Are you preparing for an AWS Lambda interview? AWS Lambda is a serverless compute service provided by Amazon Web Services (AWS) that automatically manages the underlying infrastructure and scales applications in response to events. To help you out, we have compiled 50 essential AWS Lambda interview questions with detailed answers to help you prepare effectively.
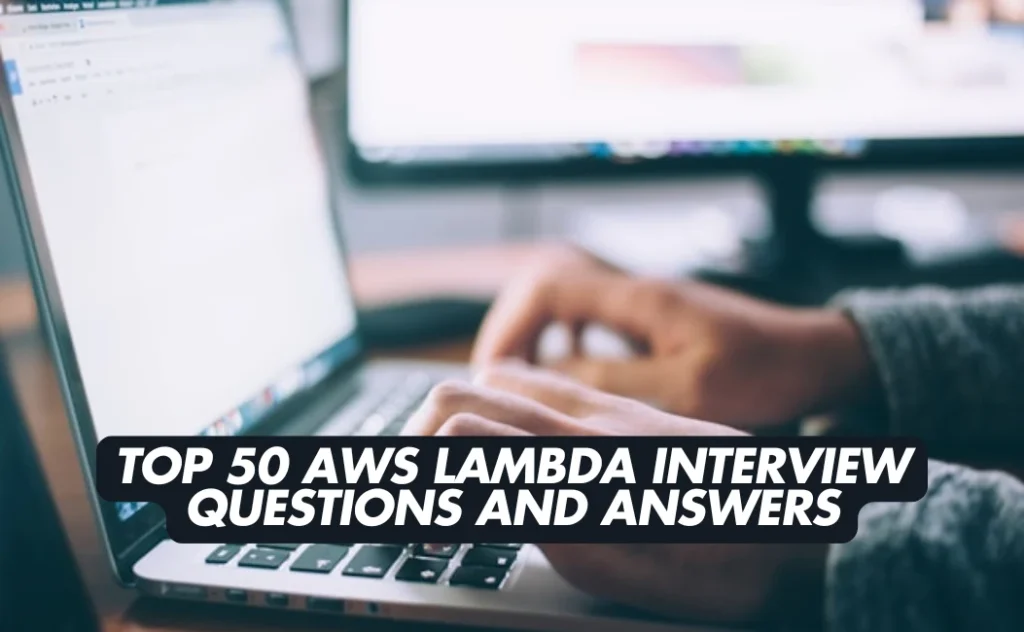
Top 50 AWS Lambda Interview Questions and Answers
- What is AWS Lambda?
- What are the main components of a Lambda function?
- How does AWS Lambda handle scaling?
- What are some common use cases for AWS Lambda?
- How do you monitor and troubleshoot AWS Lambda functions?
- What are the limitations of AWS Lambda?
- How do you secure AWS Lambda functions?
- Can you explain the concept of cold starts in AWS Lambda?
- How do you optimize the performance of AWS Lambda functions?
- How does AWS Lambda integrate with other AWS services?
- What is the AWS Lambda execution environment?
- How does AWS Lambda handle failures during function execution?
- What are AWS Lambda Layers?
- How do you manage environment variables in AWS Lambda?
- What is the maximum execution timeout for an AWS Lambda function?
- How does AWS Lambda handle concurrent executions?
- What is AWS Lambda Provisioned Concurrency?
- How can you reduce the cold start time in AWS Lambda?
- What is the /tmp directory in AWS Lambda?
- How do you manage dependencies in AWS Lambda functions?
- How does AWS Lambda integrate with Amazon API Gateway?
- What is AWS Lambda@Edge?
- How can you manage different environments (e.g., development, staging, production) in AWS Lambda?
- What is the role of AWS Lambda in a microservices architecture?
- How do you handle exceptions in AWS Lambda functions?
- Can you explain the concept of idempotency in AWS Lambda functions?
