Are you preparing for a Chella Software interview? To help you out, we have compiled a list of the top 20 interview questions commonly asked at Chella Software, along with detailed answers to help you prepare effectively.
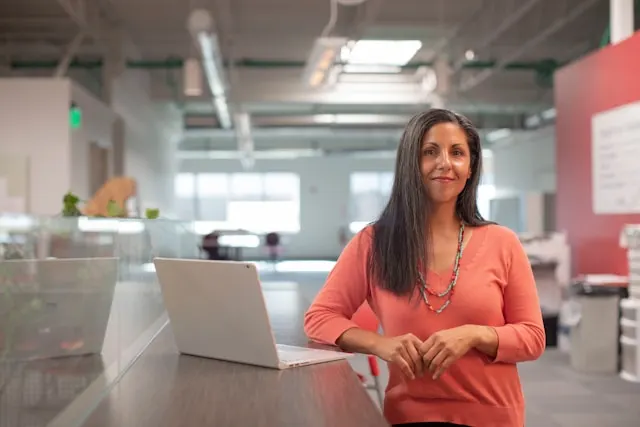
Chella Software interview questions and answers
- What is your understanding of the software development lifecycle (SDLC)?
- Can you explain the difference between functional and non-functional requirements?
- What programming languages are you proficient in?
- Describe a challenging technical problem you’ve encountered and how you solved it.
- What is Agile methodology?
- How do you ensure code quality?
- What are design patterns? Can you name a few?
- Explain what RESTful services are.
- What is version control? Why is it important?
- Describe your experience with databases.
- How do you handle tight deadlines?
- What tools do you use for debugging?
- Explain what microservices architecture is.
- How do you stay updated with technology trends?
- What is continuous integration/continuous deployment (CI/CD)?
- Can you explain what dependency injection is?
- Describe your experience working in teams.
- What steps do you take when troubleshooting an issue?
- What are some common security vulnerabilities in software applications?
- How would you approach learning a new technology?
1. What is your understanding of the software development lifecycle (SDLC)?
Answer:
The Software Development Lifecycle (SDLC) is a structured process that outlines the stages involved in developing software applications. It typically includes the following phases:
- Requirement Analysis: Gathering and analyzing user requirements to define what the software should accomplish.
- Design: Creating architecture and design specifications based on requirements. This phase can involve high-level design (HLD) and low-level design (LLD).
- Implementation: The actual coding of the software occurs here, where developers write code based on the design specifications.
- Testing: After implementation, the software undergoes various testing phases (unit testing, integration testing, system testing) to identify and fix defects.
- Deployment: The software is deployed to a production environment where users can access it.
- Maintenance: Post-deployment, the software requires updates and patches to fix bugs or improve functionality.
Understanding SDLC is crucial as it helps ensure that software is delivered on time, within budget, and meets quality standards.
2. Can you explain the difference between functional and non-functional requirements?
Answer:
Functional requirements specify what a system should do. They describe specific behaviors or functions of the system, such as:
- User authentication
- Data processing
- Business rules
Examples include “The system shall allow users to log in” or “The application must generate monthly reports.”
Non-functional requirements, on the other hand, define how a system performs its functions. They cover aspects such as:
- Performance (e.g., response time)
- Usability (e.g., user interface standards)
- Reliability (e.g., uptime requirements)
An example of a non-functional requirement might be “The system shall support 1000 concurrent users with a response time of less than 2 seconds.”
Both types are critical for ensuring that the software meets user expectations and business goals.
3. What programming languages are you proficient in?
Answer: In an interview, it’s important to mention specific programming languages relevant to the position. For instance:
- Java: Strongly typed language often used for enterprise applications.
- Python: Known for its readability and versatility; used in web development, data analysis, and automation.
- JavaScript: Essential for web development, enabling interactive web pages.
Be prepared to discuss your experience with each language, including any projects you’ve completed using them and frameworks you are familiar with (e.g., Spring for Java or Django for Python).
4. Describe a challenging technical problem you’ve encountered and how you solved it.
Answer: When answering this question, use the STAR method (Situation, Task, Action, Result):
- Situation: Briefly describe the context of the problem.
- Task: Explain what your responsibilities were in addressing it.
- Action: Detail the steps you took to solve the problem.
- Result: Share the outcome of your actions and any lessons learned.
For example, you might discuss a situation where you had to optimize a slow-running query in a database. You could explain how you analyzed query performance using tools like EXPLAIN PLAN, indexed certain columns, and ultimately reduced query execution time by 50%.
5. What is Agile methodology?
Answer: Agile is an iterative approach to software development that emphasizes flexibility, collaboration, and customer feedback. Key principles include:
- Iterative Development: Software is developed in small increments called sprints (typically 2-4 weeks), allowing teams to adapt based on feedback.
