For developers with five years of experience, preparing for an Angular interview requires knowledge of both fundamental and advanced concepts of the framework. Angular has evolved significantly since its inception, and understanding its core principles, lifecycle hooks, dependency injection, and error management is crucial. This guide compiles over 35 top Angular interview questions for 5 years experienced along with detailed answers.
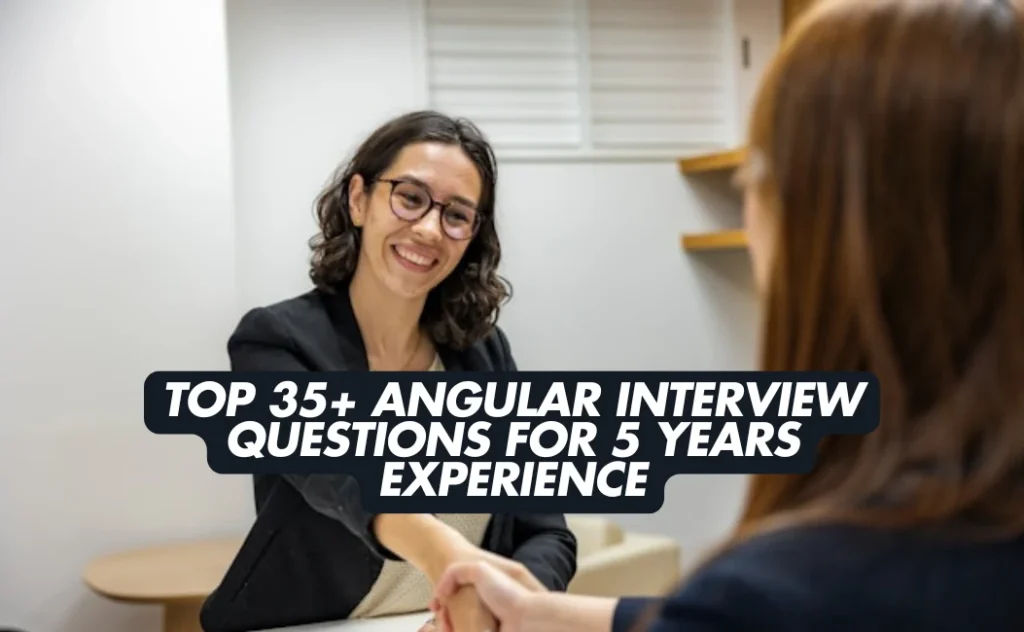
Top 35+ Angular Interview Questions for 5 Years Experience with Answers
- What is Angular and how does it differ from AngularJS?
- Can you explain the component lifecycle hooks in Angular?
- How does Angular’s dependency injection system work?
- What are Angular modules, and why are they important?
- Can you differentiate between template-driven and reactive forms in Angular?
- How does Angular handle error management in HTTP requests?
- What is the purpose of Angular’s RouterModule, and how does it function?
- How do you implement two-way data binding in Angular?
- What are Angular directives, and what are their types?
- How does Angular’s change detection mechanism work?
- What is Angular Universal, and what are its benefits?
- How do you implement lazy loading in Angular?
- What are Angular services, and how are they used?
- How does Angular handle forms, and what are the differences between template-driven and reactive forms?
- What is NgZone, and how does it relate to change detection?
- How do you optimize the performance of an Angular application?
- What are Angular pipes, and how do you create a custom pipe?
- What is the difference between ngOnInit() and a constructor in Angular components?
- How does Angular handle routing, and what are route guards?
- What is the purpose of Angular’s HttpInterceptor, and how do you implement one?
- What is the Angular Compiler, and what are the differences between JIT and AOT compilation?
- How does Angular’s change detection strategy work, and what are the differences between Default and OnPush strategies?
- What are Angular decorators, and how are they used?
- How do you implement internationalization (i18n) in Angular applications?
- What is NgRx, and how does it facilitate state management in Angular applications?
- How does Angular handle dependency injection, and what are the different provider scopes?
- What are Angular zones, and how do they assist with asynchronous operations?
- How do you implement route animations in Angular?
- What is the purpose of Angular’s Renderer2 service, and when should it be used?
- How do you manage state in Angular applications without NgRx?
- What are Angular elements, and how can they be used to integrate Angular components into non-Angular applications?
- How does Angular’s HttpClient handle error responses, and how can you implement global error handling for HTTP requests?
