Microservices architecture is a popular design pattern in modern software development, particularly for Java applications. Below are over 35 commonly asked interview questions along with detailed answers to help you prepare effectively.
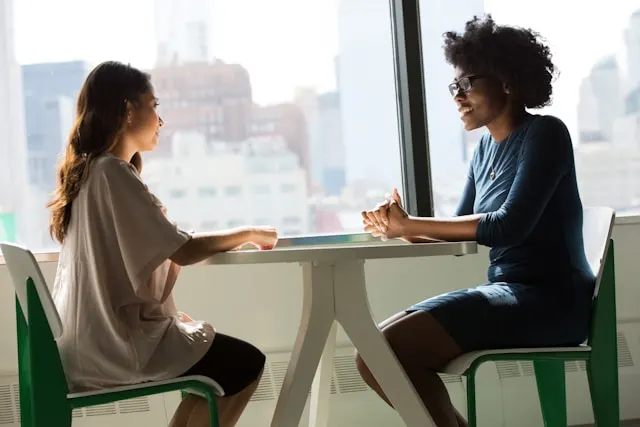
Java Microservices Interview Questions and Answers
- What are Microservices?
- How do Microservices differ from Monolithic Architecture?
- What are the key benefits of using Microservices?
- Explain the concept of API Gateway in Microservices.
- What is Service Discovery?
- How do Microservices communicate with each other?
- What is Circuit Breaker Pattern?
- What is the role of Docker in Microservices?
- Explain Continuous Integration/Continuous Deployment (CI/CD) in Microservices.
- What challenges do you face while implementing Microservices?
- How do you handle security in Microservices?
- What is Spring Cloud?
- Describe how you would implement logging in Microservices.
- What is Event Sourcing?
- Explain how you would implement monitoring in a Microservices architecture.
- What is Saga Pattern?
- How do you manage configuration in Microservices?
- What is Load Balancing in Microservices?
- Describe how you would handle versioning in Microservices APIs.
- How do you ensure data consistency across Microservices?
- How does inter-service communication work in a Microservices architecture, and what protocols are commonly used?
- What is a Sidecar Pattern, and how is it used in Microservices?
- How do you ensure fault tolerance in a Microservices architecture?
- What is Service Registry, and how is it different from Service Discovery?
- What role does API versioning play in Microservices, and how do you implement it?
- Explain the concept of distributed tracing in Microservices.
- What is an Aggregator Pattern in Microservices?
- How do you handle transactions in a Microservices environment?
- What is a Service Mesh, and how does it benefit Microservices?
- How would you implement data partitioning in Microservices?
- What is asynchronous messaging, and why is it used in Microservices?
- How do you implement caching in Microservices, and what are the benefits?
- What is a distributed database, and how does it apply to Microservices?
- How do you test Microservices?
- What is the role of API Gateway in Microservices?
- How do you implement monitoring and alerting in a Microservices environment?
- What is Reactive Microservices, and when would you use it?
1. What are Microservices?
Microservices are an architectural style that structures an application as a collection of small, loosely coupled services. Each microservice is designed to perform a specific business function and can be developed, deployed, and maintained independently. This approach contrasts with monolithic architectures, where all components are tightly integrated.
Benefits of Microservices:
- Scalability: Individual services can be scaled independently based on demand.
- Flexibility: Different services can be developed in different programming languages or frameworks.
- Resilience: Failure in one service does not affect the entire application.
2. How do Microservices differ from Monolithic Architecture?
Microservices and monolithic architecture differ fundamentally in their structure:
Feature | Microservices | Monolithic Architecture |
---|---|---|
Structure | Composed of independent services | Single, unified codebase |
Deployment | Services can be deployed independently | Requires the entire application to be redeployed |
Scalability | Individual services can be scaled | Scaling requires scaling the entire application |
Technology Stack | Different technologies for different services | Single technology stack |
Fault Isolation | Failure in one service does not impact others | A failure can bring down the entire application |
3. What are the key benefits of using Microservices?
The key benefits include:
- Improved Agility: Teams can develop, test, and deploy services independently, accelerating time-to-market.
- Better Fault Isolation: Issues in one service do not cascade to others, enhancing overall system reliability.
- Technology Diversity: Teams can choose the best technology stack for each service without being constrained by a single technology choice.
