CSS (Cascading Style Sheets) is a fundamental technology for web development, allowing developers to design visually engaging and responsive web pages. Mastering CSS is essential for any front-end developer, and interviewers often test candidates on various CSS properties, positioning techniques, and layout models. This guide covers essential CSS interview questions for freshers, helping you build confidence and be well-prepared for your next job interview.
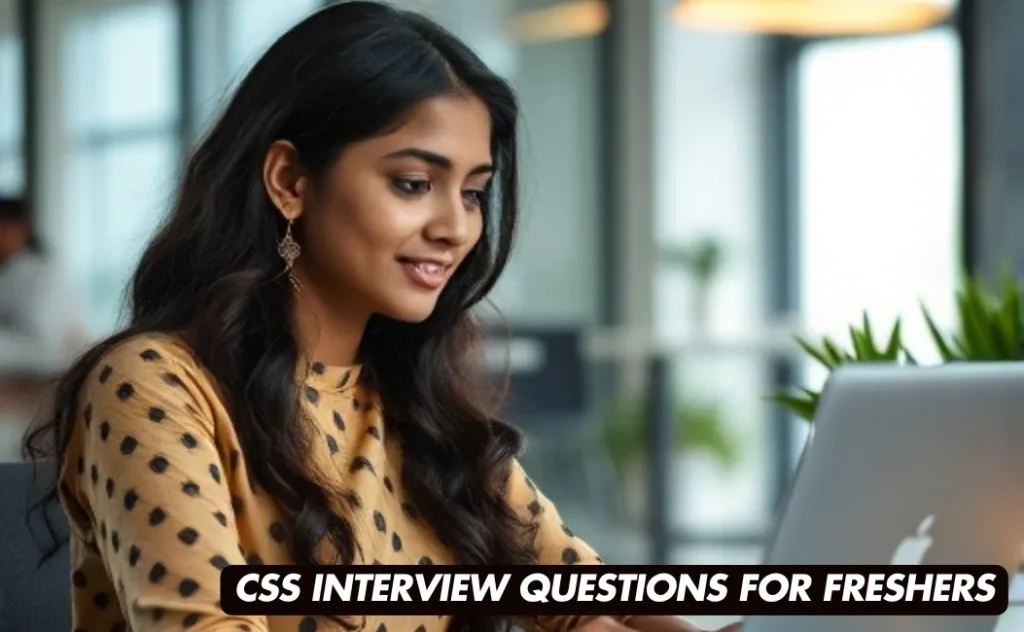
1. Basic CSS Concepts
- What is CSS, and what does it stand for?
- How do you include CSS in a web page?
- What are the benefits of using CSS?
- Explain the CSS box model and its components.
- What is the difference between class and id selectors in CSS?
- How do you center a block element horizontally in CSS?
- What is the difference between padding and margin?
- How can you make a background image responsive in CSS?
- What is the difference between inline, inline-block, and block elements in CSS?
- How do you select all paragraph elements inside a div using CSS?
2. CSS Selectors and Specificity
- What are the different types of selectors in CSS?
- How does CSS specificity work?
- What is a pseudo-class in CSS? Provide examples.
- What is a pseudo-element in CSS? Provide examples.
- How do attribute selectors work in CSS?
- Explain the difference between the child (>) and descendant (space) combinators in CSS.
- How can you apply styles to an element when the user hovers over it?
- What is the universal selector in CSS, and how is it used?
- How do you target elements that are the first child of their parent in CSS?
- What is the :nth-child() selector in CSS, and how does it work?
3. CSS Layout and Positioning
- What are the different positioning schemes in CSS?
- Explain the difference between relative, absolute, fixed, and static positioning.
- How does the CSS float property work?
- What is Flexbox in CSS, and when would you use it?
- How do CSS Grid and Flexbox differ?
- How can you create a two-column layout using CSS?
- What is the z-index property in CSS, and how does it function?
- How do you make an element stick to the top of the page when scrolling?
- What is the CSS display property, and what are some of its values?
- How can you create a responsive design using CSS?
4. Advanced CSS Features
- What are CSS preprocessors, and can you name a few?
- How do you implement CSS animations?
- What are CSS transitions, and how do they differ from animations?
- How can you optimize CSS for better performance?
