Are you preparing for a JDBC (Java Database Connectivity) interview? We compiled a list of the most commonly asked interview questions, which will help you understand all core concepts and functionalities. Below are 40 comprehensive JDBC interview questions along with detailed answers to help you prepare effectively.
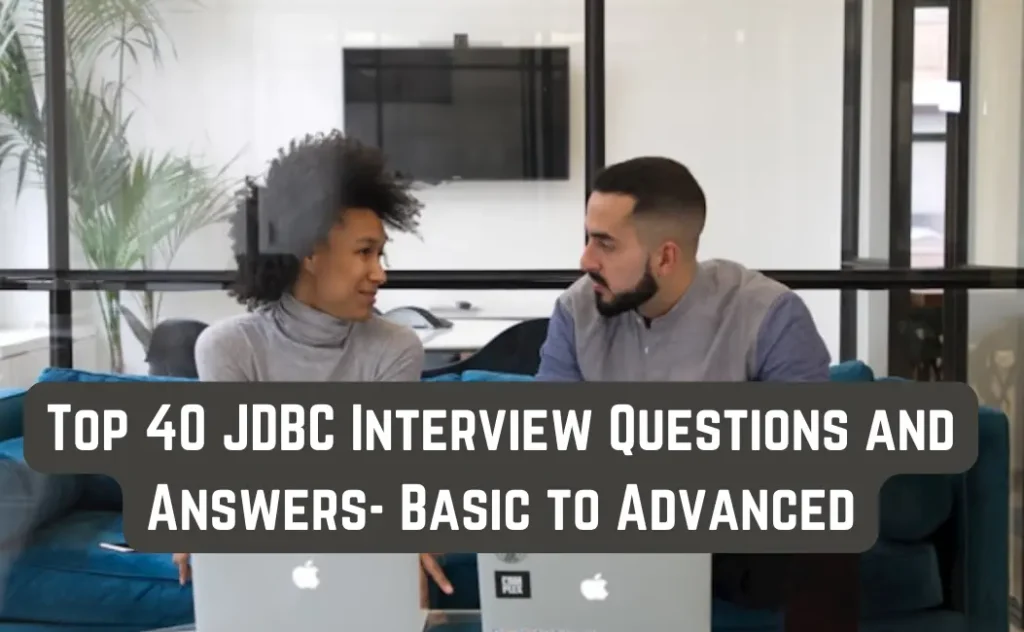
JDBC Interview Questions and Answers- Basic to Advanced
- What is JDBC?
- What are the main components of JDBC?
- How do you establish a connection to a database using JDBC?
- What are the different types of JDBC drivers?
- What is the difference between Statement and PreparedStatement in JDBC?
- How do you execute a SQL query using JDBC?
- What is ResultSet in JDBC?
- What are the different types of ResultSet in JDBC?
- How do you handle transactions in JDBC?
- What is a PreparedStatement, and how does it differ from a Statement?
- How do you execute a stored procedure in JDBC?
- What is connection pooling, and why is it used in JDBC?
- How do you handle exceptions in JDBC?
- How do you handle exceptions in JDBC?
- What is the role of the DriverManager class in JDBC?
- What is a Batch Update in JDBC, and how is it performed?
- What is the difference between
execute()
,executeQuery()
, andexecuteUpdate()
methods in JDBC? - How do you retrieve metadata in JDBC?
- What is the purpose of the
setFetchSize()
andsetMaxRows()
methods in JDBC? - How do you handle BLOB and CLOB data types in JDBC?
- What is the difference between
java.sql.Date
andjava.util.Date
? - What is the difference between a CallableStatement and a
- How do you implement transaction management in JDBC?
- What is the purpose of the DatabaseMetaData interface in JDBC?
- Explain how to handle BLOB and CLOB data types in JDBC.
- What are the different types of locking mechanisms in JDBC?
- How do you perform batch processing in JDBC?
- Explain how to retrieve metadata about ResultSet in JDBC.
- What are some common exceptions thrown by JDBC?
- How do you set up a connection with a database using JDBC?
- What is the significance of setFetchSize() method in ResultSet?
- Describe how you would use savepoints in JDBC transactions.
- How do you perform error handling in JDBC?
- Explain what an SQL Injection attack is and how can it be prevented in JDBC?
- What are Type 1, Type 2, Type 3, and Type 4 JDBC drivers?
- How do you execute multiple SQL statements in one go using JDBC?
