Are you preparing for a Node js interview? To ease your journey, we’ve compiled a comprehensive guide of Node js interview questions and answers for experienced professionals. This guide covers all essential advanced concepts, performance optimization techniques, and provides clear, concise answers to the most commonly asked questions.
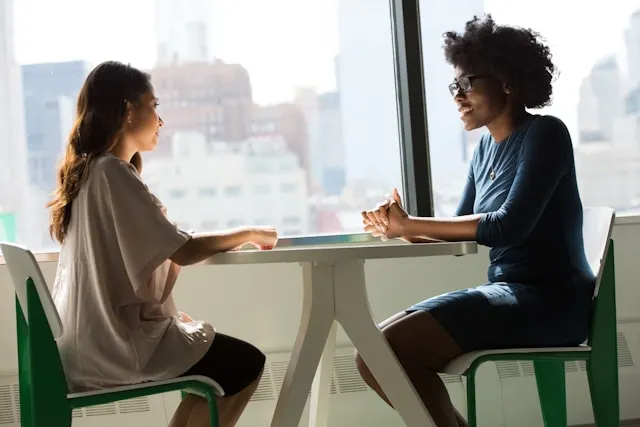
Node js interview questions and answers for experienced
1. How does the Node.js Event Loop work?
2. What are the limitations of Node.js?
3. How can you handle error handling efficiently in Node.js?
4. What is the role of streams in Node.js, and how do they improve performance?
5. Explain clustering in Node.js and how it improves performance.
6. How do you handle authentication and authorization in a Node.js application?
7. What are worker threads in Node.js, and when should you use them?
8. How do you handle real-time data in Node.js?
9. What is the purpose of middleware in Express.js, and what are the types?
10. Explain how you’d optimize a Node.js application for high performance.
11. How do you debug a Node.js application?
12. What is the async_hooks module, and how is it used?
13. Explain process management with PM2 in Node.js.
14. How do you secure a Node.js application?
15. What is the purpose of environment variables in Node.js?
16. Explain event emitters in Node.js and how they work.
17. What is middleware chaining in Express.js, and why is it useful?
18. How does Node.js handle asynchronous I/O?
19. How can you prevent memory leaks in a Node.js application?
20. What are the advantages of using TypeScript with Node.js?
21. How do you implement logging in Node.js?
22. What are child processes in Node.js, and how do you use them?
23. How do you ensure high availability and fault tolerance in a Node.js application?
24. What is the purpose of the zlib module in Node.js?
25. How do you implement request validation in Node.js?
26. Explain async/await versus Promises in Node.js.
27. How does Node.js handle backpressure in streams?
28. What is dependency injection, and how do you implement it in Node.js?
29. What is garbage collection in Node.js, and how can you tune it?
30. How do you handle unhandled promise rejections in Node.js?
31. Explain server-side rendering (SSR) and client-side rendering (CSR) in Node.js.
32. How can you improve the scalability of a Node.js application?
33. What is the difference between a Node.js process and a thread?
34. How do you handle large file uploads in Node.js?
35. What are the different ways to deploy a Node.js application to production?
36. Explain the concept of a microservices architecture and how it can be implemented with Node.js.
37. How do you optimize database queries in a Node.js application?
38. What are the best practices for writing clean and maintainable Node.js code?
39. How do you test a Node.js application?
40. How do you handle rate limiting in a Node.js application?
41. What is the difference between synchronous and asynchronous programming in Node.js?
42. How do you handle web sockets in Node.js?
43. What is the purpose of the cluster module in Node.js?
44. How do you implement server-sent events (SSE) in Node.js?
45. What are the security best practices for Node.js applications?
46. How do you optimize Node.js applications for memory usage?
47. How do you implement internationalization and localization in a Node.js application?
1. How does the Node.js Event Loop work?
Answer:
The Event Loop is a key component in Node.js that processes asynchronous callbacks and allows non-blocking I/O operations. It operates through phases: timers, I/O callbacks, idle/prepare, poll, check, and close callbacks, ensuring efficient handling of multiple tasks without blocking the main thread.
2. What are the limitations of Node.js?
Answer:
Node.js struggles with CPU-bound tasks because it’s single-threaded. High computation tasks block the event loop, affecting performance. Additionally, callback-based asynchronous code can lead to callback hell, though Promises and async/await help mitigate this.
