Are you preparing for a Kotlin interview? To help you out, we have compiled the top 40 commonly asked Kotlin interview questions and detailed answers. From understanding Kotlin’s key features to mastering advanced concepts like null safety, coroutines, and data classes, this resource covers all the essential topics.
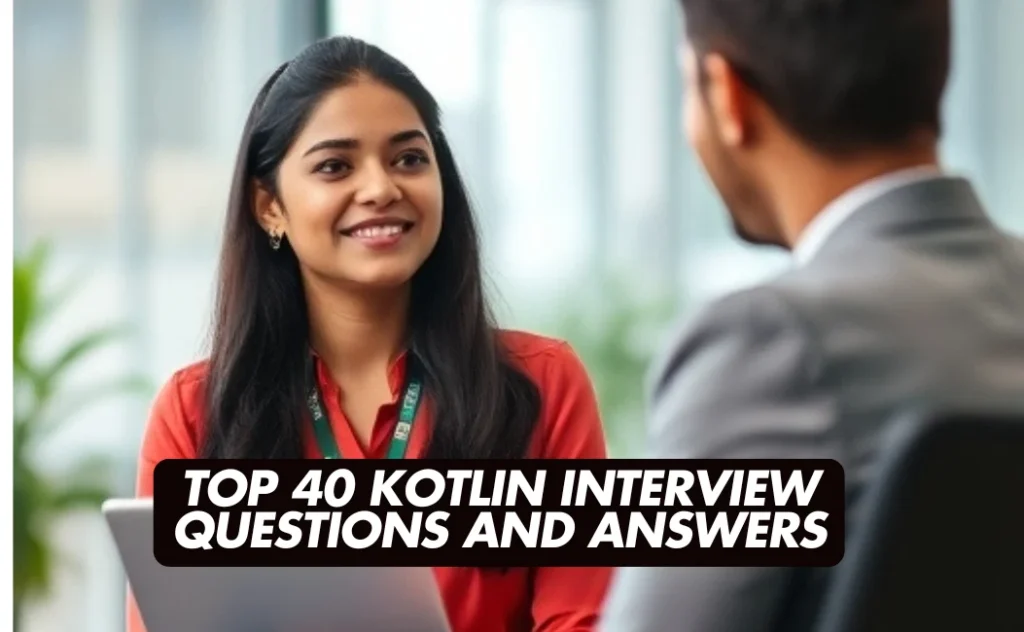
Top 40 Kotlin Interview Questions and Answers
- What is Kotlin, and what are its key features?
- What is the difference between
val
andvar
in Kotlin? - How does Kotlin handle null safety?
- What are extension functions in Kotlin?
- What is a data class in Kotlin, and when would you use it?
- How does Kotlin achieve interoperability with Java?
- What are coroutines in Kotlin, and how do they simplify asynchronous programming?
- What is the purpose of the
companion object
in Kotlin? - What are higher-order functions in Kotlin?
- How does Kotlin’s
when
expression differ from Java’sswitch
statement? - What are sealed classes in Kotlin, and when should you use them?
- How does Kotlin’s type inference work?
- What is the difference between
==
and===
in Kotlin? - How do you handle exceptions in Kotlin?
- What are inline functions in Kotlin, and why are they used?
- How does Kotlin’s
lazy
initialization work? - What is the purpose of the
lateinit
modifier in Kotlin? - What is the difference between
lateinit
andlazy
in Kotlin? - How does Kotlin’s
apply
function work, and when should you use it? - What are coroutines in Kotlin, and how do they simplify asynchronous programming?
- What is operator overloading in Kotlin, and how is it implemented?
- How does Kotlin handle null safety, and what are the key operators involved?
- What are extension functions in Kotlin, and how do you create them?
- How does Kotlin handle null safety, and what are nullable types?
- What is the difference between
val
andvar
in Kotlin? - How does Kotlin’s data class differ from a regular class?
- What is the purpose of the by keyword in Kotlin?
- How does Kotlin’s object keyword differ from class?
- What is the purpose of the sealed keyword in Kotlin?
- How does Kotlin’s inline function work, and when should you use it?
- What is the difference between const val and val in Kotlin?
- How does Kotlin achieve interoperability with Java?
- What are higher-order functions in Kotlin, and how are they used?
- Explain the concept of smart casts in Kotlin.
- How does Kotlin handle checked exceptions compared to Java?
- What is the purpose of the in and out keywords in Kotlin generics?
- How do you create a singleton in Kotlin?
- What are coroutines in Kotlin, and how do they simplify asynchronous programming?
- Explain the use of the lateinit modifier in Kotlin.
- How does Kotlin’s when expression differ from Java’s switch statement?
1. What is Kotlin, and what are its key features?
Kotlin is a statically typed, cross-platform programming language developed by JetBrains. It is fully interoperable with Java and is widely used for Android development. Key features of Kotlin include:
- Conciseness: Reduces boilerplate code compared to Java.
- Null Safety: Designed to eliminate null pointer exceptions by incorporating null safety into its type system.
- Interoperability: Seamlessly integrates with Java, allowing the use of existing Java libraries and frameworks.
- Coroutines: Provides support for coroutines, simplifying asynchronous programming.
- Extension Functions: Allows adding new functions to existing classes without modifying their source code.
- Smart Casts: Automatically handles type casting, reducing the need for explicit casts.
2. What is the difference between val
and var
in Kotlin?
In Kotlin, val
and var
are used to declare variables:
val
: Declares a read-only (immutable) variable. Once assigned, its value cannot be changed.
val name = "Alice"
// name = "Bob" // Error: Val cannot be reassigned
var
: Declares a mutable variable. Its value can be changed after assignment.
var age = 30
age = 31 // Allowed
3. How does Kotlin handle null safety?
Kotlin’s type system differentiates between nullable and non-nullable types to prevent null pointer exceptions:
- Non-nullable types: Cannot hold a null value.
var name: String = "Alice"
// name = null // Error: Null can not be a value of a non-null type String
- Nullable types: Can hold a null value, denoted by adding a
?
to the type.
var name: String? = "Alice"
name = null // Allowed
To access nullable variables safely, Kotlin provides:
- Safe call operator (
?.
): Executes an operation only if the variable is not null.
val length = name?.length // Returns length if name is not null, otherwise null
- Elvis operator (
?:
): Provides a default value if the expression on the left is null.
val length = name?.length ?: 0 // Returns length if name is not null, otherwise 0
4. What are extension functions in Kotlin?
Extension functions allow adding new functions to existing classes without modifying their source code. This enhances the functionality of classes in a clean and modular way.
fun String.greet() {
println("Hello, $this!")
}
fun main() {
"World".greet() // Output: Hello, World!
}
In this example, greet
is an extension function for the String
class. It can be called on any String
instance.
5. What is a data class in Kotlin, and when would you use it?
A data class in Kotlin is a class primarily used to hold data. The compiler automatically generates standard functions like equals()
, hashCode()
, toString()
, and copy()
based on the properties declared in the primary constructor.
data class User(val name: String, val age: Int)
fun main() {
val user1 = User("Alice", 30)
println(user1) // Output: User(name=Alice, age=30)
}
Data classes are ideal for creating classes that are meant to store state or value objects without requiring explicit implementation of utility methods.
6. How does Kotlin achieve interoperability with Java?
Kotlin is fully interoperable with Java, meaning you can call Java code from Kotlin and vice versa. This interoperability is achieved because both Kotlin and Java compile to JVM bytecode. Kotlin’s compiler ensures that Kotlin features are translated into Java-compatible code, allowing seamless integration between the two languages.