- What are some best practices when working with JDBC?
- How do you retrieve specific columns from a ResultSet?
- Explain what AutoCommit mode means in JDBC.
- What steps would you follow to connect to an Oracle database using JDBC?
1. What is JDBC?
Answer: JDBC stands for Java Database Connectivity. It is a Java API that enables Java applications to interact with various databases. JDBC provides methods to query and update data in a database, facilitating seamless interaction between Java code and SQL statements.
JDBC acts as a bridge between Java applications and databases, allowing developers to execute SQL statements, retrieve results, and manage database connections. It supports a wide range of databases, including relational databases like MySQL, Oracle, and PostgreSQL.
2. What are the main components of JDBC?
Answer: The main components of JDBC include:
- DriverManager: Manages a list of database drivers and establishes a connection between the application and the database.
- Driver: Interfaces that handle the communications with the database server.
- Connection: Represents a session with a specific database.
- Statement: Used to execute SQL queries against the database.
- PreparedStatement: A subclass of Statement that allows precompiled SQL queries with parameterized inputs.
- CallableStatement: Used to execute stored procedures in the database.
- ResultSet: Represents the result set of a query.
- SQLException: Handles any errors that occur in a database application.
These components work together to facilitate database operations in Java applications. The DriverManager class manages database drivers, while the Connection interface establishes a session with the database. Statements are used to execute SQL queries, and ResultSet holds the data retrieved from the database.
3. How do you establish a connection to a database using JDBC?
Answer: To establish a connection to a database using JDBC, follow these steps:
- Load the JDBC driver: Use
Class.forName()
to load the driver class. - Establish a connection: Use
DriverManager.getConnection()
with the database URL, username, and password.
Example:
Class.forName("com.mysql.cj.jdbc.Driver");
Connection connection = DriverManager.getConnection(
"jdbc:mysql://localhost:3306/mydatabase", "username", "password");
Loading the JDBC driver ensures that the driver is registered with the DriverManager. Establishing a connection involves providing the database URL, which includes the protocol (jdbc
), subprotocol (mysql
), host (localhost
), port (3306
), and database name (mydatabase
). The username and password authenticate the connection.
4. What are the different types of JDBC drivers?
Answer: There are four types of JDBC drivers:
Type 1: JDBC-ODBC Bridge Driver
- Translates JDBC calls into ODBC calls.
- Requires ODBC drivers to be installed on the client machine.
- Platform-dependent and not recommended for production use.
Type 2: Native-API Driver
- Converts JDBC calls into native database API calls.
- Requires native database libraries on the client machine.
- Offers better performance than Type 1 but is platform-dependent.
Type 3: Network Protocol Driver
- Translates JDBC calls into a database-independent network protocol.
- Requires a middleware server to convert the protocol to database-specific calls.
- Platform-independent and suitable for internet-based applications.
Type 4: Thin Driver
- Converts JDBC calls directly into the database-specific protocol.
- Pure Java driver, platform-independent.
- Offers high performance and is widely used in production environments.
Each driver type has its advantages and limitations. Type 4 drivers are commonly preferred due to their platform independence and performance benefits. Type 1 and Type 2 drivers are less commonly used due to their platform dependencies and the need for additional client-side configurations.
5. What is the difference between Statement and PreparedStatement in JDBC?
Answer:
Statement:
- Used for executing static SQL queries without parameters.
- Each execution of a Statement incurs the overhead of compiling the SQL query.
PreparedStatement:
- Used for executing precompiled SQL queries with or without parameters.
- Offers better performance for repeated executions of the same query.
- Helps prevent SQL injection attacks by safely handling input parameters.
PreparedStatement is preferred over Statement when executing the same query multiple times with different parameters, as it reduces the overhead of query compilation. Additionally, PreparedStatement provides a safer way to handle user inputs, mitigating the risk of SQL injection attacks.
6. How do you execute a SQL query using JDBC?
Answer: To execute a SQL query using JDBC:
- Create a Statement or PreparedStatement object:
Statement statement = connection.createStatement();
// or
PreparedStatement preparedStatement = connection.prepareStatement(sqlQuery);
- Execute the query:
- For SELECT queries:
ResultSet resultSet = statement.executeQuery(sqlQuery); // or ResultSet resultSet = preparedStatement.executeQuery();
- For INSERT, UPDATE, DELETE queries:
java int rowsAffected = statement.executeUpdate(sqlQuery); // or int rowsAffected = preparedStatement.executeUpdate();
The executeQuery()
method is used for SQL statements that retrieve data (e.g., SELECT), returning a ResultSet object. The executeUpdate()
method is used for SQL statements that modify data (e.g., INSERT, UPDATE, DELETE), returning an integer indicating the number of rows affected.