- What is a CSS sprite, and how is it used?
- How do media queries work in CSS?
- What is the purpose of the box-sizing property in CSS?
- How can you create a CSS triangle?
- What is the difference between visibility: hidden and display: none in CSS?
- How do you apply a style only to specific devices or screen sizes using CSS?
1. What is CSS, and what does it stand for?
CSS stands for Cascading Style Sheets. It is a stylesheet language used to describe the presentation of a document written in HTML or XML. CSS controls the layout, colors, fonts, spacing, and overall visual appearance of web pages, enabling developers to separate content from design. This separation enhances maintainability, allows for consistent styling across multiple pages, and improves website performance by reducing redundancy.
Key Points:
- Cascading: Refers to the priority scheme to determine which styles apply when multiple rules match an element.
- Style Sheets: Files containing CSS rules that dictate the look and feel of web pages.
Example:
body {
background-color: #f0f0f0;
font-family: Arial, sans-serif;
}
h1 {
color: #333333;
text-align: center;
}
2. How do you include CSS in a web page?
There are three primary methods to include CSS in a web page:
- Inline CSS:
- Styles are applied directly within an HTML element using the
style
attribute.Best used for quick, specific styling but not recommended for larger projects due to maintainability issues.
- Styles are applied directly within an HTML element using the
<p style="color: blue; font-size: 16px;">This is a blue paragraph.</p>
- Internal CSS:
- Styles are defined within a
<style>
tag inside the<head>
section of the HTML document.Suitable for single-page styling.
- Styles are defined within a
<head>
<style>
body {
background-color: #ffffff;
}
.container {
width: 80%;
margin: 0 auto;
}
</style>
</head>
- External CSS:
- Styles are written in a separate
.css
file and linked to the HTML document using the<link>
tag.Preferred method for larger websites as it promotes reusability and better organization.
- Styles are written in a separate
<head>
<link rel="stylesheet" href="styles.css">
</head>
/* styles.css */
header {
background-color: #333;
color: #fff;
padding: 20px;
text-align: center;
}
3. What are the benefits of using CSS?
Using CSS offers numerous advantages:
- Separation of Content and Presentation:
- Keeps HTML focused on structure and semantics while CSS handles the visual aspects, enhancing code readability and maintainability.
- Reusability:
- Styles defined in external CSS files can be reused across multiple pages, ensuring consistency and reducing duplication.
- Improved Load Times:
- Browsers cache external CSS files, leading to faster page loads after the initial visit.
- Responsive Design:
- CSS facilitates creating responsive layouts that adapt to different screen sizes and devices.
- Enhanced Accessibility:
- Proper use of CSS can improve the accessibility of web content for users with disabilities.
- Easier Maintenance:
- Updating styles in a single CSS file reflects changes across all linked pages, simplifying maintenance.
- Advanced Styling Capabilities:
- CSS offers a wide range of styling options, including animations, transitions, and complex layouts.
Example: By changing a single external CSS file, you can update the look of an entire website without modifying individual HTML pages.
4. Explain the CSS box model and its components.
The CSS box model is a fundamental concept that defines how elements are structured and how they interact with each other in terms of layout and spacing. Each element is considered as a rectangular box consisting of four main components:
- Content:
- The actual content of the element, such as text, images, or other media.
- Its size is defined by
width
andheight
properties.
- Padding:
- The space between the content and the border.
- Transparent and can be set uniformly or individually on each side using properties like
padding-top
,padding-right
, etc.
- Border:
- A visible line that surrounds the padding and content.
- Defined using properties like
border-width
,border-style
, andborder-color
.
- Margin:
- The space outside the border, separating the element from other elements.
- Like padding, it can be set uniformly or individually on each side.
Visualization:
+-----------------------+
| Margin |
| +-----------------+ |
| | Border | |
| | +-----------+ | |
| | | Padding | | |
| | | +-------+ | | |
| | | |Content| | | |
| | | +-------+ | | |
| | +-----------+ | |
| +-----------------+ |
+-----------------------+
Example:
.box {
width: 200px; /* Content width */
padding: 20px; /* Padding around content */
border: 5px solid #000; /* Border */
margin: 30px; /* Margin outside the border */
}
In this example:
- The total width of the
.box
element is calculated as:Content width
+Padding (left + right)
+Border (left + right)
= 200px + 40px + 10px = 250px.
