Are you preparing for a Django interview? Django is a high-level Python web framework that helps in rapid development and clean, pragmatic design. This guide compiles over 30 essential Django interview questions and answers, covering topics like the Model-View-Template (MVT) architecture, URL routing, ORM, and more. Whether you’re an experienced developer or a fresher, this resource will help you hone your skills and ensure you’re well-prepared for any Django-related interview.
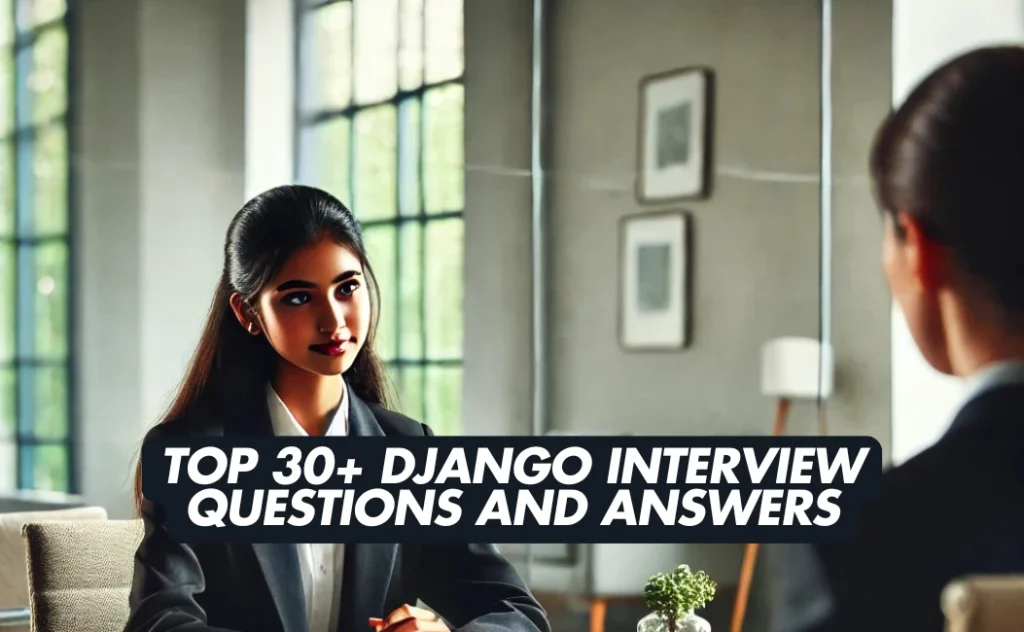
Top 30+ Django Interview Questions and Answers
- What is Django, and why is it used in web development?
- Explain the Model-View-Template (MVT) architectural pattern in Django.
- How do you create a new Django project?
- What is the purpose of Django apps?
- How do you define models in Django, and what are migrations used for?
- Explain the role of templates in Django.
- How does Django handle URL routing?
- What is the Django admin site, and how do you register a model with it?
- What is a QuerySet in Django, and how is it different from a raw SQL query?
- How does Django handle form validation?
- What is middleware in Django, and how does it work?
- How does Django’s authentication system work?
- What are Django signals, and how are they used?
- How do you implement file uploads in Django?
- What is the purpose of Django’s settings.py file?
- How does Django’s ORM handle relationships between models?
- How do you optimize database queries in Django?
- How does Django’s caching framework work?
- What is the purpose of Django’s manage.py file?
- How do you handle static and media files in Django?
- What is the purpose of Django’s urls.py file?
- How does Django’s ModelForm simplify form handling?
- What are Django’s class-based views (CBVs), and how do they differ from function-based views (FBVs)?
- How can you implement pagination in Django?
- What is the role of the as_view() method in Django’s class-based views?
- How do you create and use custom template tags and filters in Django?
- What is the purpose of Django’s sites framework?
- How does Django support internationalization and localization?
- What are Django’s generic views, and how do they simplify development?
- How do you implement user authorization and permissions in Django?
- How does Django handle security, and what measures are in place to protect against common web vulnerabilities?
