Preparing for a full-stack developer interview requires a deep understanding of both front-end and back-end technologies. Below are 40+ essential and most commonly asked full-stack developer interview questions each with detailed answers to help you demonstrate your expertise.
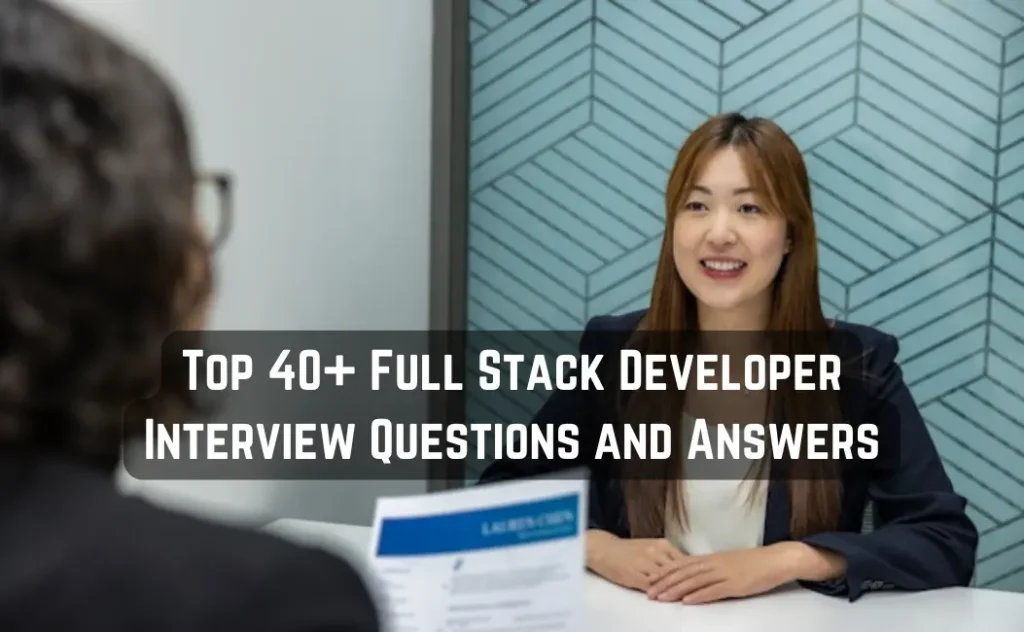
Top 40+ Full Stack Developer Interview Questions and Answers
- What is Full-Stack Development?
- Can you explain the Model-View-Controller (MVC) architecture?
- What are RESTful APIs, and how do they work?
- How do you ensure the security of a web application?
- What is the difference between SQL and NoSQL databases?
- How do you optimize the performance of a web application?
- What is Cross-Origin Resource Sharing (CORS), and why is it important?
- Can you explain the concept of Promises in JavaScript?
- What is the purpose of Node.js, and how does it work?
- How do you manage state in a React application?
- What is the difference between synchronous and asynchronous programming?
- How do you handle errors in a web application?
- What are microservices, and how do they differ from monolithic architectures?
- What is the purpose of Docker, and how does it work?
- How do you implement authentication and authorization in a web application?
- What is Continuous Integration and Continuous Deployment (CI/CD), and why are they important?
- How do you handle state management in a React application?
- What is the purpose of Webpack in modern web development?
- How do you ensure the scalability of a web application?
- What are Progressive Web Apps (PWAs), and what benefits do they offer?
- What is the Document Object Model (DOM), and how does it relate to web development?
- Can you explain the concept of closures in JavaScript?
- What is the purpose of middleware in web development?
- How do you handle cross-browser compatibility issues in web development?
- What is the difference between HTTP and HTTPS?
- What is RESTful API, and how does it differ from SOAP?
- How does the virtual DOM work in React?
- What is the purpose of WebSockets, and how do they differ from HTTP?
- How do you optimize the performance of a web application?
- What is GraphQL, and how does it compare to REST?
- What is the purpose of a Content Delivery Network (CDN), and how does it work?
- How do you manage environment variables in a full-stack application?
- What is the role of WebAssembly in modern web development?
- How do you implement server-side rendering (SSR) in a React application?
- What are Web Workers, and how do they enhance web application performance?
- What is the purpose of a package manager in web development, and can you name a few commonly used ones?
- How do you implement authentication and authorization in a web application?
- What is the purpose of a build tool in web development, and can you name a few commonly used ones?
- What is the purpose of a build tool in web development, and can you name a few commonly used ones?
- How do you handle state management in a React application?
- What is the purpose of a linter in web development, and can you name a few commonly used ones?
1. What is Full-Stack Development?
Answer: Full-stack development refers to the practice of working on both the front-end and back-end portions of a web application. A full-stack developer is proficient in handling all aspects of development, including:
- Front-End Development: Involves creating the user interface and user experience elements using technologies like HTML, CSS, and JavaScript, along with frameworks such as React, Angular, or Vue.js.
- Back-End Development: Encompasses server-side logic, database interactions, and application architecture using languages and frameworks like Node.js, Python with Django or Flask, Ruby on Rails, or Java with Spring.
- Database Management: Involves designing, implementing, and managing databases using systems like MySQL, PostgreSQL, MongoDB, or Oracle.
- Version Control and Deployment: Utilizes tools like Git for version control and platforms such as Docker, Jenkins, or Kubernetes for deployment and continuous integration/continuous deployment (CI/CD) pipelines.
By mastering both client-side and server-side technologies, full-stack developers can build comprehensive web applications from start to finish.
2. Can you explain the Model-View-Controller (MVC) architecture?
Answer: The Model-View-Controller (MVC) architecture is a design pattern that separates an application into three interconnected components:
- Model: Represents the application’s data and business logic. It directly manages the data, logic, and rules of the application.
- View: Displays the data to the user and sends user commands to the controller. It is responsible for the presentation layer.
- Controller: Acts as an intermediary between the Model and View. It listens to user input from the View, processes it (often by invoking methods on the Model), and returns the output display to the View.
