In Optum interviews, candidates can expect a variety of questions focused on data structures and algorithms. Here are some of the most common topics and specific examples that frequently arise during the interview process:
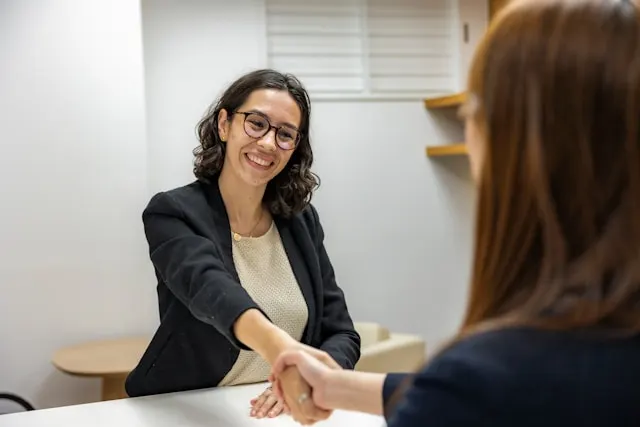
Common Data Structures
1. Arrays
Common Questions:
- Reverse an array.
- Find the maximum/minimum element in an array.
- Merge two sorted arrays.
2. Strings
Common Questions:
- Check if a string is a palindrome.
- Find the first non-repeating character in a string.
- Count the number of vowels in a string.
3. Linked Lists
Common Questions:
- Reverse a linked list.
- Detect a cycle in a linked list (Floyd’s Cycle Detection).
- Merge two sorted linked lists.
4. Stacks
Common Questions:
- Implement a stack using arrays or linked lists.
- Evaluate a postfix expression.
- Check for balanced parentheses in an expression.
5. Queues
Common Questions:
- Implement a queue using stacks.
- Design a circular queue.
- Find the maximum element in a sliding window (using deque).
6. Trees
Common Questions:
- Traverse a binary tree (in-order, pre-order, post-order).
- Find the height of a binary tree.
- Check if two trees are identical.
7. Graphs
Common Questions:
- Implement depth-first search (DFS) and breadth-first search (BFS).
- Detect cycles in a graph.
- Find the shortest path using Dijkstra’s algorithm.
8. Hash Tables
Common Questions:
- Count frequencies of elements in an array.
- Check for anagrams using hash maps.
- Find two numbers that add up to a specific target.
Common Algorithms
1. Sorting Algorithms
Common Questions:
- Implement bubble sort, selection sort, or quicksort.
- Discuss time complexity differences between sorting algorithms.
2. Searching Algorithms
Common Questions:
- Implement binary search on a sorted array.
- Discuss linear search vs binary search.
3. Dynamic Programming
Common Questions:
- Solve the Fibonacci sequence using dynamic programming.
- Find the longest increasing subsequence.
- Solve the knapsack problem.
4. Recursion
Common Questions:
- Solve problems that can be broken down recursively (e.g., factorial calculation).
- Generate all subsets of a set.
5. Backtracking
Common Questions:
- Solve the N-Queens problem.
- Generate permutations of an array or string.
Interview Structure
Interviews at Optum typically consist of multiple rounds, including:
- Online Assessment: This may include multiple-choice questions on data structures, algorithms, and other technical topics, along with coding challenges on platforms like HackerRank.
- Technical Interview: Candidates are often asked to solve live coding problems related to data structures and algorithms, explain their thought process, and discuss their previous projects.
- Behavioral Interview: This round focuses on assessing soft skills and cultural fit within the company.
Preparation Tips
- Practice coding problems on platforms like LeetCode or HackerRank to strengthen your understanding of data structures and algorithms.
- Review fundamental concepts in OOP, operating systems, and databases as they are also commonly covered during interviews at Optum.
- Be prepared to explain your thought process clearly while solving problems during technical interviews.
By focusing on these areas and practicing extensively, candidates can enhance their chances of success in Optum’s interview process.
Learn More: Carrer Guidance [Most common data structures and algorithms asked in Optum interviews]
Optum Technical Interview Questions with detailed answering
Machine learning interview questions and answers for all levels
Kubernetes interview questions and answers for all levels
Flask interview questions and answers
Software Development Life Cycle (SDLC) interview questions and answers