- What is the Django Rest Framework (DRF)?
- How do you create a custom database backend in Django?
1. What is Django, and why is it used in web development?
Django is a high-level, open-source web framework written in Python that promotes rapid development and clean, pragmatic design. It follows the “batteries-included” philosophy, providing a wide array of built-in features such as an ORM (Object-Relational Mapping), authentication mechanisms, and an administrative interface. Django is used in web development to streamline the process of building robust, scalable, and secure web applications, allowing developers to focus on writing application-specific code without reinventing common functionalities.
2. Explain the Model-View-Template (MVT) architectural pattern in Django.
Django’s architecture is based on the Model-View-Template (MVT) pattern:
- Model: Defines the data structure and database schema, serving as an interface for data management.
- View: Handles the business logic and processes user requests, interacting with the model to fetch data and rendering the appropriate template.
- Template: Manages the presentation layer, defining the HTML structure and dynamically displaying data provided by the view.
This separation of concerns enhances code maintainability and scalability.
3. How do you create a new Django project?
To create a new Django project:
Install Django: Use pip to install Django
pip install Django
Create a Project: Navigate to the desired directory and run:
django-admin startproject projectname
Navigate to Project Directory: Move into the project directory:
cd projectname
Run the Development Server: Start the server to verify the setup:
python manage.py runserver
This will set up the initial project structure with necessary configurations.
4. What is the purpose of Django apps?
In Django, an app is a self-contained module that encapsulates related functionality for a specific aspect of a project. This modular approach promotes code reusability and maintainability, allowing developers to plug in or reuse apps across different projects. Each app manages its own models, views, templates, and static files, facilitating organized and scalable development.
5. How do you define models in Django, and what are migrations used for?
In Django, models are defined as Python classes that represent the structure of database tables and the relationships between them. Each attribute of the class corresponds to a database field. For example:
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=100)
author = models.CharField(max_length=100)
published_date = models.DateField()
Migrations are used to propagate changes made to models (such as adding or modifying fields) into the database schema. They are created using:
python manage.py makemigrations
And applied using:
python manage.py migrate
This ensures that the database schema stays in sync with the current state of the models.
6. Explain the role of templates in Django.
Templates in Django are used to define the structure and layout of the HTML pages that are presented to the user. They allow developers to separate the presentation layer from the business logic by embedding dynamic content within static HTML. Django’s templating engine facilitates this by using template tags and filters to render dynamic data, enabling a clear distinction between design and functionality.
7. How does Django handle URL routing?
Django uses a URL dispatcher to map URL patterns to the appropriate view functions. This is managed through the urls.py
file, where developers define URL patterns using the path()
function. For example:
from django.urls import path
from . import views
urlpatterns = [
path('home/', views.home, name='home'),
path('about/', views.about, name='about'),
]
This setup directs incoming requests to the corresponding view based on the URL pattern, facilitating clean and organized URL management.
8. What is the Django admin site, and how do you register a model with it?
The Django admin site is a built-in interface that allows developers to manage and interact with the application’s data models. It provides a user-friendly platform for performing CRUD (Create, Read, Update, Delete) operations without the need to create custom administrative interfaces. To register a model with the admin site, add the following to the admin.py
file of your app:
from django.contrib import admin
from .models import Book
admin.site.register(Book)
This makes the Book
model accessible and manageable through the admin interface.
9. What is a QuerySet in Django, and how is it different from a raw SQL query?
QuerySets are Django’s way to retrieve data from the database in an efficient and convenient manner. They allow developers to filter, order, and manipulate data without writing raw SQL queries. For example:
# Retrieve all books authored by 'John Doe'
books = Book.objects.filter(author='John Doe')
This QuerySet fetches all Book
instances where the author is ‘John Doe’.
Differences between QuerySets and raw SQL queries:
- Abstraction: QuerySets provide a high-level, Pythonic interface to interact with the database, abstracting the underlying SQL. Raw SQL requires manual crafting of queries, which can be more error-prone.
