Are you preparing for a Mocha interview? Mocha is a powerful JavaScript test framework running on Node.js, designed for both synchronous and asynchronous testing. This guide compiles 40 commonly asked Mocha interview questions and detailed answers to help you prepare effectively. From understanding the basics of Mocha installation to mastering advanced concepts like hooks, assertions, and asynchronous code testing, this comprehensive resource covers all the key topics.
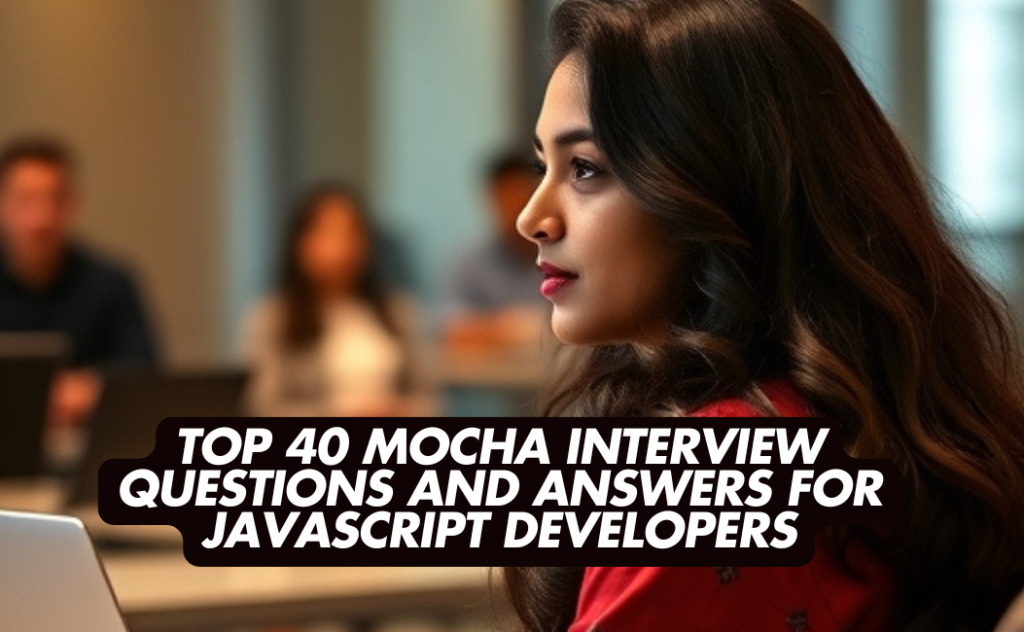
Top 40 Mocha Interview Questions and Answers
- What is Mocha and what is its role in testing?
- How do you install Mocha?
- What is the difference between describe() and it() in Mocha?
- What is the significance of before() and after() hooks in Mocha?
- What is the significance of beforeEach() and afterEach() hooks in Mocha?
- What is the role of assertions in Mocha?
- What is the difference between assert and expect in Mocha?
- How do you test asynchronous code using Mocha?
- What is the purpose of the –watch option in Mocha?
- How do you measure test coverage using Mocha?
- How do you handle asynchronous tests with timeouts in Mocha?
- How can you skip a test in Mocha?
- How do you run a specific test file or test case in Mocha?
- What are Mocha reporters, and how do you use them?
- How do you debug tests in Mocha?
- How do you organize test files in a Mocha project?
- How do you handle global variables in Mocha tests?
- How do you mock dependencies in Mocha tests?
- How do you test promises with Mocha?
- How do you generate test reports in Mocha?
- How do you handle dynamic tests in Mocha?
- What is the purpose of the –recursive option in Mocha?
- How can you share variables between hooks and test cases in Mocha?
- How do you handle uncaught exceptions in Mocha tests?
- What is the role of the done callback in asynchronous Mocha tests?
- How can you run Mocha tests in parallel?
- How do you test code that interacts with databases using Mocha?
- What is the significance of the –exit flag in Mocha?
- How do you handle environment-specific configurations in Mocha tests?
- How can you test private functions in Mocha?
- How do you perform data-driven testing in Mocha?
- How do you test code that uses timers (e.g., setTimeout) in Mocha?
- How do you test code that interacts with the file system in Mocha?
- How do you test code that makes HTTP requests in Mocha?
- How do you test middleware in an Express application using Mocha?
- How do you test error handling in Mocha?
- How do you test code that uses process.env variables in Mocha?
- How do you test code that interacts with databases in Mocha?
- How do you test code that uses external APIs in Mocha?
- How do you test code that involves user authentication in Mocha?
1. What is Mocha and what is its role in testing?
Mocha is a feature-rich JavaScript test framework that runs on Node.js and in the browser. It provides a flexible and powerful environment for writing both synchronous and asynchronous tests, supporting various testing methodologies like TDD (Test-Driven Development) and BDD (Behavior-Driven Development). Mocha allows developers to structure test cases using describe()
and it()
functions, manage asynchronous code with ease, and integrate with assertion libraries such as Chai. Its role in testing is to facilitate the creation of organized, maintainable, and efficient test suites that ensure code reliability and quality.
2. How do you install Mocha?
To install Mocha globally using npm, you can run the following command:
npm install -g mocha
This command installs Mocha globally, allowing you to run the mocha
command from anywhere on your system. Alternatively, to install Mocha as a development dependency in a specific project, navigate to your project directory and execute:
npm install --save-dev mocha
This adds Mocha to your project’s package.json
file under devDependencies
, ensuring consistency across different environments.
3. What is the difference between describe()
and it()
in Mocha?
In Mocha, describe()
and it()
are used to structure test suites and test cases:
describe()
: This function is used to group related tests. It takes a string describing the group and a callback function containing the individual tests or nesteddescribe()
blocks. It helps in organizing tests into logical collections.it()
: This function defines an individual test case. It takes a string describing the specific test and a callback function containing the test code. Eachit()
represents a single test scenario.
