Laravel, a popular PHP framework, has gained significant traction among developers due to its elegant syntax, expressive API, and robust features. If you’re preparing for a Laravel interview, it’s essential to have a solid understanding of its core concepts, popular packages, and best practices.
This article provides list of collection of most frequently asked Laravel interview questions and answers, covering a wide range of topics from fundamental concepts to advanced techniques. Whether you’re a experienced Laravel developer or just starting your journey, these questions will help you assess your knowledge and prepare for your interview with confidence.
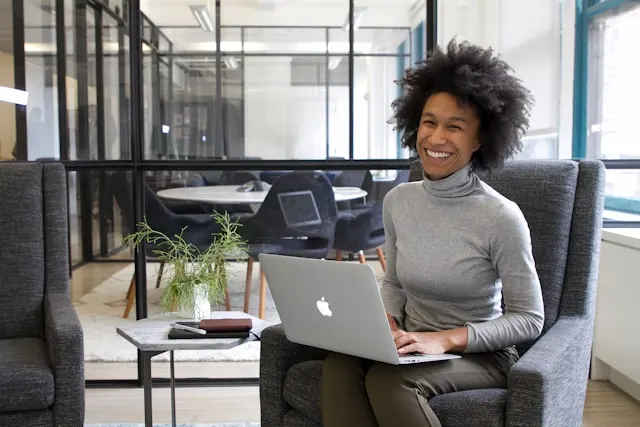
Laravel interview questions and answers
Core Laravel Concepts:
- What is Laravel?
- What are the main features of Laravel?
- What is Composer in Laravel?
- What is Artisan?
- What is Eloquent in Laravel?
- Explain Middleware in Laravel.
- What is the purpose of service providers in Laravel?
- How does routing work in Laravel?
- What are Controllers in Laravel?
- Explain CSRF protection in Laravel.
- What are Laravel Facades?
- What is Dependency Injection in Laravel?
- What is Blade Template Engine?
- What are Laravel Migrations?
- What are Seeders in Laravel?
- Explain Queues in Laravel.
- What is a Repository pattern in Laravel?
- What is Laravel Scout?
- How does Event Handling work in Laravel?
- What is Rate Limiting in Laravel?
- Explain Soft Deletes in Laravel.
- What are Observers in Laravel?
- How can you improve performance in Laravel?
- How to enable maintenance mode in Laravel?
- What is Lazy Loading in Laravel?
- What are Guards and Providers in Laravel’s authentication system?
- What is a Job in Laravel?
Advanced Laravel Concepts:
- Explain the Laravel Service Container and its use cases.
- What is the difference between include and yield in Blade templating?
- Explain how the Repository pattern is implemented in Laravel.
- What is the purpose of Laravel’s bind and singleton methods in the Service Container?
- How does Laravel handle multi-database setups (connections to multiple databases)?
- What are Laravel’s query scopes, and how can they be used?
- How does Laravel handle Eager Loading and Lazy Loading in Eloquent?
- Explain Laravel’s Middleware and how to create a custom middleware.
- What are Jobs and Queues in Laravel, and how do they improve performance?
- Describe how you would handle file uploads in Laravel.
- What is Event Broadcasting in Laravel, and how does it work?
- How does Laravel implement rate limiting, and where is it useful?
- How can you optimize a Laravel application for better performance?
- How would you test routes in Laravel?
- What is Laravel Nova and how does it differ from Laravel Admin?
- Explain how to use API Resource collections in Laravel.
- How do you handle database transactions in Laravel?
- Explain how the policy and gate authorization mechanisms work in Laravel.
- How does the Laravel Scheduler work, and what are its advantages?
- How does Laravel’s Passport package facilitate API authentication?
- What are Traits in Laravel, and when should they be used?
- How does Laravel prevent SQL Injection?
- What are the benefits of using Laravel Horizon for managing queues?
- How does Laravel’s Localization feature work?
- What are Laravel’s custom casts and when would you use them?
- Explain how to implement task scheduling using Laravel Task Scheduler.
- How do you implement multi-tenancy in Laravel?
- What is a Job Chain in Laravel, and how do you use it?
- How do you use Laravel’s broadcast helper to send notifications in real time?
- Explain Laravel’s rate limiting using Redis.