- How can you implement authentication and authorization in AWS Lambda?
- What are some best practices for writing AWS Lambda functions?
- How does AWS Lambda support versioning and aliases?
- What is the AWS Lambda execution model?
- How can you test AWS Lambda functions locally?
- What is the difference between AWS Lambda and AWS Fargate?
- How does AWS Lambda handle environment variables?
- What is the AWS Lambda function lifecycle?
- How can you automate the deployment of AWS Lambda functions?
- What are the security best practices for AWS Lambda?
- How does AWS Lambda pricing work?
- What is AWS Lambda Power Tuning?
- How can you schedule AWS Lambda functions?
- What is the maximum payload size for AWS Lambda function invocation?
- How does AWS Lambda integrate with Amazon S3?
- What are AWS Lambda Destinations?
- How can you control and manage concurrency in AWS Lambda?
- What is the purpose of AWS Lambda Layers?
- How does AWS Lambda handle function timeouts?
- Can you explain the AWS Lambda pricing model?
- How can you implement versioning in AWS Lambda?
- What are the deployment package size limits for AWS Lambda?
- How does AWS Lambda integrate with Amazon DynamoDB?
- What is the role of AWS Lambda in event-driven architectures?
1. What is AWS Lambda?
AWS Lambda is a serverless compute service provided by Amazon Web Services (AWS) that allows you to run code without provisioning or managing servers. It automatically scales applications by running code in response to events and manages the underlying compute resources, enabling developers to focus solely on writing code.
2. What are the main components of a Lambda function?
The main components of a Lambda function include:
- Handler: The entry point method in your code that processes the invocation event.
- Event: The JSON-formatted input data that triggers the function’s execution.
- Context: Provides methods and properties that provide information about the invocation, function, and execution environment.
3. How does AWS Lambda handle scaling?
AWS Lambda automatically scales by running code in response to each trigger. It manages the infrastructure to handle varying workloads, scaling from a few requests per day to thousands per second without manual intervention.
4. What are some common use cases for AWS Lambda?
Common use cases for AWS Lambda include:
- Real-time file processing: Processing files as they are uploaded to services like Amazon S3.
- Data transformation: Modifying data streams in real-time.
- Backend services: Serving as the backend for web and mobile applications.
- Event-driven applications: Responding to events from other AWS services or external sources.
5. How do you monitor and troubleshoot AWS Lambda functions?
Monitoring and troubleshooting AWS Lambda functions can be achieved using:
- AWS CloudWatch: For logging and monitoring metrics such as invocation count, duration, and error rates.
- AWS X-Ray: For tracing and analyzing requests to identify performance bottlenecks and errors.
6. What are the limitations of AWS Lambda?
Limitations of AWS Lambda include:
- Execution timeout: Maximum of 15 minutes per function execution.
- Deployment package size: Up to 50 MB for the zipped package.
- Memory allocation: Between 128 MB and 10,240 MB.
- Ephemeral disk space: 512 MB in the
/tmp
directory.
7. How do you secure AWS Lambda functions?
Securing AWS Lambda functions involves:
- Using AWS Identity and Access Management (IAM): To control access to Lambda functions and associated resources.
- Encrypting environment variables: To protect sensitive information.
- Implementing VPCs: For network isolation and security.
8. Can you explain the concept of cold starts in AWS Lambda?
A cold start occurs when a Lambda function is invoked after being idle, requiring AWS to provision a new container for execution, leading to increased latency. Warm starts reuse existing containers, resulting in faster execution.
9. How do you optimize the performance of AWS Lambda functions?
Performance optimization strategies include:
- Minimizing package size: To reduce deployment and initialization time.
- Reusing connections: By initializing SDK clients and database connections outside the handler function.
- Allocating appropriate memory: Higher memory allocation provides more CPU power, reducing execution time.
10. How does AWS Lambda integrate with other AWS services?
AWS Lambda integrates seamlessly with various AWS services, such as:
- Amazon S3: Triggering functions upon object uploads.