- Database Independence: QuerySets are database-agnostic, meaning the same code can work across different database backends. Raw SQL is often specific to a particular database dialect, reducing portability.
- Security: QuerySets automatically handle parameter escaping, protecting against SQL injection attacks. When using raw SQL, developers must manually ensure proper escaping and parameterization to maintain security.
- Maintainability: Code written with QuerySets is generally more readable and maintainable. Raw SQL can become complex and harder to manage, especially as the application grows.
While QuerySets cover most use cases, there are scenarios where raw SQL is necessary, such as when leveraging database-specific features or optimizing complex queries. Django provides the raw()
method and direct cursor operations for executing raw SQL when needed.
10. How does Django handle form validation?
Django provides a robust form handling and validation framework through its forms
module. Developers can define forms as Python classes, specifying the fields and their respective validation criteria. When a form is submitted, Django automatically validates the input data against the defined rules. If the data is valid, it can be processed (e.g., saved to the database); if not, Django generates appropriate error messages for each invalid field. This framework ensures that user input is consistently validated, enhancing the security and reliability of web applications.
11. What is middleware in Django, and how does it work?
Middleware in Django is a series of hooks into the request/response processing. It’s a lightweight, low-level plugin system for globally altering Django’s input or output. Each middleware component is a Python class that processes requests and responses. Middleware can be used for various purposes, such as handling sessions, user authentication, cross-site request forgery protection, and more. They are executed in a specific order, as defined in the MIDDLEWARE
setting, allowing developers to customize the processing of requests and responses globally.
12. How does Django’s authentication system work?
Django’s authentication system manages user accounts, groups, permissions, and cookie-based user sessions. It provides built-in views and forms for handling login, logout, and password management. The system uses middleware to associate users with requests, allowing developers to access the currently logged-in user via request.user
. Permissions and groups can be assigned to users to control access to different parts of the application, facilitating robust user management and authentication mechanisms.
13. What are Django signals, and how are they used?
Django signals are a way to allow decoupled applications to get notified when certain events occur elsewhere in the application. They enable a “listener” pattern, where certain senders notify a set of receivers when specific actions have taken place. For example, the post_save
signal can be used to execute some code right after a model instance is saved. Developers can connect receiver functions to signals to perform actions like updating related models, sending notifications, or logging activities, promoting a clean and decoupled codebase.
14. How do you implement file uploads in Django?
Django simplifies file uploads by providing a FileField
or ImageField
in models to handle file storage. Developers need to ensure that the MEDIA_URL
and MEDIA_ROOT
settings are properly configured to define the URL and filesystem location where uploaded files will be stored. In forms, the enctype="multipart/form-data"
attribute must be set to handle file data. Django takes care of saving the uploaded files to the designated location and provides mechanisms to access and manage these files efficiently.
15. What is the purpose of Django’s settings.py
file?
The settings.py
file in a Django project contains all the configuration for the application. This includes database settings, installed applications, middleware, template configurations, static and media file settings, security options, and more. It serves as the central place to manage the project’s settings, allowing developers to customize and control the behavior of the Django application across different environments.
16. How does Django’s ORM handle relationships between models?
Django’s ORM provides several field types to define relationships between models:
- ForeignKey: Defines a many-to-one relationship.
- OneToOneField: Defines a one-to-one relationship.
- ManyToManyField: Defines a many-to-many relationship.
These fields enable developers to establish and navigate relationships between different models easily. Django also provides related managers and querysets to access related objects, facilitating complex queries and data manipulations involving multiple related models.
17. How do you optimize database queries in Django?
Optimizing database queries in Django is crucial for enhancing application performance. Here are several strategies to achieve this:
1. Use select_related
and prefetch_related
: These methods help in fetching related objects efficiently.
select_related
: Performs a single SQL query with a JOIN to retrieve related objects forForeignKey
andOneToOneField
relationships.
# Retrieves books along with their authors in a single query
books = Book.objects.select_related('author').all()
prefetch_related
: Executes separate queries and does the joining in Python, suitable forManyToManyField
and complex relationships.
