Are you preparing for a Node js interview? To ease your journey, we’ve compiled a comprehensive guide of Node js interview questions and answers for freshers. This guide covers all essential topics and provides clear, concise answers to the most commonly asked questions.
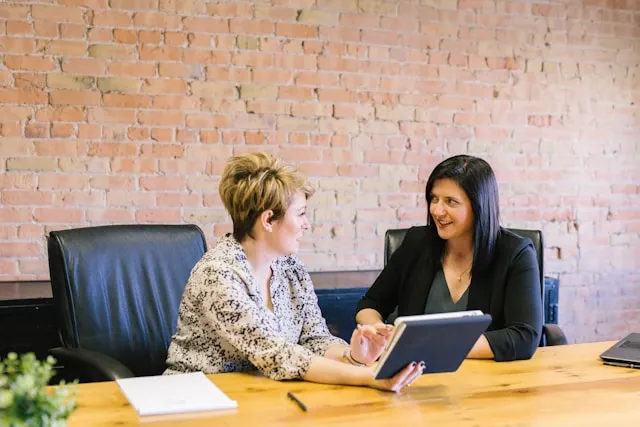
Node js interview questions and answers for freshers
1. What is Node.js?
2. What are the main features of Node.js?
3. Explain the difference between Node.js and JavaScript.
4. What are the benefits of using Node.js?
5. What is the purpose of the package.json file?
6. How do you install Node.js?
7. What are the Node.js modules?
8. What is NPM, and how is it used?
9. How do you create a simple HTTP server in Node.js?
10. Explain the concept of middleware in Node.js.
11. What is the Event Loop in Node.js?
12. What is the purpose of async and await in Node.js?
13. Explain the difference between synchronous and asynchronous code in Node.js.
14. How does error handling work in Node.js?
15. What is Express.js?
16. Explain the difference between require and import in Node.js.
17. What is callback hell, and how can you avoid it?
18. What is a stream in Node.js?
19. Explain the purpose of process.nextTick() in Node.js.
20. How do you handle file operations in Node.js?
21. What is REPL in Node.js?
22. Explain the concept of a Buffer in Node.js.
23. What is the path module in Node.js used for?
24. What is CORS, and how do you handle it in Node.js?
25. How do you connect to a database in Node.js?
26. What is clustering in Node.js?
27. How does Node.js manage memory?
28. Explain the difference between setImmediate() and setTimeout() in Node.js.
29. What is a Promise in Node.js?
30. What are the types of HTTP methods used in Node.js?
31. Explain the role of EventEmitter in Node.js.
32. What is the difference between Node.js and a traditional web server like Apache or Nginx?
33. What is the role of the V8 engine in Node.js?
34. How does Node.js handle asynchronous operations?
35. What is the purpose of the global object in Node.js?
36. What is the difference between require() and import() in Node.js?
37. What are the different types of streams in Node.js?
38. What is the purpose of the cluster module in Node.js?
39. How do you handle timeouts in Node.js?
40. What is the purpose of the util module in Node.js?
41. What is the readline module in Node.js used for?
42. What is the crypto module in Node.js used for?
43. What is the dns module in Node.js used for?
44. What is the child_process module in Node.js used for?
45. What is the http module in Node.js used for?
46. What is the os module in Node.js used for?
1. What is Node.js?
Answer:
Node.js is an open-source, cross-platform JavaScript runtime environment built on Chrome’s V8 JavaScript engine. It allows developers to execute JavaScript code server-side, making it possible to build scalable and efficient network applications.
2. What are the main features of Node.js?
Answer: Key features include:
- Event-driven architecture: Handles multiple requests simultaneously.
- Non-blocking I/O: Improves efficiency and speed by using asynchronous functions.
- Cross-platform: Compatible with Linux, Windows, and macOS.
- Single-threaded: Optimized for network-heavy applications.
3. Explain the difference between Node.js and JavaScript.
Answer:
JavaScript is a language used for web development, primarily on the client side. Node.js, on the other hand, is a runtime that allows JavaScript to run server-side, enabling developers to use JavaScript for backend development.
4. What are the benefits of using Node.js?
Answer: Benefits include:
- High performance due to V8 engine.
- Efficient handling of concurrent requests.
- Strong ecosystem with the Node Package Manager (NPM).
- Excellent for real-time applications (e.g., chat, online gaming).
5. What is the purpose of the package.json
file?
Answer:
The package.json
file is used to define project metadata, list dependencies, scripts, and specify versions of Node.js and NPM. It is essential for managing the project’s dependencies and configurations.
