Are you preparing for a Terraform interview? To help you get ready, we’ve compiled an extensive list of over 65 questions and answers covering everything from basic concepts to advanced techniques. This guide includes topics like Terraform’s core components, state management, commands, and best practices.
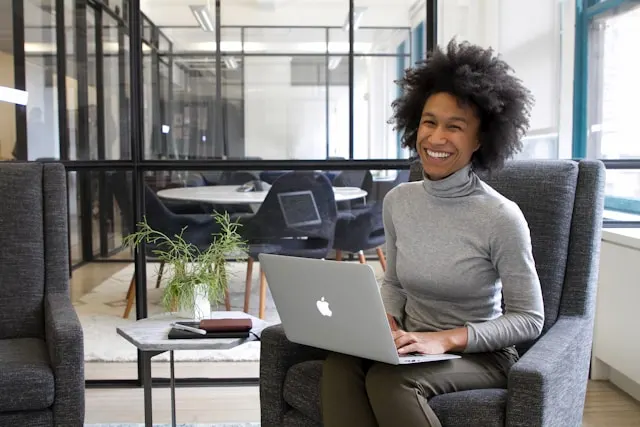
Terraform interview questions and answers for all levels
1. What is Terraform?
2. What is Infrastructure as Code (IaC)?
3. What are Terraform’s main components?
4. Explain the role of Providers in Terraform.
5. What are Resources in Terraform?
6. What is the difference between a resource and a data source in Terraform?
7. What is a Terraform configuration file?
8. What is the Terraform CLI?
9. What is Terraform state? Why is it important?
10. How do you manage Terraform state files?
11. What is a Remote Backend in Terraform?
12. What is Terraform’s ‘.tfstate’ file, and where is it stored?
13. Explain how Terraform ensures consistency between state and resources.
14. Explain terraform init.
15. What does terraform plan do?
16. Describe the terraform apply command.
17. What does terraform destroy do?
18. Explain the purpose of terraform fmt.
19. What is terraform validate used for?
20. What does terraform output do?
21. What is a Terraform Module?
22. How are variables used in Terraform?
23. How do you define variables in Terraform?
24. What are output values in Terraform?
25. How do you reference variables in Terraform?
26. Explain the difference between input and output variables in Terraform.
27. How do you manage secrets in Terraform?
28. What is a Terraform provider lock file?
29. What is Terraform Cloud?
30. How can Terraform be integrated into CI/CD pipelines?
31. How do you handle resource dependencies in Terraform?
32. Explain the concept of the count parameter in Terraform.
33. What is the for_each feature in Terraform, and when would you use it?
34. What are the pros and cons of using Terraform over other IaC tools like Ansible, CloudFormation, or Chef?
35. Explain how Terraform handles state management and how you can secure sensitive data in state files.
36. How does Terraform manage drift detection, and how would you handle drift in a large environment?
37. What are terraform import and terraform taint, and when would you use them?
38. How would you split a large Terraform configuration into multiple modules, and what are the benefits?
39. Explain the purpose of terraform.workspace and its use cases.
40. What is the role of locals in Terraform, and how would you use it in a project?
41. How do you use the count and for_each arguments effectively in Terraform configurations?
42. Explain the difference between depends_on and implicit dependencies in Terraform.
43. How would you version and share Terraform modules across teams?
44. What is the best way to manage Terraform modules in a large-scale, multi-cloud environment?
45. How do you handle cross-account resources in Terraform, especially in AWS?.
46. What are some best practices for writing reusable, maintainable Terraform modules?
47. What are the challenges of managing state files in collaborative environments, and how do you address them?
48. How do you handle state file migration in Terraform, such as moving from local to remote backends?
49. Explain the purpose and benefits of using Terraform Cloud for state management.
50. How would you handle state file corruption or loss in a production environment?
51. How do you manage sensitive data in Terraform, especially in state files?
52. What is the difference between terraform refresh and terraform plan, and when would you use each?
53. What are override files in Terraform, and when would you use them?.
54. Explain how you would handle multi-provider configurations in a single Terraform project.
55. How would you write a custom Terraform provider?
56. How do you use terraform graph, and what insights does it provide?
57. Explain the terraform apply -refresh-only command and when it might be useful.
58. How would you handle breaking changes in Terraform modules or providers?
59. How do you approach dependency management across modules in a large Terraform project?