4. Explain the concept of API Gateway in Microservices.
An API Gateway acts as a single entry point for all client requests to various microservices. It handles requests by routing them to the appropriate microservice and aggregating the results. Key functions include:
- Request Routing: Directs incoming requests to the correct microservice.
- Load Balancing: Distributes incoming traffic across multiple instances of a service.
- Security: Provides authentication and authorization mechanisms.
- Rate Limiting: Controls how many requests a client can make in a given time period.
5. What is Service Discovery?
Service Discovery is a mechanism that allows microservices to find each other dynamically. In a microservices architecture, as instances of services come and go (due to scaling or failures), they register themselves with a Service Registry (like Eureka or Consul). Other services query this registry to discover the location of services they need to communicate with.
6. How do Microservices communicate with each other?
Microservices typically communicate through:
- RESTful APIs: Using HTTP/HTTPS protocols for synchronous communication.
- Message Brokers: For asynchronous communication (e.g., RabbitMQ, Kafka).
- gRPC: A high-performance RPC framework that uses HTTP/2 for transport and Protocol Buffers for serialization.
7. What is Circuit Breaker Pattern?
The Circuit Breaker Pattern is used to prevent cascading failures in microservices. It monitors calls to a service and opens a circuit when failures exceed a certain threshold. This prevents further calls to the failing service for a specified period, allowing it time to recover before attempting to call it again.
States of Circuit Breaker:
- Closed: Normal operation; requests are allowed.
- Open: Requests are blocked; errors are returned immediately.
- Half-Open: A limited number of requests are allowed; if they succeed, the circuit closes again.
8. What is the role of Docker in Microservices?
Docker is used extensively in microservices architecture for containerization. Each microservice runs in its own container, which encapsulates everything needed to run it (code, runtime, libraries). This ensures consistency across different environments (development, testing, production) and simplifies deployment processes.
9. Explain Continuous Integration/Continuous Deployment (CI/CD) in Microservices.
CI/CD refers to practices that automate the integration and deployment of code changes:
- Continuous Integration (CI): Developers frequently merge their code changes into a shared repository where automated builds and tests run to ensure code quality.
- Continuous Deployment (CD): Changes that pass CI tests are automatically deployed to production, enabling rapid delivery of new features and bug fixes.
10. What challenges do you face while implementing Microservices?
Common challenges include:
- Data Management: Ensuring data consistency across multiple services can be complex.
- Service Coordination: Managing interactions between numerous services can lead to increased complexity.
- Monitoring and Logging: Keeping track of various services requires robust monitoring tools and centralized logging solutions.
11. How do you handle security in Microservices?
Security in microservices involves several strategies:
- API Security: Use OAuth2 or JWT for securing APIs.
- Service-to-Service Security: Implement mutual TLS for secure communication between services.
- Data Encryption: Encrypt sensitive data both at rest and in transit.
12. What is Spring Cloud?
Spring Cloud provides tools for developers to quickly build some of the common patterns in distributed systems such as configuration management, service discovery (Eureka), circuit breakers (Hystrix), and API gateway (Zuul). It simplifies the development of microservices by providing ready-to-use solutions for these patterns.
13. Describe how you would implement logging in Microservices.
Implementing logging involves:
- Centralized Logging Solutions: Use tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk to aggregate logs from different services.
- Structured Logging: Use structured logs (JSON format) for easier searching and filtering.
- Correlation IDs: Include unique identifiers in logs for tracing requests across multiple services.
14. What is Event Sourcing?
Event Sourcing is an architectural pattern where state changes are stored as a sequence of events rather than just storing the current state. This allows reconstruction of past states by replaying events which can be beneficial for auditing and debugging purposes.
15. Explain how you would implement monitoring in a Microservices architecture.
Monitoring can be implemented through:
- Metrics Collection: Use tools like Prometheus or Grafana to collect metrics from each service.
- Health Checks: Implement health endpoints that return the status of each service.
- Alerting Systems: Set up alerts based on specific metrics thresholds using tools like Alertmanager or PagerDuty.
16. What is Saga Pattern?
The Saga Pattern manages distributed transactions across multiple microservices by breaking them into smaller transactions that can be executed independently. Each transaction publishes events upon completion which trigger subsequent transactions or compensating actions if needed.
17. How do you manage configuration in Microservices?
Configuration management can be handled using:
- Centralized Configuration Servers: Tools like Spring Cloud Config allow external configuration management across all environments.
- Environment Variables: Use environment-specific variables during deployment for sensitive information like database credentials or API keys.
