In software development, Java remains a cornerstone language, widely used for building robust, scalable applications. For developers with a decade of experience, Java interviews can be a rigorous test of both their technical skills and their ability to apply these skills in real-world scenarios. This article aims to provide a comprehensive guide to Java interview questions and answers tailored for candidates with 10 years of experience.
Here is a list of 45+ Java interview questions for with 10 years of experience, along with detailed answers. These questions cover advanced topics in Java, concurrency, performance, design patterns, and more.
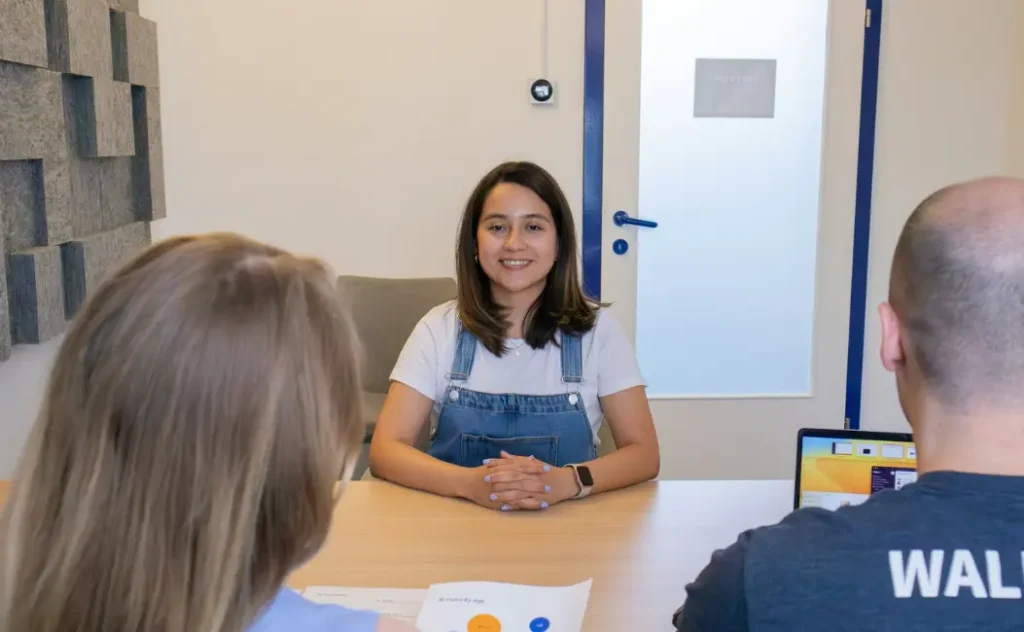
Java interview questions and answers for 10 years experience
1. What are the new features introduced in Java 8?
2. What is the difference between Callable
and Runnable
?
3. Explain the Java Memory Model (JMM).
4. What is a volatile variable in Java, and when would you use it?
5. How does the synchronized
keyword work in Java?
6. What is the difference between HashMap
and ConcurrentHashMap
?
7. Explain the Java Stream
API introduced in Java 8.
8. How does garbage collection work in Java?
9. What is the difference between strong, weak, soft, and phantom references in Java?
10. What is the difference between ReentrantLock
and synchronized
?
11. What is the Fork/Join framework in Java?
12. What is the difference between checked and unchecked exceptions?
13. What is the purpose of final
, finally
, and finalize
?
14. What are the differences between Comparable
and Comparator
?
15. What is a ThreadLocal
in Java?
16. Explain the term “immutability”. How can you make a class immutable in Java?
17. Explain the double-checked locking idiom in Singleton pattern.
18. Explain wait()
, notify()
, and notifyAll()
methods in Java.
19. What is Java Reflection?
20. Explain the term “deadlock” and how to prevent it.
22: What are the new features introduced in Java 8, 9, 10, 11, and subsequent versions? How have they improved Java development?
23: Describe the differences between CompletableFuture and Future. How does CompletableFuture enhance asynchronous programming in Java?
24: What is the Java Memory Leak? How can you identify and fix memory leaks in a Java application?
25: Describe the Java ClassLoader system. How can you implement a custom ClassLoader?