# Retrieves books and their related genres efficiently
books = Book.objects.prefetch_related('genres').all()
- Avoid the N+1 Query Problem: This occurs when a separate database query is executed for each related object. Using
select_related
orprefetch_related
can mitigate this issue by reducing the number of queries.
- Utilize Database Indexing: Adding indexes to frequently queried fields can significantly speed up data retrieval. In Django models, you can specify indexes using the
Meta
class:
class Book(models.Model):
title = models.CharField(max_length=100)
author = models.ForeignKey(Author, on_delete=models.CASCADE)
class Meta:
indexes = [
models.Index(fields=['title']),
]
However, be cautious, as excessive indexing can slow down write operations.
- Retrieve Only Necessary Fields: Use
only()
ordefer()
to load only the fields you need, reducing the amount of data fetched:
# Retrieves only the 'title' and 'author' fields
books = Book.objects.only('title', 'author')
- Use
values()
orvalues_list()
for Specific Fields: These methods return dictionaries or tuples of the specified fields, which can be more efficient:
# Retrieves a list of book titles
titles = Book.objects.values_list('title', flat=True)
- Leverage QuerySet Caching: QuerySets are lazy and can be cached to avoid redundant database hits:
# Cache the queryset to reuse without additional database queries
books = Book.objects.all()
list_of_books = list(books) # Evaluates the queryset
- Perform Bulk Operations: For multiple inserts or updates, use bulk methods to minimize database hits:
# Bulk create books
Book.objects.bulk_create([
Book(title='Book 1', author=author1),
Book(title='Book 2', author=author2),
])
- Avoid Using
count()
on Large QuerySets: Instead, useexists()
to check for the presence of records without counting all entries:
# Checks if any books exist without counting them
if Book.objects.exists():
# Do something
- Profile and Monitor Queries: Utilize tools like Django Debug Toolbar to analyze and optimize query performance.
By implementing these strategies, you can significantly enhance the efficiency and performance of your Django application’s database interactions.
18. How does Django’s caching framework work?
Django’s caching framework provides a way to store the results of expensive computations so that they can be reused, reducing the need for repeated processing and database queries. It supports various caching backends, including in-memory caches like Memcached and Redis, as well as file-based and database caches. The framework allows for caching at different levels:
- Per-View Caching: Caches the output of entire views.
- Template Fragment Caching: Caches specific parts of templates.
- Low-Level Caching: Allows manual caching of arbitrary data.
Configuration is managed through Django’s settings, where you define the cache backend and related options. By effectively utilizing caching, you can significantly improve the performance and scalability of your application.
19. What is the purpose of Django’s manage.py
file?
The manage.py
file is a command-line utility that serves as the entry point for various Django administrative tasks. It allows developers to interact with the Django project in several ways:
Running the Development Server: Start the local server during development.
python manage.py runserver
Applying Migrations: Synchronize the database schema with the current state of models.
python manage.py migrate
Creating Migrations: Generate migration files based on model changes.
python manage.py makemigrations
Creating Superusers: Create administrative users for the Django admin interface.
python manage.py createsuperuser
Running Tests: Execute test cases to ensure code quality.
python manage.py test
The manage.py
file sets the appropriate environment variables and configurations, enabling seamless management of the Django project.
20. How do you handle static and media files in Django?
Managing static and media files in Django involves configuring settings and organizing files to ensure they are correctly served during development and production.
Static Files:
Static files include assets like CSS, JavaScript, and images that are part of your application’s codebase.
1. Configuration:
In your settings.py
, define the following:
STATIC_URL = '/static/'
STATICFILES_DIRS = [BASE_DIR / 'static']
STATIC_ROOT = BASE_DIR / 'staticfiles'
STATIC_URL
: The URL prefix for static files.STATICFILES_DIRS
: Directories where Django will search for additional static files.STATIC_ROOT
: The directory wherecollectstatic
will collect static files for deployment.