For example:
describe('Array', function() {
describe('#indexOf()', function() {
it('should return -1 when the value is not present', function() {
// Test implementation
});
});
});
In this structure, describe()
groups the tests related to the Array
object and its indexOf()
method, while it()
defines a specific test case.
4. What is the significance of before()
and after()
hooks in Mocha?
In Mocha, before()
and after()
hooks are used for setup and teardown processes that need to run once before all tests and once after all tests in a describe()
block, respectively:
before()
: Executed once before all the tests in adescribe()
block. It’s typically used for one-time setup tasks, such as initializing resources or establishing database connections.after()
: Executed once after all the tests in adescribe()
block. It’s used for cleanup tasks, like closing connections or deleting test data.
These hooks help in preparing the test environment and ensuring that resources are properly managed, leading to more reliable and maintainable tests.
5. What is the significance of beforeEach()
and afterEach()
hooks in Mocha?
The beforeEach()
and afterEach()
hooks in Mocha are used to execute code before and after each individual test case within a describe()
block:
beforeEach()
: Runs before each test case. It’s useful for setting up a consistent state before each test, such as resetting variables or initializing objects.afterEach()
: Runs after each test case. It’s used for cleaning up after tests, like resetting mocks or clearing data to ensure tests do not affect each other.
These hooks ensure that each test runs in isolation, maintaining test independence and reliability.
6. What is the role of assertions in Mocha?
Assertions are statements that check whether a condition is true. In Mocha, assertions are used to verify that the code under test behaves as expected. While Mocha itself doesn’t provide assertion functions, it integrates seamlessly with assertion libraries like Chai, Node’s built-in assert
module, or Should.js. These libraries offer a variety of assertion styles and methods to validate test outcomes.
For example, using Node’s built-in assert
module:
const assert = require('assert');
assert.equal(actual, expected);
Here, assert.equal()
checks if actual
is equal to expected
. If the assertion fails, an error is thrown, and Mocha reports the test as failed.
7. What is the difference between assert
and expect
in Mocha?
In Mocha, assert
and expect
are two distinct interfaces provided by assertion libraries to validate test outcomes:
assert
: This is a function-based assertion style, commonly provided by Node.js’s built-inassert
module or libraries like Chai. It uses functions such asassert.equal(actual, expected)
to perform assertions. This style is straightforward and resembles traditional assertion methods.Example using Node.js’s built-inassert
module:
const assert = require('assert');
assert.equal(actual, expected);
expect
: This is a more expressive, chainable assertion style provided by libraries like Chai. It allows for natural language assertions, making tests more readable. For instance,expect(actual).to.equal(expected)
expresses the expectation in a readable format. Example using Chai’sexpect
interface:
const { expect } = require('chai');
expect(actual).to.equal(expected);
Key Differences:
- Syntax and Readability: The
expect
style offers a more natural language syntax, which can make tests easier to read and understand, especially for those familiar with BDD (Behavior-Driven Development) practices. In contrast, theassert
style is more concise but may be less readable for complex assertions. - Chainability: The
expect
interface supports chainable assertions, allowing for more expressive tests. For example, you can chain multiple assertions together:
expect(array).to.be.an('array').that.includes(3);
This assertion checks that array
is an array and includes the number 3.
- Extensibility: Libraries like Chai, which provide the
expect
interface, often allow for custom assertions and plugins, offering greater flexibility. Theassert
interface is typically more rigid and limited to predefined assertion methods.
Choosing Between assert
and expect
:
The choice between assert
and expect
depends on the project’s needs and the team’s preferences:
- Use
assert
if you prefer a straightforward, function-based approach and are working on simpler tests where readability is less of a concern. It’s also suitable if you want to avoid adding external dependencies, as Node.js provides a built-inassert
module. - Use
expect
if you value expressive, readable, and chainable assertions, especially in larger projects where test clarity is crucial. Theexpect
style is particularly beneficial when writing BDD-style tests.
In summary, both assert
and expect
serve the purpose of making assertions in tests, but they offer different styles and capabilities. The choice between them should be guided by the specific requirements of your testing strategy and the preferences of your development team.
8. How do you test asynchronous code using Mocha?
Mocha provides robust support for testing asynchronous code, accommodating callbacks, Promises, and async/await syntax. Here’s how to handle each scenario:
- Using Callbacks: When testing functions that accept a callback, Mocha allows you to signal the completion of the test by invoking the
done
function. Example:
it('should complete asynchronously', function(done) {
asyncFunction(arg1, arg2, function(err, result) {
if (err) return done(err);
assert.equal(result, expected);
done();
});
});
In this setup, the test will wait until done()
is called, allowing for proper handling of asynchronous operations.
- Using Promises: For functions that return Promises, you can return the Promise directly from the test. Mocha will wait for the Promise to resolve or reject. Example:
it('should resolve with the expected value', function() {
return asyncFunction(arg1, arg2).then(function(result) {
assert.equal(result, expected);
});
});
If the Promise is rejected, Mocha will consider the test as failed.
- Using async/await: With modern JavaScript, you can use
async
functions and theawait
keyword to handle asynchronous code more intuitively. Example:
it('should return the expected value', async function() {
const result = await asyncFunction(arg1, arg2);
assert.equal(result, expected);
});
This approach allows for cleaner and more readable asynchronous tests.
By leveraging these methods, Mocha enables comprehensive testing of asynchronous code, ensuring that your functions behave as expected in various scenarios.
9. What is the purpose of the --watch
option in Mocha?
The --watch
option in Mocha enables watch mode, which monitors your test files and source code for changes. When a change is detected, Mocha automatically re-runs the relevant tests. This feature is particularly beneficial during development, as it provides immediate feedback on how code modifications impact your tests, facilitating a more efficient and responsive workflow.