3. How can you handle error handling efficiently in Node.js?
Answer:
Efficient error handling involves using try-catch
blocks for synchronous code, .catch()
for promises, and error
event listeners for streams and EventEmitter. For unhandled rejections, setting up process-level listeners can prevent app crashes.
4. What is the role of streams in Node.js, and how do they improve performance?
Answer:
Streams allow handling data in chunks rather than loading it all at once into memory, which is memory-efficient and faster for handling large files or data transfers. Streams can be readable, writable, duplex, or transform.
5. Explain clustering in Node.js and how it improves performance.
Answer:
Clustering in Node.js enables creating multiple child processes that share the same server port, leveraging multi-core processors. This improves app performance by balancing loads across CPU cores.
6. How do you handle authentication and authorization in a Node.js application?
Answer:
Authentication and authorization can be managed using tokens (JWT), sessions, or OAuth. JWT is often used for stateless authentication, while sessions and cookies work well for stateful authentication.
7. What are worker threads in Node.js, and when should you use them?
Answer:
Worker threads allow multi-threading in Node.js, useful for CPU-intensive tasks, allowing offloading of such operations to avoid blocking the event loop. They are ideal for complex computations, data processing, or machine learning tasks.
8. How do you handle real-time data in Node.js?
Answer:
Real-time data can be managed using WebSockets or libraries like Socket.IO, allowing continuous, bidirectional communication between server and client. This is essential for applications like live chats, gaming, or collaborative tools.
9. What is the purpose of middleware in Express.js, and what are the types?
Answer:
Middleware functions in Express.js intercept requests and perform operations before they reach the route handler. Types include application-level, router-level, error-handling, and built-in middleware.
10. Explain how you’d optimize a Node.js application for high performance.
Answer: Techniques include:
- Using asynchronous and non-blocking functions.
- Enabling caching to reduce data load times.
- Utilizing clusters and worker threads for load balancing.
- Compressing responses and minimizing data transfers.
11. How do you debug a Node.js application?
Answer:
Debugging can be done using tools like Node.js Inspector, VS Code debugger, or Chrome DevTools. Running Node with the --inspect
flag enables debugging, while console.log()
statements are also commonly used for simpler debugging.
12. What is the async_hooks
module, and how is it used?
Answer:
async_hooks
provides APIs to track asynchronous resources in a Node.js application. It is helpful for monitoring the lifecycle of async operations, allowing for better tracking and debugging of callbacks and promises.
13. Explain process management with PM2 in Node.js.
Answer:
PM2 is a process manager for Node.js that facilitates running applications in the background, automatically restarting crashed applications, load balancing, and monitoring performance in production.
14. How do you secure a Node.js application?
Answer: Security best practices include:
- Validating and sanitizing user input.
- Using HTTPS.
- Implementing rate limiting to prevent brute-force attacks.
- Setting secure HTTP headers using
helmet
. - Avoiding vulnerable packages by regularly updating dependencies.
15. What is the purpose of environment variables in Node.js?
Answer:
Environment variables store sensitive configuration details (e.g., API keys, database URLs) outside the source code, improving security. They are accessed using process.env
in Node.js.
16. Explain event emitters in Node.js and how they work.
Answer:
The EventEmitter
module allows objects to emit and listen to events. When an event is emitted, all listeners attached to that event are triggered, enabling a decoupled, event-driven architecture.
17. What is middleware chaining in Express.js, and why is it useful?
Answer:
Middleware chaining allows multiple middleware functions to be executed in a sequence. Each middleware calls next()
to pass control to the next one, useful for modular and organized request handling.
18. How does Node.js handle asynchronous I/O?
Answer:
Node.js handles asynchronous I/O using the Event Loop and asynchronous APIs, delegating I/O operations to the system kernel and using callbacks or promises once the operations complete.
19. How can you prevent memory leaks in a Node.js application?
Answer: Prevent memory leaks by:
- Releasing resources and clearing unused references.
- Using
process.memoryUsage()
to monitor memory. - Avoiding global variables for short-lived objects.
20. What are the advantages of using TypeScript with Node.js?
Answer:
TypeScript provides static typing, making code more robust and easier to debug. It also enhances code readability, supports modern JavaScript features, and improves overall maintainability.
21. How do you implement logging in Node.js?
Answer:
Logging can be implemented using tools like winston
or morgan
. winston
is highly customizable, allowing for different log levels and log transports, while morgan
is excellent for HTTP request logging.
