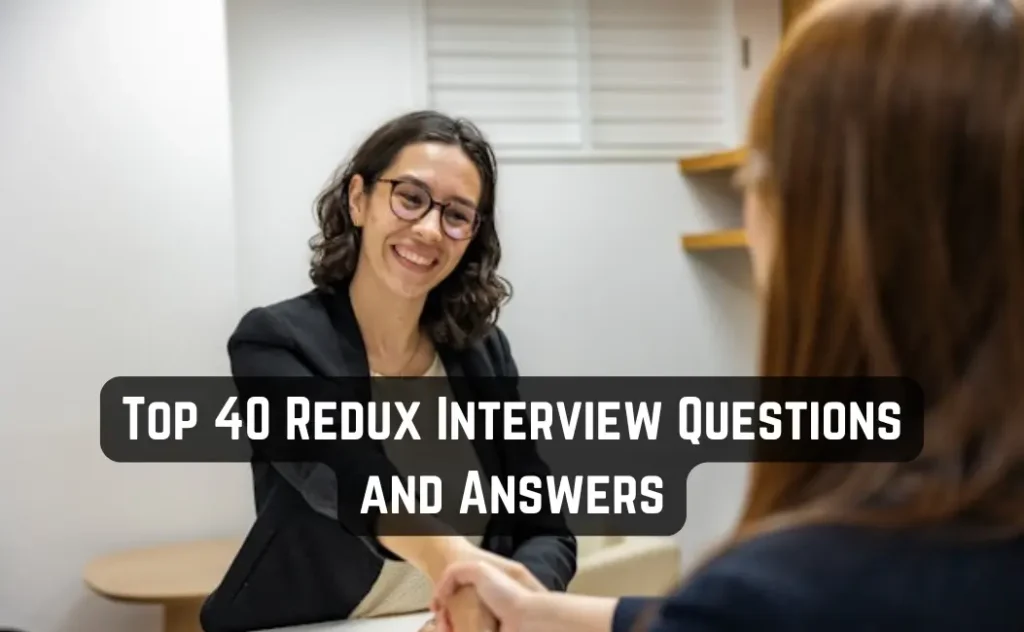
Top 40 Redux Interview Questions and Answers
- What is Redux, and why is it used in web development?
- What are the three core principles of Redux?
- What is an action in Redux?
- How do actions change the state in a Redux application?
- What is a reducer in Redux, and what role does it play?
- How does Redux differ from local component state in React?
- What is the purpose of the Redux store?
- How do you handle asynchronous actions in Redux?
- What is middleware in Redux, and how does it work?
- What are the advantages of using Redux?
- What are the limitations or downsides of using Redux?
- How does Redux ensure immutability, and why is it important?
- What is the role of the connect function in React-Redux?
- How can you optimize performance in a Redux application?
- What are selectors in Redux, and how do they work?
- How does Redux handle side effects, and what are common middleware solutions?
- What is the difference between Redux and the Context API in React?
- How do you integrate Redux with a React application?
- What are Redux DevTools, and how do they assist in debugging?
- How does Redux Toolkit simplify Redux development?
- How does Redux handle form state management?
- What is the purpose of the combineReducers function in Redux?
- How do you implement code splitting in a Redux application?
- What are higher-order reducers, and how are they used in Redux?
- How can you persist Redux state across page reloads?
- What is the role of selectors in Redux, and how do they improve performance?
- How do you handle errors in Redux applications?
- What is the difference between Redux Thunk and Redux Saga?
- How do you test Redux reducers and actions?
- How does Redux integrate with server-side rendering (SSR)?
- What are the best practices for structuring a Redux application?
- How do you handle authentication in a Redux application?
- What is the significance of immutability in Redux, and how is it maintained?
- How do you handle nested state in Redux?
- What are some common pitfalls when using Redux, and how can they be avoided?
- How does Redux handle optimistic updates?
- What is the purpose of the applyMiddleware function in Redux?
- How do you handle undo and redo functionality in a Redux application?
- How do you handle pagination in a Redux application?
- How can you implement internationalization (i18n) in a Redux application?