7. What is ResultSet in JDBC?
Answer: A ResultSet is an object in JDBC that represents the result of executing a SQL query. It maintains a cursor pointing to its current row of data and provides methods to traverse and retrieve data from the database result.
Key features:
- Row Navigation:
// Move cursor forward
next()
// Move cursor backward (if supported)
previous()
// Move to first row
first()
// Move to last row
last()
- Data Retrieval:
// Get data by column name
getString("column_name")
getInt("column_name")
getDouble("column_name")
// Get data by column index (1-based)
getString(1)
getInt(2)
getDouble(3)
- ResultSet Types:
- TYPE_FORWARD_ONLY: Default, cursor moves forward only
- TYPE_SCROLL_INSENSITIVE: Can scroll both ways, not sensitive to database changes
- TYPE_SCROLL_SENSITIVE: Can scroll both ways, reflects database changes
Basic usage example:
Statement stmt = connection.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM employees");
while (rs.next()) {
String name = rs.getString("name");
int age = rs.getInt("age");
double salary = rs.getDouble("salary");
// Process the data
}
8. What are the different types of ResultSet in JDBC?
Answer: In JDBC, ResultSet
types are defined based on their scrollability and sensitivity to changes in the underlying data. The main types are:
Type: ResultSet.TYPE_FORWARD_ONLY
- Description: The cursor can only move forward, one row at a time.
- Use Case: Suitable for simple, read-only operations where backward navigation isn’t required.
Type: ResultSet.TYPE_SCROLL_INSENSITIVE
- Description: The cursor can move both forward and backward. However, it does not reflect changes made to the database after the
ResultSet
was created. - Use Case: Useful when you need to navigate through the result set multiple times, and consistency is more important than real-time accuracy.
Type: ResultSet.TYPE_SCROLL_SENSITIVE
- Description: The cursor can move both forward and backward and reflects changes made to the database after the
ResultSet
was created. - Use Case: Ideal when you need to navigate through the result set multiple times and require the most up-to-date data.
Choosing the appropriate ResultSet
type depends on the application’s requirements for data navigation and sensitivity to concurrent data modifications. For instance, if the application needs to detect changes made by others to the database while processing the result set, TYPE_SCROLL_SENSITIVE
would be appropriate.
9. How do you handle transactions in JDBC?
Answer: Transactions in JDBC are managed using the Connection
object’s auto-commit mode and explicit commit or rollback operations.
Steps:
- Disable Auto-Commit Mode:
connection.setAutoCommit(false);
- Execute SQL Statements:
// Execute multiple SQL statements
statement.executeUpdate(sql1);
statement.executeUpdate(sql2);
- Commit the Transaction:
connection.commit();
- Handle Exceptions and Rollback if Necessary:
try {
// Execute SQL statements
statement.executeUpdate(sql1);
statement.executeUpdate(sql2);
// Commit transaction
connection.commit();
} catch (SQLException e) {
// Rollback transaction in case of error
connection.rollback();
throw e;
} finally {
// Restore auto-commit mode
connection.setAutoCommit(true);
}
By default, JDBC connections operate in auto-commit mode, meaning each SQL statement is committed immediately after execution. Disabling auto-commit allows multiple statements to be grouped into a single transaction, ensuring atomicity. Explicitly committing the transaction applies all changes, while rolling back undoes them in case of errors.
10. What is a PreparedStatement, and how does it differ from a Statement?
Answer: A PreparedStatement
is a precompiled SQL statement that can be executed multiple times with different input parameters.
Differences between Statement
and PreparedStatement
:
Aspect | Statement | PreparedStatement |
---|---|---|
Compilation | Compiled each time it is executed. | Compiled once; can be executed multiple times. |
Performance | Slower for repeated executions. | Faster for repeated executions due to precompilation. |
Parameterization | Does not support parameters. | Supports parameterized queries using placeholders (? ). |
Security | Prone to SQL injection attacks. | Helps prevent SQL injection by safely handling inputs. |
Example:
Using Statement
:
String sql = "INSERT INTO users (username, password) VALUES ('" + user + "', '" + pass + "')";
statement.executeUpdate(sql);
Using PreparedStatement
:
String sql = "INSERT INTO users (username, password) VALUES (?, ?)";
PreparedStatement pstmt = connection.prepareStatement(sql);
pstmt.setString(1, user);
pstmt.setString(2, pass);
pstmt.executeUpdate();
PreparedStatement
enhances performance for repeated executions and provides a safer way to handle user inputs, mitigating the risk of SQL injection attacks.
