Preparing for a Java 8 coding interview requires a solid understanding of the features and enhancements introduced in this version of the Java programming language. In this guide, we have compiled the top 30 Java 8 coding interview questions and answers to help you get ready. From understanding lambda expressions to mastering the Stream API, these questions cover all essential topics you need to know to ace your interview.
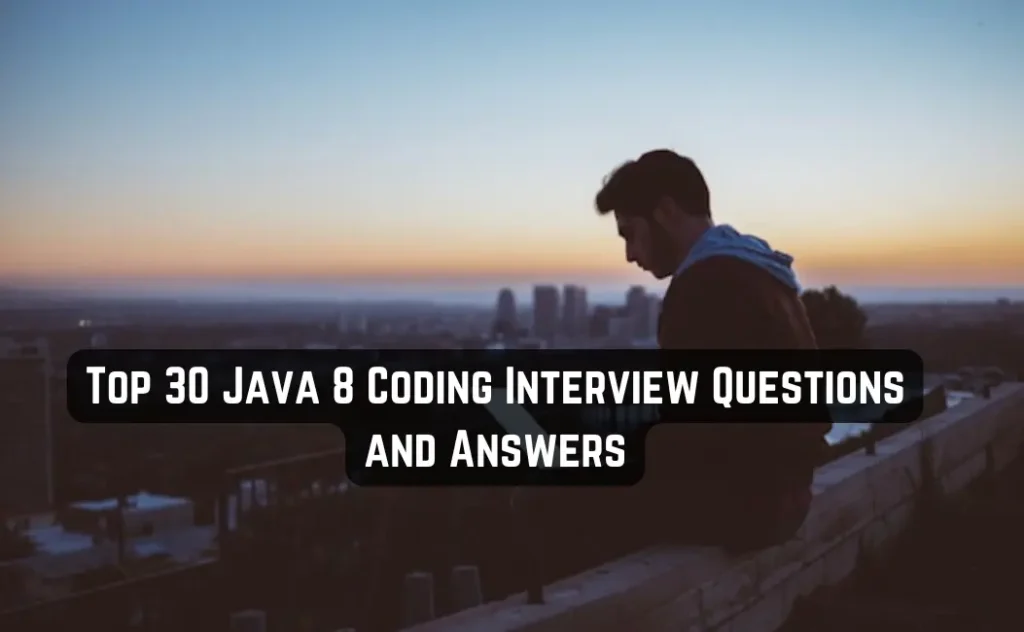
Top 30 Java 8 Coding Interview Questions and Answers
- What are the key features introduced in Java 8?
- What is a lambda expression, and how is it used in Java 8?
- What is the Stream API in Java 8, and how does it differ from collections?
- What is a functional interface, and how does it relate to lambda expressions?
- How do you filter a list of strings to find those starting with a specific letter using the Stream API?
- How can you sort a list of integers in descending order using Java 8 features?
- What is the purpose of the Optional class in Java 8, and how do you use it?
- What are method references in Java 8, and how do they simplify lambda expressions?
- How do you create a stream in Java 8, and what are the different ways to obtain a stream?
- What is the difference between map() and flatMap() methods in the Stream API?
- How can you handle exceptions in lambda expressions?
- What are default methods in interfaces, and why were they introduced in Java 8?
- How do you remove duplicates from a list using the Stream API?
- What is the purpose of the Collectors class in Java 8?
- How can you concatenate two streams in Java 8?
- How do you convert a list to a map using streams in Java 8?
- What are parallel streams in Java 8, and how do they work?
- How do you use the filter() method in the Stream API?
- What is the reduce() method in the Stream API, and how does it work?
- How do you sort a collection using streams in Java 8?
- What is the peek() method in the Stream API, and how is it used?
- What is the difference between findFirst() and findAny() in streams?
- How does the map() function differ from flatMap() in streams?
- How does Java 8 handle type inference with generics and lambda expressions?
- What are method references in Java 8, and how do they differ from lambda expressions?
- How do you create a custom functional interface in Java 8?
- What is the Collectors class in Java 8, and how is it used?
- How do you handle date and time in Java 8?
- What is the Instant class in Java 8, and how is it used?
- How do you convert between Instant and LocalDateTime in Java 8?
1. What are the key features introduced in Java 8?
Java 8 introduced several significant features that enhanced the language’s capabilities:
- Lambda Expressions: Enable treating functionality as a method argument, promoting a functional programming approach.
- Stream API: Facilitates functional-style operations on sequences of elements, such as filtering, mapping, and reducing.
- Functional Interfaces: Interfaces with a single abstract method, which can be implemented using lambda expressions.
- Default Methods: Allow interfaces to have methods with default implementations, aiding in interface evolution without breaking existing implementations.
- Optional Class: Provides a container object to represent the presence or absence of a value, reducing the risk of
NullPointerException
. - New Date and Time API: Offers a comprehensive and immutable approach to date and time manipulation, addressing the limitations of previous APIs.
These features collectively enhance code readability, facilitate functional programming paradigms, and improve overall developer productivity.
2. What is a lambda expression, and how is it used in Java 8?
A lambda expression is an anonymous function that provides a concise way to represent a method interface using an expression. It enables treating functionality as a method argument or storing it in a variable. Lambda expressions are primarily used to define the behavior of functional interfaces.
Syntax:
(parameters) -> {expression body}
Example:
// Traditional approach using an anonymous inner class
Runnable runnable = new Runnable() {
@Override
public void run() {
System.out.println("Hello, World!");
}
};
// Using a lambda expression
Runnable lambdaRunnable = () -> System.out.println("Hello, World!");
In this example, both runnable
and lambdaRunnable
perform the same action, but the lambda expression provides a more concise and readable way to implement the Runnable
interface.
3. What is the Stream API in Java 8, and how does it differ from collections?
The Stream API in Java 8 provides a functional approach to processing sequences of elements, such as collections. It allows for operations like filtering, mapping, and reducing, enabling a more declarative style of programming.
Key Differences Between Streams and Collections:
- Processing: Collections are primarily used for storing and accessing data, whereas streams are designed for processing data.
- Laziness: Streams support lazy operations, meaning intermediate operations are not executed until a terminal operation is invoked.