1. What is Redux, and why is it used in web development?
Redux is an open-source JavaScript library designed for managing and centralizing application state. It is commonly used with libraries like React or Angular to build user interfaces. Redux provides a predictable state container, ensuring that applications behave consistently across different environments. By centralizing the application’s state in a single store, Redux simplifies state management, making it easier to debug and test applications.
2. What are the three core principles of Redux?
The three core principles of Redux are:
- Single Source of Truth: The entire state of the application is stored in a single object within the Redux store, providing a centralized view of the state.
- State is Read-Only: The state cannot be modified directly. To change the state, an action must be dispatched, ensuring a clear and predictable state transition.
- Changes are Made with Pure Functions: Reducers, which are pure functions, specify how the state changes in response to actions. They take the current state and an action as inputs and return a new state, ensuring predictability and ease of testing.
3. What is an action in Redux?
An action in Redux is a plain JavaScript object that describes an event or change that should occur in the application state. Every action must have a type
property that indicates the type of action being performed. Actions may also contain a payload
property with additional data needed to update the state. For example:
const addTodoAction = {
type: 'ADD_TODO',
payload: {
id: 1,
text: 'Buy groceries'
}
};
4. How do actions change the state in a Redux application?
In Redux, actions are dispatched to the store using the dispatch()
method. Reducers listen for these dispatched actions and, based on the action type, determine how to update the state. Reducers are pure functions that take the current state and an action as arguments and return a new state. This unidirectional data flow ensures that state changes are predictable and traceable.
5. What is a reducer in Redux, and what role does it play?
A reducer in Redux is a pure function that specifies how the application’s state changes in response to an action. It takes the current state and an action as inputs and returns a new state. Reducers must be pure functions, meaning they do not modify the existing state but return a new state object. They are responsible for handling specific slices of the application state and are combined to form the overall state.
6. How does Redux differ from local component state in React?
In React, local component state is managed within individual components using the useState
or this.setState
methods. This approach is suitable for simple applications with minimal state management needs. However, as applications grow in complexity, managing state across multiple components becomes challenging. Redux addresses this by providing a centralized store for the entire application state, allowing for consistent and predictable state management across all components. This centralization simplifies debugging and testing and facilitates data sharing between components.
7. What is the purpose of the Redux store?
The Redux store is a centralized object that holds the entire state of the application. It provides methods to access the current state (getState()
), dispatch actions (dispatch(action)
), and subscribe to state changes (subscribe(listener)
). The store serves as the single source of truth for the application state, ensuring consistency and predictability.
8. How do you handle asynchronous actions in Redux?
Redux by default handles synchronous actions. To manage asynchronous actions, middleware like Redux Thunk or Redux Saga is used. Redux Thunk allows action creators to return a function instead of an action object. This function can perform asynchronous operations and dispatch actions based on the results. For example:
function fetchData() {
return (dispatch) => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => dispatch({ type: 'FETCH_DATA_SUCCESS', payload: data }))
.catch(error => dispatch({ type: 'FETCH_DATA_ERROR', error }));
};
}
9. What is middleware in Redux, and how does it work?
Middleware in Redux provides a way to extend the store’s capabilities by intercepting actions before they reach the reducers. Middleware can perform tasks such as logging, crash reporting, or handling asynchronous actions. It is applied using the applyMiddleware()
function when creating the Redux store. Middleware functions receive the dispatch
and getState
methods as arguments and return a function that takes the next middleware in the chain. This allows for composing multiple middleware functions to handle various tasks.
10. What are the advantages of using Redux?
Some advantages of using Redux include:
- Predictable State Management: The state is centralized and immutable, making it easier to understand and debug.
- Centralized State: All application state is stored in a single store, simplifying data management and sharing between components.
- Ease of Testing: Pure functions (reducers) and predictable state transitions make testing straightforward.
- Time-Travel Debugging: Tools like Redux DevTools allow developers to inspect every state and action, enabling features like time-travel debugging.
- Middleware Support: Redux’s middleware architecture allows for the extension of its capabilities, enabling tasks such as logging, crash reporting, and handling asynchronous operations.
- Community and Ecosystem: A robust ecosystem of tools, extensions, and a supportive community enhances development efficiency and provides ample resources for problem-solving.
