Are you preparing for a Flask interview? To help you out, we have compiled a list of over 55 commonly asked Flask interview questions with detailed answers. These questions cover a broad range of Flask concepts that assess understanding of both basic and advanced topics in Flask, suitable for interview preparation at various levels. Here is the list of 55+ Flask interview questions and answers.
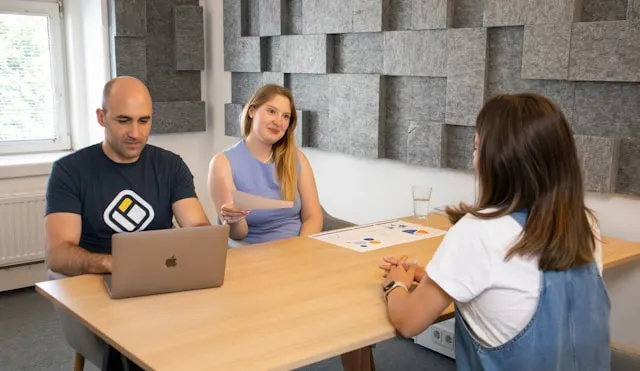
Flask interview questions and answers
1. What is Flask, and how is it different from Django?
2. Explain the concept of routing in Flask.
3. What is the purpose of the app.run() method in Flask?
4. How do you handle form data in Flask?
5. What are Flask Blueprints, and how are they used?
6. Explain Flask’s render_template() function.
7. What is Jinja2, and why is it used in Flask?
8. How does Flask handle sessions, and where are they stored?
9. Explain the flash() function in Flask.
10. How do you enable debugging in Flask?
11. What is Flask-RESTful, and how does it help in API development?
12. What is a WSGI server, and why is it important for Flask?
13. How does Flask support request methods like GET, POST, PUT, and DELETE?
14. How can you handle file uploads in Flask?
15. Explain Flask-SQLAlchemy and its purpose.
16. How do you handle errors in Flask?
17. How does Flask handle middleware, and what is it used for?
18. What are Flask extensions, and how do they enhance functionality?
19. How do you implement authentication in Flask?
20. Explain CSRF protection in Flask.
21. What is the difference between Flask.g and Flask.config?
22. How does Flask handle cookies?
23. What is the Flask request object?
24. How do you implement pagination in Flask?
25. Explain how you would deploy a Flask application.
26. What are the best practices for securing a Flask application?
27. What are Flask contexts, and what are the differences between the Application Context and Request Context?
28. How does Flask handle JSON requests and responses?
29. What are Flask’s before_request and after_request functions, and when are they useful?
30. Explain how Flask’s abort() function works and when to use it.
31. How would you manage environment-specific configurations in Flask?
32. What is Flask’s current_app and how is it used?
33. What are Flask signals, and what are they used for?
34. How can you implement caching in a Flask application?
35. How would you handle long-running tasks in Flask?
36. Explain the concept of Flask decorators.
37. What is the purpose of the app.config dictionary in Flask?
38. How do you handle static files in Flask?
39. Explain Flask’s teardown_request and teardown_appcontext.
40. How do you implement RESTful APIs in Flask?
41. What is Flask’s request.args and when should you use it?
42. Explain the difference between redirect() and url_for() in Flask.
43. How does Flask support cross-origin resource sharing (CORS)?
44. How would you structure a large Flask application?
45. Explain Flask’s Flask-Script and its uses.
46. What is a Flask factory function, and why is it used?
47. How does Flask support unit testing?
48. How can you log errors and requests in Flask?
49. Explain how you can add custom CLI commands in Flask.
50. What are HTTP status codes, and how are they used in Flask?
51. How do you handle database migrations in Flask?
52. What is the purpose of Flask’s request.endpoint?
53. How would you manage user roles and permissions in Flask?
54. Explain Flask’s make_response() function.
55. What are some Flask security best practices?
56. How do you implement WebSockets in a Flask application?
57. What is Flask-Login, and how does it simplify user authentication?
58. What is the purpose of request.endpoint and request.blueprint in Flask?
1. What is Flask, and how is it different from Django?
Answer:
Flask is a lightweight, WSGI (Web Server Gateway Interface) web framework in Python designed for simplicity and flexibility. It’s often called a “micro-framework” because it lacks many of the features of larger frameworks, like a built-in ORM (Object Relational Mapper). In contrast, Django is a full-featured, high-level web framework that follows the “batteries-included” philosophy, offering many pre-built tools such as an ORM, admin interface, and user authentication.
2. Explain the concept of routing in Flask.
Answer:
Routing in Flask is the process of mapping URLs to specific functions in the application. Flask uses the @app.route()
decorator to bind a function to a URL. When a user visits that URL, Flask invokes the associated function, which processes the request and returns a response.
