Are you preparing for Golang interview? Golang, or simply Go, has rapidly become one of the most sought-after programming languages for building scalable and high-performance applications. Developed by Google, it combines the simplicity of Python with the efficiency of C, making it an ideal choice for developers working on cloud services, networking tools, and distributed systems. This article provides 40 essential Golang interview questions, covering basic to advanced concepts such as concurrency, error handling, and memory management.
Top 40 Golang Interview Questions and Answers
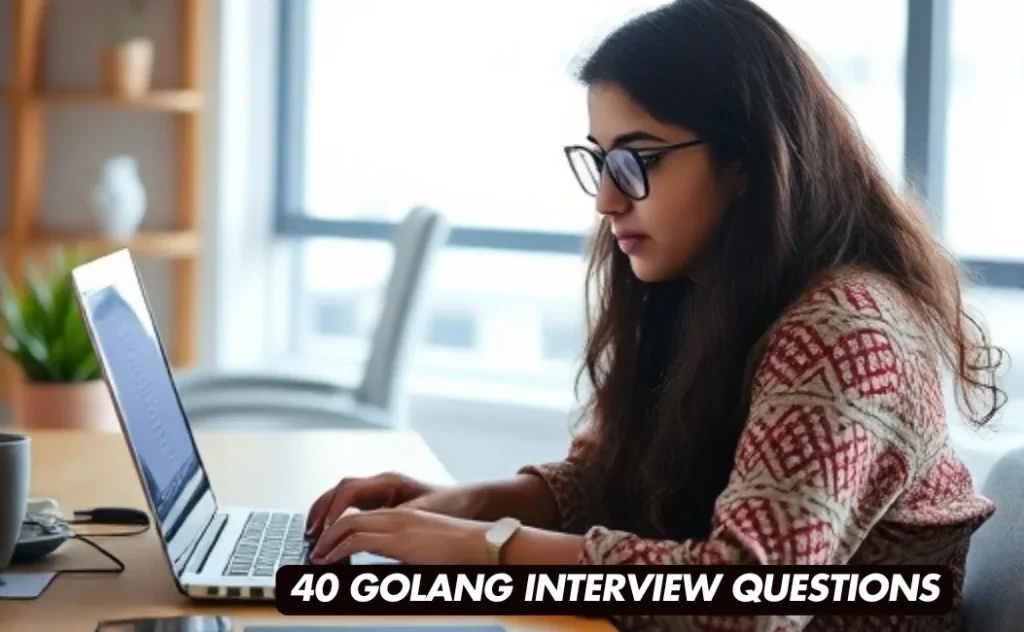
Beginner Level:
- What is Golang, and why is it used?
- How do you declare variables in Go?
- What are the basic data types in Go?
- What is a package in Go, and why is it important?
- How does Go handle strings? What are string literals?
- What are slices in Go, and how do they differ from arrays?
- How does Go implement error handling?
- What is the purpose of the
defer
statement in Go? - How do you create and use maps in Go?
- What is the significance of the
main
package in Go? - How does Go support concurrency at the language level?
- What are interfaces in Go, and how are they implemented?
- What is a pointer in Go, and how is it used?
- How does Go handle type conversion?
- What is a goroutine, and how do you stop it?
Intermediate Level:
- What is the difference between
var
and:=
in Go? - How does Go manage memory?
- What are channels in Go, and how are they used?
- How does Go handle variable scope?
- What is the role of the
init()
function in Go? - How do you handle JSON data in Go?
- What is the Go workspace, and how is it structured?
- How does Go’s garbage collector work?
- What are the different ways to concatenate strings in Go?
- How do you perform unit testing in Go?
- What is a struct in Go, and how do you define one?
- How do you handle time and date operations in Go?
- What is the purpose of the
select
statement in Go? - How do you implement inheritance in Go?
- What are the common tools used for dependency management in Go?
Advanced Level:
- How does Go’s scheduler manage goroutines?
- What are the best practices for error handling in Go?
