Java Database Connectivity (JDBC) is a fundamental technology used for connecting and interacting with databases in Java applications. As a critical skill for Java developers, proficiency in JDBC is often tested in technical interviews. Whether you’re an experienced developer or a fresh graduate, being well-prepared for JDBC-related questions can significantly boost your chances of landing a job.
In this article, we have given some of the most common JDBC interview questions and their answers, especially for experienced candidates. These questions cover various aspects of JDBC (Java Database Connectivity) that are critical for database interaction in Java applications.
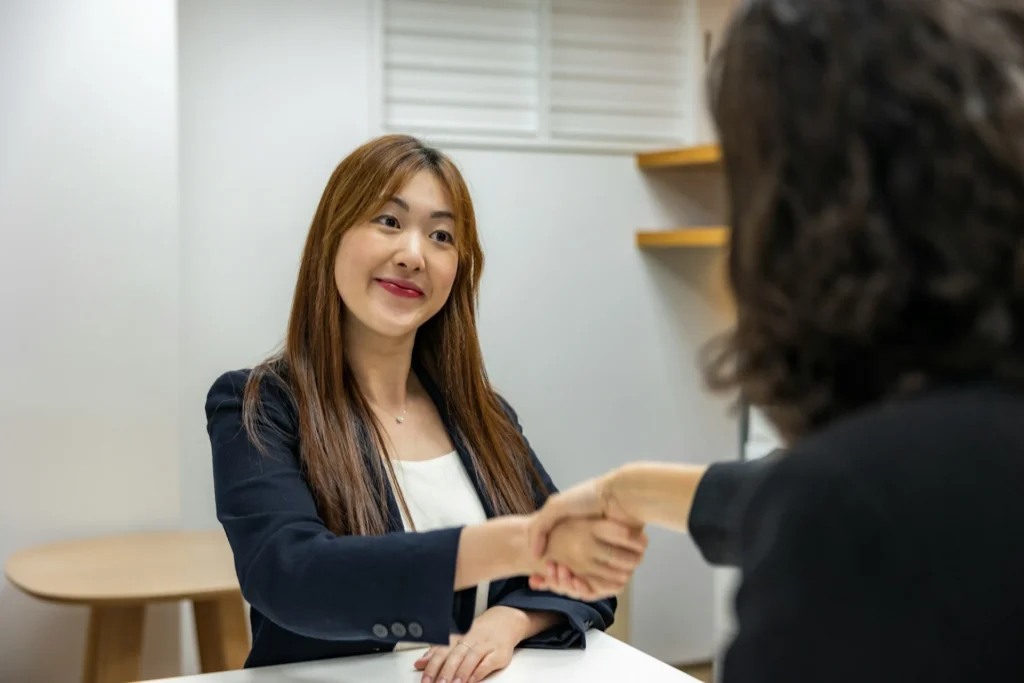
JDBC interview questions and answers
1. What is JDBC?
2. Explain the different types of JDBC drivers.
3. What are the core components of JDBC?
4. What is the difference between Statement and PreparedStatement?
5. How does JDBC handle transactions?
6. What is a ResultSet in JDBC?
7. What are the different types of ResultSets?
8. What is the purpose of the execute() method in JDBC?
9. How do you handle batch processing in JDBC?
10. What is the difference between executeQuery() and executeUpdate()?
11. What are CallableStatement and its use in JDBC?
12. How do you handle SQL exceptions in JDBC?
13. What is the role of DriverManager in JDBC?
14. Explain the concept of connection pooling in JDBC.
15. What is the use of setFetchSize() in JDBC?
16. What is RowSet in JDBC, and how is it different from ResultSet?
17. What is Savepoint in JDBC?
18. Explain how to set parameters in a PreparedStatement.
19. What is Metadata in JDBC?
20. How do you retrieve auto-generated keys in JDBC?
21. What is the JDBC API’s role in database independence?
22. What is the use of DataSource in JDBC?
23. What is the difference between a thin driver and a native-API driver?
24. What are the advantages of using PreparedStatement over Statement?
25. How do you manage database transactions using JDBC?
26. Explain how to implement connection pooling using Apache DBCP or HikariCP.
1. What is JDBC?
Answer:
JDBC stands for Java Database Connectivity. It is a Java API that allows Java programs to interact with relational databases like MySQL, Oracle, SQL Server, etc. JDBC provides methods to query and update data in a database.
2. Explain the different types of JDBC drivers.
Answer: There are 4 types of JDBC drivers:
- Type 1 (JDBC-ODBC Bridge Driver): Converts JDBC calls into ODBC calls.
- Type 2 (Native-API Driver): Converts JDBC calls into database-specific calls using native libraries.
- Type 3 (Network Protocol Driver): Sends JDBC calls to a middle-tier server, which then sends database calls to the backend database.
- Type 4 (Thin Driver): Directly converts JDBC calls to database-specific protocol calls without native libraries or middleware.
3. What are the core components of JDBC?
Answer:
- DriverManager
- Connection
- Statement
- PreparedStatement
- ResultSet
- CallableStatement
4. What is the difference between Statement and PreparedStatement?
Answer:
- Statement: Used for executing static SQL queries without parameters.
- PreparedStatement: Used for executing precompiled SQL queries with input parameters. It’s faster and helps prevent SQL injection attacks.
5. How does JDBC handle transactions?
Answer:
JDBC allows the control of transactions with methods like setAutoCommit(boolean autoCommit)
, commit()
, and rollback()
. By default, each SQL statement is committed automatically. You can disable auto-commit for handling transactions manually.