// Kotlin code
fun greet() {
println("Hello from Kotlin")
}
// Java code
public class Main {
public static void main(String[] args) {
MainKt.greet(); // Calling Kotlin function from Java
}
}
In this example, the Kotlin function greet
is called from Java code without any issues.
7. What are coroutines in Kotlin, and how do they simplify asynchronous programming?
Coroutines are a feature in Kotlin that provide a simplified approach to asynchronous programming. They allow writing asynchronous code in a sequential manner, making it more readable and maintainable. Coroutines are lightweight and can be suspended and resumed without blocking threads.
import kotlinx.coroutines.*
fun main() = runBlocking {
launch {
delay(1000L)
println("World!")
}
println("Hello,")
}
In this example, launch
starts a new coroutine that delays for 1 second before printing “World!”. The runBlocking
block waits for the coroutine to complete. This allows writing asynchronous code that looks synchronous, simplifying complex asynchronous operations.
8. What is the purpose of the companion object
in Kotlin?
In Kotlin, a companion object
is a special object declared within a class using the companion
keyword. It allows you to define members that are tied to the class itself rather than to instances of the class, effectively providing a way to create static-like members. This means you can access the members of the companion object without creating an instance of the class.
Key purposes of companion objects include:
- Defining Factory Methods: Companion objects can be used to implement the Factory Design Pattern, providing methods to create instances of the class.
class MyClass private constructor(val name: String) {
companion object Factory {
fun create(name: String): MyClass {
return MyClass(name)
}
}
}
fun main() {
val instance = MyClass.create("Kotlin")
println(instance.name) // Output: Kotlin
}
- Accessing Private Members: Companion objects can access private members (properties and functions) of their containing class, allowing for encapsulation and controlled access.
class MyClass private constructor(val name: String) {
companion object {
fun create(name: String): MyClass {
return MyClass(name)
}
}
}
fun main() {
val instance = MyClass.create("Kotlin")
println(instance.name) // Output: Kotlin
}
- Implementing Interfaces: Companion objects can implement interfaces, allowing the class to provide a singleton implementation of an interface.
interface Factory<T> {
fun create(): T
}
class MyClass {
companion object : Factory<MyClass> {
override fun create(): MyClass {
return MyClass()
}
}
}
fun main() {
val instance = MyClass.create()
}
- Java Interoperability: When working with Java code, companion object members can be annotated with
@JvmStatic
to expose them as true static members, enhancing interoperability.
class MyClass {
companion object {
@JvmStatic
fun staticMethod() {
println("Called from Java")
}
}
}
In Java, you can call this method as:
public class Main {
public static void main(String[] args) {
MyClass.staticMethod();
}
}
In summary, companion objects in Kotlin serve as a powerful tool to define class-level functionality, similar to static members in Java, but with additional capabilities such as implementing interfaces and accessing private class members. They promote better organization and encapsulation of code within classes.
9. What are higher-order functions in Kotlin?
In Kotlin, higher-order functions are functions that can take other functions as parameters or return functions as results. This feature allows for more flexible and reusable code, enabling functional programming paradigms.
Example of a higher-order function:
fun <T> List<T>.customFilter(predicate: (T) -> Boolean): List<T> {
val result = mutableListOf<T>()
for (item in this) {
if (predicate(item)) {
result.add(item)
}
}
return result
}
fun main() {
val numbers = listOf(1, 2, 3, 4, 5)
val evenNumbers = numbers.customFilter { it % 2 == 0 }
println(evenNumbers) // Output: [2, 4]
}
In this example, customFilter
is a higher-order function that takes a predicate function as a parameter. This predicate defines the condition to filter the list. The customFilter
function iterates over the list and applies the predicate to each item, collecting those that match the condition.
Higher-order functions are fundamental in Kotlin for implementing functional programming techniques, enabling operations like mapping, filtering, and reducing collections in a concise and expressive manner.
10. How does Kotlin’s when
expression differ from Java’s switch
statement?
Kotlin’s when
expression is a powerful construct that serves a similar purpose to Java’s switch
statement but with enhanced capabilities and flexibility.
Key differences:
- Expression vs. Statement: In Kotlin,
when
is an expression, meaning it returns a value. In contrast, Java’sswitch
is a statement and does not return a value.
val result = when (value) {
1 -> "One"
2 -> "Two"
else -> "Other"
}
- No Fall-Through: Kotlin’s
when
does not have fall-through behavior, eliminating the need for explicitbreak
statements to prevent unintended code execution.
when (value) {
1 -> println("One")
2 -> println("Two")
else -> println("Other")
}
- Multiple Conditions: Kotlin allows multiple conditions to be handled in a single branch using commas.
when (value) {
0, 1 -> println("Zero or One")
else -> println("Other")
}
- Arbitrary Expressions:
when
can evaluate arbitrary expressions, not just constants, providing greater flexibility.
when {
value.isOdd() -> println("Odd")
value.isEven() -> println("Even")
else -> println("Unknown")
}
- Smart Casting: Kotlin’s
when
works seamlessly with smart casting, allowing for type checks and automatic casting within branches.
when (val obj = getObject()) {
is String -> println("String of length ${obj.length}")
is Int -> println("Integer value")
else -> println("Unknown type")
}
These features make Kotlin’s when
expression more concise, expressive, and less error-prone.
11. What are sealed classes in Kotlin, and when should you use them?
Sealed classes in Kotlin are used to represent restricted class hierarchies, where a value can only be of one of the specified types. They are particularly useful in scenarios where you have a known set of subclasses and want to enforce type safety.
Key characteristics of sealed classes:
- Restricted Hierarchy: All subclasses of a sealed class must be defined within the same file.
- Exhaustive
when
Expressions: When using sealed classes in awhen
expression, all possible subclasses can be covered without requiring anelse
branch, enhancing code safety.
Example:
sealed class Result {
data class Success(val data: String) : Result()
data class Error(val exception: Throwable) : Result()
object Loading : Result()
}
fun handleResult(result: Result) {
when (result) {
is Result.Success -> println("Data: ${result.data}")
is Result.Error -> println("Error: ${result.exception.message}")
Result.Loading -> println("Loading...")
}
}
In this example, Result
is a sealed class with three possible types: Success
, Error
, and Loading
. The when
expression in handleResult
covers all possible cases, ensuring comprehensive handling of each state.
12. How does Kotlin’s type inference work?
Kotlin’s type inference allows the compiler to automatically deduce the type of a variable or expression based on its context, reducing the need for explicit type declarations and leading to more concise code.
Examples:
- Variable Declaration:
val number = 10 // Compiler infers 'Int' type
val text = "Hello" // Compiler infers 'String' type
- Function Return Type:
fun add(a: Int, b: Int) = a + b
// Compiler infers return type as 'Int'
While type inference simplifies code, explicit type declarations can enhance readability and are necessary for public APIs to ensure clarity.
13. What is the difference between ==
and ===
in Kotlin?
In Kotlin, ==
and ===
are used to compare objects, but they serve different purposes:
==
(Structural Equality): Checks if two objects have the same value. It translates to theequals()
method.
val a = "Kotlin"
val b = "Kotlin"
println(a == b) // Output: true
===
(Referential Equality): Checks if two references point to the same object instance.
val a = "Kotlin"
val b = "Kotlin"
println(a === b) // Output: true or false, depending on JVM string interning
Understanding the distinction between structural and referential equality is crucial for correctly comparing objects in Kotlin.
