Are you preparing for an interview with Avasoft? To help you out, we’ve compiled a list of over 30 top Avasoft interview questions and answers to help you confidently in your technical interview. These questions cover a wide range of topics, including Object-Oriented Programming (OOP), Python concepts, software testing methodologies, and more.
Whether you’re asked to explain a complex programming concept, discuss your approach to problem-solving, or demonstrate your understanding of software development processes, our guide provides detailed answers to help showcase your skills and experiences.
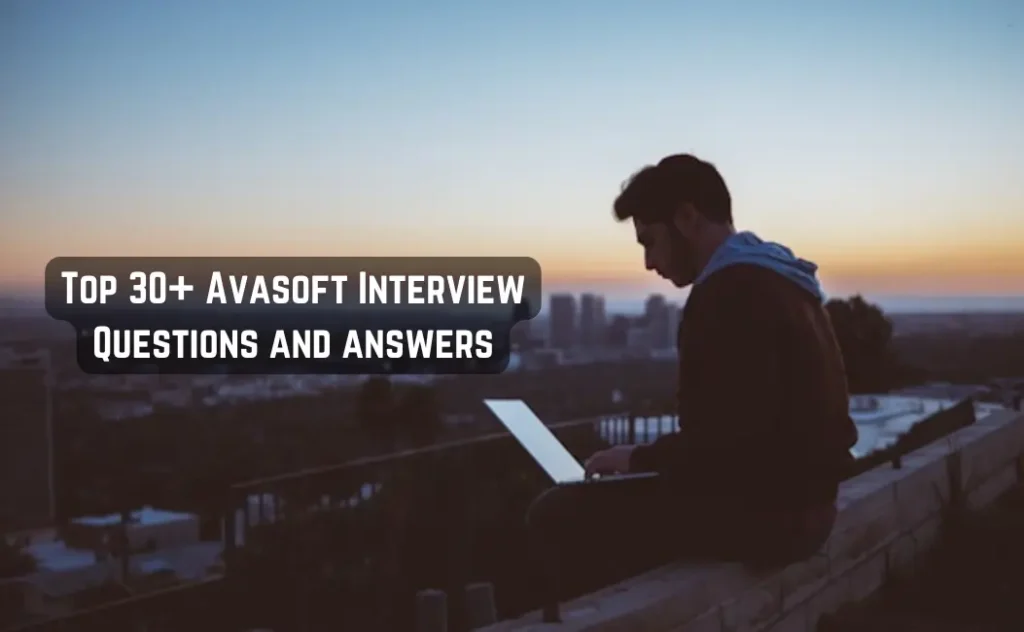
Top 30+ Avasoft Interview Questions and answers
- Explain the concept of Object-Oriented Programming (OOP) and its fundamental principles.
- What is the difference between method overloading and method overriding?
- Can you write a program to check if a number is an Armstrong number?
- What is regression testing, and how is it performed?
- Explain the difference between arrays and lists in Python.
- What is a stack, and how is it implemented in Python?
- What is a queue, and how is it implemented in Python?
- Explain the concept of recursion and provide an example in Python.
- What is the difference between a process and a thread?
- How does garbage collection work in Python?
- What is a lambda function in Python?
- Explain the concept of exception handling in Python.
- What is the purpose of the self parameter in Python class methods?
- What is a decorator in Python, and how is it used?
- What is the difference between manual testing and automated testing?
- Explain the Software Development Life Cycle (SDLC) and its phases.
- What is the purpose of version control systems, and how do they work?
- Describe the Agile methodology and its key principles.
- What is a RESTful API, and how does it differ from SOAP?
- Explain the concept of continuous integration and continuous deployment (CI/CD).
- What is the difference between black-box testing and white-box testing?
- Describe the Model-View-Controller (MVC) architecture.
- What is the purpose of unit testing, and how is it performed?
- What is the difference between functional and non-functional testing?
- Explain the concept of polymorphism in object-oriented programming.
- What is a deadlock in operating systems, and how can it be prevented?
- Describe the difference between synchronous and asynchronous communication.
- What is the purpose of middleware in software architecture?
- Explain the concept of normalization in database design.