Level 1: Core Laravel Concepts-Laravel interview questions and Interview
1. What is Laravel?
Answer:
Laravel is an open-source PHP web framework that follows the MVC (Model-View-Controller) architectural pattern. It is designed for building robust web applications with an expressive and elegant syntax.
2. What are the main features of Laravel?
Answer:
- Artisan CLI
- Eloquent ORM
- Blade Templating Engine
- Routing
- Middleware
- Queue Management
- Authentication and Authorization
- Database Migrations
3. What is Composer in Laravel?
Answer:
Composer is a dependency manager for PHP. It allows developers to manage libraries and packages that Laravel or any PHP project relies on.
4. What is Artisan?
Answer:
Artisan is Laravel’s command-line interface (CLI) used for automating repetitive tasks, such as generating migrations, models, controllers, etc.
5. What is Eloquent in Laravel?
Answer:
Eloquent is Laravel’s built-in ORM (Object-Relational Mapping) tool that allows developers to interact with the database using an active record pattern, making database operations simple and easy to read.
6. Explain Middleware in Laravel.
Answer:
Middleware is a filtering mechanism that intercepts HTTP requests coming into the application and processes them before passing them to controllers, allowing things like authentication, logging, and CORS (Cross-Origin Resource Sharing) handling.
7. What is the purpose of service providers
in Laravel?
Answer:
Service providers are the central place where Laravel applications bootstrap. They are used to bind classes and services into the Laravel’s service container for dependency injection.
8. How does routing work in Laravel?
Answer:
Routes in Laravel map URLs to their corresponding controllers and methods. You define routes in the routes/web.php
file for web interfaces and in routes/api.php
for API routes.
9. What are Controllers in Laravel?
Answer:
Controllers handle user requests by interacting with models and returning a view or response. They help in separating the business logic from the presentation layer in an MVC architecture.
10. Explain CSRF protection in Laravel.
Answer:
Laravel automatically generates a CSRF token for each active user session. This token is used to verify that the authenticated user is the one actually making the request. CSRF tokens help to protect against cross-site request forgery attacks.
11. What are Laravel Facades?
Answer:
Facades provide a static-like interface to classes available in the application’s service container. They are used to call functions that are tied to a specific component of Laravel, such as DB::select()
for database operations.
12. What is Dependency Injection in Laravel?
Answer:
Dependency Injection is a design pattern where a class’s dependencies are injected into it instead of being created inside the class. Laravel uses dependency injection to resolve and manage dependencies automatically.
13. What is Blade Template Engine?
Answer:
Blade is Laravel’s powerful templating engine that allows you to use plain PHP code and provides control structures like loops and conditionals directly in the view files.
14. What are Laravel Migrations?
Answer:
Migrations are a version control system for your database. They allow you to define the structure of your database in PHP code, making it easy to modify and share the database schema.
15. What are Seeders in Laravel?
Answer:
Seeders allow you to insert default or sample data into the database. You define seeders in database/seeds
directory and run them using Artisan commands.
16. Explain Queues in Laravel.
Answer:
Laravel queues provide a unified API across different queue backends and allow you to defer the processing of time-consuming tasks, such as sending emails, to background processes.
17. What is a Repository pattern in Laravel?
Answer:
The Repository pattern is a design pattern used to abstract data access logic from the business logic. It helps to centralize data fetching and persistence, making it easier to maintain and test.
18. What is Laravel Scout?
Answer:
Laravel Scout provides a simple driver-based solution for adding full-text search to your Eloquent models. It integrates with search engines like Algolia and makes it easy to sync and search models.
19. How does Event Handling work in Laravel?
Answer:
Laravel’s event handling system allows you to subscribe and listen to various application events. It provides an observer pattern for responding to specific actions, such as user registration or order creation.
20. What is Rate Limiting in Laravel?
Answer:
Laravel provides rate limiting functionality to restrict the number of requests a user can make within a certain time frame. It is useful for protecting your application against DDoS attacks.
21. Explain Soft Deletes in Laravel.
Answer:
Soft deletes allow you to keep records in the database even after they have been deleted. Instead of being removed, a deleted_at
timestamp is set, indicating the deletion date.
22. What are Observers in Laravel?
Answer:
Observers are used to listen to the model’s lifecycle events, such as creating, updating, deleting, etc. They help to centralize the event logic for a model.