14. How do you handle exceptions in Kotlin?
Kotlin handles exceptions similarly to Java but with some differences:
- Try-Catch Block: Used to catch exceptions.
try {
// Code that may throw an exception
} catch (e: IOException) {
// Handle IOException
} catch (e: Exception) {
// Handle other exceptions
} finally {
// Optional: Execute code regardless of exception
}
- No Checked Exceptions: Kotlin does not have checked exceptions; it doesn’t force you to catch or declare exceptions, leading to cleaner code.
Nothing
Type: Functions that always throw exceptions can have a return type ofNothing
, indicating that the function never returns.
fun fail(message: String): Nothing {
throw IllegalArgumentException(message)
}
Kotlin’s streamlined exception handling allows for more concise and readable error management.
15. What are inline functions in Kotlin, and why are they used?
Inline functions in Kotlin are functions marked with the inline
keyword, suggesting to the compiler to insert the function’s body directly into the call site. This can improve performance by eliminating the overhead of function calls, especially for higher-order functions.
Usage:
- Performance Optimization: Particularly beneficial for functions that take other functions as parameters (higher-order functions), reducing the overhead of lambda allocations.
inline fun performOperation(operation: () -> Unit) {
// Function body
operation()
}
- Non-Local Returns: Allows returning from the calling function within a lambda.
inline fun foo(action: () -> Unit) {
action()
}
fun main() {
foo {
println("Before return")
return // Returns from main function
}
println("This will not be printed")
}
While inline functions can enhance performance, they should be used judiciously, as excessive inlining can lead to code bloat.
16. How does Kotlin’s lazy
initialization work?
Kotlin’s lazy
initialization is a feature that allows the initialization of a property to be deferred until it is first accessed. This is particularly useful for properties that require significant resources to initialize or are not always needed.
Usage:
- Declaration:
val lazyValue: String by lazy {
println("Computed!")
"Hello"
}
- Access:
fun main() {
println(lazyValue) // Output: Computed! Hello
println(lazyValue) // Output: Hello
}
In this example, the lazyValue
is initialized only upon its first access, and subsequent accesses return the cached result without recomputation.
17. What is the purpose of the lateinit
modifier in Kotlin?
In Kotlin, the lateinit
modifier allows you to declare a non-nullable var
property without an initial value, with the expectation that it will be initialized before its first use. This is particularly useful in scenarios where immediate initialization is not feasible, such as dependency injection, unit testing, or Android view binding.
Key Points:
- Declaration: Apply
lateinit
to a mutable property (var
) with a non-nullable type. It cannot be used with immutable properties (val
) or primitive types.
lateinit var example: String
- Initialization: Ensure the property is assigned a value before accessing it to avoid an
UninitializedPropertyAccessException
.
example = "Initialized Value"
println(example) // Output: Initialized Value
- Checking Initialization: Use the
::propertyName.isInitialized
property to verify if alateinit
variable has been initialized before accessing it.
if (::example.isInitialized) {
println(example)
} else {
println("Property not initialized")
}
Use Cases:
- Dependency Injection: In frameworks where dependencies are injected after object creation,
lateinit
allows for non-nullable property declarations without immediate initialization.
class Service {
lateinit var repository: Repository
fun initialize(repo: Repository) {
repository = repo
}
}
- Android Development: When dealing with view binding in Android activities or fragments,
lateinit
enables the declaration of view properties that are initialized in lifecycle methods likeonCreate
oronViewCreated
.
class MainActivity : AppCompatActivity() {
private lateinit var binding: ActivityMainBinding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityMainBinding.inflate(layoutInflater)
setContentView(binding.root)
}
}
Considerations:
- Initialization Responsibility: It’s the developer’s responsibility to ensure that
lateinit
properties are initialized before use to prevent runtime exceptions. - Non-Nullable Types:
lateinit
is designed for non-nullable types. For nullable types or properties that require lazy initialization with thread safety, consider using thelazy
delegate.
By using lateinit
, you can declare non-nullable properties without immediate initialization, leading to cleaner and more concise code, especially in scenarios where properties cannot be initialized at the point of declaration.
18. What is the difference between lateinit
and lazy
in Kotlin?
In Kotlin, both lateinit
and lazy
are used for deferred initialization of properties, but they serve different purposes and have distinct characteristics.
lateinit
:
- Usage: Applicable only to
var
properties with non-nullable types. - Initialization: The property must be initialized before its first use; otherwise, accessing it will throw an
UninitializedPropertyAccessException
. - Thread Safety: Not inherently thread-safe; synchronization must be managed manually if needed.
- Common Use Cases: Dependency injection, Android view binding, and unit testing where properties are initialized after object creation.
lateinit var example: String
fun initialize() {
example = "Initialized"
}
fun use() {
println(example)
}
lazy
:
- Usage: Applicable only to
val
properties. - Initialization: The property is initialized upon first access, and the initialization lambda is executed only once.
- Thread Safety: By default,
lazy
initialization is thread-safe and ensures that the property is initialized in a thread-safe manner. - Common Use Cases: Properties that require lazy initialization, especially when the initialization is resource-intensive or should be deferred until the property is accessed.
val example: String by lazy {
println("Initializing")
"Initialized"
}
fun use() {
println(example)
}
Key Differences:
- Mutability:
lateinit
is used with mutablevar
properties, whilelazy
is used with immutableval
properties. - Initialization Timing:
lateinit
properties must be explicitly initialized before use, whereaslazy
properties are initialized automatically upon first access. - Null Safety:
lateinit
is for non-nullable types and does not handle nullability, whilelazy
can work with nullable types if specified. - Thread Safety:
lazy
provides built-in thread safety by default, whereaslateinit
does not, requiring manual handling if needed.
Choosing Between lateinit
and lazy
:
- Use
lateinit
when:- You have a non-nullable
var
property that cannot be initialized at the time of object creation. - You are certain that the property will be initialized before any access.
- You need to reassign the property after its initial assignment.
- You have a non-nullable
- Use
lazy
when:- You have an immutable
val
property that should be initialized upon first access. - The initialization is resource-intensive, and you want to defer it until necessary.
- You require thread-safe initialization without additional synchronization.
- You have an immutable
Understanding the differences between lateinit
and lazy
helps in choosing the appropriate strategy for property initialization based on the specific requirements of your application.
19. How does Kotlin’s apply
function work, and when should you use it?
In Kotlin, the apply
function is a scope function that allows you to configure an object within a block and returns the object itself. This is particularly useful for initializing or configuring objects in a concise and readable manner.
Syntax:
val result = object.apply {
// Configuration
}
Key Characteristics:
- Context Object: Within the
apply
block, the context object is referenced asthis
, allowing direct access to its members without explicit qualification. - Return Value: The
apply
function returns the original object after executing the block, facilitating method chaining.
Example:
data class Person(var name: String, var age: Int, var city: String)
val person = Person("John", 30, "New York").apply {
age = 31
city = "San Francisco"
}
println(person) // Output: Person(name=John, age=31, city=San Francisco)
In this example, the apply
function is used to update the age
and city
properties of the Person
object. The object is configured within the block, and the modified object is returned and assigned to person
.
Use Cases:
- Object Initialization:
apply
is commonly used for initializing objects where multiple properties need to be set.
val builder = StringBuilder().apply {
append("Hello")
append(" ")
append("World")
}
println(builder.toString()) // Output: Hello World
- DSL Construction: In domain-specific languages (DSLs),
apply
facilitates building complex objects in a readable manner.
val person = Person().apply {
name = "Alice"
age = 28
city = "Seattle"
}
When to Use apply
:
- When you need to configure an object and prefer to access its properties and methods directly within a block.