10. How do you measure test coverage using Mocha?
While Mocha doesn’t include built-in test coverage tools, it integrates seamlessly with external libraries like Istanbul or its command-line interface, nyc
. To measure test coverage:
- Install
nyc
: Executenpm install --save-dev nyc
to addnyc
to your project. - Run Tests with Coverage: Use the command
npx nyc mocha
to run your tests with coverage instrumentation. - View Coverage Reports: After the tests complete,
nyc
generates a coverage report, typically in thecoverage
directory. You can open the HTML report to analyze which parts of your codebase are covered by tests and identify untested areas.
This process helps ensure comprehensive test coverage, enhancing code reliability.
11. How do you handle asynchronous tests with timeouts in Mocha?
Mocha sets a default timeout of 2 seconds for tests. If an asynchronous test exceeds this duration without completing, Mocha will consider it failed. To handle longer asynchronous operations, you can adjust the timeout:
- Set Timeout for a Single Test:
it('should take longer than default timeout', function(done) {
this.timeout(5000); // Set timeout to 5 seconds
asyncFunction(arg1, arg2, function() {
done();
});
});
- Set Timeout for a Test Suite:
describe('Long Running Tests', function() {
this.timeout(10000); // Set timeout to 10 seconds for all tests in this suite
// Tests go here
});
Adjusting timeouts ensures that Mocha accurately handles tests involving longer asynchronous operations without prematurely failing them.
12. How can you skip a test in Mocha?
Mocha provides mechanisms to skip tests, allowing you to focus on specific parts of your test suite during development:
- Skip a Single Test: Use
.skip()
on the individual test:
it.skip('should be implemented later', function() {
// Test code
});
- Skip a Test Suite: Use
.skip()
on adescribe
block:
describe.skip('Feature X Tests', function() {
// Tests
});
- Conditionally Skip Tests: Use
this.skip()
within a test:
it('should only run in production', function() {
if (process.env.NODE_ENV !== 'production') {
this.skip();
}
// Test code
});
Skipping tests is useful for temporarily disabling them without removal, aiding in focused development and debugging.
13. How do you run a specific test file or test case in Mocha?
Mocha provides several methods to execute specific test files or individual test cases, allowing for focused testing during development:
- Running a Specific Test File: You can specify the path to the test file you wish to run:
mocha path/to/test-file.js
This command executes only the tests defined in test-file.js
.
- Running Tests Matching a Pattern: Mocha’s
--grep
(or-g
) option allows you to run tests that match a specific pattern:
mocha --grep "pattern"
This command runs all tests with descriptions matching the provided pattern. For example, to run tests related to “User Authentication”:
mocha --grep "User Authentication"
This will execute all tests whose descriptions include “User Authentication”.
- Running a Specific Test or Suite Using
.only()
: Within your test files, Mocha provides the.only()
method to focus on a particular test or suite
describe.only('User Authentication', function() {
// Only tests in this suite will run
it('should log in successfully', function() {
// Test implementation
});
});
it.only('should log out successfully', function() {
// Only this test will run
});
Using .only()
ensures that only the specified test(s) or suite(s) are executed, ignoring others. This is particularly useful for isolating and debugging specific parts of your test suite.
By utilizing these methods, you can efficiently run specific tests or suites in Mocha, streamlining the development and debugging process.
14. What are Mocha reporters, and how do you use them?
Mocha reporters are modules that define how test results are presented. They format and display the outcomes of your test suites, providing insights into test performance and failures. Mocha includes several built-in reporters, such as:
- Spec: Provides a hierarchical view of test cases, indicating pass/fail status.
- Dot: Displays a dot for each test, useful for large test suites.
- Nyan: A whimsical reporter that shows a Nyan Cat with test progress.
- JSON: Outputs test results in JSON format, suitable for further processing.
To use a reporter, specify it with the --reporter
(or -R
) option when running Mocha:
mocha --reporter spec
You can also set the reporter in your project’s configuration file or package.json. Additionally, Mocha supports custom reporters, allowing you to create tailored reporting formats to suit your project’s needs.
15. How do you debug tests in Mocha?
Debugging Mocha tests can be accomplished using various methods:
- Using Node.js Debugger: Run Mocha with the
--inspect
flag to enable the Node.js debugger:
node --inspect node_modules/.bin/mocha
Then, open chrome://inspect
in Google Chrome to connect to the debugger.
- Using
console.log()
Statements: Insertconsole.log()
statements within your tests to output variable values and track execution flow. - Using Debugger Statements: Place
debugger;
statements in your code where you want to pause execution. When running Mocha with a debugger attached, execution will halt at these points, allowing you to inspect the current state. - Using Integrated Development Environment (IDE) Debugging Tools: Many IDEs, such as Visual Studio Code and WebStorm, offer integrated debugging tools that can be configured to work with Mocha, providing breakpoints, call stacks, and variable inspection.
By employing these debugging techniques, you can effectively identify and resolve issues within your Mocha tests.
16. How do you organize test files in a Mocha project?
Organizing test files in a Mocha project enhances maintainability and clarity. A common approach is to create a test
directory at the root of your project, containing test files that mirror the structure of your source code. For example:
project-root/
├── src/
│ ├── module1.js
│ └── module2.js
└── test/
├── module1.test.js
└── module2.test.js
This organization ensures that each test file corresponds to a specific module, making it easier to locate and manage tests. Additionally, you can group related tests using subdirectories within the test
directory.
When running Mocha, you can specify the test directory:
mocha test/
This command executes all test files within the test
directory.
Maintaining a well-structured test hierarchy facilitates efficient testing and project scalability.
17. How do you handle global variables in Mocha tests?
Managing global variables in Mocha tests is crucial to prevent unintended side effects and ensure test isolation. Here are best practices to handle global variables effectively:
- Avoid Using Global Variables: Define variables within the scope of individual tests or suites to prevent unintended interactions. This ensures that each test runs independently without being affected by shared state.
