If you’re building a React application and encounter an error like this: Module not found: Can’t resolve ‘web-vitals’, don’t worry, we’ll fix it. This error typically occurs when your project attempts to import the web-vitals package in your reportWebVitals.js file, but the package isn’t installed in your dependencies.
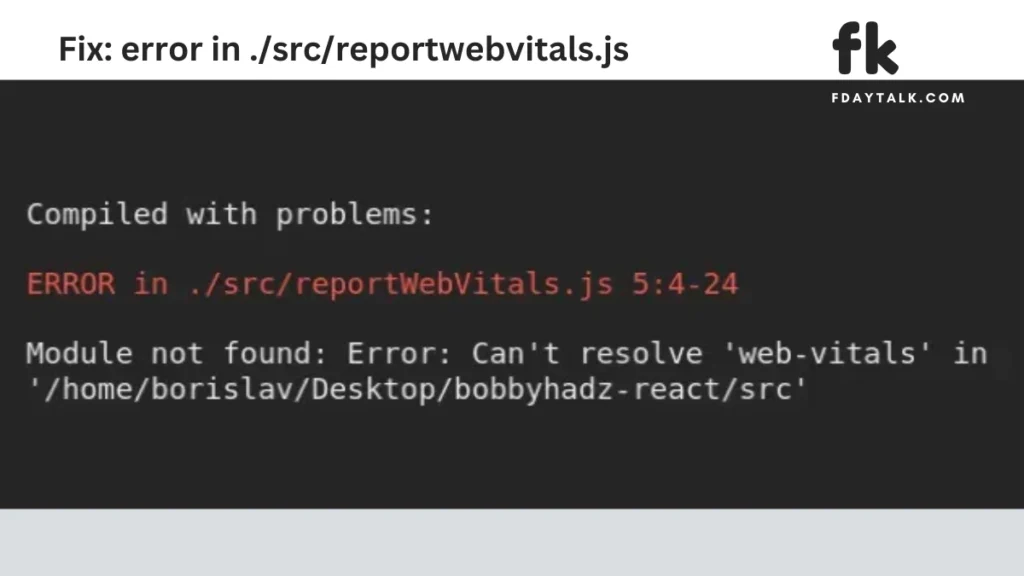
In this article, we’ll walk through the reasons behind this error and provide a step-by-step guide to resolving it.
What is React Module not found: Can’t resolve web-vitals Error
Modern React applications, especially those generated with Create React App, include a file called reportWebVitals.js
. This file is designed to help you measure and analyze your application’s performance metrics using the web-vitals package. A typical snippet from this file might look like this:
const reportWebVitals = (onPerfEntry) => {
if (onPerfEntry && onPerfEntry instanceof Function) {
import('web-vitals').then(({ getCLS, getFID, getFCP, getLCP, getTTFB }) => {
getCLS(onPerfEntry);
getFID(onPerfEntry);
getFCP(onPerfEntry);
getLCP(onPerfEntry);
getTTFB(onPerfEntry);
});
}
};
export default reportWebVitals;
When the package web-vitals is missing, the dynamic import fails, leading to the error:
Module not found: Can't resolve 'web-vitals'
Steps to Resolve the Error
Follow these simple steps to fix the issue:
1. Install the web-vitals Package
The first step is to install the missing package. Open your terminal, navigate to your project’s root directory, and run one of the following commands depending on your package manager:
- Using npm:
npm install web-vitals --save
- Using yarn:
yarn add web-vitals
This command adds the web-vitals package to your project’s dependencies.
2. Verify Installation
After the installation, check your package.json
file. You should see web-vitals listed under the dependencies section, like so:
"dependencies": {
"react": "your-react-version",
"react-dom": "your-react-dom-version",
"web-vitals": "^2.x.x"
// ...other dependencies
}
3. Restart Your Development Server
Sometimes, the development server needs a restart to recognize the new package. Stop your running server and start it again:
npm start
or
yarn start
Your React application should now compile without the error.
Why Does This Error Occur?
The error occurs because the reportWebVitals.js
file dynamically imports the web-vitals package:
import('web-vitals').then(({ getCLS, getFID, getFCP, getLCP, getTTFB }) => {
// Calls to performance measurement functions
});
Without installing the package, the module resolution fails, leading to the “Module not found” error. Installing the package ensures that all the necessary modules are available for your application.
Final Thoughts
Encountering errors during development can be frustrating, but they often have simple solutions. In this case, installing the web-vitals package and restarting your server usually does the trick. This quick fix not only resolves the error but also ensures that your application can accurately measure key performance metrics.