- How do you implement reflection in Go?
- What is the
unsafe
package in Go, and when would you use it? - How do you optimize Go code for performance?
- What are the implications of Go’s pass-by-value system?
- How do you implement a worker pool in Go?
- What is the purpose of build tags in Go?
- How do you manage configuration in Go applications?
- What are the considerations for handling large datasets in Go?
1. What is Golang, and why is it used?
Golang (or Go) is an open-source programming language developed by Google. It is designed for simplicity, efficiency, and performance. Go is known for its fast execution, static typing, and built-in support for concurrency, making it an excellent choice for cloud services, distributed systems, and command-line tools.
2. How do you declare variables in Go?
Variables in Go can be declared using the var
keyword or shorthand notation :=
for implicit type inference.
- Example (Explicit Declaration):
var x int = 10
- Example (Implicit Declaration):
y := 20
3. What are the basic data types in Go?
Go provides several fundamental data types:
- Integer types: int, int8, int16, int32, int64
- Floating-point types: float32, float64
- Boolean: bool
- String: string
- Unsigned integers: uint, uint8, uint16, uint32, uint64
- Complex numbers: complex64, complex128
- Byte and rune: byte (alias for uint8), rune (alias for int32)
4. What is a package in Go, and why is it important?
A package in Go is a way to organize code into reusable modules. Each Go file belongs to a package, and the main
package is special because it defines the entry point for executable programs. Packages help in code modularity and reusability.
- Example:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
5. How does Go handle strings? What are string literals?
Strings in Go are immutable sequences of bytes, representing Unicode text. String literals are enclosed in double quotes (""
) for plain strings or backticks (`
) for raw strings, preserving newlines and escape sequences.
- Example:
str := "Hello"
rawStr := `This is
a raw string`
6. What are slices in Go, and how do they differ from arrays?
Slices in Go are dynamic, resizable arrays that provide more flexibility than fixed-size arrays. Slices are built on top of arrays and reference a portion of an array.
- Example:
arr := [5]int{1, 2, 3, 4, 5}
slice := arr[1:4] // slice[2, 3, 4]
7. How does Go implement error handling?
Go uses a simple, explicit error handling mechanism. Functions often return an error
type as the last return value.
- Example:
result, err := divide(4, 0)
if err != nil {
fmt.Println("Error:", err)
}
8. What is the purpose of the defer
statement in Go?
The defer
statement postpones the execution of a function until the surrounding function returns. It is commonly used for cleanup tasks such as closing files or releasing resources.
Example:
func main() {
defer fmt.Println("World")
fmt.Println("Hello")
}
Output:
Hello
World
9. How do you create and use maps in Go?
Maps in Go are key-value data structures.
- Example:
m := make(map[string]int)
m["apple"] = 5
fmt.Println(m["apple"])
10. What is the significance of the main
package in Go?
The main
package is required to create an executable program in Go. The main
function within this package serves as the entry point for program execution.
11. How does Go support concurrency at the language level?
Go provides built-in support for concurrency through goroutines, which are lightweight threads managed by the Go runtime. Channels facilitate communication between goroutines.
- Example:
go myFunction()
12. What are interfaces in Go, and how are they implemented?
Interfaces in Go define a set of method signatures. A type implements an interface by implementing all its methods implicitly.
- Example:
type Speaker interface {
Speak()
}
type Dog struct{}
func (d Dog) Speak() {
fmt.Println("Woof!")
}
13. What is a pointer in Go, and how is it used?
Pointers in Go store the memory address of a variable. They are used to reference and manipulate data directly.
- Example:
var x int = 10
var p *int = &x
fmt.Println(*p) // Dereferencing the pointer
14. How does Go handle type conversion?
Go requires explicit type conversion using the syntax T(value)
, where T
is the target type.
- Example:
var x int = 42
var y float64 = float64(x)
15. What is a goroutine, and how do you stop it?
A goroutine is a lightweight thread managed by the Go runtime. It can be stopped by signaling through channels or using context
for cancellation.
