PySpark, the Python API for Apache Spark, is increasingly popular for big data processing and analytics. Below are 55+ essential PySpark interview questions, along with detailed answers to help you prepare for your next interview.
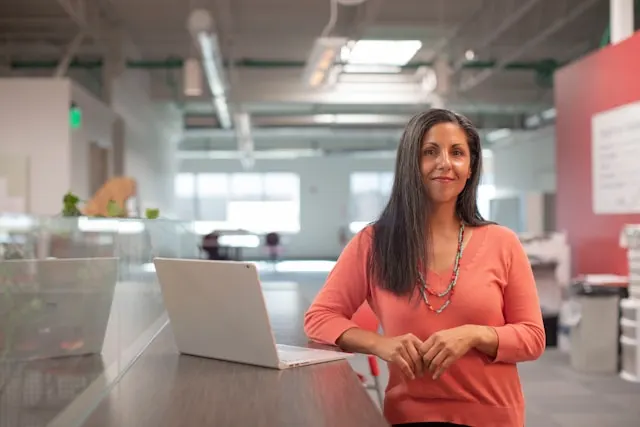
Top PySpark interview questions and answers
1. What is PySpark, and how does it differ from Apache Spark?
2. Explain the concept of an RDD in PySpark.
3. Explain the difference between RDD, DataFrame, and DataSet.
4. What are the advantages of using PySpark?
5. How does PySpark handle missing data?
6. What is the role of the Spark Driver?
7. Explain transformations and actions in PySpark.
8. What are wide and narrow transformations?
9. How do you optimize performance in PySpark?
10. What is the purpose of checkpoints in PySpark?
11. Describe how you would implement a custom transformation in PySpark.
12. What is MLlib in PySpark?
13. How do you handle errors in PySpark?
14. What are the key differences between DataFrames and Pandas DataFrames?
15. What is the significance of Partitions in PySpark?
16. How do you create a DataFrame from an existing RDD?
17. Explain how you would read data from different sources using PySpark.
18. What are User Defined Functions (UDFs) in PySpark?
19. How do you manage dependencies in PySpark applications?
20. What are DataFrames in PySpark, and why are they preferred over RDDs?
21. How do you create a DataFrame in PySpark?
22. Describe PySpark’s Catalyst Optimizer.
23. Explain the role of the SparkSession in PySpark.
24. How can PySpark be used for handling missing data?
25. What are transformations and actions in PySpark? Provide examples.
26. What is lazy evaluation in PySpark, and why is it beneficial?
27. What are accumulators and broadcast variables in PySpark?
28. How do you handle schema definition in PySpark DataFrames?
29. Explain the difference between map() and flatMap() in PySpark.
30. How is partitioning managed in PySpark?
31. How does PySpark handle data persistence and caching?
32. Describe a use case for join operations in PySpark.
33. What is groupBy() in PySpark, and how is it used?
34. How does PySpark handle file formats like CSV, JSON, and Parquet?
35. What are PySpark UDFs, and how are they used?
36. Explain the difference between narrow and wide transformations in PySpark.
37. What are window functions in PySpark, and how are they used?
38. What are the different ways of persisting data in PySpark, and how do they impact performance?
39. How does PySpark handle parallel processing, and what configurations can optimize it?
40. Explain the purpose and usage of foreachPartition() in PySpark.
41. What is PySpark’s explain() function, and how can it be used for query optimization?
42. How does PySpark handle data skew, and what techniques mitigate it?
43. What are broadcast joins in PySpark, and how do they improve performance?
44. Describe the role of Window functions in data analysis with PySpark.
45. How do you handle large file ingestion in PySpark for efficient processing?
46. Explain repartition() vs. coalesce() in PySpark.
47. What is the difference between collect() and take() in PySpark?
48. Explain how PySpark manages fault tolerance.
49. How can you optimize joins in PySpark?
50. What is PySpark SQL, and how does it integrate with DataFrames?
51. How do you handle time-based data in PySpark?
52. What is Spark MLlib, and how does it work in PySpark?
53. Explain the concept of shuffling in PySpark.
54. What is the role of mapPartitions() in PySpark, and how does it differ from map()?
55. How does PySpark integrate with Hive, and what are its benefits?
56. Describe the purpose and structure of a PySpark pipeline.
57. What are PySpark’s partitioning strategies, and how do they affect performance?
1. What is PySpark, and how does it differ from Apache Spark?
Answer:
PySpark is the Python API for Apache Spark, allowing users to interface Spark with Python. Spark itself is a powerful open-source framework for big data processing that supports various languages (Java, Scala, Python, R). PySpark offers the flexibility of Spark’s fast in-memory processing along with Python’s ease of use.