60. What are some methods to troubleshoot Terraform issues when configurations are not working as expected?
61. How would you implement a CI/CD pipeline with Terraform for continuous deployment?
62. What is Sentinel in Terraform, and how is it used?
63. How do you handle complex conditional logic in Terraform?
64. Explain lifecycle meta-arguments in Terraform and give examples of their use.
65. How do you ensure security compliance in a Terraform-managed environment?
66. What are some of the challenges of using Terraform in a production environment, and how do you address them?
Basic Terraform Concepts
1. What is Terraform?
Answer:
Terraform is an open-source Infrastructure as Code (IaC) tool created by HashiCorp. It allows users to define and provision infrastructure in a declarative configuration language.
2. What is Infrastructure as Code (IaC)?
Answer:
IaC is a practice where infrastructure is provisioned and managed using code rather than manual processes. It allows for automation, consistency, and version control of the infrastructure.
3. What are Terraform’s main components?
Answer:
The main components include Providers, Resources, Modules, Variables, and State.
4. Explain the role of Providers in Terraform.
Answer:
Providers are plugins that allow Terraform to interact with various platforms (e.g., AWS, Azure, Google Cloud). They define the resources for managing services and are configured in the Terraform configuration file.
5. What are Resources in Terraform?
Answer:
Resources are the most fundamental building blocks in Terraform configurations. They represent infrastructure components such as virtual machines, storage, and networks.
6. What is the difference between a resource and a data source in Terraform?
Answer:
Resources are used to create or manage infrastructure, while data sources allow Terraform to fetch data from existing resources without modifying them.
7. What is a Terraform configuration file?
Answer:
The Terraform configuration file (with .tf
extension) contains the code that defines the infrastructure and is written in the HashiCorp Configuration Language (HCL).
8. What is the Terraform CLI?
Answer:
The Terraform Command Line Interface (CLI) is a tool used to interact with Terraform to execute commands like terraform init
, terraform apply
, and terraform plan
.
State Management in Terraform
9. What is Terraform state? Why is it important?
Answer:
Terraform state is a record of infrastructure managed by Terraform. It is essential for tracking resources, mapping configurations to real-world resources, and enabling incremental changes.
10. How do you manage Terraform state files?
Answer:
Terraform state files can be stored locally or in remote backends (e.g., AWS S3, Google Cloud Storage). Remote storage is recommended for collaboration and to prevent loss of state.
11. What is a Remote Backend in Terraform?
Answer:
A remote backend is a backend that stores Terraform state files in a remote location like AWS S3, Azure Blob Storage, or HashiCorp Consul.
12. What is Terraform’s ‘.tfstate’ file, and where is it stored?
Answer:
The .tfstate
file is a JSON file that stores the current state of the infrastructure. It is stored locally by default but can be stored remotely for collaborative environments.
13. Explain how Terraform ensures consistency between state and resources.
Answer:
Terraform compares the state file with actual infrastructure during operations like terraform plan
and terraform apply
to detect configuration drift and make necessary adjustments.
Terraform Commands
14. Explain terraform init
.
Answer:
This command initializes the working directory by downloading necessary provider plugins and preparing the backend configuration for state storage.
15. What does terraform plan
do?
Answer:
terraform plan
creates an execution plan by comparing the current state with the desired state in the configuration files, showing what changes Terraform will make.
16. Describe the terraform apply
command.
Answer:
This command applies the changes required to reach the desired state of the configuration. It prompts the user to approve changes before applying them.
17. What does terraform destroy
do?
Answer:
terraform destroy
is used to delete all infrastructure managed by the Terraform configuration.