23. How can you improve performance in Laravel?
Answer:
- Use eager loading
- Cache configurations, routes, and views
- Use Redis or Memcached for caching
- Optimize database queries
- Minimize use of
foreach
and other loop-based operations
24. How to enable maintenance mode in Laravel?
Answer:
You can enable maintenance mode using the Artisan command:
php artisan down
To disable maintenance mode, use:
php artisan up
25. What is Lazy Loading in Laravel?
Answer:
Lazy loading is a technique where related models are not loaded until they are referenced in the code. This can lead to N+1 query problems if not managed properly.
26. What are Guards and Providers in Laravel’s authentication system?
Answer:
- Guards: Define how users are authenticated for each request.
- Providers: Define how users are retrieved from the storage backend (e.g., database).
27. What is a Job in Laravel?
Answer:
Jobs are tasks that are queued for background processing. Jobs allow you to defer the execution of time-consuming tasks, such as sending emails or processing large datasets, until a later time
Level 2: Advanced Laravel Concepts- Laravel interview questions and Interview
28. Explain the Laravel Service Container and its use cases.
Answer:
The Laravel Service Container is a powerful tool for managing class dependencies and performing dependency injection. It acts as a container that binds components (services) together, facilitating the resolution of objects via automatic dependency injection.
Example Use Case: It can be used to resolve interfaces to concrete implementations, or to manage singletons in complex applications.
29. What is the difference between include
and yield
in Blade templating?
Answer:
@include
is used to insert a Blade view file into another file, allowing for partial views (like headers or footers).@yield
is a placeholder for sections that child templates can fill, used in layout inheritance (like main content areas).
30. Explain how the Repository pattern is implemented in Laravel.
Answer:
The Repository pattern separates the logic that retrieves data from the database from the business logic. It provides an abstraction layer between the data source and the application, allowing for more flexible testing and code reuse.
31. What is the purpose of Laravel’s bind
and singleton
methods in the Service Container?
Answer:
bind
: Registers a class or interface with the service container. Each time the container resolves the class, a new instance is returned.singleton
: Registers a class as a singleton in the container. Once resolved, the same instance will be returned on subsequent calls.
32. How does Laravel handle multi-database setups (connections to multiple databases)?
Answer:
Laravel allows connecting to multiple databases by configuring them in the config/database.php
file and defining separate connections. You can switch between connections in queries using the DB::connection('connection_name')
method or by configuring models to use different connections.
33. What are Laravel’s query scopes, and how can they be used?
Answer:
Query scopes are a way to encapsulate repetitive query logic within a model. Global scopes apply to all queries for the model, while local scopes allow for reusable query constraints.
Example:
public function scopeActive($query) { return $query->where('status', 1); }
34. How does Laravel handle Eager Loading and Lazy Loading in Eloquent?
Answer:
- Eager Loading: It retrieves related models as part of the initial query, avoiding the “N+1” query problem. You use
with()
for eager loading. - Lazy Loading: It retrieves related models on-demand, when the relationship is accessed for the first time.
35. Explain Laravel’s Middleware and how to create a custom middleware.
Answer:
Middleware filters HTTP requests entering your application. It’s commonly used for tasks such as authentication, CORS, or logging. To create custom middleware:
php artisan make:middleware CustomMiddleware
36. What are Jobs and Queues in Laravel, and how do they improve performance?
Answer:
Jobs represent units of work that can be dispatched to queues for deferred execution. Queues allow you to defer tasks like sending emails, notifications, or processing data, which improves performance by offloading long-running tasks from the main thread.
37. Describe how you would handle file uploads in Laravel.
Answer:
Laravel provides simple methods for handling file uploads via Request
. You can use the store
, move
, or storeAs
methods to upload files and manage their storage.
38. What is Event Broadcasting in Laravel, and how does it work?
Answer:
Event Broadcasting is used to broadcast events to a real-time interface (like WebSockets). It involves using events, listeners, and broadcasting mechanisms to sync real-time data between the server and the client.
39. How does Laravel implement rate limiting, and where is it useful?
Answer:
Laravel provides rate limiting via middleware (ThrottleRequests
). It can be used to limit API requests to prevent abuse, DOS attacks, or excessive usage of resources.
40. How can you optimize a Laravel application for better performance?
Answer:
Some strategies include:
- Using eager loading instead of lazy loading.