- When you want to perform multiple operations on an object and return the object itself for further use.
- When constructing objects in a builder pattern or initializing objects with multiple properties.
By using apply
, you can streamline object configuration, leading to more concise and readable code, especially when setting multiple properties or initializing complex objects.
20. What are coroutines in Kotlin, and how do they simplify asynchronous programming?
Coroutines in Kotlin are a feature that enables simple and efficient asynchronous programming. They allow you to write asynchronous code in a sequential and readable manner, making it easier to manage tasks such as network calls, file I/O, or any operations that would otherwise block the main thread.
Key Features of Coroutines:
- Lightweight: Coroutines are much lighter than threads; you can run thousands of coroutines without significant overhead.
- Suspension: Coroutines can suspend their execution at a certain point without blocking the thread, allowing other coroutines to run.
- Structured Concurrency: Kotlin provides structured concurrency, ensuring that coroutines are launched in a specific scope, making it easier to manage their lifecycle.
Example:
import kotlinx.coroutines.*
fun main() = runBlocking {
launch {
delay(1000L)
println("World!")
}
println("Hello,")
}
In this example, runBlocking
creates a coroutine that blocks the main thread until its execution completes. Within this scope, launch
starts a new coroutine that delays for 1 second before printing “World!”. Meanwhile, “Hello,” is printed immediately. The output will be:
Hello,
World!
Benefits of Using Coroutines:
- Simplified Code: Coroutines allow you to write asynchronous code that looks and behaves like synchronous code, reducing complexity.
- Cancellation Support: Coroutines can be easily canceled, allowing for responsive and controllable asynchronous operations.
- Timeout Handling: Kotlin coroutines provide built-in support for handling timeouts, making it easier to manage long-running tasks.
Use Cases:
- Network Operations: Performing network requests without blocking the main thread.
- Concurrency: Running multiple tasks concurrently, such as parallel processing or handling multiple user inputs.
- Background Processing: Executing background tasks like database operations or file I/O.
By leveraging coroutines, Kotlin developers can write efficient, readable, and maintainable asynchronous code, enhancing application performance and responsiveness.
21. What is operator overloading in Kotlin, and how is it implemented?
Operator overloading in Kotlin allows developers to provide custom implementations for predefined operators, enabling them to be used with user-defined types. This feature enhances code readability and allows for intuitive operations on custom objects.
Implementation:
To overload an operator, define a function with a specific name corresponding to the operator and mark it with the operator
keyword.
Example:
data class Vector(val x: Int, val y: Int) {
operator fun plus(other: Vector): Vector {
return Vector(x + other.x, y + other.y)
}
}
fun main() {
val v1 = Vector(1, 2)
val v2 = Vector(3, 4)
val v3 = v1 + v2
println(v3) // Output: Vector(x=4, y=6)
}
In this example, the plus
function is overloaded to allow the +
operator to add two Vector
instances.
Common Operators and Their Corresponding Functions:
+
->plus
-
->minus
*
->times
/
->div
%
->rem
==
->equals
[]
->get
andset
()
->invoke
Considerations:
- Operator overloading should be used judiciously to maintain code clarity.
- Ensure that the overloaded operators perform intuitive and expected operations to avoid confusion.
By leveraging operator overloading, Kotlin allows for more expressive and natural syntax when working with custom types.
22. How does Kotlin handle null safety, and what are the key operators involved?
Kotlin’s type system is designed to eliminate the danger of null references, commonly known as the Billion Dollar Mistake. By distinguishing between nullable and non-nullable types, Kotlin ensures that null-related errors are caught at compile time.
Key Features and Operators:
- Nullable Types (
?
): By appending?
to a type, you indicate that the variable can hold a null value.
val name: String? = null
- Safe Call Operator (
?.
): Allows you to access a property or call a method on a nullable object without risking aNullPointerException
. If the object is null, the expression evaluates to null.
val length = name?.length // length is of type Int?
- Elvis Operator (
?:
): Provides a default value to return when the expression on its left is null.
val length = name?.length ?: 0 // Returns 0 if name is null
- Non-Null Assertion Operator (
!!
): Asserts that a nullable variable is not null, throwing aNullPointerException
if it is. Use this operator cautiously.
val length = name!!.length // Throws NullPointerException if name is null
- Safe Cast Operator (
as?
): Attempts to cast a value to a specified type, returning null if the cast is unsuccessful.
val obj: Any = "Kotlin"
val str: String? = obj as? String // str is "Kotlin"
Example:
fun printNameLength(name: String?) {
val length = name?.length ?: "Unknown"
println("Name length: $length")
}
fun main() {
printNameLength("Kotlin") // Output: Name length: 6
printNameLength(null) // Output: Name length: Unknown
}
In this example, the safe call operator and Elvis operator are used to handle the nullable name
parameter gracefully.
By incorporating these null safety features, Kotlin significantly reduces the likelihood of runtime null pointer exceptions, leading to more robust and reliable code.
23. What are extension functions in Kotlin, and how do you create them?
Extension functions in Kotlin allow you to add new functions to existing classes without modifying their source code or inheriting from them. This feature enables you to enhance the functionality of classes from third-party libraries or standard libraries in a clean and concise manner.
Creating an Extension Function:
To define an extension function, prefix the function name with the type you want to extend.
Syntax:
fun ClassName.functionName(parameters): ReturnType {
// function body
}
Example:
// Extension function to reverse a string
fun String.reverseText(): String {
return this.reversed()
}
fun main() {
val original = "Kotlin"
val reversed = original.reverseText()
println(reversed) // Output: niltoK
}
In this example, reverseText
is an extension function for the String
class that returns the reversed string. You can call reverseText()
on any String
instance as if it were a member function of the String
class.
Key Points:
- No Actual Modification: Extension functions do not modify the original class; they are resolved statically at compile time. This means that the function is not actually added to the class but is available for use as if it were a member function.
- Access to Public Members: Within an extension function, you can access the public members of the class you’re extending.
- Nullable Receivers: You can define extension functions on nullable types, allowing you to call the function on a nullable receiver without additional null checks.
fun String?.isNullOrEmpty(): Boolean {
return this == null || this.isEmpty()
}
fun main() {
val str: String? = null
println(str.isNullOrEmpty()) // Output: true
}
Use Cases:
- Enhancing Libraries: Add utility functions to classes from libraries without modifying their source code.
- Improving Readability: Create domain-specific functions that make the code more expressive and easier to read.
- Organizing Code: Group related functions together, even if they operate on different types, without the need for utility classes.
Extension functions are a powerful feature in Kotlin that promote cleaner and more maintainable code by allowing you to extend existing classes with new functionality seamlessly.
24. How does Kotlin handle null safety, and what are nullable types?
Kotlin addresses null safety by incorporating nullable and non-nullable types into its type system, thereby reducing the risk of NullPointerException
(NPE) occurrences.
Nullable and Non-Nullable Types:
- Non-Nullable Types: By default, variables in Kotlin cannot hold a null value.
var nonNullable: String = "Kotlin"
nonNullable = null // Compile-time error
- Nullable Types: To allow a variable to hold a null value, append a question mark
?
to its type.
var nullable: String? = "Kotlin"
nullable = null // Allowed
Safe Calls (?.
