Low Level Design (LLD) interviews are critical in evaluating a candidate’s ability to translate high-level system requirements into detailed and well-structured designs. These interviews focus on the candidate’s understanding of class structures, interactions, design patterns, and their ability to create scalable and maintainable systems. This guide offers 20+ common LLD interview questions with detailed answers to help you prepare effectively.
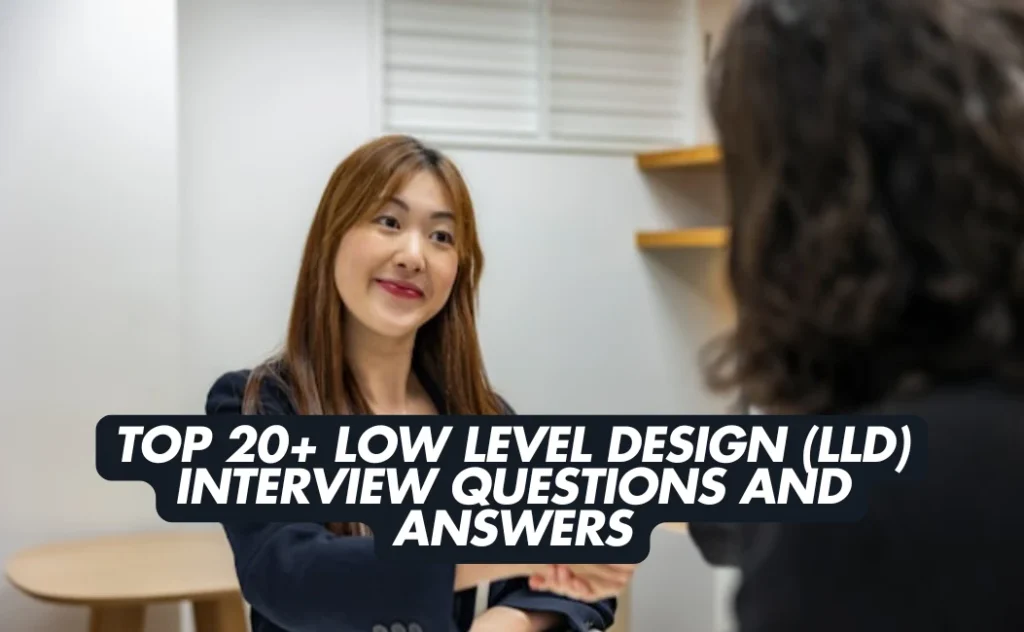
Top 20+ Low Level Design (LLD) Interview Questions and Answers
- Design a Parking Lot System
- Design a Chat Application
- Design a Search Autocomplete System
- Design a Video Streaming Service
- Design a Messaging Queue System
- Design a Banking System
- Design a Ticket Booking System
- Design a Blogging Platform
- Design a Real-time Collaboration Tool
- Design a Cryptocurrency Exchange
- Design a Content Management System (CMS)
- Implement a URL Shortener
- Design a Library Management System
- Design an E-commerce Shopping Cart
- Design a Rate Limiter
- Design a Cache System
- Design a Notification System
- Design a Ride-Sharing Service
- Design a Hotel Booking System
- Design a Food Delivery System
- Design a Social Media Feed
- Design a File Storage System
- Design a Hotel Management System
1. Design a Parking Lot System
Answer: To design a parking lot system, identify the primary entities: ParkingLot, ParkingSpace, Vehicle, and Ticket.
- Classes:
- ParkingLot: Manages multiple ParkingSpaces, tracks available spaces, and issues Tickets.
- ParkingSpace: Represents individual parking spots, each with a unique identifier and size (e.g., small, medium, large).
- Vehicle: Abstract class with subclasses like Car, Bike, and Truck, each having attributes such as licensePlate and size.
- Ticket: Records entry time, assigned ParkingSpace, and associated Vehicle.
- Methods:
- ParkingLot:
assignParkingSpace(vehicle: Vehicle): Ticket
releaseParkingSpace(ticket: Ticket): void
- ParkingSpace:
isAvailable(): boolean
assignVehicle(vehicle: Vehicle): void
removeVehicle(): void
- Vehicle: Getters for vehicle attributes.
- Ticket: Getters for ticket details.
- ParkingLot:
This design ensures scalability and efficient management of parking operations.
2. Design a Chat Application
Answer: A chat application allows users to send messages in real-time. It can support one-on-one chats, group chats, multimedia sharing, and more. Key considerations include scalability, real-time communication, message persistence, and security.
Components:
- Client Application:
- Interface for users to send and receive messages.
- Handles user authentication and message display.
- API Gateway:
- Routes requests to appropriate services.
- Handles authentication and rate limiting.
- Authentication Service: Manages user sign-up, login, and authentication tokens.
- Message Service:
- Handles sending and receiving messages.
- Utilizes WebSockets for real-time communication.
- Chat Service: Manages chat rooms, participants, and permissions.
- Database:
- Stores user data, chat histories, and media files.
- Could use NoSQL databases like MongoDB for flexibility.
- Notification Service: Sends push notifications for new messages.
- Media Storage:
- Stores images, videos, and other media shared in chats.
- Uses services like AWS S3.
Data Flow:
- User Authentication:
- User logs in through the client.
- Authentication service verifies credentials and issues a token.
- Establishing Connection: Client establishes a WebSocket connection for real-time messaging.
- Sending Messages:
- User sends a message via the client.
- Message service receives the message and stores it in the database.
- Message is pushed to recipients through WebSocket connections.
- Receiving Messages:
- Recipients receive the message in real-time.
- Notification service alerts users if they are offline.
Scalability Considerations:
- Load Balancing: Distribute traffic across multiple servers to handle high loads.
- Database Sharding: Split the database into smaller, more manageable pieces.
- Message Queues: Use message brokers like Kafka or RabbitMQ to handle asynchronous processing.
- CDN for Media: Use Content Delivery Networks to serve media files efficiently.
Security Considerations:
- Encryption:
- Encrypt messages in transit using TLS.
- Optionally, use end-to-end encryption for added security.
- Authentication & Authorization: Ensure only authorized users can access chats.
- Data Privacy: Comply with data protection regulations like GDPR.
Technologies:
- Frontend: React, Flutter, or native mobile frameworks.
- Backend: Node.js, Java, or Python with frameworks like Express, Spring, or Django.
- Real-time Communication: WebSockets, Socket.io.