These advantages contribute to Redux’s effectiveness in managing complex state in large-scale applications.
11. What are the limitations or downsides of using Redux?
While Redux offers numerous benefits, it also has certain limitations:
- Boilerplate Code: Implementing Redux can introduce additional boilerplate, including actions, action creators, and reducers, which may increase the complexity of the codebase.
- Learning Curve: Understanding Redux’s concepts, such as the unidirectional data flow and immutability, can be challenging for beginners.
- Overhead for Simple Applications: For smaller applications with minimal state management needs, integrating Redux might be unnecessary and could add unwarranted complexity.
- Performance Considerations: Improper use of Redux, such as unnecessary re-renders due to state changes, can lead to performance issues if not managed correctly.
It’s essential to assess whether Redux is appropriate for a project based on its specific requirements and complexity.
12. How does Redux ensure immutability, and why is it important?
Redux enforces immutability by requiring that reducers return new state objects rather than modifying the existing state directly. This is typically achieved using techniques like the spread operator or utility functions that create copies of objects or arrays. Immutability is crucial because it ensures that state changes are predictable and traceable, facilitating debugging and enabling features like time-travel debugging. It also prevents unintended side effects, as components cannot alter the state directly.
13. What is the role of the connect
function in React-Redux?
In React-Redux, the connect
function is a higher-order function that connects React components to the Redux store. It allows components to access the state and dispatch actions without directly interacting with the store. The connect
function takes two optional arguments:
mapStateToProps
: A function that selects the parts of the state that the component needs and maps them to the component’s props.mapDispatchToProps
: A function that creates callback props to dispatch actions to the store.
By using connect
, components become “smart” or “container” components, aware of the Redux state and capable of dispatching actions.
14. How can you optimize performance in a Redux application?
To optimize performance in a Redux application, consider the following strategies:
- Use
shouldComponentUpdate
orReact.memo
: Prevent unnecessary re-renders by implementingshouldComponentUpdate
in class components or wrapping functional components withReact.memo
. - Reselect Library: Utilize memoized selectors with the Reselect library to prevent expensive computations on each render.
- Normalize State Shape: Structure the state to minimize nested data, making it easier to update and compare.
- Batch Actions: Dispatch multiple actions together to reduce the number of renders.
- Avoid Inline Functions and Objects: Define functions and objects outside of render methods to prevent re-creation on each render, which can cause unnecessary re-renders of child components.
Implementing these practices helps maintain a responsive and efficient Redux application.
15. What are selectors in Redux, and how do they work?
Selectors are functions that extract specific pieces of data from the Redux store state. They encapsulate the logic for retrieving data, promoting reusability and maintainability. Selectors can be simple functions or more complex ones that compute derived data. Using libraries like Reselect, selectors can be memoized to prevent unnecessary recalculations, enhancing performance.
16. How does Redux handle side effects, and what are common middleware solutions?
Redux handles side effects—operations like data fetching or impure computations—using middleware. Common middleware solutions include:
- Redux Thunk: Allows action creators to return functions (thunks) instead of plain objects, enabling delayed dispatching of actions and access to the store’s state.
- Redux Saga: Utilizes generator functions to create sagas that can handle complex asynchronous workflows, offering more control over side effects.
- Redux Observable: Leverages RxJS to manage side effects as reactive streams, providing powerful capabilities for handling asynchronous operations.
These middleware solutions enable Redux applications to manage side effects in a structured and maintainable manner.
17. What is the difference between Redux and the Context API in React?
Both Redux and React’s Context API are used for state management, but they differ in scope and capabilities:
- Redux: A standalone library that provides a predictable state container with features like middleware support, time-travel debugging, and a unidirectional data flow. It’s suitable for complex state management across large applications.
- Context API: A React feature that allows for passing data through the component tree without prop drilling. It’s ideal for simpler state management needs but lacks advanced features like middleware and time-travel debugging.
Choosing between them depends on the application’s complexity and specific requirements.
18. How do you integrate Redux with a React application?
To integrate Redux with a React application:
- Install Redux and React-Redux: Use npm or yarn to install both libraries.