- Immutability: Operations on a stream do not modify its source; instead, they return a new stream with the transformed data.
- Traversal: Streams can be traversed only once, while collections can be traversed multiple times.
Example:
List<String> list = Arrays.asList("apple", "banana", "cherry");
// Using Stream API to filter and collect
List<String> result = list.stream()
.filter(s -> s.startsWith("a"))
.collect(Collectors.toList());
System.out.println(result); // Output: [apple]
In this example, the Stream API is used to filter elements that start with the letter “a” and collect them into a new list.
4. What is a functional interface, and how does it relate to lambda expressions?
A functional interface is an interface that contains exactly one abstract method. It can have multiple default or static methods, but only one abstract method. Functional interfaces serve as the target types for lambda expressions and method references.
Example:
@FunctionalInterface
public interface MyFunctionalInterface {
void execute();
}
In this example, MyFunctionalInterface
is a functional interface with a single abstract method execute()
. A lambda expression can be used to provide an implementation for this method:
MyFunctionalInterface func = () -> System.out.println("Executing...");
func.execute(); // Output: Executing...
Here, the lambda expression () -> System.out.println("Executing...")
implements the execute()
method of the functional interface.
5. How do you filter a list of strings to find those starting with a specific letter using the Stream API?
To filter a list of strings to find those that start with a specific letter using the Stream API, you can utilize the filter()
method in combination with a lambda expression.
Example:
List<String> list = Arrays.asList("apple", "banana", "cherry", "apricot", "blueberry");
// Filter strings starting with 'a'
List<String> result = list.stream()
.filter(s -> s.startsWith("a"))
.collect(Collectors.toList());
System.out.println(result); // Output: [apple, apricot]
In this example, filter(s -> s.startsWith("a"))
selects only the strings that start with the letter ‘a’, and collect(Collectors.toList())
gathers the filtered elements into a new list.
6. How can you sort a list of integers in descending order using Java 8 features?
To sort a list of integers in descending order using Java 8 features, you can use the sorted()
method of the Stream API with a custom comparator.
Example:
List<Integer> list = Arrays.asList(5, 3, 8, 1, 9);
// Sort in descending order
List<Integer> sortedList = list.stream()
.sorted(Comparator.reverseOrder())
.collect(Collectors.toList());
System.out.println(sortedList); // Output: [9, 8, 5, 3, 1]
In this example, Comparator.reverseOrder()
provides a comparator that sorts elements in descending order.
7. What is the purpose of the Optional
class in Java 8, and how do you use it?
The Optional
class in Java 8 serves as a container that may or may not hold a non-null value, providing a more expressive alternative to traditional null checks and helping to prevent NullPointerException
occurrences. By encapsulating the potential absence of a value, Optional
encourages developers to handle cases where a value might be missing more explicitly and safely.
Creating an Optional:
Empty Optional: Represents the absence of a value.
Optional<String> emptyOpt = Optional.empty();
Non-Empty Optional: Wraps a non-null value.
Optional<String> nonEmptyOpt = Optional.of("Hello");
Note: Passing a null value to Optional.of()
will throw a NullPointerException
.
Nullable Optional: Can hold a value that might be null
Optional<String> nullableOpt = Optional.ofNullable(nullValue);
This approach prevents exceptions by returning an empty Optional
if the provided value is null.
Checking for a Value:
isPresent(): Returns true
if a value is present; otherwise, false
.
if (optional.isPresent()) {
// Process the value
}
ifPresent(): Executes a specified action if a value is present.
optional.ifPresent(value -> System.out.println(value));
Retrieving the Value:
get(): Retrieves the value if present; throws NoSuchElementException
if not.
String value = optional.get();
Note: It’s safer to use methods like orElse()
or orElseGet()
to avoid exceptions.
orElse(): Returns the value if present; otherwise, returns a specified default value.
String value = optional.orElse("Default Value");
orElseGet(): Returns the value if present; otherwise, invokes a supplier to provide a default value.
String value = optional.orElseGet(() -> "Generated Default Value");
orElseThrow(): Returns the value if present; otherwise, throws an exception supplied by a provided supplier.
String value = optional.orElseThrow(() -> new IllegalArgumentException("Value is missing"));
Transforming the Value:
map(): Applies a function to the value if present, and returns an Optional
of the result.
Optional<Integer> lengthOpt = optional.map(String::length);
flatMap(): Similar to map()
, but the function must return an Optional
.
Optional<String> upperOpt = optional.flatMap(val -> Optional.of(val.toUpperCase()));
Filtering the Value:
filter(): Returns an Optional
describing the value if it matches a given predicate; otherwise, returns an empty Optional
.
Optional<String> filteredOpt = optional.filter(val -> val.startsWith("H"));
Example Usage:
public Optional<String> getUsernameById(String userId) {
User user = userRepository.findById(userId);
return Optional.ofNullable(user.getUsername());
}
// Usage
Optional<String> usernameOpt = getUsernameById("12345");
usernameOpt.ifPresent(username -> System.out.println("Username: " + username));
In this example, getUsernameById
returns an Optional<String>
, allowing the caller to handle the potential absence of a username gracefully.
By leveraging the Optional
class, developers can write more robust and readable code, explicitly handling cases where values may be absent and reducing the likelihood of encountering NullPointerException
.
8. What are method references in Java 8, and how do they simplify lambda expressions?
Method references provide a shorthand for invoking existing methods using the ::
operator, enhancing code readability by eliminating redundant lambda expressions. They allow you to refer to methods without executing them, serving as concise alternatives to lambda expressions that merely call a method.
Types of Method References:
Reference to a Static Method:
// Lambda expression
list.forEach(s -> System.out.println(s));
// Method reference
list.forEach(System.out::println);
Here, System.out::println
is a method reference to the static method println
of the PrintStream
class.
Reference to an Instance Method of a Particular Object:
// Lambda expression
myStringList.forEach(s -> this.processString(s));
// Method reference
myStringList.forEach(this::processString);
In this case, this::processString
refers to an instance method processString
of the current object.
Reference to an Instance Method of an Arbitrary Object of a Particular Type:
// Lambda expression
stringList.sort((s1, s2) -> s1.compareToIgnoreCase(s2));
// Method reference
stringList.sort(String::compareToIgnoreCase);
Here, String::compareToIgnoreCase
refers to the instance method compareToIgnoreCase
of the String
class.