- The margin adds additional space outside the element but does not affect its size.
Understanding the box model is crucial for accurately designing layouts and controlling spacing in web development.
5. What is the difference between class and id selectors in CSS?
Both class and id selectors are used to apply styles to HTML elements, but they serve different purposes and have distinct characteristics:
Class Selectors:
- Syntax: Prefixed with a dot (
.
), e.g.,.classname
. - Usage:
- Can be applied to multiple elements on a page.
- Ideal for grouping elements that share the same styling.
- Specificity: Lower specificity compared to
id
selectors. - Example:
<div class="highlight">This is highlighted.</div>
<p class="highlight">This paragraph is also highlighted.</p>
.highlight {
background-color: yellow;
}
ID Selectors:
- Syntax: Prefixed with a hash (
#
), e.g.,#uniqueid
. - Usage:
- Should be unique within an HTML document; only one element should have a particular
id
. - Often used for specific styling or JavaScript interactions.
- Should be unique within an HTML document; only one element should have a particular
- Specificity: Higher specificity, meaning styles defined with
id
selectors will override those with class selectors if there’s a conflict. - Example:
<div id="header">This is the header.</div>
#header {
background-color: blue;
color: white;
}
Key Differences:
- Multiplicity: Classes can be reused on multiple elements, whereas IDs should be unique.
- Specificity: IDs have higher specificity, making them more powerful in overriding styles.
- Use Cases: Classes are generally preferred for styling multiple elements, while IDs are used for single, unique elements or for JavaScript targeting.
Best Practices:
- Use classes for styling purposes.
- Use IDs sparingly, primarily for unique elements or JavaScript hooks.
- Avoid overusing IDs to prevent specificity conflicts.
6. How do you center a block element horizontally in CSS?
To center a block-level element horizontally within its parent container, you can use the margin
property with auto values on the left and right sides. This method requires the element to have a specified width.
Steps:
- Set a width for the block element. If no width is specified, the element will take up the full width of its parent, making centering ineffective.
- Apply
margin: 0 auto;
to center the element horizontally.
Example:
<div class="container">
<div class="centered-box">
This box is centered horizontally.
</div>
</div>
.centered-box {
width: 50%; /* Specify the width */
margin: 0 auto; /* Top and bottom margins set to 0, left and right margins set to auto */
background-color: #f2f2f2;
padding: 20px;
text-align: center;
}
Explanation:
width: 50%;
sets the width of.centered-box
to 50% of its parent container.margin: 0 auto;
automatically calculates equal left and right margins, effectively centering the element horizontally.- The first value
0
sets the top and bottom margins, whileauto
adjusts the left and right margins.
Alternative Method Using Flexbox: If the parent container uses Flexbox, you can center the child element horizontally without specifying a width.
.container {
display: flex;
justify-content: center; /* Centers horizontally */
}
.centered-box {
background-color: #f2f2f2;
padding: 20px;
text-align: center;
}
Note: Flexbox offers more flexibility and is particularly useful for responsive designs.
7. What is the difference between padding and margin?
Padding and margin are both CSS properties used to create space around elements, but they serve different purposes and affect the layout in distinct ways.
Padding:
- Definition: The space between the content of an element and its border.
- Location: Inside the element’s border.
- Effect on Element Size: Increases the overall size of the element by adding space inside its boundaries.
- Usage: Used to create breathing space within an element, ensuring that content doesn’t touch the borders.
Example:
.box {
padding: 20px; /* Adds space inside the box */
background-color: #e0e0e0;
}
Margin:
- Definition: The space outside the border of an element, separating it from other elements.
- Location: Outside the element’s border.
- Effect on Element Position: Creates space between elements without affecting the element’s own size.
- Usage: Used to control the spacing between different elements on a page.
