Developers commonly encounter the “npm ERR could not determine executable to run” error when working with Node.js and npm. npm fails to locate the executable file specified in your project’s scripts or commands, causing this error. In this article, we’ll explore the most common causes of this error and provide practical solutions to resolve it.
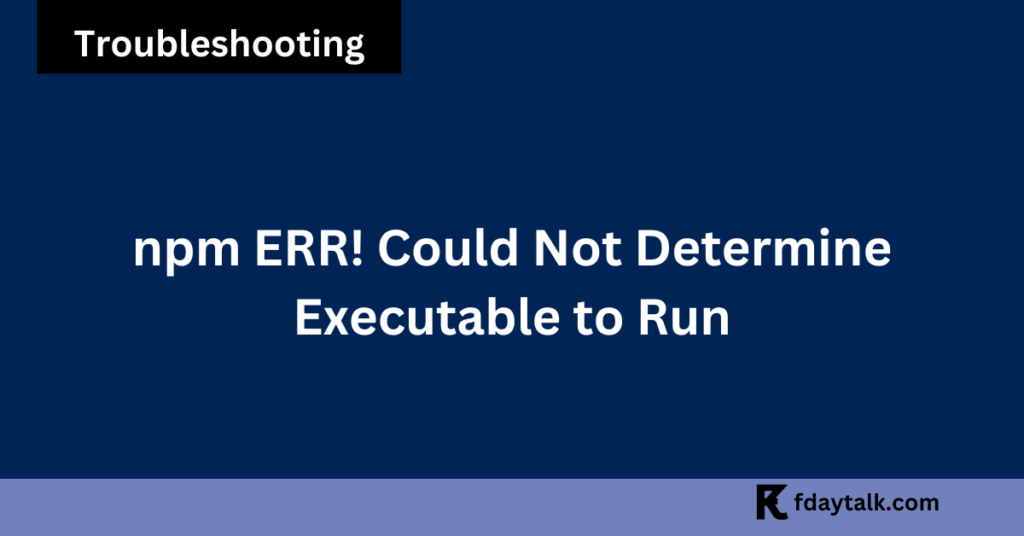
Common Causes of the Error
- Missing Dependencies:
- A dependency referenced in your scripts might not be installed.
- Incorrect Script Definitions:
- The command in the
scripts
section of yourpackage.json
may be incorrect or incomplete.
- The command in the
- PATH Environment Issues:
- Your system’s PATH might not include the directory containing npm executables (e.g.,
node_modules/.bin
).
- Your system’s PATH might not include the directory containing npm executables (e.g.,
- Git Hooks or Husky Conflicts:
- Tools like Husky, which manage Git hooks, can sometimes cause this error if improperly configured.
- Corrupted npm Installation:
- An outdated or corrupted npm installation can also result in this error.
How to Fix the “npm ERR! Could Not Determine Executable to Run” Error
1. Verify Script Definitions in package.json
Ensure that all scripts in your package.json
file are correctly defined and reference valid executables. For example:
{
"scripts": {
"start": "webpack serve"
}
}
If the webpack
command is not found, it means the package is missing or not properly installed.
2. Install Missing Dependencies
If an executable referenced in your scripts is missing, install the required package. For example, if webpack
is missing:
npm install webpack --save-dev
Always use --save-dev
for development dependencies.
3. Check Your PATH Environment Variable
Ensure that the node_modules/.bin
directory is included in your system’s PATH. You can add it by updating your shell configuration file (e.g., .bashrc
or .zshrc
):
export PATH=$PATH:./node_modules/.bin
After editing the file, reload your shell configuration:
source ~/.bashrc
4. Clean and Reinstall Dependencies
Corrupted files in the node_modules
directory sometimes cause issues. To fix this:
rm -rf node_modules package-lock.json
npm install
This will delete the node_modules
folder and the package-lock.json
file, then reinstall all dependencies.
5. Update npm
This error can occur if npm is outdated. Update npm to the latest version:
npm install -g npm@latest
6. Resolve Git Hooks Conflicts
If you are using Git hooks with tools like Husky, conflicts might arise. To resolve this, remove the existing Git hooks and reinstall dependencies:
rm -rf .git/hooks
npm install
Additional Tips
- Run
npm doctor
to diagnose potential issues with your npm setup. - Use
npm ci
instead ofnpm install
for a clean, reproducible installation in CI/CD pipelines. - Check the error log for more details, usually located in
npm-debug.log
.
By following these steps, you should be able to resolve the “npm ERR could not determine executable to run” error efficiently. If the problem persists, consider reaching out to community forums or consulting the official npm documentation for further assistance.