Reference to a Constructor:
// Lambda expression
Supplier<List<String>> listSupplier = () -> new ArrayList<>();
// Method reference
Supplier<List<String>> listSupplier = ArrayList::new;
In this example, ArrayList::new
is a reference to the ArrayList
constructor.
By using method references, you can make your code more concise and easier to read, especially when the lambda expression simply calls an existing method.
9. How do you create a stream in Java 8, and what are the different ways to obtain a stream?
In Java 8, streams provide a sequence of elements supporting various operations to process data declaratively. You can create streams in several ways:
From a Collection:
List<String> list = Arrays.asList("a", "b", "c");
Stream<String> stream = list.stream();
Here, list.stream()
creates a sequential stream from the list.
From an Array:
String[] array = {"a", "b", "c"};
Stream<String> stream = Arrays.stream(array);
Alternatively:
Stream<String> stream = Stream.of(array);
From Individual Values:
Stream<String> stream = Stream.of("a", "b", "c");
From a File:
try (Stream<String> lines = Files.lines(Paths.get("file.txt"))) {
lines.forEach(System.out::println);
} catch (IOException e) {
e.printStackTrace();
}
This creates a stream of lines from a file.
From a Generator Function:
Stream<Double> randomNumbers = Stream.generate(Math::random).limit(10);
This generates an infinite stream of random numbers, limited to 10 elements.
From an Iterator Function:
Stream<Integer> evenNumbers = Stream.iterate(0, n -> n + 2).limit(10);
This creates an infinite stream of even numbers, limited to 10 elements.
These methods provide flexible ways to create streams from various data sources, enabling functional-style operations on collections and sequences of elements.
10. What is the difference between map()
and flatMap()
methods in the Stream API?
Both map()
and flatMap()
are intermediate operations in the Stream API used for transforming elements, but they serve different purposes:
map()
: Applies a function to each element of the stream, producing a stream of the transformed elements
List<String> list = Arrays.asList("a", "b", "c");
List<String> upperList = list.stream()
.map(String::toUpperCase)
.collect(Collectors.toList());
// Result: ["A", "B", "C"]
In this example, map()
transforms each string to its uppercase form.
flatMap()
: Applies a function to each element, which returns a stream of elements, and then flattens these streams into a single stream.
List<List<String>> listOfLists = Arrays.asList(
Arrays.asList("a", "b"),
Arrays.asList("c", "d")
);
List<String> flatList = listOfLists.stream()
.flatMap(List::stream)
.collect(Collectors.toList());
// Result: ["a", "b", "c", "d"]
Here, flatMap()
flattens the nested lists into a single list.
In summary, map()
is used for one-to-one transformations, while flatMap()
is used for one-to-many transformations, flattening nested structures into a single stream.
11. How can you handle exceptions in lambda expressions?
Handling exceptions within lambda expressions, especially checked exceptions, can be challenging due to the nature of functional interfaces in Java. Functional interfaces like Consumer<T>
do not declare any checked exceptions in their abstract methods, which means that lambda expressions implementing these interfaces cannot throw checked exceptions directly.
Approaches to Handle Exceptions in Lambda Expressions:
Using Try-Catch Blocks Within the Lambda:
You can enclose the code that might throw an exception within a try-catch block inside the lambda expression.
list.forEach(item -> {
try {
// Code that may throw a checked exception
} catch (Exception e) {
// Handle exception
}
});
This approach allows you to handle exceptions locally within the lambda but can lead to verbose code.
Wrapping Checked Exceptions in Runtime Exceptions:
Another approach is to catch the checked exception and rethrow it as an unchecked exception, such as RuntimeException
.
list.forEach(item -> {
try {
// Code that may throw a checked exception
} catch (Exception e) {
throw new RuntimeException(e);
}
});
This method propagates the exception up the call stack but converts it into an unchecked exception.
Creating Custom Functional Interfaces That Declare Exceptions:
Define a custom functional interface that declares the checked exception in its abstract method.
@FunctionalInterface
public interface ThrowingConsumer<T> {
void accept(T t) throws Exception;
}
Then, create a wrapper method to handle the exception:
public static <T> Consumer<T> throwingConsumerWrapper(ThrowingConsumer<T> throwingConsumer) {
return i -> {
try {
throwingConsumer.accept(i);
} catch (Exception ex) {
throw new RuntimeException(ex);
}
};
}
Usage:
list.forEach(throwingConsumerWrapper(item -> {
// Code that may throw a checked exception
}));
This approach allows you to handle checked exceptions in a more structured way within lambda expressions.
By employing these techniques, you can effectively manage exceptions within lambda expressions, maintaining code readability and robustness.
12. What are default methods in interfaces, and why were they introduced in Java 8?
Default methods, introduced in Java 8, are methods within an interface that have a default implementation. They are declared using the default
keyword.
Purpose of Default Methods:
- Interface Evolution: They allow the addition of new methods to interfaces without breaking existing implementations. This is particularly useful for evolving APIs.
Example:
public interface MyInterface {
void existingMethod();
default void newDefaultMethod() {
System.out.println("This is a default method.");
}
}
In this example, newDefaultMethod
is a default method with an implementation. Classes implementing MyInterface
can use this method without overriding it, ensuring backward compatibility.
13. How do you remove duplicates from a list using the Stream API?
To remove duplicates from a list using the Stream API, you can utilize the distinct()
method, which returns a stream consisting of distinct elements.
Example:
List<Integer> listWithDuplicates = Arrays.asList(1, 2, 2, 3, 4, 4, 5);
List<Integer> listWithoutDuplicates = listWithDuplicates.stream()
.distinct()
.collect(Collectors.toList());
System.out.println(listWithoutDuplicates); // Output: [1, 2, 3, 4, 5]
In this example, distinct()
filters out duplicate elements, and collect(Collectors.toList())
gathers the distinct elements into a new list.
14. What is the purpose of the Collectors
class in Java 8?
The Collectors
class in Java 8 provides a set of static methods that implement various reduction operations, such as accumulating elements into collections, summarizing elements according to various criteria, and more. It is commonly used in conjunction with the Stream API’s collect()
method to transform the elements of a stream into a desired result.