- What are Angular lifecycle hooks, and how do they differ from each other?
- How does Angular’s Renderer2 differ from direct DOM manipulation, and why is it recommended?
- What is the role of Angular’s Injector, and how does it facilitate dependency injection?
- How can you optimize an Angular application for better performance?
1. What is Angular and how does it differ from AngularJS?
Angular is a TypeScript-based open-source front-end web application framework developed by Google. It is a complete rewrite of AngularJS, introducing features like a component-based architecture, improved performance with Ahead-of-Time (AOT) compilation, and enhanced dependency injection. Unlike AngularJS, which uses JavaScript and follows the Model-View-Controller (MVC) architecture, Angular leverages TypeScript and adopts a component-based structure, leading to better modularity and maintainability.
2. Can you explain the component lifecycle hooks in Angular?
Angular provides several lifecycle hooks that allow developers to tap into key events in a component’s lifecycle:
- ngOnChanges: Called when an input-bound property changes.
- ngOnInit: Invoked once after the first ngOnChanges.
- ngDoCheck: Detects and acts upon changes that Angular can’t or won’t detect on its own.
- ngAfterContentInit: Called after content projection into the component.
- ngAfterContentChecked: Called after every check of projected content.
- ngAfterViewInit: Invoked after the component’s view and child views are initialized.
- ngAfterViewChecked: Called after every check of the component’s views and child views.
- ngOnDestroy: Cleans up just before Angular destroys the component.
These hooks provide insights into the component’s initialization, change detection, and destruction phases, enabling developers to manage resources and application behavior effectively.
3. How does Angular’s dependency injection system work?
Angular’s dependency injection (DI) is a design pattern that allows a class to receive its dependencies from external sources rather than creating them itself. This promotes modularity and testability. In Angular, services or objects are injected into components or other services using constructors. The Angular injector is responsible for creating service instances and injecting them as needed. Providers define how to create instances of dependencies. By specifying providers in modules or components, Angular knows how to inject the necessary services.
4. What are Angular modules, and why are they important?
Angular modules, defined using the NgModule decorator, are containers that group related components, directives, pipes, and services. They help organize an application into cohesive blocks of functionality. Modules can import other modules, allowing for a modular architecture. The root module, often called AppModule, bootstraps the application. Feature modules can encapsulate specific functionalities, enabling lazy loading and better separation of concerns.
5. Can you differentiate between template-driven and reactive forms in Angular?
Angular provides two approaches for handling user input through forms:
- Template-Driven Forms: These rely on directives in the template to create and manage forms. They are suitable for simple forms and use two-way data binding with [(ngModel)]. Validation is declared in the template using attributes.
- Reactive Forms: Also known as model-driven forms, they are built programmatically in the component class using FormControl and FormGroup. They provide more control and are suitable for complex forms. Validation is defined in the component class, offering more flexibility and predictability.
Reactive forms are generally preferred for larger applications due to their scalability and robustness.
6. How does Angular handle error management in HTTP requests?
Angular’s HttpClient module provides mechanisms to handle errors in HTTP operations. By using RxJS operators like catchError, developers can intercept failed HTTP responses and handle them appropriately. For example:
import { HttpClient, HttpErrorResponse } from '@angular/common/http';
import { catchError } from 'rxjs/operators';
import { throwError } from 'rxjs';
constructor(private http: HttpClient) {}
getData() {
return this.http.get('api/data').pipe(
catchError(this.handleError)
);
}
private handleError(error: HttpErrorResponse) {
// Handle the error based on error status or message
return throwError('An error occurred');
}
This approach ensures that the application can gracefully handle errors, providing feedback to users and maintaining stability.
7. What is the purpose of Angular’s RouterModule, and how does it function?
The RouterModule is a core part of Angular’s routing mechanism. It enables navigation between different views or components within a single-page application. By defining routes, developers can map URL paths to components. The RouterModule provides directives like routerLink and router-outlet to facilitate navigation and view rendering. Lazy loading can be implemented by configuring child routes, which helps in loading modules only when needed, improving application performance.
8. How do you implement two-way data binding in Angular?
Two-way data binding in Angular is achieved using the ngModel directive combined with the [( )] syntax, known as banana-in-a-box. This allows synchronization of data between the component class and the template. For example:
<input [(ngModel)]="userName">
<p>{{ userName }}</p>
In this example, any changes to the input field update the userName property in the component, and any changes to userName in the component reflect in the input field.