A key difference between PySpark and Spark is the language API, where PySpark enables Pythonic programming, and it integrates Python libraries such as Pandas and NumPy, useful for data manipulation and analysis. However, Python code in PySpark may perform slightly slower than native Spark in Scala due to inter-language operations.
2. Explain the concept of an RDD in PySpark.
Answer:
An RDD (Resilient Distributed Dataset) is PySpark’s core data structure representing a distributed collection of data across clusters. RDDs are fault-tolerant, meaning they can recover from node failures and recompute missing data via lineage (the history of transformations applied to the RDD).
RDDs support two types of operations: transformations (like map
, filter
) which define a new RDD and are lazily evaluated, and actions (like collect
, count
) which trigger the execution. This lazy evaluation enables PySpark to optimize execution plans and improve performance.
3. Explain the difference between RDD, DataFrame, and DataSet.
Answer:
Feature | RDD | DataFrame | DataSet |
---|---|---|---|
Type | Unstructured | Structured | Strongly typed |
Schema | No schema | Schema defined by columns | Schema defined with types |
Performance | Lower due to lack of optimizations | Higher due to Catalyst optimizer | Higher due to both optimizations |
API | Functional API | SQL-like API | Combination of both |
RDDs are ideal for low-level transformations, while DataFrames and Datasets provide higher-level abstractions with optimizations.
4. What are the advantages of using PySpark?
Answer:
- Scalability: PySpark can handle large datasets across multiple nodes.
- Performance: It leverages in-memory processing to speed up computations.
- Fault Tolerance: RDDs can recover from failures through lineage.
- Integration: Works seamlessly with other big data tools in the Apache ecosystem.
- Ease of Use: Provides a simple interface for complex operations.
5. How does PySpark handle missing data?
Answer: PySpark provides several methods to handle missing data:
- Drop Rows: Use
dropna()
to remove rows with null values. - Fill Values: Use
fillna(value)
to replace null values with a specified value. - Imputation: For more advanced handling, use
Imputer
frompyspark.ml.feature
to fill missing values based on statistical methods like mean or median.
6. What is the role of the Spark Driver?
Answer:
The Spark Driver is the main program that coordinates the execution of tasks in a Spark application. It converts user code into tasks and schedules them across the cluster. The driver maintains information about the Spark application and communicates with the cluster manager to allocate resources.
7. Explain transformations and actions in PySpark.
Answer:
Transformations are operations that create a new RDD from an existing one without executing immediately (lazy evaluation). Examples include map()
, filter()
, and flatMap()
.Actions trigger execution and return results to the driver program or write data to external storage. Examples include collect()
, count()
, and saveAsTextFile()
.
8. What are wide and narrow transformations?
Answer:
- Narrow Transformations: Each input partition contributes to at most one output partition (e.g.,
map()
,filter()
). They do not require data shuffling. - Wide Transformations: Each input partition can contribute to multiple output partitions (e.g.,
reduceByKey()
,groupByKey()
). They require shuffling of data across the network.
9. How do you optimize performance in PySpark?
Answer: To optimize performance in PySpark:
- Caching: Use
persist()
orcache()
to store intermediate results. - Data Serialization: Choose efficient serialization formats like Parquet or Avro.
- Partitioning: Ensure proper partitioning of datasets based on key distributions.
- Broadcast Variables: Use broadcast variables for large read-only datasets to reduce communication costs.
10. What is the purpose of checkpoints in PySpark?
Answer:
Checkpoints save RDDs to a reliable storage system (like HDFS) at specified points during computation. This helps recover from failures without recomputing everything from scratch, improving fault tolerance and performance for long-running jobs.
