TanStack Suite continues to redefine how developers create high-performance, full-stack applications. With its robust set of tools—powered by Vinxi, a JavaScript SDK for building full-stack apps with Vite—the TanStack Suite integrates seamlessly into the modern developer’s workflow. From client-side data management to server-side features, it bridges the gap between functionality and user experience.
This guide explores the key tools in the TanStack Suite and their practical applications for building modern React applications.
What is the TanStack Suite?
The TanStack Suite is a collection of JavaScript libraries that provide developers with a first-class front-end experience and robust back-end capabilities. In 2025, it is a popular choice alongside frameworks like Astro, Next.js, and Remix. Its core tools include:
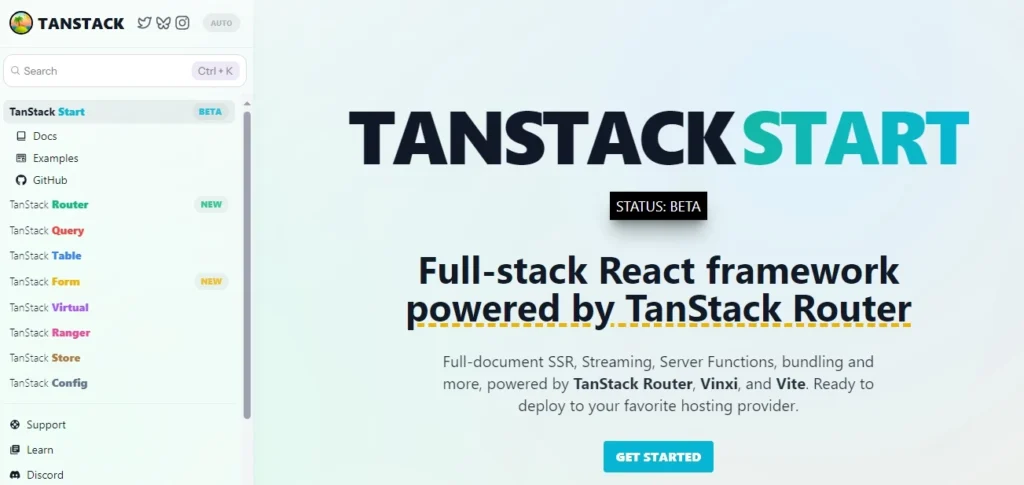
- TanStack Query: Advanced data fetching and state management.
- TanStack Router: Flexible, high-performance routing.
- TanStack Table: Headless table creation.
- TanStack Virtual: Efficient virtualization for large datasets.
- TanStack Form: Streamlined form management with validation.
Why Choose the TanStack Suite for React Applications?
The TanStack Suite offers:
- Unified Development: Consistent API design across tools simplifies learning and integration.
- Performance: Optimized for speed and scalability.
- Flexibility: Works seamlessly with React and other frameworks like Remix and Astro.
- Active Community: Backed by extensive documentation and a growing ecosystem.
Key Tools in the TanStack Suite
1. TanStack Query: Efficient Data Management
TanStack Query excels at managing server state with features like caching, background synchronization, and automatic retries. To set it up:
npm install @tanstack/react-query @tanstack/react-query-devtools
In your main.tsx
, initialize the Query Client:
import { QueryClient, QueryClientProvider } from '@tanstack/react-query';
import { ReactQueryDevtools } from '@tanstack/react-query-devtools';
const queryClient = new QueryClient({
defaultOptions: {
queries: { staleTime: 1000 * 60 * 5, gcTime: 1000 * 60 * 60 },
},
});
<QueryClientProvider client={queryClient}>
<YourApp />
<ReactQueryDevtools initialIsOpen={false} />
</QueryClientProvider>;
2. TanStack Router: High-Performance Routing
With TanStack Router, you can create nested, file-based, or dynamic routes effortlessly. Setup involves:
npm create @tanstack/router@latest --legacy-peer-deps
For a basic router setup:
import { createRouter, createRouteConfig } from '@tanstack/router';
const rootRoute = createRouteConfig({ component: () => <Root /> });
const childRoute = rootRoute.createRoute({ path: '/child', component: () => <Child /> });
export const router = createRouter({ routeConfig: rootRoute.addChildren([childRoute]) });
3. TanStack Table: Headless Table Management
TanStack Table provides a headless API for creating customizable tables. Install it with:
npm install @tanstack/react-table
Example of a basic data table:
import { useReactTable, getCoreRowModel, ColumnDef } from '@tanstack/react-table';
const columns = [
{ accessorKey: 'name', header: 'Name' },
{ accessorKey: 'age', header: 'Age' },
];
const table = useReactTable({
data: [{ name: 'John', age: 30 }],
columns,
getCoreRowModel: getCoreRowModel(),
});
4. TanStack Virtual: Efficient Virtualization
For large datasets, TanStack Virtual ensures efficient rendering of only visible elements. Install it:
npm install @tanstack/react-virtual
5. TanStack Form: Simplified Form Management
Streamline form validation with TanStack Form and Zod:
npm install @tanstack/react-form zod
Example of a validated form:
import { useForm } from '@tanstack/react-form';
import { z } from 'zod';
const schema = z.object({ name: z.string().min(1), age: z.number().positive() });
const form = useForm({ schema, onSubmit: (data) => console.log(data) });
Step-by-Step: Setting Up a TanStack React Project
1. Create a New React Project
Use Vite for an optimized setup:
npm create vite@latest my-tanstack-app --template react-ts
cd my-tanstack-app
npm install
2. Install Required TanStack Tools
npm install @tanstack/react-query @tanstack/router @tanstack/react-table @tanstack/react-virtual
3. Build the Application
- Add routes with TanStack Router.
- Fetch and manage data with TanStack Query.
- Create tables and handle large datasets with TanStack Table and TanStack Virtual.
- Implement forms with TanStack Form for user input.
Advanced Features of the TanStack Suite
The suite offers additional tools for advanced use cases:
- TanStack Virtual: Ideal for scrollable data and virtualized lists.
- TanStack Config: Configure and publish JavaScript packages.
- TanStack Store: Advanced state management.
Conclusion
The TanStack Suite is a game-changer for building modern React applications in 2025. With tools like TanStack Query, Router, Table, Virtual, and Form, developers can create efficient, scalable, and maintainable applications. Get official TanStack documentation for more in-depth guidance and unleash the full potential of your React projects.