- Create a Redux Store: Define the store using
createStore
and apply any necessary middleware. - Provide the Store to React: Wrap the root component with the
Provider
component from React-Redux, passing the store as a prop. - Connect Components: Use the
connect
function or theuseSelector
anduseDispatch
hooks to access state and dispatch actions within components.
This setup enables React components to interact seamlessly with the Redux store.
19. What are Redux DevTools, and how do they assist in debugging?
Redux DevTools is a powerful toolset that enhances the development and debugging experience for Redux applications. They provide a visual interface to monitor dispatched actions and state changes, enabling developers to:
- Inspect Actions and State: View the details of each dispatched action and the resulting state, facilitating a clear understanding of how actions affect the application.
- Time-Travel Debugging: Navigate through the action history to observe previous states, allowing developers to “travel back in time” to debug issues effectively.
- State Persistence: Persist the state across page reloads, which is particularly useful during development to maintain the application’s state without reinitializing it.
- Error Tracking: Identify errors by examining the state and actions leading up to them, aiding in pinpointing the root cause of issues.
To integrate Redux DevTools into a project, developers can install the browser extension available for Chrome, Firefox, and Edge. Additionally, when configuring the Redux store, it’s essential to enable the DevTools extension. This can be achieved using the composeWithDevTools
function from the redux-devtools-extension
package:
import { createStore } from 'redux';
import { composeWithDevTools } from 'redux-devtools-extension';
const store = createStore(
rootReducer,
composeWithDevTools(
// other store enhancers if any
)
);
By incorporating Redux DevTools, developers gain valuable insights into the application’s behavior, making the debugging process more efficient and effective.
20. How does Redux Toolkit simplify Redux development?
Redux Toolkit is the official, opinionated, batteries-included toolset for efficient Redux development. It addresses common concerns about Redux, such as boilerplate code and complex store setup, by providing utilities that simplify standard tasks:
configureStore
: Simplifies store creation with good default settings, including combining reducers, adding middleware, and enabling Redux DevTools.createSlice
: Automatically generates action creators and action types corresponding to the reducers and state you define, reducing boilerplate.createAsyncThunk
: Facilitates writing standard Redux logic for fetching data and handling loading states, streamlining asynchronous actions.createEntityAdapter
: Provides a set of functions to manage normalized data in the store, simplifying the handling of collections of items.
By utilizing Redux Toolkit, developers can write Redux logic more concisely and effectively, adhering to best practices and reducing the likelihood of common errors.
21. How does Redux handle form state management?
Redux can manage form state by storing form data within the Redux store. This approach centralizes form state, allowing for consistent handling across the application. Libraries like Redux Form or React Final Form integrate seamlessly with Redux, providing utilities to manage form state, validation, and submission. However, for simple forms, using React’s local state might be more efficient, as managing form state in Redux can introduce unnecessary complexity.
22. What is the purpose of the combineReducers
function in Redux?
The combineReducers
function is a utility that merges multiple reducer functions into a single reducing function. Each reducer manages its own slice of the application state. By combining them, combineReducers
produces a single root reducer that can be passed to the Redux store. This modular approach enhances code maintainability and scalability.
23. How do you implement code splitting in a Redux application?
Code splitting in a Redux application involves loading parts of the application asynchronously to improve performance. This can be achieved using dynamic import()
statements and React’s Suspense
component. For Redux, it’s essential to ensure that the reducers for the dynamically loaded components are available when needed. Libraries like redux-dynamic-modules
facilitate adding reducers dynamically, enabling effective code splitting.
24. What are higher-order reducers, and how are they used in Redux?
Higher-order reducers are functions that take a reducer as an argument and return a new reducer with enhanced behavior. They allow for reusing reducer logic across different parts of the state. For example, a higher-order reducer can add logging functionality or handle specific actions across multiple reducers, promoting code reuse and separation of concerns.
25. How can you persist Redux state across page reloads?
To persist Redux state across page reloads, you can use middleware like redux-persist
. This library automatically saves the Redux state to storage (e.g., localStorage) and rehydrates it upon app initialization. By configuring redux-persist
, you can specify which parts of the state to persist and ensure data consistency across sessions.