9. What are Angular directives, and what are their types?
Angular directives are classes that add behavior to elements in your Angular applications. They allow you to attach specific behavior to the DOM. There are three main types of directives in Angular:
- Components: These are directives with a template. They are the most common directive type and are defined using the
@Component
decorator. Components control a patch of screen called a view. - Structural Directives: These directives change the DOM layout by adding or removing elements. They are denoted with an asterisk (
*
) before the directive name. Common examples include*ngIf
and*ngFor
. - Attribute Directives: These directives change the appearance or behavior of an element, component, or another directive. They are applied as attributes to elements. Examples include
ngClass
andngStyle
.
Understanding these directive types is crucial for effectively manipulating the DOM and enhancing the user interface in Angular applications.
10. How does Angular’s change detection mechanism work?
Angular’s change detection mechanism is responsible for synchronizing the model and the view by tracking changes and updating the DOM accordingly. It uses a unidirectional data flow and a tree of components to detect changes. When an event occurs, such as user input or an HTTP request, Angular runs change detection to check all components for changes. It compares the current and previous values of component properties and updates the view if differences are found. This process ensures that the user interface remains consistent with the underlying data model.
11. What is Angular Universal, and what are its benefits?
Angular Universal is a technology that enables server-side rendering (SSR) of Angular applications. It allows Angular applications to be rendered on the server, generating static application pages that are later bootstrapped on the client. The benefits of Angular Universal include improved performance, better search engine optimization (SEO), and faster initial load times. By rendering pages on the server, users can see the content more quickly, and search engines can index the content more effectively.
12. How do you implement lazy loading in Angular?
Lazy loading in Angular is implemented by loading feature modules asynchronously when the user navigates to a specific route. This approach reduces the initial load time of the application. To implement lazy loading, define routes using the loadChildren
property with dynamic imports. For example:
const routes: Routes = [
{
path: 'feature',
loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule)
}
];
This configuration tells Angular to load the FeatureModule
only when the user navigates to the ‘feature’ path, thereby optimizing the application’s performance.
13. What are Angular services, and how are they used?
Angular services are singleton objects that encapsulate reusable business logic, data access, or other functionalities that can be shared across components. They are typically defined as classes and can be injected into components or other services using Angular’s dependency injection system. Services promote code modularity and reusability. To create a service, use the @Injectable
decorator, and then provide it in a module or component. For example:
@Injectable({
providedIn: 'root'
})
export class DataService {
// Service logic
}
This service can then be injected into a component’s constructor:
constructor(private dataService: DataService) {}
This approach allows components to access shared functionalities provided by the service.
14. How does Angular handle forms, and what are the differences between template-driven and reactive forms?
Angular provides two approaches to handling forms: template-driven and reactive forms.
- Template-Driven Forms: These forms are declared in the template using directives like
ngModel
. They are suitable for simple forms and rely on two-way data binding. Validation is defined using attributes in the template. - Reactive Forms: Also known as model-driven forms, they are defined programmatically in the component class using
FormControl
andFormGroup
. Reactive forms provide more control and are suitable for complex forms. Validation is defined in the component class, offering greater flexibility.
The choice between the two depends on the complexity of the form and the level of control required.
15. What is NgZone, and how does it relate to change detection?
NgZone
is a service in Angular that provides an execution context that persists across asynchronous tasks. It allows Angular to detect changes and update the view when asynchronous operations, such as HTTP requests or timers, complete. By running code inside or outside Angular’s zone, developers can control when change detection is triggered, optimizing performance for certain operations.
16. How do you optimize the performance of an Angular application?
Optimizing an Angular application involves several strategies:
- Lazy Loading: Load feature modules only when needed to reduce initial load time.
- Ahead-of-Time (AOT) Compilation: Compile the application during the build process to improve rendering speed.
- OnPush Change Detection Strategy: Use the
OnPush
strategy to limit change detection checks to specific components. - TrackBy with ngFor: Implement
trackBy
functions withngFor
to optimize rendering of lists. - Code Splitting: Divide the application into smaller bundles to load only necessary code.
- Caching: Utilize browser caching and service workers to cache assets and API responses.
These techniques help in enhancing the application’s responsiveness and load times.