- Example:
done := make(chan bool)
go func() {
// Goroutine logic
done <- true
}()
<-done
16. What is the difference between var
and :=
in Go?
var
is used for declaring variables with explicit type declarations, which can be at the package or function level.:=
is a shorthand for declaring and initializing variables within functions without explicitly specifying the type; the type is inferred from the assigned value.
// Using var
var x int = 10
// Using :=
y := 20
17. How does Go manage memory?
- Go uses automatic memory management with a built-in garbage collector that handles allocation and deallocation.
- It employs stack and heap memory allocation; short-lived variables are typically allocated on the stack, while longer-lived objects reside on the heap.
- Developers can optimize memory usage by understanding variable lifetimes and using pointers judiciously.
18. What are channels in Go, and how are they used?
- Channels are conduits that allow goroutines to communicate and synchronize by sending and receiving values.
- They ensure safe data exchange between concurrent processes, preventing race conditions.
// Creating a channel
ch := make(chan int)
// Sending to a channel
ch <- 5
// Receiving from a channel
value := <-ch
19. How does Go handle variable scope?
- Go has block-level scope, meaning variables are accessible within the block they are declared and its nested blocks.
- Variables declared inside functions are local to those functions, while package-level variables are accessible throughout the package.
func example() {
var a int = 10 // Accessible only within this function
if a > 5 {
var b int = 20 // Accessible only within this if block
}
// b is not accessible here
}
20. What is the role of the init()
function in Go?
- The
init()
function is automatically executed when a package is initialized, before themain()
function runs. - It’s used to set up initial states, such as initializing variables, checking conditions, or registering types.
func init() {
// Initialization code
fmt.Println("Initialization complete")
}
21. How do you handle JSON data in Go?
- Go provides the
encoding/json
package to encode and decode JSON data. - Structs can be annotated with JSON tags to map JSON fields to Go struct fields.
import "encoding/json"
// Defining a struct with JSON tags
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
}
// Decoding JSON
var p Person
json.Unmarshal([]byte(`{"name":"Alice","age":30}`), &p)
// Encoding to JSON
data, _ := json.Marshal(p)
22. What is the Go workspace, and how is it structured?
- The Go workspace is the directory structure where Go code resides, typically organized with
src
,pkg
, andbin
directories. src
contains source code,pkg
holds compiled package objects, andbin
stores executable binaries.- With Go modules, the workspace structure is more flexible, allowing projects to be located outside the traditional
GOPATH
.
workspace/
├── src/
│ └── github.com/
│ └── user/
│ └── project/
├── pkg/
└── bin/
23. How does Go’s garbage collector work?
- Go’s garbage collector automatically identifies and frees memory that is no longer in use.
- It uses a concurrent, mark-and-sweep algorithm to minimize pause times and maintain application performance.
- The garbage collector runs in the background, allowing developers to focus on writing efficient code without manual memory management.
24. What are the different ways to concatenate strings in Go?
- Using the
+
operator: Simple and straightforward for small numbers of strings.
fullName := "John" + " " + "Doe"
- Using
fmt.Sprintf
: Useful for formatting strings with variables.
fullName := fmt.Sprintf("%s %s", firstName, lastName)
- Using
strings.Join
: Efficient for concatenating slices of strings.
parts := []string{"Hello", "World"}
combined := strings.Join(parts, " ")
25. How do you perform unit testing in Go?
- Go provides the
testing
package to write unit tests. - Test functions start with
Test
and take a pointer totesting.T
. - Use
go test
to run tests.
import "testing"
func Add(a, b int) int {
return a + b
}
func TestAdd(t *testing.T) {
result := Add(2, 3)
if result != 5 {
t.Errorf("Expected 5, got %d", result)
}
}
26. What is a struct in Go, and how do you define one?
- A struct is a composite data type that groups together variables under a single name, allowing the creation of complex data structures.