- Amazon DynamoDB: Responding to data modifications.
- Amazon API Gateway: Handling HTTP requests.
- Amazon SNS and SQS: Processing messages and notifications.
11. What is the AWS Lambda execution environment?
The execution environment is a runtime environment that AWS Lambda provides to run your function code. It includes the operating system, system libraries, and language runtime. Each function execution occurs in a secure and isolated environment.
12. How does AWS Lambda handle failures during function execution?
AWS Lambda handles failures differently based on the invocation type:
- Synchronous invocations: Returns the error to the caller application.
- Asynchronous invocations: Retries the execution twice, and if it still fails, the event can be sent to a Dead Letter Queue (DLQ) if configured.
13. What are AWS Lambda Layers?
AWS Lambda Layers are a distribution mechanism for libraries, custom runtimes, and other dependencies that can be used by multiple Lambda functions. By separating these components from the main function code, layers promote code reuse and can significantly reduce the size of deployment packages. Each function can include up to five layers, and during execution, AWS Lambda extracts the layer contents into the /opt
directory within the execution environment, making them accessible to your function code.
14. How do you manage environment variables in AWS Lambda?
Environment variables in AWS Lambda are key-value pairs that allow you to pass configuration settings to your function code without embedding them directly. You can set these variables using the AWS Management Console, AWS CLI, AWS SDKs, or infrastructure as code tools like AWS CloudFormation. In the console, navigate to your function’s configuration page, locate the “Environment variables” section, and add the desired key-value pairs.
For sensitive information, such as database credentials or API keys, AWS Lambda encrypts environment variables at rest. To enhance security, you can configure Lambda to use a customer-managed AWS Key Management Service (KMS) key for encryption. Additionally, enabling encryption helpers in the console ensures that environment variables are encrypted in transit.
Within your function code, you can access environment variables using standard methods provided by your programming language. For example, in Python, you can use os.environ['VARIABLE_NAME']
to retrieve the value.
By effectively managing environment variables and implementing proper encryption, you can securely and flexibly configure your Lambda functions.
15. What is the maximum execution timeout for an AWS Lambda function?
The maximum execution timeout for an AWS Lambda function is 15 minutes (900 seconds). This means that any function must complete its execution within this timeframe; otherwise, AWS Lambda will terminate the function, and the invocation will result in a timeout error.
It’s important to note that while AWS Lambda allows a maximum timeout of 15 minutes, other AWS services that interact with Lambda may have different timeout limits. For instance, Amazon API Gateway has a maximum integration timeout of 29 seconds when invoking a Lambda function. Therefore, when designing your Lambda functions, ensure that their execution time aligns with the timeout constraints of any integrated services to prevent unexpected timeouts.
To configure the timeout setting for a Lambda function, you can specify the desired timeout value in the function’s configuration. This can be done through the AWS Management Console, AWS CLI, or infrastructure as code tools like AWS CloudFormation. Adjusting the timeout setting allows you to control how long a function is allowed to run before it is forcibly terminated.
16. How does AWS Lambda handle concurrent executions?
AWS Lambda manages concurrency by allocating a separate execution environment for each concurrent invocation of a function. The default concurrency limit per AWS Region is 1,000 concurrent executions, but this can be adjusted by requesting a limit increase through AWS Support. To control the number of concurrent executions for a specific function, you can set a reserved concurrency limit, which ensures that the function doesn’t exceed the specified number of concurrent instances. This is useful for controlling costs and managing the load on downstream resources.
17. What is AWS Lambda Provisioned Concurrency?
Provisioned Concurrency is a feature that initializes a specified number of execution environments so that they are prepared to respond immediately to your function’s invocations. This reduces the latency caused by cold starts, providing more consistent performance for latency-sensitive applications. When you configure Provisioned Concurrency, AWS Lambda keeps the specified number of environments warm and ready to handle incoming requests. This feature is particularly beneficial for applications that require predictable start-up latency.