26: Explain the Java Memory Model’s happens-before relationship and its importance in concurrent programming.
27: What are the differences between checked and unchecked exceptions in Java? When would you choose one over the other in your designs?
28: Describe the Fork/Join framework in Java. How does it differ from the standard ExecutorService?
29: What is the Reactive Streams specification in Java? How does it relate to the Java 9 Flow API?
30: Explain the concept of method references in Java 8+. How do they relate to lambda expressions?
31: What are the differences between abstract classes and interfaces in Java 8 and later versions?
32: Describe the Garbage-First (G1) garbage collector. How does it differ from other GC algorithms?
33: Explain the concept of soft leaks in Java and how they differ from traditional memory leaks.
34: How does the Java module system (introduced in Java 9) improve application development and deployment?
35: Describe the concept of ThreadLocal in Java. What are its use cases and potential pitfalls?
36: What are the differences between volatile and synchronized keywords in Java?
37: Explain the concept of CompletionStage in Java. How does it enhance asynchronous programming?
38: What are the best practices for implementing hashCode() and equals() methods in Java?
39: Describe the Java Virtual Thread feature (introduced in Java 19 as a preview). How does it differ from platform threads?
40: What are the differences between static and default methods in interfaces? When would you choose one over the other?
41: Explain the concept of off-heap memory in Java. What are its use cases and how can it be managed?
42: Describe the Java Flight Recorder (JFR) and Java Mission Control (JMC). How can they be used for performance analysis and troubleshooting?
43. What is the CompletableFuture
class in Java?
44. What is a JavaAgent
? How is it used?
45. Explain the role of Phaser
in Java.
46. What is java.lang.instrument
and how does it relate to the JVM?
47. Explain the concept of a Java WeakHashMap
.
1. What are the new features introduced in Java 8?
Answer: Key Java 8 features include:
- Lambda Expressions: Allow functional programming by passing methods as arguments.
- Streams API: Provides a functional approach to process sequences of elements.
- Default Methods: Interface methods with default implementation.
- Optional: Avoids null references.
- Method References: Refers to methods using
::
. - Functional Interfaces: An interface with only one abstract method (e.g.,
Runnable
). - Nashorn JavaScript Engine: Integrates JavaScript engine.
2. What is the difference between Callable
and Runnable
?
Answer:
Runnable
does not return a result or throw checked exceptions, whereasCallable
returns a result and can throw checked exceptions.Callable
is used with executors that can return aFuture
.
3. Explain the Java Memory Model (JMM).
Answer: The Java Memory Model defines how threads interact through memory, and it ensures visibility and ordering of variables between threads. JMM guarantees:
- Visibility: Changes made by one thread are visible to others.
- Happens-Before Relationship: Ensures that memory writes by one thread are visible to another thread.
4. What is a volatile variable in Java, and when would you use it?
Answer:
A volatile variable ensures that the value of the variable is read from the main memory and not from the thread’s local cache. It’s used to guarantee visibility of changes across threads for simple flags, counters, etc., but does not guarantee atomicity.
5. How does the synchronized
keyword work in Java?
Answer:
synchronized
ensures mutual exclusion, i.e., only one thread can access a block of code or method at a time. It also establishes a happens-before relationship, ensuring visibility of changes in variables made by one thread to others. It can be applied at method or block level.
6. What is the difference between HashMap
and ConcurrentHashMap
?
Answer:
- HashMap is not thread-safe and may lead to data inconsistency when modified by multiple threads.
- ConcurrentHashMap is thread-safe, using a segmented locking mechanism (in Java 7) or a CAS-based mechanism (in Java 8) to allow concurrent updates without locking the entire map.
7. Explain the Java Stream
API introduced in Java 8.
Answer:
The Stream API is used for processing collections of objects in a declarative way. It supports operations like map
, filter
, reduce
, and is designed to work on sequences of elements (streams), supporting both sequential and parallel execution.
8. How does garbage collection work in Java?
Answer:
Java’s garbage collector automatically reclaims memory by removing objects that are no longer referenced. There are different types of garbage collectors:
- Serial GC: Single-threaded.
- Parallel GC: Multiple threads used for garbage collection.
