Cucumber is a widely used tool in Behavior Driven Development (BDD) that allows teams to write test cases in a human-readable format. Below are 40 common interview questions about Cucumber, along with detailed answers to help you prepare.
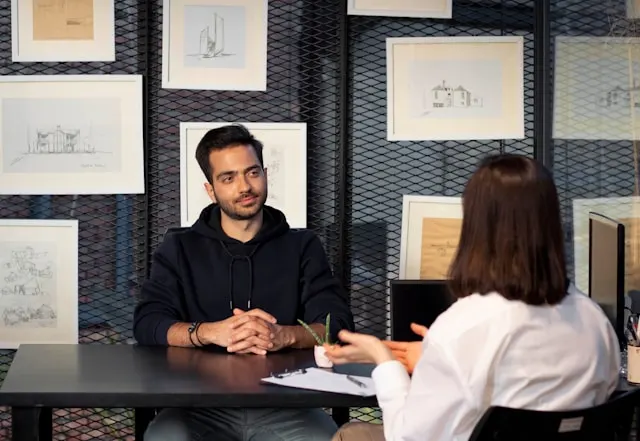
Cucumber Interview Questions with Detailed Answers
- What is Cucumber?
- What are the advantages of using Cucumber?
- Explain the Gherkin language.
- What are the main keywords used in Cucumber?
- What is the purpose of the Background keyword?
- How does Scenario Outline work in Cucumber?
- What is the role of step definitions in Cucumber?
- Explain how tags work in Cucumber.
- What is the significance of hooks in Cucumber?
- Describe how Cucumber integrates with Selenium.
- What are some best practices for writing Cucumber tests?
- Can you explain what a Data Table is in Cucumber?
- What is the difference between Scenario and Scenario Outline?
- How do you handle exceptions in Cucumber?
- What tools can be integrated with Cucumber for reporting?
- How does BDD differ from traditional testing approaches?
- What challenges might one face when implementing BDD with Cucumber?
- Can you explain what “Given”, “When”, “Then” represent in a scenario?
- How do you execute tests written in Cucumber?
- Why is it important to keep feature files organized?
- How do you set up Cucumber in a project?
- What are Cucumber expressions, and how do they differ from regular expressions in step definitions?
- How can Cucumber be configured to run only a subset of scenarios in a feature file?
- Explain the purpose and usage of the
@DataTableType
annotation in Cucumber. - What is ParameterType in Cucumber, and how does it work?
- How does Cucumber support parallel test execution?
- Can Cucumber handle API testing, and if so, how?
- How would you implement conditional steps in Cucumber?
- What are some common anti-patterns to avoid in Cucumber?
- How do you manage test data in Cucumber scenarios?
- How does Cucumber handle asynchronous operations, and what challenges does this present?
- Can you explain the usage of custom transformers in Cucumber?
- What are the main advantages of using Scenario hooks over Global hooks in Cucumber?
- How do you implement parameterized testing in Cucumber?
- Describe the differences between
@BeforeStep
and@AfterStep
hooks in Cucumber. - How can you handle dependencies between scenarios in Cucumber?
- Explain how you would execute Cucumber tests in a CI/CD pipeline.
- What are the different types of reports that can be generated in Cucumber?
- How do you debug issues in Cucumber tests?
- Can Cucumber be used for non-functional testing? Explain.
1. What is Cucumber?
Answer:
Cucumber is an open-source testing tool that supports Behavior Driven Development (BDD). It enables developers and testers to write acceptance tests in a format that is easily understandable by non-technical stakeholders. Cucumber uses the Gherkin language, which allows for writing scenarios in plain English, making it accessible for business analysts and other non-developers to participate in the testing process.
2. What are the advantages of using Cucumber?
Answer: Cucumber offers several advantages:
- Collaboration: It bridges the gap between technical and non-technical team members, facilitating better communication.
- Readability: Tests written in Gherkin are easy to read and understand, which helps stakeholders grasp the requirements without deep technical knowledge.
- Automation: Cucumber allows for automated testing of applications, improving efficiency and reducing manual errors.
- Reusability: Step definitions can be reused across different scenarios, promoting DRY (Don’t Repeat Yourself) principles.
3. Explain the Gherkin language.
Answer:
Gherkin is a domain-specific language used by Cucumber to write test scenarios. It employs a simple syntax that includes keywords such as Feature, Scenario, Given, When, Then, and Background. Gherkin files are saved with a .feature
extension and describe the behavior of the application in a structured format that is both human-readable and machine-executable.
4. What are the main keywords used in Cucumber?
Answer: The primary keywords in Cucumber include:
- Feature: Defines a feature of the application under test.