- Database: MongoDB, Cassandra, or PostgreSQL.
- Message Broker: Kafka, RabbitMQ.
- Storage: AWS S3, Google Cloud Storage.
03. Design a Search Autocomplete System
Answer: An autocomplete system suggests possible completions for a user’s query as they type. It enhances user experience by speeding up the search process and guiding users towards popular or relevant queries.
Components:
- Frontend Interface: Captures user input and displays suggestions in real-time.
- API Gateway: Receives autocomplete requests and routes them to the appropriate service.
- Autocomplete Service: Processes input and retrieves relevant suggestions.
- Search Index: Stores searchable terms in a manner optimized for quick retrieval.
- Analytics Service: Tracks user interactions to improve suggestion relevance.
- Caching Layer: Stores frequently accessed suggestions to reduce latency.
Data Flow:
- User Input: User starts typing in the search bar.
- Request Handling: Frontend sends the current input to the API Gateway.
- Fetching Suggestions:
- Autocomplete Service queries the Search Index for matching terms.
- Utilizes caching to quickly return popular suggestions.
- Returning Results: Suggestions are sent back to the frontend for display.
- Learning from Interactions: Analytics Service logs which suggestions are selected to refine future results.
Key Features:
- Real-time Suggestions: Provide instant feedback as the user types.
- Relevance Ranking: Order suggestions based on popularity, personalization, or relevance.
- Handling Typos: Implement fuzzy matching to account for user typos.
- Personalization: Tailor suggestions based on user history and preferences.
Scalability Considerations:
- Distributed Search Index: Use scalable search engines like Elasticsearch or Solr.
- Caching: Implement distributed caches like Redis or Memcached to handle high read loads.
- Load Balancing: Distribute requests across multiple instances of the Autocomplete Service.
Performance Optimization:
- Prefetching: Anticipate user queries based on typing patterns.
- Efficient Data Structures: Use tries or prefix trees in the search index for fast lookup.
- Asynchronous Processing: Handle suggestion generation without blocking user interactions.
Security Considerations:
- Input Validation: Sanitize user input to prevent injection attacks.
- Rate Limiting: Prevent abuse by limiting the number of requests per user.
Technologies:
- Frontend: JavaScript frameworks like React or Angular.
- Backend: Node.js, Python, or Java.
- Search Engine: Elasticsearch, Apache Solr.
- Caching: Redis, Memcached.
- Data Storage: NoSQL databases for flexibility.
04. Design a Video Streaming Service
Answer: A video streaming service allows users to watch videos on-demand. Key features include video upload, storage, transcoding, content delivery, and user management. Scalability, low latency, and high availability are crucial.
Components:
- Client Application: Web, mobile, or smart TV interfaces for users to watch videos.
- API Gateway: Manages client requests and routes them to appropriate services.
- User Service: Handles user registration, authentication, and profiles.
- Video Upload Service:
- Manages video uploads from users.
- Integrates with storage and transcoding services.
- Storage Service:
- Stores original and transcoded video files.
- Uses distributed storage systems like AWS S3.
- Transcoding Service: Converts uploaded videos into multiple formats and resolutions.
- Content Delivery Network (CDN): Distributes video content globally to reduce latency.
- Streaming Server: Serves video content to users via protocols like HLS or DASH.
- Database: Stores metadata about videos, users, and interactions.
- Recommendation Engine: Suggests videos to users based on their viewing history.
Data Flow:
- Video Upload:
- User uploads a video through the client.
- Video Upload Service stores the file and triggers the Transcoding Service.
- Transcoding:
- Transcoding Service processes the video into various formats and resolutions.
- Transcoded videos are stored in the Storage Service.
- Content Delivery: Videos are distributed via CDN to ensure low-latency access.
- Video Playback:
- User selects a video to watch.
- Client requests the video stream from the Streaming Server through CDN.
- User Interaction:
- Viewing history and preferences are updated in the Database.
- Recommendation Engine updates suggestions based on interactions.
Scalability Considerations:
- Distributed Storage: Use scalable storage solutions like AWS S3 or Google Cloud Storage.
- CDN Utilization: Offload traffic to CDNs to handle high demand and reduce server load.
- Microservices Architecture: Break down services to scale independently based on demand.
- Load Balancing: Distribute incoming traffic across multiple streaming servers.
Performance Optimization:
- Adaptive Streaming: Adjust video quality in real-time based on user bandwidth.
- Caching: Cache popular videos at edge locations in the CDN.
- Efficient Encoding: Optimize transcoding pipelines for faster processing.
Security Considerations:
- Content Protection: Implement DRM to prevent unauthorized access and piracy.
- Authentication & Authorization: Ensure only authorized users can access restricted content.
- Data Encryption: Encrypt data in transit and at rest.
Technologies:
- Frontend: React, Vue.js, native mobile frameworks.
- Backend: Node.js, Python, Java.
- Transcoding: FFmpeg, AWS Elastic Transcoder.
- CDN: Cloudflare, Akamai, AWS CloudFront.
- Database: PostgreSQL, MongoDB.
- Streaming Protocols: HLS, DASH.
05. Design a Messaging Queue System
Answer: A messaging queue system facilitates communication between different parts of an application by decoupling producers and consumers. It ensures reliable message delivery, scalability, and fault tolerance.
Components:
- Producers: Applications or services that send messages to the queue.
- Consumers: Applications or services that receive and process messages from the queue.
- Message Broker: Core component that manages queues, stores messages, and routes them to consumers.
- Examples include RabbitMQ, Kafka, and AWS SQS.
- Queues: Logical containers that hold messages until they are processed.
- Message Store: Persistent storage for messages to ensure durability.
- Management Interface: Tools for monitoring, configuring, and managing the messaging system.
Data Flow:
- Message Production: Producers send messages to a specific queue in the Message Broker.
- Message Storage: Messages are stored persistently in the Message Store.
- Message Consumption: Consumers retrieve messages from the queue for processing.
- Acknowledgment: Consumers acknowledge successful processing, prompting the broker to remove the message from the queue.
- Retry Mechanism: If a consumer fails to process a message, the broker can retry or route it to a dead-letter queue.
Key Features:
- Durability: Ensure messages are not lost by persisting them to disk.
- Scalability: Handle increasing load by distributing queues and brokers across multiple servers.
- Fault Tolerance: Replicate queues and brokers to prevent single points of failure.