Common Collectors:
toList(): Collects elements into a List
.
List<String> list = stream.collect(Collectors.toList());
toSet(): Collects elements into a Set
.
Set<String> set = stream.collect(Collectors.toSet());
toMap(): Collects elements into a Map
.
Map<Integer, String> map = stream.collect(Collectors.toMap(String::length, Function.identity()));
joining(): Concatenates elements into a single String
.
String result = stream.collect(Collectors.joining(", "));
groupingBy(): Groups elements by a classifier function.
Map<Integer, List<String>> groupedByLength = stream.collect(Collectors.groupingBy(String::length));
partitioningBy(): Partitions elements based on a predicate.
Map<Boolean, List<String>> partitioned = stream.collect(Collectors.partitioningBy(s -> s.length() > 3));
The Collectors
class facilitates complex mutable reduction operations, enabling the collection of stream elements into various data structures and forms.
15. How can you concatenate two streams in Java 8?
To concatenate two streams in Java 8, you can use the Stream.concat()
method, which combines two streams into a single sequential stream. This method takes two streams as input and returns a new stream containing all elements from both input streams.
Example:
Stream<String> stream1 = Stream.of("A", "B", "C");
Stream<String> stream2 = Stream.of("D", "E", "F");
// Concatenating two streams
Stream<String> concatenatedStream = Stream.concat(stream1, stream2);
// Collecting the concatenated stream into a list
List<String> resultList = concatenatedStream.collect(Collectors.toList());
System.out.println(resultList); // Output: [A, B, C, D, E, F]
In this example, Stream.concat(stream1, stream2)
merges stream1
and stream2
into a single stream, which is then collected into a list containing all elements from both streams.
Key Points:
- Order Preservation: The resulting stream maintains the order of elements, with all elements from the first stream followed by all elements from the second stream.
- Parallel Streams: If either of the input streams is parallel, the resulting stream will also be parallel.
- Stream Closure: Closing the concatenated stream will close both input streams.
- Multiple Streams: To concatenate more than two streams, you can use nested
Stream.concat()
calls or leverage theStream.of()
method combined withflatMap()
.
Concatenating Multiple Streams:
Stream<String> stream1 = Stream.of("A", "B");
Stream<String> stream2 = Stream.of("C", "D");
Stream<String> stream3 = Stream.of("E", "F");
// Using nested Stream.concat()
Stream<String> concatenatedStream = Stream.concat(Stream.concat(stream1, stream2), stream3);
// Collecting the concatenated stream into a list
List<String> resultList = concatenatedStream.collect(Collectors.toList());
System.out.println(resultList); // Output: [A, B, C, D, E, F]
Alternatively, using Stream.of()
and flatMap()
:
Stream<String> stream1 = Stream.of("A", "B");
Stream<String> stream2 = Stream.of("C", "D");
Stream<String> stream3 = Stream.of("E", "F");
// Using Stream.of() and flatMap()
Stream<String> concatenatedStream = Stream.of(stream1, stream2, stream3)
.flatMap(s -> s);
// Collecting the concatenated stream into a list
List<String> resultList = concatenatedStream.collect(Collectors.toList());
System.out.println(resultList); // Output: [A, B, C, D, E, F]
In this approach, Stream.of(stream1, stream2, stream3)
creates a stream of streams, and flatMap(s -> s)
flattens it into a single stream containing all elements.
By utilizing these methods, you can effectively concatenate multiple streams in Java 8, enabling flexible and efficient data processing pipelines.
16. How do you convert a list to a map using streams in Java 8?
In Java 8, you can convert a List
to a Map
using the Stream API along with the Collectors.toMap()
method. This approach allows you to define how to extract keys and values from the list elements.
Example:
Suppose you have a list of Person
objects and want to create a map where the keys are the person’s IDs and the values are the person objects themselves:
import java.util.*;
import java.util.stream.Collectors;
class Person {
private int id;
private String name;
// Constructor, getters, and setters
public Person(int id, String name) {
this.id = id;
this.name = name;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
}
public class ListToMapExample {
public static void main(String[] args) {
List<Person> personList = Arrays.asList(
new Person(1, "Alice"),
new Person(2, "Bob"),
new Person(3, "Charlie")
);
// Converting List to Map
Map<Integer, Person> personMap = personList.stream()
.collect(Collectors.toMap(Person::getId, person -> person));
// Displaying the Map
personMap.forEach((id, person) ->
System.out.println("ID: " + id + ", Name: " + person.getName()));
}
}
Output:
ID: 1, Name: Alice
ID: 2, Name: Bob
ID: 3, Name: Charlie
Explanation:
personList.stream()
converts the list into a stream.Collectors.toMap(Person::getId, person -> person)
collects the elements of the stream into a map:Person::getId
specifies that the key for each map entry should be the person’s ID.person -> person
specifies that the value for each map entry should be the person object itself.
Handling Duplicate Keys:
If the list contains duplicate keys (i.e., multiple persons with the same ID), the above approach will throw an IllegalStateException
. To handle duplicates, you can provide a merge function to toMap()
. For example, to keep the first occurrence:
Map<Integer, Person> personMap = personList.stream()
.collect(Collectors.toMap(
Person::getId,
person -> person,
(existing, replacement) -> existing
));
In this case, (existing, replacement) -> existing
ensures that the first occurrence is retained in case of duplicates.
Specifying a Specific Map Implementation:
By default, Collectors.toMap()
returns a HashMap
. If you want to use a different map implementation, such as a TreeMap
, you can provide a map supplier:
Map<Integer, Person> personMap = personList.stream()
.collect(Collectors.toMap(
Person::getId,
person -> person,
(existing, replacement) -> existing,
TreeMap::new
));
This approach allows you to control the type of map returned.
By utilizing the Stream API and Collectors.toMap()
, you can efficiently convert a list to a map in Java 8, with control over key collisions and the specific map implementation used.
17. What are parallel streams in Java 8, and how do they work?
Parallel streams in Java 8 allow for parallel processing of data, enabling operations to be executed concurrently across multiple threads. This can lead to performance improvements on multi-core processors by dividing the workload into smaller chunks that are processed simultaneously.