- CMS (Concurrent Mark-Sweep) GC: Focuses on minimizing pause times.
- G1 GC: Divides heap into regions and works to balance memory reclamation with performance.
9. What is the difference between strong, weak, soft, and phantom references in Java?
Answer:
- Strong Reference: The default type, objects are not eligible for GC as long as a strong reference exists.
- Weak Reference: Objects can be reclaimed by GC if only weak references exist.
- Soft Reference: Similar to weak references but more lenient, GC will collect these if memory is needed.
- Phantom Reference: Used to do some cleanup before GC, accessed only through a
ReferenceQueue
.
10. What is the difference between ReentrantLock
and synchronized
?
Answer:
ReentrantLock
offers advanced features such as timeouts for lock acquisition, ability to check if the lock is held, and fairness policy.synchronized
is simpler but does not provide such flexibility.
11. What is the Fork/Join framework in Java?
Answer:
The Fork/Join framework introduced in Java 7 is designed for parallelism. It allows splitting tasks into smaller subtasks (fork
) and combining the results (join
). It is optimized for divide-and-conquer algorithms.
12. What is the difference between checked and unchecked exceptions?
Answer:
- Checked Exceptions: These are checked at compile-time (e.g.,
IOException
). You must either handle or declare them. - Unchecked Exceptions: These are checked at runtime (e.g.,
NullPointerException
). They can be handled but are not enforced.
13. What is the purpose of final
, finally
, and finalize
?
Answer:
- final: Keyword used to declare constants, immutable methods, or to prevent inheritance.
- finally: A block that executes regardless of exceptions in try-catch.
- finalize: A method called by GC before an object is garbage collected.
14. What are the differences between Comparable
and Comparator
?
Answer:
Comparable
defines a natural ordering for objects (e.g.,compareTo
method in the same class).Comparator
allows defining multiple ways of ordering objects and is external to the object (e.g.,compare
method in a separate class).
15. What is a ThreadLocal
in Java?
Answer:
ThreadLocal
provides each thread with its own independently initialized variable. It helps in thread isolation, especially useful in maintaining user session or transaction contexts.
16. Explain the term “immutability”. How can you make a class immutable in Java?
Answer: Immutability means that once an object is created, it cannot be changed. To make a class immutable:
- Declare the class as
final
. - Make all fields
private final
. - Do not provide any setters.
- Ensure that methods do not expose mutable objects (e.g., by returning deep copies).
17. Explain the double-checked locking idiom in Singleton pattern.
Answer: Double-checked locking ensures that a Singleton instance is only created once and is thread-safe. The idiom is:
public class Singleton {
private static volatile Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
synchronized (Singleton.class) {
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
}
18. Explain wait()
, notify()
, and notifyAll()
methods in Java.
Answer: These methods are used for inter-thread communication:
- wait(): A thread releases the lock and waits until notified.
- notify(): Wakes up one waiting thread.
- notifyAll(): Wakes up all waiting threads.
19. What is Java Reflection?
Answer:
Reflection allows Java code to inspect and manipulate classes, fields, methods, and constructors at runtime. It is used for creating frameworks, debugging, and testing. However, it has performance and security implications.
20. Explain the term “deadlock” and how to prevent it.
Answer:
Deadlock occurs when two or more threads are waiting on each other to release resources, leading to an infinite wait. Prevention strategies include:
- Avoiding circular dependencies.
- Using lock ordering.
- Using
tryLock()
with timeouts.
21: Explain the concept of Java memory model and how it relates to concurrency.
Answer:
The Java Memory Model (JMM) defines how the Java virtual machine (JVM) works with the computer’s memory. It specifies how and when different threads can see values written to shared variables by other threads, and how to synchronize access to shared variables. Key concepts include happens-before relationship, volatile keyword, and memory barriers.
22: Describe the differences between CompletableFuture and Future. How does CompletableFuture enhance asynchronous programming in Java?
Answer:
CompletableFuture extends the Future interface and provides a more robust and flexible way to handle asynchronous computations. Unlike Future, CompletableFuture allows chaining of asynchronous operations, combining multiple futures, and handling exceptions more gracefully. It also provides a way to manually complete the future, making it useful for scenarios where the result might come from multiple sources.