Example:
.box {
margin: 20px; /* Adds space outside the box */
background-color: #c0c0c0;
}
Key Differences:
Aspect | Padding | Margin |
---|---|---|
Location | Inside the border | Outside the border |
Affects Element Size | Yes, increases the element’s size | No, does not affect the element’s size |
Collapsing | Does not collapse with other margins | Adjacent margins can collapse |
Background | Background color extends into padding | Background color does not apply to margins |
Visual Representation:
+-----------------------+
| Margin |
| +-----------------+ |
| | Border | |
| | +-----------+ | |
| | | Padding | | |
| | | +-------+ | | |
| | | |Content| | | |
| | | +-------+ | | |
| | +-----------+ | |
| +-----------------+ |
+-----------------------+
Practical Example:
<div class="container">
<div class="box">
Content goes here.
</div>
</div>
.container {
background-color: #f9f9f9;
padding: 30px; /* Padding inside the container */
}
.box {
margin: 20px; /* Margin outside the box */
padding: 15px; /* Padding inside the box */
background-color: #ffffff;
border: 1px solid #cccccc;
}
In this example:
- The
.container
has padding, creating space inside its border. - The
.box
has margin, creating space between itself and other elements (in this case, the container’s padding). - The
.box
also has padding, adding space between its content and its own border.
8. How can you make a background image responsive in CSS?
Making a background image responsive ensures that it adjusts smoothly to different screen sizes and devices without losing its visual integrity. CSS provides several properties to achieve responsive background images effectively.
Steps to Make a Background Image Responsive:
- Use
background-size: cover;
:- Scales the background image to cover the entire container, maintaining its aspect ratio.
- The image may be cropped to fit the container.
- Use
background-position: center;
:- Centers the background image within the container, ensuring the focal point remains visible.
- Use
background-repeat: no-repeat;
:- Prevents the background image from repeating if it’s smaller than the container.
- Set the Container’s Dimensions Responsively:
- Use relative units like percentages or viewport units to define the container’s size.
Example:
<div class="responsive-bg">
<!-- Content goes here -->
</div>
.responsive-bg {
width: 100%;
height: 400px; /* Can use relative units or set based on content */
background-image: url('path-to-image.jpg');
background-size: cover;
background-position: center;
background-repeat: no-repeat;
}
Explanation:
background-size: cover;
ensures the image always covers the entire container, scaling as needed.background-position: center;
keeps the image centered, so the most important part remains visible.background-repeat: no-repeat;
prevents tiling of the image.
Alternative: Using Media Queries for Enhanced Responsiveness: Sometimes, different background images or styles are needed for various screen sizes. Media queries allow for such adjustments.
.responsive-bg {
width: 100%;
height: 400px;
background-image: url('desktop-image.jpg');
background-size: cover;
background-position: center;
background-repeat: no-repeat;
}
@media (max-width: 768px) {
.responsive-bg {
background-image: url('tablet-image.jpg');
height: 300px;
}
}
@media (max-width: 480px) {
.responsive-bg {
background-image: url('mobile-image.jpg');
height: 200px;
}
}
Using background-attachment: fixed;
for Parallax Effect: To create a parallax scrolling effect, you can fix the background image relative to the viewport.
.responsive-bg {
background-attachment: fixed;
background-size: cover;
background-position: center;
}
Note:
- Ensure that the background image has a high enough resolution to look good on larger screens.
- Optimize images for web to reduce loading times, especially for mobile users.
9. What is the difference between inline, inline-block, and block elements in CSS?
Inline, inline-block, and block are different display properties in CSS that define how elements behave in the document flow. Understanding their differences is crucial for controlling layout and spacing.
1. Block Elements:
- Characteristics:
- Start on a new line.
- Take up the full width available by default.
- Respect
width
,height
,margin
, andpadding
properties. - Examples:
<div>
,<p>
,<h1>
to<h6>
,<section>
,<article>
.
- Usage:
- Ideal for creating larger structural components like containers, sections, and paragraphs.
- Example:
.block-element {
display: block;
width: 50%;
height: 100px;
margin: 20px auto;
background-color: #f0f0f0;
}
2. Inline Elements:
- Characteristics:
- Do not start on a new line.
- Only take up as much width as necessary.
- Do not respect
width
andheight
properties. - Respect horizontal
margin
andpadding
, but not vertical. - Examples:
<span>
,<a>
,<strong>
,<em>
,<img>
.
- Usage:
- Suitable for styling small portions of text or inline content within block elements.
- Example:
.inline-element {
display: inline;
margin: 10px; /* Only horizontal margins have effect */
padding: 5px 10px;
background-color: #e0e0e0;
}
3. Inline-Block Elements:
- Characteristics:
- Similar to inline elements in that they do not start on a new line.