- Structs can have fields of different types and methods associated with them.
type Person struct {
Name string
Age int
}
// Creating an instance of a struct
p := Person{
Name: "Alice",
Age: 30,
}
27. How do you handle time and date operations in Go?
- Go provides the
time
package for managing time and date operations. - It supports parsing, formatting, measuring durations, and working with time zones.
import "time"
// Getting the current time
now := time.Now()
// Formatting time
formatted := now.Format("2006-01-02 15:04:05")
// Parsing time
t, err := time.Parse("2006-01-02", "2024-12-24")
28. What is the purpose of the select
statement in Go?
- The
select
statement allows a goroutine to wait on multiple communication operations (channels) simultaneously. - It enables handling of channel operations in a non-blocking manner and implementing timeouts or multiplexing.
select {
case msg1 := <-ch1:
fmt.Println("Received", msg1)
case ch2 <- msg2:
fmt.Println("Sent", msg2)
default:
fmt.Println("No communication")
}
29. How do you implement inheritance in Go?
- Go does not support traditional inheritance; instead, it uses composition and embedding.
- By embedding one struct within another, the outer struct inherits the fields and methods of the embedded struct.
type Animal struct {
Name string
}
func (a Animal) Speak() {
fmt.Println(a.Name, "makes a sound")
}
type Dog struct {
Animal
Breed string
}
// Using the embedded struct
d := Dog{
Animal: Animal{Name: "Buddy"},
Breed: "Golden Retriever",
}
d.Speak() // Outputs: Buddy makes a sound
30. What are the common tools used for dependency management in Go?
- Go Modules (
go.mod
andgo.sum
): The standard dependency management system introduced in Go 1.11, allowing versioning and reproducible builds.
go mod init github.com/user/project
go get github.com/some/dependency
- Vendoring: Storing dependencies locally within the project’s
vendor
directory to ensure consistent builds. - Third-party Tools:
- Dep: An older dependency management tool, now deprecated in favor of Go Modules.
- Glide: Another third-party tool, also largely replaced by Go Modules.
Go Modules are now the recommended approach for managing dependencies in Go projects.
31. How does Go’s scheduler manage goroutines?
- Go’s scheduler is responsible for managing the execution of goroutines, which are lightweight threads managed by the Go runtime.
- It uses a work-stealing scheduler with a model based on M (machine), P (processor), and G (goroutine) to efficiently distribute goroutines across available OS threads.
- The scheduler multiplexes thousands of goroutines onto a limited number of OS threads, handling preemption and ensuring fair execution.
- It optimizes for low latency and high throughput by minimizing context switching and leveraging efficient scheduling algorithms.
32. What are the best practices for error handling in Go?
- Explicit Error Checking: Always check for errors returned by functions to ensure robust code.
result, err := someFunction()
if err != nil {
// handle error
}
- Use Custom Error Types: Define custom error types to provide more context and enable error type assertions.
type MyError struct {
Msg string
}
func (e *MyError) Error() string {
return e.Msg
}
- Wrap Errors: Use
fmt.Errorf
with%w
to wrap errors, preserving the original error for unwrapping.
if err != nil {
return fmt.Errorf("operation failed: %w", err)
}
- Avoid Panic for Regular Errors: Reserve
panic
for unrecoverable situations, not for routine error handling. - Provide Context: Add context to errors to make debugging easier, indicating where and why the error occurred.
33. How do you implement reflection in Go?
- Reflection in Go is implemented using the
reflect
package, which allows inspection and manipulation of variables at runtime. - It is used to dynamically discover the type and value of variables, enabling generic programming and operations like serialization.
import (
"fmt"
"reflect"
)
func main() {
var x float64 = 3.4
v := reflect.ValueOf(x)
fmt.Println("Type:", v.Type())
fmt.Println("Value:", v.Float())
}
- Key Components:
reflect.Type
: Represents the type of a variable.reflect.Value
: Represents the value of a variable.
- Use Cases:
- Implementing generic libraries.
- Serialization and deserialization.
- Building frameworks that require runtime type information.