):
The safe call operator ?.
allows you to access properties or call methods on a nullable object without risking an NPE. If the object is null, the expression evaluates to null.
val length: Int? = nullable?.length
Elvis Operator (?:
):
The Elvis operator ?:
provides a default value when an expression evaluates to null.
val length: Int = nullable?.length ?: 0
Not-Null Assertion (!!
):
The not-null assertion operator !!
converts a nullable type to a non-nullable type, throwing an NPE if the value is null. Use it cautiously.
val length: Int = nullable!!.length // Throws NullPointerException if nullable is null
Safe Casting (as?
):
The safe cast operator as?
attempts to cast a value to a specified type, returning null if the cast is unsuccessful.
val obj: Any = "Kotlin"
val str: String? = obj as? String // str is "Kotlin"
Benefits of Kotlin’s Null Safety:
- Compile-Time Checks: Many potential null reference errors are caught at compile time, reducing runtime crashes.
- Explicit Nullability: Developers must explicitly declare when a variable can be null, leading to more predictable and robust code.
By integrating null safety into its type system, Kotlin helps developers write safer and more reliable code, minimizing the common pitfalls associated with null references.
25. What is the difference between val
and var
in Kotlin?
In Kotlin, val
and var
are used to declare variables, but they differ in mutability:
val
(Immutable Reference): Defines a read-only variable whose reference cannot be reassigned after initialization. However, if the variable refers to a mutable object, the object’s internal state can still be modified.
val immutableList = mutableListOf(1, 2, 3)
immutableList.add(4) // Allowed, as the list is mutable
// immutableList = mutableListOf(5, 6) // Compile-time error: Val cannot be reassigned
var
(Mutable Reference): Defines a mutable variable whose reference can be reassigned to a different value.
var mutableString = "Hello"
mutableString = "World" // Allowed
Key Differences:
- Mutability:
val
is immutable (cannot be reassigned), whilevar
is mutable (can be reassigned). - Use Cases: Use
val
for variables that should not change after initialization, promoting immutability and thread safety. Usevar
for variables that need to change values during their lifecycle.
Understanding the distinction between val
and var
is crucial for writing clear and maintainable Kotlin code.
26. How does Kotlin’s data class
differ from a regular class?
In Kotlin, data class
is a special type of class designed to hold data. It automatically provides several standard functionalities, reducing boilerplate code.
Features of data class
:
- Automatic
equals()
andhashCode()
: Generatesequals()
andhashCode()
methods based on the primary constructor properties. toString()
Implementation: Provides a readabletoString()
method that includes the class name and its properties.copy()
Function: Enables creating a copy of the object with optional property modifications.- Component Functions: Generates
componentN()
functions corresponding to the properties, facilitating destructuring declarations.
Example:
data class User(val name: String, val age: Int)
fun main() {
val user1 = User("Alice", 30)
val user2 = user1.copy(age = 31)
println(user1) // Output: User(name=Alice, age=30)
println(user2) // Output: User(name=Alice, age=31)
}
In contrast, a regular class does not provide these functionalities automatically, requiring manual implementation if needed.
Use Cases for data class
:
- Representing simple data structures, such as entities, DTOs, or value objects.
- Situations where value-based equality is essential.
By using data class
, Kotlin developers can create concise and expressive data-holding classes with minimal boilerplate.
27. What is the purpose of the by
keyword in Kotlin?
In Kotlin, the by
keyword is used for delegation, allowing a class to delegate functionality to another object. This is particularly useful for implementing interfaces or extending class behavior without inheritance.
Types of Delegation Using by
:
- Property Delegation: Delegates the getter and setter of a property to another object.
import kotlin.properties.Delegates
var observedProperty: String by Delegates.observable("Initial Value") { _, old, new ->
println("Changed from $old to $new")
}
- Class Delegation: A class can delegate the implementation of an interface to another object.
interface Printer {
fun print()
}
class PrinterImpl(val text: String) : Printer {
override fun print() = println(text)
}
class Document(printer: Printer) : Printer by printer
fun main() {
val doc = Document(PrinterImpl("Hello, World!"))
doc.print() // Output: Hello, World!
}
Benefits of Using by
for Delegation:
- Code Reusability: Promotes composition over inheritance, allowing reuse of existing implementations.
- Separation of Concerns: Enables separation of responsibilities by delegating specific functionalities.
- Flexibility: Provides a flexible mechanism to extend class behavior without modifying existing code.
By utilizing the by
keyword, Kotlin offers a powerful delegation mechanism that enhances code modularity and maintainability.
28. How does Kotlin’s object
keyword differ from class
?
In Kotlin, the object
keyword is used to declare a singleton, which is a class with a single instance. This contrasts with the class
keyword, which defines a blueprint for creating multiple instances.
Key Differences:
- Singleton Declaration: The
object
keyword creates a singleton instance that is initialized lazily and thread-safe.
object DatabaseConnection {
fun connect() = println("Connected to database")
}
fun main() {
DatabaseConnection.connect() // Output: Connected to database
}
- Companion Objects: Within a class, the
object
keyword can define a companion object, providing a place for factory methods and static-like members.
class MyClass {
companion object {
fun create(): MyClass = MyClass()
}
}
fun main() {
val instance = MyClass.create()
}
- Anonymous Objects: The
object
keyword can create anonymous objects, often used for implementing interfaces or abstract classes on the fly.
interface ClickListener {
fun onClick()
}
val listener = object : ClickListener {
override fun onClick() = println("Clicked")
}
Use Cases:
- Singletons: Use
object
to create a singleton instance when only one instance of a class is needed throughout the application. - Companion Objects: Use companion objects to define members that belong to the class rather than to any specific instance, similar to static members in Java.
- Anonymous Objects: Use anonymous objects for one-time implementations of interfaces or abstract classes, especially when a class needs to be extended for a specific purpose without creating a separate subclass.
In summary, the object
keyword in Kotlin provides a way to create single instances, companion objects, and anonymous objects, offering flexibility beyond the traditional class-based instantiation.
29. What is the purpose of the sealed
keyword in Kotlin?
In Kotlin, the sealed
keyword is used to define sealed classes, which are classes that restrict the hierarchy to a specific set of subclasses. This means all possible subclasses of a sealed class are known at compile time, providing more control over inheritance and enabling exhaustive when
expressions.
Example:
sealed class Result {
data class Success(val data: String) : Result()
data class Error(val exception: Exception) : Result()
}
fun handleResult(result: Result) {
when (result) {
is Result.Success -> println("Data: ${result.data}")
is Result.Error -> println("Error: ${result.exception.message}")
}
}
In this example, Result
is a sealed class with two subclasses: Success
and Error
. The when
expression in the handleResult
function can handle all possible subclasses without needing an else
branch, ensuring all cases are covered.
Benefits of Sealed Classes:
- Exhaustive
when
Expressions: The compiler can verify that all possible cases are handled in awhen
expression, reducing runtime errors. - Controlled Inheritance: Sealed classes allow you to control the subclassing of a class, ensuring that all subclasses are known and defined within the same file.
- Enhanced Readability: By grouping related subclasses together, sealed classes improve code organization and readability.
Use Cases:
- Representing Restricted Hierarchies: Use sealed classes when you have a fixed set of types that represent a closed hierarchy, such as different states in a state machine or different outcomes of an operation.
- Modeling Algebraic Data Types: Sealed classes are useful for modeling algebraic data types, where a type can have several different but fixed forms.
By using the sealed
keyword, Kotlin provides a powerful tool for creating restricted class hierarchies, enhancing type safety and code clarity.