- Behave like block elements in that they can have
width
andheight
set. - Respect all
margin
andpadding
properties. - Allow elements to sit next to each other horizontally.
- Usage:
- Useful for creating horizontal navigation menus, buttons, or any elements that need both inline and block characteristics.
- Example:
.inline-block-element {
display: inline-block;
width: 150px;
height: 50px;
margin: 10px;
padding: 10px;
background-color: #d0d0d0;
text-align: center;
vertical-align: middle;
}
Visual Comparison:
Property | Block | Inline | Inline-Block |
---|---|---|---|
Line Break | Yes | No | No |
Width/Height | Yes | No | Yes |
Margin/Padding | All sides | Horizontal only | All sides |
Flow | Stacks vertically | Flows horizontally | Flows horizontally |
Use Cases | Containers, sections | Text styling, links | Buttons, menu items |
Practical Example:
<div class="block-element">Block Element</div>
<span class="inline-element">Inline Element</span>
<span class="inline-block-element">Inline-Block Element</span>
.block-element {
display: block;
width: 80%;
background-color: #f9c2ff;
padding: 10px;
margin-bottom: 10px;
}
.inline-element {
display: inline;
background-color: #c2f9ff;
padding: 5px;
}
.inline-block-element {
display: inline-block;
width: 100px;
background-color: #ffc2f9;
padding: 10px;
}
Outcome:
- The block element will occupy the full width and start on a new line.
- The inline element will flow within the line without starting a new line.
- The inline-block element will allow setting width and height while still flowing inline.
10. How do you select all paragraph elements inside a div using CSS?
To select all <p>
(paragraph) elements that are descendants of a <div>
element, you can use the descendant selector in CSS. The descendant selector targets elements that are nested within another specified element, regardless of their depth in the hierarchy.
Syntax:
div p {
/* CSS properties */
}
Explanation:
div p
selects all<p>
elements that are inside any<div>
element.- This includes
<p>
elements that are direct children or nested deeper within other elements inside the<div>
.
Example:
<div class="content">
<p>This paragraph is inside the div.</p>
<section>
<p>This paragraph is also inside the div, within a section.</p>
</section>
</div>
<p>This paragraph is outside the div.</p>
div p {
color: blue;
font-size: 16px;
}
Result:
- The first two
<p>
elements inside the<div>
with classcontent
will have blue text and a font size of 16px. - The third
<p>
outside the<div>
will not be affected by this CSS rule.
If You Want to Select Only Direct Children: To target only the <p>
elements that are direct children of a <div>
, use the child selector (>
).
Syntax:
div > p {
/* CSS properties */
}
Example:
<div class="content">
<p>This paragraph is a direct child of the div.</p>
<section>
<p>This paragraph is inside a section within the div.</p>
</section>
</div>
div > p {
color: green;
font-weight: bold;
}
Result:
- Only the first
<p>
inside.content
will have green, bold text. - The second
<p>
inside the<section>
will not be affected.
Additional Tips:
- Multiple Selectors:
- You can combine selectors for more specific targeting.
- Example:
div.content p.intro { /* styles */ }
targets<p>
elements with classintro
inside<div>
elements with classcontent
.
- Universal Descendant Selector:
div p
selects all<p>
elements inside any<div>
.- To limit it to a specific
<div>
, use a class or ID. - Example:
.main-content p { /* styles */ }
targets<p>
elements inside elements with classmain-content
.
Understanding selector specificity and the different types of selectors allows for precise and efficient styling in CSS.
11. What are the different types of selectors in CSS?
CSS selectors are used to select and apply styles to HTML elements. Different types of selectors help target elements in various ways:
- Universal Selector (
*
) – Selects all elements.* { margin: 0; padding: 0; }
- Type Selector (Element Selector) – Targets all instances of a specific HTML element.
p { color: blue; }
- Class Selector (
.