26. What is the role of selectors in Redux, and how do they improve performance?
Selectors are functions that extract specific pieces of data from the Redux state. They encapsulate the logic for retrieving data, promoting reusability and maintainability. Memoized selectors, created using libraries like Reselect, cache the computed output, preventing unnecessary recalculations and improving performance, especially for derived data.
27. How do you handle errors in Redux applications?
Error handling in Redux can be managed by:
- Actions: Dispatching actions that represent error states.
- Reducers: Updating the state to reflect error information.
- Middleware: Intercepting actions to handle errors globally, such as logging or displaying notifications.
By structuring error handling through Redux’s flow, you maintain a predictable state and centralized error management.
28. What is the difference between Redux Thunk and Redux Saga?
Both Redux Thunk and Redux Saga are middleware for handling asynchronous actions in Redux:
- Redux Thunk: Allows action creators to return functions (thunks) instead of plain objects. These functions can perform asynchronous operations and dispatch actions. It’s simple and suitable for basic async scenarios.
- Redux Saga: Uses generator functions to create sagas that handle complex asynchronous workflows. It provides more control over side effects and is suitable for complex scenarios like orchestrating multiple async operations.
Choosing between them depends on the application’s complexity and specific requirements.
29. How do you test Redux reducers and actions?
Testing Redux reducers and actions involves:
- Reducers: Since reducers are pure functions, you can test them by providing an initial state and an action, then asserting that the returned state matches the expected state.
- Actions: Testing action creators involves ensuring they return the correct action objects. For asynchronous actions, you can use middleware like Redux Thunk and test the sequence of dispatched actions using tools like
redux-mock-store
.
By isolating and testing these components, you ensure the reliability of the state management logic.
30. How does Redux integrate with server-side rendering (SSR)?
In server-side rendering, Redux can be integrated by:
- Creating a Store on the Server: Initialize the Redux store on the server with any necessary initial state.
- Rendering the App: Render the React application to a string using the server-side store.
- Hydrating the State: Pass the initial state to the client, which uses it to create the client-side Redux store, ensuring consistency between server and client.
This approach enables the application to render on the server and hydrate on the client with the same state.
31. What are the best practices for structuring a Redux application?
Best practices for structuring a Redux application include:
- Modular Structure: Organize code by feature or domain, grouping related actions, reducers, and components.
- Consistent Naming: Use clear and consistent naming conventions for actions and action types.
- Immutable Updates: Ensure state updates are immutable, using tools like the spread operator or libraries like Immer.
- Selective State Storage: Only store necessary state in Redux; keep local UI state within components when appropriate.
Following these practices enhances maintainability and scalability.
32. How do you handle authentication in a Redux application?
Handling authentication in Redux involves:
- Storing Tokens: Saving authentication tokens in the Redux state or more securely in cookies or localStorage.
- Managing User State: Using reducers to update the state based on authentication actions (e.g., login, logout).
- Protecting Routes: Implement higher-order components (HOCs) or utilize React Router’s
<Route>
components to create protected routes that render components conditionally based on the user’s authentication status. This ensures that only authenticated users can access certain parts of the application. - Refreshing Tokens: Implement mechanisms to handle token expiration and refreshing. This can involve setting up interceptors with libraries like Axios to automatically refresh tokens when they expire, ensuring a seamless user experience without requiring frequent logins.
By integrating these strategies, Redux can effectively manage authentication, providing a secure and consistent user experience across the application.
33. What is the significance of immutability in Redux, and how is it maintained?
Immutability in Redux ensures that the state is not directly modified but instead produces new copies of the state with the necessary changes. This approach is significant because:
- Predictability: Immutable state ensures that state transitions are predictable, as previous states remain unchanged, allowing for reliable debugging and testing.
- Change Detection: Immutability facilitates efficient change detection, as comparing references of objects can quickly determine if a state has changed, optimizing rendering performance.
- Time-Travel Debugging: Tools like Redux DevTools leverage immutability to enable features like time-travel debugging, allowing developers to traverse through previous states.
To maintain immutability:
- Spread Operator: Use the spread operator (
...
) to create shallow copies of objects or arrays when updating the state. - Immutable Libraries: Utilize libraries like Immer or Immutable.js to handle complex state updates, ensuring immutability is preserved.
