Cypress is a popular end-to-end testing framework designed for modern web applications. Here are over 40 essential Cypress interview questions along with detailed answers to help you prepare effectively.
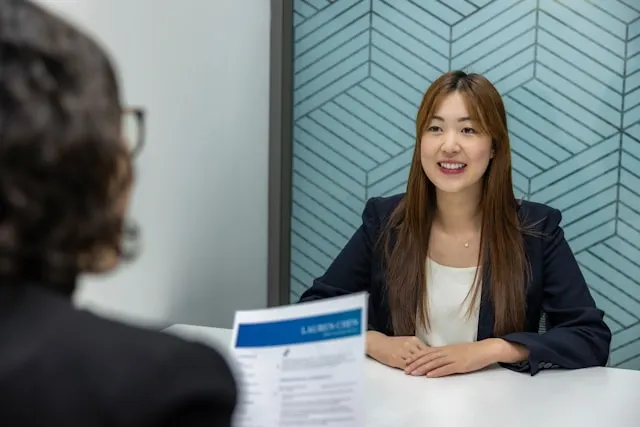
Cypress Interview Questions and Answers
1. What is Cypress?
2. How do you install Cypress?
3. How do you open Cypress?
4. What are some key features of Cypress?
5. What is the difference between cy.get() and cy.contains()?
6. How do you handle asynchronous operations in Cypress?
7. Explain how fixtures work in Cypress.
8. How do you perform API testing with Cypress?
9. What are custom commands in Cypress?
10. How do you handle timeouts in Cypress?
11. What is the purpose of beforeEach() and afterEach() hooks?
12. How can you manage environment variables in Cypress?
13. Describe how to handle iframes in Cypress.
14. What are some common assertions used in Cypress?
15. How do you take screenshots and record videos during tests?
16. Explain how to work with dynamic content in Cypress.
17. How do you implement Page Object Model (POM) with Cypress?
18. How do you debug failing tests in Cypress?
19. Can you explain what Shadow DOM is and how to interact with it using Cypress?
20. What are some best practices for writing tests in Cypress?
21. How does Cypress differ from Selenium, and what are some advantages of Cypress?
22. How does Cypress handle cross-browser testing, and what are the limitations?
23. What is the Cypress Test Runner, and how does it help in debugging?
24. Explain the role of the Cypress Dashboard Service. How is it beneficial?
25. How can Cypress be integrated with Continuous Integration (CI) systems?
26. What is the difference between cy.route() and cy.intercept() in Cypress?
27. How do you handle flaky tests in Cypress?
28. How do you perform data-driven testing in Cypress?
29. What is Cypress’s approach to handling browser security policies, like CORS?
30. How does Cypress support mobile testing, and what are its limitations?
31. How can you verify HTTP response status codes in Cypress?
32. How do you test file uploads in Cypress?
33. What are cy.spy() and cy.stub(), and how are they used?
34. How does Cypress handle JavaScript alerts and confirm dialogs?
35. How do you work with date and time in Cypress tests?
36. What is the purpose of cy.wrap(), and when should you use it?
37. Explain Cypress’s support for BDD-style testing.
38. What are Cypress commands cy.exec() and cy.task(), and when do you use them?
39. How can you validate UI elements that are hidden or visible only on user interaction?
40. What is the best way to clean up or reset data between Cypress tests?
1. What is Cypress?
Answer:
Cypress is an open-source JavaScript-based testing framework primarily used for end-to-end testing of web applications. It operates directly in the browser, allowing developers to write and execute tests in real-time. Key features include:
- Automatic Waiting: Cypress automatically waits for elements to appear before interacting with them, eliminating the need for manual waits.
- Real-Time Reloads: Any changes made to the tests are reflected immediately in the browser.
- Debugging Capabilities: Cypress provides an interactive test runner that allows developers to inspect elements and debug tests easily.
- Screenshots and Videos: Cypress can capture screenshots and record videos of tests, which helps in analyzing test failures.
2. How do you install Cypress?
Answer:
To install Cypress, you first need Node.js installed on your machine. Once Node.js is set up, you can install Cypress using npm (Node Package Manager) with the following command:
npm install cypress --save-dev
This command installs Cypress as a development dependency in your project.
3. How do you open Cypress?
Answer:
After installing Cypress, you can open it using the following command:
npx cypress open
This command launches the Cypress Test Runner, where you can select and run your test files interactively.
4. What are some key features of Cypress?
Answer:
Cypress offers several features that make it stand out among testing frameworks:
- Time Travel: You can hover over commands in the Test Runner to see snapshots of your application at each step.
- Network Traffic Control: Cypress allows you to stub and control network requests, making it easier to test various scenarios without relying on an external server.