) – Selects elements with a specific class..box { border: 1px solid black; }
- ID Selector (
#
) – Selects a single element with a specific ID.#header { font-size: 24px; }
- Group Selector (
,
) – Selects multiple elements at once.h1, h2, h3 { font-family: Arial; }
- Attribute Selector – Targets elements based on attributes.
input[type="text"] { background-color: yellow; }
- Combinator Selectors – Target elements based on their relationship to other elements.
div > p { color: red; }
12. How does CSS specificity work?
CSS specificity determines which styles are applied when multiple rules target the same element. It follows a hierarchical approach:
- Inline styles – Highest specificity (e.g.,
style="color: red;"
) - IDs – More specific than classes and elements.
- Classes, attributes, and pseudo-classes – Medium specificity.
- Element selectors and pseudo-elements – Lowest specificity.
Example of Specificity Calculation:
h1 { color: blue; } /* Specificity = 1 */
.title { color: red; } /* Specificity = 10 */
#main-title { color: green; } /* Specificity = 100 */
The element will be green because the ID selector has the highest specificity.
13. What is a pseudo-class in CSS? Provide examples.
A pseudo-class is used to define the special state of an element. It allows you to style elements based on user interaction or element state.
Common Pseudo-Classes:
:hover
– When the user hovers over an element.:focus
– When an element gains focus.:nth-child()
– Selects elements based on their position in the parent.
Example:
button:hover {
background-color: blue;
}
input:focus {
border-color: green;
}
14. What is a pseudo-element in CSS? Provide examples.
Pseudo-elements allow you to style specific parts of an element, such as the first letter or first line.
Common Pseudo-Elements:
::before
– Inserts content before an element.::after
– Inserts content after an element.::first-letter
– Styles the first letter of an element.
Example:
p::first-letter {
font-size: 2em;
color: red;
}
.box::before {
content: "[Start] ";
}
15. How do attribute selectors work in CSS?
Attribute selectors target elements based on their attributes and values.
Types of Attribute Selectors:
[attr]
– Selects elements with the specified attribute.[attr="value"]
– Selects elements with the exact attribute value.[attr^="value"]
– Selects elements with an attribute value starting with a specific string.[attr$="value"]
– Selects elements with an attribute value ending with a specific string.[attr*="value"]
– Selects elements with an attribute value containing a specific string.
Example:
input[type="checkbox"] {
accent-color: blue;
}
16. Explain the difference between the child (>) and descendant (space) combinators in CSS.
- Child Combinator (
>
) – Selects direct children of a specified element. - Descendant Combinator (space) – Selects all descendants (children, grandchildren, etc.) of a specified element.
Example:
div > p {
color: red; /* Direct child <p> inside <div> */
}
div p {
color: blue; /* All <p> inside <div> */
}
17. How can you apply styles to an element when the user hovers over it?
The :hover
pseudo-class is used to apply styles when the user hovers over an element.
Example:
a:hover {
text-decoration: underline;
color: green;
}
18. What is the universal selector in CSS, and how is it used?
The universal selector (*
) targets all elements on the page.
Example:
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
This resets margin and padding for all elements, ensuring consistency across browsers.
19. How do you target elements that are the first child of their parent in CSS?
Use the :first-child
pseudo-class to target the first child of a parent.
Example:
p:first-child {
font-weight: bold;
}
This makes the first <p>
child of any parent bold.
20. What is the :nth-child() selector in CSS, and how does it work?
The :nth-child()
selector allows you to select elements based on their position in the parent element.
Syntax:
:nth-child(n)
Where n
can be a number, keyword, or formula (e.g., odd
, even
, 3n+1
).
Example:
li:nth-child(odd) {
background-color: lightgray;
}
li:nth-child(2) {
color: blue;
}
This applies styles to odd list items and the second list item specifically.
21. What are the different positioning schemes in CSS?
CSS provides five main positioning schemes that control how elements are placed within a web page:
- Static (default): Elements appear in the normal document flow. This is the default positioning for all elements.
- Relative: The element is positioned relative to its normal position. It can be shifted using
top
,right
,bottom
, andleft
properties without affecting surrounding elements. - Absolute: The element is removed from the normal document flow and is positioned relative to the nearest positioned ancestor (other than
static
). If no such ancestor exists, it is positioned relative to the document body. - Fixed: The element is removed from the normal document flow and positioned relative to the viewport, meaning it stays in the same position even when the page is scrolled.
- Sticky: The element toggles between
relative
andfixed
depending on the scroll position. It behaves likerelative
until it reaches a specified threshold, after which it acts likefixed
.
