Are you preparing for MongoDB interview? To help you out, we complied over 40+ most commonly asked MongoDB interview questions along with comprehensive answers that cover key concepts, functionalities, and practical applications of MongoDB.
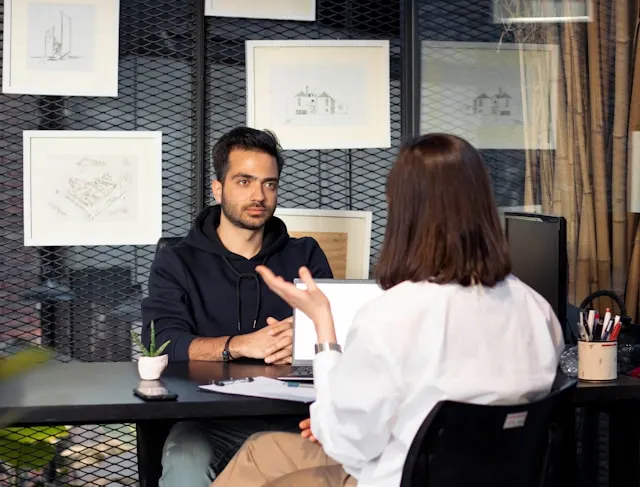
MongoDB interview questions and answers
- What is MongoDB, and how does it differ from traditional SQL databases?
- Explain the structure of BSON in MongoDB.
- What are indexes in MongoDB, and why are they important?
- What is sharding in MongoDB?
- How does replication work in MongoDB?
- Can you explain what a replica set election is?
- What are transactions in MongoDB?
- What are read and write concerns in MongoDB?
- How do you optimize query performance in MongoDB?
- What is GridFS, and when would you use it?
- Describe how you would handle schema design in MongoDB.
- What are capped collections in MongoDB?
- How do you perform aggregation operations in MongoDB?
- Explain what a sharding key is and its significance.
- What are some common pitfalls in MongoDB data modeling?
- How does MongoDB handle schema migrations?
- Can you explain what MapReduce is in MongoDB?
- What monitoring tools can be used with MongoDB?
- How do you perform backups in MongoDB?
- What are some best practices for using MongoDB?
- What are MongoDB Atlas and MongoDB Enterprise?
- What is a MongoDB database profiler?
- How does MongoDB handle concurrency?
- What is a change stream, and when would you use it?
- How does MongoDB handle ACID properties?
- What is the aggregation pipeline, and how does it work?
- Explain the $lookup operation and when to use it.
- What is a MongoDB projection, and why is it useful?
- How do you manage user authentication and roles in MongoDB?
- What are MongoDB Atlas triggers, and how do they work?
- Describe MongoDB’s support for geospatial data and queries.
- How does MongoDB’s WiredTiger storage engine work?
- What are time-series collections in MongoDB, and when would you use them?
- How does MongoDB handle data partitioning across shards?
- What is the oplog, and what role does it play in replication?
- How do you use MongoDB with programming languages like Python or Java?
- Explain the $facet stage in the aggregation pipeline.
- How does MongoDB handle large data sets and ensure scalability?
- What are field-level encryption and client-side encryption in MongoDB?
- How does MongoDB implement zero-downtime deployment?
1. What is MongoDB, and how does it differ from traditional SQL databases?
Answer:
MongoDB is a NoSQL database that utilizes a document-oriented data model, storing data in BSON (Binary JSON) format. Unlike traditional SQL databases, which organize data into tables with fixed schemas, MongoDB allows for a flexible schema where documents can have varying structures. This flexibility facilitates rapid development and iteration by allowing changes to the data model without requiring extensive database migrations.
Key Differences:
- Schema: SQL databases have a rigid schema; MongoDB has a dynamic schema.
- Data Storage: SQL uses rows and columns; MongoDB uses documents and collections.
- Query Language: SQL uses structured query language (SQL); MongoDB uses its own query language based on JSON-like syntax.