This separation facilitates modular development, making it easier to manage and scale applications.
3. What are RESTful APIs, and how do they work?
Answer: RESTful APIs (Representational State Transfer) are web services that adhere to REST architectural principles, enabling communication between client and server over HTTP. Key characteristics include:
- Statelessness: Each request from a client to a server must contain all the information needed to understand and process the request.
- Client-Server Architecture: Separates the user interface concerns from the data storage concerns, improving the portability of the user interface across multiple platforms.
- Uniform Interface: Defines a standardized way of communicating between client and server, typically using HTTP methods like GET, POST, PUT, DELETE, and PATCH.
- Resource-Based: Everything is considered a resource, identified by URIs (Uniform Resource Identifiers).
RESTful APIs are widely used due to their simplicity and scalability, allowing different systems to interact seamlessly.
4. How do you ensure the security of a web application?
Answer: Ensuring web application security involves implementing multiple layers of defense to protect against various threats:
- Input Validation: Sanitize and validate all user inputs to prevent injection attacks like SQL injection and cross-site scripting (XSS).
- Authentication and Authorization: Implement robust authentication mechanisms (e.g., multi-factor authentication) and enforce strict authorization checks to ensure users have appropriate access levels.
- Data Encryption: Use HTTPS to encrypt data in transit and apply encryption algorithms to protect data at rest.
- Secure Coding Practices: Follow best practices such as avoiding hard-coded credentials, using parameterized queries, and regularly updating dependencies to patch known vulnerabilities.
- Regular Security Audits: Conduct periodic security assessments, including vulnerability scanning and penetration testing, to identify and mitigate potential risks.
By adopting these practices, developers can significantly reduce the risk of security breaches.
5. What is the difference between SQL and NoSQL databases?
Answer: SQL (Structured Query Language) and NoSQL (Not Only SQL) databases differ in several key aspects:
Data Model:
- SQL Databases: Use a structured, table-based schema with predefined relationships.
- NoSQL Databases: Employ flexible schemas, including document, key-value, column-family, or graph models.
Scalability:
- SQL Databases: Typically scale vertically by increasing hardware resources.
- NoSQL Databases: Designed to scale horizontally by adding more servers.
Use Cases:
- SQL Databases: Ideal for complex queries and transactions requiring ACID (Atomicity, Consistency, Isolation, Durability) compliance.
- NoSQL Databases: Suitable for unstructured data, large-scale data storage, and applications requiring high scalability and flexibility.
Choosing between SQL and NoSQL depends on the specific requirements of the application, including data structure, scalability needs, and consistency requirements.
6. How do you optimize the performance of a web application?
Answer: Optimizing web application performance involves several strategies:
- Efficient Code: Write clean, efficient, and maintainable code to reduce execution time.
- Caching: Implement caching mechanisms (e.g., browser caching, server-side caching) to store frequently accessed data and reduce server load.
- Database Optimization: Use indexing, query optimization, and normalization to enhance database performance.
- Content Delivery Network (CDN): Utilize CDNs to distribute content geographically, reducing latency for users.
- Asynchronous Processing: Handle time-consuming tasks asynchronously to prevent blocking the main application thread.
- Minification and Compression: Minify CSS and JavaScript files and compress images to reduce load times.
Implementing these techniques can lead to faster response times and an improved user experience.
7. What is Cross-Origin Resource Sharing (CORS), and why is it important?
Answer: Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers to control how web pages can request resources from a different domain than the one that served the web page.
By default, web applications are restricted by the Same-Origin Policy, which prevents scripts on a web page from making requests to a different domain. CORS provides a way to relax this restriction securely.
Importance of CORS:
- Security: CORS helps prevent malicious websites from accessing sensitive data on another domain without explicit permission.
- Flexibility: It allows legitimate cross-domain requests, enabling functionalities like integrating third-party APIs or resources.
How CORS Works:
When a web page makes a cross-origin request, the browser sends an Origin
header indicating the source of the request. The server can respond with specific headers such as Access-Control-Allow-Origin
to indicate whether the request is allowed. If permitted, the browser allows the response; otherwise, it blocks it.
Proper implementation of CORS is crucial for web application security and functionality.
8. Can you explain the concept of Promises in JavaScript?
Answer: In JavaScript, a Promise is an object representing the eventual completion or failure of an asynchronous operation. It allows developers to write asynchronous code in a more synchronous and manageable fashion.
States of a Promise:
- Pending: Initial state; neither fulfilled nor rejected.
- Fulfilled: Operation completed successfully.
- Rejected: Operation failed.
Using Promises:
Promises can be created using the Promise
constructor and are typically consumed using .then()
for fulfilled cases and .catch()
for handling rejections.
Example:
let promise = new Promise((resolve, reject) => {
// Asynchronous operation
if (success) {
resolve(result);
} else {
reject(error);
}
});
promise
.then((result) => {
// Handle success
})
.catch((error) => {
// Handle error
});
Promises enhance code readability and maintainability, especially when dealing with multiple asynchronous operations.
9. What is the purpose of Node.js, and how does it work?
Answer: Node.js is a runtime environment that allows developers to execute JavaScript code on the server side. Built on Chrome’s V8 JavaScript engine, it enables the development of scalable and high-performance network applications.
Key Features:
- Event-Driven Architecture: Utilizes an event loop to handle asynchronous operations, making it efficient for I/O-bound tasks.
- Non-Blocking I/O: Performs I/O operations asynchronously, allowing the server to handle multiple requests concurrently without blocking the execution thread.
- Single-Threaded: Despite being single-threaded, Node.js can manage numerous connections simultaneously through its event-driven model.
Use Cases:
- Real-time applications (e.g., chat applications, live streaming).
- APIs for mobile and web applications.
- Microservices architecture.
Node.js’s efficiency and scalability make it a popular choice for modern web development.