3. What is the purpose of the app.run()
method in Flask?
Answer:
The app.run()
method in Flask is used to start the Flask application’s built-in development server. This server is useful for testing and debugging during development but is not suitable for production use.
4. How do you handle form data in Flask?
Answer:
Form data can be handled in Flask by importing request
from flask
and then accessing request.form
. This dictionary-like object contains all form data as key-value pairs, allowing us to retrieve values based on the input field names.
5. What are Flask Blueprints, and how are they used?
Answer:
Flask Blueprints are a way to organize a Flask application into smaller and reusable modules. They allow us to split the application into components and create a more modular structure by grouping related views, templates, and static files together.
6. Explain Flask’s render_template()
function.
Answer:
render_template()
in Flask is used to render HTML files and pass data to these templates. Flask uses the Jinja2 templating engine to allow Python code to be inserted in HTML. For example, render_template('index.html', data=data)
passes the data
variable to the template.
7. What is Jinja2, and why is it used in Flask?
Answer:
Jinja2 is a templating engine for Python, used in Flask to embed Python code in HTML templates. It allows us to dynamically generate HTML based on variables and conditional logic, making it easier to create dynamic web pages.
8. How does Flask handle sessions, and where are they stored?
Answer:
Flask provides session management via the session
object, which is stored in a client-side cookie. Flask encrypts this data with a secret key to prevent tampering, but sensitive data should not be stored in sessions due to security concerns.
9. Explain the flash()
function in Flask.
Answer:
The flash()
function in Flask is used to display messages to the user. Messages are stored in session data, and the get_flashed_messages()
function retrieves them for display. Flash messages are commonly used for feedback notifications after form submissions.
10. How do you enable debugging in Flask?
Answer:
To enable debugging in Flask, set the debug
parameter to True
in app.run(debug=True)
. This activates the debug mode, allowing automatic reloading of the server on code changes and providing a detailed error traceback in the browser.
11. What is Flask-RESTful, and how does it help in API development?
Answer:
Flask-RESTful is an extension for Flask that simplifies the development of RESTful APIs by providing tools for creating and managing API endpoints. It enables easier handling of HTTP methods (GET, POST, PUT, DELETE) and provides classes for resources and request parsing.
12. What is a WSGI server, and why is it important for Flask?
Answer:
A WSGI (Web Server Gateway Interface) server acts as an intermediary between the web server and Python applications. Flask’s built-in server is for development, so a production-ready WSGI server (e.g., Gunicorn or uWSGI) is recommended to handle requests in production.
13. How does Flask support request methods like GET, POST, PUT, and DELETE?
Answer:
Flask allows specifying HTTP methods for each route by setting the methods
parameter in @app.route()
. For instance, @app.route('/post', methods=['POST'])
will route only POST requests to that endpoint. This helps define CRUD operations in REST APIs.
14. How can you handle file uploads in Flask?
Answer:
File uploads in Flask can be managed by using the request.files
dictionary, which holds uploaded files. Files can then be saved using the save()
method. Flask-Uploads is an extension that adds more features for file handling.
15. Explain Flask-SQLAlchemy
and its purpose.
Answer:
Flask-SQLAlchemy
is an ORM (Object Relational Mapper) for Flask, based on SQLAlchemy. It allows developers to interact with databases using Python classes and objects instead of writing raw SQL queries, improving readability and maintainability.
16. How do you handle errors in Flask?
Answer:
Error handling in Flask can be managed using custom error pages and the @app.errorhandler()
decorator. For instance, @app.errorhandler(404)
can be used to define a custom 404 error page.
17. How does Flask handle middleware, and what is it used for?
Answer:
Middleware in Flask can be implemented using before_request
and after_request
decorators, which allow functions to run before and after each request. This is useful for logging, authentication, or data processing.
18. What are Flask extensions, and how do they enhance functionality?
Answer:
Flask extensions are additional libraries or tools that add functionality to a Flask application. Examples include Flask-WTF
for forms, Flask-Login
for authentication, and Flask-Mail
for email support.
19. How do you implement authentication in Flask?
Answer:
Flask-Login is an extension for managing user sessions and authentication in Flask. It provides features like login/logout functionality, session management, and decorators like @login_required
to restrict access to authenticated users.
20. Explain CSRF protection in Flask.
Answer:
CSRF (Cross-Site Request Forgery) protection in Flask can be enabled using Flask-WTF, which automatically includes CSRF tokens in forms and validates them upon form submission. This prevents unauthorized actions from being performed on behalf of a logged-in user.
21. What is the difference between Flask.g
and Flask.config
?
Answer:
Flask.g
is a context-specific global variable used to store data during a request cycle, whereas Flask.config
holds configuration variables for the application that are shared across all requests.