17. What are Angular pipes, and how do you create a custom pipe?
Angular pipes are functions that transform data directly within templates, allowing for easy and declarative data formatting in HTML. To create a custom pipe:
1. Generate the Pipe: Use Angular CLI:
ng generate pipe pipe-name
2. Implement the Pipe Logic: In the generated .ts
file, implement the PipeTransform
interface and define the transform
method. For example, a kebab-case pipe:
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({ name: 'kebabCase' })
export class KebabCasePipe implements PipeTransform {
transform(value: string): string {
return value.toLowerCase().replace(/ /g, '-');
}
}
3. Register the Pipe: Add the pipe to the declarations
array of an Angular module:
@NgModule({
declarations: [KebabCasePipe],
exports: [KebabCasePipe]
})
export class SharedModule {}
4. Use the Pipe in Templates:
<p>{{ 'Hello World' | kebabCase }}</p> <!-- Outputs: hello-world -->
Custom pipes can also accept parameters to modify their behavior by adding arguments to the transform
method and passing them in the template.
18. What is the difference between ngOnInit()
and a constructor in Angular components?
In Angular components, both the constructor and the ngOnInit()
lifecycle hook are used for initialization, but they serve different purposes:
- Constructor: The constructor is a default method of the TypeScript class that is invoked when Angular instantiates the component class. It’s typically used for simple initialization tasks, such as setting up basic variables. However, at this point, the component’s inputs are not yet bound, and the view is not initialized.
- ngOnInit(): This lifecycle hook is called by Angular after the component’s inputs have been bound and the component’s view has been initialized. It’s the appropriate place to perform complex initialization tasks, such as fetching data from a service or setting up the component’s state based on its inputs.
In summary, use the constructor for simple, synchronous initialization, and ngOnInit()
for tasks that depend on Angular’s bindings or require the component’s view to be initialized.
19. How does Angular handle routing, and what are route guards?
Angular’s routing mechanism enables navigation between different views or components within a single-page application. It uses the RouterModule
to define routes, which map URL paths to components.
Route Guards are interfaces that allow developers to control access to routes based on certain conditions. Angular provides several types of route guards:
- CanActivate: Determines if a route can be activated.
- CanActivateChild: Determines if child routes can be activated.
- CanDeactivate: Determines if a route can be deactivated.
- CanLoad: Determines if a module can be loaded asynchronously.
Implementing these guards involves creating a service that implements the respective interface and defining the logic that returns a boolean or an Observable
/Promise
resolving to a boolean. This service is then applied to routes in the routing configuration to protect them based on the specified conditions.
20. What is the purpose of Angular’s HttpInterceptor
, and how do you implement one?
An HttpInterceptor
in Angular is a service that intercepts HTTP requests and responses, allowing developers to modify or handle them before they reach the server or the application code. This is useful for tasks such as adding authentication tokens, logging, error handling, or modifying request headers.
To implement an HttpInterceptor
:
1. Create a Service: Implement a class that implements the HttpInterceptor
interface.
import { Injectable } from '@angular/core';
import { HttpInterceptor, HttpRequest, HttpHandler, HttpEvent } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable()
export class AuthInterceptor implements HttpInterceptor {
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
// Clone the request to add the new header
const authReq = req.clone({
headers: req.headers.set('Authorization', 'Bearer YOUR_TOKEN_HERE')
});
// Pass on the cloned request instead of the original request
return next.handle(authReq);
}
}
2. Provide the Interceptor: Register the interceptor in the application’s module by adding it to the providers
array with the HTTP_INTERCEPTORS
token.
import { HTTP_INTERCEPTORS } from '@angular/common/http';
@NgModule({
// ...
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: AuthInterceptor, multi: true },
],
})
export class AppModule {}
By setting multi: true
, you allow multiple interceptors to be registered. The order in which interceptors are provided determines their execution order.
Implementing HttpInterceptor
provides a powerful way to handle cross-cutting concerns related to HTTP communication in a centralized and reusable manner.
21. What is the Angular Compiler, and what are the differences between JIT and AOT compilation?
The Angular Compiler, known as the Angular Compiler (NGC), translates Angular HTML and TypeScript code into efficient JavaScript code during the build process. Angular supports two types of compilation:
- Just-in-Time (JIT) Compilation: Compiles the application in the browser at runtime. This approach is beneficial during development due to faster build times and the absence of a build step. However, it can lead to slower application startup times and may expose the application to injection attacks.