- Pure Functions: Write reducers as pure functions that return new state objects without mutating the existing state.
Adhering to immutability principles in Redux leads to more maintainable and robust applications.
34. How do you handle nested state in Redux?
Managing nested state in Redux requires careful handling to maintain immutability and ensure efficient updates:
- Shallow Copies: When updating nested state, create shallow copies of each level of the state that is being modified. This can be achieved using the spread operator
const newState = {
...state,
nestedLevel1: {
...state.nestedLevel1,
nestedLevel2: {
...state.nestedLevel2,
// updated properties
}
}
};
- Utility Libraries: Employ libraries like Immer, which allow you to write code that appears to mutate the state directly but under the hood ensures immutability by producing new state copies.
- Normalization: Normalize the state structure to flatten nested data. This involves storing entities in a normalized form, such as using an object with IDs as keys, which simplifies updates and access. Libraries like normalizr can assist in this process.
By adopting these practices, you can effectively manage nested state in Redux, ensuring updates are predictable and maintainable.
35. What are some common pitfalls when using Redux, and how can they be avoided?
Common pitfalls when using Redux include:
- Overusing Redux: Storing every piece of state in Redux, including local UI state, can lead to unnecessary complexity. To avoid this, determine whether the state truly needs to be global or if it can reside locally within components.
- Mutating State: Directly mutating the state in reducers breaks immutability, leading to unpredictable behavior. Always return new state objects in reducers to maintain immutability.
- Improper Middleware Usage: Misconfiguring middleware or using it inappropriately can cause unexpected side effects. Ensure middleware is set up correctly and used for its intended purpose, such as handling asynchronous actions.
- Neglecting Performance Optimization: Failing to optimize component re-renders can degrade performance. Use memoization techniques, such as
React.memo
for functional components orshouldComponentUpdate
for class components, to prevent unnecessary re-renders. - Complex State Shape: Having deeply nested state structures can make updates cumbersome and error-prone. Normalize the state to flatten nested data, simplifying state management.
By being aware of these pitfalls and implementing best practices, you can develop more efficient and maintainable Redux applications.
36. How does Redux handle optimistic updates?
Optimistic updates in Redux involve updating the UI before receiving confirmation from the server, providing a more responsive user experience. To implement optimistic updates:
- Dispatch Optimistic Action: Immediately dispatch an action that updates the state as if the operation succeeded.
- Perform Async Operation: Proceed with the asynchronous operation (e.g., API call).
- Handle Response: If the operation succeeds, no further action is needed. If it fails, dispatch an action to revert the state to its previous form or handle the error appropriately.
This approach ensures the UI remains responsive, but it’s crucial to handle potential errors gracefully to maintain data consistency.
37. What is the purpose of the applyMiddleware
function in Redux?
The applyMiddleware
function in Redux is used to enhance the store’s dispatch function by allowing middleware to intercept and act upon actions before they reach the reducers. Middleware can perform tasks such as logging, handling asynchronous actions, or modifying actions. To apply middleware:
import { createStore, applyMiddleware } from 'redux';
import thunk from 'redux-thunk';
const store = createStore(
rootReducer,
applyMiddleware(thunk)
);
In this example, redux-thunk
middleware is applied to handle asynchronous actions.
38. How do you handle undo and redo functionality in a Redux application?
To implement undo and redo functionality in a Redux application, you can utilize the redux-undo
library, which simplifies the process by providing higher-order reducers that manage state history. Here’s how to integrate it:
- Install
redux-undo
:
npm install redux-undo
- Wrap Your Reducer with
undoable
:
Import the undoable
function from redux-undo
and wrap your existing reducer:
import { combineReducers } from 'redux';
import undoable from 'redux-undo';
const rootReducer = combineReducers({
todos: undoable(todosReducer),
// other reducers
});
This enhances your reducer to handle undo and redo actions.