- Ordering Guarantees: Maintain the order of messages if required by the application.
- Delivery Guarantees: Support at-least-once, at-most-once, or exactly-once delivery semantics.
Scalability Considerations:
- Partitioning: Split queues across multiple brokers to balance the load.
- Replication: Replicate queues to ensure high availability and fault tolerance.
- Load Balancing: Distribute message processing across multiple consumers.
Performance Optimization:
- Batch Processing: Allow consumers to process messages in batches to increase throughput.
- Asynchronous Processing: Decouple message production and consumption to improve responsiveness.
- Efficient Serialization: Use lightweight serialization formats like Protocol Buffers or Avro.
Security Considerations:
- Authentication & Authorization: Ensure only authorized producers and consumers can access queues.
- Encryption: Encrypt messages in transit and at rest to protect sensitive data.
- Access Control: Implement fine-grained permissions for different queues and operations.
Technologies:
- Message Brokers: RabbitMQ, Apache Kafka, AWS SQS, Google Pub/Sub.
- Serialization Formats: JSON, XML, Protocol Buffers, Avro.
- Monitoring Tools: Prometheus, Grafana, Kibana.
06. Design a Banking System
Answer: A banking system manages financial transactions, customer accounts, loans, and other banking services. It requires high security, reliability, and compliance with financial regulations.
Components:
- User Interface: Web and mobile applications for customers to interact with their accounts.
- Authentication Service: Manages user login, multi-factor authentication, and session management.
- Account Management Service: Handles creation, updating, and deletion of customer accounts.
- Transaction Service: Manages deposits, withdrawals, transfers, and other financial transactions.
- Loan Service: Handles loan applications, approvals, repayments, and interest calculations.
- Notification Service: Sends alerts and notifications via email, SMS, or in-app messages.
- Database: Stores customer data, account information, transaction history, and loan details.
- Fraud Detection Service: Monitors transactions for suspicious activities and potential fraud.
- Compliance Service: Ensures the system adheres to financial regulations and standards.
- Reporting and Analytics: Provides insights into customer behavior, financial trends, and system performance.
Data Flow:
- User Authentication:
- Customer logs in through the UI.
- Authentication Service verifies credentials and issues a session token.
- Account Operations:
- Customer views account details, initiates transactions, or applies for loans via the UI.
- Requests are processed by the respective services and updated in the Database.
- Transaction Processing:
- Transaction Service ensures atomicity and consistency of financial operations.
- Updates account balances and records transaction history.
- Loan Management:
- Loan Service processes applications, approves or rejects based on criteria.
- Manages repayment schedules and interest calculations.
- Fraud Detection:
- Fraud Detection Service analyzes transactions in real-time to identify anomalies.
- Triggers alerts or blocks suspicious transactions as needed.
- Notifications: Notification Service informs customers about transaction statuses, loan approvals, etc.
Scalability Considerations:
- Microservices Architecture: Decompose the system into independent services to scale components based on demand.
- Database Sharding: Split databases to handle large volumes of transactions and customer data.
- Load Balancing: Distribute incoming traffic across multiple servers to ensure availability.
- Caching: Implement caching for frequently accessed data to reduce database load.
Security Considerations:
- Data Encryption: Encrypt sensitive data both in transit (TLS) and at rest.
- Access Control: Implement role-based access controls to restrict functionalities based on user roles.
- Audit Logging: Maintain detailed logs of all operations for accountability and compliance.
- Secure Coding Practices: Protect against common vulnerabilities like SQL injection, XSS, and CSRF.
Compliance:
- Regulations: Adhere to financial regulations such as PCI DSS, GDPR, and local banking laws.
- Data Privacy: Ensure customer data is handled according to privacy standards.
Technologies:
- Frontend: React, Angular, or native mobile frameworks.
- Backend: Java with Spring Boot, .NET, or Node.js.
- Database: PostgreSQL, Oracle, or SQL Server.
- Security: OAuth 2.0, JWT, SSL/TLS.
- Monitoring: Splunk, ELK Stack, Prometheus.
07. Design a Ticket Booking System
Answer: A ticket booking system allows users to browse, select, and purchase tickets for events such as movies, concerts, or flights. It requires real-time availability, secure payment processing, and efficient seat management.
Components:
- User Interface: Web and mobile applications for users to search and book tickets.
- API Gateway: Manages client requests and routes them to appropriate services.
- Authentication Service: Handles user registration, login, and session management.
- Event Management Service: Manages events, schedules, venues, and available seats.
- Search Service: Allows users to search for events based on various criteria.
- Booking Service: Handles seat selection, reservation, and booking confirmation.
- Payment Service: Processes payments securely through integrations with payment gateways.
- Notification Service: Sends booking confirmations, reminders, and updates via email or SMS.
- Database: Stores user data, event details, bookings, and transaction records.
- Inventory Management: Manages seat availability and prevents double-booking.
Data Flow:
- User Browsing:
- User searches for events using the Search Service.
- Event details and available seats are displayed.
- Seat Selection:
- User selects desired seats.
- Booking Service reserves the seats temporarily to prevent others from booking them.
- Payment Processing:
- User proceeds to payment.
- Payment Service handles the transaction through a secure gateway.
- Booking Confirmation:
- Upon successful payment, Booking Service finalizes the booking.
- Notification Service sends confirmation details to the user.
- Seat Allocation: Inventory Management updates seat availability in real-time.
Scalability Considerations:
- Load Balancing: Distribute incoming traffic across multiple servers to handle peak loads.
- Database Optimization: Use indexing and sharding to manage large volumes of booking data.
- Caching: Implement caching for frequently accessed data like event details.
- Microservices Architecture: Scale individual services based on demand, such as the Booking Service during ticket releases.
Performance Optimization:
- Real-time Availability: Ensure seat availability is updated instantly to prevent overbooking.
- Asynchronous Processing: Handle non-critical tasks like sending notifications asynchronously.
- Efficient Queries: Optimize database queries for faster search and retrieval.
Security Considerations:
- Secure Payments: Comply with PCI DSS standards for handling payment information.
- Data Protection: Encrypt sensitive user data and ensure secure storage.
- Fraud Prevention: Implement mechanisms to detect and prevent fraudulent bookings.
Reliability Considerations:
- High Availability: Ensure system uptime through redundant services and failover mechanisms.
- Transaction Management: Use ACID transactions to maintain data consistency during bookings.
