In software development, many developers rush into choosing technologies—like PostgreSQL, MongoDB, or Object-Relational Mappers (ORMs) such as Hibernate or Sequelize—without first establishing a solid data modeling foundation. Skipping this foundational step often leads to hidden data inconsistencies, business logic errors, and security gaps.
Entity Relationship Diagrams (ERDs) help prevent these problems. They provide a visual roadmap of how your data fits together, ensuring your database structure aligns with your application’s needs before you write any code or commit to a specific database technology.
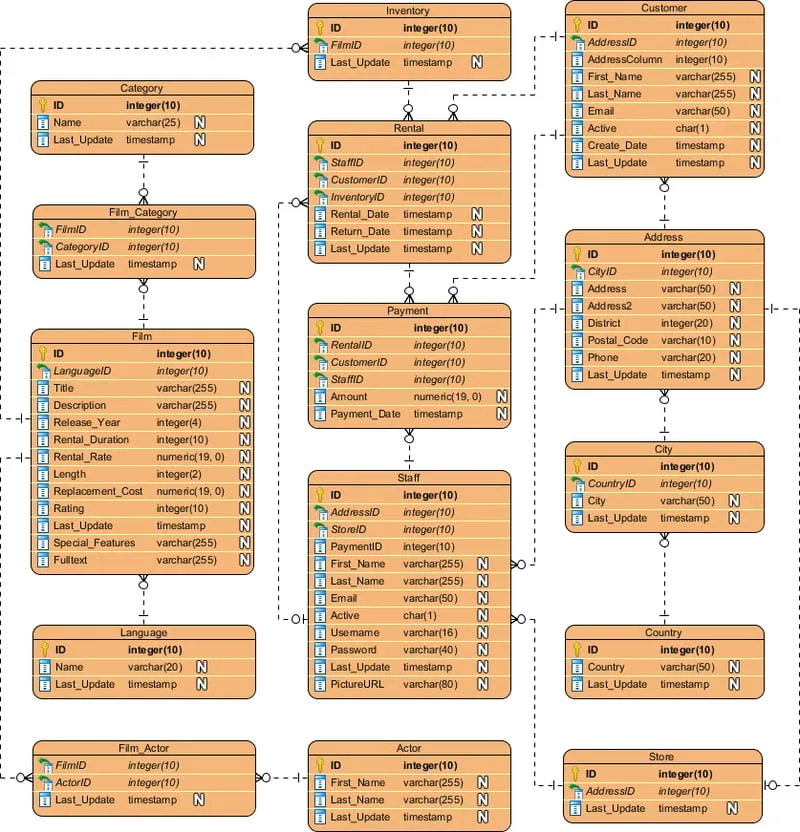
What Are Entity Relationship Diagrams (ERDs)?
An ERD is essentially a flowchart that represents how data is structured within your system. It clarifies:
- Entities: Think of these as “things” or “objects.” In an e-commerce platform, entities might be Users, Products, and Orders.
- Attributes: Each entity has attributes (or fields) that describe it. For a User, you might have
user_id
,email
,name
. For a Product, attributes could includeproduct_id
,name
, andprice
. - Relationships: These show how entities are connected. Does one User have many Orders? Does one Order contain many Products? By making relationships explicit, ERDs highlight data dependencies and constraints early on.
Key Benefit: An ERD is like a high-level blueprint. Just as architects wouldn’t build a house without blueprints, developers shouldn’t design a database without visualizing relationships and constraints first.
How ERDs Reduce Bugs in Business Logic
1. Clear Alignment with Business Rules:
If your business rule says every Order must belong to a User, your ERD makes that rule explicit. Without this visual step, you risk designing tables or ORM models that allow invalid or “orphaned” orders.
Example: In an online store, your ERD shows a one-to-many relationship from User to Order. This ensures no order record exists without a valid user reference, minimizing data errors later.
2. Stronger Data Integrity:
By defining relationships and constraints early, ERDs help you avoid problems like duplicate entries or referencing non-existent records. This leads to cleaner queries, simpler debugging, and fewer runtime surprises.
