Are you preparing for a jQuery interview? jQuery, a fast, small, and feature-rich JavaScript library, has revolutionized web development by simplifying HTML document traversal, event handling, animation, and AJAX interactions. To help you out, we have compiled the top 50 jQuery interview questions with answers. This guide covers everything from the basics of jQuery inclusion and element selection to advanced topics like event delegation, AJAX requests, and method chaining.
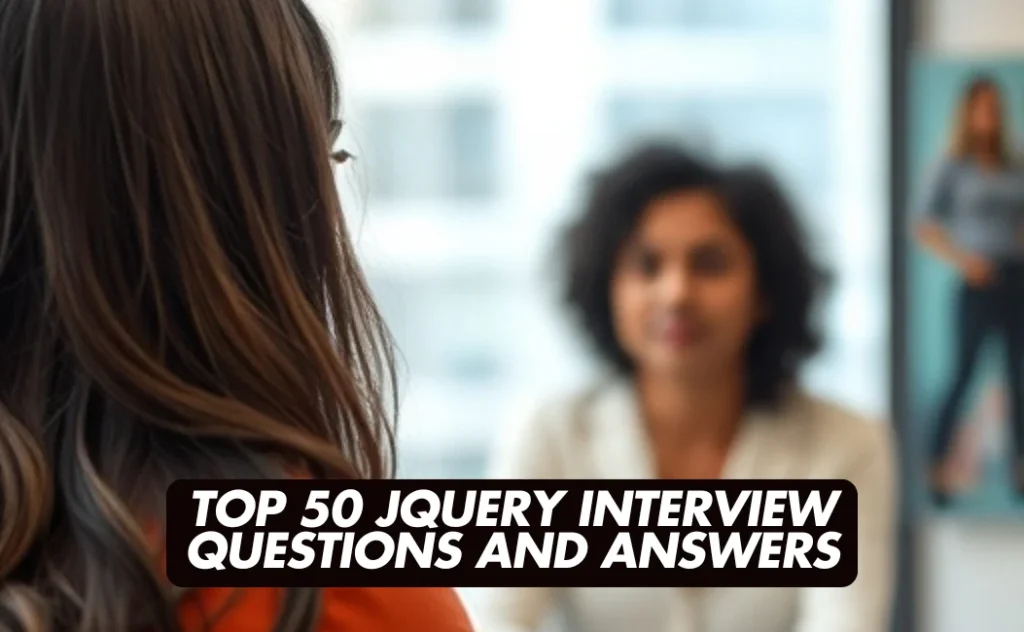
Top 50 jQuery Interview Questions and Answers
- What is jQuery, and why is it used in web development?
- How do you include jQuery in a web page?
- Explain the difference between $(document).ready() and $(window).load().
- How can you select elements by class and ID in jQuery?
- What is event delegation in jQuery?
- How do you perform an AJAX request using jQuery?
- What is the difference between .empty(), .remove(), and .detach() in jQuery?
- How can you add and remove CSS classes using jQuery?
- Explain the concept of method chaining in jQuery.
- How do you handle events for dynamically added elements in jQuery?
- What is the purpose of the $.each() function in jQuery?
- How can you stop event propagation in jQuery?
- What is the difference between .on() and .bind() methods in jQuery?
- How do you perform form validation using jQuery?
- How can you animate elements using jQuery?
- What is the purpose of the $.noConflict() method in jQuery?
- How do you get and set the value of form elements using jQuery?
- How can you load content from a server and place it into an element using jQuery?
- What is the difference between .prop() and .attr() methods in jQuery?
- How do you traverse the DOM using jQuery?
- How can you check if an element exists in the DOM using jQuery?
- What is the difference between .fadeIn() and .fadeTo() methods in jQuery?
- How can you stop an animation or effect in jQuery?
- How do you get the parent element of a selected element in jQuery?
- What is the purpose of the .serialize() method in jQuery?
- How can you bind multiple event handlers to a single element in jQuery?
- How do you remove an attribute from an HTML element using jQuery?
- How can you get the index of an element within its parent using jQuery?
- What is the difference between .slideUp() and .slideDown() methods in jQuery?
- How do you delegate events to dynamically added elements in jQuery?
- How can you prevent the default action of an event in jQuery?
- What is the purpose of the .data() method in jQuery?
- How do you perform asynchronous HTTP requests using jQuery?
- How can you load JSON data using jQuery?
- What is the difference between .children() and .find() methods in jQuery?
- How can you get the text content of an element using jQuery?
- How do you set the HTML content of an element using jQuery?
- What is the difference between .eq() and .get() methods in jQuery?