22. Explain the difference between relative, absolute, fixed, and static positioning.
- Static: This is the default positioning for all elements. The element follows the normal flow of the document.
- Relative: The element is positioned relative to its original position. For example:
.box {
position: relative;
top: 20px;
left: 10px;
}
This shifts the element 20px down and 10px to the right from its original spot.
- Absolute: The element is positioned relative to the closest ancestor with
position: relative
,absolute
, orfixed
. If no such ancestor exists, it positions relative to the body:
.box {
position: absolute;
top: 50px;
left: 30px;
}
- Fixed: The element is positioned relative to the viewport and does not move when the page is scrolled:
.box {
position: fixed;
top: 0;
left: 0;
}
23. How does the CSS float property work?
The float
property in CSS allows elements to be positioned to the left or right of their container, allowing text or inline elements to wrap around it. This is commonly used for creating layouts with images and text.
- Syntax:
.box {
float: left;
width: 200px;
}
- Values:
left
– The element floats to the left.right
– The element floats to the right.none
– The element does not float (default).inherit
– The element inherits the float value from its parent.
24. What is Flexbox in CSS, and when would you use it?
Flexbox, or Flexible Box Layout, is a one-dimensional layout model that allows you to design complex layouts with ease by aligning and distributing space among items in a container.
- Use Cases:
- Aligning items vertically or horizontally.
- Distributing space between items.
- Making items grow or shrink to fill available space.
- Example:
.container {
display: flex;
justify-content: center;
align-items: center;
}
This centers the items both horizontally and vertically.
25. How do CSS Grid and Flexbox differ?
- Flexbox: One-dimensional layout (either row or column).
- Grid: Two-dimensional layout (both rows and columns).
- Flexbox Use Case: Best for linear, single-axis layouts.
- Grid Use Case: Best for complex layouts with multiple rows and columns.
- Example (Grid):
.container {
display: grid;
grid-template-columns: repeat(3, 1fr);
}
26. How can you create a two-column layout using CSS?
A simple two-column layout can be created using Flexbox or Grid.
Flexbox Example:
.container {
display: flex;
}
.left, .right {
width: 50%;
}
Grid Example:
.container {
display: grid;
grid-template-columns: 1fr 1fr;
}
27. What is the z-index property in CSS, and how does it function?
The z-index
property controls the stacking order of elements. Elements with higher z-index
values are placed in front of those with lower values.
- Syntax:
.box {
position: absolute;
z-index: 10;
}
- Important Notes:
z-index
only works with positioned elements (relative
,absolute
,fixed
,sticky
).
28. How do you make an element stick to the top of the page when scrolling?
You can achieve this using position: sticky
.
Example:
.navbar {
position: sticky;
top: 0;
}
This ensures the element sticks to the top when the user scrolls.
29. What is the CSS display property, and what are some of its values?
The display
property defines how an element is displayed in the document.
- Common Values:
block
– Element takes full width.inline
– Element takes only necessary width.inline-block
– Similar to inline but allows block properties.flex
– Enables Flexbox.grid
– Enables CSS Grid.none
– Hides the element.
- Example:
.box { display: flex; }
30. How can you create a responsive design using CSS?
Responsive design ensures a website looks good on different screen sizes. This can be achieved using:
- Media Queries:
@media (max-width: 768px) {
.container {
flex-direction: column;
}
}
- Flexbox and Grid:
.container {
display: flex;
flex-wrap: wrap;
}
- Percentage Widths:
.box {
width: 50%;
}
- Viewport Units:
.box {
width: 100vw;
}
31. What are CSS preprocessors, and can you name a few?
- CSS preprocessors are scripting languages that extend the capabilities of CSS by introducing features like variables, nesting, mixins, and functions. They help in writing more maintainable and efficient CSS code by allowing developers to use programming constructs that are not available in standard CSS.
Examples include:
- Sass (Syntactically Awesome Stylesheets)
$primary-color: #333;
body {
color: $primary-color;
}
- Less (Leaner CSS)
@primary-color: #333;
body {
color: @primary-color;
}
- Stylus
primary-color = #333
body
color primary-color
32. How do you implement CSS animations?
- CSS animations are implemented using the
@keyframes
rule to define the animation sequence and theanimation
property to apply the animation to an element. Animations can control various CSS properties over time, allowing for complex visual effects.