6. How do you install Node.js?
Answer:
Node.js can be installed by downloading the installer from the official Node.js website or using package managers like nvm
for version management.
7. What are the Node.js modules?
Answer:
Modules are libraries or files that contain related code that can be imported and used in Node.js applications. Examples include core modules like fs
, http
, path
, and third-party modules like express
.
8. What is NPM, and how is it used?
Answer:
NPM (Node Package Manager) is a tool that comes with Node.js. It is used to manage packages and dependencies for Node.js applications. Commands like npm install
are used to add packages.
9. How do you create a simple HTTP server in Node.js?
Answer:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello, World!');
});
server.listen(3000, () => {
console.log('Server running on port 3000');
});
10. Explain the concept of middleware in Node.js.
Answer:
Middleware functions are functions that have access to the request object, response object, and the next middleware function in the application’s request-response cycle. They are used for handling requests, processing, and sending responses.
11. What is the Event Loop in Node.js?
Answer:
The Event Loop is a core feature in Node.js that handles asynchronous operations, allowing Node.js to be non-blocking by managing callbacks and I/O operations.
12. What is the purpose of async
and await
in Node.js?
Answer:
async
and await
are used for handling asynchronous operations in a more readable way. async
makes a function return a promise, while await
pauses execution until the promise resolves.
13. Explain the difference between synchronous and asynchronous code in Node.js.
Answer:
Synchronous code executes sequentially, blocking further code until the current task completes. Asynchronous code does not block the thread and allows for other code execution while waiting for an operation to complete.
14. How does error handling work in Node.js?
Answer:
Errors are handled using try-catch
blocks for synchronous code and .catch()
for promises. For callback-based functions, errors are usually passed as the first argument in the callback function.
15. What is Express.js?
Answer:
Express.js is a lightweight web application framework for Node.js, designed to simplify routing, middleware management, and handling HTTP requests.
16. Explain the difference between require
and import
in Node.js.
Answer:
require
is a Node.js module syntax used in CommonJS modules, while import
is an ES6 module syntax. Both are used to include modules, but import
requires the use of .mjs
files or adding "type": "module"
in package.json
.
17. What is callback hell, and how can you avoid it?
Answer:
Callback hell is the excessive nesting of callback functions, which makes code harder to read and maintain. It can be avoided by using Promises or async/await.
18. What is a stream in Node.js?
Answer:
A stream is a continuous flow of data that allows reading or writing in chunks rather than loading the entire data into memory. Types of streams include readable, writable, duplex, and transform streams.
19. Explain the purpose of process.nextTick()
in Node.js.
Answer:
process.nextTick()
defers the execution of a callback function until the next iteration of the event loop. It’s used to handle asynchronous operations without blocking the event loop.
20. How do you handle file operations in Node.js?
Answer:
Node.js provides the fs
module to handle file operations like reading, writing, and deleting files. Example:
const fs = require('fs');
fs.readFile('file.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
21. What is REPL in Node.js?
Answer:
REPL stands for Read-Eval-Print Loop. It’s a command-line tool that evaluates JavaScript code one line at a time, useful for testing code snippets.
22. Explain the concept of a Buffer in Node.js.
Answer:
A Buffer is a temporary storage area for handling binary data streams. It’s used in Node.js to manage binary data from files, network streams, or other resources.
23. What is the path
module in Node.js used for?
Answer:
The path
module provides utilities for handling and transforming file paths, making it easier to work with directory and file structures.
24. What is CORS, and how do you handle it in Node.js?
Answer:
CORS (Cross-Origin Resource Sharing) is a security feature that restricts resources on a web server to be requested from another domain. In Node.js, it can be managed by configuring headers or using the cors
package.
25. How do you connect to a database in Node.js?
Answer:
Node.js connects to databases (e.g., MongoDB, MySQL) using packages like mongoose
for MongoDB or mysql
for MySQL, through functions that establish and manage database connections.
26. What is clustering in Node.js?
Answer:
Clustering enables a Node.js application to create child processes (workers) to handle multiple requests concurrently, improving performance in multi-core systems.
27. How does Node.js manage memory?
Answer:
Node.js uses the V8 garbage collection process, which automatically clears unused data from memory, and also employs memory limits for each application instance.
28. Explain the difference between setImmediate()
and setTimeout()
in Node.js.
Answer:
setImmediate()
executes code after the current event loop, while setTimeout()
schedules code to execute after a specified delay.
29. What is a Promise in Node.js?
Answer:
A Promise represents an eventual completion (or failure) of an asynchronous operation. It allows chaining .then()
for success and .catch()
for errors.