18. How can you reduce the cold start time in AWS Lambda?
To minimize cold start times in AWS Lambda, consider the following strategies:
- Use Provisioned Concurrency: Keeps execution environments warm to eliminate cold starts.
- Optimize Deployment Package Size: Smaller packages reduce initialization time.
- Choose Appropriate Runtimes: Some runtimes have faster initialization times than others.
- Initialize Outside the Handler: Load dependencies and set up connections outside the handler function to reuse them across invocations.
By implementing these practices, you can achieve more consistent and lower latency in your Lambda functions.
19. What is the /tmp directory in AWS Lambda?
The /tmp
directory in AWS Lambda provides ephemeral storage of up to 512 MB that can be used to store temporary data during function execution. This storage is unique to each function instance and persists only for the lifetime of the execution environment. It’s useful for tasks that require temporary file storage, such as processing large files or caching data between invocations. However, since the storage is ephemeral, it’s important to not rely on it for persistent data storage.
20. How do you manage dependencies in AWS Lambda functions?
Managing dependencies in AWS Lambda functions involves packaging your function code along with any required libraries. For Python functions, this can be done by creating a deployment package that includes your function code and dependencies installed in the appropriate directory structure. Alternatively, you can use AWS Lambda Layers to separate dependencies from your function code, allowing you to manage and reuse them across multiple functions. This approach simplifies deployment and reduces package size.
21. How does AWS Lambda integrate with Amazon API Gateway?
AWS Lambda integrates with Amazon API Gateway to create RESTful APIs. API Gateway acts as a front door for applications to access data, business logic, or functionality from backend services. When an HTTP request is made to an API Gateway endpoint, it can trigger a Lambda function to process the request and return a response. This setup enables the creation of serverless web applications without managing any infrastructure.
22. What is AWS Lambda@Edge?
AWS Lambda@Edge allows you to run Lambda functions at AWS Edge locations worldwide in response to Amazon CloudFront events. This enables you to customize content delivery and execute logic closer to users, reducing latency. Common use cases include header manipulation, URL rewrites, and user authentication at the edge.
23. How can you manage different environments (e.g., development, staging, production) in AWS Lambda?
Managing different environments in AWS Lambda can be achieved by:
- Using separate Lambda functions: Deploy distinct functions for each environment.
- Employing aliases and versions: Create versions of a function and use aliases to point to different versions representing environments.
- Utilizing environment variables: Define environment-specific variables within the same function to adjust behavior based on the environment.
This approach ensures that changes in one environment do not affect others, facilitating safe development and deployment practices.
24. What is the role of AWS Lambda in a microservices architecture?
In a microservices architecture, AWS Lambda serves as a lightweight compute service to run individual microservices. Each Lambda function can encapsulate a single microservice, allowing for independent deployment, scaling, and management. This promotes a decoupled and modular system where each service can evolve separately.
25. How do you handle exceptions in AWS Lambda functions?
Exception handling in AWS Lambda functions involves:
- Try-catch blocks: Wrapping code in try-catch blocks to handle exceptions gracefully.
- Returning error messages: Providing meaningful error messages to the caller.
- Configuring Dead Letter Queues (DLQ): Setting up DLQs to capture failed events for asynchronous invocations, allowing for later analysis and reprocessing.
Proper exception handling ensures robustness and reliability in function execution.
26. Can you explain the concept of idempotency in AWS Lambda functions?
Idempotency refers to the property of an operation where performing it multiple times has the same effect as performing it once. In AWS Lambda, designing idempotent functions ensures that even if an event is processed multiple times (due to retries or duplicate events), the outcome remains consistent. This is crucial for maintaining data integrity and avoiding unintended side effects.