- Use Mocha’s
--check-leaks
Option: Mocha provides a--check-leaks
flag that detects global variable leaks during test execution. Running Mocha with this option will alert you to any unintended global variables:
mocha --check-leaks
If certain global variables are intentional, you can specify them using the --globals
option:
mocha --check-leaks --globals myGlobalVar
This configuration helps maintain control over global variables and prevents accidental leaks.
- Reset Global State Between Tests: If global variables are necessary, use Mocha’s hooks to reset their state:
beforeEach(function() {
global.someVariable = initialValue;
});
afterEach(function() {
delete global.someVariable;
});
This ensures that each test starts with a clean slate, maintaining test isolation.
- Isolate Test Environments: Consider using tools that provide isolated environments for each test, such as virtualization or containerization, to prevent global state interference.
By following these practices, you can manage global variables effectively in Mocha tests, ensuring reliability and maintainability.
18. How do you mock dependencies in Mocha tests?
Mocking dependencies is essential for isolating the unit of code under test. While Mocha doesn’t include built-in mocking capabilities, it integrates seamlessly with libraries like Sinon.js. Here’s how to mock dependencies using Sinon.js:
- Install Sinon.js: Add Sinon.js to your project:
npm install --save-dev sinon
- Require Sinon.js in Your Test File:
const sinon = require('sinon');
- Mock a Function: Use Sinon.js to create a mock function:
const myMock = sinon.mock(myObject);
- Set Expectations: Define the expected behavior of the mock:
myMock.expects('myMethod').once().returns(expectedValue);
- Verify Expectations: After executing the test, verify that the expectations were met:
myMock.verify();
myMock.restore();
By mocking dependencies, you can test components in isolation, ensuring that tests are focused and reliable.
19. How do you test promises with Mocha?
Mocha provides native support for testing promises. By returning a promise from your test, Mocha will wait for it to resolve or reject before determining the test’s outcome. Here’s how to test promises:
- Testing a Resolved Promise:
it('should resolve with the expected value', function() {
return myPromiseFunction().then(function(result) {
assert.equal(result, expectedValue);
});
});
- Testing a Rejected Promise: Ensure that the promise is rejected as expected:
it('should reject with an error', function() {
return myPromiseFunction().catch(function(error) {
assert.equal(error.message, 'Expected error message');
});
});
By returning the promise, Mocha manages the asynchronous behavior, ensuring accurate test results.
20. How do you generate test reports in Mocha?
Mocha offers various reporters to format and display test results. To generate test reports:
- Use a Built-in Reporter: Specify a reporter using the
--reporter
(or-R
) option:
mocha --reporter spec
Mocha includes several built-in reporters, such as spec
, dot
, nyan
, and json
.
- Use a Third-Party Reporter: Install a reporter like
mocha-junit-reporter
:
npm install --save-dev mocha-junit-reporter
Then, run Mocha with the specified reporter:
mocha --reporter mocha-junit-reporter
- Configure Report Output: Some reporters allow configuration of the output file:
mocha --reporter mocha-junit-reporter --reporter-options mochaFile=results.xml
Generating test reports is essential for continuous integration and tracking test results over time.
21. How do you handle dynamic tests in Mocha?
In Mocha, you can generate dynamic tests by programmatically creating test cases within a loop or function. This approach is useful when you have multiple similar tests that differ only in their input data or expected outcomes.
Example:
const testCases = [
{ input: 1, expected: 2 },
{ input: 2, expected: 4 },
{ input: 3, expected: 6 },
];
describe('Dynamic Tests', function() {
testCases.forEach(({ input, expected }) => {
it(`should double ${input} to get ${expected}`, function() {
const result = input * 2;
assert.equal(result, expected);
});
});
});
In this example, the forEach
loop iterates over the testCases
array, creating a new test for each set of inputs and expected outputs. This method keeps your test code DRY (Don’t Repeat Yourself) and maintainable.
22. What is the purpose of the --recursive
option in Mocha?
The --recursive
option in Mocha tells the test runner to include subdirectories when searching for test files. By default, Mocha looks for test files only in the specified directory. Using the --recursive
flag ensures that all nested test files are also executed.
Example:
mocha --recursive test/
This command runs all test files in the test
directory and its subdirectories, ensuring comprehensive test coverage across your project’s hierarchy.
23. How can you share variables between hooks and test cases in Mocha?
To share variables between hooks (before
, beforeEach
, after
, afterEach
) and test cases (it
) in Mocha, define the variables in the outer scope of the describe
block. This allows all nested functions to access and modify these variables.
Example:
describe('Shared Variables', function() {
let sharedVar;
before(function() {
sharedVar = initializeValue();
});
it('should use the shared variable', function() {
assert.equal(sharedVar, expectedValue);
});
after(function() {
sharedVar = null;
});
});
Here, sharedVar
is accessible within the before
hook, the test case, and the after
hook, allowing for shared state management across the test suite.
24. How do you handle uncaught exceptions in Mocha tests?
Mocha provides mechanisms to handle uncaught exceptions during test execution, ensuring that unexpected errors don’t cause the test runner to crash. You can use the process.on('uncaughtException')
event to catch these errors and handle them appropriately.
Example:
process.on('uncaughtException', function(err) {
console.error('Uncaught Exception:', err);
// Handle the error or fail the test
});
Additionally, Mocha has a built-in mechanism to detect uncaught exceptions during asynchronous tests. If an uncaught exception occurs, Mocha will automatically fail the test and report the error, helping you identify and address issues promptly.
25. What is the role of the done
callback in asynchronous Mocha tests?
In Mocha, the done
callback is used to signal the completion of an asynchronous test. When testing asynchronous code that doesn’t return a promise, you pass done
as an argument to the test function and call it once the asynchronous operations are complete. This informs Mocha to proceed to the next test.
Example:
it('should complete async operation', function(done) {
asyncFunction(arg1, arg2, function(err, result) {
if (err) return done(err);
assert.equal(result, expectedValue);
done();
});
});
Calling done()
indicates that the test has finished. If an error occurs, passing the error to done(err)
will cause the test to fail, providing proper error handling in asynchronous scenarios.