Example:
@keyframes fadeIn {
from { opacity: 0; }
to { opacity: 1; }
}
.element {
animation: fadeIn 2s ease-in-out;
}
33. What are CSS transitions, and how do they differ from animations?
- CSS transitions allow property changes in CSS values to occur smoothly over a specified duration when a triggering event happens (like hover or click). They are simpler than animations and are typically used for minor changes.
- Difference from animations: Transitions require a trigger to start and are limited to the change between two states, whereas animations can have multiple keyframes and run automatically or be controlled more precisely.
Example of a transition:
.button {
background-color: blue;
transition: background-color 0.3s ease;
}
.button:hover {
background-color: green;
}
34. How can you optimize CSS for better performance?
- Optimize CSS performance by:
- Minimizing and compressing CSS files to reduce load times.
- Removing unused CSS to decrease file size.
- Using shorthand properties to reduce code length.
- Placing CSS in the
<head>
to ensure styles are loaded before rendering. - Avoiding excessive use of complex selectors which can slow down rendering.
- Leveraging CSS caching by setting appropriate cache headers.
- Using CSS sprites to reduce the number of HTTP requests.
Example of using shorthand:
/* Instead of */
margin-top: 10px;
margin-right: 10px;
margin-bottom: 10px;
margin-left: 10px;
/* Use */
margin: 10px;
35. What is a CSS sprite, and how is it used?
- CSS sprites are a technique where multiple images are combined into a single image file. This reduces the number of HTTP requests needed to load multiple images, improving page load performance.
- Usage involves setting the combined image as a background and adjusting the
background-position
to display the desired part of the sprite.
Example:
.icon {
background: url('sprite.png') no-repeat;
}
.icon-home {
background-position: 0 0;
}
.icon-search {
background-position: -50px 0;
}
36. How do media queries work in CSS?
- Media queries allow you to apply CSS styles based on the characteristics of the user’s device, such as screen size, resolution, or orientation. They enable responsive design by adapting the layout to different environments.
Example:
@media (max-width: 600px) {
.container {
flex-direction: column;
}
}
37. What is the purpose of the box-sizing property in CSS?
- The
box-sizing
property defines how the total width and height of an element are calculated. By default,box-sizing
is set tocontent-box
, which excludes padding and border from the total size. Setting it toborder-box
includes padding and border within the element’s total width and height, making layout calculations more predictable.
Example:
.element {
box-sizing: border-box;
width: 200px; /* Includes padding and border */
}
38. How can you create a CSS triangle?
- CSS triangles are created using borders. By setting the width and height of an element to zero and manipulating the border properties, you can create a triangle pointing in different directions.
Example of a downward-pointing triangle:
.triangle {
width: 0;
height: 0;
border-left: 50px solid transparent;
border-right: 50px solid transparent;
border-top: 100px solid red;
}
39. What is the difference between visibility: hidden and display: none in CSS?
visibility: hidden
hides the element but still occupies space in the layout. The element is invisible, but its position and space are preserved.display: none
removes the element from the document flow entirely. It does not occupy any space, and the layout behaves as if the element does not exist.
Example:
.hidden {
visibility: hidden; /* Element is hidden but space is kept */
}
.none {
display: none; /* Element is removed from the layout */
}
40. How do you apply a style only to specific devices or screen sizes using CSS?
- Applying styles to specific devices or screen sizes is achieved using media queries. By defining conditions based on device characteristics like width, height, or resolution, you can target and apply styles to particular devices or screen sizes.
Example targeting devices with a maximum width of 768px:
@media (max-width: 768px) {
.sidebar {
display: none;
}
.content {
width: 100%;
}
}
Learn More: Carrer Guidance | Hiring Now!
AWS Interview Guide: Top 30 Questions and Answers
SSIS Interview Questions for Freshers with Answers
Selenium Cucumber scenario based Interview Questions and Answers
Tableau Scenario based Interview Questions and Answers
Unix Interview Questions and Answers
RabbitMQ Interview Questions and Answers
Kotlin Interview Questions and Answers for Developers
Mocha Interview Questions and Answers for JavaScript Developers