How Parallel Streams Work:
- Splitting: The stream is divided into multiple substreams. This is typically handled by the stream’s
Spliterator
, which partitions the data source. - Processing: Each substream is processed independently in separate threads, utilizing the Fork/Join framework introduced in Java 7.
- Combining: The results from each substream are combined to produce the final result.
Creating Parallel Streams:
From a Collection:
List<String> list = Arrays.asList("a", "b", "c");
Stream<String> parallelStream = list.parallelStream();
From an Existing Stream:
Stream<String> stream = list.stream();
Stream<String> parallelStream = stream.parallel();
Example:
List<String> list = Arrays.asList("a", "b", "c", "d");
// Using a parallel stream to process elements
list.parallelStream().forEach(System.out::println);
In this example, parallelStream()
creates a parallel stream from the list, and forEach
processes each element concurrently.
Considerations When Using Parallel Streams:
- Data Size: Parallel streams are more beneficial with large datasets where the overhead of parallelism is outweighed by performance gains.
- Data Source: Some data sources, like
ArrayList
, are more efficiently split for parallel processing than others, like linked lists. - Boxing/Unboxing: Excessive boxing and unboxing can negate the benefits of parallelism.
- Thread Safety: Ensure that operations performed in parallel are thread-safe to avoid concurrency issues.
- Performance Testing: Always measure performance, as parallel streams can sometimes be slower than sequential streams due to overhead.
By leveraging parallel streams appropriately, you can harness the power of multi-core processors to improve the performance of data processing tasks in Java 8.
18. How do you use the filter()
method in the Stream API?
The filter()
method in Java 8’s Stream API is an intermediate operation that allows you to process a sequence of elements, retaining only those that match a specified condition. It takes a Predicate
(a functional interface) as an argument and returns a new stream consisting of elements that satisfy the predicate.
Syntax:
Stream<T> filter(Predicate<? super T> predicate)
Example:
Suppose you have a list of integers and want to filter out the even numbers:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println(evenNumbers); // Output: [2, 4, 6]
Explanation:
numbers.stream()
converts the list into a sequential stream..filter(n -> n % 2 == 0)
applies a predicate to each element, retaining only those that are even..collect(Collectors.toList())
collects the filtered elements into a new list.
Key Points:
- Lazy Evaluation: The
filter()
method is lazy; it doesn’t process elements until a terminal operation (likecollect()
) is invoked. - Multiple Filters: You can chain multiple
filter()
operations to apply multiple conditions. - Parallel Streams: The
filter()
operation is compatible with parallel streams, allowing for concurrent processing.
Example with Multiple Filters:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
List<String> result = names.stream()
.filter(name -> name.startsWith("A"))
.filter(name -> name.length() > 3)
.collect(Collectors.toList());
System.out.println(result); // Output: [Alice]
In this example, the stream is filtered to include only names that start with “A” and have a length greater than three.
By utilizing the filter()
method, you can efficiently process and extract subsets of data from collections based on specific criteria, leading to more readable and concise code.
19. What is the reduce()
method in the Stream API, and how does it work?
The reduce()
method in Java 8’s Stream API is a terminal operation that aggregates the elements of a stream into a single result. It applies a binary operator (a function that takes two arguments and produces a single result) repeatedly to the elements of the stream, combining them into one.
Common Variants of reduce()
:
Without Identity:
Optional<T> reduce(BinaryOperator<T> accumulator)
Description: Performs a reduction on the elements using the provided accumulator function. Returns an Optional
describing the result, or an empty Optional
if the stream is empty.
With Identity:
T reduce(T identity, BinaryOperator<T> accumulator)
Description: Performs a reduction on the elements using the provided identity value and accumulator function. Returns the result of the reduction.
With Identity and Combiner (for Parallel Processing):
<U> U reduce(U identity, BiFunction<U, ? super T, U> accumulator, BinaryOperator<U> combiner)
Description: Performs a reduction on the elements using the provided identity value, accumulator function, and combiner function. Suitable for parallel processing.
Example: Summing a List of Integers
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream()
.reduce(0, Integer::sum);
System.out.println(sum); // Output: 15
Explanation:
numbers.stream()
creates a stream from the list..reduce(0, Integer::sum)
reduces the stream to a single integer by summing the elements, starting with an identity value of0
.
Example: Concatenating Strings
List<String> letters = Arrays.asList("a", "b", "c", "d");
String concatenated = letters.stream()
.reduce("", String::concat);
System.out.println(concatenated); // Output: abcd
Explanation:
letters.stream()
creates a stream from the list..reduce("", String::concat)
concatenates the strings in the stream, starting with an empty string as the identity value.
Key Points:
- Identity Value: The identity value is the initial value for the reduction and the default result if the stream is empty. It should be the identity element for the operation (e.g.,
0
for addition,1
for multiplication). - Accumulator Function: The accumulator function takes two parameters: a partial result and the next element, and returns a new partial result.
- Combiner Function: In parallel streams, the combiner function combines the results of the substreams. It’s used to merge the partial results produced by the accumulator function.
Example: Finding the Maximum Value
List<Integer> numbers = Arrays.asList(3, 5, 2, 8, 7);
int max = numbers.stream()
.reduce(Integer.MIN_VALUE, Integer::max);
System.out.println(max); // Output: 8
Explanation:
numbers.stream()
creates a stream from the list..reduce(Integer.MIN_VALUE, Integer::max)
finds the maximum value in the stream, starting withInteger.MIN_VALUE
as the identity value.
The reduce()
method is a powerful tool for performing aggregation operations on streams, enabling the transformation of a sequence of elements into a single result through a specified combining operation.
20. How do you sort a collection using streams in Java 8?
In Java 8, you can sort a collection using the Stream API’s sorted()
method, which returns a stream with elements sorted according to their natural order or a specified comparator.
Sorting in Natural Order:
List<String> list = Arrays.asList("Banana", "Apple", "Mango");
List<String> sortedList = list.stream()
.sorted()
.collect(Collectors.toList());
System.out.println(sortedList); // Output: [Apple, Banana, Mango]
In this example, sorted()
arranges the strings in ascending alphabetical order.