11. Describe how you would implement a custom transformation in PySpark.
Answer:
To implement a custom transformation, define a Python function that takes a DataFrame as input and returns a transformed DataFrame. You can then use the .transform()
method:
def add_discounted_price(df):
return df.withColumn("discounted_price", df.price - (df.price * df.discount) / 100)
df_transformed = df.transform(add_discounted_price)
12. What is MLlib in PySpark?
Answer:
MLlib is Spark’s scalable machine learning library that provides various algorithms for classification, regression, clustering, collaborative filtering, and more. It offers APIs for building machine learning pipelines and supports model persistence.
13. How do you handle errors in PySpark?
Answer:
Error handling can be done using try-except blocks around your transformations or actions. Additionally, you can use logging mechanisms to capture errors during execution:
try:
df = spark.read.csv("data.csv")
except Exception as e:
print(f"Error occurred: {e}")
14. What are the key differences between DataFrames and Pandas DataFrames?
Answer:
Feature | PySpark DataFrame | Pandas DataFrame |
---|---|---|
Scale | Distributed across clusters | In-memory on single machine |
Performance | Optimized with Catalyst | Limited by memory |
Size Limit | Can handle larger datasets | Limited by available RAM |
API | SQL-like operations | Pythonic operations |
PySpark DataFrames are designed for big data processing while Pandas is suitable for smaller datasets that fit into memory.
15. What is the significance of Partitions in PySpark?
Answer:
Partitions are fundamental units of parallelism in Spark. They allow distributed processing of data across multiple nodes, enhancing performance by enabling concurrent execution of tasks. Proper partitioning can significantly improve job execution time and resource utilization.
16. How do you create a DataFrame from an existing RDD?
Answer:
You can create a DataFrame from an existing RDD using the createDataFrame()
method provided by SparkSession:
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("Example").getOrCreate()
rdd = spark.sparkContext.parallelize([(1, "Alice"), (2, "Bob")])
df = spark.createDataFrame(rdd, ["id", "name"])
17. Explain how you would read data from different sources using PySpark.
Answer: PySpark supports reading data from various sources such as:
- CSV Files: Use
spark.read.csv("path/to/file.csv")
. - JSON Files: Use
spark.read.json("path/to/file.json")
. - Parquet Files: Use
spark.read.parquet("path/to/file.parquet")
. - Databases: Use JDBC connections with
spark.read.format("jdbc").options(...)
.
18. What are User Defined Functions (UDFs) in PySpark?
Answer:
UDFs allow users to define custom functions that can be applied on DataFrames or RDDs similar to built-in functions. They enable more complex transformations that cannot be achieved using standard functions:
from pyspark.sql.functions import udf
from pyspark.sql.types import StringType
def capitalize_name(name):
return name.capitalize()
capitalize_udf = udf(capitalize_name, StringType())
df = df.withColumn("capitalized_name", capitalize_udf(df.name))
19. How do you manage dependencies in PySpark applications?
Answer: Dependencies can be managed using:
- Virtual Environments: Create isolated environments using tools like virtualenv or conda.
- Packaging Libraries: Bundle required libraries with your application using tools like pip or conda.
- Cluster Configuration: Install necessary libraries directly on cluster nodes or use cluster management tools like Apache Mesos or YARN.
20. What are DataFrames in PySpark, and why are they preferred over RDDs?
Answer:
DataFrames are PySpark’s distributed data structures similar to relational tables and built on top of RDDs. They provide named columns and can handle structured and semi-structured data. DataFrames are preferred over RDDs as they offer better optimization, leveraging Catalyst (Spark’s query optimizer) and Tungsten (execution engine), and they allow integration with SQL for complex queries. DataFrames are also easier to work with due to their schema and allow for Python, Scala, Java, and R API interoperability.
21. How do you create a DataFrame in PySpark?
Answer: DataFrames in PySpark can be created from various data sources, such as:
- RDDs:
spark.createDataFrame(rdd, schema)
- Pandas DataFrames:
spark.createDataFrame(pandas_df)
- CSV/JSON files:
spark.read.csv("path")
orspark.read.json("path")
- Existing Tables:
spark.sql("SELECT * FROM table")
Each method allows for schema specification, either inferred or explicitly defined, making PySpark DataFrames versatile for data ingestion.