- How can you clone an element along with its event handlers using jQuery?
- How do you bind an event handler that executes only once using jQuery?
- How can you check if a checkbox is checked using jQuery?
- How do you disable or enable an input element using jQuery?
- How can you get the width and height of an element using jQuery?
- How do you get the position of an element relative to the document using jQuery?
- How can you hide an element and remove it from the DOM using jQuery?
- How do you attach multiple event handlers to the same element for the same event in jQuery?
- How can you trigger an event programmatically using jQuery?
- How can you prevent event propagation in jQuery?
- How do you check if an element is visible in the viewport using jQuery?
- How can you debounce a function in jQuery to limit its execution rate?
1. What is jQuery, and why is it used in web development?
jQuery is a fast, small, and feature-rich JavaScript library designed to simplify HTML document traversal and manipulation, event handling, animation, and AJAX interactions. It enables developers to write less code while achieving more functionality, ensuring cross-browser compatibility and enhancing user experience.
2. How do you include jQuery in a web page?
You can include jQuery in a web page by:
Using a CDN: Include the following <script>
tag in your HTML:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Hosting Locally: Download the jQuery library from the official website and include it:
<script src="path/to/jquery-3.6.0.min.js"></script>
3. Explain the difference between $(document).ready()
and $(window).load()
.
$(document).ready()
: Executes the specified function as soon as the DOM is fully loaded, without waiting for images or other resources.$(window).load()
: Executes the function after the entire page, including all assets like images, has fully loaded.
Using $(document).ready()
ensures that your code runs as soon as the HTML is ready, improving performance.
4. How can you select elements by class and ID in jQuery?
By ID: Use the #
symbol followed by the ID:
$('#elementID')
By Class: Use the .
symbol followed by the class name:
$('.className')
These selectors allow precise targeting of HTML elements for manipulation.
5. What is event delegation in jQuery?
Event delegation involves attaching a single event handler to a parent element to manage events for its child elements, even those added dynamically. This approach improves performance and simplifies code management.
Example:
$(document).on('click', '.child-class', function() {
// Event handler code
});
Here, the click event is handled for all current and future elements with the class .child-class
.
6. How do you perform an AJAX request using jQuery?
jQuery provides the $.ajax()
method for AJAX requests. A simple GET request example:
$.ajax({
url: 'https://api.example.com/data',
method: 'GET',
success: function(response) {
// Handle success
},
error: function(error) {
// Handle error
}
});
This method allows for asynchronous data retrieval without reloading the page.
7. What is the difference between .empty()
, .remove()
, and .detach()
in jQuery?
.empty()
: Removes all child elements and content from the selected elements..remove()
: Removes the selected elements and their child elements from the DOM entirely..detach()
: Similar to.remove()
, but retains the data and event handlers associated with the removed elements, allowing for potential reattachment.
These methods provide flexibility in manipulating the DOM based on specific requirements.
8. How can you add and remove CSS classes using jQuery?
Add a Class: Use .addClass()
:
$('selector').addClass('className');
Remove a Class: Use .removeClass()
:
$('selector').removeClass('className');
These methods facilitate dynamic styling of elements.
9. Explain the concept of method chaining in jQuery.
Method chaining allows multiple jQuery methods to be called sequentially on the same element(s) in a single statement. This is possible because most jQuery methods return the jQuery object itself.
Example:
$('#element').addClass('active').slideDown().html('Content');
This approach leads to more concise and readable code.
10. How do you handle events for dynamically added elements in jQuery?
For dynamically added elements, use event delegation with the .on()
method:
$(document).on('event', 'selector', function() {
// Event handler code
});
This ensures that the event handler is applied to both existing and future elements matching the selector.
11. What is the purpose of the $.each()
function in jQuery?
The $.each()
function iterates over objects and arrays, executing a function for each matched element.
Example:
$.each(arrayOrObject, function(index, value) {
// Code to execute for each element
});
This function simplifies iteration over collections.
12. How can you stop event propagation in jQuery?
To stop event propagation, use the event.stopPropagation()
method within the event handler:
$('selector').on('event', function(event) {
event.stopPropagation();
// Additional code
});
This prevents the event from bubbling up to parent elements.
13. What is the difference between .on()
and .bind()
methods in jQuery?
.bind()
: Attaches an event handler directly to elements; does not work for dynamically added elements..on()
: A versatile method that attaches event handlers and supports event delegation, making it suitable for both existing and future elements.
Due to its flexibility, .on()
is preferred over .bind()
.