11. How do you execute a stored procedure in JDBC?
Answer: To execute a stored procedure in JDBC, use the CallableStatement
interface.
Steps:
- Create a
CallableStatement
Object:
CallableStatement cstmt = connection.prepareCall("{call procedure_name(?, ?)}");
- Set Input Parameters (if any):
cstmt.setInt(1, parameterValue);
- Register Output Parameters (if any):
cstmt.registerOutParameter(2, Types.INTEGER);
- Execute the Stored Procedure:
cstmt.execute();
- Retrieve Output Parameters:
int result = cstmt.getInt(2);
CallableStatement
allows the execution of stored procedures in the database. Input parameters are set using set
methods, and output parameters are registered using registerOutParameter
. After execution, output parameters can be retrieved using appropriate get
methods.
12. What is connection pooling, and why is it used in JDBC?
Answer: Connection pooling is a technique used to manage a pool of reusable database connections. Instead of creating a new connection for each database operation, applications reuse existing connections from the pool, improving performance and resource utilization.
Benefits:
- Performance Improvement: Reduces the overhead of establishing and closing connections.
- Resource Management: Limits the number of connections, preventing resource exhaustion.
- Scalability: Supports a higher number of concurrent users by efficiently managing connections.
Establishing a database connection is resource-intensive. Connection pooling mitigates this by maintaining a pool of active connections that can be reused, leading to faster response times and better resource management.
Q13. How do you handle exceptions in JDBC?
Answer: In JDBC, exceptions are primarily handled using the SQLException
class, which provides detailed information about database access errors.
Key Methods of SQLException
:
getMessage()
: Retrieves the description of the error.getErrorCode()
: Returns the vendor-specific error code.getSQLState()
: Provides the SQLState string, which follows the X/Open SQLState conventions.getNextException()
: Retrieves the nextSQLException
in the chain.
Example:
try {
Connection connection = DriverManager.getConnection(dbURL, username, password);
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM users");
// Process the result set
} catch (SQLException e) {
System.err.println("Error Message: " + e.getMessage());
System.err.println("SQL State: " + e.getSQLState());
System.err.println("Error Code: " + e.getErrorCode());
SQLException nextException = e.getNextException();
while (nextException != null) {
System.err.println("Next Exception: " + nextException.getMessage());
nextException = nextException.getNextException();
}
} finally {
// Close resources
}
Handling exceptions in JDBC involves catching SQLException
and utilizing its methods to obtain detailed information about the error. This approach aids in debugging and provides clarity on the nature of the issue encountered during database operations.
14. What is the role of the DriverManager class in JDBC?
Answer: The DriverManager
class in JDBC acts as an interface between the user and the drivers. It manages a list of database drivers and establishes a connection between the application and the appropriate database driver.
Key Responsibilities:
- Driver Registration: Loads and registers database drivers using
Class.forName()
orDriverManager.registerDriver()
. - Connection Establishment: Provides methods like
getConnection()
to establish a connection to the database. - Driver Management: Maintains a list of registered drivers and matches connection requests to the appropriate driver.
Example:
Class.forName("com.mysql.cj.jdbc.Driver");
Connection connection = DriverManager.getConnection(
"jdbc:mysql://localhost:3306/mydatabase", "username", "password");
The DriverManager
class is essential for managing database drivers and establishing connections. It ensures that the appropriate driver is used for each connection request, facilitating seamless communication between the Java application and the database.
15. What is a Batch Update in JDBC, and how is it performed?
Answer: A Batch Update in JDBC allows multiple SQL statements to be executed as a single batch, reducing the number of database calls and improving performance.