Technologies:
- Frontend: React, Angular, or native mobile frameworks.
- Backend: Node.js, Java with Spring Boot, or Python with Django.
- Database: PostgreSQL, MySQL, or NoSQL databases like MongoDB.
- Payment Gateways: Stripe, PayPal, Braintree.
- Caching: Redis, Memcached.
- Search Engine: Elasticsearch for efficient event searching.
08. Design a Blogging Platform
Answer: A blogging platform allows users to create, publish, and manage blog posts. Features include user authentication, content creation, commenting, tagging, and content discovery. It should support scalability, content management, and user engagement.
Components:
- User Interface: Web and mobile applications for users to read and write blogs.
- API Gateway: Routes client requests to the appropriate backend services.
- Authentication Service: Manages user sign-up, login, and authorization.
- User Profile Service: Handles user profiles, settings, and preferences.
- Content Management Service: Manages creation, editing, publishing, and deletion of blog posts.
- Media Service: Handles uploading and storing images, videos, and other media assets.
- Commenting Service: Allows users to comment on blog posts and manage comment threads.
- Tagging and Categorization Service: Manages tags and categories for content organization.
- Search Service: Enables users to search for blog posts based on keywords, tags, or authors.
- Notification Service: Sends updates to users about new posts, comments, or interactions.
- Database: Stores user data, blog content, comments, tags, and other metadata.
- Analytics Service: Tracks user interactions, content popularity, and engagement metrics.
Data Flow:
- User Registration: Users sign up and create profiles via the Authentication and User Profile Services.
- Content Creation:
- Authenticated users create blog posts using the Content Management Service.
- Media files are uploaded through the Media Service.
- Publishing: Users publish posts, which are stored in the Database and indexed by the Search Service.
- Content Consumption: Readers access blog posts through the User Interface, fetched via the API Gateway.
- Engagement:
- Users comment on posts, managed by the Commenting Service.
- Notifications are sent for new comments or interactions.
- Content Discovery: Users search for content using the Search Service, filtering by tags or categories.
Scalability Considerations:
- Load Balancing: Distribute traffic across multiple servers to handle high user loads.
- Database Scaling: Implement read replicas and sharding to manage large volumes of data.
- Caching: Use caching for frequently accessed content to reduce database load.
- Microservices Architecture: Allow individual services to scale independently based on demand.
Performance Optimization:
- Content Delivery Network (CDN): Serve static assets like images and videos via CDNs for faster load times.
- Efficient Indexing: Optimize the Search Service for quick retrieval of blog posts.
- Asynchronous Processing: Handle non-critical tasks like sending notifications asynchronously.
Security Considerations:
- Data Protection: Encrypt sensitive data and ensure secure storage.
- Access Control: Implement role-based access to manage permissions for content creation and moderation.
- Input Validation: Prevent XSS, SQL injection, and other common vulnerabilities by validating user inputs.
Reliability Considerations:
- Backup and Recovery: Regularly backup data and have recovery plans in place.
- High Availability: Ensure services are redundant and can failover seamlessly in case of outages.
Technologies:
- Frontend: React, Vue.js, or Angular.
- Backend: Node.js with Express, Python with Django or Flask, Java with Spring Boot.
- Database: PostgreSQL, MySQL, or NoSQL databases like MongoDB.
- Search Engine: Elasticsearch or Apache Solr.
- Storage: AWS S3 for media files.
- Caching: Redis or Memcached.
- CDN: Cloudflare, AWS CloudFront.
09. Design a Real-time Collaboration Tool
Answer: A real-time collaboration tool allows multiple users to work simultaneously on shared documents, spreadsheets, or other resources. Features include real-time editing, version control, user presence indication, and conflict resolution.
Components:
- User Interface: Web and desktop applications where users can create and edit documents.
- API Gateway: Manages client requests and routes them to appropriate backend services.
- Authentication Service: Handles user registration, login, and access control.
- Document Management Service: Manages creation, storage, and retrieval of documents.
- Real-time Sync Service: Ensures that changes made by one user are propagated to all collaborators in real-time.
- WebSocket Server: Maintains persistent connections for real-time data exchange.
- Conflict Resolution Engine: Handles simultaneous edits and ensures consistency across all clients.
- Version Control Service: Tracks changes and maintains version history for documents.
- Presence Service: Indicates which users are currently viewing or editing a document.
- Notification Service: Sends alerts for document updates, comments, or access requests.
- Database: Stores user data, documents, change logs, and metadata.
- Storage Service: Stores large files or media associated with documents.
Data Flow:
- User Authentication: Users log in through the Authentication Service and receive a session token.
- Document Creation: Users create or open a document via the Document Management Service.
- Real-time Editing:
- Changes are sent through the Real-time Sync Service via WebSocket connections.
- Sync Service broadcasts updates to all connected clients.
- Conflict Resolution: Conflict Resolution Engine ensures that simultaneous edits do not overwrite each other, maintaining document integrity.
- Version Tracking: Changes are logged by the Version Control Service, allowing users to revert to previous versions if needed.
- User Presence: Presence Service updates the UI to show which users are currently collaborating on the document.
- Notifications: Notification Service alerts users about relevant updates or actions.
Scalability Considerations:
- Distributed WebSocket Servers: Scale WebSocket connections horizontally to handle numerous concurrent users.
- Load Balancing: Distribute traffic across multiple instances of services to manage high loads.
- Efficient Data Synchronization: Use operational transforms or CRDTs (Conflict-free Replicated Data Types) for efficient real-time synchronization.
- Database Scaling: Implement sharding and replication to handle large volumes of documents and change logs.
Performance Optimization:
- Delta Updates: Send only the changes (deltas) instead of the entire document to minimize bandwidth usage.
- Local Caching: Implement client-side caching to reduce latency and improve responsiveness.
- Efficient Data Structures: Use optimized data structures for representing documents and changes.
Security Considerations:
- Data Encryption: Encrypt data in transit using TLS and at rest in the storage systems.
- Access Control: Implement fine-grained permissions to control who can view or edit documents.
- Input Sanitization: Prevent injection attacks by sanitizing user inputs.
Reliability Considerations:
- Redundancy: Deploy services across multiple availability zones to ensure high availability.
- Backup and Recovery: Regularly backup documents and provide mechanisms for data recovery.
Technologies:
- Frontend: React, Angular, or Vue.js with real-time libraries like Socket.io.