- Scenario: Describes a specific situation or test case.
- Given: Sets up the context or initial state for the scenario.
- When: Specifies an action or event.
- Then: Describes the expected outcome or result.
- Background: Provides common steps that apply to all scenarios within a feature file.
5. What is the purpose of the Background keyword?
Answer:
The Background keyword allows you to define steps that are common to all scenarios within a feature file. This helps reduce redundancy by executing these steps before each scenario, ensuring that all necessary preconditions are met without repeating them in every scenario.
6. How does Scenario Outline work in Cucumber?
Answer:
A Scenario Outline is used to run the same scenario multiple times with different sets of data. It defines placeholders (e.g., <username>
) within steps, which are replaced by actual values provided in an accompanying Examples table. This approach supports data-driven testing.
7. What is the role of step definitions in Cucumber?
Answer:
Step definitions link Gherkin steps to code that executes those steps. Each step written in a feature file must have a corresponding step definition implemented in code (usually Java, Ruby, etc.). Step definitions use regular expressions or annotations to match Gherkin syntax with executable code.
8. Explain how tags work in Cucumber.
Answer:
Tags are used to categorize scenarios and features, allowing selective execution of tests based on specific criteria. Tags can be added to features or scenarios using the @tagname
syntax. You can run tests with specific tags using command-line options, enabling targeted testing during development or CI/CD processes.
9. What is the significance of hooks in Cucumber?
Answer:
Hooks are blocks of code that run at specific points during the test execution lifecycle. They can be used for setup and teardown activities, such as initializing test data or cleaning up after tests. Common hooks include Before
, After
, BeforeStep
, and AfterStep
.
10. Describe how Cucumber integrates with Selenium.
Answer:
Cucumber can be integrated with Selenium WebDriver to automate web application testing. While Cucumber manages test scenarios written in Gherkin, Selenium executes browser interactions based on those scenarios. This combination allows for behavior-driven testing of web applications, where business requirements drive automated tests.
11. What are some best practices for writing Cucumber tests?
Answer: Best practices include:
- Write clear and concise scenarios that focus on user behavior.
- Use meaningful names for features and scenarios to improve readability.
- Avoid complex logic within step definitions; keep them simple and focused.
- Reuse step definitions whenever possible to reduce duplication.
- Regularly review and refactor scenarios to maintain clarity as requirements evolve.
12. Can you explain what a Data Table is in Cucumber?
Answer:
A Data Table is a way to pass multiple sets of data into a single step definition within a scenario. It allows you to provide structured input data directly within your Gherkin file using rows and columns, making it easier to manage complex scenarios with multiple variables.
13. What is the difference between Scenario and Scenario Outline?
Answer:
A Scenario represents a single test case with specific inputs and expected outcomes, while a Scenario Outline serves as a template for running multiple iterations of similar tests using different sets of data defined in an Examples table.
14. How do you handle exceptions in Cucumber?
Answer:
Exceptions can be handled by implementing error handling within step definitions using try-catch blocks or custom exception classes. Additionally, you can use hooks like @After
to log errors or take screenshots when tests fail, providing better insights into issues encountered during execution.
15. What tools can be integrated with Cucumber for reporting?
Answer: Cucumber can be integrated with various reporting tools such as:
- Allure Reports: Provides detailed reports with visual representations of test results.
- ExtentReports: Allows for customizable HTML reports that include screenshots and logs.
- JUnit/TestNG Reports: If using Java, you can leverage built-in reporting features from these frameworks.
16. How does BDD differ from traditional testing approaches?
Answer:
Behavior Driven Development focuses on collaboration between stakeholders (developers, testers, business analysts) by using natural language specifications (Gherkin) that everyone can understand. Traditional testing often relies on technical specifications and may not involve non-technical stakeholders as closely, leading to potential misunderstandings about requirements.
17. What challenges might one face when implementing BDD with Cucumber?
Answer: Challenges include:
- Ensuring all team members understand BDD principles and practices.
- Maintaining clear communication between technical and non-technical team members.
- Keeping feature files organized as they grow over time.
- Balancing between too much detail (leading to complexity) and too little detail (leading to ambiguity).
18. Can you explain what “Given”, “When”, “Then” represent in a scenario?
Answer: In Cucumber:
- Given represents the initial context or setup required before executing actions.
- When describes the action taken by the user or system during the scenario.
- Then specifies the expected outcome or result after performing the action(s).
These keywords help structure scenarios clearly, making it easier for all stakeholders to understand what each part represents.
19. How do you execute tests written in Cucumber?
Answer:
Tests written in Cucumber can be executed using command-line interfaces or through integrated development environments (IDEs) like Eclipse or IntelliJ IDEA that support running JUnit or TestNG tests configured with Cucumber options.