Steps to Perform Batch Update:
- Disable Auto-Commit Mode:
connection.setAutoCommit(false);
- Create a Statement or PreparedStatement:
Statement statement = connection.createStatement();
// or
PreparedStatement preparedStatement = connection.prepareStatement(sql);
- Add SQL Statements to the Batch:
statement.addBatch("INSERT INTO users (username) VALUES ('user1')");
statement.addBatch("INSERT INTO users (username) VALUES ('user2')");
// or
preparedStatement.setString(1, "user1");
preparedStatement.addBatch();
preparedStatement.setString(1, "user2");
preparedStatement.addBatch();
- Execute the Batch:
int[] updateCounts = statement.executeBatch();
// or
int[] updateCounts = preparedStatement.executeBatch();
- Commit the Transaction:
connection.commit();
Batch updates are beneficial when executing multiple similar queries, such as inserting multiple records. By batching these operations, the application reduces the overhead of multiple database round-trips, leading to improved performance.
16. What is the difference between execute()
, executeQuery()
, and executeUpdate()
methods in JDBC?
Answer:
Method | Return Type | Usage |
---|---|---|
execute() | boolean | Used for executing any SQL statement. Returns true if the result is a ResultSet ; false if it is an update count or no result. |
executeQuery() | ResultSet | Used for executing SELECT statements that return data. |
executeUpdate() | int | Used for executing INSERT , UPDATE , DELETE , or DDL statements. Returns the number of rows affected. |
Explanation:
execute()
: Suitable for executing any SQL statement. The return value indicates whether the result is aResultSet
or an update count.executeQuery()
: Specifically used forSELECT
statements that retrieve data from the database.executeUpdate()
: Used for statements that modify data or the database schema, such asINSERT
,UPDATE
,DELETE
, or DDL statements likeCREATE TABLE
.
Q17. How do you retrieve metadata in JDBC?
Answer: In JDBC, metadata can be retrieved using the DatabaseMetaData
and ResultSetMetaData
interfaces.
Retrieving Database Metadata:
DatabaseMetaData dbMetaData = connection.getMetaData();
String databaseProductName = dbMetaData.getDatabaseProductName();
String databaseProductVersion = dbMetaData.getDatabaseProductVersion();
Retrieving ResultSet Metadata:
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM users");
ResultSetMetaData rsMetaData = resultSet.getMetaData();
int columnCount = rsMetaData.getColumnCount();
for (int i = 1; i <= columnCount; i++) {
String columnName = rsMetaData.getColumnName(i);
String columnType = rsMetaData.getColumnTypeName(i);
}
ResultSetMetaData
: Provides information about the columns in a ResultSet
, including column names, types, and
DatabaseMetaData
: Provides information about the database as a whole, such as its capabilities, supported features, and version details.
18. What is the purpose of the setFetchSize()
and setMaxRows()
methods in JDBC?
Answer: In JDBC, the setFetchSize()
and setMaxRows()
methods are used to optimize the retrieval of query results by controlling the number of rows processed.
setFetchSize(int rows)
:
- Purpose: Suggests to the JDBC driver the number of rows to fetch from the database when more rows are needed for
ResultSet
processing. - Usage: Helps in optimizing performance, especially when dealing with large result sets, by reducing the number of database round-trips.
Example:
Statement stmt = connection.createStatement();
stmt.setFetchSize(100);
ResultSet rs = stmt.executeQuery("SELECT * FROM large_table");
setMaxRows(int max)
:
- Purpose: Limits the maximum number of rows that a
ResultSet
can contain. - Usage: Useful when only a subset of the query results is needed, thereby reducing memory consumption and processing time.
Example:
Statement stmt = connection.createStatement();
stmt.setMaxRows(50);
ResultSet rs = stmt.executeQuery("SELECT * FROM large_table");
setFetchSize()
: By setting an appropriate fetch size, applications can balance between memory usage and performance. A larger fetch size may reduce the number of database trips but increase memory usage, while a smaller fetch size does the opposite.
setMaxRows()
: This method ensures that the ResultSet
does not exceed a specified number of rows, which is beneficial when only a limited number of records are required from a potentially large dataset.
Q19. How do you handle BLOB and CLOB data types in JDBC?
Answer: In JDBC, BLOB (Binary Large Object) and CLOB (Character Large Object) are used to store large binary and text data, respectively. Handling these data types involves specific methods provided by the PreparedStatement
and ResultSet
interfaces.