6. What is a ResultSet in JDBC?
Answer:
A ResultSet
represents a table of data retrieved from a database using a SQL query. It maintains a cursor pointing to its current row of data.
7. What are the different types of ResultSets?
Answer:
- TYPE_FORWARD_ONLY: The cursor can only move forward.
- TYPE_SCROLL_INSENSITIVE: The cursor can scroll forward and backward but does not reflect changes made to the database after the
ResultSet
is created. - TYPE_SCROLL_SENSITIVE: The cursor can scroll forward and backward, and the
ResultSet
reflects database changes after its creation.
8. What is the purpose of the execute()
method in JDBC?
Answer:
The execute()
method is used for executing SQL queries that may return multiple results, such as when executing a Stored Procedure
. It returns a boolean indicating the type of result returned: true
for a ResultSet and false
for an update count.
9. How do you handle batch processing in JDBC?
Answer:
Batch processing in JDBC is handled using the addBatch()
and executeBatch()
methods. This allows multiple SQL statements to be executed in a batch, which improves performance by reducing database communication overhead.
10. What is the difference between executeQuery()
and executeUpdate()
?
Answer:
- executeQuery(): Used for executing SELECT queries. It returns a
ResultSet
. - executeUpdate(): Used for executing INSERT, UPDATE, or DELETE statements. It returns an integer representing the number of affected rows.
11. What are CallableStatement
and its use in JDBC?
Answer:
CallableStatement
is used to execute stored procedures in the database. It allows parameters to be passed into a stored procedure and to retrieve output parameters.
12. How do you handle SQL exceptions in JDBC?
Answer:
SQLExceptions are handled using try-catch blocks. The SQLException
class provides methods like getErrorCode()
, getSQLState()
, and getMessage()
to retrieve details about the exception.
13. What is the role of DriverManager
in JDBC?
Answer:
The DriverManager
class manages a list of database drivers and establishes a connection to a database using the getConnection()
method.
14. Explain the concept of connection pooling in JDBC.
Answer:
Connection pooling allows multiple database connections to be reused instead of opening and closing them repeatedly. This improves performance, especially in web applications. A pool of connections is maintained and shared among different clients.
15. What is the use of setFetchSize()
in JDBC?
Answer:
The setFetchSize()
method is used to give a hint to the database on the number of rows that should be fetched from the database at a time when more rows are needed.
16. What is RowSet in JDBC, and how is it different from ResultSet?
Answer:
A RowSet
is a wrapper around a ResultSet
with additional functionality like scrolling, updating rows, and event notification. Unlike ResultSet
, RowSet
is disconnected and can be serialized.
17. What is Savepoint in JDBC?
Answer:
Savepoints allow transactions to be partially rolled back to a designated point. You can create a savepoint using the setSavepoint()
method and roll back to it using the rollback(Savepoint savepoint)
method.
18. Explain how to set parameters in a PreparedStatement.
Answer:
Parameters in PreparedStatement
are set using methods like setInt()
, setString()
, setDate()
, etc., corresponding to the type of parameter.
19. What is Metadata in JDBC?
Answer: JDBC provides two types of metadata:
- DatabaseMetaData: Provides information about the database itself (e.g., database product name, version, tables, etc.).
- ResultSetMetaData: Provides information about the structure of a
ResultSet
(e.g., number of columns, column types, etc.).
20. How do you retrieve auto-generated keys in JDBC?
Answer:
After executing an INSERT statement using executeUpdate()
, you can retrieve auto-generated keys by calling the getGeneratedKeys()
method.
21. What is the JDBC API’s role in database independence?
Answer:
JDBC API provides a common interface for accessing different relational databases. By using JDBC, Java applications can remain database-independent, as changing the underlying database only requires a different JDBC driver.
22. What is the use of DataSource
in JDBC?
Answer:
DataSource
is an alternative to DriverManager
for establishing a database connection. It allows for connection pooling and is typically used in enterprise applications for better scalability.
23. What is the difference between a thin driver and a native-API driver?
Answer:
A thin driver (Type 4) communicates directly with the database using the database’s native protocol, while a native-API driver (Type 2) requires native database libraries to convert JDBC calls into database-specific calls.
24. What are the advantages of using PreparedStatement over Statement?
Answer:
- Precompiled SQL: PreparedStatement is precompiled by the database, so it runs faster for repeated executions.
- Security: It helps prevent SQL injection attacks by automatically escaping special characters in input parameters.
- Parameterization: PreparedStatement allows for easy parameterization of SQL queries.
25. How do you manage database transactions using JDBC?
Answer: Transactions in JDBC can be managed by:
- Disabling auto-commit using
setAutoCommit(false)
. - Executing multiple SQL queries as part of the transaction.
- Committing the transaction using
commit()
or rolling it back usingrollback()
if an error occurs.
26. Explain how to implement connection pooling using Apache DBCP or HikariCP.
Answer:
Connection pooling can be implemented by configuring a DataSource
object provided by libraries like Apache DBCP or HikariCP. These libraries handle the pooling logic, ensuring that a pool of connections is available for reuse, enhancing performance in multi-threaded applications.
These questions and answers should provide a solid foundation for an experienced developer preparing for a JDBC-related interview.
Learn More: Carrer Guidance
1. Data Analyst Interview Questions for fresher
2. Data Analyst Interview Questions for Experienced
3. Redux Interview Question and Answers for experienced