30. What are the types of HTTP methods used in Node.js?
Answer:
Common HTTP methods are GET
, POST
, PUT
, DELETE
, PATCH
, used to perform CRUD (Create, Read, Update, Delete) operations.
31. Explain the role of EventEmitter in Node.js.
Answer:
EventEmitter is a core module that provides an event-driven pattern in Node.js, allowing objects to emit and listen to events.
32. What is the difference between Node.js and a traditional web server like Apache or Nginx?
Answer:
Node.js is a JavaScript runtime environment that allows you to execute JavaScript code outside of a browser. It’s event-driven, non-blocking, and highly scalable, making it ideal for real-time applications, I/O-bound tasks, and microservices.
Traditional web servers like Apache and Nginx are process-based, meaning they create a new process for each incoming request. This can be inefficient for high-traffic applications. Node.js, on the other hand, handles multiple requests concurrently using a single thread, making it more efficient.
33. What is the role of the V8 engine in Node.js?
Answer:
The V8 engine is a high-performance JavaScript engine developed by Google. It’s responsible for compiling and executing JavaScript code in Node.js. It optimizes code execution by converting it into machine code, which significantly improves performance.
34. How does Node.js handle asynchronous operations?
Answer:
Node.js uses an event-driven, non-blocking I/O model to handle asynchronous operations efficiently. When a request comes in, Node.js delegates the task to the operating system and continues processing other requests.
Once the operation is complete, the OS notifies Node.js, which triggers the appropriate callback function. This allows Node.js to handle many concurrent requests without blocking the main thread.
35. What is the purpose of the global
object in Node.js?
Answer:
The global
object is a special object that provides access to global variables and functions in Node.js. It’s used to define variables and functions that can be accessed from anywhere in your Node.js application.
36. What is the difference between require()
and import()
in Node.js?
Answer:
require()
is the CommonJS module system used in Node.js. It’s synchronous and is used to load modules at runtime.
import()
is the ECMAScript module system, which is asynchronous and is used to load modules at compile time. It’s more modern and offers features like tree-shaking and lazy loading.
37. What are the different types of streams in Node.js?
Answer:
- Readable streams: Used for reading data from a source, such as a file or network socket.
- Writable streams: Used for writing data to a destination, such as a file or network socket.
- Duplex streams: Can be read from and written to, such as a TCP socket.
- Transform streams: Modify or transform data as it flows through, such as a gzip stream.
38. What is the purpose of the cluster
module in Node.js?
Answer:
The cluster
module allows you to create multiple worker processes to handle incoming requests, improving the scalability and performance of your Node.js application. Each worker process runs independently and shares the same server port, allowing you to efficiently handle a large number of concurrent connections.
39. How do you handle timeouts in Node.js?
Answer:
You can use the setTimeout()
function to set a timer that executes a callback function after a specified time. You can also use the clearTimeout()
function to cancel a timeout. Additionally, you can use libraries like async
or bluebird
to handle timeouts and asynchronous operations more elegantly.
40. What is the purpose of the util
module in Node.js?
Answer:
The util
module provides utility functions for common tasks, such as inheritance, introspection, and debugging. It also includes functions for formatting objects and inspecting values.
41. What is the readline
module in Node.js used for?
Answer:
The readline
module provides an interface for reading data from a readable stream one line at a time. It’s often used for creating command-line interfaces and reading input from the user.
42. What is the crypto
module in Node.js used for?
Answer:
The crypto
module provides cryptographic functionalities, such as creating hashes, generating random numbers, and encrypting and decrypting data. It’s essential for securing your Node.js applications.
43. What is the dns
module in Node.js used for?
Answer:
The dns
module provides functions for resolving domain names to IP addresses. It’s useful for tasks like fetching website information or building network applications.
44. What is the child_process
module in Node.js used for?
Answer:
The child_process
module allows you to spawn child processes, execute shell commands, and communicate with them. It’s useful for tasks like running external programs, executing system commands, and implementing complex workflows.
45. What is the http
module in Node.js used for?
Answer:
The http
module provides the foundation for creating HTTP servers and clients in Node.js. It’s used to handle incoming requests, send responses, and implement web servers.
46. What is the os
module in Node.js used for?
Answer:
The os
module provides information about the operating system, such as the platform, CPU architecture, and memory usage. It’s useful for tailoring your application to the specific environment it’s running in.
Learn More: Carrer Guidance
Hibernate interview questions and answers for freshers
Terraform interview questions and answers for all levels
Rest API interview questions and answers for all levels
Mysql interview questions and answers for experienced
Mysql interview questions and answers for freshers
Python interview questions and answers for data analyst experienced
Python interview questions and answers for data analyst freshers