14. How do you perform form validation using jQuery?
Form validation using jQuery involves attaching event handlers to form elements and checking input values before submission. This ensures user inputs meet specific criteria.
Example:
$('#form').on('submit', function(event) {
if ($('#input').val().trim() === '') {
event.preventDefault();
alert('Input cannot be empty');
}
});
Explanation:
- Event Handling: The
submit
event is bound to the form. - Validation: Checks if the input field is empty.
- Prevent Submission: Uses
event.preventDefault()
to stop form submission. - User Feedback: Alerts the user if input is invalid.
For multiple fields, you can add regex checks, real-time validation, and display error messages next to each field for a better user experience.
15. How can you animate elements using jQuery?
jQuery provides the .animate()
method to create custom animations by changing CSS properties over time.
Example:
$('#element').animate({
opacity: 0.5,
height: 'toggle'
}, 1000, function() {
// Animation complete callback
});
In this example, the #element
‘s opacity is reduced to 0.5, and its height is toggled over 1000 milliseconds. The optional callback function executes after the animation completes.
16. What is the purpose of the $.noConflict()
method in jQuery?
The $.noConflict()
method releases control of the $
identifier, preventing conflicts with other libraries that use $
as a shortcut.
Example:
$.noConflict();
// Now, use jQuery instead of $
jQuery(document).ready(function() {
jQuery('#element').hide();
});
This approach ensures compatibility when multiple libraries are used on the same page.
17. How do you get and set the value of form elements using jQuery?
Get Value: Use the .val()
method without arguments:
var value = $('#inputField').val();
Set Value: Pass the new value as an argument to .val()
:
$('#inputField').val('New Value');
The .val()
method is versatile for handling form input values.
18. How can you load content from a server and place it into an element using jQuery?
Use the .load()
method to fetch content from the server and insert it into a selected element:
$('#result').load('content.html', function(response, status, xhr) {
if (status == 'error') {
$('#result').html('An error occurred: ' + xhr.status + ' ' + xhr.statusText);
}
});
This method simplifies AJAX calls for loading HTML content.
19. What is the difference between .prop()
and .attr()
methods in jQuery?
.attr()
: Retrieves or sets attributes of elements, reflecting the initial HTML value..prop()
: Retrieves or sets properties of elements, reflecting the current state.
Example:
// Using .attr()
var href = $('#link').attr('href');
// Using .prop()
var checked = $('#checkbox').prop('checked');
Understanding the distinction is crucial for accurate manipulation of element attributes and properties.
20. How do you traverse the DOM using jQuery?
jQuery provides various methods to navigate the DOM:
- Parent Elements:
.parent()
,.parents()
,.closest()
- Child Elements:
.children()
,.find()
- Siblings:
.siblings()
,.next()
,.prev()
Example:
// Find the parent of #element
$('#element').parent();
// Find all children with class .child
$('#element').children('.child');
// Find the next sibling
$('#element').next();
These methods enable efficient traversal and manipulation of the DOM structure.
21. How can you check if an element exists in the DOM using jQuery?
To determine if an element exists in the DOM, you can use the .length
property of a jQuery selector. If the length is greater than zero, the element exists.
if ($('#elementID').length) {
// Element exists
} else {
// Element does not exist
}
22. What is the difference between .fadeIn()
and .fadeTo()
methods in jQuery?
.fadeIn()
: Gradually changes the opacity of the selected elements from hidden to visible..fadeTo()
: Animates the opacity of the selected elements to a specified value.
Example of .fadeTo()
:
$('#element').fadeTo(1000, 0.5); // Fades the element to 50% opacity over 1 second
23. How can you stop an animation or effect in jQuery?
Use the .stop()
method to stop the currently running animation on the selected elements.
$('#element').stop();
You can also pass parameters to .stop()
to control whether the current animation completes and whether queued animations are executed.
24. How do you get the parent element of a selected element in jQuery?
Use the .parent()
method to retrieve the immediate parent of the selected element.
$('#childElement').parent();
To traverse up multiple levels, you can use .parents()
or .closest()
.
25. What is the purpose of the .serialize()
method in jQuery?
The .serialize()
method creates a URL-encoded string representation of form data, which is useful for submitting form data via AJAX.
var formData = $('#form').serialize();
This string can then be sent to the server using an AJAX request.
26. How can you bind multiple event handlers to a single element in jQuery?
You can bind multiple event handlers to a single element by chaining .on()
methods or by passing multiple events as a space-separated string.