30. How does Kotlin’s inline
function work, and when should you use it?
In Kotlin, the inline
keyword is used to optimize higher-order functions by reducing the overhead of function calls, especially when functions are passed as parameters. When a function is marked as inline
, the compiler replaces the function call with the actual code of the function during compilation.
Example:
inline fun performOperation(operation: () -> Unit) {
println("Before operation")
operation()
println("After operation")
}
fun main() {
performOperation {
println("Executing operation")
}
}
In this example, the performOperation
function is marked as inline
. During compilation, the compiler will replace the call to performOperation
with its body, inlining the operation
lambda directly into the main
function.
Benefits of Using inline
:
- Performance Improvement: By eliminating the overhead of function calls, inlining can improve performance, particularly in performance-critical code sections.
- Non-Local Returns: Inline functions allow the use of non-local returns, enabling a lambda passed to an inline function to return from the enclosing function.
inline fun inlined(block: () -> Unit) {
block()
}
fun foo() {
inlined {
println("Before return")
return // Returns from foo
}
println("This will not be printed")
}
Considerations:
- Code Size Increase: Excessive use of inlining can lead to code bloat, as the function body is duplicated at each call site. Use inlining judiciously to balance performance and code size.
- Recursive Functions: Inline functions cannot be recursive, as inlining would result in infinite expansion.
- Function References: Passing an inline function as a reference to another function will prevent it from being inlined.
31. What is the difference between const val
and val
in Kotlin?
val
:- Runtime Constant:
val
is used to declare a read-only (immutable) variable whose value is assigned at runtime. Once assigned, it cannot be reassigned. - Usage: Suitable for values that are determined during program execution.
- Example:
- Runtime Constant:
val currentTime = System.currentTimeMillis()
const val
:- Compile-Time Constant:
const val
is used to declare compile-time constants. These must be of primitive types orString
and initialized with a value known at compile time. - Restrictions: Can only be used at the top-level or inside
object
declarations. Not allowed inside classes or functions. - Usage: Ideal for constants like configuration keys, fixed strings, or numeric values.
- Example:
- Compile-Time Constant:
const val MAX_USERS = 100
Key Differences:
- Initialization Time:
const val
is initialized at compile time, whereasval
is initialized at runtime. - Scope:
const val
can only be top-level or inside objects, whileval
can be used anywhere. - Usage Constraints:
const val
is limited to primitive types andString
, whereasval
can hold any type.
32. How does Kotlin achieve interoperability with Java?
Kotlin is designed to be fully interoperable with Java, allowing developers to use Java libraries and frameworks seamlessly within Kotlin projects. Here’s how Kotlin achieves this interoperability:
- Bytecode Compatibility:
- Both Kotlin and Java compile to Java Virtual Machine (JVM) bytecode, ensuring they run on the same platform without issues.
- Seamless Syntax Integration:
- Kotlin can call Java code and vice versa without requiring special adapters or converters.
- Example:
// Java class
public class JavaClass {
public String greet(String name) {
return "Hello, " + name;
}
}
// Kotlin usage
val javaObject = JavaClass()
println(javaObject.greet("Kotlin"))
- Null Safety:
- Kotlin handles Java’s nullability annotations to enforce null safety, reducing
NullPointerException
risks.
- Kotlin handles Java’s nullability annotations to enforce null safety, reducing
- Annotations:
- Kotlin uses annotations like
@JvmOverloads
,@JvmStatic
, and@JvmField
to enhance Java interoperability, allowing better integration and usage of Kotlin features from Java.
- Kotlin uses annotations like
- Default Parameters and Overloads:
- Kotlin’s default parameters can generate overloaded methods in the bytecode, making them accessible from Java which doesn’t support default parameters.
- Extension Functions:
- While Kotlin supports extension functions, they are compiled as static methods, which can be called from Java as regular static methods.
- Data Classes and Other Features:
- Kotlin’s data classes, sealed classes, and other features are compatible with Java, providing enhanced functionality without sacrificing interoperability.
- Tooling Support:
- IDEs like IntelliJ IDEA and Android Studio provide robust support for mixed Java-Kotlin projects, facilitating smooth development experiences.
Benefits:
- Gradual Migration: Projects can migrate from Java to Kotlin incrementally without complete rewrites.
- Library Usage: Kotlin can utilize the vast ecosystem of existing Java libraries and frameworks.
- Developer Flexibility: Teams can leverage the strengths of both languages as needed.
33. What are higher-order functions in Kotlin, and how are they used?
Higher-Order Functions: Higher-order functions are functions that can take other functions as parameters or return functions. This feature allows for more abstract, reusable, and concise code.
Characteristics:
- Function Parameters: They can accept functions as arguments.
- Function Return Types: They can return functions as results.
- Lambda Expressions: Often used with lambda expressions for inline function definitions.
Usage Examples:
- Passing a Function as a Parameter:
// Higher-order function
fun calculate(a: Int, b: Int, operation: (Int, Int) -> Int): Int {
return operation(a, b)
}
// Usage with lambda
val sum = calculate(5, 3) { x, y -> x + y }
println(sum) // Outputs: 8
- Returning a Function:
// Higher-order function returning another function
fun operationFactory(): (Int, Int) -> Int {
return { x, y -> x * y }
}
val multiply = operationFactory()
println(multiply(4, 5)) // Outputs: 20
- Using with Standard Library Functions: Kotlin’s standard library extensively uses higher-order functions like
map
,filter
, andfold
.
val numbers = listOf(1, 2, 3, 4, 5)
val doubled = numbers.map { it * 2 }
println(doubled) // Outputs: [2, 4, 6, 8, 10]
Benefits:
- Code Reusability: Abstract common patterns and behaviors.
- Conciseness: Reduce boilerplate code by using lambda expressions.
- Flexibility: Enable functional programming paradigms within Kotlin.
34. Explain the concept of smart casts in Kotlin.
Smart Casts: Smart casts in Kotlin automatically cast variables to their target types after performing certain type checks (like is
checks), eliminating the need for explicit casting.
How It Works:
- When the compiler can guarantee that a variable has a certain type based on control flow (e.g., after an
is
check), it allows the variable to be treated as that type without explicit casting.
Example:
fun printLength(obj: Any) {
if (obj is String) {
// obj is automatically cast to String in this branch
println(obj.length)
} else {
println("Not a string")
}
}
Under the Hood:
- The Kotlin compiler inserts necessary type casts where safe.
- Ensures type safety without compromising on performance.
Limitations:
- Mutable Variables: If a variable is mutable (
var
) and could be changed between the type check and usage, smart casts are not applied.
var obj: Any = "Hello"
fun getLength(): Int? {
if (obj is String) {
// Cannot smart cast because obj is mutable
return obj.length // Compiler error
}
return null
}
- Complex Control Flow: In cases where the compiler cannot guarantee the type due to complex control flows, smart casts may not be applied.
Benefits:
- Reduced Boilerplate: No need for explicit casting (
as
keyword) after type checks. - Enhanced Readability: Code is cleaner and more concise.
- Safety: Maintains type safety by relying on compiler checks.
35. How does Kotlin handle checked exceptions compared to Java?
Checked Exceptions in Java:
- Java enforces checked exceptions at compile-time, requiring methods to declare them with
throws
and callers to handle them usingtry-catch
blocks or propagate them further. - This can lead to verbose code and potential clutter with exception handling.
Kotlin’s Approach:
- No Checked Exceptions: Kotlin does not have checked exceptions. All exceptions are treated as unchecked, similar to Java’s
RuntimeException
. - Implications:
- Simpler Code: Developers are not forced to handle or declare exceptions, leading to cleaner and more readable code.