- Scalability: SQL databases typically scale vertically, while MongoDB supports horizontal scaling through sharding.
2. Explain the structure of BSON in MongoDB.
Answer: BSON (Binary JSON) is the data format used by MongoDB to represent documents. It extends JSON’s capabilities by including additional data types such as Date
, ObjectId
, and binary data. BSON is designed to be efficient in both storage space and speed of traversal.
Structure:
- Format: BSON is binary-encoded, which allows for faster parsing compared to JSON.
- Data Types: Supports various types including strings, integers, arrays, embedded documents, and more.
- Size: BSON documents can be up to 16 MB in size, accommodating large amounts of data within a single document.
3. What are indexes in MongoDB, and why are they important?
Answer: Indexes in MongoDB are special data structures that improve the speed of data retrieval operations on a collection. They function similarly to indexes in traditional databases by allowing the database engine to find documents more quickly without scanning every document in a collection.
Importance of Indexes:
- Performance Improvement: Indexes significantly reduce the time required to execute queries.
- Types of Indexes: Includes single-field indexes, compound indexes (multiple fields), text indexes (for string searches), geospatial indexes (for location-based queries), and hashed indexes (for sharding).
- Default Indexes: By default, an index on the
_id
field is created for every collection.
4. What is sharding in MongoDB?
Answer: Sharding is a method used by MongoDB to distribute data across multiple servers or clusters. It enables horizontal scaling by partitioning large datasets into smaller chunks called shards.
Key Aspects:
- Sharding Key: A specific field or fields that determine how data is distributed across shards. The choice of sharding key affects performance and scalability.
- Benefits: Improves performance by balancing loads across multiple servers and allows for handling large datasets that exceed the capacity of a single server.
- Automatic Balancing: MongoDB automatically balances the distribution of data across shards as new shards are added or removed.
5. How does replication work in MongoDB?
Answer: Replication in MongoDB involves creating multiple copies of data across different servers to ensure high availability and redundancy. This is typically achieved through replica sets.
Replica Set Components:
- Primary Node: The main node that accepts write operations.
- Secondary Nodes: Replicas of the primary node that can serve read requests and provide failover capabilities.
- Automatic Failover: If the primary node fails, an election process occurs among secondary nodes to select a new primary automatically.
6. Can you explain what a replica set election is?
Answer: A replica set election occurs when the primary node in a replica set becomes unavailable due to failure or maintenance. The remaining nodes participate in an election process to select a new primary node.
Election Process:
- Each secondary node votes for a candidate based on criteria such as health status and last heartbeat time.
- The candidate with the majority of votes becomes the new primary.
- Elections ensure continuous availability of write operations even during failures.
7. What are transactions in MongoDB?
Answer: MongoDB supports multi-document transactions since version 4.0, allowing multiple operations on different documents to be executed atomically.
Key Features:
- ACID Compliance: Transactions ensure that all operations within them either succeed or fail together, maintaining data integrity.
- Usage: Transactions can be used for complex operations involving multiple collections or documents where consistency is critical.
- Syntax Example:
session.startTransaction();
try {
// Perform multiple operations
session.commitTransaction();
} catch (error) {
session.abortTransaction();
}
8. What are read and write concerns in MongoDB?
Answer: Read and write concerns define the level of acknowledgment required from the database for read and write operations.
Write Concern Levels:
w: 1
: Acknowledgment from the primary only.w: "majority"
: Acknowledgment from the majority of nodes in the replica set.
Read Concern Levels:
local
: Returns the most recent data available on the primary.majority
: Ensures that the read operation returns data acknowledged by the majority of nodes.
9. How do you optimize query performance in MongoDB?
Answer: Optimizing query performance involves several strategies:
- Indexing: Create appropriate indexes based on query patterns to speed up searches.
db.collection.createIndex({ fieldName: 1 }); // Ascending index
- Query Patterns: Use efficient query patterns that leverage existing indexes rather than performing full collection scans.
db.collection.find({ indexedField: value });
- Aggregation Framework: Utilize aggregation pipelines for complex queries instead of multiple round trips to the database.