- What is the difference between a primary key and a foreign key in a database?
- Describe the concept of multithreading and its benefits.
1. Explain the concept of Object-Oriented Programming (OOP) and its fundamental principles.
Answer: Object-Oriented Programming (OOP) is a programming paradigm centered around objects rather than actions. It emphasizes the following core principles:
- Encapsulation: This involves bundling the data (attributes) and methods (functions) that operate on the data into a single unit, known as a class. Encapsulation restricts direct access to some of an object’s components, which is a means of preventing unintended interference and misuse.
- Inheritance: This allows a new class to inherit attributes and methods from an existing class, promoting code reusability. The existing class is referred to as the parent or base class, and the new class is the child or derived class.
- Polymorphism: This enables methods to do different things based on the object it is acting upon, even though they share the same name. Polymorphism allows for methods to be overridden or overloaded, providing flexibility in code.
- Abstraction: This principle involves hiding the complex implementation details and showing only the necessary features of an object. Abstraction helps in reducing programming complexity and effort.
2. What is the difference between method overloading and method overriding?
Answer:
- Method Overloading: This occurs when multiple methods in the same class have the same name but different parameters (different type, number, or both). It allows a class to perform a single action in different ways. Example:
class MathOperations {
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
}
- Method Overriding: This happens when a subclass provides a specific implementation for a method that is already defined in its superclass. The method in the child class should have the same name, return type, and parameters as in the parent class. Example:
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
@Override
void sound() {
System.out.println("Dog barks");
}
}
3. Can you write a program to check if a number is an Armstrong number?
Answer: An Armstrong number (also known as a Narcissistic number) is a number that is equal to the sum of its own digits each raised to the power of the number of digits. For example, 153 is an Armstrong number because 1³ + 5³ + 3³ = 153.
Python Implementation:
def is_armstrong_number(num):
digits = [int(d) for d in str(num)]
num_digits = len(digits)
return num == sum(d ** num_digits for d in digits)
# Example usage:
number = 153
if is_armstrong_number(number):
print(f"{number} is an Armstrong number.")
else:
print(f"{number} is not an Armstrong number.")
4. What is regression testing, and how is it performed?
Answer: Regression testing is a type of software testing that ensures that recent code changes have not adversely affected existing functionalities. It involves re-running previously completed tests to verify that the software continues to perform as expected after modifications.
Steps to Perform Regression Testing:
- Identify Test Cases: Select test cases that cover the modified code and the affected functionalities.
- Prioritize Test Cases: Determine the order of execution based on the criticality and impact of the functionalities.
- Execute Test Cases: Run the selected test cases manually or using automated testing tools.
- Analyze Results: Compare the outcomes with expected results to identify any discrepancies.
- Report and Fix Bugs: Document any defects found and collaborate with the development team to resolve them.
- Re-test: After fixes are applied, re-run the tests to confirm that the issues have been resolved.
5. Explain the difference between arrays and lists in Python.
Answer:
- Arrays: In Python, arrays are provided by the
array
module and can only hold elements of the same data type. They are more memory-efficient for large sequences of homogeneous data. Example:
import array
# Creating an array of integers
int_array = array.array('i', [1, 2, 3, 4])
- Lists: Lists are built-in Python data structures that can hold elements of different data types. They are more flexible but may consume more memory compared to arrays. Example:
# Creating a list with mixed data types
mixed_list = [1, 'two', 3.0, [4, 5]]
6. What is a stack, and how is it implemented in Python?
Answer: A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle, meaning the last element added is the first to be removed.
Implementation in Python:
Python’s list
can be used to implement a stack, utilizing the append()
method to add elements and the pop()
method to remove them.
Example:
stack = []
# Pushing elements onto the stack
stack.append(1)
stack.append(2)
stack.append(3)
# Popping elements from the stack
top_element = stack.pop() # Returns 3
7. What is a queue, and how is it implemented in Python?
Answer: A queue is a linear data structure that follows the First-In-First-Out (FIFO) principle, meaning the first element added is the first one to be removed. This structure is analogous to a real-life queue, such as a line of people waiting their turn.