- Flexibility: Developers can choose to handle exceptions as needed without compiler enforcement.
Example in Kotlin:
fun readFile(path: String): String {
val file = File(path)
return file.readText() // May throw IOException, but not required to declare
}
fun main() {
try {
val content = readFile("example.txt")
println(content)
} catch (e: IOException) {
println("Error reading file: ${e.message}")
}
}
Handling Exceptions:
- While Kotlin doesn’t enforce handling, it’s still good practice to handle exceptions where appropriate to ensure robust applications.
Reasons for Kotlin’s Design Choice:
- Interoperability: Seamless interoperability with Java, which means Kotlin can work with Java methods that throw checked exceptions without needing to declare them.
- Philosophical Preference: Favoring simplicity and reducing boilerplate code.
Trade-offs:
- Pros:
- Cleaner and less verbose code.
- Greater flexibility in exception handling.
- Cons:
- Potential for unhandled exceptions at runtime.
- Reliance on developer discipline to handle exceptions appropriately.
36. What is the purpose of the in
and out
keywords in Kotlin generics?
The in
and out
keywords in Kotlin are used to define variance in generic types, ensuring type safety while maintaining flexibility. They correspond to contravariance and covariance, respectively.
Covariance (out
):
- Purpose: Allows a generic type to be a subtype of another generic type if the type parameter is a subtype.
- Usage Scenario: When a generic class produces or outputs data of type
T
. - Declaration:
out T
- Example:
interface Producer<out T> {
fun produce(): T
}
val stringProducer: Producer<String> = ...
val anyProducer: Producer<Any> = stringProducer // Valid due to covariance
Contravariance (in
):
- Purpose: Allows a generic type to accept a supertype of the specified type parameter.
- Usage Scenario: When a generic class consumes or takes in data of type
T
. - Declaration:
in T
- Example:
interface Consumer<in T> {
fun consume(item: T)
}
val anyConsumer: Consumer<Any> = ...
val stringConsumer: Consumer<String> = anyConsumer // Valid due to contravariance
Use Cases:
- Read-Only (Producer):
- Use
out
when the generic type is only producing or returning data. - Ensures that you can safely assign a producer of a subtype to a producer of a supertype.
- Example:
- Use
interface Source<out T> {
fun get(): T
}
- Write-Only (Consumer):
- Use
in
when the generic type is only consuming or accepting data. - Ensures that you can safely assign a consumer of a supertype to a consumer of a subtype.
- Example:
- Use
interface Sink<in T> {
fun put(item: T)
}
- Invariant:
- If a generic type can both consume and produce data, it should remain invariant (no
in
orout
). - Example:
- If a generic type can both consume and produce data, it should remain invariant (no
class Box<T>(var content: T)
Benefits:
- Type Safety: Prevents type mismatch errors by enforcing correct usage patterns.
- Flexibility: Allows for more flexible and reusable code by supporting subtype relationships.
Summary:
out
: Covariant – used when the generic type is an output (producer).in
: Contravariant – used when the generic type is an input (consumer).
37. How do you create a singleton in Kotlin?
In Kotlin, creating a singleton is straightforward using the object
declaration. The object
keyword ensures that only one instance of the class exists throughout the application.
Creating a Singleton with object
:
object DatabaseConnection {
init {
// Initialization code, e.g., establishing a connection
println("DatabaseConnection initialized")
}
fun query(sql: String): List<String> {
// Execute query and return results
println("Executing query: $sql")
return listOf("Result1", "Result2")
}
}
Usage:
fun main() {
// Accessing the singleton instance and its methods
DatabaseConnection.query("SELECT * FROM users")
}
Key Features:
- Thread-Safe Initialization:
- The
object
declaration ensures thread-safe lazy initialization, meaning the instance is created when it is first accessed.
- The
- No Need for
getInstance()
:- Unlike Java’s singleton pattern, Kotlin’s
object
provides direct access without the need for explicit getter methods.
- Unlike Java’s singleton pattern, Kotlin’s
- Inheritance and Interfaces:
- Singletons can inherit from classes or implement interfaces.
interface Logger {
fun log(message: String)
}
object ConsoleLogger : Logger {
override fun log(message: String) {
println(message)
}
}
- Companion Objects:
- For singleton-like behavior within a class, Kotlin provides companion objects.
class MyClass {
companion object {
fun create(): MyClass = MyClass()
}
}
val instance = MyClass.create()
Alternative: Using object
with Delegation: For more complex scenarios, such as lazy initialization or dependency injection, you might combine object
declarations with delegation patterns, but for most singleton needs, the object
declaration suffices.
Example with Initialization:
object Logger {
fun log(message: String) {
println("Log: $message")
}
}
fun main() {
Logger.log("Application started")
}
Advantages:
- Simplicity: Minimal boilerplate compared to traditional singleton implementations.
- Safety: Ensures single instance creation with thread safety.
- Clarity: Clear and concise syntax enhances code readability.
38. What are coroutines in Kotlin, and how do they simplify asynchronous programming?
Coroutines: Coroutines are Kotlin’s way of handling asynchronous programming by providing a lightweight, efficient, and straightforward method to perform non-blocking operations. They enable writing asynchronous code in a sequential, readable manner without the complexity typically associated with callbacks or reactive programming.
Key Characteristics:
- Lightweight:
- Coroutines are not bound to any particular thread and can be started in the background without the overhead of traditional threads.
- Thousands of coroutines can run concurrently with minimal memory usage.
- Suspension:
- Coroutines can suspend their execution without blocking the underlying thread, allowing other coroutines to run.
- Suspension points are marked by the
suspend
keyword.
- Structured Concurrency:
- Coroutines are organized hierarchically, ensuring that they are properly managed and canceled when no longer needed.
Simplifying Asynchronous Programming:
- Sequential Code Style:
- Allows writing asynchronous code that looks and behaves like synchronous code, improving readability and maintainability.
suspend fun fetchData(): String {
val data = networkCall() // Suspends without blocking
return processData(data)
}
fun main() = runBlocking {
val result = fetchData()
println(result)
}
- Managing Concurrency:
- Coroutines can easily handle multiple concurrent tasks using constructs like
launch
andasync
.
- Coroutines can easily handle multiple concurrent tasks using constructs like
fun main() = runBlocking {
val job1 = launch { /* Task 1 */ }
val job2 = launch { /* Task 2 */ }
job1.join()
job2.join()
}
- Cancellation and Timeout:
- Coroutines support cooperative cancellation, allowing tasks to be canceled gracefully. Timeouts can be implemented using functions like
withTimeout
.
- Coroutines support cooperative cancellation, allowing tasks to be canceled gracefully. Timeouts can be implemented using functions like
suspend fun doTask() {
withTimeout(1000L) {
// Task that should complete within 1 second
}
}
- Error Handling:
- Structured error handling with
try-catch
blocks works seamlessly within coroutines.
- Structured error handling with
suspend fun safeCall() {
try {
// Potentially failing operation
} catch (e: Exception) {
// Handle exception
}
}
- Integration with Existing APIs:
- Many Kotlin libraries and frameworks support coroutines, allowing smooth integration with existing asynchronous APIs.
Example: Fetching Data Asynchronously:
import kotlinx.coroutines.*
suspend fun fetchData(): String {
delay(1000) // Simulates a long-running task
return "Data fetched"
}
fun main() = runBlocking {
println("Fetching data...")
val result = fetchData()
println(result)
}
Output:
Fetching data...
