Seeing the dreaded message:
“Cannot connect to the Docker daemon at unix:///var/run/docker.sock. Is the docker daemon running?”
This is one of the most common Docker errors developers face. This guide will walk you through exactly why this happens and how to fix it across different environments (local, Docker Desktop, CI/CD, etc.).
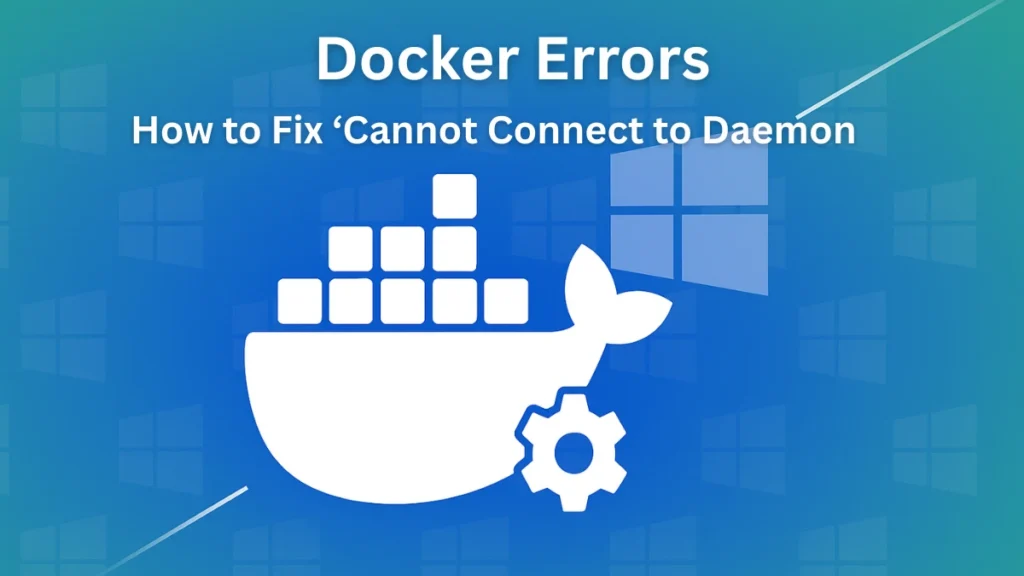
Fix 1: Ensure the Docker Daemon Is Running
The Docker daemon (dockerd
) is the background service that powers all container actions. If it’s not running, Docker commands will fail because there’s no active process to handle them.
Steps:
- Check if Docker is active:
sudo systemctl status docker
- If not active, start it:
sudo systemctl start docker
- For older Linux distributions:
sudo service docker start
- If you use Rootless Docker, run:
systemctl --user status docker
Fix 2: Run Docker as a Non-root User
By default, Docker requires root-level privileges. If your user is not part of the docker
group, the CLI cannot connect to the socket — even if the daemon is running.
Steps:
- Temporarily try:
sudo docker ps
- To fix it permanently:
sudo groupadd -f docker
sudo usermod -aG docker $USER
- Log out and back in, or run:
newgrp docker
Fix 3: Restart the Docker Daemon
Sometimes the daemon enters a stale or unstable state. Restarting the service resets its status and clears potential memory or socket issues..
Steps:
- Restart using systemd:
sudo systemctl restart docker
- Or with service:
sudo service docker restart
Fix 4: Check Docker Socket Permissions
Docker uses the Unix socket file at /var/run/docker.sock
for communication. If your user can’t access it due to incorrect permissions, commands will fail.
Steps:
- Inspect the socket:
ls -l /var/run/docker.sock
You should see:
srw-rw---- 1 root docker ...
- Change ownership if needed:
sudo chown $USER:docker /var/run/docker.sock
Fix 5: Check or Switch Docker Context
Docker CLI can interact with different environments (local, remote, or Docker Desktop) using contexts. If the wrong one is active, your CLI might be targeting a non-existent or misconfigured daemon.
Steps:
- List current context:
docker context ls
- Switch back to default:
docker context use default
- Verify socket path:
docker context inspect --format '{{ .Endpoints.docker.Host }}'
Fix 6: Check Environment Variable DOCKER_HOST
If the DOCKER_HOST
variable is set incorrectly, Docker may try to connect to a non-existent daemon location — such as a wrong TCP address or unavailable VM socket.
Steps:
- Check if it’s set:
echo $DOCKER_HOST
- If unnecessary, unset it:
unset DOCKER_HOST
- Inspect
.bashrc
or.zshrc
for persistent entries.
Fix 7: Start Docker Daemon Manually for Debugging
Manually launching the daemon helps expose any errors preventing it from starting — including port conflicts, socket issues, or missing dependencies.
Steps:
- Run in terminal:
sudo dockerd
- Observe logs and messages. Then, in another terminal:
docker ps
Fix 8: Validate File Permissions During Build
If Docker can’t access files in your build context, it may throw this error mid-build — even if the daemon is active. Ownership and access control issues are common here.
Steps:
- Run:
docker build .
Observe file-related errors. - Solutions:
- Exclude files using
.dockerignore
- Change ownership:
- Exclude files using
sudo chown $USER:docker path/to/file
Bonus Tip: Know Your Docker Variant
Docker comes in multiple install formats (CE, Desktop, Snap). Each uses its own service management and socket paths. Mixing installations can cause the CLI to target the wrong daemon.
Quick Checks:
- For Snap:
snap services docker.dockerd
- For Docker Desktop:
systemctl --user status docker-desktop
Conclusion
The error “Cannot connect to the Docker daemon” is not always what it seems—it’s a general message covering multiple causes. The key is to methodically check:
- Is the Docker daemon running?
- Does your user have the correct group permissions?
- Is the correct socket accessible?
- Are you in the right Docker context?
Once you troubleshoot the above areas, your Docker CLI should be fully functional again.
FAQs
Q1: Can I use Docker without sudo?
Yes, add your user to the docker
group and re-login.
Q2: How do I know if Docker is using the correct context?
Use docker context ls
and switch with docker context use default
.
Q3: What does /var/run/docker.sock
do?
It’s the Unix socket Docker uses to communicate between CLI and daemon.
Q4: Why does this happen on CI/CD systems?
Some pipelines override DOCKER_HOST
or lack daemon access. Always validate your context and environment variables.
Q5: I’m using Docker Desktop, but still getting this error. Why?
Docker CLI might be pointed at a different context or the Docker CE daemon. Switch to the default
context.