- Accessing the Present State:
After wrapping your reducer, the state structure includes past
, present
, and future
properties. Access the current state using state.present
const mapStateToProps = (state) => ({
todos: state.todos.present,
});
- Dispatch Undo and Redo Actions:
Use the action creators provided by redux-undo
to dispatch undo and redo actions:
import { ActionCreators } from 'redux-undo';
// To undo the last action
dispatch(ActionCreators.undo());
// To redo the last undone action
dispatch(ActionCreators.redo());
- Configure Undoable Reducer:
redux-undo
allows configuration to customize its behavior, such as setting a history limit or filtering actions:
const undoableTodos = undoable(todosReducer, {
limit: 10, // History limit
filter: includeAction(['ADD_TODO', 'REMOVE_TODO']), // Only include specific actions
});
By integrating redux-undo
, you can efficiently implement undo and redo functionality, enhancing user experience by allowing users to revert and reapply actions as needed.
39. How do you handle pagination in a Redux application?
Managing pagination in a Redux application involves:
- State Management: Storing pagination-related data, such as the current page number, total pages, and items per page, in the Redux store.
- Actions and Reducers: Defining actions to update pagination state (e.g.,
SET_CURRENT_PAGE
) and corresponding reducers to handle these actions. - Data Fetching: Fetching data based on the current page and items per page, often involving asynchronous actions handled by middleware like Redux Thunk.
- UI Components: Creating components that dispatch pagination actions and display paginated data, ensuring they reflect the current pagination state.
This approach centralizes pagination logic, making it easier to manage and maintain across the application.
40. How can you implement internationalization (i18n) in a Redux application?
Implementing internationalization in a Redux application involves:
- Language State: Storing the current language selection in the Redux store to ensure consistency across the application.
- Actions and Reducers: Defining actions to change the language and reducers to update the state accordingly.
- Translation Files: Maintaining separate translation files for each supported language, containing key-value pairs for translatable text.
- Integration with i18n Libraries: Utilizing libraries like
react-i18next
to handle translation logic, integrating them with Redux to synchronize language state.
By managing language selection through Redux, you ensure that the application’s language setting is consistent and easily accessible across all components.
Preparation Tips
All the above interview questions cover a comprehensive range of Redux topics, from basic to advanced levels. It is beneficial to focus on practical application and problem-solving skills to excel in interviews. Here are some additional strategies to enhance your preparation:
1. Practical Implementation:
- Build Projects: Develop small to medium-sized projects utilizing Redux to solidify your understanding and demonstrate your ability to apply concepts in real-world scenarios.
- Code Reviews: Engage in code reviews to learn best practices and identify common pitfalls in Redux implementations.
2. Performance Optimization:
- Profiling: Learn to profile Redux applications to identify and address performance bottlenecks.
- Memoization Techniques: Understand and implement memoization strategies to prevent unnecessary re-renders and computations.
3. Advanced Middleware:
- Custom Middleware: Gain experience in writing custom middleware to handle specific application needs beyond standard solutions.
- Middleware Integration: Explore integrating multiple middleware in complex applications and managing their interactions.
4. Testing Strategies:
- Comprehensive Testing: Develop a robust testing strategy that includes unit tests for reducers and actions, as well as integration tests for components connected to the Redux store.
- Mocking State: Practice mocking Redux state in tests to isolate components and test them effectively.
5. Integration with Other Technologies:
- GraphQL and Redux: Explore how Redux can be integrated with GraphQL for state management in data-driven applications.
- TypeScript with Redux: Learn to implement Redux in TypeScript projects, focusing on typing actions, reducers, and the store.
6. Community Engagement:
- Open Source Contribution: Contribute to Redux-related open-source projects to gain deeper insights and practical experience.
- Discussion Forums: Participate in forums and discussion groups to stay updated on best practices and emerging patterns in Redux development.
By focusing on these areas, you can enhance your practical skills and problem-solving abilities, which are crucial for performing well in technical interviews involving Redux.
Learn More: Carrer Guidance | Hiring Now!
Top 40+ Jira Interview Questions and Answers- Basic to Advanced
PostgreSQL interview Questions and Answers- Basic to Advanced
Palo Alto Interview Questions and Answers- Basic to Advanced
Deep Learning Interview Questions and Answers- Basic to Advanced
Data Modelling Interview Questions and Answers- Basic to Advanced
Tosca Interview Questions for Freshers with detailed Answers
Ansible Interview Questions and Answers- Basic to Advanced
Scrum Master Interview Questions and Answers- Basic to Advanced