- Backend: Node.js, Go, or Java with frameworks supporting WebSockets.
- WebSocket Server: Socket.io, ws for Node.js, or custom implementations.
- Database: MongoDB, PostgreSQL, or distributed databases like Cassandra.
- Real-time Sync: Operational Transform (OT) or CRDT libraries.
- Storage: AWS S3 or Google Cloud Storage for media files.
- Version Control: Git-based systems or custom versioning solutions.
10. Design a Cryptocurrency Exchange
Answer: A cryptocurrency exchange platform enables users to buy, sell, and trade various cryptocurrencies. Key features include user authentication, wallet management, order matching, transaction processing, and security measures to protect user assets.
Components:
- User Interface: Web and mobile applications for users to interact with the exchange.
- API Gateway: Manages client requests and routes them to appropriate backend services.
- Authentication Service: Handles user registration, login, two-factor authentication, and security protocols.
- User Management Service: Manages user profiles, KYC (Know Your Customer) processes, and account settings.
- Wallet Service: Manages cryptocurrency wallets for users, including deposits, withdrawals, and balance tracking.
- Order Management System (OMS): Handles the creation, matching, and execution of buy and sell orders.
- Matching Engine: Core component that matches buy and sell orders based on price and time priority.
- Transaction Processing Service: Processes completed trades and updates user balances accordingly.
- Market Data Service: Provides real-time and historical market data, including price charts and order books.
- Notification Service: Sends alerts for order confirmations, trade executions, and account activities.
- Security Service: Monitors for suspicious activities, implements fraud detection, and ensures compliance.
- Database: Stores user data, order books, transaction history, and wallet information.
- Compliance Service: Ensures the platform adheres to financial regulations and reporting requirements.
Data Flow:
- User Registration and Verification: Users sign up and complete KYC through the User Management Service.
- Depositing Funds: Users deposit cryptocurrencies or fiat money into their wallets via the Wallet Service.
- Placing Orders:
- Users place buy or sell orders through the User Interface.
- Orders are sent to the OMS and stored in the Database.
- Order Matching: Matching Engine processes incoming orders, matches compatible buy and sell orders, and executes trades.
- Transaction Processing:
- Completed trades update user balances in the Wallet Service.
- Transaction details are recorded in the Database.
- Withdrawing Funds: Users withdraw funds from their wallets, initiated via the Wallet Service.
- Market Data Updates: Market Data Service broadcasts real-time price updates and order book changes to users.
- Notifications: Notification Service informs users about order statuses, trades, and account activities.
Scalability Considerations:
- High Throughput: Ensure the Matching Engine can handle a large number of orders per second.
- Distributed Architecture: Deploy services across multiple servers and data centers to manage load and ensure availability.
- Database Sharding: Split databases to handle large volumes of transactions and user data.
- Caching: Implement caching for frequently accessed data like market prices and order books.
Performance Optimization:
- Low Latency: Optimize the Matching Engine for minimal delay in order matching and execution.
- Efficient Data Structures: Use optimized data structures for order books to speed up matching operations.
- Asynchronous Processing: Handle non-critical tasks asynchronously to maintain system responsiveness.
Security Considerations:
- Cold and Hot Wallets: Store the majority of funds in cold wallets (offline) to prevent hacking, and a small portion in hot wallets for transactions.
- Encryption: Encrypt all sensitive data in transit and at rest.
- Access Control: Implement strict access controls and multi-factor authentication for user accounts and administrative access.
- Regular Audits: Conduct security audits and penetration testing to identify and fix vulnerabilities.
Compliance Considerations:
- Regulatory Compliance:
- Adhere to AML (Anti-Money Laundering) and KYC regulations.
- Implement reporting mechanisms for regulatory authorities.
- Data Privacy: Comply with data protection laws like GDPR.
Reliability Considerations:
- Redundancy: Ensure services are redundant to prevent downtime.
- Disaster Recovery: Implement backup and recovery plans to handle data loss or system failures.
Technologies:
- Frontend: React, Angular, or Vue.js.
- Backend: Node.js, Java with Spring Boot, or Go.
- Database: PostgreSQL, MySQL, or NoSQL databases like Cassandra.
- Matching Engine: Custom-built for performance, possibly using in-memory data stores.
- Wallet Management: Integrate with blockchain nodes or use third-party wallet services.
- Security Tools: OAuth 2.0, JWT, SSL/TLS.
- Monitoring: Prometheus, Grafana, ELK Stack.
11. Design a Content Management System (CMS)
Answer: A Content Management System (CMS) allows users to create, manage, and publish digital content, typically for websites. Features include content creation, editing, workflow management, user roles, and publishing capabilities. It should be flexible, scalable, and user-friendly.
Components:
- User Interface: Web-based dashboard for content creators, editors, and administrators.
- API Gateway: Manages client requests and routes them to appropriate backend services.
- Authentication Service: Handles user registration, login, and role-based access control.
- Content Creation Service: Provides tools for creating and editing content, including rich text editors and media uploads.
- Content Storage Service: Stores content in a structured format, supporting various content types like articles, images, and videos.
- Workflow Management Service: Manages content approval processes, drafts, and publication schedules.
- Media Management Service: Handles uploading, storing, and managing media assets like images and videos.
- Search Service: Enables users to search for content based on keywords, tags, or categories.
- SEO Management Service: Provides tools for optimizing content for search engines, including metadata management.
- Version Control Service: Tracks changes to content, allowing users to revert to previous versions if needed.
- Publishing Service: Manages the deployment of content to the live website or other platforms.
- Analytics Service: Tracks content performance, user engagement, and other metrics.
- Database: Stores user data, content, media references, and metadata.
- Caching Layer: Caches frequently accessed content to improve load times and reduce database queries.
Data Flow:
- User Authentication: Users log in through the Authentication Service and are granted permissions based on roles.
- Content Creation:Users create or edit content using the Content Creation Service, utilizing rich text editors and media uploads.
- Saving Content: Content is saved to the Content Storage Service, with references to media managed by the Media Management Service.
- Workflow Management: Content moves through approval stages managed by the Workflow Management Service, ensuring proper review before publication.
- Version Control: Each change to the content is tracked by the Version Control Service, allowing for rollback if necessary.
- Publishing: Approved content is published via the Publishing Service, making it live on the website or other platforms.
- Content Delivery: Published content is served to users, utilizing the Caching Layer and CDN for optimal performance.