- Consistent Execution: Tests run in the same run loop as the application, providing faster execution compared to traditional frameworks like Selenium.
5. What is the difference between cy.get()
and cy.contains()
?
Answer:
cy.get(selector)
is used to select elements based on a CSS selector. It returns a jQuery object containing all matching elements.
Example: cy.get('.btn-submit').click();
cy.contains(text)
searches for an element that contains specific text. This method is particularly useful when you want to find an element without knowing its exact selector.
Example: cy.contains('Submit').click();
6. How do you handle asynchronous operations in Cypress?
Answer:
Cypress handles asynchronous operations automatically through its built-in waiting mechanism. When you use commands like cy.get()
, it waits for the element to appear before proceeding to the next command. You can also use .then()
to work with promises explicitly if needed.
Example:
cy.get('.btn-submit').should('be.visible').click().then(() => {
// Additional actions after click
});
7. Explain how fixtures work in Cypress.
Answer:
Fixtures are used in Cypress to manage test data separately from your test scripts. They allow you to load static data from files (usually JSON) that can be reused across multiple tests.To use fixtures:
- Place your fixture files in the
cypress/fixtures
directory. - Load them using
cy.fixture()
within your tests.
Example:
cy.fixture('user.json').then((user) => {
cy.get('input[name="username"]').type(user.username);
});
8. How do you perform API testing with Cypress?
Answer:
Cypress provides a straightforward way to perform API testing using the cy.request()
command, which allows you to send HTTP requests and validate responses.
Example:
cy.request('GET', 'https://api.example.com/users')
.its('status')
.should('eq', 200);
9. What are custom commands in Cypress?
Answer:
Custom commands allow you to define reusable functions that encapsulate complex actions or sequences of actions within your tests. You can add custom commands globally by extending the Cypress.Commands
object.
Example:
Cypress.Commands.add('login', (username, password) => {
cy.get('#username').type(username);
cy.get('#password').type(password);
cy.get('#login-button').click();
});
You can then call this command in your tests like so:
cy.login('myUser', 'myPassword');
10. How do you handle timeouts in Cypress?
Answer:
Cypress has default timeout settings for various commands, which can be adjusted globally or per command basis using .timeout()
. You can also set custom timeouts in your configuration file (cypress.json
).
Example of per-command timeout:
cy.get('.btn-submit', { timeout: 10000 }).should('be.visible');
11. What is the purpose of beforeEach()
and afterEach()
hooks?
Answer:
These hooks are used for setting up preconditions and cleaning up after tests:
beforeEach()
: Runs before each test case within a suite, allowing you to set up common state or reset conditions.
Example:
beforeEach(() => {
cy.visit('/login');
});
afterEach()
: Runs after each test case, useful for cleanup tasks like logging out or resetting data.
Example:
afterEach(() => {
cy.logout();
});
12. How can you manage environment variables in Cypress?
Answer:
You can define environment variables in the cypress.json
configuration file or pass them via CLI when running tests. To access these variables within your tests, use Cypress.env('variableName')
.
Example:
{
Accessing it in a test:
"env": {
"apiUrl": "https://api.example.com"
}
}
cy.request(`${Cypress.env('apiUrl')}/users`);
13. Describe how to handle iframes in Cypress.
Answer:
Handling iframes requires a specific approach since they create a separate browsing context. You typically need to use a plugin like cypress-iframe
or manually access iframe contents using JavaScript.Using the plugin:
- Install it via npm:
npm install -D cypress-iframe
- Include it in your support file (
cypress/support/index.js
):import 'cypress-iframe';
- Access iframe elements:
cy.frameLoaded('#iframe-id');
cy.iframe().find('.inside-iframe-element').click();
14. What are some common assertions used in Cypress?
Answer:
Cypress integrates with Chai assertion library, allowing various assertions such as:
.should()
: For asserting conditions.
Example: cy.get('.todo-item').should('have.length', 3);
.expect()
: For traditional assertion style.
Example: expect(true).to.equal(true);
15. How do you take screenshots and record videos during tests?
Answer:
Cypress automatically captures screenshots on failure by default, but you can also manually trigger screenshots using cy.screenshot()
within your tests.To enable video recording during test runs, ensure it’s configured in your cypress.json
file:
{
"video": true,
}
Videos will be recorded during CI runs by default.
16. Explain how to work with dynamic content in Cypress..
Answer:
When dealing with dynamic content that may take time to load (like AJAX calls), utilize assertions that wait for conditions rather than fixed timeouts.For example:
cy.get('.dynamic-element').should('be.visible');
This command will wait until .dynamic-element
appears before proceeding.
17. How do you implement Page Object Model (POM) with Cypress?
Answer:
The Page Object Model is a design pattern that helps organize code by creating separate classes or objects for different pages of your application.