23: How does Garbage Collection work in Java? Explain different GC algorithms and how to tune GC performance.
Answer:
Garbage Collection in Java automatically frees memory by removing objects that are no longer reachable. Common GC algorithms include:
- Serial GC
- Parallel GC
- Concurrent Mark Sweep (CMS)
- G1 (Garbage First)
ZGC (Z Garbage Collector) Tuning involves adjusting heap size, choosing the appropriate GC algorithm, and fine-tuning GC parameters based on application needs and performance metrics.
24: Explain the concept of reflection in Java. What are its use cases and potential drawbacks?
Answer:
Reflection allows examination or modification of the runtime behavior of applications running in the JVM. It can be used to:
- Inspect classes, interfaces, fields, and methods at runtime
- Instantiate objects and invoke methods dynamically
Access and modify private fields and methods Drawbacks include reduced performance, security restrictions, and potential for breaking encapsulation.
25: What is the Java Memory Leak? How can you identify and fix memory leaks in a Java application?
Answer:
A Java memory leak occurs when objects are no longer used by the application but are still referenced, preventing garbage collection. Identification methods include:
- Using profiling tools (e.g., VisualVM, JProfiler)
- Analyzing heap dumps
Monitoring memory usage over time Fixes typically involve finding and removing unnecessary object references, often in static fields, caches, or improperly managed resources.
26: What are Java agents? How can they be used for application monitoring and instrumentation?
Answer:
Java agents are programs that can instrument or modify Java bytecode at runtime. They can be used for:
- Performance monitoring
- Logging
- Debugging
Profiling Java agents are typically implemented by creating a class with a premain or agentmain method and packaging it as a JAR file with the appropriate manifest entries.
27: Explain the Java Memory Model’s happens-before relationship and its importance in concurrent programming.
Answer:
The happens-before relationship in the Java Memory Model defines a partial ordering of program actions, ensuring that memory operations in one thread are correctly observed by another thread. It’s crucial for writing correct concurrent programs, as it guarantees visibility of changes across threads without excessive synchronization.
28: What is the Reactive Streams specification in Java? How does it relate to the Java 9 Flow API?
Answer:
Reactive Streams is a specification for asynchronous stream processing with non-blocking back pressure. The Java 9 Flow API is the standard implementation of this specification, providing interfaces like Publisher, Subscriber, and Subscription. It enables building reactive, scalable applications that can handle back pressure effectively.
29: Explain the concept of method references in Java 8+. How do they relate to lambda expressions?
Answer:
Method references are shorthand notations of lambda expressions to call methods. They can be used to refer to static methods, instance methods, or constructors. There are four types:
- Reference to a static method
- Reference to an instance method of a particular object
- Reference to an instance method of an arbitrary object of a particular type
- Reference to a constructor They provide a more readable and concise way to express simple lambda expressions.
30: What are the differences between abstract classes and interfaces in Java 8 and later versions?
Answer: Key differences include:
- Abstract classes can have state, interfaces cannot (except static final fields)
- A class can extend only one abstract class but implement multiple interfaces
- Abstract classes can have constructors, interfaces cannot
- Interfaces can have default and static methods (since Java 8)
Interfaces can have private methods (since Java 9) Choose abstract classes when you want to share code among several closely related classes. Use interfaces to define a contract for a set of classes to implement, especially when these classes are not closely related.
31: Describe the Garbage-First (G1) garbage collector. How does it differ from other GC algorithms?
Answer:: G1 is a server-style garbage collector designed for multiprocessor machines with large memories. Key features:
- Concurrent and parallel phases
- Incremental compaction
- Predictable pause times
Regions-based heap layout It differs from other algorithms by its ability to prioritize garbage collection in areas of the heap that are likely to reclaim the most space, hence “Garbage-First”.
32: What is bytecode instrumentation? How can it be used to enhance application performance or add functionality?
Answer:
Bytecode instrumentation is the process of modifying Java bytecode after it has been compiled. It can be used to:
- Add logging or monitoring code
- Implement aspect-oriented programming features
- Optimize performance by modifying compiled code
- Add security checks or enforce policies Tools like ASM, Javassist, or ByteBuddy can be used for bytecode instrumentation.