22. How does Flask handle cookies?
Answer:
Flask uses the set_cookie()
method to store cookies on the client side. Cookies can be retrieved using request.cookies
and deleted using response.delete_cookie()
.
23. What is the Flask request
object?
Answer:
The request
object in Flask is an instance of Request
and contains information about the HTTP request, such as form data, headers, cookies, and the request method. It enables accessing all data sent by the client.
24. How do you implement pagination in Flask?
Answer:
Pagination in Flask can be implemented by using SQL queries with LIMIT
and OFFSET
. Flask extensions like Flask-Paginate
can also help manage pagination by providing built-in pagination views and controls.
25. Explain how you would deploy a Flask application.
Answer:
To deploy a Flask application, use a WSGI server (e.g., Gunicorn) and a web server (e.g., Nginx) as a reverse proxy. The setup may also include Docker for containerization and cloud platforms like AWS, Heroku, or DigitalOcean for hosting.
26. What are the best practices for securing a Flask application?
Answer: Security best practices for Flask include:
- Using HTTPS for secure data transmission.
- Enabling CSRF protection.
- Validating and sanitizing user input.
- Securing sensitive data in environment variables.
- Restricting SQL injection with ORM usage or parameterized queries.
27. What are Flask contexts, and what are the differences between the Application Context and Request Context?
Answer:
Flask contexts allow us to keep track of global variables in a thread-safe manner. The Application Context holds the application data (e.g., config), while the Request Context holds request-specific data (e.g., request
, session
). app_context()
is used for app-wide data, and request_context()
is used for data specific to an individual request.
28. How does Flask handle JSON requests and responses?
Answer:
Flask provides a built-in method request.get_json()
to parse JSON data from incoming requests. For responses, Flask’s jsonify()
method serializes data into JSON format and sets the correct Content-Type
header, making it ideal for API responses.
29. What are Flask’s before_request
and after_request
functions, and when are they useful?
Answer:
The before_request
function runs before each request and is useful for tasks like authentication or logging. The after_request
function executes after each request, ideal for post-processing tasks, such as modifying headers or logging the response.
30. Explain how Flask’s abort()
function works and when to use it.
Answer:
The abort()
function in Flask immediately stops a request and returns an HTTP error response. For example, abort(404)
returns a 404 error. It’s useful when an invalid input or unauthorized access needs to be flagged quickly.
31. How would you manage environment-specific configurations in Flask?
Answer:
Flask allows different configurations by setting FLASK_ENV
to “development,” “production,” or “testing.” Flask also supports environment-based config files, loading specific configurations based on the environment, and setting environment variables for sensitive data.
32. What is Flask’s current_app
and how is it used?
Answer:
current_app
is a context variable that gives access to the active application instance, useful when writing code outside the main application file, such as in Blueprints, extensions, or helper functions.
33. What are Flask signals, and what are they used for?
Answer:
Signals in Flask allow different parts of an application to react to specific events. Flask-Signals provides events such as before_request
, after_request
, teardown_request
, and custom events to decouple code and improve modularity.
34. How can you implement caching in a Flask application?
Answer:
Caching in Flask can be achieved using the Flask-Caching extension, which supports caching mechanisms like Redis, Memcached, or in-memory cache. Caching is used to improve performance by storing and retrieving precomputed data rather than recalculating it.
35. How would you handle long-running tasks in Flask?
Answer:
For long-running tasks, Flask integrates with task queues like Celery, combined with a message broker like RabbitMQ or Redis. This lets the main application stay responsive while the task runs in the background, avoiding timeouts and improving scalability.
36. Explain the concept of Flask decorators.
Answer:
Flask uses decorators extensively for routing (@app.route
), error handling (@app.errorhandler
), and request management (@app.before_request
). These decorators wrap functions with additional functionality, allowing clear organization of code logic and reusable functionality.
37. What is the purpose of the app.config
dictionary in Flask?
Answer:
app.config
stores configuration variables for a Flask application, such as DEBUG
, SECRET_KEY
, and database URIs. Developers can add custom settings to this dictionary to make configurations accessible globally.
38. How do you handle static files in Flask?
Answer:
Static files (e.g., CSS, JavaScript, images) are stored in the static
folder by default in Flask. Flask provides url_for('static', filename='file.ext')
to generate URLs for these files, enabling easy access and cache-busting.
39. Explain Flask’s teardown_request
and teardown_appcontext
.
Answer:
teardown_request
and teardown_appcontext
are used to clean up resources after a request or after the app context ends, respectively. They are often used for closing database connections or releasing resources.