Sorting in Reverse Order:
List<String> list = Arrays.asList("Banana", "Apple", "Mango");
List<String> sortedList = list.stream()
.sorted(Comparator.reverseOrder())
.collect(Collectors.toList());
System.out.println(sortedList); // Output: [Mango, Banana, Apple]
Here, sorted(Comparator.reverseOrder())
arranges the strings in descending order.
Sorting with a Custom Comparator:
For custom objects, you can use a comparator to define the sorting logic.
class Person {
private String name;
private int age;
// Constructor, getters, and setters
}
List<Person> people = Arrays.asList(
new Person("Alice", 30),
new Person("Bob", 25),
new Person("Charlie", 28)
);
List<Person> sortedByAge = people.stream()
.sorted(Comparator.comparingInt(Person::getAge))
.collect(Collectors.toList());
sortedByAge.forEach(person ->
System.out.println(person.getName() + ": " + person.getAge()));
// Output:
// Bob: 25
// Charlie: 28
// Alice: 30
In this example, sorted(Comparator.comparingInt(Person::getAge))
sorts the list of Person
objects by age in ascending order.
Key Points:
- Stability: The
sorted()
method is stable; it preserves the relative order of equal elements. - Intermediate Operation:
sorted()
is an intermediate operation; it returns a new stream and does not modify the original collection. - Terminal Operation Required: To produce a result, a terminal operation like
collect()
must followsorted()
.
By leveraging the sorted()
method in the Stream API, you can efficiently sort collections in Java 8 according to various criteria.
21. What is the peek()
method in the Stream API, and how is it used?
The peek()
method in Java 8’s Stream API is an intermediate operation that allows you to perform a specified action on each element of the stream as it is processed. It is primarily used for debugging purposes, enabling you to inspect the elements as they flow through the stream pipeline without modifying them.
Syntax:
Stream<T> peek(Consumer<? super T> action)
Example:
List<String> list = Arrays.asList("apple", "banana", "cherry");
List<String> result = list.stream()
.filter(s -> s.startsWith("a"))
.peek(s -> System.out.println("Filtered value: " + s))
.collect(Collectors.toList());
In this example, peek()
is used to print each element that passes the filter condition. The output will be:
Filtered value: apple
Key Points:
- Non-Interfering: The
peek()
method does not modify the elements; it only performs the specified action. - Lazy Evaluation: Like other intermediate operations,
peek()
is lazy and will not execute until a terminal operation is invoked. - Use Cases: While useful for debugging,
peek()
should be used cautiously in production code to avoid unintended side effects.
By utilizing peek()
, developers can gain insights into the processing of stream elements, facilitating debugging and understanding of the stream pipeline.
22. What is the difference between findFirst()
and findAny()
in streams?
Both findFirst()
and findAny()
are terminal operations in the Stream API that return an Optional
describing some element of the stream. The key differences lie in their behavior, especially concerning parallel streams:
findFirst()
:
- Sequential Streams: Returns the first element in the encounter order.
- Parallel Streams: Still returns the first element in the encounter order, which may involve additional overhead to ensure order preservation.
findAny()
:
- Sequential Streams: Typically returns the first element but is not guaranteed.
- Parallel Streams: May return any element, allowing for more efficient execution as it doesn’t require preserving encounter order.
Example:
List<String> list = Arrays.asList("apple", "banana", "cherry");
Optional<String> first = list.stream().findFirst();
Optional<String> any = list.parallelStream().findAny();
Key Points:
- Deterministic vs. Non-Deterministic:
findFirst()
is deterministic, always returning the first element in encounter order, whilefindAny()
is non-deterministic, especially in parallel streams. - Performance Considerations:
findAny()
can be more performant in parallel streams as it allows for greater optimization opportunities.
Understanding these differences enables developers to choose the appropriate method based on the requirements for order and performance.
23. How does the map()
function differ from flatMap()
in streams?
Both map()
and flatMap()
are intermediate operations in the Stream API used for transforming elements, but they differ in their behavior:
map()
:
- Functionality: Applies a function to each element, transforming it into a new element.
- Output: Produces a stream of the same structure as the input, with each element transformed.
flatMap()
:
- Functionality: Applies a function to each element that produces a stream of new values, then flattens these streams into a single stream.
- Output: Produces a flattened stream containing all the elements from the streams generated by the function.
Example:
List<List<String>> list = Arrays.asList(
Arrays.asList("a", "b"),
Arrays.asList("c", "d")
);
// Using map()
List<Stream<String>> mapped = list.stream()
.map(Collection::stream)
.collect(Collectors.toList());
// Using flatMap()
List<String> flatMapped = list.stream()
.flatMap(Collection::stream)
.collect(Collectors.toList());
Key Points:
- Nested Structures:
map()
is suitable for one-to-one transformations, whileflatMap()
is ideal for flattening nested structures. - Use Cases: Use
map()
when each input element maps to exactly one output element; useflatMap()
when each input element maps to multiple output elements.
By choosing the appropriate method, developers can effectively transform and process data within streams.
24. How does Java 8 handle type inference with generics and lambda expressions?
Java 8 enhances type inference capabilities, allowing the compiler to deduce types in various contexts, particularly with generics and lambda expressions.
Generics:
- Diamond Operator (
<>
): Simplifies instantiation of generic types by inferring type parameters from the context.
List<String> list = new ArrayList<>();
Lambda Expressions:
- Parameter Types: The compiler infers parameter types based on the target functional interface.
Predicate<String> predicate = s -> s.isEmpty();
In this example, the compiler infers that s
is of type String
because Predicate<String>
‘s test
method accepts a String
.
Key Points:
- Improved Readability: Enhanced type inference reduces boilerplate code, leading to more concise and readable code.
- Compiler Capabilities: The compiler uses contextual information to infer types, streamlining the development process.
These enhancements in Java 8 facilitate more expressive and succinct code, improving developer productivity.
25. What are method references in Java 8, and how do they differ from lambda expressions?
Method references in Java 8 provide a concise way to refer to existing methods or constructors using the ::
operator. They serve as shorthand for lambda expressions that invoke existing methods, enhancing code readability and reducing verbosity.