10. How do you manage state in a React application?
Answer: Managing state in a React application is crucial for maintaining data consistency and ensuring a responsive user interface. State can be managed at different levels:
- Component-Level State: Managed using the
useState
hook in functional components orthis.state
in class components. Suitable for local state specific to a component. - Context API: Provides a way to pass data through the component tree without having to pass props down manually at every level. Useful for global state that needs to be accessed by multiple components.
- State Management Libraries: Tools like Redux or MobX offer more robust solutions for complex state management needs, especially in large applications. They provide a centralized store and mechanisms to handle state changes predictably.
Choosing the appropriate state management approach depends on the application’s complexity and specific requirements.
11. What is the difference between synchronous and asynchronous programming?
Answer: Synchronous and asynchronous programming define how tasks are executed in a program:
- Synchronous Programming: Tasks are performed sequentially; each task waits for the previous one to complete before starting. This can lead to blocking, where a time-consuming task halts the execution of subsequent tasks.
- Asynchronous Programming: Tasks can be initiated and continue running in the background, allowing the program to proceed without waiting for the task to finish. This is achieved through callbacks, promises, or async/await syntax, enabling non-blocking operations.
Asynchronous programming is essential for improving performance and responsiveness, especially in I/O-bound operations like network requests or file system access.
12. How do you handle errors in a web application?
Answer: Effective error handling in a web application involves:
- Client-Side Error Handling:
- Implementing try-catch blocks in JavaScript to catch exceptions.
- Using error boundaries in React to catch errors in the component tree.
- Displaying user-friendly error messages and providing options to retry or navigate to a safe state.
- Server-Side Error Handling:
- Validating and sanitizing inputs to prevent errors.
- Using structured exception handling to catch and log errors.
- Returning appropriate HTTP status codes and messages to inform the client of errors.
- Logging and Monitoring:
- Implementing logging mechanisms to record errors for debugging purposes.
- Setting up monitoring tools to detect and alert on errors in real-time.
Proactive error handling enhances application stability and user experience.
13. What are microservices, and how do they differ from monolithic architectures?
Answer: Microservices are an architectural style that structures an application as a collection of small, autonomous services, each responsible for a specific business capability. These services communicate over well-defined APIs and can be developed, deployed, and scaled independently.
Differences between Microservices and Monolithic Architectures:
Structure:
- Monolithic Architecture: The entire application is built as a single, unified unit where all components are interconnected and interdependent.
- Microservices Architecture: The application is divided into multiple independent services, each handling a specific function.
Development and Deployment:
- Monolithic: Requires the entire application to be redeployed for any change, making the process cumbersome.
- Microservices: Allows individual services to be developed, tested, and deployed independently, facilitating continuous integration and continuous deployment (CI/CD).
Scalability:
- Monolithic: Scaling requires duplicating the entire application, which can be resource-intensive.
- Microservices: Enables scaling of individual services based on demand, optimizing resource utilization.
Technology Stack:
- Monolithic: Typically constrained to a single technology stack throughout the application.
- Microservices: Permits the use of diverse technologies and languages for different services, allowing teams to choose the best tools for specific tasks.
Fault Isolation:
- Monolithic: A failure in one component can potentially bring down the entire application.
- Microservices: Failures are isolated to individual services, reducing the risk of a complete system outage.
While microservices offer advantages in scalability, flexibility, and resilience, they also introduce complexities in terms of service coordination, data consistency, and increased operational overhead. Choosing between monolithic and microservices architectures depends on the specific needs, scale, and resources of the project.
14. What is the purpose of Docker, and how does it work?
Answer: Docker is an open-source platform designed to automate the deployment, scaling, and management of applications using containerization. Containers encapsulate an application and its dependencies into a standardized unit, ensuring consistency across various environments.
Purpose of Docker:
- Consistency: Ensures applications run the same way across different environments, eliminating the “it works on my machine” problem.
- Isolation: Provides isolated environments for applications, preventing conflicts between dependencies.
- Efficiency: Containers are lightweight and share the host system’s kernel, allowing for efficient resource utilization.
How Docker Works:
- Images: Docker images are read-only templates that contain the application’s code, runtime, libraries, and dependencies. They serve as the blueprint for creating containers.
- Containers: A container is a runnable instance of a Docker image. Containers are isolated from each other and the host system but can communicate through defined channels.
- Docker Engine: The core component that enables the creation, management, and execution of Docker containers.
- Dockerfile: A script containing a series of instructions on how to build a Docker image. It defines the application’s environment and dependencies.
Workflow:
- Developers write a
Dockerfile
specifying the application’s environment. - Docker uses this file to build an image.
- The image is then deployed as a container in any environment that supports Docker.
By leveraging Docker, developers can achieve consistent and efficient application deployment across various platforms.
15. How do you implement authentication and authorization in a web application?
Answer: Implementing authentication and authorization is crucial for securing a web application.
Authentication: Verifies the identity of a user.
- Methods:
- Username and Password: The most common method where users provide credentials.
- OAuth: Allows users to authenticate using third-party services like Google or Facebook.
- Multi-Factor Authentication (MFA): Combines multiple verification methods, such as passwords and one-time codes.
- Implementation Steps:
- Collect user credentials securely.
- Validate credentials against stored data.
- Generate a session or token upon successful validation.
- Store session information securely (e.g., using HTTP-only cookies or secure tokens).
Authorization: Determines what authenticated users are allowed to do.
- Role-Based Access Control (RBAC): Assigns permissions to roles, and users are assigned roles.
- Implementation Steps:
- Define roles and associated permissions.
- Assign roles to users.
- Check user roles before granting access to resources or actions.
Best Practices:
- Use secure protocols (e.g., HTTPS) to protect data in transit.
- Store passwords securely using hashing algorithms like bcrypt.
- Implement proper session management to prevent hijacking.
- Regularly update and patch authentication mechanisms to address vulnerabilities.
By effectively implementing authentication and authorization, developers can ensure that only legitimate users have appropriate access to the application’s resources.