26. How can you run Mocha tests in parallel?
By default, Mocha runs tests serially to ensure predictable outcomes, especially when tests share resources or modify global state. However, for performance optimization, you can run tests in parallel using tools like mocha-parallel-tests
or by configuring Mocha’s built-in parallel mode (available in newer versions).
Using Mocha’s Built-in Parallel Mode:
mocha --parallel
Using mocha-parallel-tests
:
Install the package:
npm install --save-dev mocha-parallel-tests
Run tests:
npx mocha-parallel-tests test/
Ensure that your tests are independent and do not share state to prevent race conditions when running in parallel.
27. How do you test code that interacts with databases using Mocha?
Testing code that interacts with databases requires careful setup and teardown to ensure a consistent test environment. Use Mocha’s hooks to manage database connections and state.
Example:
describe('Database Tests', function() {
before(async function() {
await connectToDatabase();
});
beforeEach(async function() {
await clearDatabase();
});
it('should retrieve the correct data', async function() {
const data = await getDataFromDatabase();
assert.deepEqual(data, expectedData);
});
after(async function() {
await disconnectFromDatabase();
});
});
This setup ensures that each test starts with a clean database state, preventing tests from affecting each other and leading to more reliable results.
28. What is the significance of the --exit
flag in Mocha?
In Mocha version 4.0.0 and later, the test runner does not forcefully exit after tests complete. This change means that if there are lingering asynchronous operations or open handles (such as active timers, open database connections, or pending network requests), the Node.js process will remain active, potentially causing the test run to hang indefinitely.
The --exit
flag instructs Mocha to forcefully terminate the process after all tests have finished running, regardless of any remaining asynchronous operations. This behavior mirrors Mocha’s pre-4.0.0 functionality, where the process would exit automatically upon test completion.
Usage:
mocha --exit
Considerations:
- Resource Management: Relying on the
--exit
flag can mask issues where tests or application code fail to properly clean up resources. It’s generally better to ensure that all asynchronous operations are correctly handled and that resources are appropriately released at the end of each test. - Debugging: If your tests hang without the
--exit
flag, it indicates that there are unfinished asynchronous tasks or open handles. Identifying and resolving these issues leads to more robust and reliable tests.
In summary, while the --exit
flag can be a quick fix to prevent hanging tests, it’s advisable to use it sparingly and focus on proper resource management within your test suites.
29. How do you handle environment-specific configurations in Mocha tests?
Handling environment-specific configurations in Mocha tests ensures that tests run correctly across different environments (e.g., development, testing, production). This can be achieved by utilizing environment variables and configuration files.
Approach:
- Environment Variables: Set environment variables to control behavior based on the environment. In Node.js, you can access these variables using
process.env
. Example:
if (process.env.NODE_ENV === 'production') {
// Production-specific logic
} else {
// Development or testing logic
}
- Configuration Files: Maintain separate configuration files for each environment (e.g.,
config.dev.js
,config.prod.js
). Load the appropriate configuration based on the environment variable. Example
const config = require(`./config.${process.env.NODE_ENV}.js`);
- Setting Environment Variables: Set the
NODE_ENV
variable when running tests to specify the environment. Example:
NODE_ENV=testing mocha test/
Best Practices:
- Consistency: Ensure that environment variables are consistently set across different environments to avoid unexpected behavior.
- Security: Avoid hardcoding sensitive information in configuration files; instead, use environment variables to manage secrets securely.
By effectively managing environment-specific configurations, you can ensure that your Mocha tests are adaptable and reliable across various deployment scenarios.
30. How can you test private functions in Mocha?
Testing private functions (i.e., functions not exported from a module) can be challenging, as they are not directly accessible from test files. However, there are several approaches to test their functionality:
- Refactor to Export for Testing: Modify the module to export private functions conditionally, specifically for testing purposes. Example:
// module.js
function privateFunction() {
// Implementation
}
module.exports = {
publicFunction,
// Export privateFunction only in test environment
...(process.env.NODE_ENV === 'test' && { privateFunction }),
};
// test.js
const { privateFunction } = require('./module');
// Test privateFunction
- Use a Testing Framework with Access Capabilities: Some testing frameworks or tools allow access to private functions through reflection or other mechanisms. However, this approach can be complex and is less common in JavaScript.
- Test Through Public Interfaces: The recommended approach is to test private functions indirectly by testing the public functions that utilize them. This ensures that the internal logic is tested as part of the overall functionality without exposing private implementation details. Example:
// module.js
function privateFunction() {
// Implementation
}
function publicFunction() {
// Calls privateFunction
return privateFunction();
}
module.exports = { publicFunction };
// test.js
const { publicFunction } = require('./module');
// Test publicFunction, which indirectly tests privateFunction
Considerations:
- Encapsulation: Exposing private functions solely for testing can break encapsulation and lead to brittle tests that are tightly coupled to implementation details.
- Refactoring: If a private function is complex and requires direct testing, consider refactoring the code to make the function a public method of a separate module, thereby maintaining encapsulation while allowing direct testing.
In summary, while it’s possible to test private functions by exposing them conditionally or using specialized tools, it’s generally better to test them indirectly through public interfaces to maintain proper encapsulation and robust test design.
31. How do you perform data-driven testing in Mocha?
Data-driven testing involves running the same test logic with multiple sets of input data to ensure that a function behaves correctly under various conditions. In Mocha, this can be achieved by iterating over an array of test cases and dynamically creating tests for each set of inputs.