- Analytics: User interactions with content are tracked by the Analytics Service, providing insights into engagement and performance.
Scalability Considerations:
- Microservices Architecture: Break down the CMS into independent services that can scale based on demand.
- Distributed Storage: Use scalable storage solutions like AWS S3 for media assets.
- Load Balancing: Distribute incoming traffic across multiple servers to handle high user loads.
- Database Sharding: Split databases to manage large volumes of content and user data efficiently.
Performance Optimization:
- Caching: Implement caching for frequently accessed content to reduce latency and server load.
- Content Delivery Network (CDN): Use CDNs to serve static assets and reduce load times for users globally.
- Efficient Querying: Optimize database queries and use indexing to speed up content retrieval.
Security Considerations:
- Access Control: Implement role-based permissions to control who can create, edit, approve, and publish content.
- Data Encryption: Encrypt sensitive data both in transit and at rest.
- Input Validation: Prevent XSS, SQL injection, and other vulnerabilities by validating and sanitizing user inputs.
- Backup and Recovery: Regularly backup content and have recovery plans in place to prevent data loss.
Reliability Considerations:
- Redundancy: Deploy services across multiple availability zones to ensure high availability.
- Monitoring and Alerting: Continuously monitor system performance and set up alerts for any anomalies or failures.
- Disaster Recovery: Implement strategies to recover from system failures or data corruption quickly.
Technologies:
- Frontend: React, Angular, or Vue.js with CMS-specific frameworks like Gatsby or Next.js.
- Backend: Node.js with Express, Python with Django, or PHP with Laravel.
- Database: PostgreSQL, MySQL, or NoSQL databases like MongoDB for flexible content storage.
- Search Engine: Elasticsearch or Apache Solr for efficient content searching.
- Storage: AWS S3, Google Cloud Storage for media assets.
- Caching: Redis, Memcached.
- CDN: Cloudflare, AWS CloudFront.
- Version Control: Git-based systems integrated with the Version Control Service.
12. Implement a URL Shortener
Answer: A URL shortener maps long URLs to shorter, unique aliases. Key components include:
- Data Structures:
- HashMap (Dictionary): Stores mappings from short URLs to original URLs for quick retrieval.
- Reverse HashMap: Maps original URLs to their corresponding short versions to handle duplicates.
- Methods:
shortenURL(originalURL: String): String
: Generates a unique short URL, stores the mapping, and returns the short URL.expandURL(shortURL: String): String
: Retrieves the original URL associated with the given short URL.
- Short URL Generation:
- Use a base-62 encoding (characters a-z, A-Z, 0-9) to convert a unique identifier (e.g., database auto-increment ID) into a short string.
This approach ensures efficient storage and retrieval, with scalability to handle a large number of URLs.
13. Design a Library Management System
Answer: A library management system involves entities like Library, Book, Member, and Transaction.
- Classes:
- Library: Manages collections of Books and Members.
- Book: Attributes include title, author, ISBN, and availability status.
- Member: Attributes include memberID, name, and a list of borrowed Books.
- Transaction: Records details of book borrowings and returns, including dates and involved Members and Books.
- Methods:
- Library:
addBook(book: Book): void
removeBook(isbn: String): void
registerMember(member: Member): void
issueBook(isbn: String, memberID: String): Transaction
returnBook(isbn: String, memberID: String): Transaction
- Book: Getters and setters for book attributes.
- Member: Methods to borrow and return books.
- Transaction: Getters for transaction details.
- Library:
This design facilitates efficient management of library operations, including book inventory and member transactions.
14. Design an E-commerce Shopping Cart
Answer: An e-commerce shopping cart involves entities like Cart, Item, and User.
- Classes:
- Cart: Contains a list of Items and is associated with a User.
- Item: Attributes include itemID, name, price, and quantity.
- User: Attributes include userID, name, and associated Cart.
- Methods:
- Cart:
addItem(item: Item): void
removeItem(itemID: String): void
updateQuantity(itemID: String, quantity: int): void
calculateTotal(): double
checkout(): Order
- Item: Getters and setters for item attributes.
- User: Methods to view and manage the Cart.
- Cart:
This design ensures a seamless shopping experience, allowing users to manage their cart items and proceed to checkout efficiently.
15. Design a Rate Limiter
Answer: A rate limiter controls the rate of incoming requests to ensure fair usage and protect the system from abuse.
- Approaches:
- Token Bucket Algorithm: Tokens are added to a bucket at a fixed rate. Each request consumes a token; if no tokens are available, the request is denied or delayed. This allows for bursts of traffic while enforcing an average rate limit.
- Leaky Bucket Algorithm: Requests are added to a queue and processed at a constant rate. Excess requests are discarded or delayed, ensuring a steady outflow of requests.
- Fixed Window Counter: Counts the number of requests in fixed time windows (e.g., per minute). If the count exceeds the limit, subsequent requests are denied until the next window.
- Sliding Window Log: Maintains a log of request timestamps and counts requests within a rolling time window, providing a more accurate rate limit.
- Implementation Steps:
- Identify the Limiting Criteria: Determine whether to limit based on user ID, IP address, API key, etc.
- Choose an Algorithm: Select an appropriate rate-limiting algorithm based on requirements.
- Set Thresholds: Define the maximum number of requests allowed per time unit.
- Monitor Requests: Track incoming requests and apply the rate-limiting logic.
- Handle Violations: Decide on actions for exceeding limits, such as rejecting requests with a 429 status code or delaying processing.
- Considerations:
- Distributed Systems: Ensure consistency across multiple servers, possibly using centralized data stores like Redis to track request counts.
- Burst Handling: Allow short bursts of traffic while maintaining overall rate limits.
- Fairness: Implement user-specific limits to prevent a single user from consuming disproportionate resources.
Implementing a rate limiter effectively requires balancing system protection with user experience, ensuring legitimate users are not unduly affected.
16. Design a Cache System
Answer: A cache system temporarily stores frequently accessed data to improve retrieval times and reduce load on underlying resources.
- Key Components:
- Cache Storage: Holds the cached data, implemented using in-memory data structures like hash maps for O(1) access time.
- Eviction Policy: Determines which items to remove when the cache reaches its capacity.
- Common Eviction Policies:
- Least Recently Used (LRU): Evicts the least recently accessed items first.
- Least Frequently Used (LFU): Evicts items accessed the least number of times.