10. What is GridFS, and when would you use it?
Answer: GridFS is a specification for storing and retrieving large files such as images or videos within MongoDB. It divides files into smaller chunks (default size is 255 KB) stored as individual documents.
Use Cases:
- Storing large binary files that exceed BSON document size limits (16 MB).
- Efficient retrieval of large files using metadata stored alongside file chunks.
11. Describe how you would handle schema design in MongoDB.
Answer: Schema design in MongoDB requires careful consideration due to its flexible nature:
Key Considerations:
- Embedding vs Referencing: Decide whether to embed related data within a single document (denormalization) or reference documents across collections (normalization).
// Embedded Document Example
{
name: "John Doe",
address: { street: "123 Main St", city: "Anytown" }
}
- Document Structure: Design documents according to application query patterns for efficient access.
12. What are capped collections in MongoDB?
Answer: Capped collections are fixed-size collections that maintain insertion order and automatically overwrite old documents when they reach their size limit.
Characteristics:
- They offer high-performance logging capabilities due to their fixed size.
db.createCollection("logs", { capped: true, size: 100000 }); // Size in bytes
13. How do you perform aggregation operations in MongoDB?
Answer: Aggregation operations allow you to process data records and return computed results using the aggregation framework.
Common Stages in Aggregation Pipeline:
$match
: Filters documents based on specified criteria.
db.collection.aggregate([{ $match: { status: "active" } }]);
$group
: Groups documents by specified fields and performs aggregations like sum or average.
db.collection.aggregate([{ $group: { _id: "$category", totalSales: { $sum: "$amount" } } }]);
14. Explain what a sharding key is and its significance.
Answer:
A sharding key determines how data is distributed across shards in a sharded cluster. Choosing an appropriate sharding key is crucial for balancing load evenly across shards and optimizing query performance.
Significance:
- A well-chosen sharding key ensures even distribution of documents across shards, preventing hotspots where one shard handles more traffic than others.
15. What are some common pitfalls in MongoDB data modeling?
Answer: Common pitfalls include:
- Not choosing an appropriate sharding key leading to uneven distribution of data.
- Failing to understand query patterns which can result in inefficient indexing strategies.
- Over-normalizing or under-normalizing data can lead to performance issues; striking the right balance between embedding and referencing is essential.
16. How does MongoDB handle schema migrations?
Answer: MongoDB’s flexible schema allows for easier schema migrations compared to traditional relational databases:
Migration Strategies:
- In-place updates can be used for minor changes without downtime.
db.collection.updateMany({}, { $set: { newField: "defaultValue" } });
- Background processes can manage larger migrations while keeping the application online.
17. Can you explain what MapReduce is in MongoDB?
Answer: MapReduce is a programming model used for processing large datasets with parallel algorithms on distributed systems. In MongoDB, it allows you to perform complex aggregations beyond what can be achieved with simple queries or aggregation frameworks.
Process Steps:
- Map Function: Processes input records and emits key-value pairs.
- Reduce Function: Merges values associated with the same key into a single output value.
db.collection.mapReduce(
function() { emit(this.category, this.amount); },
function(key, values) { return Array.sum(values); },
{ out: "resultCollection" }
);
18. What monitoring tools can be used with MongoDB?
Answer: Monitoring tools help track performance metrics and system health:
- MongoDB Atlas Monitoring: Provides real-time insights into database performance through cloud-based monitoring tools.
- MongoDB Ops Manager: Offers on-premise monitoring solutions with alerts and backup capabilities.
- Third-party Tools: Tools like Prometheus or Grafana can also be integrated for advanced monitoring solutions.