34. What is the unsafe
package in Go, and when would you use it?
- The
unsafe
package provides operations that step around Go’s type safety, allowing low-level memory manipulation. - It enables:
- Converting between arbitrary pointer types.
- Inspecting the memory layout of variables.
- Implementing performance optimizations that require direct memory access.
import (
"fmt"
"unsafe"
)
func main() {
var x int = 42
p := unsafe.Pointer(&x)
fmt.Println(p)
}
- When to Use:
- Interfacing with low-level system components or hardware.
- Optimizing performance-critical code where standard abstractions are insufficient.
- Implementing certain types of serialization/deserialization.
- Cautions:
- Use with care as it can lead to undefined behavior, memory corruption, and security vulnerabilities.
- Bypasses the Go compiler’s safety guarantees, making code harder to maintain and reason about.
35. How do you optimize Go code for performance?
- Efficient Algorithms and Data Structures: Choose the right algorithms and data structures to minimize computational complexity.
- Minimize Memory Allocations: Reuse objects and use stack allocation where possible to reduce garbage collector pressure.
// Using sync.Pool for object reuse
var pool = sync.Pool{
New: func() interface{} { return new(MyStruct) },
}
obj := pool.Get().(*MyStruct)
// Use obj
pool.Put(obj)
- Avoid Unnecessary Goroutines: Limit the number of goroutines to what is necessary to prevent excessive context switching.
- Profile and Benchmark: Use Go’s profiling tools (
pprof
,benchmarks
) to identify and address performance bottlenecks.
go test -bench=.
go tool pprof cpu.prof
- Use Buffering and Pipelining: Optimize I/O operations with buffered channels and pipeline patterns to enhance throughput.
- Leverage Concurrency: Utilize goroutines and channels effectively to parallelize tasks and make full use of multi-core processors.
- Inlining and Compiler Optimizations: Write simple functions to encourage the compiler to inline them, reducing function call overhead.
36. What are the implications of Go’s pass-by-value system?
- Copy Semantics: When variables are passed to functions, a copy of the variable is made. Modifications inside the function do not affect the original variable.
func modify(x int) {
x = 10
}
func main() {
a := 5
modify(a)
fmt.Println(a) // Outputs: 5
}
- Performance Considerations: Passing large structs by value can be inefficient due to the overhead of copying. Use pointers to pass references instead.
func modify(x *int) {
*x = 10
}
func main() {
a := 5
modify(&a)
fmt.Println(a) // Outputs: 10
}
- Immutability of Arguments: Since functions receive copies, the original data remains unchanged unless pointers are used, promoting safer code.
- Value vs. Reference Types:
- Value Types: Integers, floats, structs, etc., are copied on assignment and when passed to functions.
- Reference Types: Slices, maps, channels, pointers, etc., reference the same underlying data even when passed by value.
37. How do you implement a worker pool in Go?
- A worker pool allows multiple goroutines (workers) to process tasks concurrently from a shared task queue, improving efficiency and resource utilization.
import (
"fmt"
"sync"
)
func worker(id int, jobs <-chan int, wg *sync.WaitGroup) {
defer wg.Done()
for j := range jobs {
fmt.Printf("Worker %d processing job %d\n", id, j)
}
}
func main() {
const numWorkers = 3
jobs := make(chan int, 5)
var wg sync.WaitGroup
for w := 1; w <= numWorkers; w++ {
wg.Add(1)
go worker(w, jobs, &wg)
}
for j := 1; j <= 5; j++ {
jobs <- j
}
close(jobs)
wg.Wait()
}
- Components:
- Jobs Channel: A buffered or unbuffered channel that holds the tasks to be processed.
- Workers: Goroutines that listen on the jobs channel and process incoming tasks.
- WaitGroup: Synchronizes the completion of all workers before exiting the program.
- Advantages:
- Limits the number of concurrent workers, preventing resource exhaustion.
- Improves throughput by distributing tasks among multiple workers.