- Collaboration: Agile promotes close collaboration between cross-functional teams including developers, testers, and stakeholders.
- Customer Involvement: Regular feedback from customers ensures that the product meets their needs throughout development.
Agile methodologies include frameworks like Scrum and Kanban, which help manage tasks and workflows effectively.
6. How do you ensure code quality?
Answer: Ensuring code quality involves several practices:
- Code Reviews: Regular reviews by peers help catch issues early and promote knowledge sharing among team members.
- Unit Testing: Writing tests for individual components ensures they function correctly before integration.
- Static Code Analysis: Tools like SonarQube can analyze code for potential issues without executing it.
- Continuous Integration/Continuous Deployment (CI/CD): Automating builds and tests helps maintain code quality by ensuring that changes do not introduce new bugs.
By implementing these practices consistently, developers can maintain high standards of code quality throughout the project lifecycle.
7. What are design patterns? Can you name a few?
Answer: Design patterns are reusable solutions to common problems in software design. They provide templates for solving issues related to object creation, structure, or behavior within applications. Some common design patterns include:
- Singleton Pattern: Ensures a class has only one instance and provides a global point of access to it.
- Observer Pattern: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified.
- Factory Pattern: Provides an interface for creating objects in a superclass but allows subclasses to alter the type of created objects.
Understanding these patterns can help improve code maintainability and scalability.
8. Explain what RESTful services are.
Answer:
RESTful services are web services that adhere to REST (Representational State Transfer) architectural principles. They use standard HTTP methods such as GET, POST, PUT, DELETE to perform operations on resources identified by URLs. Key characteristics include:
- Statelessness: Each request from client to server must contain all information needed to understand and process it; no session state is stored on the server.
- Resource-Based: Resources are represented using URIs (Uniform Resource Identifiers), allowing clients to interact with them through standard HTTP methods.
- JSON/XML Format: Data exchanged between client and server is typically formatted in JSON or XML for easy parsing.
RESTful services are widely used due to their simplicity and scalability in web applications.
9. What is version control? Why is it important?
Answer: Version control systems (VCS) track changes to files over time, allowing multiple collaborators to work on projects without conflict. Key benefits include:
- Collaboration: Multiple developers can work on different features simultaneously without overwriting each other’s changes.
- History Tracking: VCS maintains a history of changes made over time, making it easy to revert back if necessary.
- Branching/Merging: Developers can create branches for new features or experiments without affecting the main codebase; changes can later be merged back into the main branch after testing.
Popular version control systems include Git and Subversion (SVN).
10. Describe your experience with databases.
Answer: When discussing your database experience:
- Mention specific databases you’ve worked with (e.g., MySQL, PostgreSQL, MongoDB).
- Highlight your familiarity with SQL for relational databases or query languages for NoSQL databases.
- Discuss any experience with database design principles such as normalization or indexing strategies that improve performance.
You could also mention any projects where you designed schemas or optimized queries for better performance.
11. How do you handle tight deadlines?
Answer:
Handling tight deadlines requires effective time management strategies:
- Prioritization: Assess tasks based on urgency and importance; focus on high-impact tasks first.
- Breakdown Tasks: Divide larger tasks into smaller manageable units; this makes progress easier to track.
- Communication: Keep stakeholders informed about progress; if delays occur, communicate them early along with potential solutions.
- Avoid Perfectionism: Aim for functional deliverables rather than perfect ones; iterative improvements can follow after initial delivery.
Implementing these strategies helps manage workload effectively under pressure while maintaining quality output.
12. What tools do you use for debugging?
Answer:
Debugging tools vary by programming language but commonly include:
- Integrated Development Environments (IDEs) like Visual Studio or Eclipse that offer built-in debugging features such as breakpoints and variable watches.
- Logging Libraries, such as Log4j or Winston in Node.js applications; these help track application behavior during runtime.
- Profiling Tools, like Chrome DevTools for web applications; they provide insights into performance bottlenecks and memory usage.
Using these tools effectively can significantly reduce debugging time and improve overall application stability.
13. Explain what microservices architecture is.
Answer:
Microservices architecture is an approach where an application is developed as a collection of small independent services that communicate over APIs. Each service focuses on a specific business capability and can be developed, deployed, and scaled independently. Key benefits include:
- Scalability: Services can be scaled individually based on demand without affecting other parts of the application.
- Resilience: Failure in one service does not necessarily bring down the entire application; other services continue functioning normally.
- Technology Agnostic: Different services can be built using different technologies best suited for their specific needs.