16. What is Continuous Integration and Continuous Deployment (CI/CD), and why are they important?
Answer: Continuous Integration (CI) and Continuous Deployment (CD) are pivotal practices in modern software development, aiming to enhance code quality and expedite the delivery process.
Continuous Integration (CI):
CI involves the frequent integration of code changes into a shared repository, followed by automated builds and testing.
- Purpose:
- Detect integration issues early.
- Ensure that new code changes do not disrupt the existing application.
- Benefits:
- Reduces integration problems.
- Encourages collaborative development.
- Maintains a consistently deployable codebase.
Continuous Deployment (CD):
CD extends CI by automating the release of code changes to production environments.
- Purpose:
- Deliver new features and fixes to users rapidly.
- Maintain a continuous flow of updates.
- Benefits:
- Accelerates time-to-market.
- Reduces manual deployment errors.
- Enhances user satisfaction through timely updates.
Importance of CI/CD:
Implementing CI/CD pipelines fosters a culture of continuous improvement, allowing teams to respond swiftly to feedback and evolving requirements. This approach not only improves software quality but also aligns development processes with business objectives, ensuring a competitive edge in the market.
17. How do you handle state management in a React application?
Answer: State management in React is crucial for maintaining data consistency and ensuring a responsive user interface. React offers several approaches to manage state effectively:
- Component-Level State: Utilize the
useState
hook in functional components orthis.state
in class components to manage local state specific to a component. - Context API: React’s Context API allows for the creation of global state accessible by multiple components, eliminating the need for prop drilling. It’s suitable for managing themes, user authentication, and other global data.
- State Management Libraries: For complex applications, libraries like Redux or MobX provide robust solutions. They offer a centralized store and predictable state transitions, facilitating easier debugging and testing.
- React Query: For managing server state, React Query simplifies data fetching, caching, and synchronization with the server, reducing the need for manual state management.
Choosing the appropriate state management strategy depends on the application’s complexity and specific requirements.
18. What is the purpose of Webpack in modern web development?
Answer: Webpack is a powerful module bundler for JavaScript applications. It processes and compiles modules (JavaScript files, CSS, images, etc.) into a single or multiple bundles, optimizing them for browser consumption.
Key Features:
- Module Bundling: Combines various modules and their dependencies into cohesive bundles.
- Code Splitting: Allows splitting code into smaller chunks, enabling lazy loading and improving application performance.
- Loaders and Plugins: Utilizes loaders to preprocess files (e.g., transpiling ES6 to ES5 using Babel) and plugins to extend functionality (e.g., minification, environment variables).
Purpose in Modern Web Development:
- Optimization: Reduces file sizes and load times through minification and compression.
- Compatibility: Transpiles modern JavaScript syntax to ensure compatibility across different browsers.
- Development Experience: Offers features like hot module replacement, enhancing the development workflow.
By leveraging Webpack, developers can create efficient, maintainable, and high-performance web applications.
19. How do you ensure the scalability of a web application?
Answer: Ensuring the scalability of a web application involves designing and implementing strategies that allow the application to handle increased load and user demand effectively. Key considerations include:
Architecture Design:
- Microservices: Decompose the application into independent services that can be developed, deployed, and scaled individually.
- Load Balancing: Distribute incoming traffic across multiple servers to prevent any single server from becoming a bottleneck.
Database Optimization:
- Sharding: Partition the database to distribute data across multiple servers.
- Caching: Implement caching mechanisms (e.g., Redis, Memcached) to reduce database load.
Asynchronous Processing:
- Message Queues: Use systems like RabbitMQ or Kafka to handle background tasks and reduce synchronous processing load.
Horizontal Scaling:
- Auto-Scaling: Deploy additional instances of services automatically based on demand.
Performance Monitoring:
- Monitoring Tools: Utilize tools to track performance metrics and identify bottlenecks.
By incorporating these strategies, developers can build applications that maintain performance and reliability under varying loads.
20. What are Progressive Web Apps (PWAs), and what benefits do they offer?
Answer: Progressive Web Apps (PWAs) are web applications that leverage modern web technologies to deliver an app-like experience to users. They combine the best features of web and mobile applications.
Key Characteristics:
- Responsive: Adapt to various screen sizes and orientations.
- Offline Capability: Utilize service workers to cache resources, allowing functionality without an internet connection.
- App-Like Feel: Provide a user experience similar to native apps, including smooth navigation and interactions.
- Installable: Can be added to the user’s home screen without the need for an app store.
Benefits:
- Improved Performance: Faster load times and smoother interactions enhance user satisfaction.
- Increased Engagement: Features like push notifications and offline access keep users engaged.
- Cost-Effective Development: Developing a single PWA can serve both web and mobile users, reducing development and maintenance costs.
By adopting PWAs, businesses can provide a seamless and engaging user experience across different platforms.
21. What is the Document Object Model (DOM), and how does it relate to web development?
Answer: The Document Object Model (DOM) is a programming interface for web documents. It represents the structure of a document as a tree of objects, allowing programs and scripts to dynamically access and update the content, structure, and style of web pages.
Key Aspects:
- Structure Representation: The DOM represents the document’s structure as a tree of nodes, where each node corresponds to a part of the document, such as elements, attributes, and text.
- Language Agnostic: While commonly associated with JavaScript, the DOM can be manipulated using various programming languages.
- Dynamic Interaction: Developers can use the DOM to create, delete, or modify elements and attributes, enabling dynamic content updates without reloading the page.
Relation to Web Development:
In web development, the DOM is crucial for creating interactive and dynamic web pages. JavaScript, in particular, interacts with the DOM to manipulate HTML and CSS, allowing developers to respond to user events, validate forms, and update content dynamically. Understanding the DOM is essential for tasks such as:
- Event Handling: Attaching event listeners to elements to respond to user actions like clicks or key presses.
- Content Manipulation: Adding, removing, or modifying elements and their attributes to update the user interface.
- Styling Changes: Dynamically altering CSS properties to change the appearance of elements based on user interactions or other conditions.
A solid grasp of the DOM enables developers to build responsive and interactive web applications that enhance user experience.