20. Why is it important to keep feature files organized?
Answer:
Keeping feature files organized is crucial for maintaining clarity as projects scale up. Well-organized files help team members quickly locate relevant tests, understand their purpose without confusion, and facilitate easier updates as requirements change over time.
21. How do you set up Cucumber in a project?
Answer:
Setting up Cucumber involves several steps depending on your development environment and programming language. In a Maven-based Java project, you start by adding the cucumber-java
and cucumber-junit
dependencies in the pom.xml
file. Then, you create the directory structure for feature files (typically in src/test/resources
). Cucumber requires a runner class annotated with @RunWith(Cucumber.class)
to execute tests, and in this class, you can configure various aspects of the test execution. Finally, you write feature files in Gherkin and corresponding step definitions in Java to implement each scenario.
22. What are Cucumber expressions, and how do they differ from regular expressions in step definitions?
Answer:
Cucumber expressions are simpler, more readable placeholders used in step definitions to match steps in a feature file. They allow parameters in curly braces, e.g., {int}
or {string}
, to match variables in the scenario steps. Unlike regular expressions, Cucumber expressions are easier to understand and use, especially for non-technical stakeholders. While regular expressions use special syntax (e.g., \\d+
for numbers), Cucumber expressions directly use {int}
, making step definitions more readable and maintainable.
23. How can Cucumber be configured to run only a subset of scenarios in a feature file?
Answer:
Cucumber allows you to filter scenarios using tags. Tags are annotations prefixed with @
, and you can assign them to individual scenarios or feature files. By specifying tags in the Cucumber options of the test runner (e.g., @CucumberOptions(tags = "@SmokeTest")
), you can run only the scenarios with those tags. You can also use logical operators like AND
, OR
, and NOT
to include or exclude multiple tags in your selection criteria.
24. Explain the purpose and usage of the @DataTableType
annotation in Cucumber.
Answer:
The @DataTableType
annotation is used in Cucumber to define a custom mapping between data tables in feature files and Java objects. It converts each row in a data table into an instance of a specified class, allowing for structured data to be passed into step definitions. This approach simplifies data handling by removing the need for manual parsing, making tests more concise and readable. This annotation is commonly used when dealing with complex data structures.
25. What is ParameterType in Cucumber, and how does it work?
Answer:
ParameterType
is a mechanism in Cucumber to define custom data types for matching specific parts of a step definition. By defining a ParameterType
, you can transform matched text into a specified type directly in the step definition, making steps more expressive and reusable. This is done by registering ParameterType
in the step definition file, which maps patterns to custom types.
26. How does Cucumber support parallel test execution?
Answer:
Parallel execution in Cucumber is supported by configuring the test runner to run multiple scenarios simultaneously. In a Java environment, this can be achieved using tools like JUnit 5, TestNG, or plugins such as cucumber-jvm-parallel-plugin
. Parallel execution helps reduce test time significantly, especially in larger test suites. However, it requires careful setup to ensure that test data and shared resources are isolated to prevent interference between tests.
27. Can Cucumber handle API testing, and if so, how?
Answer:
Yes, Cucumber can handle API testing by combining it with libraries like RestAssured or HttpClient in Java. In this approach, you can write Gherkin scenarios that describe API interactions, such as sending HTTP requests and validating responses. The actual API calls and response validations are implemented in step definitions, where you can perform assertions on response status codes, headers, and payload data. This setup allows for BDD testing at the API level, which is beneficial in microservices and backend testing.
28. How would you implement conditional steps in Cucumber?
Answer:
In Cucumber, conditional steps can be managed by using control logic within step definitions. For instance, after performing an action in one step, the subsequent step definition can check a condition to decide if it should proceed. While Cucumber doesn’t natively support branching in feature files (to maintain readability), this approach allows flexibility within the execution flow. However, overuse of conditional steps can make tests complex and hard to maintain, so they should be used sparingly.
29. What are some common anti-patterns to avoid in Cucumber?
Answer: Common Cucumber anti-patterns include:
- Writing overly detailed steps, which reduces readability and makes maintenance challenging.
- Mixing Gherkin syntax with technical details that non-technical stakeholders may not understand.
- Using long, complex step definitions that perform multiple actions, violating the single-responsibility principle.
- Using Cucumber for non-behavioral tests, such as unit tests, which detracts from the tool’s primary purpose of describing user behavior.
Avoiding these anti-patterns ensures that Cucumber remains an effective tool for BDD.