Development:
- Django automatically serves static files during development when
DEBUG
is set toTrue
. - Ensure
'django.contrib.staticfiles'
is included inINSTALLED_APPS
.
Production:
- Run
collectstatic
to gather all static files into theSTATIC_ROOT
directory:
python manage.py collectstatic
- Configure your web server (e.g., Nginx, Apache) to serve files from the
STATIC_ROOT
directory.
Media Files:
Media files are user-uploaded content, such as profile pictures or documents.
1. Configuration:
In settings.py
, define:
MEDIA_URL = '/media/'
MEDIA_ROOT = BASE_DIR / 'media'
MEDIA_URL
: The URL prefix for media files.MEDIA_ROOT
: The filesystem path to the directory that will hold user-uploaded files.
2. Development:
- To serve media files during development, add the following to your project’s
urls.py
:
from django.conf import settings
from django.conf.urls.static import static
urlpatterns = [
# ... your url patterns
] + static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
- This setup enables Django to serve media files when
DEBUG
isTrue
.
3. Production:
- It’s recommended to serve media files using a dedicated media server or cloud storage service (e.g., Amazon S3) for better performance and scalability.
- Configure your web server to serve files from the
MEDIA_ROOT
directory or set up your cloud storage to handle media files.
Referencing Files in Templates:
- To include static files in templates, use the
{% static %}
template tag:
{% load static %}
<img src="{% static 'images/logo.png' %}" alt="Logo">
- For media files, store the file path in the model and access it via the model instance:
<img src="{{ user.profile.image.url }}" alt="Profile Picture">
Proper configuration and management of static and media files ensure that your Django application serves these assets efficiently in both development and production environments.
21. What is the purpose of Django’s urls.py
file?
In Django, the urls.py
file is responsible for mapping URLs to their corresponding view functions. This URL configuration allows the framework to direct incoming HTTP requests to the appropriate view based on the request’s URL pattern. By defining URL patterns in urls.py
, developers can create a clean and organized URL structure for their application, enhancing both user experience and SEO.
22. How does Django’s ModelForm
simplify form handling?
Django’s ModelForm
is a helper class that creates a form based on a specific model. It automatically generates form fields that correspond to the model’s fields, streamlining the process of creating forms for model data. This approach reduces boilerplate code and ensures consistency between the form and the model, making it easier to handle form validation and data saving.
23. What are Django’s class-based views (CBVs), and how do they differ from function-based views (FBVs)?
Django’s class-based views (CBVs) provide a way to organize view logic using classes instead of functions. CBVs offer inheritance and mixins, promoting code reuse and modularity. They differ from function-based views (FBVs) in that CBVs encapsulate view behavior within class methods, allowing for more structured and maintainable code, especially in complex applications.
24. How can you implement pagination in Django?
Django provides a built-in Paginator
class to handle pagination. To implement pagination:
- Import the
Paginator
,PageNotAnInteger
, andEmptyPage
classes. - Create a
Paginator
object with the list of items and the number of items per page. - Retrieve the desired page number from the request.
- Use the
page()
method to get the items for that page.
This approach divides large datasets into manageable pages, improving user experience and performance.
25. What is the role of the as_view()
method in Django’s class-based views?
The as_view()
method in Django’s class-based views is a class method that returns a callable view that can be used in URLconf. It serves as the entry point for the view, processing HTTP requests and dispatching them to the appropriate handler methods (e.g., get()
, post()
). This method bridges the gap between the class-based view and Django’s URL routing system.
26. How do you create and use custom template tags and filters in Django?
To create custom template tags and filters in Django:
- Create a
templatetags
directory within your app. - Inside
templatetags
, create a Python file (e.g.,custom_tags.py
). - In this file, import
template
fromdjango
and register your custom tags or filters using the@register
decorator. - Load the custom tags/filters in your template using
{% load custom_tags %}
.
This allows you to extend Django’s templating language with custom functionality tailored to your application’s needs.
27. What is the purpose of Django’s sites
framework?
