When moving a Node.js application from your local development environment to a production server, ensuring security and stability should be top priorities. Production environments often face more threats and scaling challenges than test environments, and your deployment strategy must reflect that. In this guide, we’ll explore how to securely set up an Ubuntu server for Node.js, manage the deployment pipeline, and harden the system to keep it stable, performant, and secure.
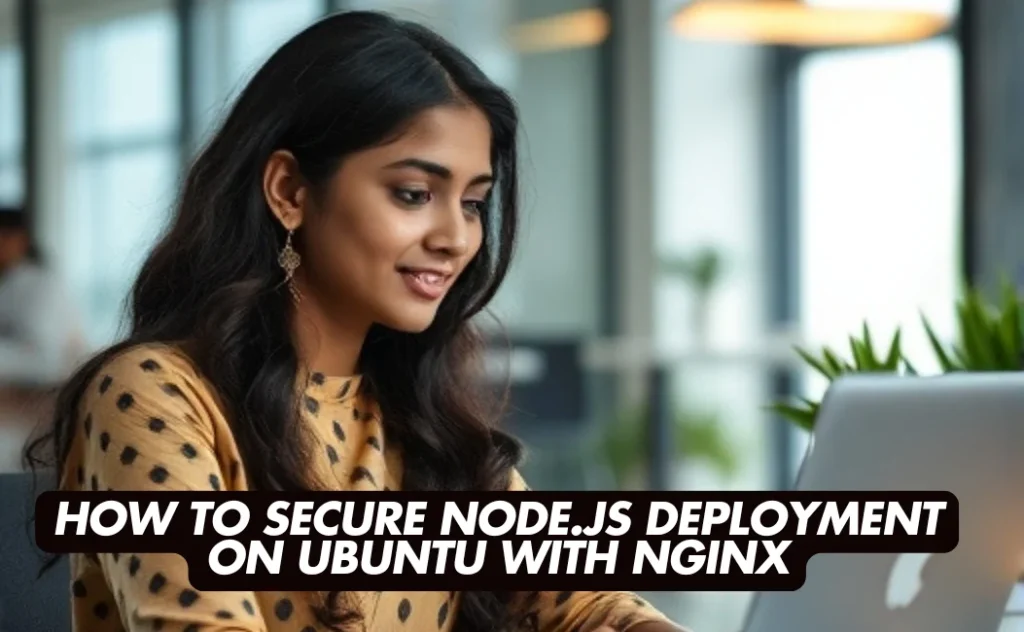
1. Preparing the Ubuntu Server
Create a Non-Root User
Start by deploying a fresh Ubuntu server through your cloud provider of choice (e.g., AWS, DigitalOcean, GCP). Once your server is ready, log in as the root
user and create a dedicated non-root user. This user will handle deployments and running the application.
adduser deployuser
usermod -aG sudo deployuser
Now, log out and log back in as deployuser
. The principle of least privilege ensures that if the application is compromised, attackers cannot easily escalate to root
.
Securing SSH Access
Default SSH settings are often too permissive. Harden them by disabling remote root
access and password-based logins. Open /etc/ssh/sshd_config
:
PermitRootLogin no
PasswordAuthentication no
You should rely on SSH keys for authentication. Consider changing the SSH port from the default 22
to something less obvious (e.g., 2222
) for additional security through obscurity:
Port 2222
After making these changes, apply them:
sudo systemctl restart ssh
Keep the System Updated
Regular updates prevent known vulnerabilities from going unpatched:
sudo apt update && sudo apt upgrade -y
sudo apt install -y build-essential git ufw fail2ban
- build-essential: Essential tools for building native Node.js dependencies.
- git: Useful for code deployment.
- ufw (Uncomplicated Firewall): For managing firewall rules.
- fail2ban: To protect against brute-force login attempts.
2. Firewall and Network Hardening
A firewall controls access points to your server. Ubuntu’s ufw
makes this straightforward:
sudo ufw allow 2222/tcp # Adjust if you changed SSH port
sudo ufw allow http
sudo ufw allow https
sudo ufw enable
With this, only SSH, HTTP (80), and HTTPS (443) are accessible. All other ports are blocked by default.
3. Installing and Managing Node.js
For a stable production environment, use a long-term support (LTS) version of Node.js. NodeSource provides an easy installation script:
curl -fsSL https://deb.nodesource.com/setup_lts.x | sudo -E bash -
sudo apt-get install -y nodejs
Validate the installation:
node -v
npm -v
To ensure consistent runtime environments, stick to the LTS release and avoid experimental versions for production.
4. Organize Application Files and Deployments
Establish a dedicated directory for your application:
sudo mkdir -p /var/www/myapp
sudo chown deployuser:deployuser /var/www/myapp
This isolates application code and ensures the deployuser
can manage it without root
privileges.
Handling Sensitive Information
Never store API keys, database credentials, or other secrets in version control. Instead, use environment variables or a .env
file that’s excluded from version control. Additionally, consider using a secrets manager such as HashiCorp Vault or AWS Secrets Manager for added security.