30. How do you manage test data in Cucumber scenarios?
Answer:
Test data in Cucumber can be managed using data tables in the feature files, external files (such as CSV or JSON), or even dedicated test data generators within step definitions. Data tables allow for straightforward, in-line data input, while external files offer greater flexibility and maintainability. Using Dependency Injection frameworks, such as Spring or Guice, is also a viable approach to manage test data in more complex scenarios.
31. How does Cucumber handle asynchronous operations, and what challenges does this present?
Answer:
Cucumber step definitions can handle asynchronous operations by using language-specific constructs, such as await
in JavaScript or CompletableFuture
in Java. Asynchronous operations may lead to timing issues, where assertions are made before the action completes. To address this, it’s essential to add waits or retries and to structure scenarios so that steps explicitly wait for each asynchronous operation to finish before proceeding.
32. Can you explain the usage of custom transformers in Cucumber?
Answer:
Custom transformers in Cucumber allow you to convert complex input data into custom objects, simplifying step definitions. Using annotations like @DocStringType
or @DataTableType
, you can define transformers that take raw input and transform it into structured types. This enables a clean, reusable way to handle data transformation and supports a wide range of data formats in tests.
33. What are the main advantages of using Scenario hooks over Global hooks in Cucumber?
Answer:
Scenario hooks (like @Before
and @After
specific to each scenario) offer a more granular level of control than global hooks. They allow you to define setup and teardown logic specific to individual scenarios, which is useful for isolating test data, ensuring tests are independent, and reducing test flakiness. Global hooks apply to all scenarios, which might not be appropriate for certain test setups.
34. How do you implement parameterized testing in Cucumber?
Answer:
Parameterized testing in Cucumber is achieved through Scenario Outlines. By defining placeholders in the scenario, you can pass multiple sets of values in an example table, which results in the scenario running once per data set. This method allows for efficient testing with multiple inputs and keeps the feature files clean and organized.
35. Describe the differences between @BeforeStep
and @AfterStep
hooks in Cucumber.
Answer:
@BeforeStep
and @AfterStep
hooks allow actions to be performed before and after each step in a scenario. This is useful for operations like logging, capturing screenshots, or other verification tasks at each step. Unlike @Before
and @After
hooks, which apply to the entire scenario, @BeforeStep
and @AfterStep
add more granularity by focusing on each individual step.
36. How can you handle dependencies between scenarios in Cucumber?
Answer:
Dependencies between scenarios are generally discouraged in BDD, as they create coupling and make tests less robust. However, if necessary, dependencies can be handled by sharing data through a common context or state, often implemented with Dependency Injection frameworks or singleton classes. This approach allows for shared state without creating tight coupling, though it requires careful management to avoid unintended interactions.
37. Explain how you would execute Cucumber tests in a CI/CD pipeline.
Answer:
To execute Cucumber tests in a CI/CD pipeline, integrate Cucumber with build tools like Jenkins, GitLab CI, or CircleCI. You configure the pipeline to run test commands (e.g., mvn test
for Maven projects) and generate reports. Cucumber supports generating JUnit, HTML, and JSON reports, which can be used to visualize test results. Ensuring tests run in isolated environments and handling dependencies effectively is critical for successful CI/CD integration.
38. What are the different types of reports that can be generated in Cucumber?
Answer:
Cucumber can generate various reports, including HTML, JSON, and XML reports. HTML reports provide a visual summary of test results, while JSON and XML formats are commonly used for integration with other tools. Plugins like cucumber-reporting
offer enhanced visualizations, which can be used in CI/CD dashboards to track test results over time.
39. How do you debug issues in Cucumber tests?
Answer:
Debugging Cucumber tests can involve adding logging statements in step definitions, using breakpoints in the IDE, or using Cucumber hooks to capture contextual information.
Many IDEs support debugging Cucumber step definitions directly, allowing you to step through the code and inspect variables. Additionally, error messages in the Cucumber console output help trace issues back to specific scenarios or steps.
40. Can Cucumber be used for non-functional testing? Explain.
Answer:
While Cucumber is primarily used for functional testing, it can be adapted for certain types of non-functional tests, such as performance and load testing, by integrating it with other tools like JMeter or Gatling. However, Cucumber’s Gherkin syntax is not well-suited for describing non-functional requirements, so these scenarios might require customization or a more appropriate testing framework.
Learn More: Carrer Guidance
Accounts Payable Interview Questions with Detailed Answers
Entity Framework Interview Questions with detailed answers- Basic to Advanced
Vue Js interview questions and answers- Basic to Advance
CN Interview Questions and Answers for Freshers
Desktop Support Engineer Interview Questions and Detailed Answer- Basic to Advanced
Apex Interview Questions and Answers- Basic to Advance