Implementation in Python:
Python provides multiple ways to implement a queue:
- Using
collections.deque
: Thedeque
(double-ended queue) from thecollections
module allows efficient appends and pops from both ends, making it suitable for implementing queues. Example:
from collections import deque
# Initialize an empty deque
queue = deque()
# Enqueue elements
queue.append('A')
queue.append('B')
queue.append('C')
# Dequeue elements
first_element = queue.popleft() # Returns 'A'
- Using
queue.Queue
: Thequeue
module provides aQueue
class that is especially useful in multi-threaded environments, as it is synchronized and provides thread-safe operations. Example:
from queue import Queue
# Initialize an empty queue
q = Queue()
# Enqueue elements
q.put('A')
q.put('B')
q.put('C')
# Dequeue elements
first_element = q.get() # Returns 'A'
8. Explain the concept of recursion and provide an example in Python.
Answer: Recursion is a programming technique where a function calls itself to solve smaller instances of the same problem. It is particularly useful for problems that can be broken down into simpler, repetitive tasks.
Example: Calculating the factorial of a number:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
# Example usage:
result = factorial(5) # Returns 120
In this example, the factorial
function calls itself with a decremented value of n
until it reaches the base case (n == 0
).
9. What is the difference between a process and a thread?
Answer:
- Process: A process is an independent program in execution, with its own memory space. Processes are isolated from each other, and communication between them requires inter-process communication mechanisms.
- Thread: A thread is a smaller unit of a process that can be scheduled for execution. Threads within the same process share the same memory space and resources, allowing for efficient communication but also requiring careful synchronization to avoid conflicts.
10. How does garbage collection work in Python?
Answer: Python uses automatic garbage collection to manage memory. It primarily employs reference counting and a cyclic garbage collector to reclaim memory occupied by objects that are no longer in use.
- Reference Counting: Each object maintains a count of references pointing to it. When this count drops to zero, the object’s memory is deallocated.
- Cyclic Garbage Collector: To handle reference cycles (where objects reference each other, preventing their reference counts from reaching zero), Python’s cyclic garbage collector periodically identifies and collects groups of objects that are unreachable from any active references.
11. What is a lambda function in Python?
Answer: A lambda function is an anonymous, inline function defined using the lambda
keyword. It can have any number of input parameters but only a single expression. Lambda functions are often used for short, throwaway functions or as arguments to higher-order functions.
Example:
# Regular function
def add(x, y):
return x + y
# Equivalent lambda function
add_lambda = lambda x, y: x + y
# Usage
result = add_lambda(3, 5) # Returns 8
12. Explain the concept of exception handling in Python.
Answer: Exception handling in Python is a mechanism to handle runtime errors gracefully, allowing the program to continue execution or terminate cleanly.
try
block: Contains code that might raise an exception.except
block: Catches and handles the exception if it occurs.else
block: Executed if no exception occurs in thetry
block.finally
block: Executed regardless of whether an exception occurred or was handled, typically used for cleanup actions.
Example:
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero.")
else:
print("Division successful.")
finally:
print("Execution completed.")
13. What is the purpose of the self
parameter in Python class methods?
Answer: In Python, the self
parameter refers to the instance of the class on which a method is called. It allows access to the instance’s attributes and other methods. By convention, self
is the first parameter of instance methods.
Example:
class Person:
def __init__(self, name):
self.name = name
def greet(self):
print(f"Hello, my name is {self.name}.")
# Usage
p = Person("Alice")
p.greet() # Outputs: Hello, my name is Alice.
14. How do you implement inheritance in Python?
Answer: Inheritance in Python allows a class (child class) to inherit attributes and methods from another class (parent class), promoting code reuse and organization.
Example:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
return f"{self.name} says Woof!"
# Usage
dog = Dog("Buddy")
print(dog.speak()) # Outputs: Buddy says Woof!
In this example, Dog
inherits from Animal
and overrides the speak
method.
15. What is a decorator in Python, and how is it used?
Answer: A decorator in Python is a function that takes another function as an argument, extends its behavior without explicitly modifying it, and returns a new function. Decorators are commonly used to add functionality such as logging, access control, or performance measurement to existing functions in a clean and maintainable way.