Continuous Deployment (CD) Considerations
For seamless deployments, integrate a CI/CD pipeline. Platforms like GitHub Actions or GitLab CI can push changes automatically to the server and run build scripts. By automating testing and deployments, you reduce human error and improve the reliability of updates.
5. Process Management With PM2 or systemd
Your Node.js application should run continuously and restart automatically if it crashes. There are two popular approaches:
Using PM2
PM2 is a production-grade process manager for Node.js:
sudo npm install pm2@latest -g
cd /var/www/myapp
pm2 start app.js --name myapp
pm2 startup systemd
pm2 save
Now PM2 will automatically start your app on boot and keep it alive, restarting it if it crashes.
Using systemd
If you prefer native tools, create a systemd service file at /etc/systemd/system/myapp.service
:
[Unit]
Description=My Node.js App
After=network.target
[Service]
User=deployuser
WorkingDirectory=/var/www/myapp
ExecStart=/usr/bin/node /var/www/myapp/app.js
Restart=always
Environment=NODE_ENV=production
[Install]
WantedBy=multi-user.target
Then enable and start the service:
sudo systemctl daemon-reload
sudo systemctl enable myapp
sudo systemctl start myapp
This ensures the application is treated as a first-class citizen in your system’s service ecosystem.
6. Setting Up a Reverse Proxy with Nginx
A reverse proxy like Nginx provides several benefits:
- Handles static file serving and caching.
- Manages SSL/TLS termination.
- Offers load balancing and rate limiting.
Installing Nginx
sudo apt install nginx -y
Configure a server block at /etc/nginx/sites-available/myapp
:
server {
listen 80;
server_name example.com;
location / {
proxy_pass http://localhost:3000; # Replace with your Node.js app port
proxy_http_version 1.1;
proxy_set_header Upgrade $http_upgrade;
proxy_set_header Connection 'upgrade';
proxy_set_header Host $host;
proxy_cache_bypass $http_upgrade;
}
}
Enable the configuration:
sudo ln -s /etc/nginx/sites-available/myapp /etc/nginx/sites-enabled/myapp
sudo nginx -t
sudo systemctl restart nginx
7. Enabling HTTPS with Let’s Encrypt and Certbot
Traffic encryption is no longer optional. HTTPS protects data in transit and improves SEO and user trust.
Install Certbot and fetch a free TLS certificate from Let’s Encrypt:
sudo apt install certbot python3-certbot-nginx -y
sudo certbot --nginx -d example.com
Certbot modifies your Nginx configuration to redirect HTTP to HTTPS. It also sets up automatic certificate renewal. You can test the renewal process:
sudo certbot renew --dry-run
8. Ongoing Security and Monitoring
Intrusion Detection and Prevention
- Fail2ban: Already installed, it monitors log files for repeated failed authentication attempts and bans offending IPs.
- Consider installing an Intrusion Detection System (IDS) or Host-based Intrusion Detection System (HIDS) like OSSEC for extra security layers.
Automatic Security Updates
Enable unattended-upgrades
for security patches:
sudo apt install unattended-upgrades
sudo dpkg-reconfigure --priority=low unattended-upgrades
This ensures critical fixes are applied automatically, reducing the attack surface.
Logging and Observability
- Application Logs: If you’re using PM2,
pm2 logs
provides a quick look at real-time logs. For long-term storage and analysis, consider a centralized logging system like the ELK stack (Elasticsearch, Logstash, Kibana) or Graylog. - Server Metrics: Tools like
htop
,glances
, or Prometheus with Grafana dashboards give insights into CPU, memory usage, and network performance. - APM (Application Performance Monitoring): For more in-depth metrics and tracing, consider solutions like New Relic, Datadog, or OpenTelemetry.
9. Backup and Recovery Strategy
Backups are your insurance policy. Even if your application is secure, hardware failures and accidental deletions can occur.
- Code Backups: Keep your code in a remote Git repository.
- Configuration Files: Regularly back up
/etc/nginx
,/etc/systemd/system
, and any custom scripts or configuration files. - Database Backups: If you’re running a database, schedule regular dumps or snapshots. Test restoring them periodically.
A well-tested disaster recovery plan ensures you can get back online quickly and confidently after unexpected incidents.
Conclusion
Securing a Node.js application in a production environment on Ubuntu requires a layered approach. By following these best practices—securing SSH, keeping the system updated, using a non-root deploy user, employing a reverse proxy, enabling HTTPS, and setting up continuous monitoring—you create a robust, secure foundation.
Over time, continue to improve your setup. As threats evolve and your application grows, you may add more sophisticated tools like Web Application Firewalls (WAFs), load balancers, containers or orchestration with Kubernetes, and advanced logging and alerting systems. Every iteration should aim for enhanced reliability, security, and performance.
By treating security as a continuous process rather than a one-time checklist, your Node.js application on Ubuntu will remain dependable and safe throughout its lifecycle.