Approach:
- Define Test Cases: Create an array of objects, each representing a set of inputs and the expected output. Example:
const testCases = [
{ input: 1, expected: 2 },
{ input: 2, expected: 4 },
{ input: 3, expected: 6 },
];
- Iterate Over Test Cases: Use
forEach
or a similar loop to iterate over the test cases and create a test for each one. Example:
describe('multiplyByTwo function', function() {
testCases.forEach(({ input, expected }) => {
it(`should return ${expected} when input is ${input}`, function() {
const result = multiplyByTwo(input);
assert.equal(result, expected);
});
});
});
In this setup, multiplyByTwo
is the function under test, and assert.equal
is used to verify that the function’s output matches the expected value for each input.
Using External Libraries:
For more complex scenarios, you can use libraries like mocha-data-driven
, which provide utilities to facilitate data-driven testing.
Example with mocha-data-driven
:
- Install the Library:
npm install --save-dev mocha-data-driven
- Implement Data-Driven Tests:
const dataDriven = require('mocha-data-driven');
describe('multiplyByTwo function', function() {
dataDriven([
{ input: 1, expected: 2 },
{ input: 2, expected: 4 },
{ input: 3, expected: 6 },
], function() {
it('should return {expected} when input is {input}', function(ctx) {
const result = multiplyByTwo(ctx.input);
assert.equal(result, ctx.expected);
});
});
});
In this example, dataDriven
facilitates the iteration over test cases, and placeholders in the test description ({input}
, {expected}
) are replaced with actual values during execution.
Benefits:
- Efficiency: Reduces code duplication by consolidating similar tests into a single, parameterized structure.
- Coverage: Ensures that various input scenarios are tested, improving the robustness of your code.
By implementing data-driven testing in Mocha, you can streamline your test suite and enhance its effectiveness in validating different input scenarios.
32. How do you test code that uses timers (e.g., setTimeout
) in Mocha?
Testing code that relies on timers, such as setTimeout
or setInterval
, requires controlling the passage of time to ensure predictable and efficient tests. Sinon.js, a popular JavaScript testing utility, provides functionality to mock timers, allowing you to simulate the passage of time.
Approach:
- Install Sinon.js:
npm install --save-dev sinon
- Use Fake Timers:
const sinon = require('sinon');
describe('Timer-based function', function() {
let clock;
before(function() {
// Create a fake timer
clock = sinon.useFakeTimers();
});
after(function() {
// Restore the real timers
clock.restore();
});
it('should execute after 1 second', function() {
const callback = sinon.spy();
function timerFunction() {
setTimeout(callback, 1000);
}
timerFunction();
// Fast-forward time by 1 second
clock.tick(1000);
// Assert that the callback was called
sinon.assert.calledOnce(callback);
});
});
In this example, sinon.useFakeTimers()
replaces the native timer functions with Sinon’s implementations, allowing you to control time. The clock.tick(1000)
call advances the virtual clock by 1000 milliseconds, triggering the setTimeout
callback immediately.
Benefits:
- Deterministic Tests: Eliminates the unpredictability associated with real-time delays.
- Performance: Speeds up tests by simulating time progression without actual waiting.
By mocking timers in your Mocha tests, you can effectively test time-dependent code in a controlled and efficient manner.
33. How do you test code that interacts with the file system in Mocha?
Testing code that interacts with the file system requires careful handling to ensure tests are reliable and do not have unintended side effects on the actual file system. Here are common approaches to achieve this:
Approach 1: Using mock-fs
mock-fs
is a Node.js package that allows developers to create a mock file system for testing purposes. It simulates a file system environment, enabling you to test file operations without affecting the actual file system.
- Install
mock-fs
:
npm install --save-dev mock-fs
- Mock File System Operations:
const mock = require('mock-fs');
const fs = require('fs');
describe('File System Interaction', function() {
before(function() {
// Mock the file system
mock({
'path/to/fake/dir': {
'file.txt': 'file content here',
},
});
});
after(function() {
// Restore the real file system
mock.restore();
});
it('should read file content', function() {
const content = fs.readFileSync('path/to/fake/dir/file.txt', 'utf8');
assert.equal(content, 'file content here');
});
});
In this setup, mock-fs
creates an in-memory file system based on the provided structure, allowing you to test file operations without affecting the actual file system.
Approach 2: Using Temporary Directories and Files
Alternatively, you can use Node.js modules like fs
and os
to create temporary directories and files during testing. This ensures that any file system interactions are isolated and do not interfere with the real file system.
- Create Temporary Directories and Files:
const fs = require('fs');
const os = require('os');
const path = require('path');
describe('File System Interaction', function() {
let tempDir;
before(function() {
// Create a temporary directory
tempDir = fs.mkdtempSync(path.join(os.tmpdir(), 'test-'));
});
after(function() {
// Clean up the temporary directory
fs.rmdirSync(tempDir, { recursive: true });
});
it('should create a new file', function() {
const filePath = path.join(tempDir, 'test.txt');
fs.writeFileSync(filePath, 'Hello, world!');
const content = fs.readFileSync(filePath, 'utf8');
assert.equal(content, 'Hello, world!');
});
});
This approach ensures that file system interactions during tests are confined to temporary locations, preventing any unintended modifications to the actual file system.
Best Practices:
- Isolation: Ensure that each test has its own isolated file system environment to prevent tests from affecting each other.
- Cleanup: Always clean up any temporary files or directories created during tests to maintain a clean testing environment.
- Error Handling: Implement proper error handling to manage scenarios where file operations may fail.
By following these practices, you can effectively test code that interacts with the file system in Mocha, ensuring reliability and preventing side effects on the actual file system.
34. How do you test code that makes HTTP requests in Mocha?
Testing code that makes HTTP requests can be challenging due to the need to control external service interactions. To handle this, you can use libraries like nock
to mock HTTP requests and responses, allowing you to test your code in isolation.
Approach: Using nock
nock
is a HTTP mocking and expectations library for Node.js that allows you to intercept and mock HTTP requests.