22. Describe PySpark’s Catalyst Optimizer.
Answer:
Catalyst is Spark’s optimizer designed to enhance query processing performance. It automatically optimizes logical execution plans for transformations on DataFrames or SQL queries before converting them into physical execution plans. Catalyst applies rule-based optimizations, like predicate pushdown, column pruning, and filter pushdown, reducing unnecessary data shuffling. This leads to reduced computation time and efficient resource usage, crucial for big data tasks.
23. Explain the role of the SparkSession in PySpark.
Answer:
SparkSession
is the entry point for any PySpark application, acting as the environment handler for creating DataFrames, reading/writing data, and running SQL queries. It replaced SparkContext
in Spark 2.x, unifying different entry points for managing resources, configurations, and application lifecycle. SparkSession.builder.appName("app_name").getOrCreate()
is typically used to create a session. It helps streamline various APIs and enhance user productivity.
24. How can PySpark be used for handling missing data?
Answer:
PySpark offers various methods for handling missing data in DataFrames, such as:
drop()
: Removes rows with null values, optionally specifying columns or a threshold.fill()
: Fills missing values with specified values for columns or across the entire DataFrame.replace()
: Allows for more complex handling by replacing certain values in a DataFrame.
These functions enable efficient data preprocessing, ensuring data consistency for analysis.
25. What are transformations and actions in PySpark? Provide examples.
Answer:
Transformations are operations that define a new RDD/DataFrame from an existing one and are lazily evaluated. Examples include:
map()
: Applies a function to each element.filter()
: Filters rows based on conditions.groupBy()
: Groups data based on a column.
Actions trigger execution, returning results to the driver. Examples include:
count()
: Counts elements.collect()
: Returns all elements.first()
: Returns the first element.
The separation allows for better performance through optimization.
26. What is lazy evaluation in PySpark, and why is it beneficial?
Answer:
Lazy evaluation means that PySpark doesn’t execute transformations immediately; instead, it builds a lineage or execution plan, only triggering on actions. This benefits performance by allowing PySpark to analyze and optimize the execution plan, batch up operations, and minimize data shuffling.
27. What are accumulators and broadcast variables in PySpark?
Answer:
Accumulators are write-only variables that aggregate information (e.g., counters) across executors and are updated only by actions.
Broadcast variables are read-only variables sent to all nodes to reduce the overhead of duplicating data (e.g., look-up tables). These enhance distributed processing by ensuring only necessary data is communicated.
28. How do you handle schema definition in PySpark DataFrames?
Answer:
PySpark allows for both explicit and inferred schema definitions. For explicit schemas, users define a StructType
composed of StructField
s specifying data types (StringType
, IntegerType
, etc.), which provides consistency and enforces data integrity.
29. Explain the difference between map()
and flatMap()
in PySpark.
Answer:
map()
: Transforms each element in an RDD and returns an RDD of the same length.flatMap()
: Similar but can return multiple elements per input element, resulting in an RDD with a variable length.
30. How is partitioning managed in PySpark?
Answer:
PySpark automatically partitions data for distributed processing. Users can adjust partitions using repartition()
or coalesce()
for optimal memory use and reduced data shuffling, depending on the workload’s data distribution requirements.
31. How does PySpark handle data persistence and caching?
Answer:
PySpark provides persist()
and cache()
methods to store intermediate RDD/DataFrame states in memory or on disk, improving performance for iterative computations by avoiding recomputation of the RDD lineage.
32. Describe a use case for join
operations in PySpark.
Answer:
Joins are used for combining DataFrames based on shared columns (keys). PySpark supports various joins (inner, outer, left, right) and can handle large datasets efficiently by distributing join operations across nodes.
33. What is groupBy()
in PySpark, and how is it used?
Answer:
groupBy()
groups rows based on one or more columns, enabling aggregation operations like sum()
, avg()
, and count()
, useful for summarizing and aggregating large datasets.
34. How does PySpark handle file formats like CSV, JSON, and Parquet?
Answer:
PySpark supports reading/writing various formats through spark.read.format().load()
and .write.format().save()
, with Parquet offering optimal storage and performance through columnar storage.
35. What are PySpark UDFs, and how are they used?
Answer:
User-defined functions (UDFs) extend PySpark’s SQL capabilities by allowing custom Python functions on DataFrame columns. UDFs are registered via udf()
and used in select()
or withColumn()
.