19. How do you perform backups in MongoDB?
Answer: Backups can be performed using several methods:
- Mongodump/Mongorestore: Command-line tools that create binary backups of your database collections.
mongodump --db myDatabase --out /backup/location
- Cloud Backups: If using Atlas or Ops Manager, automated backups can be scheduled easily through their interfaces.
- File System Snapshots: For replica sets, file system snapshots can also serve as effective backup solutions if properly managed.
20. What are some best practices for using MongoDB?
Answer: Best practices include:
- Always use indexes appropriately based on query patterns for optimal performance.
- Regularly monitor your database using built-in tools or third-party solutions to catch issues early.
- Design your schema considering future scalability needs—plan for potential growth when choosing sharding keys or embedding versus referencing strategies.
21. What are MongoDB Atlas and MongoDB Enterprise?
Answer:
MongoDB Atlas is a cloud-hosted database-as-a-service (DBaaS) that simplifies deployment and management by offering managed MongoDB clusters. It is hosted on platforms like AWS, Google Cloud, and Azure and provides features like automated backups, monitoring, and scaling. MongoDB Enterprise, on the other hand, is a self-managed, on-premise version intended for organizations needing advanced security, operational support, and compliance features. MongoDB Enterprise includes access to the BI Connector for SQL-based analytics and LDAP support for user authentication.
22. What is a MongoDB database profiler?
Answer:
The MongoDB database profiler tracks performance-related statistics for operations. This tool helps identify slow queries, bottlenecks, and problematic write operations by logging queries that exceed a certain execution threshold. Profiling data is stored in the system.profile
collection, and profiling levels can be adjusted based on performance needs.
23. How does MongoDB handle concurrency?
Answer:
MongoDB uses a multi-granular locking system. It employs a global lock, database-level locks, and collection-level locks to control concurrency. Document-level locks ensure high concurrency, meaning individual documents are locked rather than collections, which optimizes performance by allowing multiple read and write operations on different documents concurrently.
24. What is a change stream, and when would you use it?
Answer:
Change streams in MongoDB allow applications to receive real-time notifications on data changes, such as inserts, updates, or deletes, in collections or databases. Change streams are useful for tracking changes in data, implementing real-time analytics, or syncing changes to another system. They rely on the replica set oplog to monitor and stream changes.
25. How does MongoDB handle ACID properties?
Answer:
MongoDB provides ACID compliance for single-document operations. In version 4.0 and later, MongoDB introduced multi-document ACID transactions for replica sets, and in 4.2, extended this to sharded clusters. MongoDB transactions enable atomicity and isolation for operations across multiple documents within a collection, supporting complex workflows.
26. What is the aggregation pipeline, and how does it work?
Answer:
The aggregation pipeline in MongoDB is a powerful framework for data transformation and analysis. It processes documents in stages, where each stage applies an operation (e.g., filtering, grouping, sorting) on the input documents. This feature is highly efficient for handling large datasets and performing data manipulations directly on the server side.
27. Explain the $lookup operation and when to use it.
Answer:
The $lookup
operation in MongoDB’s aggregation pipeline is used for performing left outer joins between collections. It allows you to combine documents from multiple collections based on a common field. $lookup
is particularly useful for joining related documents without embedding, which helps keep collections modular and reduces redundancy.
28. What is a MongoDB projection, and why is it useful?
Answer:
Projections in MongoDB are used to include or exclude specific fields in query results. By specifying only the required fields, projections reduce the amount of data transferred from the database, which can improve performance. Projections are specified in a query by setting fields to 1 (include) or 0 (exclude).
29. How do you manage user authentication and roles in MongoDB?
Answer:
MongoDB supports role-based access control (RBAC) for managing user permissions. Administrators can create roles with specific privileges (e.g., read, readWrite, dbAdmin) and assign them to users. Authentication methods include username/password, LDAP integration, and x.509 certificate authentication, which provides flexibility based on security requirements.