- Install
nock
:
npm install --save-dev nock
- Mock HTTP Requests:
const nock = require('nock');
const axios = require('axios');
describe('HTTP Request Tests', function() {
before(function() {
// Mock the external API
nock('https://api.example.com')
.get('/data')
.reply(200, { key: 'value' });
});
it('should fetch data from API', async function() {
const response = await axios.get('https://api.example.com/data');
assert.deepEqual(response.data, { key: 'value' });
});
after(function() {
// Clean up all mocks
nock.cleanAll();
});
});
In this example, nock
intercepts the HTTP GET request to https://api.example.com/data
and responds with a predefined JSON object. This allows you to test how your code handles the response without making actual network requests.
Best Practices:
- Isolation: Mock external HTTP requests to ensure tests are not dependent on external services, leading to more reliable and faster tests.
- Coverage: Test various scenarios, including different response statuses and error conditions, to ensure your code handles all possible outcomes.
- Cleanup: Ensure that mocks are cleaned up after tests to prevent interference with other tests.
By mocking HTTP requests, you can effectively test code that interacts with external APIs, ensuring that your application behaves as expected under various conditions.
35. How do you test middleware in an Express application using Mocha?
Testing middleware in an Express application involves isolating the middleware function and simulating HTTP requests and responses to verify its behavior. This can be achieved using tools like supertest
and assertion libraries such as chai
.
Approach: Using supertest
and chai
- Install Necessary Packages: Ensure that
supertest
,chai
, andmocha
are installed as development dependencies:
npm install --save-dev supertest chai mocha
- Set Up the Middleware and Express Application: Define your middleware function and integrate it into an Express application.
// middleware.js
function myMiddleware(req, res, next) {
req.customProperty = 'customValue';
next();
}
module.exports = myMiddleware;
// app.js
const express = require('express');
const myMiddleware = require('./middleware');
const app = express();
app.use(myMiddleware);
app.get('/test', (req, res) => {
res.json({ customProperty: req.customProperty });
});
module.exports = app;
- Write Tests Using
supertest
andchai
: Create a test file to verify the middleware’s functionality.
// test/middleware.test.js
const request = require('supertest');
const { expect } = require('chai');
const app = require('../app');
describe('Middleware Tests', function() {
it('should add customProperty to the request object', async function() {
const response = await request(app).get('/test');
expect(response.status).to.equal(200);
expect(response.body).to.have.property('customProperty', 'customValue');
});
});
- In this test,
supertest
is used to simulate an HTTP GET request to the/test
endpoint. The response is then checked to ensure that thecustomProperty
added by the middleware is present and has the expected value. - Run the Tests:Execute the tests using Mocha:
npx mocha test/middleware.test.js
Best Practices:
- Isolation: Test middleware functions in isolation to ensure they behave as expected without interference from other parts of the application.
- Comprehensive Testing: Cover various scenarios, including edge cases, to verify the middleware’s robustness.
- Mocking Dependencies: If the middleware relies on external services or databases, consider mocking these dependencies to maintain test isolation and speed.
By following this approach, you can effectively test middleware in an Express application using Mocha, ensuring that your middleware functions perform as intended within the application’s request-response cycle.
36. How do you test error handling in Mocha?
Testing error handling ensures that your application gracefully manages unexpected situations. In Mocha, you can simulate errors and verify that your code responds appropriately.
Approach:
- Simulate Errors:Trigger errors within your functions or use mocking libraries like
sinon
to simulate exceptions.
const sinon = require('sinon');
it('should handle errors gracefully', function() {
const error = new Error('Something went wrong');
const stub = sinon.stub(myModule, 'functionThatThrows').throws(error);
try {
myModule.functionUnderTest();
} catch (err) {
assert.equal(err.message, 'Something went wrong');
}
stub.restore();
});
- Test Asynchronous Error Handling:For asynchronous functions, use the
done
callback to handle errors.
it('should handle async errors', function(done) {
asyncFunctionThatThrows().catch((err) => {
assert.equal(err.message, 'Async error');
done();
});
});
Best Practices:
- Comprehensive Coverage: Test both synchronous and asynchronous error scenarios.
- Clear Assertions: Ensure that error messages and types are as expected to validate proper error handling.
By simulating errors and verifying your application’s responses, you can ensure robust error handling in your codebase.
37. How do you test code that uses process.env
variables in Mocha?
Testing code that relies on environment variables (process.env
) requires controlling these variables within your test environment to ensure predictable behavior.
Approach:
- Set Environment Variables in Tests:Assign values to
process.env
before running your tests.
before(function() {
process.env.MY_VARIABLE = 'testValue';
});
it('should use the environment variable', function() {
const result = functionThatUsesEnvVariable();
assert.equal(result, 'testValue');
});
after(function() {
delete process.env.MY_VARIABLE;
});
- Use a
.env
File for Configuration:Utilize packages likedotenv
to load environment variables from a.env
file during testing.
require('dotenv').config({ path: './test.env' });
it('should read environment variable from .env file', function() {
const result = functionThatUsesEnvVariable();
assert.equal(result, process.env.MY_VARIABLE);
});
Best Practices:
- Isolation: Ensure that setting environment variables in tests does not affect other tests or the development environment.
- Cleanup: Restore or delete environment variables after tests to maintain a clean state.
By managing process.env
variables within your tests, you can accurately simulate different environments and validate your code’s behavior under various configurations.
38. How do you test code that interacts with databases in Mocha?
Testing code that interacts with databases requires careful handling to ensure tests are reliable, repeatable, and do not affect the actual database state. Here are common approaches to achieve this:
Approach 1: Using an In-Memory Database
Utilizing an in-memory database allows you to run tests without affecting your production database. For example, if you’re using MongoDB, you can use mongodb-memory-server
to spin up an in-memory instance.