Handling BLOB Data:
Inserting BLOB Data:
String sql = "INSERT INTO files (file_data) VALUES (?)";
PreparedStatement pstmt = connection.prepareStatement(sql);
InputStream inputStream = new FileInputStream(new File("path/to/file"));
pstmt.setBlob(1, inputStream);
pstmt.executeUpdate();
Retrieving BLOB Data:
String sql = "SELECT file_data FROM files WHERE file_id = ?";
PreparedStatement pstmt = connection.prepareStatement(sql);
pstmt.setInt(1, fileId);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
Blob blob = rs.getBlob("file_data");
InputStream inputStream = blob.getBinaryStream();
// Process the input stream as needed
}
Handling CLOB Data:
Inserting CLOB Data:
String sql = "INSERT INTO documents (doc_text) VALUES (?)";
PreparedStatement pstmt = connection.prepareStatement(sql);
Reader reader = new FileReader(new File("path/to/textfile"));
pstmt.setClob(1, reader);
pstmt.executeUpdate();
Retrieving CLOB Data:
String sql = "SELECT doc_text FROM documents WHERE doc_id = ?";
PreparedStatement pstmt = connection.prepareStatement(sql);
pstmt.setInt(1, docId);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
Clob clob = rs.getClob("doc_text");
Reader reader = clob.getCharacterStream();
// Process the reader as needed
}
- BLOBs are used to store large binary data such as images, audio, or video files. The
setBlob()
andgetBlob()
methods facilitate inserting and retrieving BLOB data. - CLOBs are used to store large text data. The
setClob()
andgetClob()
methods are used for inserting and retrieving CLOB data. - It’s important to manage resources properly by closing streams and readers after their usage to prevent memory leaks.
20. What is the difference between java.sql.Date
and java.util.Date
?
Answer: Both java.sql.Date
and java.util.Date
represent date values in Java, but they serve different purposes and have distinct characteristics.
java.util.Date
:
- Purpose: Represents both date and time information.
- Usage: Commonly used in general Java programming to handle date and time.
- Precision: Includes both date and time components down to milliseconds.
java.sql.Date
:
- Purpose: Represents only the date (year, month, day) without time information.
- Usage: Specifically designed for JDBC to interact with SQL
DATE
type, which does not include time. - Precision: Contains only the date component; the time part is set to the start of the day (00:00:00).
Key Differences:
Aspect | java.util.Date | java.sql.Date |
---|---|---|
Purpose | General-purpose date and time | JDBC-specific date representation |
Time Info | Includes time | Excludes time |
Usage | General Java applications | Database operations via JDBC |
When working with databases through JDBC, it’s essential to use java.sql.Date
for date columns to ensure proper handling of date values without time components. For general-purpose date and time manipulation in Java applications, java.util.Date
is appropriate.
21. What is the difference between a CallableStatement and a PreparedStatement in JDBC?
Answer: CallableStatement is used to execute stored procedures in the database, while PreparedStatement is used for executing parameterized SQL queries.
- CallableStatement allows you to call stored procedures that may have input and output parameters. It can handle complex SQL statements and can return multiple result sets.
- PreparedStatement is more efficient for executing repeated SQL statements since it is precompiled. It helps prevent SQL injection attacks by using placeholders for parameters.
Example of CallableStatement:
CallableStatement cs = conn.prepareCall("{call my_procedure(?, ?)}");
cs.setInt(1, 123);
cs.registerOutParameter(2, Types.VARCHAR);
cs.execute();
String result = cs.getString(2);
22. How do you implement transaction management in JDBC?
Answer:
Transaction management in JDBC can be implemented using the setAutoCommit
method of the Connection
interface. By default, JDBC operates in auto-commit mode, which means every SQL statement is treated as a transaction and committed automatically.
To manage transactions manually:
- Disable auto-commit mode using
setAutoCommit(false)
. - Execute your SQL statements.
- Commit the transaction using
commit()
or roll back usingrollback()
if an error occurs.
Example:
try {
conn.setAutoCommit(false);
// Execute SQL statements
conn.commit();
} catch (SQLException e) {
conn.rollback();
}
23. What is the purpose of the DatabaseMetaData interface in JDBC?
Answer: The DatabaseMetaData interface provides methods to retrieve metadata about the database, such as its structure, capabilities, and features. This includes information about tables, columns, supported SQL types, and more.
You can obtain an instance of DatabaseMetaData from a Connection object:
DatabaseMetaData metaData = conn.getMetaData();
String dbName = metaData.getDatabaseProductName();
String dbVersion = metaData.getDatabaseProductVersion();
24. Explain how to handle BLOB and CLOB data types in JDBC.
Answer: BLOB (Binary Large Object) and CLOB (Character Large Object) are used to store large binary and character data respectively.