18. Explain the purpose of terraform fmt
.
Answer:
terraform fmt
formats the code in Terraform configuration files to make them more readable and maintainable.
19. What is terraform validate
used for?
Answer:
terraform validate
checks the configuration files for syntax errors without executing the plan.
20. What does terraform output
do?
Answer:
terraform output
displays the values of outputs from the last terraform apply
command, which are typically used to expose data outside of the configuration.
Terraform Modules and Variables
21. What is a Terraform Module?
Answer:
A Terraform module is a collection of Terraform files that encapsulates and reuses code for provisioning specific infrastructure. Modules can be local or retrieved from a registry.
22. How are variables used in Terraform?
Answer:
Variables are used to parameterize the configuration, allowing users to pass values for resource properties instead of hardcoding them. They are defined in .tf
files and can be referenced elsewhere in the configuration.
23. How do you define variables in Terraform?
Answer:
Variables are defined using the variable
keyword followed by the variable name and optional default value and description.
For example:
variable "instance_type" {
default = "t2.micro"
description = "EC2 instance type"
}
24. What are output values in Terraform?
Answer:
Output values allow users to access information about resources managed by Terraform. They are helpful for passing values between modules or for displaying essential information to the user.
25. How do you reference variables in Terraform?
Answer:
Variables are referenced using the syntax var.<variable_name>
. For example: var.instance_type
.
26. Explain the difference between input and output variables in Terraform.
Answer:
Input variables are used to pass data into configurations, while output variables are used to extract data from configurations, typically for reference after apply
.
Best Practices and Advanced Concepts
27. How do you manage secrets in Terraform?
Answer:
Secrets can be managed using encrypted remote backends, environment variables, or secret management services (e.g., AWS Secrets Manager). Avoid hardcoding secrets in Terraform files.
28. What is a Terraform provider lock file?
Answer:
The provider lock file (.terraform.lock.hcl
) locks the versions of the providers, ensuring consistency across different environments and runs.
29. What is Terraform Cloud?
Answer:
Terraform Cloud is a SaaS offering by HashiCorp that provides remote state management, collaboration, CI/CD integrations, and policy enforcement for Terraform environments.
30. How can Terraform be integrated into CI/CD pipelines?
Answer:
Terraform can be integrated into CI/CD pipelines using commands like terraform plan
and terraform apply
, allowing for automated deployment of infrastructure with tools like Jenkins, GitHub Actions, or GitLab CI.
31. How do you handle resource dependencies in Terraform?
Answer:
Terraform manages dependencies automatically using its configuration language, but explicit dependencies can be declared using depends_on
if necessary.
32. Explain the concept of the count
parameter in Terraform.
Answer:
The count
parameter allows for the creation of multiple instances of a resource based on a specified number. It enables easy scaling of resources by duplicating them.
33. What is the for_each
feature in Terraform, and when would you use it?
Answer:
The for_each
feature enables iteration over a set of values (e.g., maps or lists) to create multiple instances of a resource. It’s useful when each instance requires specific attributes.
Advanced Terraform Concepts– Terraform interview questions and answers
34. What are the pros and cons of using Terraform over other IaC tools like Ansible, CloudFormation, or Chef?
Answer:
Pros include Terraform’s support for a wide range of providers, declarative syntax, and powerful state management. Cons include lack of imperative-style configuration and limited on-the-fly configuration management, unlike Ansible or Chef.
35. Explain how Terraform handles state management and how you can secure sensitive data in state files.
Answer:
Terraform stores state in a JSON file that maps resources to configurations. For security, sensitive data can be protected by using remote backends with encryption (e.g., AWS S3 with server-side encryption), environment variables, and avoiding outputting secrets directly in Terraform state.
36. How does Terraform manage drift detection, and how would you handle drift in a large environment?
Answer:
Terraform detects drift by comparing the actual state to the desired configuration during terraform plan
and apply
. To handle drift, especially in large environments, regularly scheduled terraform plan
jobs in a CI/CD pipeline can help identify and correct drift.