$('#element').on('click', function() {
// Click handler
}).on('mouseover', function() {
// Mouseover handler
});
// Or using a space-separated string
$('#element').on('click mouseover', function(event) {
if (event.type === 'click') {
// Click handler
} else if (event.type === 'mouseover') {
// Mouseover handler
}
});
27. How do you remove an attribute from an HTML element using jQuery?
Use the .removeAttr()
method to remove an attribute from the selected element.
$('#element').removeAttr('disabled');
This will remove the ‘disabled’ attribute from the element.
28. How can you get the index of an element within its parent using jQuery?
Use the .index()
method to get the zero-based index of an element relative to its siblings.
var index = $('#element').index();
This returns the position of #element
among its sibling elements.
29. What is the difference between .slideUp()
and .slideDown()
methods in jQuery?
.slideUp()
: Animates the height of the selected elements to zero, effectively hiding them with a sliding effect..slideDown()
: Animates the height of the selected elements from zero to their natural height, effectively showing them with a sliding effect.
These methods are useful for creating accordion-style UI components.
30. How do you delegate events to dynamically added elements in jQuery?
Use the .on()
method with event delegation by specifying a parent element and a child selector.
$(document).on('click', '.dynamic-element', function() {
// Event handler code
});
This ensures that the event handler works for both existing and future elements matching the selector.
31. How can you prevent the default action of an event in jQuery?
Within an event handler, use the event.preventDefault()
method to prevent the default action associated with the event.
$('a').on('click', function(event) {
event.preventDefault();
// Additional code
});
This prevents the default behavior of the anchor tag, which is navigation to the href attribute.
32. What is the purpose of the .data()
method in jQuery?
The .data()
method is used to store and retrieve data associated with DOM elements without modifying the DOM structure.
$('#element').data('key', 'value'); // Store data
var value = $('#element').data('key'); // Retrieve data
This is useful for associating arbitrary data with elements.
33. How do you perform asynchronous HTTP requests using jQuery?
Use the $.ajax()
method to perform asynchronous HTTP requests.
$.ajax({
url: 'https://api.example.com/data',
method: 'GET',
success: function(response) {
// Handle success
},
error: function(error) {
// Handle error
}
});
jQuery also provides shorthand methods like $.get()
and $.post()
for common HTTP request types.
34. How can you load JSON data using jQuery?
Use the $.getJSON()
method to load JSON data from the server.
$.getJSON('https://api.example.com/data.json', function(data) {
// Process JSON data
});
This method simplifies the process of fetching JSON-formatted data.
35. What is the difference between .children()
and .find()
methods in jQuery?
.children()
: This method retrieves all direct child elements of the selected element. It traverses only a single level down the DOM tree. For example, $('ul').children('li')
will select all <li>
elements that are immediate children of the <ul>
element.
.find()
: This method searches through all descendant elements of the selected element, at all levels down the DOM tree. For instance, $('ul').find('li')
will select all <li>
elements that are descendants of the <ul>
, including those nested within other child elements.
In summary, use .children()
when you need to select only the immediate children of an element, and use .find()
when you need to select all descendants, regardless of their depth in the DOM hierarchy.
36. How can you get the text content of an element using jQuery?
To retrieve the text content of an element, use the .text()
method without any arguments:
var text = $('#element').text();
This will return the combined text contents of the selected element and its descendants.
37. How do you set the HTML content of an element using jQuery?
To set the HTML content of an element, use the .html()
method with the desired HTML string as an argument:
$('#element').html('<p>New content</p>');
This will replace the existing content of #element
with the new HTML.
38. What is the difference between .eq()
and .get()
methods in jQuery?
.eq()
: Returns a jQuery object containing the element at the specified zero-based index within the matched set.
var element = $('div').eq(2); // Gets the third div as a jQuery object
.get()
: Returns the DOM element at the specified index within the matched set. If no index is provided, it returns all matched elements as an array of DOM elements.
var element = $('div').get(2); // Gets the third div as a DOM element
In summary, .eq()
returns a jQuery object, allowing for further jQuery method chaining, while .get()
returns the raw DOM element.
39. How can you clone an element along with its event handlers using jQuery?
Use the .clone()
method with the true
argument to clone an element along with its data and event handlers:
var clonedElement = $('#element').clone(true);
This creates a deep copy of #element
, including its event handlers.
40. How do you bind an event handler that executes only once using jQuery?
Use the .one()
method to attach an event handler that executes only once for each element:
$('#element').one('click', function() {
// Handler code
});
After the first click, the handler is automatically removed.