18. What is Load Balancing in Microservices?
Load balancing distributes incoming network traffic across multiple instances of a service to ensure no single instance becomes overwhelmed with traffic. This improves responsiveness and availability while ensuring optimal resource utilization.
19. Describe how you would handle versioning in Microservices APIs.
API versioning can be implemented through:
- URI Versioning: Including version numbers directly in the URL (e.g.,
/api/v1/resource
). - Header Versioning: Using custom headers to specify versions.
- Query Parameter Versioning: Adding version information as query parameters (e.g.,
/api/resource?version=1
).
20. How do you ensure data consistency across Microservices?
Data consistency can be ensured through:
- Eventual Consistency: Accept that immediate consistency may not always be possible; instead, use mechanisms like message queues or event sourcing.
- Distributed Transactions: Implement patterns like Saga or Two-phase commit where applicable but be cautious about their complexity and performance implications.
21. How does inter-service communication work in a Microservices architecture, and what protocols are commonly used?
Answer:
In Microservices, inter-service communication is typically handled using RESTful HTTP APIs or messaging systems. For synchronous communication, HTTP/REST or gRPC is commonly used due to their ease of use and stateless nature. For asynchronous communication, message brokers like RabbitMQ, Apache Kafka, or ActiveMQ are preferred as they allow for event-driven interactions and higher resilience to service failures. Choosing between synchronous and asynchronous communication depends on the use case; synchronous communication is usually more suitable for immediate responses, while asynchronous can handle longer processing times and decouple services effectively.
22. What is a Sidecar Pattern, and how is it used in Microservices?
Answer:
The Sidecar Pattern involves deploying a helper service (sidecar) alongside the main Microservice to handle supplementary functions like logging, monitoring, or security. This sidecar runs in the same environment as the main service but is isolated, ensuring that ancillary functionalities are handled without modifying the core service logic. This pattern is commonly used with service meshes like Istio, which implement sidecars to manage networking and security in Microservices architectures. The sidecar facilitates modularity and reduces code duplication.
23. How do you ensure fault tolerance in a Microservices architecture?
Answer:
Fault tolerance is achieved by implementing patterns like Circuit Breaker, Retry, and Bulkhead patterns. The Circuit Breaker prevents cascading failures by cutting off requests to a failing service, allowing it time to recover. The Retry pattern automatically retries failed requests to increase resilience, while the Bulkhead pattern isolates service failures by allocating resources per service to prevent overloading. These patterns, coupled with monitoring tools, ensure that the system can handle individual service failures without collapsing.
24. What is Service Registry, and how is it different from Service Discovery?
Answer:
A Service Registry is a database that stores information about available Microservices and their instances. Service Discovery, on the other hand, is the process of finding instances of a service, often using information from the Service Registry. Service Registries often use tools like Eureka or Consul to register services dynamically, enabling other services to locate and communicate with them. In short, the registry holds information, while discovery is the mechanism to access that information for communication purposes.
25. What role does API versioning play in Microservices, and how do you implement it?
Answer:
API versioning is crucial in Microservices to allow for backward compatibility and to ensure that older clients can still interact with updated services. Common versioning techniques include URI versioning (e.g., /api/v1/resource
), header versioning, and query parameter versioning. URI versioning is straightforward and commonly used in REST APIs, while header versioning keeps URIs clean. Choosing a versioning strategy depends on the system’s needs, but ensuring backward compatibility is a critical part of maintaining a stable Microservices ecosystem.
26. Explain the concept of distributed tracing in Microservices.
Answer:
Distributed tracing allows you to track requests as they propagate across various Microservices, providing insights into each service’s latency and potential bottlenecks. Tools like Zipkin and Jaeger capture trace data, often including unique trace IDs passed across services. Distributed tracing is invaluable for debugging complex flows, identifying slow services, and providing end-to-end visibility across the entire request chain. It’s an essential component of observability in Microservices environments.
27. What is an Aggregator Pattern in Microservices?
Answer:
The Aggregator Pattern is used when a service needs to gather data from multiple Microservices and aggregate it into a single response. This pattern is often employed in API Gateway implementations, where the gateway makes concurrent calls to several services, collects responses, and returns a consolidated result to the client. It helps in reducing round-trip time and simplifies client interaction by providing a single entry point to access multiple services’ data.