- Install the In-Memory Server:
npm install --save-dev mongodb-memory-server
- Set Up the In-Memory Database in Tests:
const { MongoMemoryServer } = require('mongodb-memory-server');
const mongoose = require('mongoose');
let mongoServer;
before(async () => {
mongoServer = await MongoMemoryServer.create();
const uri = mongoServer.getUri();
await mongoose.connect(uri, { useNewUrlParser: true, useUnifiedTopology: true });
});
after(async () => {
await mongoose.disconnect();
await mongoServer.stop();
});
it('should save a user to the database', async () => {
const user = new User({ name: 'John Doe' });
const savedUser = await user.save();
assert.equal(savedUser.name, 'John Doe');
});
In this setup, mongodb-memory-server
provides a fresh MongoDB instance for each test run, ensuring isolation and repeatability.
Approach 2: Mocking Database Calls
For unit tests, mocking database interactions can isolate the code under test. Libraries like sinon
can mock functions and simulate database behavior.
- Install Sinon:
npm install --save-dev sinon
- Mock Database Functions:
const sinon = require('sinon');
const database = require('../database');
const { getUser } = require('../userService');
describe('getUser', () => {
it('should return user data', async () => {
const mockUser = { id: 1, name: 'John Doe' };
const stub = sinon.stub(database, 'findUserById').resolves(mockUser);
const user = await getUser(1);
assert.deepEqual(user, mockUser);
stub.restore();
});
});
By mocking the findUserById
function, the test avoids actual database calls, ensuring faster and more reliable tests.
Best Practices:
- Isolation: Ensure tests do not depend on the state of a real database to prevent flaky tests.
- Cleanup: Always clean up any test data or mock setups to maintain test integrity.
- Consistency: Use consistent data and states across tests to ensure reliability.
By following these practices, you can effectively test database interactions in Mocha, ensuring your data-related code functions correctly without unintended side effects.
39. How do you test code that uses external APIs in Mocha?
Testing code that relies on external APIs can be challenging due to factors like network variability and API rate limits. To handle this, you can mock external API calls to simulate various responses and ensure your code handles them correctly.
Approach: Using nock
to Mock HTTP Requests
nock
is a library that allows you to intercept and mock HTTP requests in Node.js, making it ideal for testing code that interacts with external APIs.
- Install
nock
:
npm install --save-dev nock
- Mock External API Calls:
const nock = require('nock');
const axios = require('axios');
describe('External API Interaction', () => {
before(() => {
nock('https://api.example.com')
.get('/data')
.reply(200, { key: 'value' });
});
it('should fetch data from external API', async () => {
const response = await axios.get('https://api.example.com/data');
assert.deepEqual(response.data, { key: 'value' });
});
after(() => {
nock.cleanAll();
});
});
In this example, nock
intercepts the HTTP GET request to https://api.example.com/data
and returns a predefined response, allowing you to test how your code handles the API response without making actual network requests.
Best Practices:
- Isolation: Mock external API calls to ensure tests are not dependent on external services, leading to more reliable and faster tests.
- Coverage: Test various scenarios, including different response statuses and error conditions, to ensure your code handles all possible outcomes.
- Cleanup: Ensure that mocks are cleaned up after tests to prevent interference with other tests.
By mocking external API calls, you can effectively test how your code interacts with external services, ensuring robustness and reliability in various scenarios.
40. How do you test code that involves user authentication in Mocha?
To test user authentication in Mocha, simulate authenticated and unauthenticated requests using supertest and chai. This ensures your authentication mechanisms work correctly.
Setup
- Install Dependencies:
npm install --save-dev supertest chai mocha
- Express Application:
Set up an Express app with authentication middleware and protected routes.
Example Code
// app.js
const express = require('express');
const passport = require('passport');
const session = require('express-session');
const app = express();
app.use(express.json());
app.use(session({ secret: 'your_secret_key', resave: false, saveUninitialized: true }));
app.use(passport.initialize());
app.use(passport.session());
function isAuthenticated(req, res, next) {
req.isAuthenticated() ? next() : res.status(401).json({ message: 'Unauthorized' });
}
app.post('/login', passport.authenticate('local'), (req, res) => {
res.status(200).json({ message: 'Logged in' });
});
app.get('/protected', isAuthenticated, (req, res) => {
res.status(200).json({ message: 'Access granted' });
});
module.exports = app;
Write Tests
Create a test file to verify the authentication flow.
// test/auth.test.js
const request = require('supertest');
const { expect } = require('chai');
const app = require('../app');
describe('Authentication Tests', function() {
it('should log in a user with valid credentials', function(done) {
request(app)
.post('/login')
.send({ username: 'validUser', password: 'validPassword' })
.expect(200)
.end((err, res) => {
if (err) return done(err);
expect(res.body).to.have.property('message', 'Logged in');
done();
});
});
it('should prevent access to protected route without authentication', function(done) {
request(app)
.get('/protected')
.expect(401)
.end((err, res) => {
if (err) return done(err);
expect(res.body).to.have.property('message', 'Unauthorized');
done();
});
});
it('should allow access to protected route after login', function(done) {
const agent = request.agent(app);
agent
.post('/login')
.send({ username: 'validUser', password: 'validPassword' })
.expect(200)
.end((err) => {
if (err) return done(err);
agent
.get('/protected')
.expect(200)
.end((err, res) => {
if (err) return done(err);
expect(res.body).to.have.property('message', 'Access granted');
done();
});
});
});
});
Run the Tests
Execute the tests with Mocha:
npx mocha test/auth.test.js
Best Practices
- Test Various Scenarios: Include tests for valid/invalid logins and session management.
- Use Test Databases: Avoid manipulating real user data.
- Mock External Services: Ensure tests are reliable by mocking dependencies.
This approach effectively tests user authentication in your Express application using Mocha.
Learn More: Carrer Guidance | Hiring Now!
Top 25+ Java Multi Threading Interview Questions and Answers
Tosca Real-Time Scenario Questions and Answers
Advanced TOSCA Test Automation Engineer Interview Questions and Answers with 5+ Years of Experience
Tosca Interview Questions for Freshers with detailed Answers
Tosca interview question and answers- Basic to Advanced