40. How do you implement RESTful APIs in Flask?
Answer:
RESTful APIs in Flask are built using @app.route
decorators with HTTP methods like GET, POST, PUT, and DELETE. Flask-RESTful is an extension that further simplifies API creation by providing resource classes and request parsing tools.
41. What is Flask’s request.args
and when should you use it?
Answer:
request.args
is a dictionary-like object for handling query string parameters in GET requests. Use it to access data sent in the URL, like request.args.get('param')
, for filter, pagination, or sorting in APIs.
42. Explain the difference between redirect()
and url_for()
in Flask.
Answer:
redirect()
sends a response that tells the browser to navigate to a different URL, while url_for()
generates URLs based on the function name and parameters. They are commonly used together to create a redirect to a URL dynamically.
43. How does Flask support cross-origin resource sharing (CORS)?
Answer:
Flask-CORS is an extension that allows cross-origin resource sharing by setting headers on HTTP responses to specify which domains can access the API. This is crucial for enabling API calls from other origins, like JavaScript apps hosted on different domains.
44. How would you structure a large Flask application?
Answer:
In large applications, it’s common to use Blueprints to separate concerns, place templates and static files in dedicated folders, and create separate modules for models, views, and configurations. A factory function can also be used to initialize the app and extensions.
45. Explain Flask’s Flask-Script
and its uses.
Answer:
Flask-Script
is an extension that allows managing tasks like running the server, database migrations, and custom commands from the command line. Although Flask-Script has been deprecated, alternatives like Flask CLI provide similar functionality.
46. What is a Flask factory function, and why is it used?
Answer:
A factory function in Flask is used to create an instance of the app. This approach allows for better configurability, such as configuring the app based on the environment, and is especially helpful in testing as it allows multiple app instances.
47. How does Flask support unit testing?
Answer:
Flask provides a test_client
for simulating requests without starting a server. Combined with Python’s unittest
or pytest
frameworks, developers can test views, endpoints, and logic within a controlled testing environment.
48. How can you log errors and requests in Flask?
Answer:
Flask uses Python’s logging
module for logging. Configure it to log messages to files or console with levels like ERROR
, WARNING
, or INFO
. This helps trace requests, errors, and other runtime information crucial for debugging.
49. Explain how you can add custom CLI commands in Flask.
Answer:
Flask allows adding custom CLI commands via the @app.cli.command()
decorator or using the Click library. These commands are useful for running scripts, database migrations, or starting development servers with specific configurations.
50. What are HTTP status codes, and how are they used in Flask?
Answer:
HTTP status codes represent the response state. Flask functions can return status codes alongside responses, e.g., return jsonify(data), 200
or abort(404)
, helping clients understand the response and troubleshoot issues.
51. How do you handle database migrations in Flask?
Answer:
Flask-Migrate, an extension for handling database migrations, integrates with SQLAlchemy. It uses Alembic under the hood to generate migration scripts for schema changes, making it easy to manage database version control in a Flask app.
52. What is the purpose of Flask’s request.endpoint
?
Answer:
request.endpoint
stores the endpoint name (function name) of the matched route. It’s helpful in conditional logic based on which route is accessed, for instance, to modify behavior or render specific templates.
53. How would you manage user roles and permissions in Flask?
Answer:
Flask-Principal and Flask-User are extensions that manage roles and permissions, allowing role-based access control. Developers can restrict route access based on user roles to implement a more secure and role-specific experience.
54. Explain Flask’s make_response()
function.
Answer:
make_response()
allows greater control over response creation by letting developers customize headers, status codes, and content. It’s helpful when modifying responses beyond simple text or JSON.
55. What are some Flask security best practices?
Answer: Important Flask security practices include:
- Using
SECRET_KEY
for secure sessions. - Validating and sanitizing user input.
- Implementing HTTPS and secure cookie flags.
- Using CSRF protection for forms.
- Implementing secure headers using Flask-Talisman.
56. How do you implement WebSockets in a Flask application?
Answer:
WebSockets are supported in Flask through Flask-SocketIO. This extension enables real-time, bidirectional communication for applications, like live notifications or chat features, by establishing a persistent connection with the client.
57. What is Flask-Login, and how does it simplify user authentication?
Answer:
Flask-Login manages user sessions, enabling easy login, logout, and user session persistence. It provides the @login_required
decorator to protect routes and handles session expiration, user loading, and session data securely.
58. What is the purpose of request.endpoint
and request.blueprint
in Flask?
Answer:
request.endpoint
provides the function name of the current route, while request.blueprint
gives the name of the current Blueprint (if any), helping organize logic based on the specific route or module.
Learn More: Carrer Guidance
System Design Interview questions and answers
Software Development Life Cycle (SDLC) interview questions and answers
Manual testing interview questions for 5 years experience
Manual testing interview questions and answers for all levels
Node js interview questions and answers for experienced