37. What are terraform import
and terraform taint
, and when would you use them?
Answer:
terraform import
allows you to import existing resources into Terraform’s state, while terraform taint
marks resources for recreation on the next apply
. They’re useful for onboarding unmanaged resources or handling specific resources that require recreation.
38. How would you split a large Terraform configuration into multiple modules, and what are the benefits?
Answer:
Large configurations can be split into reusable modules (e.g., VPC, compute instances, databases) for easier management, readability, and scalability. This approach allows for better separation of concerns, reuse, and parallel development.
39. Explain the purpose of terraform.workspace
and its use cases.
Answer:
terraform.workspace
allows the creation of separate, isolated environments (e.g., dev, staging, prod) within the same configuration. It’s useful for managing multiple deployments with different configurations without duplicating code.
40. What is the role of locals
in Terraform, and how would you use it in a project?
Answer:
locals
define temporary values within a configuration to avoid repetition and improve readability. They’re useful for creating computed values or formatting data that can be reused across multiple resources.
41. How do you use the count
and for_each
arguments effectively in Terraform configurations?
Answer:
count
is useful for duplicating resources, while for_each
is better suited for managing multiple resources with unique identifiers. for_each
is often preferred in production environments for managing dynamic lists or maps.
42. Explain the difference between depends_on
and implicit dependencies in Terraform.
Answer: Terraform handles dependencies implicitly by recognizing resource relationships, but depends_on
can explicitly enforce dependency order when needed, such as for resources that don’t inherently reference each other.
Terraform Modules and Reusability
43. How would you version and share Terraform modules across teams?
Answer:
Modules can be versioned using semantic versioning in a central repository like Terraform Registry, GitHub, or an internal repository. Using module version constraints in configurations ensures consistency and control over updates.
44. What is the best way to manage Terraform modules in a large-scale, multi-cloud environment?
Answer:
In a multi-cloud environment, creating provider-specific modules and managing them in a version-controlled repository can ensure consistency across clouds. Utilizing Terraform’s provider configurations within each module is key for modularity.
45. How do you handle cross-account resources in Terraform, especially in AWS?
Answer:
Cross-account resources in AWS can be managed by assuming roles in other accounts and using Terraform providers configured with those roles. Each provider can be configured with unique alias
values to manage resources across accounts.
46. What are some best practices for writing reusable, maintainable Terraform modules?
Answer:
Best practices include using input variables with defaults, keeping outputs minimal, encapsulating logic within modules, documenting code, and enforcing naming conventions. A well-structured module should be version-controlled and self-contained.
Terraform State Management and Backend
47. What are the challenges of managing state files in collaborative environments, and how do you address them?
Answer:
In collaborative environments, state locking and consistent remote storage are crucial. Using remote backends (e.g., S3 with DynamoDB for locking) and enforcing access controls are recommended to avoid concurrent modifications and ensure security.
48. How do you handle state file migration in Terraform, such as moving from local to remote backends?
Answer:
State migration can be achieved by configuring a new backend in the configuration and running terraform init
, which prompts for migration. Careful planning and backup of the state file are essential to avoid data loss during migration.
49. Explain the purpose and benefits of using Terraform Cloud for state management.
Answer:
Terraform Cloud provides remote state management, collaboration, access controls, and versioning, making it suitable for large teams. It also offers locking and history tracking, which enhance security and coordination.
50. How would you handle state file corruption or loss in a production environment?
Answer:
To handle state corruption, regular backups should be kept, and remote backends with versioned storage are preferred. In case of loss, restoring from a backup or reimporting resources using terraform import
may be necessary.
51. How do you manage sensitive data in Terraform, especially in state files?
Answer:
Sensitive data should be stored in secure backends with encryption, and using sensitive
variables can help hide them in outputs. Alternatively, external secret management solutions (e.g., AWS Secrets Manager) are recommended.