- Implementing caching for configurations, queries, and routes.
- Optimizing database queries and indexes.
- Using queue workers for time-consuming tasks.
41. How would you test routes in Laravel?
Answer:
Using Laravel’s TestResponse
class, routes can be tested with methods like get()
, post()
, and assertions like assertStatus()
, assertSee()
, or assertRedirect()
.
42. What is Laravel Nova and how does it differ from Laravel Admin?
Answer:
Nova is a premium admin panel package for Laravel applications that provides a sleek, customizable interface for managing resources and models. It differs from other admin panels due to its ease of integration, advanced metrics, and resource tools.
43. Explain how to use API Resource collections in Laravel.
Answer:
Laravel Resources transform models and collections into JSON responses. Resource collections wrap a collection of models, providing a standardized JSON structure.
44. How do you handle database transactions in Laravel?
Answer:
Database transactions ensure that a series of database operations either fully succeed or fully fail. You can use DB::beginTransaction()
, DB::commit()
, and DB::rollBack()
to manage transactions manually.
45. Explain how the policy
and gate
authorization mechanisms work in Laravel.
Answer:
Policies are used for authorizing actions on specific models. Gates provide a simple closure-based authorization that is not tied to any model. Both help control user access to actions in the application.
46. How does the Laravel Scheduler work, and what are its advantages?
Answer:
The Laravel Scheduler allows you to define and manage task execution at specific intervals via a single cron entry. It offers a more expressive way of defining tasks compared to raw cron jobs.
47. How does Laravel’s Passport
package facilitate API authentication?
Answer:
Passport
is used to create OAuth2 servers for API authentication. It offers a full OAuth2 implementation, including access tokens, refresh tokens, and personal access clients.
48. What are Traits in Laravel, and when should they be used?
Answer:
Traits in Laravel allow you to reuse methods across different classes without inheritance. They are useful for sharing logic between models or controllers, like handling user authentication logic.
49. How does Laravel prevent SQL Injection?
Answer:
Laravel uses PDO (PHP Data Objects), which performs automatic parameter binding in queries. This prevents SQL injection attacks by escaping inputs when constructing SQL statements.
50. What are the benefits of using Laravel Horizon for managing queues?
Answer:
Laravel Horizon provides a real-time dashboard to monitor queue metrics, job throughput, and failure rates. It simplifies queue management and job prioritization.
51. How does Laravel’s Localization feature work?
Answer:
Localization helps in translating the application into different languages. Laravel uses lang
files where translations can be stored and retrieved via the trans()
function or the __()
helper.
52. What are Laravel’s custom casts and when would you use them?
Answer:
Custom casts transform how attributes are stored and retrieved from the database. You can define your own logic for attribute casting by creating a custom cast class that implements the CastsAttributes
interface.
53. Explain how to implement task scheduling using Laravel Task Scheduler.
Answer:
Laravel’s Task Scheduler allows scheduling Artisan commands to run periodically without creating Cron entries for each task. You define them in the app/Console/Kernel.php
file’s schedule
method.
54. How do you implement multi-tenancy in Laravel?
Answer:
Multi-tenancy in Laravel can be implemented via database partitioning (separate databases or tables for tenants), or via a shared schema with a tenant identifier. Tools like tenancy
or Stancl/tenancy
simplify this process.
55. What is a Job Chain in Laravel, and how do you use it?
Answer:
A job chain in Laravel allows you to dispatch multiple jobs in a specific sequence, where each job waits for the previous one to complete before starting. This ensures that jobs are executed in a strict order.
56. How do you use Laravel’s broadcast
helper to send notifications in real time?
Answer:
The broadcast
helper is used to broadcast events to external services like Pusher, allowing real-time notifications. You can define broadcast events that clients can listen to using Laravel Echo.
57. Explain Laravel’s rate limiting using Redis.
Answer:
Redis can be used as the backing store for Laravel’s rate limiter, storing the number of requests allowed per minute or hour. Redis’ efficiency makes it ideal for high-volume applications.
Learn More: Carrer Guidance [Laravel interview questions and answers]
Data analyst interview questions and answers
HTML CSS Interview Questions and Answers
API Testing Interview Questions and Answers
Salesforce Integration Interview Questions and Answers for fresher
Salesforce integration interview questions and answers for experienced