This architecture supports agile development practices by allowing teams to work independently on different services simultaneously.
14. How do you stay updated with technology trends?
Answer:
To stay current with technology trends:
- Follow industry blogs and websites like TechCrunch or Hacker News.
- Participate in online courses through platforms like Coursera or Udemy focusing on emerging technologies.
- Attend webinars or tech meetups within your area of expertise; networking helps share knowledge with peers.
- Engage in open-source projects on GitHub; contributing keeps skills sharp while learning from others’ codebases.
Staying updated ensures that skills remain relevant in a rapidly evolving tech landscape.
15. What is continuous integration/continuous deployment (CI/CD)?
Answer:
CI/CD is a set of practices aimed at improving software development processes through automation:
- Continuous Integration (CI) involves automatically integrating code changes from multiple contributors into a shared repository several times a day. Automated tests run against these integrations to detect issues early.
- Continuous Deployment (CD) extends CI by automatically deploying all code changes that pass tests into production environments without manual intervention.
This practice reduces integration problems while allowing teams to deliver updates more frequently and reliably.
16. Can you explain what dependency injection is?
Answer:
Dependency Injection (DI) is a design pattern used primarily in object-oriented programming that allows an object’s dependencies to be provided externally rather than created internally within the object itself. This promotes loose coupling between components by allowing them to interact through interfaces rather than concrete implementations.
Benefits of DI include:
- Improved testability since dependencies can be mocked during unit tests.
- Enhanced maintainability as changes in dependencies do not require modifications within dependent classes.
- Greater flexibility as different implementations can be injected at runtime based on configuration settings or environment needs.
Frameworks such as Spring in Java utilize DI extensively for managing application components effectively.
17. Describe your experience working in teams.
Answer: When discussing team experiences:
- Highlight collaborative projects where teamwork was essential for success.
- Mention specific roles you’ve played within teams—such as lead developer or scrum master—and how you facilitated communication among team members.
- Discuss how you’ve resolved conflicts or contributed positively during challenging situations by promoting open dialogue or focusing on common goals.
Effective teamwork enhances productivity while fostering innovation through diverse perspectives.
18. What steps do you take when troubleshooting an issue?
Answer: Troubleshooting involves systematic steps:
- Identify the problem clearly—gather information about symptoms from users or logs.
- Reproduce the issue—attempting to replicate it helps confirm its existence while providing context about its occurrence.
- Analyze potential causes—consider recent changes made before problems arose; check configurations or dependencies involved.
- Implement fixes—try solutions incrementally while documenting changes made during troubleshooting efforts.
- Verify resolution—ensure that fixes resolve issues without introducing new problems before closing tickets or notifying users about resolution status.
Following this structured approach helps ensure thoroughness while minimizing disruption during troubleshooting efforts.
19. What are some common security vulnerabilities in software applications?
Answer: Common security vulnerabilities include:
- SQL Injection: Attackers manipulate SQL queries by injecting malicious input through forms or URLs leading to unauthorized data access.
- Cross-Site Scripting (XSS): Malicious scripts are injected into trusted websites viewed by users; attackers exploit this vulnerability to steal cookies or session tokens.
- Cross-Site Request Forgery (CSRF): Users unknowingly execute unwanted actions on behalf of authenticated users due to forged requests sent from malicious websites targeting their sessions.
- Insecure Direct Object References (IDOR): Attackers gain access to unauthorized resources by manipulating input parameters used in requests without proper validation checks implemented server-side.
Mitigating these vulnerabilities involves implementing secure coding practices such as input validation/sanitization techniques alongside regular security audits.
20. How would you approach learning a new technology?
Answer: When learning new technology:
- Start with foundational knowledge—read official documentation/tutorials focusing on core concepts before diving deeper into advanced topics.
- Engage hands-on—build small projects utilizing new technologies; practical experience reinforces theoretical understanding.
- Join communities/forums—participate actively in discussions around technology-related topics where experts share insights & resources.
- Stay consistent—set aside regular time weekly dedicated solely towards learning & practicing new skills ensuring steady progress over time.
By following this structured approach towards learning new technologies ensures comprehensive understanding leading towards mastery eventually.
These questions cover various aspects of software development knowledge required at Chella Software while providing insights into both technical skills & soft skills necessary within collaborative environments!
Learn More: Carrer Guidance
Tosca interview question and answers- Basic to Advanced
SQL Queries Interview Questions and Answers
PySpark Interview Questions and Answers- Basic to Advanced
Kubernetes Interview Questions and Answers- Basic to Advanced
Embedded C Interview Questions with Detailed Answers- Basic to Advanced
Zoho Technical Support Engineer Interview Questions and Answers