41. How can you check if a checkbox is checked using jQuery?
To check if a checkbox is checked, use the .prop()
method:
if ($('#checkbox').prop('checked')) {
// Checkbox is checked
}
Alternatively, you can use the :checked
selector:
if ($('#checkbox:checked').length) {
// Checkbox is checked
}
42. How do you disable or enable an input element using jQuery?
To disable an input element:
$('#input').prop('disabled', true);
To enable it:
$('#input').prop('disabled', false);
The .prop()
method is used to set the disabled
property of the input element.
43. How can you get the width and height of an element using jQuery?
Use the .width()
and .height()
methods to get the width and height of an element, respectively:
var width = $('#element').width();
var height = $('#element').height();
These methods return the computed width and height of the element, excluding padding, border, and margin.
44. How do you get the position of an element relative to the document using jQuery?
Use the .offset()
method to get the position of an element relative to the document:
var position = $('#element').offset();
var top = position.top;
var left = position.left;
The .offset()
method returns an object containing the top
and left
coordinates.
45. How can you hide an element and remove it from the DOM using jQuery?
To hide an element without removing it from the DOM, use the .hide()
method:
$('#element').hide();
To remove an element from the DOM, use the .remove()
method:
$('#element').remove();
The .hide()
method sets the element’s display property to none
, while .remove()
deletes the element from the DOM entirely.
46. How do you attach multiple event handlers to the same element for the same event in jQuery?
You can attach multiple event handlers to the same element for the same event using the .on()
method:
$('#element').on('click', handler1);
$('#element').on('click', handler2);
Both handler1
and handler2
will be executed when the element is clicked.
47. How can you trigger an event programmatically using jQuery?
To programmatically trigger an event in jQuery, you can use the .trigger()
method. This method activates the event handlers attached to the selected elements for a specified event type, effectively simulating a user-initiated event.
Example:
$('#element').trigger('click');
In this example, the click event is triggered on the element with the ID element
, causing any click event handlers attached to it to execute as if the element had been clicked by the user.
The .trigger()
method can also pass additional parameters to the event handler:
$('#element').trigger('customEvent', [param1, param2]);
Here, customEvent
is a user-defined event, and [param1, param2]
are additional parameters passed to the event handler.
It’s important to note that while .trigger()
simulates the activation of an event, it may not perfectly replicate a naturally occurring event. For instance, triggering a click event on a link using .trigger('click')
will execute the jQuery event handlers but may not follow the link and change the current page.
For scenarios where you need to trigger an event without causing the default behavior (such as form submission), you can use the .triggerHandler()
method. This method is similar to .trigger()
but does not trigger the default behavior of the event and only affects the first matched element.
By utilizing these methods, you can effectively simulate user interactions and control event handling programmatically in your jQuery applications.
48. How can you prevent event propagation in jQuery?
In jQuery, you can prevent event propagation by using the event.stopPropagation()
method within your event handler. This method stops the event from bubbling up the DOM tree, preventing parent elements from receiving the event.
Example:
$('#childElement').on('click', function(event) {
event.stopPropagation();
// Additional code
});
In this example, when #childElement
is clicked, the click event will not propagate to its parent elements.
Additionally, to prevent the default action associated with the event (such as following a link), you can use event.preventDefault()
.
49. How do you check if an element is visible in the viewport using jQuery?
To determine if an element is visible within the viewport, you can compare the element’s position to the viewport’s dimensions. jQuery doesn’t provide a built-in method for this, but you can achieve it using the following approach:
Example:
function isElementInViewport(el) {
var rect = el[0].getBoundingClientRect();
return (
rect.top >= 0 &&
rect.left >= 0 &&
rect.bottom <= (window.innerHeight || document.documentElement.clientHeight) &&
rect.right <= (window.innerWidth || document.documentElement.clientWidth)
);
}
if (isElementInViewport($('#element'))) {
// Element is in the viewport
}
This function checks if the element’s bounding rectangle is within the viewport’s boundaries.
50. How can you debounce a function in jQuery to limit its execution rate?
Debouncing ensures that a function is not called too frequently. jQuery doesn’t have a built-in debounce method, but you can implement it using JavaScript:
Example:
function debounce(func, wait) {
var timeout;
return function() {
var context = this, args = arguments;
clearTimeout(timeout);
timeout = setTimeout(function() {
func.apply(context, args);
}, wait);
};
}
// Usage
$(window).on('resize', debounce(function() {
// Resize event handler code
}, 250));
In this example, the resize event handler is debounced to execute at most once every 250 milliseconds.
Learn More: Carrer Guidance | Hiring Now!
Top 40 Network Security Interview Questions and Answers
REST Assured Interview Questions and Answers
Top Android Developer Interview Q&A for 5+ Years Experience
Ansible Interview Questions and Answers
Django Interview Questions and Answers