27. How can you implement authentication and authorization in AWS Lambda?
Authentication and authorization in AWS Lambda can be implemented by:
- Using Amazon Cognito: Integrating with Amazon Cognito to manage user authentication and access control.
- API Gateway authorizers: Configuring API Gateway to use Lambda authorizers (formerly known as custom authorizers) to control access to APIs.
- IAM roles and policies: Assigning appropriate IAM roles and policies to Lambda functions to control access to AWS resources.
These mechanisms ensure that only authorized users and services can invoke Lambda functions or access specific resources.
28. What are some best practices for writing AWS Lambda functions?
Best practices for writing AWS Lambda functions include:
- Keeping functions small and focused: Ensuring each function has a single responsibility.
- Minimizing deployment package size: Including only necessary dependencies to reduce cold start times.
- Reusing execution context: Initializing connections and variables outside the handler to leverage the execution environment reuse.
- Implementing proper error handling: Using try-catch blocks and meaningful error messages.
- Monitoring and logging: Utilizing CloudWatch for logs and setting up appropriate alarms.
Adhering to these practices leads to efficient, maintainable, and reliable Lambda functions.
29. How does AWS Lambda support versioning and aliases?
AWS Lambda allows you to publish versions of your functions, which are immutable snapshots of your function code and configuration. Aliases are pointers to specific versions and can be used to manage different environments or stages (e.g., development, production). This facilitates safe deployment practices, such as blue/green deployments and canary releases.
30. What is the AWS Lambda execution model?
The AWS Lambda execution model involves:
- Event source: An event triggers the Lambda function.
- Invocation: Lambda locates or creates an execution environment to run the function.
- Execution environment: A secure and isolated runtime environment where the function code executes.
- Handler function: The specific method within your code that Lambda calls to process the event.
- Termination: After execution, the environment may be frozen for reuse or terminated based on demand and scaling requirements.
Understanding this model is crucial for optimizing performance and resource utilization.
31. How can you test AWS Lambda functions locally?
Testing AWS Lambda functions locally can be done using:
- AWS SAM (Serverless Application Model) CLI: Provides the
sam local
command to run Lambda functions locally in a Docker container that simulates the Lambda environment. - AWS Cloud9: An integrated development environment that allows you to write, run, and debug Lambda functions in the cloud.
- LocalStack: A fully functional local AWS cloud stack that enables testing of Lambda functions and other AWS services locally.
These tools facilitate development and testing without deploying code to the AWS environment.
32. What is the difference between AWS Lambda and AWS Fargate?
AWS Lambda is a serverless, event-driven compute service that allows you to run code in response to events without provisioning or managing servers. It’s ideal for short-lived, event-driven tasks and supports various programming languages.
AWS Fargate is a serverless compute engine for containers, enabling you to run containerized applications without managing the underlying infrastructure. It integrates with Amazon Elastic Container Service (ECS) and Amazon Elastic Kubernetes Service (EKS), providing more control over the execution environment.
Key Differences:
- Execution Model:
- Lambda: Designed for short-duration, event-driven functions with a maximum execution timeout of 15 minutes.
- Fargate: Suitable for long-running, containerized applications without strict execution time limits.
- Scaling:
- Lambda: Automatically scales in response to incoming events, handling thousands of concurrent executions.
- Fargate: Scales by adjusting the number of running containers based on defined policies.
- Pricing:
- Lambda: Charges based on the number of requests and execution duration, with billing in millisecond increments.
- Fargate: Charges based on the allocated vCPU and memory resources per second for running containers.
- Use Cases:
- Lambda: Ideal for real-time file processing, data transformations, and event-driven applications.
- Fargate: Best suited for microservices architectures, batch processing, and applications requiring fine-grained control over the environment.
In summary, AWS Lambda is optimal for event-driven, short-lived tasks with minimal management overhead, while AWS Fargate is better suited for long-running, containerized applications requiring more control over the execution environment.
