When deploying an ASP.NET Core application, you might encounter the HTTP Error 500.30 – ASP.NET Core App Failed to Start error. This typically indicates that the application failed during the startup process. In this guide, we will explore the common causes of this error and how to fix them.
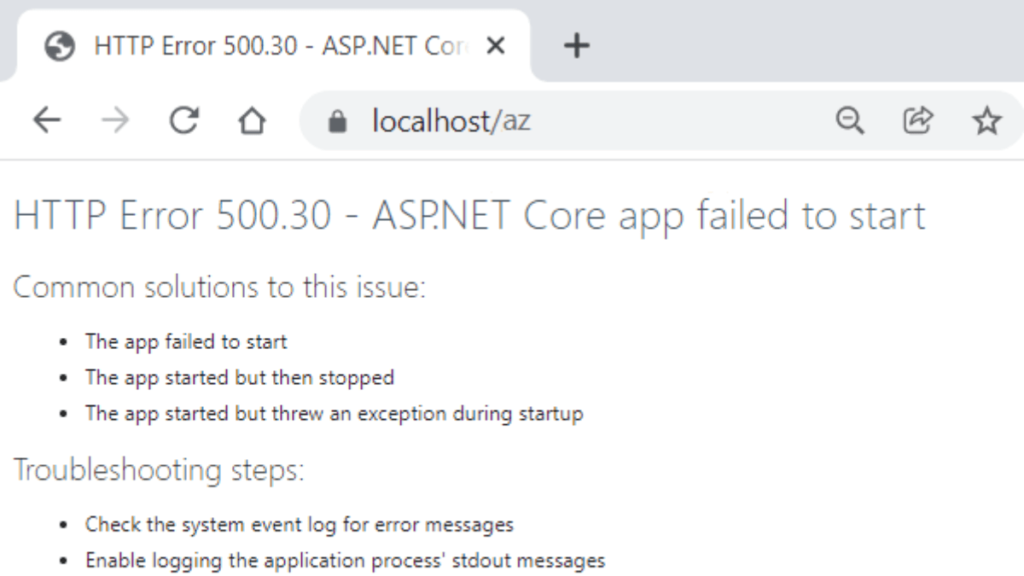
The error message usually means:
- The app failed to start.
- The app started but then crashed.
- An exception was thrown during the startup process.
To resolve this, let’s go through some common troubleshooting steps.
Common Causes and Fixes
1. Missing .NET Runtime on the Server
Since your deployment mode is Framework-Dependent, the target server must have the correct .NET runtime installed.
Solution:
- Install the required .NET 5.0 runtime from the official Microsoft .NET Download page.
- Run the following command on the server to verify the installed runtime:
dotnet --list-runtimes
Ensure that the expected version appears in the output.
2. Incorrect Hosting Bundle Installation (For IIS Hosting)
If you are deploying your app to IIS, it requires the ASP.NET Core Hosting Bundle to run properly.
Solution:
- Download and install the ASP.NET Core Hosting Bundle.
- Restart IIS after installation:
iisreset
3. Insufficient Permissions on Deployed Files
Your IIS worker process might lack the necessary permissions to access the deployed files.
Solution:
- Navigate to the published folder.
- Right-click, select Properties → Security.
- Ensure the IIS user (
IIS_IUSRS
orNETWORK SERVICE
) has read and execute permissions. - Restart IIS after updating permissions.
4. Issues in Startup.cs or Program.cs
If there is an issue in Startup.cs or Program.cs, the application will fail to start.
Solution:
- Check the ConfigureServices and Configure methods in
Startup.cs
for any errors. - Add logging to capture errors:
public void Configure(IApplicationBuilder app, IWebHostEnvironment env, ILogger<Startup> logger)
{
logger.LogInformation("Application is starting...");
}
- Deploy and check the logs for any failure messages.
5. Database Connection Issues
If your application depends on a database, incorrect connection strings can cause startup failures.
Solution:
- Verify the connection string in appsettings.json or environment variables.
- Test database connectivity using:
telnet database_server 1433
(Replace database_server
with your actual database hostname and ensure port 1433 or any relevant port is open.)
- If using Azure Key Vault, ensure the correct access policies are configured.
6. Unhandled Exceptions in Code
An unhandled exception during startup can cause the app to fail before it fully initializes.
Solution:
- Check the Windows Event Viewer:
- Open Event Viewer (
eventvwr.msc
). - Navigate to Windows Logs → Application.
- Look for errors related to your application.
- Open Event Viewer (
- Enable logging in
appsettings.json
:
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
}
- Enable stdout logs in
web.config
:
<aspNetCore stdoutLogEnabled="true" stdoutLogFile=".\logs\stdout" />
- Check logs in the
/logs
folder for errors.
7. Incorrect Target Runtime Selection
Your Target Runtime is set to win-x64
, but if the server is running a different architecture, it could cause an issue.
Solution:
- Verify the architecture of the target server (
x64
orx86
). - If necessary, change the Target Runtime in Publish Settings to match the server’s OS.
8. Application Deployment Issues
A broken deployment can prevent the app from starting.
Solution:
- Ensure the web.config file is present and correctly configured.
- Delete all files from the deployment folder and redeploy the application.
- Compare the deployed files with the contents of the publish folder.
Troubleshooting on Azure App Service and IIS
If your app is deployed on Azure App Service or IIS, additional debugging may be required.
On Azure App Service:
- Use the Kudu Debug Console (
Advanced Tools → Go → Debug Console → CMD
). - Enable Application Logging in the Azure portal.
- Check Application Event Logs via
Diagnose and solve problems
→Application Events
. - Run the app manually in the console:
cd d:\home\site\wwwroot
dotnet YourApp.dll
On IIS:
- Check IIS logs in
C:\inetpub\logs\LogFiles
. - Run the app from the command line:
dotnet YourApp.dll
- Enable detailed error messages in
web.config
.
Troubleshooting Steps Summary
- Check Windows Event Viewer for error logs.
- Enable stdout logging in
web.config
and inspect log files. - Manually run the app on the server:
dotnet YourApp.dll
- Verify database connections and any external dependencies.
- Ensure the correct .NET runtime and Hosting Bundle are installed.
- Use Azure Kudu Debug Console if hosted on Azure.
- Check IIS logs and configuration if hosted on IIS.
By following these steps, you should be able to identify and resolve the HTTP Error 500.30 issue, ensuring a smooth deployment of your ASP.NET Core application.
If you still face issues, share the error logs from Windows Event Viewer or stdout logs for further debugging.