- First In, First Out (FIFO): Evicts the oldest items first, based on insertion order.
- Random Replacement: Evicts items at random, which can be useful in certain scenarios.
- Implementation Steps:
- Determine Cache Size: Decide on the maximum number of items or total memory size for the cache.
- Choose Data Structures: Use hash maps for fast lookups and linked lists or priority queues to manage eviction order.
- Implement Eviction Policy: Ensure the chosen policy is correctly applied during insertions and when the cache reaches capacity.
- Handle Cache Misses: Define how to retrieve data not present in the cache and how to update the cache accordingly.
- Considerations:
- Consistency: Ensure cached data remains consistent with the source, possibly through time-to-live (TTL) settings or invalidation strategies.
- Concurrency: Handle concurrent access to the cache, ensuring thread safety and data integrity.
- Persistence: Decide if the cache should persist data across restarts or be entirely in-memory.
Designing an effective cache system requires understanding access patterns and choosing appropriate eviction policies to balance performance and resource utilization.
17. Design a Notification System
Answer: A notification system delivers messages to users through various channels, ensuring timely and relevant communication.
- Key Components:
- Notification Service: Central hub that manages notification creation, scheduling, and dispatching.
- Channel Handlers: Specific modules responsible for sending notifications via different channels (e.g., EmailHandler, SMSHandler, PushNotificationHandler).
- User Preferences: Stores user-specific settings indicating preferred channels and notification types.
- Message Queue: Buffers notifications to handle high throughput and ensure reliable delivery.
- Workflow:
- Notification Creation: An event triggers the creation of a notification, which includes the message content, recipient details, and preferred delivery channels.
- Queueing: The notification is placed into a message queue to handle high throughput and ensure reliable delivery.
- Processing: Worker services consume messages from the queue, consult user preferences, and delegate the notification to the appropriate Channel Handler.
- Delivery: The Channel Handler formats the message as required and sends it via the specified channel (e.g., SMTP for email, SMS gateway for SMS).
- Feedback Handling: Capture delivery statuses and user interactions (e.g., read receipts) to update the system and inform future notifications.
- Considerations:
- Scalability: Utilize distributed message queues and scalable worker services to handle varying loads.
- Reliability: Implement retry mechanisms and dead-letter queues to manage failed deliveries.
- User Preferences: Allow users to customize notification settings, including preferred channels and do-not-disturb periods.
- Compliance: Ensure adherence to regulations like GDPR, including user consent and data protection.
This design provides a flexible and extensible framework for sending notifications across multiple channels, accommodating user preferences and ensuring reliable delivery.
18. Design a Ride-Sharing Service
Answer: Designing a ride-sharing service involves multiple components interacting seamlessly to match riders with drivers.
- Key Components:
- User Management: Handles registration, authentication, and profiles for riders and drivers.
- Ride Matching: Matches ride requests from riders with available drivers based on location and other criteria.
- Geolocation Services: Tracks real-time locations of drivers and riders using GPS data.
- Pricing Engine: Calculates fares based on distance, time, demand (surge pricing), and other factors.
- Payment System: Processes payments, including fare calculation, invoicing, and driver payouts.
- Notification System: Sends updates to users about ride status, driver arrival, payment receipts, etc.
- Rating and Feedback: Allows riders and drivers to rate each other and provide feedback to maintain service quality.
- Workflow:
- Ride Request: A rider requests a ride, specifying pickup and drop-off locations.
- Driver Matching: The system identifies the nearest available driver and sends a ride offer.
- Ride Acceptance: The driver accepts the ride; the rider is notified with driver details and estimated arrival time.
- Ride Execution: The driver picks up the rider and proceeds to the destination, with real-time tracking available to both parties.
- Payment Processing: Upon ride completion, the fare is calculated, and payment is processed automatically.
- Feedback Collection: Both rider and driver are prompted to provide ratings and feedback.
- Considerations:
- Scalability: Design the system to handle high volumes of concurrent users and ride requests.
- Reliability: Ensure high availability and quick recovery from failures.
- Security: Protect user data and transactions through encryption and secure authentication mechanisms.
- Regulatory Compliance: Adhere to local transportation laws and regulations, including licensing and insurance requirements.
This design outlines the core components and workflows necessary for a functional ride-sharing service, emphasizing scalability, reliability, and user satisfaction.
19. Design a Hotel Booking System
Answer: A hotel booking system manages room availability, reservations, and customer information.
- Classes:
- Hotel: Attributes include name, location, and a list of Rooms.
- Room: Attributes include roomNumber, type (e.g., single, double), status (available, booked), and price.
- Customer: Attributes include customerID, name, contact details, and booking history.
- Reservation: Attributes include reservationID, Customer, Room, check-in and check-out dates, and payment details.
- Methods:
- Hotel:
searchRooms(checkInDate: Date, checkOutDate: Date, roomType: String): List<Room>
- Room:
isAvailable(checkInDate: Date, checkOutDate: Date): boolean
- Customer:
makeReservation(room: Room, checkInDate: Date, checkOutDate: Date): Reservation
cancelReservation(reservationID: String): boolean
- Reservation:
confirm(): void
cancel(): void
- Hotel:
- Workflow:
- Room Search: Customer searches for available rooms based on desired dates and room type.
- Reservation Creation: Customer selects a room and makes a reservation, providing necessary details.
- Payment Processing: System processes payment and confirms the reservation.
- Check-In/Check-Out: Upon arrival, the customer checks in; after the stay, checks out, updating room status accordingly.
- Considerations:
- Concurrency: Handle simultaneous booking attempts for the same room.
- Cancellation Policy: Implement rules for reservation modifications and cancellations.
- Notifications: Send confirmation and reminder notifications to customers.
This design provides a structured approach to managing hotel bookings, ensuring efficient operations and a positive customer experience.
20. Design a Food Delivery System
Workflow:
- User Registration and Authentication: Users and restaurants register on the platform, providing necessary details.
- Restaurant Management: Restaurants update their menus, prices, and availability.
- Menu Browsing: Users browse available restaurants and their menus, applying filters as needed.
- Order Placement: Users add items to their cart, specify delivery details, and place the order.
- Order Processing: The system sends the order to the restaurant for preparation.
- Delivery Assignment: A delivery partner is assigned to pick up the order and deliver it to the user.
- Payment Processing: Users make payments through integrated payment gateways.
