Are you preparing for Python interview questions for data analyst? You’ve come to the right place! This article will guide you through all essential Python interview questions and answers, focusing on foundational concepts, data manipulation, and the powerful libraries you’ll use in the field. Perfect for freshers, this guide will help you crack your interview.
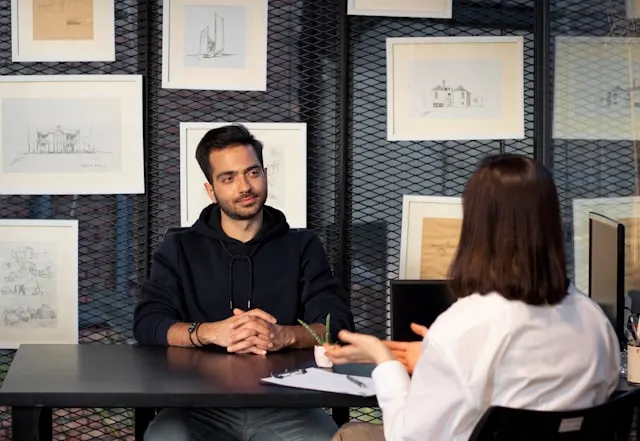
Python interview questions and answers for data analyst freshers
1. What is Python, and why is it popular for data analysis?
2. Explain the difference between lists and tuples in Python?
3. What are Python dictionaries?
4. How do you install packages in Python?
5. What is a lambda function in Python?
6. Explain the concept of list comprehension.
7. How do you handle missing values in Python?
8. What is the purpose of the pandas library?
9. How do you read data from a CSV file using pandas?
10. Explain the difference between loc
and iloc
in pandas.
11. What is NumPy, and why is it important for data analysis?
12. How do you calculate basic statistical measures like mean, median, and mode in Python?
13. How do you handle duplicate values in a DataFrame?
14. What is the purpose of the groupby()
function in pandas?
15. How do you merge two DataFrames in pandas?
16. How would you handle outliers in a dataset?
17. Explain the purpose of the apply()
function in pandas.
18. How do you concatenate DataFrames?
19. Explain how you would convert data types in pandas.
20. How do you create a pivot table in pandas?
21. What libraries can you use for data visualization in Python?
22. How do you create a simple line plot in Matplotlib?
23. How do you add labels and titles to a plot?
24. What is a heatmap, and how do you create one in Seaborn?
25. How do you calculate correlation in a DataFrame?
26. What is a Jupyter Notebook, and why is it useful for data analysis?
27. Explain exception handling in Python with try
and except
.
28. How do you export a DataFrame to a CSV file?
29. What is a Series in pandas?
30. How do you rename columns in a DataFrame?
31. What is vectorization, and why is it useful in pandas and NumPy?
32. How do you perform a time series analysis with pandas?
33. What is broadcasting in NumPy?
34. How would you identify the top 5 most frequent values in a column?
35. How do you handle large datasets in Python that exceed memory?
Basic Python Questions
1. What is Python, and why is it popular for data analysis?
Answer:
Python is a high-level, interpreted programming language known for its simplicity and readability, making it popular for data analysis. Its vast ecosystem of libraries (like pandas, numpy, matplotlib) and community support make it a go-to language for data analysts.
2. Explain the difference between lists and tuples in Python.
Answer:
Lists are mutable, meaning they can be modified after creation (elements can be added or removed), while tuples are immutable, meaning they cannot be changed once created. Lists use square brackets [ ]
, while tuples use parentheses ( )
.
3. What are Python dictionaries?
Answer:
Dictionaries are data structures in Python that store data in key-value pairs, allowing efficient retrieval of values using keys. They are created using curly braces { }
, e.g., {'key': 'value'}
.
4. How do you install packages in Python?
Answer:
You can install packages using the command pip install package_name
, which is Python’s package installer. For example, pip install pandas
.
5. What is a lambda function in Python?
Answer:
A lambda function is an anonymous, small function defined with the lambda
keyword. It can have any number of arguments but only one expression. Example: lambda x: x * 2
creates a function that doubles the input.
6. Explain the concept of list comprehension.
Answer:
List comprehension is a concise way to create lists. For example, [x**2 for x in range(5)]
generates [0, 1, 4, 9, 16]
.
7. How do you handle missing values in Python?
Answer:
Using the pandas
library, you can use functions like .fillna()
to fill missing values and .dropna()
to remove them.
Data Analysis and Libraries
8. What is the purpose of the pandas library?
Answer:
Pandas is used for data manipulation and analysis. It provides data structures like DataFrames and Series, which allow easy data handling, cleaning, and transformation.
9. How do you read data from a CSV file using pandas?
Answer:
Use pd.read_csv('filename.csv')
to load data into a DataFrame. Here, pd
is an alias for the pandas library.
10. Explain the difference between loc
and iloc
in pandas.
Answer:
.loc
is used to access data based on labels (index names), while .iloc
is used to access data based on integer positions.