22. Can you explain the concept of closures in JavaScript?
Answer: In JavaScript, a closure is a function that has access to its own scope, the outer function’s scope, and the global scope. This means a closure can access variables defined within its own scope, variables defined in the outer function’s scope, and variables in the global scope.
Key Characteristics:
- Function within a Function: Closures are created when a function is defined inside another function, allowing the inner function to access the outer function’s variables.
- Persistent Scope: Even after the outer function has executed and returned, the inner function retains access to the outer function’s variables.
Example:
function outerFunction(outerVariable) {
return function innerFunction(innerVariable) {
console.log('Outer Variable:', outerVariable);
console.log('Inner Variable:', innerVariable);
};
}
const newFunction = outerFunction('outside');
newFunction('inside');
Output:
Outer Variable: outside
Inner Variable: inside
Use Cases:
- Data Privacy: Closures can be used to create private variables that are not accessible from the global scope.
- Function Factories: Generating functions with preset parameters.
- Event Handlers: Maintaining state between event handler executions.
Understanding closures is essential for mastering JavaScript, as they are fundamental to many advanced patterns and techniques in the language.
23. What is the purpose of middleware in web development?
Answer: In web development, middleware refers to functions that execute during the request-response cycle of an application. Middleware functions have access to the request and response objects and can modify them, end the request-response cycle, or pass control to the next middleware function.
Purpose of Middleware:
- Request Processing: Middleware can parse request bodies, handle cookies, and manage sessions.
- Response Modification: Middleware can modify response headers, set cookies, or compress response data.
- Authentication and Authorization: Middleware can verify user credentials and permissions before granting access to certain routes or resources.
- Error Handling: Middleware can catch and handle errors, providing a centralized mechanism for error management.
Example in Express.js:
const express = require('express');
const app = express();
// Middleware function
function logger(req, res, next) {
console.log(`${req.method} ${req.url}`);
next(); // Pass control to the next middleware
}
app.use(logger);
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000);
In this example, the logger
middleware logs the HTTP method and URL of each request before passing control to the next middleware or route handler.
Middleware enhances modularity and reusability in web applications by allowing developers to separate concerns and apply specific functions across multiple routes or the entire application.
24. How do you handle cross-browser compatibility issues in web development?
Answer: Ensuring that a web application functions consistently across different browsers is a critical aspect of web development. Cross-browser compatibility issues arise due to variations in how browsers interpret HTML, CSS, and JavaScript.
Strategies to Handle Cross-Browser Compatibility:
- Use Standardized Code: Adhere to web standards set by organizations like the World Wide Web Consortium (W3C) to ensure consistent behavior across browsers.
- CSS Resets and Normalization: Implement CSS resets or normalization stylesheets to reduce inconsistencies in default styling across browsers.
- Feature Detection: Utilize libraries like Modernizr to detect browser capabilities and apply polyfills or alternative solutions for unsupported features.
- Progressive Enhancement: Build the core functionality using technologies supported by all browsers, and add advanced features that enhance the experience in browsers that support them.
- Testing Across Browsers: Regularly test the application in multiple browsers and devices to identify and address compatibility issues early in the development process.
- Use of Polyfills: Incorporate polyfills to add support for features that are not natively available in certain browsers.
By implementing these strategies, developers can create web applications that provide a consistent and functional experience for users, regardless of their choice of browser.
25. What is the difference between HTTP and HTTPS?
Answer: HTTP (Hypertext Transfer Protocol) and HTTPS (Hypertext Transfer Protocol Secure) are protocols used for transmitting data over the internet.
Key Differences:
- Security:
- HTTP: Transmits data in plain text, making it susceptible to interception and tampering
- HTTPS: Encrypts data using SSL/TLS protocols, providing secure communication
- Port:
- HTTP: Uses port 80 by default
- HTTPS: Uses port 443 by default
- URL Format:
- HTTP: Starts with “http://”
- HTTPS: Starts with “https://”
- Authentication:
- HTTP: No authentication of the website’s identity
- HTTPS: Uses SSL certificates to verify website authenticity
- Speed:
- HTTP: Slightly faster due to no encryption overhead
- HTTPS: Marginally slower due to encryption/decryption processes
- SEO Impact:
- HTTP: May negatively impact search rankings
- HTTPS: Preferred by search engines, can improve SEO rankings
- Usage:
- HTTP: Suitable for non-sensitive information
- HTTPS: Required for sensitive data (passwords, payment info, personal details)
26. What is RESTful API, and how does it differ from SOAP?
Answer:
A RESTful API (Representational State Transfer) is an architectural style for designing networked applications. It utilizes standard HTTP methods and is stateless, meaning each request from a client contains all the information needed for the server to fulfill that request.
Key Characteristics of RESTful APIs:
- Statelessness: Each request is independent; the server doesn’t store client context between requests.
- Resource-Based: Operations are performed on resources identified by URLs.
- Use of Standard HTTP Methods: Employs methods like GET, POST, PUT, DELETE to perform CRUD operations.
SOAP (Simple Object Access Protocol):
SOAP is a protocol for exchanging structured information in web services. It relies on XML-based messaging and can operate over various lower-level protocols, not just HTTP.
Differences Between RESTful APIs and SOAP:
- Protocol vs. Architectural Style: SOAP is a protocol with strict standards, while REST is an architectural style offering more flexibility.
- Message Format: SOAP uses XML exclusively, whereas REST can use multiple formats, including JSON, XML, and plain text.
- Complexity: SOAP is more complex due to its strict standards and extensive features, while REST is simpler and more lightweight.
- Statefulness: SOAP can be stateful or stateless, whereas REST is inherently stateless.
Choosing between RESTful APIs and SOAP depends on the specific requirements of the application, such as the need for standardized security features (favoring SOAP) or the desire for simplicity and scalability (favoring REST).
27. How does the virtual DOM work in React?
Answer:
In React, the virtual DOM is an in-memory representation of the real DOM elements generated by React components. It allows React to efficiently update and render components by minimizing direct manipulation of the actual DOM.