Usage of Decorators:
Decorators are applied to functions using the @decorator_name
syntax above the function definition. This is syntactic sugar for passing the function to the decorator and reassigning it.
Example:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
Output:
Something is happening before the function is called.
Hello!
Something is happening after the function is called.
In this example:
my_decorator
is a decorator function that takesfunc
as an argument.- Within
my_decorator
, thewrapper
function is defined to add behavior before and after callingfunc
. - The
wrapper
function is returned and replaces the originalsay_hello
function. - When
say_hello()
is called, it actually calls thewrapper
function, which adds the additional behavior.
Decorators with Arguments:
To create decorators that accept arguments, you need to define a function that returns a decorator.
Example:
def repeat(num_times):
def decorator_repeat(func):
def wrapper(*args, **kwargs):
for _ in range(num_times):
func(*args, **kwargs)
return wrapper
return decorator_repeat
@repeat(num_times=3)
def greet(name):
print(f"Hello, {name}!")
greet("Alice")
Output:
Hello, Alice!
Hello, Alice!
Hello, Alice!
In this example:
repeat
is a decorator factory that takesnum_times
as an argument and returns a decorator.decorator_repeat
is the actual decorator that takesfunc
as an argument.wrapper
is the inner function that executesfunc
num_times
times.- The
@repeat(num_times=3)
syntax applies the decorator to thegreet
function, causing it to execute three times when called.
Decorators are a powerful feature in Python that allow for the extension and modification of function behavior in a clean and readable manner.
16. What is the difference between manual testing and automated testing?
Answer: Manual testing involves human testers executing test cases without the assistance of tools or scripts. Testers manually operate the application, observe outcomes, and compare them with expected results. This approach is flexible and allows for exploratory testing but can be time-consuming and prone to human error.
Automated testing, on the other hand, utilizes specialized tools and scripts to execute test cases. Once developed, these tests can run unattended, providing quick feedback and consistent results. Automated testing is efficient for repetitive tasks and regression testing but requires an initial investment in scripting and tool setup.
17. Explain the Software Development Life Cycle (SDLC) and its phases.
Answer: The Software Development Life Cycle (SDLC) is a structured process used for developing software applications. It consists of several phases:
- Requirement Analysis: Gathering and analyzing business requirements to understand what the software should achieve.
- Design: Creating architectural and detailed designs based on the requirements.
- Implementation (Coding): Translating designs into executable code.
- Testing: Verifying that the software functions as intended and identifying defects.
- Deployment: Releasing the software to the production environment.
- Maintenance: Providing ongoing support, fixing issues, and implementing enhancements.
Each phase has specific deliverables and serves as input for the subsequent phase, ensuring a systematic approach to software development.
18. What is the purpose of version control systems, and how do they work?
Answer: Version control systems (VCS) are tools that help manage changes to source code over time. They enable multiple developers to collaborate on a project, track revisions, and revert to previous versions if necessary.
VCS work by maintaining a repository that stores the complete history of changes. Developers can check out copies of the code, make modifications, and commit changes back to the repository. Popular VCS include Git, Subversion, and Mercurial.
19. Describe the Agile methodology and its key principles.
Answer: Agile is a software development methodology that emphasizes iterative development, collaboration, and flexibility. Key principles include:
- Customer Collaboration: Engaging customers throughout the development process to gather feedback and adjust requirements.
- Iterative Development: Delivering software in small, incremental releases, allowing for frequent reassessment and adaptation.
- Cross-Functional Teams: Assembling teams with diverse skills to foster collaboration and shared responsibility.
- Responding to Change: Embracing changes in requirements, even late in development, to provide a competitive advantage.
Agile methodologies, such as Scrum and Kanban, implement these principles to enhance software development processes.
20. What is a RESTful API, and how does it differ from SOAP?
Answer: A RESTful API (Representational State Transfer) is an architectural style for designing networked applications. It uses standard HTTP methods (GET, POST, PUT, DELETE) and is stateless, meaning each request contains all the information needed to process it.
SOAP (Simple Object Access Protocol) is a protocol for exchanging structured information in web services. It relies on XML messaging and has built-in error handling and security features.