- Order Tracking: Users track the status of their order in real-time.
- Feedback Collection: After delivery, users can rate and review the service.
- Considerations:
- Scalability: Design the system to handle high volumes of users, orders, and real-time tracking.
- Reliability: Ensure the system is robust, with minimal downtime and quick recovery from failures.
- Security: Protect user data and transactions through encryption and secure authentication mechanisms.
- User Experience: Provide a seamless and intuitive interface for users, restaurants, and delivery partners.
- Regulatory Compliance: Adhere to local laws and regulations related to food delivery and data privacy.
This design outlines the core components and workflows necessary for a functional food delivery system, emphasizing scalability, reliability, and user satisfaction.
21. Design a Social Media Feed
Answer: A social media feed aggregates and displays content from a user’s connections in a personalized and timely manner.
- Key Components:
- User Profile: Stores user information, including connections (friends, followed pages).
- Post: Represents content created by users or pages, including text, images, videos, timestamps, and metadata.
- Feed Generator: Aggregates posts from a user’s connections, applies ranking algorithms, and generates the feed.
- Notification System: Notifies users about new posts, comments, likes, and other interactions.
- Workflow:
- Content Creation: Users and pages create posts, which are stored in the database with relevant metadata.
- Feed Generation:
- Aggregation: Collect posts from the user’s connections.
- Filtering: Remove irrelevant or duplicate content.
- Ranking: Apply algorithms to prioritize content based on relevance, recency, user preferences, and engagement metrics.
- Feed Delivery: The generated feed is delivered to the user’s interface, allowing for interactions like likes, comments, and shares.
- Real-Time Updates: Implement mechanisms (e.g., WebSockets) to push new content and updates to the user’s feed in real-time.
- Considerations:
- Scalability: Efficiently handle large volumes of data and user interactions.
- Personalization: Tailor the feed to individual user preferences and behaviors.
- Latency: Ensure low latency in feed generation and delivery for a responsive user experience.
- Content Moderation: Implement systems to detect and handle inappropriate or harmful content.
- Privacy: Respect user privacy settings and data protection regulations.
This design provides a framework for delivering a dynamic and personalized social media feed, balancing performance with user engagement and safety.
22. Design a File Storage System
Answer: A distributed file storage system allows users to store, share, and synchronize files across multiple devices.
- Key Components:
- Client Application: Interfaces with the user, enabling file upload, download, synchronization, and sharing.
- Metadata Server: Manages metadata, including file names, directory structures, permissions, and version history.
- Storage Nodes: Store the actual file data, distributed across multiple servers for redundancy and scalability.
- Synchronization Service: Ensures files are consistent across all user devices, handling updates and conflict resolution.
- Access Control: Manages user authentication, authorization, and permissions for file access and sharing.
- Workflow:
- File Upload:
- Client Interaction: User uploads a file via the client application.
- Metadata Handling: Client sends file metadata to the Metadata Server.
- Data Storage: File is divided into chunks, which are distributed and stored across multiple Storage Nodes.
- Metadata Update: Metadata Server records the locations of file chunks and updates the directory structure.
- File Synchronization:
- Change Detection: Client monitors local files for changes.
- Update Propagation: Changes are uploaded to the server, and the Synchronization Service propagates updates to other devices.
- Conflict Resolution: Handles simultaneous edits using versioning or user prompts to merge changes.
- File Sharing:
- Permission Setting: User sets sharing permissions (e.g., read, write) for other users.
- Access Provisioning: Metadata Server updates access control lists, and shared files appear in recipients’ directories.
- File Upload:
- Considerations:
- Data Redundancy: Implement replication strategies to ensure data availability and durability.
- Scalability: Design the system to handle increasing amounts of data and users efficiently.
- Security: Encrypt data at rest and in transit, and enforce strong authentication mechanisms.
- Performance: Optimize for fast file access and minimal synchronization delays.
- User Experience: Provide intuitive interfaces and seamless integration across devices.
This design ensures reliable, secure, and efficient file storage and synchronization across multiple devices, catering to both individual and collaborative use cases.
23. Design a Hotel Management System
Answer: A hotel management system manages room inventory, reservations, guest check-ins and check-outs, and billing operations.
- Key Components:
- Room: Attributes include room number, type (e.g., single, double, suite), status (e.g., available, occupied, reserved), and rate.
- Guest: Attributes include guest ID, name, contact information, and reservation history.
- Reservation: Details such as reservation ID, guest information, room assigned, check-in and check-out dates, and payment status.
- Billing System: Handles invoicing, payments, and refunds.
- Front Desk Interface: Used by staff to manage reservations, check-ins, check-outs, and guest inquiries.
- Workflow:
- Room Booking:
- Availability Check: Guest or staff checks room availability for desired dates.
- Reservation Creation: System creates a reservation, assigns a room, and updates the room’s status to reserved.
- Confirmation: System sends a booking confirmation to the guest.
- Check-In:
- Guest Arrival: Guest arrives at the hotel.
- Identity Verification: Staff verifies guest identity and reservation details.
- Room Assignment: System updates the room status to occupied and records the check-in time.
- Stay Management:
- Service Requests: Guests can request services (e.g., room service, housekeeping) through the system.
- Billing Updates: System adds charges for services to the guest’s bill.
- Check-Out:
- Bill Preparation: System compiles all charges and prepares the final bill.
- Payment Processing: Guest settles the bill through available payment methods.
- Room Status Update: System updates the room status to available after housekeeping clearance.
- Room Booking:
- Considerations:
- Scalability: Support multiple properties and a large number of rooms and reservations.
- Integration: Interface with external systems like online travel agencies and payment gateways.
- Security: Protect guest data and ensure secure payment processing.
- Reporting: Generate reports on occupancy rates, revenue, and guest demographics.
- User Roles: Define permissions for different roles, such as front desk staff, housekeeping, and management.
This design outlines a comprehensive hotel management system that streamlines operations, enhances guest experience, and provides valuable insights for management.
Learn More: Carrer Guidance | Hiring Now!
Top 40 SQL Interview Questions for 3 Years Experience with Answers
Advanced TOSCA Test Automation Engineer Interview Questions and Answers with 5+ Years of Experience
DSA Interview Questions and Answers
Angular Interview Questions and Answers for Developers with 5 Years of Experience
Android Interview Questions for Senior Developer with Detailed Answers
SQL Query Interview Questions for Freshers with Answers