How It Works:
- Rendering: When a component’s state or props change, React creates a new virtual DOM tree.
- Diffing: React compares the new virtual DOM with the previous one to identify changes (a process known as “diffing”).
- Reconciliation: React updates only the parts of the real DOM that have changed, rather than re-rendering the entire DOM.
Benefits:
- Performance: By reducing direct DOM manipulations, React enhances application performance, especially in complex interfaces.
- Predictability: The virtual DOM provides a predictable and efficient way to manage UI updates.
Understanding the virtual DOM is crucial for optimizing React applications and ensuring efficient rendering.
28. What is the purpose of WebSockets, and how do they differ from HTTP?
Answer:
WebSockets provide a full-duplex communication channel over a single, long-lived connection between a client and server. This enables real-time data exchange, making them ideal for applications like chat systems, live feeds, and online gaming.
Differences Between WebSockets and HTTP:
Communication Model:
- HTTP: Follows a request-response model; the client sends a request, and the server responds.
- WebSockets: Establishes a persistent connection, allowing both client and server to send data independently at any time.
Connection Persistence:
- HTTP: Connections are short-lived and closed after each request-response cycle.
- WebSockets: Connections remain open, facilitating continuous data exchange.
Overhead:
- HTTP: Each request includes headers, leading to higher overhead.
- WebSockets: After the initial handshake, data frames have minimal overhead, making communication more efficient.
WebSockets are particularly beneficial for applications requiring low-latency, real-time interactions, whereas HTTP is suitable for standard request-response scenarios.
29. How do you optimize the performance of a web application?
Answer:
Optimizing web application performance involves several strategies:
Front-End Optimization:
- Minification: Reduce the size of CSS, JavaScript, and HTML files by removing unnecessary characters.
- Image Optimization: Compress images without compromising quality.
- Lazy Loading: Defer loading of non-critical resources until needed.
- Caching: Implement browser caching to store static resources locally.
Back-End Optimization:
- Database Indexing: Use indexes to speed up query performance.
- Load Balancing: Distribute incoming traffic across multiple servers.
- Asynchronous Processing: Handle time-consuming tasks asynchronously to prevent blocking.
Network Optimization:
- Content Delivery Networks (CDNs): Serve content from servers closer to the user.
- HTTP/2: Utilize HTTP/2 for multiplexing and header compression.
Regular performance monitoring and profiling are essential to identify bottlenecks and ensure the application remains responsive and efficient.
30. What is GraphQL, and how does it compare to REST?
Answer: GraphQL is a query language for APIs and a runtime for executing those queries. It allows clients to request exactly the data they need, making APIs more efficient and flexible.
Comparison with REST:
Data Fetching:
- REST: Clients may over-fetch or under-fetch data due to fixed endpoints.
- GraphQL: Clients specify precisely the data they require, reducing unnecessary data transfer.
Endpoint Structure:
- REST: Multiple endpoints for different resources.
- GraphQL: Single endpoint handling various queries and mutations.
Versioning:
- REST: Often requires versioning to manage changes.
- GraphQL: Evolves without versioning by adding new fields and deprecating old ones.
GraphQL offers a more efficient and flexible approach to data retrieval compared to REST, especially in complex applications with diverse data requirements.
31. What is the purpose of a Content Delivery Network (CDN), and how does it work?
Answer: A Content Delivery Network (CDN) is a system of distributed servers strategically placed across various geographic locations to deliver web content to users more efficiently. The primary purpose of a CDN is to reduce latency and improve load times by serving content from a server closest to the user’s location.
How a CDN Works:
- Content Replication: The origin server’s content, such as images, videos, stylesheets, and scripts, is replicated and cached across multiple CDN servers worldwide.
- User Request Routing: When a user requests content, the CDN directs the request to the nearest server in its network, minimizing the distance data must travel.
- Content Delivery: The CDN server delivers the cached content to the user, resulting in faster load times and reduced bandwidth usage on the origin server.
Benefits of Using a CDN:
- Improved Performance: By serving content from geographically closer servers, CDNs reduce latency and enhance user experience.
- Scalability: CDNs can handle large volumes of traffic, preventing server overloads during high-demand periods.
- Reliability: With multiple servers, CDNs provide redundancy, ensuring content availability even if one server fails.
- Security: Many CDNs offer security features like DDoS protection and secure content delivery.
Implementing a CDN is a strategic approach to optimize web application performance, especially for a global user base.
32. How do you manage environment variables in a full-stack application?
Answer: Environment variables are key-value pairs used to configure applications across different environments (development, testing, production) without altering the codebase. They store sensitive information like database credentials, API keys, and configuration settings.
Managing Environment Variables:
- Environment Files: Use files like
.env
to define variables. These files are read at runtime, and variables are injected into the application’s environment. - Environment-Specific Files: Create separate files for different environments (e.g.,
.env.development
,.env.production
) and load the appropriate one based on the deployment context. - Environment Variable Libraries: Utilize libraries such as
dotenv
in Node.js to load variables from.env
files intoprocess.env
. - Secure Storage: Avoid committing
.env
files to version control. Instead, use tools like Git’s.gitignore
to exclude them and manage secrets securely. - Deployment Platforms: Many platforms (e.g., Heroku, AWS) offer built-in mechanisms to set environment variables through their interfaces or CLI tools.
Best Practices:
- Consistency: Ensure variable names are consistent across environments to prevent misconfigurations.
- Documentation: Maintain clear documentation of required environment variables and their purposes.
- Security: Handle sensitive information carefully, using encryption and access controls as needed.
Proper management of environment variables enhances application security, flexibility, and maintainability across different deployment scenarios.
33. What is the role of WebAssembly in modern web development?
Answer: WebAssembly (Wasm) is a binary instruction format designed as a portable compilation target for high-level languages like C, C++, and Rust. It enables the execution of high-performance applications on web browsers, complementing JavaScript.