38. What is the purpose of build tags in Go?
- Build tags (also known as build constraints) are annotations that specify conditions under which a file should be included or excluded during the build process.
- They allow developers to include platform-specific code, enable or disable features, and manage different build configurations.
// +build linux
package main
func main() {
// Linux-specific code
}
- Usage:
- Platform-Specific Code: Separate implementations for different operating systems or architectures.
- Conditional Compilation: Include or exclude code based on build flags, such as debug or release modes.
- Syntax:
- Placed at the top of the file, preceding the
package
statement. - Can use logical operators like
&&
,||
, and!
.
- Placed at the top of the file, preceding the
- Examples:
// +build windows
for Windows-specific files.// +build !debug
to exclude files when thedebug
tag is present.
39. How do you manage configuration in Go applications?
- Configuration Files: Use formats like JSON, YAML, TOML, or INI to store configuration settings.
i
import (
"encoding/json"
"os"
)
type Config struct {
Port int `json:"port"`
Host string `json:"host"`
}
func loadConfig(filename string) (Config, error) {
var config Config
file, err := os.Open(filename)
if err != nil {
return config, err
}
defer file.Close()
decoder := json.NewDecoder(file)
err = decoder.Decode(&config)
return config, err
}
- Environment Variables: Store configuration in environment variables for flexibility and security.
import "os"
port := os.Getenv("PORT")
host := os.Getenv("HOST")
- Command-Line Flags: Use the
flag
package to parse command-line arguments for configuration.
import "flag"
var port = flag.Int("port", 8080, "Port to run the server on")
flag.Parse()
- Configuration Libraries: Utilize libraries like
Viper
for advanced configuration management, supporting multiple formats and sources.
import (
"github.com/spf13/viper"
)
func initConfig() {
viper.SetConfigName("config")
viper.SetConfigType("json")
viper.AddConfigPath(".")
if err := viper.ReadInConfig(); err != nil {
panic(err)
}
}
- Best Practices:
- Separate configuration from code to enhance flexibility and security.
- Use environment variables for sensitive data like API keys and passwords.
- Provide default values and validate configurations at startup.
40. What are the considerations for handling large datasets in Go?
- Memory Management:
- Ensure efficient memory usage by using appropriate data structures.Consider streaming data instead of loading entire datasets into memory.
file, _ := os.Open("largefile.txt")
scanner := bufio.NewScanner(file)
for scanner.Scan() {
process(scanner.Text())
}
- Concurrency:
- Utilize goroutines and channels to process data in parallel, improving throughput.
- Implement worker pools to manage concurrent processing without overwhelming system resources.
- Performance Optimization:
- Profile the application to identify and optimize bottlenecks.
- Use efficient algorithms and minimize unnecessary computations.
- Data Storage and Access:
- Choose appropriate storage solutions (databases, in-memory caches) that can handle large volumes of data.
- Implement indexing and caching strategies to speed up data retrieval.
- Error Handling and Resilience:
- Handle errors gracefully, especially when dealing with I/O operations.
- Implement retry mechanisms and fallback strategies for robustness.
- Scalability:
- Design the application to scale horizontally by distributing the workload across multiple instances or services.
- Use distributed processing frameworks if necessary.
- Garbage Collection Considerations:
- Large allocations can impact garbage collector performance. Reuse objects and manage lifetimes to minimize GC overhead.
- Serialization and Deserialization:
- Optimize serialization formats for speed and size when transmitting or storing large datasets.
- Testing and Validation:
- Test with real-world large datasets to ensure the application performs as expected under load.
By considering these factors, Go applications can efficiently handle large datasets, maintaining performance and reliability.
Learn More: Carrer Guidance | Hiring Now!
Top 40 AI Interview Questions and Answers for 2025
CSS Interview Questions for Freshers with Detailed Answers
AWS Interview Guide: Top 30 Questions and Answers
SSIS Interview Questions for Freshers with Answers
Selenium Cucumber scenario based Interview Questions and Answers
Tableau Scenario based Interview Questions and Answers