Example: A SaaS platform’s ERD might link Company
→ User
→ UserPermissions
. By visualizing this chain, you confirm that no user can have invalid permissions, reinforcing both data integrity and security.
Why ERDs Help Secure Client Data
1. Ensures Proper Data Segmentation
Sensitive information—like payment details, social security numbers, or medical records—should be isolated and carefully controlled. An ERD helps you identify which attributes need special handling and how to partition data into separate entities.
Real-Time Example – Healthcare Management System:
- Entities:
Patient
,Doctor
,Appointment
,MedicalRecord
- The
MedicalRecord
table should link to aPatient
securely. Appointment
linksPatient
andDoctor
, ensuring only the assigned doctor can see or modify that patient’s data.
With an ERD, you clearly see the chain of relationships. If a Doctor
wants to view a MedicalRecord
, your application logic can check the Appointment
table to confirm that the doctor is authorized. If you hadn’t designed these relationships properly, you might have a scenario where a doctor or even a malicious user can view data they shouldn’t see.
2. Supports Auditability
Audits and compliance checks (HIPAA, GDPR, etc.) rely on a clear understanding of data flow. With an ERD, you can prove where every piece of data originates and how it is connected.
- Without ERD: Records might be loosely linked, making it hard to trace unauthorized edits or deletions.
- With ERD: Foreign key constraints and defined relationships ensure that any unexpected deletion or modification triggers a visible integrity error or at least a clear audit trail. Auditors can read the ERD and understand exactly how data moves through the system.
Long-Term Benefits of ERDs
Fewer Runtime Errors: By exposing logical issues before coding, ERDs help you avoid complex debugging sessions.
Streamlined Business Logic: With constraints clear from the start, implementing business rules is straightforward.
Enhanced Security and Scalability: As your application grows, adding new entities or features is easier if your foundational ERD is solid. Also, well-defined relationships reduce the “attack surface” for malicious actors.
Real-World Example: Social Media Application
Context: Imagine building a social media platform like Twitter or Instagram.
- Entities:
User
,Post
,Comment
,Like
- Relationships:
- A
User
can create manyPosts
. - A
Post
can have manyComments
and manyLikes
. - A
Comment
must reference both aUser
(who wrote it) and aPost
(it’s attached to). - A
Like
references both aUser
and aPost
.
- A
Without ERD: You might start coding with an ORM and forget to enforce that Comment
needs both user_id
and post_id
. Maybe you allow Comment.post_id
to be null. Eventually, you’ll have comments floating around with no associated post, causing confusion in the UI and skewed engagement metrics. Fixing this later requires data cleanup and potentially massive code refactoring.
With ERD: In your diagram, you connect Comment
to both User
and Post
with foreign keys. You mark them as NOT NULL
to ensure every comment is always tied to a valid user and post. Before writing queries, you realize: “If a User
is deleted, what happens to their Posts
, Comments
, and Likes
?”
The ERD encourages you to plan for cascading deletes or other cleanup strategies. By resolving these questions early, you prevent broken references and keep your platform’s data consistent and trustworthy.
Getting Started with ERDs
Learn Basic Concepts:
- Primary Key: A unique identifier for an entity’s records.
- Foreign Key: A reference to a primary key in another table, defining relationships.
- Normalization: Structuring data to reduce redundancy and improve integrity.
Use Visualization Tools: Tools like Lucidchart, Draw.io, or dbdiagram.io help you create and refine your ERDs quickly. Many can even generate database schema code from your diagrams.
Apply to Every Project First: Even a small ERD helps you think more clearly about the data. As your requirements change, you can update the ERD easily before adjusting code, saving time and preventing errors.
Conclusion
Before committing to a particular database or ORM, invest time in creating an ERD. By visually mapping out entities, attributes, and relationships, you build a stronger foundation for your entire project. This approach:
- Reduces unexpected bugs and rework.
- Ensures alignment with business logic.
- Enhances data security and compliance.
- Makes scaling and feature additions smoother over time.
Start your next project by sketching out an ERD. The time spent planning upfront pays dividends in fewer headaches, cleaner code, and a more secure, maintainable application down the road.