Data fetched
Benefits:
- Improved Readability: Code flows naturally without deep nesting of callbacks.
- Efficiency: Minimal resource consumption compared to traditional threading.
- Scalability: Easily manage thousands of concurrent operations.
- Maintainability: Easier to reason about and maintain asynchronous code.
Coroutines provide a powerful and efficient framework for handling asynchronous tasks in Kotlin, making it easier to write clean, readable, and maintainable code without sacrificing performance.
39. Explain the use of the lateinit
modifier in Kotlin.
lateinit
Modifier: The lateinit
modifier in Kotlin is used with var
(mutable) properties to indicate that the property will be initialized later, after the object’s construction. It allows developers to defer the initialization of a property without making it nullable.
Key Characteristics:
- Non-Nullable:
lateinit
properties must be non-nullable types. This means you don’t need to declare them as nullable (?
) and can avoid unnecessary null checks.
- Mutable (
var
) Only:lateinit
can only be applied tovar
properties, not toval
(immutable) properties.
- No Initializer:
- Properties marked with
lateinit
do not require an initial value at the point of declaration.
- Properties marked with
- Initialization Check:
- Accessing a
lateinit
property before it has been initialized will throw anUninitializedPropertyAccessException
.
- Accessing a
Usage Scenarios:
- Dependency Injection:
- Often used in frameworks like Spring or Android for injecting dependencies after object creation.
class Service {
lateinit var repository: Repository
fun setup() {
repository = RepositoryImpl()
}
fun performAction() {
repository.getData()
}
}
- Android Activities and Fragments:
- Commonly used for initializing UI components that are set up in lifecycle methods like
onCreate
.
- Commonly used for initializing UI components that are set up in lifecycle methods like
class MainActivity : AppCompatActivity() {
private lateinit var button: Button
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
button = findViewById(R.id.myButton)
button.setOnClickListener { /* Handle click */ }
}
}
Example:
class User {
lateinit var name: String
fun initializeName(userName: String) {
name = userName
}
fun printName() {
if (::name.isInitialized) {
println(name)
} else {
println("Name is not initialized")
}
}
}
fun main() {
val user = User()
user.printName() // Outputs: Name is not initialized
user.initializeName("Alice")
user.printName() // Outputs: Alice
}
Advantages:
- Avoids Nullability: Prevents the need to declare properties as nullable when you are certain they will be initialized before use.
- Cleaner Code: Reduces boilerplate code associated with null checks.
Limitations:
- Runtime Exceptions: Accessing an uninitialized
lateinit
property results in an exception, so developers must ensure proper initialization. - Not Suitable for Immutable Properties: Since
lateinit
requiresvar
, it cannot be used withval
properties.
Best Practices:
- Initialize Early: Initialize
lateinit
properties as soon as possible, preferably in initialization blocks or lifecycle methods. - Check Initialization: Use the
::property.isInitialized
syntax to check if alateinit
property has been initialized before accessing it. - Avoid Overuse: Use
lateinit
judiciously to prevent potential runtime issues. Consider alternatives like nullable types or constructor injection when appropriate.
40. How does Kotlin’s when
expression differ from Java’s switch
statement?
Kotlin’s when
expression is a more powerful and flexible alternative to Java’s switch
statement. While both are used for conditional branching based on the value of an expression, when
offers several enhancements and additional capabilities.
Key Differences:
- Expression vs. Statement:
- Kotlin
when
: Can be used as both an expression (returns a value) and a statement. - Java
switch
: Primarily a statement; starting from Java 14,switch
can also be used as an expression with the->
syntax.
- Kotlin
- Supported Data Types:
- Kotlin
when
: Supports a wide range of data types, including primitives, strings, enums, objects, and even arbitrary expressions. - Java
switch
: Traditionally limited to primitives (int
,char
, etc.),String
, and enum types. Java 17 introduced pattern matching forswitch
.
- Kotlin
- No Need for
break
:- Kotlin
when
: Automatically breaks after the first matching branch, eliminating the need forbreak
statements to prevent fall-through. - Java
switch
: Requires explicitbreak
statements to prevent fall-through unless intentional.
- Kotlin
- Multiple Conditions per Branch:
- Kotlin
when
: Allows multiple conditions in a single branch using commas. - Java
switch
: Requires multiplecase
labels to achieve similar functionality.
- Kotlin
- Range and Type Checks:
- Kotlin
when
: Can handle range checks (in
keyword), type checks (is
keyword), and arbitrary boolean expressions. - Java
switch
: Limited to exact matches of case values.
- Kotlin
- Default Case:
- Kotlin
when
: Useselse
as the default case, which is mandatory when thewhen
is used as an expression and not all possible cases are covered. - Java
switch
: Usesdefault
as the default case, which is optional.
- Kotlin
Examples:
- Basic Usage:
- Kotlin
when
:
- Kotlin
fun describe(obj: Any): String {
return when (obj) {
1 -> "One"
"Hello" -> "Greeting"
is Long -> "Long number"
!is String -> "Not a string"
else -> "Unknown"
}
}
- Java
switch
:
String describe(Object obj) {
switch (obj) {
case 1:
return "One";
case "Hello":
return "Greeting";
case Long l:
return "Long number"; // Requires Java 17+ for pattern matching
default:
return "Unknown";
}
}
- Multiple Conditions in Kotlin:
fun getColorName(color: String): String {
return when (color) {
"Red", "Crimson", "Maroon" -> "Red Family"
"Blue", "Azure", "Cyan" -> "Blue Family"
else -> "Unknown Color"
}
}
- Equivalent Java
String getColorName(String color) {
switch (color) {
case "Red":
case "Crimson":
case "Maroon":
return "Red Family";
case "Blue":
case "Azure":
case "Cyan":
return "Blue Family";
default:
return "Unknown Color";
}
}
- Range Check in Kotlin:
fun checkNumber(x: Int): String {
return when (x) {
in 1..10 -> "Between 1 and 10"
!in 11..20 -> "Not between 11 and 20"
else -> "Between 11 and 20"
}
}
Java switch
Equivalent: Java’s switch
does not natively support range checks, so you’d need to use if-else
statements instead.
- Using
when
as an Expression:
val result = when (val type = getType()) {
is String -> "String of length ${type.length}"
is Int -> "Integer: $type"
else -> "Unknown type"
}
Java switch
: Achieving similar functionality in Java would require more verbose code with multiple case
statements and potentially nested if-else
blocks.
Advantages of Kotlin’s when
:
- Enhanced Flexibility: Supports a broader range of conditions, including ranges, types, and complex expressions.
- Improved Readability: More concise and expressive, reducing boilerplate code.
- Safety: When used as an expression, the compiler ensures all possible cases are handled, especially when combined with sealed classes.
- No Fall-Through: Eliminates the common bugs associated with missing
break
statements inswitch
.
Conclusion: Kotlin’s when
expression offers a more powerful and flexible alternative to Java’s switch
statement, enabling developers to write clearer, more concise, and safer conditional logic.
Learn More: Carrer Guidance | Hiring Now!
Top 40 Mocha Interview Questions and Answers for JavaScript Developers
Java Multi Threading Interview Questions and Answers
Tosca Real-Time Scenario Questions and Answers
Advanced TOSCA Test Automation Engineer Interview Questions and Answers with 5+ Years of Experience
Tosca Interview Questions for Freshers with detailed Answers
Tosca interview question and answers- Basic to Advanced