22. What are child processes in Node.js, and how do you use them?
Answer:
Child processes allow executing shell commands and running other applications within a Node.js application. They are created using methods like exec()
, spawn()
, or fork()
and are ideal for parallel processing tasks.
23. How do you ensure high availability and fault tolerance in a Node.js application?
Answer:
Ensuring high availability involves using clustering, load balancing, monitoring, health checks, and auto-restart mechanisms (like PM2) to recover from crashes and handle high traffic smoothly.
24. What is the purpose of the zlib
module in Node.js?
Answer:
The zlib
module provides compression and decompression functionalities, reducing the size of files and data being transferred, which improves data transfer speed and saves bandwidth.
25. How do you implement request validation in Node.js?
Answer:
Request validation can be implemented using libraries like Joi
or express-validator
, ensuring that incoming data conforms to required formats and conditions before processing.
26. Explain async/await versus Promises in Node.js.
Answer:
Promises handle asynchronous code by chaining .then()
and .catch()
, whereas async/await offers a more readable and synchronous-looking code structure, handling Promises using await
.
27. How does Node.js handle backpressure in streams?
Answer:
Node.js uses the pipe()
method to manage backpressure by pausing readable streams when the writable stream buffers reach a limit, preventing data loss and optimizing memory usage.
28. What is dependency injection, and how do you implement it in Node.js?
Answer:
Dependency injection decouples module dependencies by providing required modules at runtime. Libraries like InversifyJS
enable dependency injection in Node.js, useful for creating modular and testable applications.
29. What is garbage collection in Node.js, and how can you tune it?
Answer:
Garbage collection in Node.js automatically frees up memory by removing unused objects. Tuning can be done with --max-old-space-size
and --optimize_for_size
flags to control memory usage.
30. How do you handle unhandled promise rejections in Node.js?
Answer:
Unhandled rejections can be caught globally by adding a listener on process.on('unhandledRejection')
. However, it’s better practice to handle all promises with .catch()
to avoid unhandled rejections.
31. Explain server-side rendering (SSR) and client-side rendering (CSR) in Node.js.
Answer:
SSR renders HTML on the server and sends it to the client, improving initial load speed and SEO. CSR renders HTML on the client using JavaScript, enhancing interactivity and load speed for dynamic content.
32. How can you improve the scalability of a Node.js application?
Answer: To improve the scalability of a Node.js application, consider the following strategies:
- Cluster Module: Utilize multiple worker processes to handle requests.
- Load Balancing: Distribute traffic across multiple instances.
- Caching: Reduce database load and improve response times.
- Asynchronous Programming: Efficiently handle concurrent requests.
- Database Optimization: Optimize database queries and connections.
- Efficient Resource Utilization: Monitor and optimize resource usage.
- Code Optimization: Write efficient and optimized code.
- Serverless Architecture: Leverage serverless platforms for automatic scaling.
- Monitoring and Logging: Track performance and identify issues.
- Continuous Testing: Test under load to identify bottlenecks.
33. What is the difference between a Node.js process and a thread?
Answer:
- Process: A process is an instance of a computer program that is being executed. Each process has its own memory space.
- Thread: A thread is the smallest unit of a process that can be scheduled and executed by the CPU. Threads within the same process share the same memory space.
34. How do you handle large file uploads in Node.js?
Answer:
- Streams: Use streams to handle large files in chunks rather than loading them entirely into memory.
- Multer: Utilize middleware like Multer for handling multipart/form-data for file uploads.
- Busboy: Use libraries like Busboy for processing file uploads efficiently.
35. What are the different ways to deploy a Node.js application to production?
Answer:
- Using Cloud Services: Deploy on cloud platforms like AWS, Azure, Google Cloud, or Heroku.
- Containerization: Use Docker to containerize the application and deploy it using Kubernetes or other orchestration tools.
- PM2: Utilize process managers like PM2 for process management and load balancing.
- Bare Metal: Deploy directly on physical servers or virtual private servers (VPS) using traditional methods.
36. Explain the concept of a microservices architecture and how it can be implemented with Node.js.
Answer:
Microservices Architecture: It is an architectural style that structures an application as a collection of small, loosely coupled, independently deployable services.
Implementation with Node.js:
- Divide Application: Split the application into multiple smaller services, each responsible for a specific functionality.