Types of Method References:
- Reference to a Static Method:
- Syntax:
ClassName::staticMethodName
- Example:
Integer::parseInt
- Syntax:
- Reference to an Instance Method of a Particular Object:
- Syntax:
instance::instanceMethodName
- Example:
myInstance::myMethod
- Syntax:
- Reference to an Instance Method of an Arbitrary Object of a Particular Type:
- Syntax:
ClassName::instanceMethodName
- Example:
String::toLowerCase
- Syntax:
- Reference to a Constructor:
- Syntax:
ClassName::new
- Example:
ArrayList::new
- Syntax:
Example:
List<String> list = Arrays.asList("apple", "banana", "cherry");
// Using a lambda expression
list.forEach(s -> System.out.println(s));
// Using a method reference
list.forEach(System.out::println);
In this example, both approaches produce the same output. The method reference System.out::println
is a shorthand for the lambda expression s -> System.out.println(s)
.
Differences Between Lambda Expressions and Method References:
- Syntax: Lambda expressions explicitly define parameters and the method body, while method references directly refer to existing methods without specifying parameters.
- Conciseness: Method references can make the code more concise and readable when they directly map to existing methods.
- Use Cases: Lambda expressions are more flexible and can include additional logic, whereas method references are suitable when the lambda expression merely calls an existing method.
When to Use:
- Method References: Prefer method references when a lambda expression simply invokes an existing method without additional logic.
- Lambda Expressions: Use lambda expressions when you need to implement custom behavior or when the logic doesn’t directly map to a single existing method.
By understanding and appropriately applying method references and lambda expressions, developers can write more concise and maintainable code in Java 8.
26. How do you create a custom functional interface in Java 8?
In Java 8, a functional interface is an interface with a single abstract method, serving as the target for lambda expressions and method references. To create a custom functional interface:
- Define an Interface with a Single Abstract Method:
@FunctionalInterface
public interface MyFunctionalInterface {
void execute();
}
- Use the
@FunctionalInterface
Annotation (Optional but Recommended):- This annotation indicates that the interface is intended to be a functional interface and prompts the compiler to enforce this constraint.
- Implement the Interface Using a Lambda Expression:
MyFunctionalInterface func = () -> System.out.println("Executing...");
func.execute(); // Output: Executing...
Key Points:
- Single Abstract Method: The interface must have exactly one abstract method.
- Default and Static Methods: The interface can include default and static methods without affecting its status as a functional interface.
@FunctionalInterface
Annotation: While optional, using this annotation enhances code clarity and ensures the interface remains functional.
By creating custom functional interfaces, developers can define specific behaviors and utilize lambda expressions to implement them concisely.
27. What is the Collectors
class in Java 8, and how is it used?
The Collectors
class in Java 8 provides a set of static methods for accumulating elements of streams into collections or summarizing them. It facilitates the reduction of stream elements into more manageable forms, such as lists, sets, maps, or summary statistics.
Commonly Used Collectors:
toList()
: Collects elements into aList
.
List<String> list = stream.collect(Collectors.toList());
toSet()
: Collects elements into aSet
.
Set<String> set = stream.collect(Collectors.toSet());
toMap()
: Collects elements into aMap
.
Map<Integer, String> map = stream.collect(Collectors.toMap(String::length, Function.identity()));
joining()
: Concatenates elements into a singleString
String result = stream.collect(Collectors.joining(", "));
groupingBy()
: Groups elements by a classifier function.
Map<Integer, List<String>> grouped = stream.collect(Collectors.groupingBy(String::length));
partitioningBy()
: Partitions elements based on a predicate.
Map<Boolean, List<String>> partitioned = stream.collect(Collectors.partitioningBy(s -> s.length() > 3));
Example:
List<String> list = Arrays.asList("apple", "banana", "cherry");
Map<Integer, List<String>> groupedByLength = list.stream()
.collect(Collectors.groupingBy(String::length));
System.out.println(groupedByLength);
Output:
{5=[apple], 6=[banana], 6=[cherry]}
Key Points:
- Immutable Collections: The collections returned are not guaranteed to be mutable; if a mutable collection is needed, consider using
toCollection()
with a specific supplier. - Thread Safety: The collectors provided are not thread-safe unless specified; caution is needed when using them with parallel streams.
- Custom Collectors: Developers can create custom collectors by implementing the
Collector
interface.
The Collectors
class provides a powerful and flexible framework for transforming and accumulating stream elements, enabling complex data processing tasks to be expressed succinctly.
28. How do you handle date and time in Java 8?
Java 8 introduced a comprehensive Date and Time API within the java.time
package, addressing the limitations of the previous java.util.Date
and java.util.Calendar
classes. This new API offers a more intuitive and flexible approach to handling dates and times.
Key Classes in the java.time
Package:
LocalDate
: Represents a date without a time zone, such as2024-11-30
.LocalTime
: Represents a time without a date or time zone, such as17:40:57
.LocalDateTime
: Combines date and time without a time zone.ZonedDateTime
: Represents a date and time with a time zone.Instant
: Represents a timestamp, typically used for machine time.Duration
: Measures time-based amounts, such as “34.5 seconds”.Period
: Measures date-based amounts, such as “2 years, 3 months, and 4 days”.ZoneId
: Represents a time zone identifier.DateTimeFormatter
: Provides formatting and parsing capabilities for dates and times.