Django’s sites
framework enables a single Django project to manage multiple websites with different domains. It provides a way to associate content and configurations with specific sites, allowing for shared functionality across multiple sites while maintaining distinct content and settings for each. This is particularly useful for applications serving multiple clients or brands from a single codebase.
28. How does Django support internationalization and localization?
Django supports internationalization (i18n) and localization (l10n) through its built-in translation system. Developers can mark strings for translation using the gettext
function or the {% trans %}
template tag. Django then uses translation files to provide content in different languages. Additionally, Django handles locale-specific formatting of dates, times, numbers, and time zones, enabling applications to cater to a global audience.
29. What are Django’s generic views, and how do they simplify development?
Django’s generic views are pre-built views that handle common patterns, such as displaying a list of objects or retrieving a single object. They simplify development by encapsulating common functionality, allowing developers to focus on customizing behavior rather than writing boilerplate code. By extending or configuring generic views, developers can quickly implement standard features with minimal code.
30. How do you implement user authorization and permissions in Django?
Django provides a permissions framework that allows developers to define what actions a user can perform. Permissions can be set at both the model and object levels. Django’s authentication system includes built-in permissions (add
, change
, delete
, view
) for each model, and developers can create custom permissions as needed. By using decorators like @permission_required
or checking permissions in views, developers can control access to various parts of the application.
31. How does Django handle security, and what measures are in place to protect against common web vulnerabilities?
Django incorporates several built-in security features to safeguard applications against common web vulnerabilities:
- Cross-Site Scripting (XSS) Protection: Django’s templating engine automatically escapes special characters in HTML templates, mitigating XSS attacks by preventing malicious scripts from being executed in the browser.
- Cross-Site Request Forgery (CSRF) Protection: Django includes middleware that generates a unique token for each user session, which must be included in forms and AJAX requests. This ensures that unauthorized commands are not executed on behalf of authenticated users.
- SQL Injection Protection: Django’s ORM uses parameterized queries, which separate SQL code from data inputs, effectively preventing SQL injection attacks.
- Clickjacking Protection: Django provides middleware that sets the
X-Frame-Options
header, preventing the site from being rendered in a frame or iframe, thereby protecting against clickjacking attacks. - Secure Password Storage: Django uses PBKDF2 by default to hash passwords, ensuring that they are stored securely.
- SSL/HTTPS Support: Django supports HTTPS to encrypt data transmitted between the client and server, protecting against eavesdropping and man-in-the-middle attacks.
- Host Header Validation: Django validates the
Host
header against theALLOWED_HOSTS
setting to prevent HTTP Host header attacks.
By leveraging these features and following best practices, developers can build robust and secure web applications with Django.
32. What is the Django Rest Framework (DRF)?
The Django Rest Framework (DRF) is a powerful and flexible toolkit for building Web APIs in Django. It provides features like serialization, authentication, and viewsets, making it easier to develop RESTful APIs. DRF supports various authentication methods, including token-based and OAuth, and offers a browsable API interface for testing and development. Its modular and customizable architecture allows developers to build robust APIs efficiently.
33. How do you create a custom database backend in Django?
Creating a custom database backend in Django involves:
- Implementing Required Classes: Create classes for database features, such as
DatabaseWrapper
,DatabaseFeatures
,DatabaseOperations
, andDatabaseClient
. - Defining Database Settings: Specify the custom backend in the
DATABASES
setting insettings.py
. - Handling Database Operations: Implement methods to handle database connections, queries, and transactions.
This approach allows Django to interact with non-standard or proprietary databases by extending its database backend capabilities.
Learn More: Carrer Guidance | Hiring Now!
Top 50 AWS Lambda Interview Questions and Answers
Low Level Design (LLD) Interview Questions and Answers
SQL Interview Questions for 3 Years Experience with Answers
Advanced TOSCA Test Automation Engineer Interview Questions and Answers with 5+ Years of Experience
DSA Interview Questions and Answers
Angular Interview Questions and Answers for Developers with 5 Years of Experience
Android Interview Questions for Senior Developer with Detailed Answers