- API Gateway: Use an API gateway to handle requests and route them to the appropriate services.
- Independent Deployment: Deploy each service independently, often using containers like Docker.
- Inter-Service Communication: Use HTTP/REST or messaging queues for communication between services.
37. How do you optimize database queries in a Node.js application?
Answer:
- Indexing: Use appropriate indexes on database tables to speed up queries.
- Query Optimization: Write optimized queries to minimize database load.
- Connection Pooling: Implement connection pooling to reuse database connections.
- ORM: Use Object-Relational Mappers (ORMs) like Sequelize to manage database interactions efficiently.
- Caching: Cache frequently accessed data to reduce database hits.
38. What are the best practices for writing clean and maintainable Node.js code?
Answer:
- Modularization: Break down the code into small, reusable modules.
- Consistent Naming Conventions: Use meaningful and consistent naming conventions.
- Linting: Use linters like ESLint to enforce code quality.
- Documentation: Write clear documentation for your code and APIs.
- Unit Testing: Implement unit tests to ensure code reliability.
- Code Reviews: Conduct regular code reviews to maintain code quality.
39. How do you test a Node.js application?
Answer:
- Unit Testing: Write unit tests using frameworks like Mocha, Chai, or Jest.
- Integration Testing: Use tools like Supertest to test the interaction between different parts of the application.
- End-to-End Testing: Implement end-to-end testing using tools like Cypress or Selenium.
- Mocking: Use libraries like Sinon for mocking dependencies in tests.
40. How do you handle rate limiting in a Node.js application?
Answer:
- Express Middleware: Use rate-limiting middleware like express-rate-limit to limit the number of requests.
- Third-Party Services: Implement rate limiting with third-party services like Redis.
- API Gateway: Use an API gateway that supports rate limiting.
41. What is the difference between synchronous and asynchronous programming in Node.js?
Answer:
- Synchronous Programming: Operations are executed sequentially, blocking further code execution until the current operation completes.
- Asynchronous Programming: Operations are executed non-blocking, allowing the execution of other code while waiting for the operation to complete, using callbacks, Promises, or async/await.
42. How do you handle web sockets in Node.js?
Answer:
- Socket.IO: Use libraries like Socket.IO to manage WebSocket connections and enable real-time communication between the client and server.
- ws Library: Use the ws library to create WebSocket servers and clients.
43. What is the purpose of the cluster module in Node.js?
Answer:
Cluster Module: It allows the creation of child processes (workers) that share the same server port, enabling the application to utilize multiple CPU cores for better performance and handling a higher number of concurrent requests.
44. How do you implement server-sent events (SSE) in Node.js?
Answer:
- Creating SSE Endpoint: Set up an endpoint that sends a stream of updates to the client.
- Response Headers: Use appropriate headers (e.g.,
Content-Type: text/event-stream
,Cache-Control: no-cache
,Connection: keep-alive
). - Send Events: Use the
res.write()
method to send event data to the client in the correct SSE format.
45. What are the security best practices for Node.js applications?
Answer:
- Input Validation: Validate and sanitize user inputs to prevent SQL injection and XSS attacks.
- HTTPS: Use HTTPS to encrypt data in transit.
- Authentication: Implement strong authentication mechanisms (e.g., JWT, OAuth).
- Environment Variables: Store sensitive information in environment variables.
- Dependencies: Regularly update and audit dependencies to avoid using vulnerable packages.
- Rate Limiting: Implement rate limiting to protect against brute-force attacks.
46. How do you optimize Node.js applications for memory usage?
Answer:
- Garbage Collection: Tune the garbage collection settings.
- Memory Profiling: Use tools like Node.js’s built-in
--inspect
flag or third-party profilers to identify memory leaks. - Efficient Code: Write efficient code to minimize memory usage.
- Streams: Use streams to handle large data efficiently.
47. How do you implement internationalization and localization in a Node.js application?
Answer:
- i18n Libraries: Use libraries like i18next or Globalize for managing translations.
- Message Files: Create separate translation files for different languages.
- Locale Detection: Detect the user’s locale from headers or user preferences and load the appropriate translations.
- Middleware: Use middleware to set up localization context for each request.
Learn More: Carrer Guidance
Node js interview questions and answers for freshers
Hibernate interview questions and answers for freshers
Terraform interview questions and answers for all levels
Rest API interview questions and answers for all levels