11. What is NumPy, and why is it important for data analysis?
Answer:
NumPy is a library used for numerical computations. It provides support for arrays and matrices, and functions to perform mathematical operations efficiently.
12. How do you calculate basic statistical measures like mean, median, and mode in Python?
Answer: Using pandas
:
df['column'].mean()
for mean,df['column'].median()
for median, anddf['column'].mode()
for mode.
13. How do you handle duplicate values in a DataFrame?
Answer:
Use df.drop_duplicates()
to remove duplicates and df.duplicated()
to identify them.
14. What is the purpose of the groupby()
function in pandas?
Answer:
groupby()
is used to split data into groups based on criteria, allowing aggregate calculations, transformations, and more within each group.
15. How do you merge two DataFrames in pandas?
Answer:
Use pd.merge(df1, df2, on='common_column')
to merge DataFrames on a common column. Other types of joins (inner, outer, left, right) can be specified using the how
parameter.
Data Cleaning and Transformation
16. How would you handle outliers in a dataset?
Answer:
Outliers can be handled by removing them, transforming data (e.g., log transformation), or using capping methods like z-score or IQR-based filtering.
17. Explain the purpose of the apply()
function in pandas.
Answer:
apply()
is used to apply a function along an axis (rows or columns) in a DataFrame. It’s useful for transformations across rows or columns.
18. How do you concatenate DataFrames?
Answer:
Use pd.concat([df1, df2], axis=0)
for row-wise concatenation or axis=1
for column-wise concatenation.
19. Explain how you would convert data types in pandas.
Answer:
You can use astype()
to convert data types, e.g., df['column'] = df['column'].astype(float)
.
20. How do you create a pivot table in pandas?
Answer:
Use pd.pivot_table(data, values, index, columns)
to create a pivot table, where data
is the DataFrame, values
are the columns to aggregate, and index
and columns
define the axes.
Data Visualization
21. What libraries can you use for data visualization in Python?
Answer:
Libraries like Matplotlib, Seaborn, and Plotly are commonly used for data visualization in Python.
22. How do you create a simple line plot in Matplotlib?
Answer:
Using plt.plot(x, y)
where x
and y
are data points, and then calling plt.show()
.
23. How do you add labels and titles to a plot?
Answer:
Use plt.xlabel()
, plt.ylabel()
, and plt.title()
for x-axis, y-axis, and title respectively.
24. What is a heatmap, and how do you create one in Seaborn?
Answer:
A heatmap shows values across a matrix using color gradients. Use sns.heatmap(data)
where data
is a 2D data array.
Intermediate Questions
25. How do you calculate correlation in a DataFrame?
Answer:
Use df.corr()
to calculate pairwise correlations between columns.
26. What is a Jupyter Notebook, and why is it useful for data analysis?
Answer:
Jupyter Notebook is an open-source, interactive environment for code, visualizations, and markdown. It’s popular for data analysis because it allows code and results to be viewed in one document.
27. Explain exception handling in Python with try
and except
.
Answer:
Exception handling manages errors gracefully. try
runs code that may raise an exception, and except
handles errors if they occur, preventing program crashes.
28. How do you export a DataFrame to a CSV file?
Answer:
Use df.to_csv('filename.csv', index=False)
to export a DataFrame to a CSV file without the index.
29. What is a Series in pandas?
Answer:
A Series is a one-dimensional labeled array, like a single column of data, with an index for each element.
30. How do you rename columns in a DataFrame?
Answer:
Use df.rename(columns={'old_name': 'new_name'})
.
Advanced Concepts
31. What is vectorization, and why is it useful in pandas and NumPy?
Answer:
Vectorization allows for applying operations to entire arrays rather than individual elements, making code faster and more efficient.
32. How do you perform a time series analysis with pandas?
Answer:
Time series analysis can be done by using pd.to_datetime()
to convert dates, setting it as an index, and using methods like resampling and rolling mean.
33. What is broadcasting in NumPy?
Answer:
Broadcasting allows NumPy to perform operations on arrays of different shapes by stretching smaller arrays along dimensions to match the larger array.
Practical Scenario Questions
34. How would you identify the top 5 most frequent values in a column?
Answer:
Use df['column'].value_counts().head(5)
to get the top 5 most frequent values in a column.
35. How do you handle large datasets in Python that exceed memory?
Answer:
Use libraries like Dask
for distributed processing, chunking
while reading files, or work with sampling techniques to analyze subsets of data.
These questions cover the core Python concepts, data manipulation with libraries, data cleaning, and visualization skills essential for data analysts, providing a solid foundation for a fresher in the data analytics field.
Learn More: Carrer Guidance [Python interview questions and answers for data analyst freshers]
Splunk interview questions and answers
React native interview questions and answers for freshers and experienced
Automation Testing Interview Questions and answers for Experienced
Automation Testing Interview Questions and answers for Freshers
SAS Interview Questions and answers- Basic to Advanced