To handle BLOBs:
- Use
PreparedStatement
to insert or update BLOB data using thesetBlob()
method. - To retrieve BLOB data, use
getBlob()
.
Example:
PreparedStatement ps = conn.prepareStatement("INSERT INTO images (img) VALUES (?)");
FileInputStream input = new FileInputStream("image.jpg");
ps.setBlob(1, input);
ps.executeUpdate();
To handle CLOBs:
- Use
setCharacterStream()
for inserting/updating CLOB data. - Use
getClob()
for retrieval.
Example:
PreparedStatement ps = conn.prepareStatement("INSERT INTO documents (doc) VALUES (?)");
Reader reader = new FileReader("document.txt");
ps.setCharacterStream(1, reader);
ps.executeUpdate();
25. What are the different types of locking mechanisms in JDBC?
Answer: JDBC supports two primary types of locking mechanisms:
- Optimistic Locking: Assumes that multiple transactions can complete without affecting each other. Locks are only applied when a transaction attempts to update data. If a conflict occurs, an exception is thrown.
- Pessimistic Locking: Locks the data as soon as it is read until the transaction completes. This prevents other transactions from accessing the locked data until the lock is released.
Example of optimistic locking might involve checking a version number before updating a record.
26. How do you perform batch processing in JDBC?
Answer: Batch processing allows you to execute multiple SQL statements in one go, improving performance by reducing round trips to the database.
To perform batch processing:
- Create a
Statement
orPreparedStatement
. - Add multiple SQL commands using
addBatch()
. - Execute all commands with
executeBatch()
.
Example:
PreparedStatement ps = conn.prepareStatement("INSERT INTO users (name) VALUES (?)");
for (String name : names) {
ps.setString(1, name);
ps.addBatch();
}
int[] results = ps.executeBatch();
27. What is connection pooling and why is it important in JDBC?
Answer: Connection pooling refers to creating a pool of database connections that can be reused rather than creating new connections for every request. This significantly improves performance by reducing the overhead associated with establishing connections.
Benefits include:
- Reduced latency in acquiring connections.
- Efficient resource management.
- Improved scalability for applications handling many concurrent users.
Connection pools can be managed by frameworks like Apache DBCP or HikariCP.
28. Explain how to retrieve metadata about ResultSet in JDBC.
Answer: You can retrieve metadata about a ResultSet using the ResultSetMetaData
interface, which provides information about the number of columns, column names, types, etc.
Example:
ResultSet rs = stmt.executeQuery("SELECT * FROM users");
ResultSetMetaData rsmd = rs.getMetaData();
int columnCount = rsmd.getColumnCount();
for (int i = 1; i <= columnCount; i++) {
String columnName = rsmd.getColumnName(i);
System.out.println("Column " + i + ": " + columnName);
}
29. What are some common exceptions thrown by JDBC?
Answer: Common exceptions include:
- SQLException: The base class for all database access errors.
- SQLTimeoutException: Indicates that a timeout has occurred while waiting for a database operation to complete.
- SQLSyntaxErrorException: Indicates that there is an error in the SQL syntax.
Handling these exceptions appropriately is crucial for robust application behavior.
30. How do you set up a connection with a database using JDBC?
Answer: To establish a connection with a database using JDBC:
- Load the JDBC driver class using
Class.forName()
. - Use
DriverManager.getConnection()
with database URL, username, and password.
Example:
Class.forName("com.mysql.cj.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password");
31. What is the significance of setFetchSize() method in ResultSet?
Answer: The setFetchSize(int rows) method specifies how many rows should be fetched from the database when more rows are needed by the application. This can improve performance by reducing network round trips when retrieving large datasets.
Example:
stmt.setFetchSize(100);
ResultSet rs = stmt.executeQuery("SELECT * FROM large_table");
32. Describe how you would use savepoints in JDBC transactions.
Answer: A Savepoint allows you to set intermediate points within a transaction so that you can roll back to that point without rolling back the entire transaction.
To use savepoints:
- Create a savepoint using
Connection.setSavepoint()
. - Roll back to that savepoint if needed using
Connection.rollback(Savepoint)
.
Example:
Savepoint sp = conn.setSavepoint();
// Perform some operations
if (someCondition) {
conn.rollback(sp); // Roll back to savepoint if condition met
}
33. How do you perform error handling in JDBC?
Answer: Error handling in JDBC involves catching exceptions thrown during database operations and taking appropriate actions based on the type of exception caught.