28. How do you handle transactions in a Microservices environment?
Answer:
Transactions in Microservices, especially those involving multiple services, are often managed using the Saga pattern instead of traditional ACID transactions. In a Saga, each service executes a local transaction and publishes an event to trigger the next service’s transaction. If any step fails, compensating actions are triggered to undo the previous steps. Two common Saga implementations are choreography (event-driven) and orchestration (centralized controller).
29. What is a Service Mesh, and how does it benefit Microservices?
Answer:
A Service Mesh is an infrastructure layer that facilitates secure and reliable service-to-service communication in Microservices. It typically manages traffic, handles service discovery, and enables advanced networking functionalities like load balancing, failure recovery, and observability. Examples include Istio and Linkerd. A Service Mesh decouples these concerns from application code, promoting cleaner code and centralized traffic management, making it easier to scale and secure the Microservices environment.
30. How would you implement data partitioning in Microservices?
Answer:
Data partitioning, or sharding, involves dividing a dataset into distinct parts to improve scalability and performance. In Microservices, each service may have its own database, and data partitioning can be achieved by partitioning based on data attributes (like customer ID or region). This approach reduces the load on individual databases, improves response times, and aligns with the concept of database per service, ensuring each service can scale independently.
31. What is asynchronous messaging, and why is it used in Microservices?
Answer:
Asynchronous messaging enables services to communicate without waiting for an immediate response, enhancing performance and decoupling services. This is achieved through message brokers like RabbitMQ or Apache Kafka, which act as intermediaries to deliver messages to intended recipients. Asynchronous messaging is especially useful in long-running tasks, such as background processing, and improves reliability since services can function independently of each other.
32. How do you implement caching in Microservices, and what are the benefits?
Answer:
Caching in Microservices can be implemented at various levels, including service-level caching (Redis, Memcached), HTTP caching, or even within API gateways. Caching reduces database load and improves response times by storing frequently accessed data. It’s essential to handle cache invalidation carefully to ensure data accuracy. In distributed systems, a distributed cache is often used, allowing data to be synchronized across different instances.
33. What is a distributed database, and how does it apply to Microservices?
Answer:
A distributed database is a system where data is stored across multiple servers, ensuring data availability and fault tolerance. In Microservices, distributed databases support the principle of a database per service by allowing data to reside close to the services that need it. Examples include Cassandra and MongoDB. Distributed databases enable horizontal scaling, essential for handling large volumes of data in Microservices architectures.
34. How do you test Microservices?
Answer:
Testing Microservices requires unit, integration, contract, and end-to-end tests. Unit tests validate individual components, while integration tests assess service interactions. Contract tests are used to verify that services meet the expectations of their consumers. End-to-end tests ensure the complete system works as intended. Each type of testing plays a unique role in verifying functionality, ensuring reliability, and detecting issues across the Microservices architecture.
35. What is the role of API Gateway in Microservices?
Answer:
The API Gateway is a single entry point for clients to interact with multiple Microservices, handling request routing, composition, and protocol translation. It simplifies client-side interactions, enables cross-cutting concerns like authentication, rate limiting, and caching, and can also implement security measures. API Gateways like Netflix Zuul and Spring Cloud Gateway are popular in Microservices environments for streamlining and managing service requests.
36. How do you implement monitoring and alerting in a Microservices environment?
Answer:
Monitoring in Microservices involves collecting metrics, logs, and traces. Tools like Prometheus (metrics), ELK Stack (logs), and Jaeger (traces) are commonly used. Alerting is set up to notify on thresholds or unusual patterns. A well-monitored system can detect performance issues and avoid service downtimes, while alerting ensures rapid response to incidents. Monitoring tools are essential to maintain observability and uphold system reliability.
37. What is Reactive Microservices, and when would you use it?
Answer:
Reactive Microservices refer to services that are designed to be resilient, elastic, and responsive, handling asynchronous data streams. They follow the principles outlined in the Reactive Manifesto. Reactive Microservices are ideal for high-throughput applications where responsiveness is critical, as they can handle high loads by distributing work across non-blocking threads. Frameworks like Spring WebFlux are used to create reactive applications in Java.
Learn More: Carrer Guidance
OOP Interview Questions with Detailed Answers
React JS Interview Questions with Detailed Answers for Freshers
Cypress Interview Questions with Detailed Answers
PySpark interview questions and answers
Salesforce admin interview questions and answers for experienced
Salesforce admin interview questions and answers for freshers
EPAM Systems Senior Java Developer Interview questions with answers