36. Explain the difference between narrow and wide transformations in PySpark.
Answer:
Narrow transformations (like map
, filter
) only require data from a single partition, while wide transformations (like join
, groupBy
) require data shuffling across partitions, impacting performance.
37. What are window functions in PySpark, and how are they used?
Answer:
Window functions perform calculations across rows related to the current row within a window frame. Common use cases include running totals, ranks, and moving averages. They are used with Window
specification and functions like row_number()
, rank()
, and sum().
38. What are the different ways of persisting data in PySpark, and how do they impact performance?
Answer:
PySpark allows data persistence with the cache()
and persist()
methods, which store intermediate results for re-use across transformations or actions. cache()
stores data in memory by default, while persist()
offers various storage levels, such as MEMORY_ONLY, MEMORY_AND_DISK, and DISK_ONLY.
Choosing the right persistence level affects performance—storing in memory improves speed for iterative tasks but can lead to memory overhead, whereas MEMORY_AND_DISK is useful when memory is limited, allowing PySpark to spill excess data to disk.
39. How does PySpark handle parallel processing, and what configurations can optimize it?
Answer:
PySpark’s parallel processing is achieved by partitioning data across executors on a cluster. It uses spark.default.parallelism
for RDDs and spark.sql.shuffle.partitions
for DataFrames, with default values based on the cluster setup. Increasing partitions enhances parallelism, but excessive partitions may increase shuffling and memory usage. Optimizing spark.executor.memory
, spark.executor.cores
, and spark.task.cpus
can ensure tasks utilize the available resources efficiently.
40. Explain the purpose and usage of foreachPartition()
in PySpark.
Answer:
The foreachPartition()
function in PySpark processes data at the partition level, providing efficient handling of resources when each partition requires separate setup, such as database connections. Unlike foreach()
, which processes each element, foreachPartition()
minimizes repetitive overhead by processing data in bulk per partition, useful in scenarios like batch database writes or external API calls.
41. What is PySpark’s explain()
function, and how can it be used for query optimization?
Answer:
The explain()
function in PySpark provides an execution plan for DataFrames, showing stages of transformations and actions. This includes details of the physical plan (e.g., project, filter, scan). Analyzing these plans helps identify performance bottlenecks, excessive shuffles, or costly transformations, guiding users in optimizing queries. For instance, explain(True)
provides both logical and physical plans, enabling in-depth analysis.
42. How does PySpark handle data skew, and what techniques mitigate it?
Answer:
Data skew occurs when some partitions are significantly larger than others, slowing down processing. PySpark mitigates skew through techniques such as salting (adding random keys to distribute skewed keys), splitting large keys into multiple partitions, and using partitioning and bucketing strategies. Identifying skew with tools like glom()
or groupByKey()
helps balance partitions, enhancing efficiency.
43. What are broadcast joins in PySpark, and how do they improve performance?
Answer:
Broadcast joins optimize joins by distributing a small table across all executors, eliminating the need for data shuffling. This approach works well when joining a small table with a larger one. In PySpark, broadcast()
is explicitly used with the join()
operation, reducing join time significantly, especially in distributed data scenarios where shuffling can be costly.
44. Describe the role of Window
functions in data analysis with PySpark.
Answer:
Window
functions allow calculations over a specific range or window of rows in DataFrames, such as ranking, cumulative sums, and moving averages. They do not reduce rows but add columns for analytical insights. Specified with Window.partitionBy()
and Window.orderBy()
, they enable sophisticated data analysis tasks, such as finding top-N records per group or calculating running totals.
45. How do you handle large file ingestion in PySpark for efficient processing?
Answer:
PySpark can handle large file ingestion by using distributed file formats like Parquet, adjusting the spark.sql.files.maxPartitionBytes
to control data partition size, and enabling schema inference where possible. Using file formats with indexing, such as ORC or Parquet, reduces I/O overhead. Optimizing cluster resources and using distributed storage (e.g., HDFS, S3) also facilitate high-volume ingestion.
46. Explain repartition()
vs. coalesce()
in PySpark.
Answer:
repartition()
evenly redistributes data across the specified number of partitions and can increase or decrease partitions. It triggers a full shuffle, useful for load balancing. coalesce()
, on the other hand, reduces partitions without triggering a shuffle, ideal for consolidating partitions. For instance, reducing partitions after a large join or aggregation can improve memory efficiency without the overhead of a shuffle.