Roles of WebAssembly:
- Performance: Allows near-native execution speed for compute-intensive tasks, enhancing performance for applications like games, simulations, and image processing.
- Language Support: Enables developers to write code in languages other than JavaScript and run it on the web, broadening the scope of web development.
- Interoperability: Works alongside JavaScript, allowing seamless integration and leveraging existing web APIs.
- Security: Operates within the browser’s security sandbox, ensuring safe execution of code.
WebAssembly is transforming web development by enabling complex applications to run efficiently in the browser, reducing the need for native applications.
34. How do you implement server-side rendering (SSR) in a React application?
Answer: Server-Side Rendering (SSR) in React involves rendering components on the server and sending the fully rendered HTML to the client. This approach improves performance and SEO by delivering content faster and making it accessible to search engines.
Implementing SSR in React:
- Set Up a Node.js Server: Use frameworks like Express.js to handle incoming requests.
- Render React Components on the Server: Utilize
ReactDOMServer.renderToString()
to convert React components into HTML strings. - Send Rendered HTML to the Client: Embed the rendered HTML into a template and send it as the response.
- Hydrate on the Client: On the client side, use
ReactDOM.hydrate()
to attach React’s event listeners to the server-rendered HTML, enabling full interactivity.
Example:
// Server.js
import express from 'express';
import React from 'react';
import ReactDOMServer from 'react-dom/server';
import App from './App';
const app = express();
app.get('*', (req, res) => {
const appString = ReactDOMServer.renderToString(<App />);
res.send(`
<!DOCTYPE html>
<html>
<head><title>SSR with React</title></head>
<body>
<div id="root">${appString}</div>
<script src="/bundle.js"></script>
</body>
</html>
`);
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Considerations:
- Data Fetching: Handle data fetching on the server to render components with the necessary data.
- Routing: Use libraries like React Router with SSR support to manage routing on both server and client.
- Code Splitting: Implement code splitting carefully to ensure all necessary code is available during server rendering.
SSR enhances performance and SEO but adds complexity to the application architecture. Tools like Next.js provide built-in SSR support, simplifying the implementation process.
35. What are Web Workers, and how do they enhance web application performance?
Answer: Web Workers are a feature of modern web browsers that allow JavaScript code to run in the background, separate from the main execution thread of a web application. This enables the execution of complex or time-consuming tasks without blocking the user interface, thereby enhancing the performance and responsiveness of web applications.
Key Characteristics of Web Workers:
- Background Execution: Web Workers operate on a separate thread from the main JavaScript execution thread, allowing tasks to run concurrently without interfering with the user interface.
- No Direct DOM Access: Web Workers do not have direct access to the Document Object Model (DOM) to prevent race conditions and ensure thread safety. They communicate with the main thread through a messaging system.
- Use Cases: Ideal for tasks such as data processing, computations, and handling large datasets that would otherwise slow down the main thread.
How Web Workers Enhance Performance:
- Non-Blocking Operations: By offloading intensive tasks to Web Workers, the main thread remains free to handle user interactions and render updates, leading to a smoother user experience.
- Parallel Processing: Web Workers enable parallel execution of scripts, making efficient use of multi-core processors and improving the overall performance of web applications.
Example Usage:
To create a Web Worker, you define a separate JavaScript file for the worker’s tasks and instantiate it in your main script:
// main.js
const worker = new Worker('worker.js');
worker.onmessage = function(event) {
console.log('Received from worker:', event.data);
};
worker.postMessage('Start processing');
// worker.js
self.onmessage = function(event) {
// Perform time-consuming task
const result = performComplexCalculation();
self.postMessage(result);
};
In this example, worker.js
performs a complex calculation without blocking the main thread, and the result is sent back to main.js
through messaging.
By leveraging Web Workers, developers can create more responsive and efficient web applications, especially when dealing with resource-intensive operations.
36. What is the purpose of a package manager in web development, and can you name a few commonly used ones?
Answer: A package manager is a tool that automates the process of installing, updating, configuring, and managing software packages or libraries. In web development, package managers streamline the inclusion of external libraries and dependencies, ensuring that projects are consistent and manageable.
Purpose of a Package Manager:
- Dependency Management: Automatically handles the installation and updating of project dependencies, including their specific versions.
- Version Control: Maintains consistent versions of packages across different environments, reducing the risk of compatibility issues.
- Simplified Installation: Allows developers to easily add or remove packages using simple commands, enhancing productivity.
- Community Access: Provides access to a vast repository of open-source packages and libraries, fostering code reuse and collaboration.
Commonly Used Package Managers:
- npm (Node Package Manager): The default package manager for Node.js, widely used for managing JavaScript packages.
- Yarn: An alternative to npm, known for its speed and reliability in managing JavaScript dependencies.
- pip: The package installer for Python, used to install and manage Python libraries and dependencies.
- Composer: A dependency manager for PHP, facilitating the management of project libraries and packages.
Utilizing package managers is essential in modern web development to efficiently manage project dependencies, ensure consistency across development environments, and leverage the vast ecosystem of open-source libraries.
37. How do you implement authentication and authorization in a web application?
Answer: Authentication and authorization are critical components of web application security.
- Authentication: The process of verifying the identity of a user or system.
- Authorization: Determining the permissions or access levels granted to an authenticated user.
Implementing Authentication:
- User Registration: Collect user credentials (e.g., username, password) and securely store them, typically using hashing algorithms for passwords.
- Login Process: Verify user credentials during login attempts by comparing the provided information with stored data.
- Session Management: Maintain user sessions using cookies, tokens (e.g., JSON Web Tokens), or server-side sessions to track authenticated users.
Implementing Authorization:
- Role-Based Access Control (RBAC): Assign roles to users (e.g., admin, user) and define permissions for each role.
- Access Control Lists (ACLs): Specify granular permissions for individual users or groups for specific resources or actions.
- Policy-Based Access Control: Define policies that determine access based on attributes like user role, resource type, and action.