30. What are MongoDB Atlas triggers, and how do they work?
Answer:
MongoDB Atlas triggers allow automatic execution of functions based on database events, HTTP requests, or a fixed schedule. These triggers can perform actions like updating other documents, calling external APIs, or sending notifications, enabling real-time automation and facilitating event-driven architecture.
31. Describe MongoDB’s support for geospatial data and queries.
Answer:
MongoDB offers extensive support for geospatial data, allowing users to store coordinates and perform spatial queries. It provides two types of geospatial indexes: 2d indexes for flat geometry and 2dsphere indexes for spherical geometry, supporting queries like $near
, $geoWithin
, and $geoIntersects
.
32. How does MongoDB’s WiredTiger storage engine work?
Answer:
WiredTiger is MongoDB’s default storage engine, optimized for high concurrency and low disk I/O. It uses a combination of B-tree and LSM (Log-Structured Merge) trees, employs document-level locking, and utilizes compression techniques to improve storage efficiency. WiredTiger ensures faster reads and writes, particularly for write-intensive workloads.
33. What are time-series collections in MongoDB, and when would you use them?
Answer:
Time-series collections are optimized for storing time-series data, like sensor readings or financial data. MongoDB provides built-in support for efficient data insertion, compaction, and querying for time-series collections, making it suitable for applications that require efficient handling of temporal data with predictable timestamp-based patterns.
34. How does MongoDB handle data partitioning across shards?
Answer:
MongoDB uses sharding to distribute data across multiple servers. Data partitioning is managed using a shard key, and MongoDB divides data into ranges or zones based on this key. Each shard contains a subset of the data, allowing horizontal scaling by adding new servers and balancing data and load distribution.
35. What is the oplog, and what role does it play in replication?
Answer:
The oplog (operations log) is a capped collection in MongoDB that logs all changes to data in a replica set. Each secondary node reads the oplog from the primary node to replicate data changes, maintaining consistency across nodes. The oplog is crucial for providing data redundancy and ensuring that all nodes have up-to-date data.
36. How do you use MongoDB with programming languages like Python or Java?
Answer:
MongoDB provides official drivers and APIs for multiple programming languages, including Python, Java, Node.js, and C#. For Python, MongoDB offers the pymongo
library, while Java uses the MongoDB Java Driver
. These libraries provide methods to connect, query, and manipulate MongoDB data directly from the application code.
37. Explain the $facet stage in the aggregation pipeline.
Answer:
The $facet
stage in MongoDB’s aggregation pipeline allows simultaneous execution of multiple aggregations on the same input data within a single query. It outputs multiple result sets as different fields within a single document, enabling efficient data analysis by combining different aggregation results in a single response.
38. How does MongoDB handle large data sets and ensure scalability?
Answer:
MongoDB scales horizontally using sharding, where data is distributed across multiple servers or clusters. This setup allows MongoDB to handle large datasets by partitioning data, reducing the load on each individual server. MongoDB’s architecture also supports adding new shards as data grows, ensuring continued scalability.
39. What are field-level encryption and client-side encryption in MongoDB?
Answer:
Field-level encryption allows data to be encrypted at the field level within documents, ensuring sensitive data remains encrypted even within the database. Client-side encryption enables data encryption before sending it to MongoDB, so encrypted data is never exposed to the server. Both methods enhance data security and compliance.
40. How does MongoDB implement zero-downtime deployment?
Answer:
MongoDB supports zero-downtime deployment by utilizing replica sets and rolling upgrades. By sequentially upgrading or changing configuration on replica set members, MongoDB maintains availability, as at least one replica set member remains online. For sharded clusters, maintenance can occur on individual shards without impacting the overall system.
Learn More: Carrer Guidance
ServiceNow Interview Questions and Answers
DevOps Interview Questions and Answers for Freshers
Record to Report (R2R) Interview Questions and Answers- Basic to Advance
Playwright interview questions and answers- Basic to Advance
Power Automate Interview Questions and Answers
Selenium Interview Questions for Candidates with 5 Years of Experience