Differences:
- Protocol: REST is an architectural style; SOAP is a protocol.
- Data Format: REST can use various formats (JSON, XML); SOAP uses XML exclusively.
- Complexity: REST is simpler and more flexible; SOAP is more rigid but offers built-in standards for security and transactions.
21. Explain the concept of continuous integration and continuous deployment (CI/CD).
Answer: CI/CD is a set of practices in software development aimed at delivering code changes more frequently and reliably.
- Continuous Integration (CI): Developers frequently integrate code into a shared repository, triggering automated builds and tests to detect issues early.
- Continuous Deployment (CD): Code changes that pass automated tests are automatically deployed to production, ensuring rapid delivery of new features and fixes.
CI/CD pipelines automate the process of building, testing, and deploying code, enhancing efficiency and reducing the risk of errors.
22. What is the difference between black-box testing and white-box testing?
Answer:
- Black-Box Testing: Focuses on testing the software’s functionality without knowledge of its internal code structure. Testers validate inputs and outputs against specifications.
- White-Box Testing: Involves testing the internal structures or workings of an application. Testers have access to the source code and design tests based on code logic, paths, and conditions.
Both approaches are essential for comprehensive software testing, addressing different aspects of quality assurance.
23. Describe the Model-View-Controller (MVC) architecture.
Answer: MVC is a design pattern that separates an application into three interconnected components:
- Model: Manages the application’s data and business logic.
- View: Displays the data (the user interface).
- Controller: Handles user input and interacts with the Model to update the View.
This separation facilitates modular development, easier maintenance, and scalability.
24. What is the purpose of unit testing, and how is it performed?
Answer: Unit testing involves testing individual components or functions of a software application to ensure they Unit testing is a software testing method where individual components of the software are tested independently to verify each part functions correctly. It’s a fundamental practice in software development, aimed at ensuring code quality and reliability by isolating each unit and validating its performance.
Purpose of Unit Testing:
- Early Detection of Defects: By testing individual units, developers can identify and fix bugs at an early stage, reducing the cost and effort required to address issues later in the development cycle.
- Code Quality Assurance: Unit tests ensure that each component behaves as expected, leading to more reliable and maintainable code.
- Facilitates Refactoring: With a comprehensive suite of unit tests, developers can confidently refactor code, knowing that existing functionality is safeguarded.
Steps to Perform Unit Testing:
- Identify Units: Determine the smallest testable parts of the application, such as functions, methods, or classes.
- Write Test Cases: Develop test cases for each unit, covering various input scenarios, including edge cases and potential error conditions.
- Execute Tests: Run the tests using a unit testing framework (e.g., JUnit for Java, pytest for Python).
- Analyze Results: Verify that the unit produces the expected output for each test case. If discrepancies are found, investigate and resolve the underlying issues.
By systematically applying unit testing, developers can ensure that individual components function correctly before integration, leading to a more robust and error-free software application.
25. What is the difference between functional and non-functional testing?
Answer: Functional testing evaluates the software’s compliance with specified requirements by verifying that each function operates in conformance with the requirement specification. It focuses on user interactions and the system’s behavior under specific conditions. Examples include unit testing, integration testing, system testing, and acceptance testing.
Non-functional testing assesses aspects not related to specific behaviors or functions of the system. It examines attributes such as performance, usability, reliability, and security. Examples include load testing, stress testing, and security testing.
Therefore, functional testing ensures the system performs its intended functions correctly, while non-functional testing ensures the system’s performance and quality attributes meet the desired standards.
26. Explain the concept of polymorphism in object-oriented programming.
Answer: Polymorphism is a fundamental concept in object-oriented programming that allows objects of different classes to be treated as objects of a common superclass. It enables a single interface to represent different underlying forms (data types). Polymorphism is achieved through:
- Method Overloading: Defining multiple methods with the same name but different parameters within the same class.
- Method Overriding: Providing a specific implementation of a method in a subclass that already exists in its superclass.
Polymorphism enhances flexibility and maintainability by allowing the same method to operate on objects of different classes, enabling code reusability and the ability to introduce new classes with minimal changes to existing code.