47. What is the difference between collect()
and take()
in PySpark?
Answer:
collect()
: Retrieves all data from executors to the driver, suitable for small datasets due to memory constraints.take(n)
: Returns the firstn
rows and is memory-efficient for previewing data as it doesn’t retrieve the entire dataset, reducing potential memory overload on the driver.
48. Explain how PySpark manages fault tolerance.
Answer:
PySpark achieves fault tolerance through lineage and data replication. Lineage records transformations, enabling recomputation of lost partitions from the original data. Additionally, cluster managers like YARN or Kubernetes replicate tasks, handling executor failures and ensuring data persistence.
49. How can you optimize joins in PySpark?
Answer: Join optimization involves:
- Broadcast joins for small tables.
- Partitioning large datasets on join keys to minimize shuffling.
- Bucketing in Hive tables for repeatable joins.
- Using DataFrames over RDDs for Catalyst optimization and query planning.
These techniques help manage memory usage, minimize shuffling, and improve performance.
50. What is PySpark SQL, and how does it integrate with DataFrames?
Answer:
PySpark SQL provides SQL-like querying capabilities on DataFrames. It allows for data manipulation with SQL syntax, beneficial for users familiar with SQL and for integrating with SQL-based ETL workflows. PySpark SQL queries integrate seamlessly with DataFrames, enabling complex analytical queries, joins, and aggregations within the PySpark ecosystem.
51. How do you handle time-based data in PySpark?
Answer:
PySpark handles time-based data using timestamp functions (date_format
, to_date
, year
, month
, etc.) and Window
functions for time-based aggregations like rolling averages. Additionally, PySpark’s time zone handling in timestamp
columns allows correct conversion and arithmetic for different time zones, crucial in time-series analysis.
52. What is Spark MLlib, and how does it work in PySpark?
Answer:
Spark MLlib is the machine learning library in PySpark, offering tools for classification, regression, clustering, and recommendation. MLlib pipelines standardize model training workflows, including data preparation and model evaluation. It provides scalable ML algorithms, utilizing PySpark’s distributed architecture for large datasets, facilitating real-time data science applications.
53. Explain the concept of shuffling in PySpark.
Answer:
Shuffling is the process of redistributing data across partitions for wide transformations like groupBy
and join
. It often involves moving data between nodes, increasing network I/O and memory usage, which can slow down performance. Shuffling optimization is achieved by partitioning data, caching, and tuning the number of shuffle partitions (spark.sql.shuffle.partitions
).
54. What is the role of mapPartitions()
in PySpark, and how does it differ from map()
?
Answer:
mapPartitions()
processes data at the partition level, enabling operations on each partition as a whole, reducing the setup overhead compared to map()
, which processes each element individually. mapPartitions()
is efficient for operations like initializing database connections or batch processing, whereas map()
is simpler for element-wise transformations.
55. How does PySpark integrate with Hive, and what are its benefits?
Answer:
PySpark integrates with Hive using enableHiveSupport()
in SparkSession
, providing access to Hive databases and tables. This integration supports querying, reading, and writing Hive data within PySpark, beneficial for ETL workflows. Hive integration enables usage of Hive’s metadata store, allowing analysts to manage and query large datasets using SQL.
56. Describe the purpose and structure of a PySpark pipeline.
Answer:
A PySpark pipeline structures machine learning workflows, standardizing stages of data processing (e.g., transformers like tokenizers, encoders) and model estimators (e.g., classifiers). Pipelines allow for building repeatable processes, optimizing model tuning, and ensuring consistent preprocessing steps, which are valuable in iterative model development.
57. What are PySpark’s partitioning strategies, and how do they affect performance?
Answer: Partitioning strategies in PySpark include:
- Range partitioning: Divides data based on range values, efficient for sorting.
- Hash partitioning: Partitions data based on a hash of keys
Learn More: Carrer Guidance
Salesforce admin interview questions and answers for experienced
Salesforce admin interview questions and answers for freshers
EPAM Systems Senior Java Developer Interview questions with answers
Flutter Interview Questions and Answers for all levels
Most common data structures and algorithms asked in Optum interviews
Optum Technical Interview Questions with detailed answering for Freshers and Experienced
Machine learning interview questions and answers for all levels