- Create a separate file for each page (e.g.,
loginPage.js
). - Define methods representing actions on that page.
- Import and use these methods in your test files.
Example of a simple page object:
// loginPage.js
class LoginPage {
visit() {
cy.visit('/login');
}
fillUsername(username) {
cy.get('#username').type(username);
}
fillPassword(password) {
cy.get('#password').type(password);
}
submit() {
cy.get('#login-button').click();
}
}
export default new LoginPage();
Using it in a test:
import loginPage from '../support/loginPage';
describe('Login Test', () => {
it('should log in successfully', () => {
loginPage.visit();
loginPage.fillUsername('user');
loginPage.fillPassword('pass');
loginPage.submit();
});
});
18. How do you debug failing tests in Cypress?
Answer:
Cypress provides several debugging tools:
- Interactive Test Runner: Use it to step through tests visually and inspect elements at each step.
- Console Logs: Add logs using
cy.log()
or standard JavaScript console logs within your tests. - Debugging Commands: Use
.debug()
on any command chain to pause execution at that point.
Example: cy.get('.element').debug().should('be.visible');
19. Can you explain what Shadow DOM is and how to interact with it using Cypress?
Answer:
Shadow DOM allows developers to encapsulate styles and markup so they don’t interfere with other parts of the document. To interact with Shadow DOM elements, you’ll need specific commands since they are not part of the main DOM tree. Using native JavaScript methods or plugins like cypress-shadow-dom
, you can access shadow elements:
Example using native methods:
cy.get('#shadow-host')
.shadow()
.find('.shadow-element')
.click();
20. What are some best practices for writing tests in Cypress?
Answer:
To write effective tests using Cypress:
- Keep Tests Independent: Ensure each test case can run independently without relying on previous ones.
- Use Descriptive Names: Name your test cases clearly so their purpose is easily understood.
- Avoid Hard-Coding Values: Use fixtures or environment variables instead of hard-coded values where possible.
- Organize Tests Logically: Group related tests together using
describe()
blocks.
By following these guidelines, you’ll create maintainable and reliable test suites that enhance productivity and reduce debugging time.
21. How does Cypress differ from Selenium, and what are some advantages of Cypress?
Answer:
Cypress and Selenium serve similar purposes in web automation testing but differ significantly in architecture and functionality. Selenium operates outside the browser and communicates with it via WebDriver, making it compatible with multiple browsers. Cypress, on the other hand, runs directly in the browser, giving it access to JavaScript events and making tests faster and more reliable. Some advantages of Cypress include automatic waiting, a robust debugging interface, time-travel feature, and simple setup without external dependencies.
22. How does Cypress handle cross-browser testing, and what are the limitations?
Answer:
Cypress initially supported only Chrome-family browsers due to its architecture, but it has expanded to include Firefox and WebKit (used by Safari). However, Cypress doesn’t offer full cross-browser support for all features, particularly advanced configurations or certain browser-specific functions. This limitation is due to its reliance on a single JavaScript execution environment, which doesn’t perfectly emulate other browser environments.
23. What is the Cypress Test Runner, and how does it help in debugging?
Answer:
The Cypress Test Runner is a visual interface that allows you to view tests as they run in real-time. It helps in debugging by allowing developers to view snapshots of each command, use “time-travel” to examine the application state at each step, and inspect DOM elements directly. This interface also logs errors and failed assertions, providing more context and easing troubleshooting.
24. Explain the role of the Cypress Dashboard Service. How is it beneficial?
Answer:
The Cypress Dashboard Service is a cloud-based tool that records test runs, tracks performance, and provides insights into test metrics. It allows you to parallelize tests across CI environments, manage test history, and get detailed failure analysis. This service is beneficial for teams needing to analyze tests over time, detect flaky tests, and improve overall test efficiency.
25. How can Cypress be integrated with Continuous Integration (CI) systems?
Answer:
Cypress can be integrated with CI systems like Jenkins, CircleCI, GitHub Actions, and others by using its CLI for running tests. This can be achieved by installing Cypress in the CI pipeline, configuring environment variables, and running cypress run
to execute tests. Cypress also provides documentation and support for setting up headless browsers in CI environments to facilitate smooth integration.
26. What is the difference between cy.route()
and cy.intercept()
in Cypress?
Answer:
cy.route()
was the original way to handle network stubbing in Cypress but is now deprecated in favor of cy.intercept()
. cy.intercept()
provides a more flexible and powerful way to intercept and mock HTTP requests, allowing you to modify requests, add conditions, and test various API responses directly.
