Encountering “npm ERR Could not determine executable to run” error can be frustrating, especially when working on a Node.js project. This error often arises when npm cannot locate the required executable to run a specific script or command. Several factors can trigger this issue, including missing dependencies, misconfigurations, or outdated packages.
npm ERR! Could not determine executable to run
Common Causes of Error ‘npm ERR Could Not Determine Executable to Run‘
Before jumping into fixes, it’s essential to understand what causes this error:
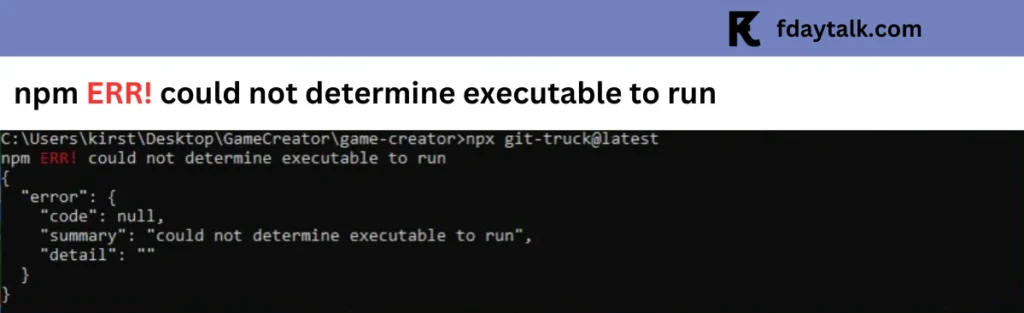
- Missing Dependencies: If npm dependencies are not installed properly, certain executables may not be available.
- Corrupt or Incomplete Package Installation: Sometimes, an interrupted installation or package corruption can lead to this issue.
- Incorrect Command Syntax: Running incorrect npm or npx commands can trigger this error.
- Version Conflicts: Using an outdated or incompatible Node.js and npm version may cause executable issues.
- Environmental Path Issues: If npm’s global bin directory is not in the system’s PATH variable, npm may struggle to find executables.
10 Fixes to Resolve “Could Not Determine Executable to Run” Error
Now that we understand the possible causes, let’s go through the best ways to fix the issue.
1. Run npm install
to Install Missing Dependencies
Often, this error occurs because required dependencies are missing. Running the following command ensures all dependencies are installed:
npm install
This will restore all packages listed in your package.json
file and might resolve missing executables.
2. Check for Missing or Outdated Packages
If you’re using an outdated or missing package, you can check and update the necessary packages:
npm outdated
npm update
For a specific package:
npm install <package-name>@latest
Ensure you’re using the latest stable version of npm itself:
npm install -g npm
3. Verify Your Command Syntax
Using an incorrect command can lead to this error. Ensure you’re using the right command:
Correct:
npm start
Incorrect:
npx start
For running scripts, use:
npm run <script-name>
4. Clear npm Cache
If a corrupt package is causing the issue, clearing the npm cache can help:
npm cache clean --force
Then reinstall dependencies:
rm -rf node_modules package-lock.json
npm install
5. Reinstall npm and Node.js
If clearing the cache doesn’t work, reinstall npm and Node.js:
- Uninstall npm globally:
npm uninstall -g npm
- Download and reinstall the latest Node.js version from Node.js Official Site.
6. Remove Corrupt or Unnecessary Git Hooks
Sometimes, corrupted Git hooks can cause this error. Remove them by running:
rm -rf .git/hooks
npm install
7. Check Environment Variables
If npm cannot find the correct executable, you might need to add its global bin directory to your PATH variable:
export PATH=~/.npm-global/bin:$PATH
For Windows users:
- Open Environment Variables.
- Add
C:\Users\<YourUser>\AppData\Roaming\npm
to the PATH variable.
8. Force a Repair of npm
If the error persists, try forcing an npm repair:
npm doctor
npm audit fix --force
9. Check If a Specific Package is Causing Issues
Some package versions might not be compatible. Try reinstalling the affected package:
npm uninstall <package-name>
npm install <package-name>
If a package (e.g., Tailwind CSS) is giving issues due to recent updates, refer to its official documentation for updated installation steps.
10. Consider Using Yarn Instead of npm
If npm continues to cause issues, switching to Yarn might be an alternative:
npm install -g yarn
yarn install
This can sometimes resolve dependency issues.
Conclusion
The “npm ERR Could not determine executable to run” error in npm is mainly caused by missing dependencies, incorrect command syntax, outdated packages, or environmental issues. By following the fixes outlined in this guide, you should be able to troubleshoot and resolve the issue efficiently.
Let us know in the comments if any of these solutions worked for you!