Examples:
- Getting the Current Date and Time:
LocalDate currentDate = LocalDate.now();
LocalTime currentTime = LocalTime.now();
LocalDateTime currentDateTime = LocalDateTime.now();
ZonedDateTime currentZonedDateTime = ZonedDateTime.now();
System.out.println("Current Date: " + currentDate);
System.out.println("Current Time: " + currentTime);
System.out.println("Current DateTime: " + currentDateTime);
System.out.println("Current ZonedDateTime: " + currentZonedDateTime);
- Creating Specific Date and Time Instances:
LocalDate specificDate = LocalDate.of(2024, Month.NOVEMBER, 30);
LocalTime specificTime = LocalTime.of(17, 40, 57);
LocalDateTime specificDateTime = LocalDateTime.of(specificDate, specificTime);
ZonedDateTime specificZonedDateTime = ZonedDateTime.of(specificDateTime, ZoneId.of("Asia/Kolkata"));
System.out.println("Specific Date: " + specificDate);
System.out.println("Specific Time: " + specificTime);
System.out.println("Specific DateTime: " + specificDateTime);
System.out.println("Specific ZonedDateTime: " + specificZonedDateTime);
- Parsing and Formatting Dates:
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd-MM-yyyy HH:mm:ss");
String dateTimeString = "30-11-2024 17:40:57";
LocalDateTime parsedDateTime = LocalDateTime.parse(dateTimeString, formatter);
String formattedDateTime = parsedDateTime.format(formatter);
System.out.println("Parsed DateTime: " + parsedDateTime);
System.out.println("Formatted DateTime: " + formattedDateTime);
- Calculating Duration and Period:
LocalDate startDate = LocalDate.of(2024, Month.JANUARY, 1);
LocalDate endDate = LocalDate.of(2024, Month.NOVEMBER, 30);
Period period = Period.between(startDate, endDate);
LocalTime startTime = LocalTime.of(10, 0);
LocalTime endTime = LocalTime.of(17, 40, 57);
Duration duration = Duration.between(startTime, endTime);
System.out.println("Period: " + period.getYears() + " years, " +
period.getMonths() + " months, " + period.getDays() + " days");
System.out.println("Duration: " + duration.toHours() + " hours, " +
duration.toMinutesPart() + " minutes, " + duration.toSecondsPart() + " seconds");
Key Features of the New Date and Time API:
- Immutability: All classes in the
java.time
package are immutable and thread-safe, ensuring consistency in multi-threaded environments. - Clarity: The API provides clear and concise methods for common date and time operations, reducing the complexity associated with earlier classes.
- Time Zone Support: Enhanced support for time zones through classes like
ZonedDateTime
andZoneId
, simplifying the handling of different time zones. - Comprehensive Functionality: Offers a wide range of classes and methods to handle various aspects of date and time, including parsing, formatting, and arithmetic operations.
By leveraging the new Date and Time API in Java 8, developers can write more readable, maintainable, and reliable code when working with dates and times.
29. What is the Instant
class in Java 8, and how is it used?
The Instant
class in Java 8, part of the java.time
package, represents a specific point on the timeline, typically modeled as the number of nanoseconds since the Unix epoch (1970-01-01T00:00:00Z). It is immutable and thread-safe, making it suitable for high-precision timestamping.
Key Characteristics:
- Precision:
Instant
provides nanosecond precision. - Time Zone Neutrality: It represents an absolute point in time without any time zone information.
- Immutability: Instances of
Instant
are immutable and thread-safe.
Common Usage:
- Obtaining the Current Timestamp:
Instant now = Instant.now();
System.out.println("Current Instant: " + now);
- Creating an Instant from Epoch Seconds or Milliseconds:
Instant fromEpochSecond = Instant.ofEpochSecond(1609459200); // 2021-01-01T00:00:00Z
Instant fromEpochMilli = Instant.ofEpochMilli(1609459200000L); // 2021-01-01T00:00:00Z
- Parsing an Instant from a String:
Instant parsedInstant = Instant.parse("2024-11-30T12:00:00Z");
- Adding or Subtracting Time:
Instant now = Instant.now();
Instant later = now.plusSeconds(3600); // Adds one hour
Instant earlier = now.minus(1, ChronoUnit.DAYS); // Subtracts one day
- Converting to Other Date-Time Classes:
// Convert Instant to LocalDateTime in system default time zone
LocalDateTime dateTime = LocalDateTime.ofInstant(instant, ZoneId.systemDefault());
// Convert Instant to ZonedDateTime in a specific time zone
ZonedDateTime zonedDateTime = instant.atZone(ZoneId.of("Asia/Kolkata"));
Key Points:
- Machine-Readable Timestamps:
Instant
is ideal for representing machine-readable timestamps, such as those used in logging or event tracking. - Arithmetic Operations: Supports addition and subtraction of time units, facilitating time-based calculations.
- Comparison: Provides methods like
isBefore()
,isAfter()
, andequals()
for comparing instants. - Interoperability: Can be converted to other date-time classes, such as
LocalDateTime
orZonedDateTime
, for human-readable representations.
By utilizing the Instant
class, developers can effectively handle precise timestamps and perform time-based calculations in a clear and efficient manner.
30. How do you convert between Instant
and LocalDateTime
in Java 8?
In Java 8, converting between Instant
and LocalDateTime
requires accounting for time zones, as Instant
represents a point in time in UTC, while LocalDateTime
is time-zone agnostic. The conversion involves associating the Instant
with a time zone to obtain a LocalDateTime
, and vice versa.
Converting Instant
to LocalDateTime
:
Using System Default Time Zone:
Instant instant = Instant.now();
LocalDateTime localDateTime = LocalDateTime.ofInstant(instant, ZoneId.systemDefault());
Using a Specific Time Zone:
Instant instant = Instant.now();
LocalDateTime localDateTime = LocalDateTime.ofInstant(instant, ZoneId.of("Asia/Kolkata"));
Converting LocalDateTime
to Instant
:
Using System Default Time Zone:
LocalDateTime localDateTime = LocalDateTime.now();
Instant instant = localDateTime.atZone(ZoneId.systemDefault()).toInstant();
Using a Specific Time Zone:
LocalDateTime localDateTime = LocalDateTime.now();
Instant instant = localDateTime.atZone(ZoneId.of("Asia/Kolkata")).toInstant();
Key Points:
- Time Zone Consideration: Converting between
Instant
andLocalDateTime
requires specifying a time zone to accurately represent the point in time. - Immutability: Both
Instant
andLocalDateTime
are immutable; conversions produce new instances without modifying the original objects. - Precision: Both classes support nanosecond precision, ensuring high-resolution time representations.
By understanding and correctly applying these conversions, developers can seamlessly work with different date-time representations in Java 8.
Learn More: Carrer Guidance | Hiring Now!
Top 20 Chipotle Interview Questions and Answers
Batch Apex Interview Questions for Freshers with Answers
Mphasis interview Questions and Answers- Basic to Advanced
Redux Interview Questions and Answers: From Basics to Advanced Concepts
Jira Interview Questions and Answers- Basic to Advanced
PostgreSQL interview Questions and Answers- Basic to Advanced
Palo Alto Interview Questions and Answers- Basic to Advanced