- Ahead-of-Time (AOT) Compilation: Compiles the application during the build process before serving it to the client. This results in faster rendering, fewer asynchronous requests, smaller Angular framework download sizes, and enhanced security by pre-compiling templates and eliminating the need for the client to compile them.
In production environments, AOT compilation is generally preferred due to its performance and security benefits.
22. How does Angular’s change detection strategy work, and what are the differences between Default and OnPush strategies?
Angular’s change detection mechanism ensures that the view reflects the current state of the model. It does this by checking the components’ data bindings and updating the DOM accordingly. Angular provides two change detection strategies:
- Default: Angular checks every component in the component tree for changes during each change detection cycle, regardless of whether the inputs have changed. This can lead to unnecessary checks and performance overhead in large applications.
- OnPush: Angular only checks the component when its input properties change, an event originates from the component or its children, or when
markForCheck()
is explicitly called. This strategy reduces the number of change detection cycles, leading to improved performance.
Using the OnPush strategy can significantly enhance performance, especially in large applications with complex component trees.
23. What are Angular decorators, and how are they used?
Decorators in Angular are special declarations that attach metadata to classes, methods, properties, or parameters. They are functions that modify the behavior of the target they are applied to. Common Angular decorators include:
- @Component: Defines a component with its selector, template, styles, and other properties.
- @Directive: Defines a directive with its selector and properties.
- @Injectable: Marks a class as available for dependency injection.
- @NgModule: Defines a module with declarations, imports, providers, and bootstrap properties.
Decorators are a fundamental part of Angular’s design, enabling a declarative approach to configuring classes and their behavior.
24. How do you implement internationalization (i18n) in Angular applications?
Internationalization (i18n) in Angular involves designing the application to support multiple languages and locales. Angular provides built-in support for i18n through the following steps:
- Mark Text for Translation: Use the
i18n
attribute in templates to mark text for translation.
<h1 i18n="@@homeTitle">Welcome to our application</h1>
- Extract Translatable Content: Use the Angular CLI to extract the marked text into a translation source file (e.g., XLIFF format).
ng extract-i18n --output-path src/locale
- Provide Translations: Translate the extracted text into the target languages and save them in separate translation files.
- Configure the Angular Compiler: Modify the Angular build configuration to use the appropriate translation file for each locale.
- Build and Deploy: Build the application for each locale and deploy the localized versions.
This process enables Angular applications to cater to a global audience by supporting multiple languages and regional settings.
25. What is NgRx, and how does it facilitate state management in Angular applications?
NgRx is a state management library for Angular applications, implementing the Redux pattern using RxJS observables. It provides a single source of truth for the application state, making it predictable and easier to manage. Key concepts in NgRx include:
- Store: Holds the application state as a single immutable object.
- Actions: Plain objects that describe state changes.
- Reducers: Pure functions that take the current state and an action to return a new state.
- Selectors: Functions that select and derive pieces of the state for use in components.
By centralizing the state and using unidirectional data flow, NgRx helps in building scalable and maintainable Angular applications.
26. How does Angular handle dependency injection, and what are the different provider scopes?
Angular’s dependency injection (DI) system allows for the efficient management of service instances within an application. By defining providers, Angular knows how to create and deliver services to components or other services that declare dependencies. The scope of these providers determines the lifecycle and availability of the service instances:
- Root Scope: When a service is provided in the root injector (using
providedIn: 'root'
), Angular creates a single instance of the service that is shared across the entire application. - Module Scope: Providing a service in a specific feature module limits the service instance to that module and its components. Each lazy-loaded module can have its own instance of the service.
- Component Scope: Providing a service at the component level ensures that each instance of the component gets its own instance of the service. This is achieved by specifying the provider in the component’s
providers
array.
Understanding these scopes is crucial for managing service lifecycles and ensuring that components receive the appropriate instances as needed.
27. What are Angular zones, and how do they assist with asynchronous operations?
Angular utilizes zones, specifically the zone.js
library, to manage and detect asynchronous operations within an application. A zone is an execution context that persists across asynchronous tasks, allowing Angular to be notified when these tasks start and complete. This mechanism enables Angular to automatically trigger change detection after asynchronous operations, ensuring that the view remains in sync with the underlying data model. By leveraging zones, developers can write asynchronous code without manually handling change detection, leading to more predictable and maintainable applications.
28. How do you implement route animations in Angular?
Angular’s @angular/animations
module provides a robust framework for implementing animations, including those triggered by route changes. To set up route animations:
1. Import Necessary Modules: Ensure that the BrowserAnimationsModule
is imported in the application’s main module.