27. How do you handle flaky tests in Cypress?
Answer:
Flaky tests in Cypress can be managed through techniques such as retrying failed assertions, using custom commands to handle retries, and employing Cypress commands like cy.wait()
to ensure application stability. Cypress also allows configuring global and command-specific timeouts, which can reduce flakiness by waiting for application states to stabilize.
28. How do you perform data-driven testing in Cypress?
Answer:
Data-driven testing in Cypress can be accomplished by using fixtures to load test data, using JavaScript arrays to iterate over sets of data, or creating custom commands to handle multiple inputs. For complex scenarios, Cypress provides cy.each()
and allows using JSON
files to store data, supporting dynamic input within the same test case.
29. What is Cypress’s approach to handling browser security policies, like CORS?
Answer:
Cypress automatically bypasses certain browser security restrictions, such as CORS, by running in the same execution context as the application. This setup allows it to access all resources directly. However, Cypress also offers options to set headers or modify the server configurations for specific needs, such as handling authentication tokens.
30. How does Cypress support mobile testing, and what are its limitations?
Answer:
Cypress provides support for responsive testing by allowing users to set viewport sizes to mimic mobile devices, using cy.viewport()
. While this works well for layout testing, Cypress currently does not support native mobile app testing, so it’s limited to testing responsive web applications rather than true mobile devices or applications.
31. How can you verify HTTP response status codes in Cypress?
Answer:
To verify HTTP response status codes, Cypress allows using the cy.intercept()
command to listen for specific network requests and inspect the response. Assertions can then be made on the status code or response body to confirm that the API behaves as expected, like cy.intercept('GET', '/api/path').as('apiCall')
.
32. How do you test file uploads in Cypress?
Answer:
Cypress supports file uploads by using the cy.get()
command to select the file input element and the cy.attachFile()
command to simulate file selection. The attachFile()
method is available via third-party plugins, such as cypress-file-upload
, which provides an easy way to upload files in test cases.
33. What are cy.spy()
and cy.stub()
, and how are they used?
Answer:
cy.spy()
is used to listen to a function and check how many times it has been called without altering its behavior. cy.stub()
replaces a function with a mock implementation, which is useful for isolating and testing specific functions or actions without depending on their actual logic.
34. How does Cypress handle JavaScript alerts and confirm dialogs?
Answer:
Cypress automatically handles alerts and confirm dialogs without manual interaction by simulating clicks on these dialog buttons. For custom handling, you can use cy.on('window:alert', (text) => {...})
or cy.on('window:confirm', () => {...})
to assert on the dialog content or customize the behavior.
35. How do you work with date and time in Cypress tests?
Answer:
Cypress provides utilities to mock dates and times using cy.clock()
and cy.tick()
, allowing you to manipulate time-sensitive functions like animations or countdowns. This is particularly useful for testing time-dependent functionality by creating a controlled environment.
36. What is the purpose of cy.wrap()
, and when should you use it?
Answer:
cy.wrap()
is used to turn a non-Cypress value, such as a JavaScript object or promise, into a Cypress object, allowing Cypress commands to interact with it. This is helpful for chaining Cypress commands or working with external functions or libraries within Cypress test cases.
37. Explain Cypress’s support for BDD-style testing.
Answer:
Cypress supports BDD (Behavior-Driven Development) by allowing integration with libraries like cypress-cucumber-preprocessor
, which enables writing test scenarios in Gherkin syntax (Given, When, Then). This makes tests more readable and bridges the gap between technical and non-technical stakeholders.
38. What are Cypress commands cy.exec()
and cy.task()
, and when do you use them?
Answer:
cy.exec()
runs terminal commands directly from Cypress, which is useful for tasks like resetting databases. cy.task()
allows executing Node.js code from the backend, such as accessing files or performing calculations. These commands enable integration with external systems or complex data manipulation.
39. How can you validate UI elements that are hidden or visible only on user interaction?
Answer:
Cypress provides commands like should('not.be.visible')
and should('be.visible')
for checking element visibility. For elements appearing on user actions, Cypress can interact with triggers like hover, focus, or click using cy.trigger()
to simulate user actions and validate the UI state change.
40. What is the best way to clean up or reset data between Cypress tests?
Answer:
To maintain test independence, Cypress offers hooks like beforeEach()
and afterEach()
to set up or reset application state. Cleaning up can be done via API calls to reset data, clearing local storage, or using Cypress commands like cy.clearCookies()
and cy.clearLocalStorage()
.
Learn More: Carrer Guidance
PySpark interview questions and answers
Salesforce admin interview questions and answers for experienced
Salesforce admin interview questions and answers for freshers
EPAM Systems Senior Java Developer Interview questions with answers
Flutter Interview Questions and Answers for all levels
Most common data structures and algorithms asked in Optum interviews