33. How does AWS Lambda handle environment variables?
AWS Lambda allows you to define environment variables that can be accessed within your function code. These variables enable you to customize function behavior without altering the code. Sensitive information, such as database credentials, can be encrypted using AWS Key Management Service (KMS) to enhance security. Environment variables can be managed through the AWS Management Console, AWS CLI, or infrastructure as code tools like AWS CloudFormation.
34. What is the AWS Lambda function lifecycle?
The AWS Lambda function lifecycle includes the following phases:
- Function Creation: You upload your code and configure settings such as memory allocation and timeout.
- Initialization: Lambda creates an execution environment, initializes the runtime, and runs the function’s initialization code.
- Invocation: The function’s handler is invoked in response to an event.
- Idle: After execution, the environment may be frozen and retained for subsequent invocations to reduce latency.
- Termination: The execution environment is eventually terminated when it’s no longer needed.
Understanding this lifecycle helps in optimizing function performance and managing resources effectively.
35. How can you automate the deployment of AWS Lambda functions?
Automating the deployment of AWS Lambda functions can be achieved using:
- AWS CloudFormation: Defines infrastructure as code, allowing you to provision and manage Lambda functions and related resources.
- AWS Serverless Application Model (SAM): An extension of CloudFormation optimized for serverless applications, simplifying the definition and deployment of Lambda functions.
- AWS CodePipeline and CodeDeploy: Facilitates continuous integration and deployment (CI/CD) of Lambda functions.
- Third-party frameworks: Tools like the Serverless Framework enable streamlined deployment processes.
These tools and services enable consistent, repeatable, and automated deployments, enhancing development efficiency and reliability.
36. What are the security best practices for AWS Lambda?
Security best practices for AWS Lambda include:
- Principle of Least Privilege: Assign minimal permissions necessary for the function to operate.
- Environment Variable Encryption: Use AWS KMS to encrypt sensitive data in environment variables.
- Network Security: Place functions within a Virtual Private Cloud (VPC) to control access to resources.
- Regular Updates: Keep dependencies and libraries up to date to mitigate vulnerabilities.
- Monitoring and Logging: Utilize AWS CloudWatch and AWS CloudTrail to monitor function activity and maintain audit trails.
Implementing these practices ensures that your Lambda functions operate securely within your AWS environment.
37. How does AWS Lambda pricing work?
AWS Lambda pricing is based on:
- Number of Requests: You are charged for the total number of requests across all functions.
- Duration: Measured from the time your code begins executing until it returns or otherwise terminates, rounded up to the nearest millisecond.
- Provisioned Concurrency: If enabled, you pay for the amount of concurrency you configure and the duration it is provisioned.
The free tier includes 1 million free requests and 400,000 GB-seconds of compute time per month, providing a cost-effective solution for many applications.
38. What is AWS Lambda Power Tuning?
AWS Lambda Power Tuning is an open-source tool that helps you optimize the memory and CPU allocation for your Lambda functions. By testing different memory configurations, it provides recommendations to achieve the best performance-to-cost ratio. This enables you to make data-driven decisions when allocating resources to your functions.
39. How can you schedule AWS Lambda functions?
You can schedule AWS Lambda functions using:
- Amazon CloudWatch Events (now Amazon EventBridge): Create rules that trigger functions at specified times or intervals using cron or rate expressions.
- AWS Lambda Console: Set up test events to manually invoke functions for testing purposes.
This scheduling capability is useful for tasks such as periodic data processing, maintenance, or generating reports.
40. What is the maximum payload size for AWS Lambda function invocation?
The maximum payload size for AWS Lambda function invocation varies based on the invocation method:
- Synchronous Invocations: The payload (event) size can be up to 6 MB.
- Asynchronous Invocations: The payload size is limited to 256 KB.
These limits include both the request and response payloads. It’s important to design your functions to handle these constraints, possibly by storing larger payloads in services like Amazon S3 and passing references to them in your events.