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
@NgModule({
imports: [BrowserAnimationsModule, /* other imports */],
/* other configurations */
})
export class AppModule {}
2. Define Animation States and Transitions: Create animations using Angular’s animation DSL, specifying states and transitions.
import { trigger, transition, style, animate } from '@angular/animations';
export const slideInAnimation = trigger('routeAnimations', [
transition('* <=> *', [
style({ opacity: 0 }),
animate('300ms', style({ opacity: 1 })),
]),
]);
3. Apply Animations to Router Outlet: Bind the defined animation to the router outlet in the template.
<div [@routeAnimations]="o && o.activatedRouteData && o.activatedRouteData['animation']">
<router-outlet #o="outlet"></router-outlet>
</div>
4. Configure Route Data: Assign animation metadata to routes in the routing module.
const routes: Routes = [
{ path: 'home', component: HomeComponent, data: { animation: 'HomePage' } },
{ path: 'about', component: AboutComponent, data: { animation: 'AboutPage' } },
// other routes
];
By following these steps, Angular will animate route transitions based on the defined animations, enhancing the user experience with smooth visual effects.
29. What is the purpose of Angular’s Renderer2
service, and when should it be used?
The Renderer2
service in Angular provides an abstraction for interacting with the DOM, allowing developers to manipulate elements without directly accessing native DOM APIs. This abstraction is particularly beneficial for:
- Cross-Platform Compatibility: Ensuring that code works across different platforms, such as web browsers and server-side rendering environments.
- Security: Mitigating security risks like Cross-Site Scripting (XSS) by sanitizing inputs and outputs.
- Testability: Facilitating easier testing by decoupling DOM manipulations from the component logic.
By using Renderer2
, developers can perform actions such as creating elements, setting attributes, adding classes, and listening to events in a platform-agnostic manner, leading to more robust and maintainable applications.
30. How do you manage state in Angular applications without NgRx?
While NgRx is a popular choice for state management in Angular applications, there are alternative approaches to handle state effectively:
- Service-Based State Management: Utilize Angular services to maintain and share state across components. Services can hold application data and provide methods to update and retrieve this data.
- BehaviorSubject: Leverage
BehaviorSubject
from RxJS to create observable streams of data that components can subscribe to. This allows for reactive state management and ensures that components are updated when the state changes. - Local Storage: For persisting state across sessions, use browser storage mechanisms like
localStorage
orsessionStorage
.
These methods provide flexibility and can be tailored to the specific needs of the application, especially when a full-fledged state management library like NgRx is not required.
31. What are Angular elements, and how can they be used to integrate Angular components into non-Angular applications?
Angular Elements allow developers to package Angular components as custom elements (web components), enabling their use in non-Angular applications or other frameworks.
How to Use Angular Elements:
1. Create an Angular Component:
// my-component.component.ts
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-my-component',
template: `<h1>Hello, {{ name }}!</h1>`
})
export class MyComponent {
@Input() name: string = 'World';
}
2. Transform into a Custom Element:
// app.module.ts
import { NgModule, Injector } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { createCustomElement } from '@angular/elements';
import { MyComponent } from './my-component.component';
@NgModule({
declarations: [MyComponent],
imports: [BrowserModule],
entryComponents: [MyComponent]
})
export class AppModule {
constructor(private injector: Injector) {
const element = createCustomElement(MyComponent, { injector });
customElements.define('my-component', element);
}
ngDoBootstrap() {}
}
3. Include Polyfills (if needed):
npm install @webcomponents/webcomponentsjs --save
4. Build the Project:
ng build --prod --output-hashing=none
5. Integrate into a Non-Angular App:
<!DOCTYPE html>
<html>
<head>
<script src="path-to-polyfills.js"></script>
<script src="path-to-main.js"></script>
</head>
<body>
<my-component name="Angular"></my-component>
</body>
</html>
Benefits of Angular Elements:
- Reusability: Use Angular components across different projects and frameworks.
- Interoperability: Integrate seamlessly with React, Vue, or plain HTML/JavaScript apps.
- Encapsulation: Isolate styles and functionality using the shadow DOM.
- Standards-Based: Leverage standard web components for broad compatibility.
By using Angular Elements, you can extend the functionality of Angular components beyond Angular applications, promoting a more versatile and modular codebase.