Use try-catch blocks around your database code:
try {
// Database operations
} catch (SQLException e) {
System.err.println("SQL Error: " + e.getMessage());
}
Additionally, always ensure resources like Connections, Statements, and ResultSets are closed properly in finally blocks or use try-with-resources for automatic resource management.
34. Explain what an SQL Injection attack is and how can it be prevented in JDBC?
Answer: SQL Injection is a code injection technique where an attacker can execute arbitrary SQL code on your database by manipulating input parameters.
To prevent SQL Injection:
- Use PreparedStatements instead of concatenating strings to create SQL queries.
- Validate user inputs rigorously.
Example of safe usage with PreparedStatement:
String sql = "SELECT * FROM users WHERE username = ?";
PreparedStatement ps = conn.prepareStatement(sql);
ps.setString(1, username); // Safe from injection
ResultSet rs = ps.executeQuery();
35. What are Type 1, Type 2, Type 3, and Type 4 JDBC drivers?
Answer: JDBC drivers are categorized into four types based on their architecture:
- Type 1 (JDBC-ODBC Bridge Driver): Translates JDBC calls into ODBC calls; not recommended for production due to performance issues.
- Type 2 (Native API Driver): Converts JDBC calls into database-specific native calls; requires native library installation on client machines.
- Type 3 (Network Protocol Driver): Uses middleware to convert JDBC calls into vendor-specific protocols; does not require client-side libraries.
- Type 4 (Thin Driver): Pure Java driver that converts JDBC calls directly into database-specific protocol; highly portable and preferred for web applications.
36. How do you execute multiple SQL statements in one go using JDBC?
Answer: You can execute multiple SQL statements by utilizing batch processing with either Statement or PreparedStatement objects as discussed earlier.
For example:
try {
conn.setAutoCommit(false); // Disable auto commit
Statement stmt = conn.createStatement();
stmt.addBatch("INSERT INTO users (name) VALUES ('Alice')");
stmt.addBatch("INSERT INTO users (name) VALUES ('Bob')");
int[] results = stmt.executeBatch(); // Execute all at once
conn.commit(); // Commit changes
} catch (SQLException e) {
conn.rollback(); // Rollback if any error occurs
}
37. What are some best practices when working with JDBC?
Answer: Best practices include:
- Always close resources like Connections, Statements, and ResultSets after use.
- Use PreparedStatements to avoid SQL injection vulnerabilities.
- Handle exceptions gracefully and log them for debugging purposes.
- Use connection pooling for better performance and resource management.
- Keep transactions small and manageable to reduce locking issues and improve concurrency.
38. How do you retrieve specific columns from a ResultSet?
Answer: When executing queries with ResultSet, you can retrieve specific columns by their index or name using methods like getInt()
, getString()
, etc., based on their type:
Example:
ResultSet rs = stmt.executeQuery("SELECT id, name FROM users");
while (rs.next()) {
int id = rs.getInt(1); // Retrieve by index
String name = rs.getString("name"); // Retrieve by column name
}
39. Explain what AutoCommit mode means in JDBC.
Answer: In AutoCommit mode, every individual SQL statement is treated as a transaction and automatically committed right after it executes successfully. This means changes are immediately saved to the database without needing explicit commit calls from your application code.
You can disable AutoCommit mode if you want finer control over transaction boundaries:
conn.setAutoCommit(false); // Disable AutoCommit mode
40. What steps would you follow to connect to an Oracle database using JDBC?
Answer: To connect to an Oracle database:
- Load the Oracle JDBC driver class using Class.forName().
- Establish a connection using DriverManager.getConnection() with appropriate URL format (
jdbc:oracle:thin:@host:port:sid
). - Handle exceptions properly during connection establishment.
Example:
Class.forName("oracle.jdbc.driver.OracleDriver");
Connection conn = DriverManager.getConnection("jdbc:oracle:thin:@localhost:1521:xe", "username", "password");
Learn More: Carrer Guidance
SAP MM interview questions and answer- Basic to Advanced
Gallagher Interview Questions and Answers for Freshers
Top Free Power BI Courses with Certificates to Boost Your Data Analysis Skills
Databricks Interview Questions and Answers- Basic to Advanced
Kafka Interview Questions and Answers- Basic to Advanced
Chella Software interview questions with detailed answers