41. How does AWS Lambda integrate with Amazon S3?
AWS Lambda integrates with Amazon S3 by allowing you to configure S3 bucket events to trigger Lambda functions. For example, when an object is created in an S3 bucket, it can trigger a Lambda function to process the object. This integration is commonly used for tasks such as image processing, data validation, and real-time file processing.
42. What are AWS Lambda Destinations?
AWS Lambda Destinations provide a way to route the outcome of asynchronous function executions to specific targets. You can configure separate destinations for successful and failed executions, directing the results to services like Amazon SQS, Amazon SNS, another Lambda function, or EventBridge. This feature enhances the ability to build event-driven architectures with clear handling of function outcomes.
43. How can you control and manage concurrency in AWS Lambda?
AWS Lambda allows you to manage concurrency through:
- Reserved Concurrency: Sets the maximum number of concurrent executions for a function, ensuring it doesn’t exceed a specified limit.
- Provisioned Concurrency: Pre-warms a specified number of execution environments, reducing latency for highly critical functions.
These controls help in managing resource utilization and ensuring consistent performance.
44. What is the purpose of AWS Lambda Layers?
AWS Lambda Layers allow you to package and share code and dependencies separately from your function code. This promotes code reuse and can reduce the size of deployment packages. Layers can include libraries, custom runtimes, or other dependencies, and can be shared across multiple functions or even with other AWS accounts.
45. How does AWS Lambda handle function timeouts?
Each AWS Lambda function has a configurable timeout setting, ranging from 1 second to 15 minutes. If a function execution exceeds the specified timeout, Lambda terminates the execution and returns an error. Properly setting the timeout value is crucial to balance between allowing sufficient processing time and preventing excessive resource consumption.
46. Can you explain the AWS Lambda pricing model?
AWS Lambda pricing is based on two main factors:
- Number of Requests: You are charged per request, with the first 1 million requests per month included in the AWS Free Tier.
- Duration: Measured from the time your code begins executing until it returns or otherwise terminates, rounded up to the nearest millisecond.
Additional charges may apply for data transfer and provisioned concurrency.
47. How can you implement versioning in AWS Lambda?
AWS Lambda supports versioning, allowing you to create immutable versions of your functions. Each version is assigned a unique ARN and can be invoked directly or through aliases. Versioning facilitates deployment strategies like blue/green deployments and canary releases, enabling safer updates and rollbacks.
48. What are the deployment package size limits for AWS Lambda?
The deployment package size limits for AWS Lambda are:
- Compressed .zip file (direct upload): Up to 50 MB.
- Compressed .zip file (using S3): Up to 250 MB.
- Uncompressed size: Up to 250 MB.
For larger packages, consider using Lambda Layers or container images, which can be up to 10 GB in size.
49. How does AWS Lambda integrate with Amazon DynamoDB?
AWS Lambda integrates with Amazon DynamoDB through DynamoDB Streams. When enabled, changes to items in a DynamoDB table are captured in a stream, which can trigger a Lambda function. This integration enables real-time processing of data modifications, such as triggering workflows or updating other systems.
50. What is the role of AWS Lambda in event-driven architectures?
In event-driven architectures, AWS Lambda acts as an event processor, executing code in response to events from various sources like S3, DynamoDB, or API Gateway. This decouples event producers and consumers, allowing for scalable and maintainable systems where components can evolve independently.
Learn More: Carrer Guidance | Hiring Now!
Top 20+ Low Level Design (LLD) Interview Questions and Answers
SQL Interview Questions for 3 Years Experience with Answers
Advanced TOSCA Test Automation Engineer Interview Questions and Answers with 5+ Years of Experience
DSA Interview Questions and Answers
Angular Interview Questions and Answers for Developers with 5 Years of Experience
Android Interview Questions for Senior Developer with Detailed Answers
SQL Query Interview Questions for Freshers with Answers