32. How does Angular’s HttpClient
handle error responses, and how can you implement global error handling for HTTP requests?
Angular’s HttpClient
module provides a streamlined API for making HTTP requests and includes mechanisms for handling errors. When an HTTP request fails, HttpClient
returns an Observable
that emits an error. To handle these errors globally, you can implement an HttpInterceptor
that intercepts all HTTP requests and responses. Within this interceptor, you can catch errors and perform actions such as logging or displaying user-friendly messages. Here’s an example of implementing global error handling using an interceptor:
import { Injectable } from '@angular/core';
import { HttpInterceptor, HttpRequest, HttpHandler, HttpEvent, HttpErrorResponse } from '@angular/common/http';
import { Observable, throwError } from 'rxjs';
import { catchError } from 'rxjs/operators';
@Injectable()
export class GlobalErrorInterceptor implements HttpInterceptor {
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
return next.handle(req).pipe(
catchError((error: HttpErrorResponse) => {
// Handle the error here
console.error('An error occurred:', error.message);
// Optionally, display a user-friendly message or perform other actions
return throwError(error);
})
);
}
}
To apply this interceptor globally, provide it in your application’s module:
import { HTTP_INTERCEPTORS } from '@angular/common/http';
@NgModule({
// ...
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: GlobalErrorInterceptor, multi: true },
],
})
export class AppModule {}
This setup ensures that all HTTP errors are intercepted and handled consistently across the application.
33. What are Angular lifecycle hooks, and how do they differ from each other?
Angular lifecycle hooks are methods that allow developers to tap into key events during a component’s lifecycle, from its creation to its destruction. Each hook serves a specific purpose:
ngOnChanges
: Called when an input property value changes.ngOnInit
: Invoked once after the firstngOnChanges
. Ideal for component initialization.ngDoCheck
: Runs during every change detection cycle. Use for custom change detection.ngAfterContentInit
: Called after content projection (<ng-content>
) is initialized.ngAfterContentChecked
: Runs after every check of projected content.ngAfterViewInit
: Invoked after the component’s view and child views are initialized.ngAfterViewChecked
: Runs after every check of the component’s view and child views.ngOnDestroy
: Called just before the component is destroyed. Use for cleanup tasks.
Implementing these hooks allows developers to manage component behavior throughout its lifecycle effectively.
34. How does Angular’s Renderer2
differ from direct DOM manipulation, and why is it recommended?
Renderer2
is Angular’s abstraction for DOM manipulation, providing a platform-agnostic way to interact with the DOM. Unlike direct DOM manipulation, Renderer2
ensures that operations are compatible across different environments, such as server-side rendering with Angular Universal. It also enhances security by preventing vulnerabilities like Cross-Site Scripting (XSS). Using Renderer2
is recommended because it:
- Ensures compatibility across various platforms.
- Enhances security by sanitizing inputs.
- Improves testability by decoupling DOM access from component logic.
By leveraging Renderer2
, developers can create more robust and secure applications.
35. What is the role of Angular’s Injector
, and how does it facilitate dependency injection?
The Injector
is a core part of Angular’s dependency injection system. It maintains a container of service instances and provides them upon request. When a component or service declares a dependency, the injector supplies the required instance, promoting loose coupling and enhancing testability. The injector resolves dependencies hierarchically, allowing for scoped service instances and efficient resource management.
36. How can you optimize an Angular application for better performance?
Optimizing an Angular application involves several strategies:
- Lazy Loading: Load modules only when needed to reduce initial load time.
- Ahead-of-Time (AOT) Compilation: Compile templates during the build process to improve rendering speed.
- OnPush Change Detection: Use the
OnPush
strategy to minimize change detection cycles. - TrackBy with
ngFor
: ImplementtrackBy
functions to optimize list rendering. - Code Splitting: Divide the application into smaller bundles for efficient loading.
- Caching: Utilize browser caching and service workers to cache assets and API responses.
Implementing these techniques enhances the application’s responsiveness and overall performance.
Learn More: Carrer Guidance | Hiring Now!
Top 35+ Android Interview Questions for Senior Developer with Detailed Answers
SQL Query Interview Questions for Freshers with Answers
GCP (Google Cloud Platform) Interview Questions and Answers
Selenium Coding Interview Questions and Answers
Power Automate Interview Questions for Freshers with Answers
Mobile Testing Interview Questions and Answers- Basic to Advanced
JMeter Interview Questions and Answers