Web application testing is evolving, and developers need faster, more reliable tools to ensure their applications perform flawlessly. Cypress, a next-generation JavaScript-based end-to-end testing framework that simplifies the testing process while providing robust tools for debugging and validation.
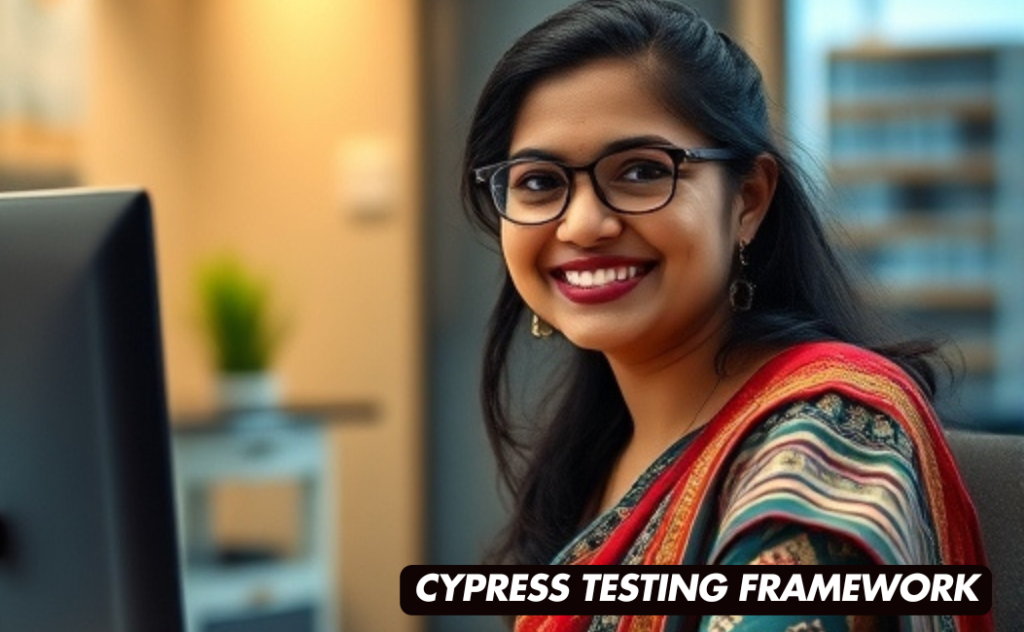
Whether you’re building a small web app or a large-scale enterprise solution, Cypress empowers developers and QA engineers to write tests faster, run them directly in the browser, and debug issues with ease. In this article, we’ll explore the key features, use cases, and benefits of Cypress, along with a practical example to get you started.
What is Cypress?
Cypress is a modern front-end testing tool designed specifically for the web. Unlike traditional testing frameworks that rely on Selenium, Cypress operates directly within the browser, delivering faster, more reliable results. This makes it an ideal choice for developers seeking simplicity and efficiency in their testing workflow.
Key Features of Cypress
- Fast and Reliable: Tests execute within the same browser context as your application.
- Real-time Reloading: Automatically reloads tests upon code changes.
- Time Travel: View snapshots of your app at different test stages, making it easier to debug.
- Automatic Waiting: Cypress automatically waits for elements to appear, reducing the need for manual wait commands.
- Network Traffic Control: Easily intercept and stub API requests and responses.
- Detailed Debugging: Provides powerful error messages and stack traces, allowing for quick identification and resolution of issues.
How Cypress Works
Cypress takes a fundamentally different approach to testing compared to traditional tools. Here’s how:
- Runs Inside the Browser: Cypress executes in the same run-loop as your application, enabling native access to DOM elements.
- No WebDriver Required: Unlike Selenium, Cypress interacts directly with the browser, eliminating the need for WebDriver and reducing the risk of flakiness.
- Direct Network Layer Access: By controlling network requests and responses, Cypress offers more predictable and consistent test behavior.
Use Cases for Cypress
Cypress can handle various types of testing scenarios, making it a versatile tool for developers and testers alike.
- End-to-End Testing: Simulate real user interactions and validate the entire application workflow.
- Integration Testing: Test how different components of your application interact with each other.
- Unit Testing: Validate individual components or functions to ensure they work as expected.
Writing Your First Cypress Test
Let’s walk through a simple Cypress test to demonstrate how easy it is to get started.
Example: Testing a Simple Webpage
describe('My First Test', () => {
it('Visits the Kitchen Sink', () => {
cy.visit('https://example.cypress.io') // Visit URL
cy.contains('type').click() // Find and click 'type'
cy.url().should('include', '/commands/actions') // Assert URL
cy.get('.action-email') // Target input
.type('[email protected]') // Type into input
.should('have.value', '[email protected]') // Assert value
})
})
In this test:
- We visit a sample webpage.
- Locate and click the “type” button.
- Verify the URL changes correctly.
- Interact with an input field and validate the typed value.
Installing Cypress
Getting started with Cypress is straightforward. You can install it using npm:
npm install cypress --save-dev
To launch Cypress, simply run:
npx cypress open
This will open the Cypress Test Runner, allowing you to execute and manage your test cases.
Key Cypress Commands
Cypress offers a comprehensive set of commands to interact with and validate web elements:
cy.visit()
– Navigate to a specified URL.cy.get()
– Select elements using CSS selectors.cy.contains()
– Locate elements by their text content.cy.type()
– Simulate typing into input fields.cy.click()
– Perform click actions.cy.should()
– Add assertions to validate expected outcomes.cy.intercept()
– Intercept and modify API requests.
Why Choose Cypress Over Selenium?
Simplicity and Speed:
- Cypress requires no additional drivers or configurations, whereas Selenium often involves complex setup and third-party dependencies.
Built for Developers:
- With intuitive commands and real-time feedback, Cypress is designed to fit naturally into a front-end developer’s workflow.
Stability and Debugging:
- Since Cypress executes tests in the browser, it avoids the flakiness often encountered in Selenium tests.
- Debugging is seamless, with time travel and detailed error logs providing greater visibility into test execution.
Conclusion
Cypress is revolutionizing web application testing by offering developers a fast, reliable, and easy-to-use framework. Its unique approach to browser-based testing, along with powerful debugging tools, makes it an invaluable asset for front-end development teams.
By incorporating Cypress into your testing workflow, you can ensure higher-quality applications, faster development cycles, and a more streamlined testing experience.