Advanced Terraform CLI and Commands– Terraform interview questions and answers
52. What is the difference between terraform refresh
and terraform plan
, and when would you use each?
Answer:
terraform refresh
updates the state file with real-world infrastructure changes without planning further changes, while terraform plan
creates an execution plan. Use refresh
when you only need to sync the state without applying updates.
53. What are override
files in Terraform, and when would you use them?
Answer:
Override files allow you to customize configurations without modifying the primary .tf
files, which can be useful for temporary changes during testing. They should be used sparingly as they can increase complexity.
54. Explain how you would handle multi-provider configurations in a single Terraform project.
Answer:
Multi-provider configurations are achieved using multiple provider
blocks with alias
attributes. Each resource can then specify which provider to use, making it possible to deploy resources across different clouds.
55. How would you write a custom Terraform provider?
Answer:
A custom provider is written using the Go SDK by implementing required CRUD functions and structuring the provider according to Terraform’s plugin architecture. This requires familiarity with Go and Terraform’s plugin SDK.
56. How do you use terraform graph
, and what insights does it provide?
Answer:
terraform graph
generates a visual representation of the resource dependency graph. It helps identify resource dependencies, possible cycles, and can assist in debugging complex configurations.
57. Explain the terraform apply -refresh-only
command and when it might be useful.
Answer:
This command refreshes the state without making changes to infrastructure. It’s useful for updating the state file after external changes without triggering additional modifications.
Best Practices and Scenarios
58. How would you handle breaking changes in Terraform modules or providers?
Answer:
Breaking changes are managed by version pinning, reviewing release notes, and testing in isolated environments before applying in production. Using version
constraints in the configuration helps avoid automatic updates to incompatible versions.
59. How do you approach dependency management across modules in a large Terraform project?
Answer:
Dependency management can be streamlined by defining outputs and inputs carefully between modules, utilizing depends_on
when necessary, and managing lifecycle policies to control resource creation and destruction.
60. What are some methods to troubleshoot Terraform issues when configurations are not working as expected?
Answer:
Troubleshooting techniques include using terraform plan
and terraform apply
with detailed logs (TF_LOG
environment variable), reviewing dependency graphs with terraform graph
, and leveraging state inspection with terraform state show
.
61. How would you implement a CI/CD pipeline with Terraform for continuous deployment?
Answer:
A CI/CD pipeline can include stages for terraform fmt
, terraform validate
, terraform plan
, and terraform apply
with approval gates. Secure credentials management and automated state management are also essential.
62. What is Sentinel in Terraform, and how is it used?
Answer:
Sentinel is a policy-as-code framework in Terraform Cloud/Enterprise. It allows users to define and enforce policies that control Terraform actions, ensuring compliance and governance.
63. How do you handle complex conditional logic in Terraform?
Answer:
Conditional logic is handled using conditional expressions (? :
syntax) and dynamic blocks. This enables flexibility in resource configuration and allows for complex scenarios based on variable conditions.
64. Explain lifecycle
meta-arguments in Terraform and give examples of their use.
Answer:
lifecycle
meta-arguments control resource behavior, with options like create_before_destroy
, prevent_destroy
, and ignore_changes
. These can be used for managing critical resources and handling rolling updates.
65. How do you ensure security compliance in a Terraform-managed environment?
Answer:
Security compliance can be ensured by using secure backends, secret management tools, regular audits, role-based access controls, and integrating with compliance tools like HashiCorp Sentinel for automated policy checks.
66. What are some of the challenges of using Terraform in a production environment, and how do you address them?
Answer:
Production challenges include managing state at scale, securing secrets, handling configuration drift, and maintaining modularity. Addressing these requires remote state management, automation, policy enforcement, and regular updates to configurations.
Learn More: Carrer Guidance
Rest API interview questions and answers for all levels
Mysql interview questions and answers for experienced
Mysql interview questions and answers for freshers
Python interview questions and answers for data analyst experienced
Python interview questions and answers for data analyst freshers