Best Practices:
- Secure Password Storage: Use strong hashing algorithms (e.g., bcrypt) to store passwords securely.
- Multi-Factor Authentication (MFA): Implement MFA to add an extra layer of security.
- Token Expiry: Set appropriate expiration times for tokens to reduce the risk of unauthorized access.
- Least Privilege Principle: Grant users the minimum permissions necessary to perform their tasks.
By implementing robust authentication and authorization mechanisms, developers can protect web applications from unauthorized access and ensure that users have appropriate permissions based on their roles and responsibilities.
38. What is the purpose of a build tool in web development, and can you name a few commonly used ones?
Answer: A build tool is a software application that automates various tasks involved in the development process, making it more efficient and reliable. In web development, build tools primarily focus on the following:
Automation of Repetitive Tasks:
- Compilation: Translating code written in one language (e.g., TypeScript, Sass) into another (e.g., JavaScript, CSS).
- Minification: Removing unnecessary characters from code to reduce file size and improve loading speed.
- Bundling: Combining multiple files into a single file to reduce the number of HTTP requests.
- Testing: Running automated tests to ensure code quality.
- Deployment: Deploying the final product to a web server.
Dependency Management:
- Managing and resolving dependencies (external libraries and frameworks) used in a project.
- Ensuring that the correct versions of dependencies are used and that conflicts are avoided.
Optimization:
- Applying various optimization techniques to improve the performance of the final product, such as:
- Tree-shaking: Removing unused code from bundles.
- Code splitting: Breaking large bundles into smaller chunks to improve initial load time.
- Caching: Implementing caching strategies to reduce server load and improve user experience.
Commonly Used Build Tools:
- Webpack: A powerful module bundler that can be used to bundle JavaScript, CSS, and other assets.
- Gulp: A flexible build tool that uses a streaming pipeline to automate tasks.
- Grunt: A configuration-based build tool that provides a wide range of tasks and plugins.
- Parcel: A fast, zero-configuration build tool that focuses on simplicity and performance.
- Rollup: A module bundler that is particularly well-suited for creating libraries and frameworks.
By using a build tool, developers can save time, reduce errors, and improve the overall quality of their web applications.
39. What is the purpose of a build tool in web development, and can you name a few commonly used ones?
Answer: In web development, a build tool automates tasks involved in transforming source code into a final, deployable product. These tasks include compiling code, minifying assets, bundling modules, running tests, and optimizing performance. By automating these processes, build tools enhance efficiency, consistency, and reliability in the development workflow.
Commonly Used Build Tools:
- Webpack: A powerful module bundler for JavaScript applications. It processes and bundles JavaScript files along with their dependencies, and can also handle assets like CSS and images.
- Gulp: A task runner that uses Node.js streams to automate tasks such as file minification, compilation, and browser reloading.
- Grunt: A JavaScript task runner that automates repetitive tasks like minification, compilation, and testing.
- Parcel: A zero-configuration web application bundler that supports HTML, JavaScript, CSS, and more, offering fast performance and simplicity.
Utilizing build tools is essential in modern web development to streamline workflows, enforce best practices, and produce optimized, production-ready code.
40. How do you handle state management in a React application?
Answer: State management in a React application involves handling the data that influences the rendering and behavior of components. Effective state management ensures that the application remains predictable, maintainable, and scalable.
Approaches to State Management:
- Component-Level State: Manage state within individual components using React’s
useState
hook for functional components orthis.state
in class components. This approach is suitable for local state that doesn’t need to be shared across components. - Context API: React’s Context API allows for sharing state across multiple components without prop drilling. It’s useful for global state that doesn’t require complex logic.
- State Management Libraries:
- Redux: A predictable state container for JavaScript applications, offering a centralized store and strict unidirectional data flow.
- MobX: A library that makes state management simple and scalable by using observable data structures.
- React Query: A library for fetching, caching, and synchronizing server state in React applications, simplifying data fetching logic.
Best Practices:
- Lift State Up: When multiple components need access to the same state, lift the state to the nearest common ancestor.
- Keep State Minimal: Store only necessary data in the state to reduce complexity and improve performance.
- Immutable Updates: Always create new copies of state objects when updating to ensure predictable behavior and enable features like time-travel debugging.
Choosing the appropriate state management approach depends on the application’s complexity and specific requirements. For simple applications, React’s built-in state and Context API may suffice, while more complex applications might benefit from libraries like Redux or MobX.
41. What is the purpose of a linter in web development, and can you name a few commonly used ones?
Answer: A linter is a tool that analyzes source code to identify and report potential errors, stylistic inconsistencies, and code quality issues. In web development, linters help maintain code quality, enforce coding standards, and catch errors early in the development process.
Commonly Used Linters:
- ESLint: A widely used linter for JavaScript and TypeScript, configurable to enforce various coding standards and detect potential issues.
- JSHint: A static code analysis tool for JavaScript, designed to detect errors and potential problems in code.
- Stylelint: A linter for CSS and SCSS that helps enforce consistent conventions and avoid errors in stylesheets.
- TSLint: A linter for TypeScript, though it’s being deprecated in favor of ESLint with TypeScript support.
Benefits of Using Linters:
- Error Detection: Identify syntax errors, potential bugs, and problematic patterns before runtime.
- Code Consistency: Enforce consistent coding styles and conventions across a codebase, improving readability and maintainability.
- Improved Code Quality: Encourage best practices and adherence to coding standards, leading to more robust and reliable code.
Integrating linters into the development workflow, often through code editors or build processes, helps developers catch issues early, maintain high code quality, and adhere to team or project coding standards.
Learn More: Carrer Guidance
Avasoft Interview Questions and answers- Basic to Advanced
Deloitte NLA Interview Questions and Answers
ADF Interview Questions Scenario based Questions with detailed Answers
Generative AI System Design Interview Questions and Answers- Basic to Advanced
Business Development Executive Interview Questions and Answers
ServiceNow Interview Questions and Answers for Experienced
ServiceNow Interview Questions and Answers-Freshers