Python is known for its simplicity and flexibility, but developers coming from other languages like C# or Java may occasionally encounter confusing errors—especially when integrating Python with .NET environments or certain libraries. One such issue is the 'Type or Namespace Not Found'
error, which typically stems from interop or incorrect module usage.
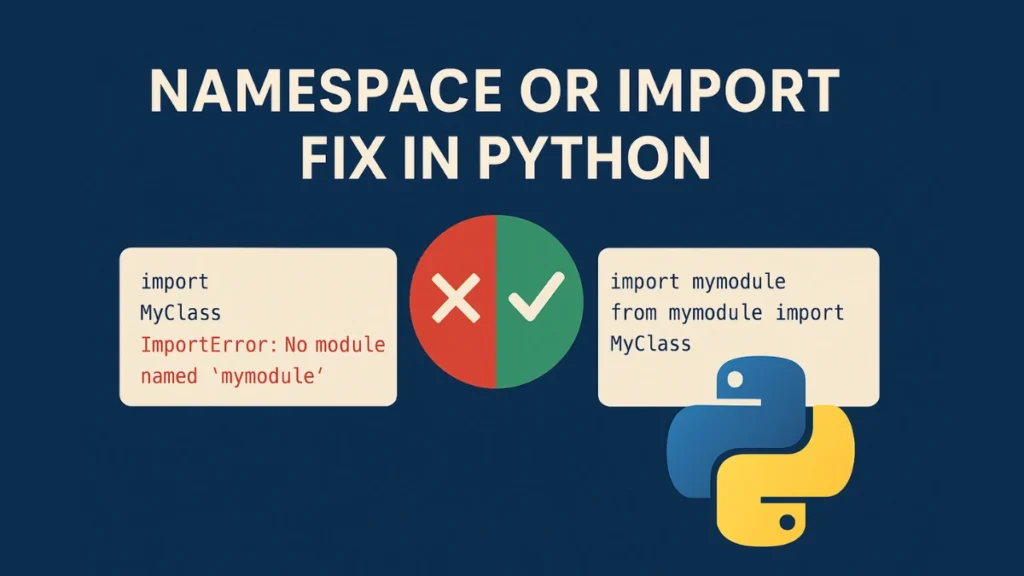
In this guide, we’ll break down what causes this error, when it’s most likely to occur in Python environments, and how to fix it effectively.
Python Import System
Python’s import system is fundamentally different from C#’s namespace resolution but shares conceptual similarities. When Python can’t find a name you’re trying to use, it raises either:
ModuleNotFoundError
(for missing modules)ImportError
(for failed imports)NameError
(for undefined names)AttributeError
(for missing attributes in found objects)
Common Causes and Solutions
1. Missing Package Installation (Equivalent to Missing Assembly Reference)
Symptoms:
ModuleNotFoundError: No module named 'requests'
Solution:
pip install requests # For the specific missing package
pip install -r requirements.txt # For project-wide dependencies
Advanced Tip: Use virtual environments to manage dependencies:
python -m venv myenv
source myenv/bin/activate # Linux/Mac
myenv\Scripts\activate # Windows
pip install package-name
2. Incorrect Import Paths (Similar to Using Directive Issues)
Common Mistakes:
# File structure:
# project/
# utils/
# helpers.py
# main.py
# Wrong:
from utils.helper import func # Typo in filename
from util.helpers import func # Wrong directory name
Solutions:
# Correct:
from utils.helpers import func # Exact filename
Pro Tip: Use absolute imports with your package name:
from myproject.utils.helpers import func
3. Python Environment Mismatch (Analogous to Target Framework Issues)
Diagnosis:
which python # Shows current Python interpreter
pip list # Shows installed packages for current environment
Solutions:
- Activate the correct virtual environment
- Ensure consistent Python versions across development and deployment
- Use
pyenv
to manage multiple Python versions
4. Dependency Version Conflicts (Like NuGet Package Issues)
Solution:
pip freeze > requirements.txt # Create exact version requirements
pip install -U package-name # Upgrade specific package
pip check # Verify dependency conflicts
Advanced: Use poetry
for better dependency management:
poetry add package-name
poetry install
5. Case Sensitivity and Typos (Same as C# Issues)
Python is case-sensitive:
import numpy # Correct
import NumPy # Will fail
Solution: Always verify:
- Exact module name casing
- Correct spelling in imports
- Proper file naming (especially on case-sensitive filesystems)
6. Project Structure Problems (Similar to Project Configuration Issues)
Proper structure example:
myproject/
├── __init__.py
├── main.py
└── utils/
├── __init__.py
└── helpers.py
Critical Files:
__init__.py
makes directories importable packagessetup.py
orpyproject.toml
for installable projects
7. Cache Issues (Like Visual Studio Cache Problems)
Solutions:
- Delete
__pycache__
directories:
find . -name "__pycache__" -exec rm -r {} +
- Clear Python bytecode:
python -B # Run with -B flag to prevent bytecode creation
8. Name Conflicts (Similar to Namespace Ambiguities)
Resolution:
# Instead of:
from package1 import utils
from package2 import utils # Overwrites previous import
# Use either:
import package1.utils as utils1
import package2.utils as utils2
# Or:
from package1.utils import specific_function
Advanced Troubleshooting Techniques
1. Debugging Import Paths
import sys
print(sys.path) # Shows where Python looks for modules
# To add custom paths temporarily:
sys.path.append('/path/to/your/module')
2. Using try-except
for Graceful Handling
try:
import optional_package
except ImportError:
optional_package = None
print("Optional feature disabled")
3. Dynamic Imports
module = __import__('module_name') # Equivalent to import module_name
4. Package Development Mode
pip install -e . # Install in editable mode during development
Special Cases
1. Relative Imports
# In myproject/utils/helpers.py:
from ..config import settings # Goes up one package level
Requirements:
- Must be part of an installable package
- Cannot be used in
__main__
module
2. Circular Imports
Solution: Restructure code or use local imports:
def function():
from module import name # Import only when needed
3. Namespace Packages
For splitting packages across multiple directories:
# __init__.py must be empty (Python 3.3+)
IDE-Specific Solutions
VS Code:
- Select correct Python interpreter (Ctrl+Shift+P > “Python: Select Interpreter”)
- Install recommended extensions (Python, Pylance)
PyCharm:
- Mark directories as “Sources Root” (right-click folder)
- Invalidate caches (File > Invalidate Caches)
Conclusion
Python’s “type or namespace not found” errors stem from similar root causes as their C# counterparts but require different solutions due to Python’s dynamic nature and import system. By methodically checking:
- Package installation
- Import paths
- Environment configuration
- Project structure
- Naming conventions
You can resolve nearly all such issues. Remember that Python’s ecosystem tools (pip, virtualenv, poetry) are your allies in preventing these problems before they occur.
For persistent issues, always:
- Check Python version compatibility
- Verify file permissions
- Consult package documentation
- Search for similar issues in project repositories
With these comprehensive strategies, you’ll be equipped to handle any Python import or namespace resolution error efficiently.