27. What is a deadlock in operating systems, and how can it be prevented?
Answer: A deadlock is a situation in operating systems where a set of processes becomes permanently blocked because each process is waiting for a resource that another process holds. The four necessary conditions for a deadlock are:
- Mutual Exclusion: At least one resource is held in a non-shareable mode.
- Hold and Wait: Processes holding resources can request additional resources.
- No Preemption: Resources cannot be forcibly taken from a process holding them.
- Circular Wait: A closed chain of processes exists, where each process holds a resource needed by the next.
Deadlock prevention strategies include:
- Resource Allocation Policies: Imposing restrictions to ensure at least one of the necessary conditions cannot hold.
- Deadlock Avoidance: Using algorithms like Banker’s Algorithm to allocate resources safely.
- Deadlock Detection and Recovery: Allowing deadlocks to occur, detecting them, and then recovering by terminating or rolling back processes.
Implementing these strategies helps maintain system stability and resource availability.
28. Describe the difference between synchronous and asynchronous communication.
Answer: In synchronous communication, the sender and receiver operate in a coordinated manner, with the sender waiting for the receiver to process the message before continuing. This approach ensures immediate feedback but can lead to inefficiencies if either party is delayed.
Asynchronous communication allows the sender to transmit a message without waiting for an immediate response from the receiver. The receiver processes the message at its convenience. This method enhances efficiency and scalability, especially in distributed systems, by decoupling the sender and receiver’s operations.
29. What is the purpose of middleware in software architecture?
Answer: Middleware is software that provides common services and capabilities to applications beyond what’s offered by the operating system. It acts as a bridge between different applications, services, or components, facilitating communication and data management. Middleware supports functions like messaging, authentication, API management, and database connectivity, enabling developers to focus on core application logic without dealing with underlying complexities.
30. Explain the concept of normalization in database design.
Answer: Normalization is a systematic approach to organizing data in a database to reduce redundancy and improve data integrity. It involves decomposing tables into smaller, related tables and defining relationships between them. The process follows a series of normal forms, each with specific rules:
- First Normal Form (1NF): Ensures that the table has a primary key and that all columns contain atomic, indivisible values.
- Second Normal Form (2NF): Achieved when the table is in 1NF, and all non-key attributes are fully functionally dependent on the primary key.
- Third Normal Form (3NF): Achieved when the table is in 2NF, and all attributes are functionally dependent only on the primary key, eliminating transitive dependencies.
Normalization enhances data consistency, reduces anomalies, and optimizes storage efficiency.
31. What is the difference between a primary key and a foreign key in a database?
Answer:
- A primary key is a unique identifier for each record in a database table. It ensures that no two rows have the same value in the primary key column(s) and that the value is not null.
- A foreign key is a field (or collection of fields) in one table that uniquely identifies a row in another table. It establishes a link between the data in the two tables, enforcing referential integrity by ensuring that the value in the foreign key column corresponds to a valid primary key value in the referenced table.
Therefore, a primary key uniquely identifies records within its own table, while a foreign key establishes a relationship between records in different tables.
32. Describe the concept of multithreading and its benefits.
Answer: Multithreading is a programming technique that allows multiple threads to exist within a single process, enabling concurrent execution of tasks. Each thread operates independently but shares the process’s resources, such as memory and file handles.
Benefits of Multithreading:
- Improved Performance: By performing multiple operations concurrently, multithreading can enhance the responsiveness and throughput of applications, especially on multi-core processors.
- Resource Sharing: Threads within the same process can share resources, leading to efficient memory usage.
- Simplified Modeling: Complex applications can be divided into smaller, manageable threads, simplifying design and maintenance.
However, multithreading introduces challenges like synchronization, deadlocks, and race conditions, requiring careful management to ensure thread safety.
Learn More: Carrer Guidance
Deloitte NLA Interview Questions and Answers
ADF Interview Questions Scenario based Questions with detailed Answers
Generative AI System Design Interview Questions and Answers- Basic to Advanced
Business Development Executive Interview Questions and Answers
ServiceNow Interview Questions and Answers for Experienced
ServiceNow Interview Questions and Answers-Freshers