33: Explain the concept of soft leaks in Java and how they differ from traditional memory leaks.
Answer:
Soft leaks occur when objects are not garbage collected despite not being strictly necessary for the application. Unlike traditional memory leaks, the objects in soft leaks can be garbage collected if memory pressure increases. Common causes include oversized caches or pools. They can be addressed by using weak references or time-based eviction policies in caches.
34: How does the Java module system (introduced in Java 9) improve application development and deployment?
Answer: The Java module system:
- Improves encapsulation by allowing explicit declaration of public APIs
- Reduces JAR hell and classpath issues
- Enables creation of smaller, more efficient runtimes with jlink
- Improves security by reducing the attack surface
- Enhances performance through better organization of packages
35: What are the differences between volatile and synchronized keywords in Java?
Answer:
- volatile ensures visibility of changes to variables across threads, but doesn’t provide atomicity
- synchronized provides both visibility and atomicity, but at a higher performance cost
- volatile is field-level, synchronized can be applied to methods or blocks
- volatile doesn’t cause thread contention, synchronized can
36: Explain the concept of CompletionStage in Java. How does it enhance asynchronous programming?
Answer: CompletionStage represents a stage of a possibly asynchronous computation. It allows for:
- Chaining of asynchronous operations
- Combining results from multiple asynchronous computations
- Handling exceptions in asynchronous flows
Transforming results asynchronously It provides a more flexible and powerful model for asynchronous programming compared to simple Future objects.
37: What are the best practices for implementing hashCode() and equals() methods in Java?
Answer: Best practices include:
- If you override equals(), you must override hashCode()
- equals() should be consistent (multiple invocations should return the same result)
- equals() should be symmetric (a.equals(b) should return the same as b.equals(a))
- hashCode() should use the same fields as equals()
- Consider using Objects.hash() for generating hash codes
- For mutable objects, use only fields that are not expected to change
38: Describe the Java Virtual Thread feature (introduced in Java 19 as a preview). How does it differ from platform threads?
Answer:
Virtual threads are lightweight threads that are cheap to create and maintain. Key differences:
- Managed by the JVM, not the OS
- Can support millions of concurrent threads
- Use a small stack and grow/shrink as needed
Automatically yield when blocked on I/O operations They enable a simpler programming model for concurrent applications, especially those with many concurrent I/O operations.
39: What are the differences between static and default methods in interfaces? When would you choose one over the other?
Answer:
- Static methods belong to the interface, not implementing classes
- Default methods are inherited by implementing classes
- Static methods cannot be overridden, default methods can
- Use static methods for utility functions related to the interface
- Use default methods to add new methods to interfaces without breaking existing implementations
40: Explain the concept of off-heap memory in Java. What are its use cases and how can it be managed?
Answer: Off-heap memory refers to memory allocated outside the Java heap. Use cases include:
- Large data sets that would cause excessive GC overhead if stored on-heap
- Memory-mapped files
Direct buffers for high-performance I/O It can be managed using libraries like ByteBuffer or specialized off-heap libraries. Care must be taken to manually free this memory when it’s no longer needed.
41: Describe the Java Flight Recorder (JFR) and Java Mission Control (JMC). How can they be used for performance analysis and troubleshooting?
Answer:: JFR is a profiling and event collection framework built into the JVM. JMC is a graphical tool for analyzing JFR data. Together, they provide:
- Low-overhead profiling
- Detailed performance metrics
- Thread analysis
- Memory leak detection
GC behavior analysis They are powerful tools for identifying performance bottlenecks and analyzing production issues with minimal impact.
42. What is the CompletableFuture
class in Java?
Answer:
CompletableFuture
is part of the java.util.concurrent
package and represents a future result of an asynchronous computation. It supports combinator operations like thenApply
, thenCombine
, and thenAccept
to chain tasks without blocking threads.
43. What is a JavaAgent
? How is it used?
Answer:
A Java Agent is a special tool that can modify bytecode at runtime or before a class is loaded. It is typically used in monitoring tools, profiling, and aspect-oriented programming. Agents are specified using the -javaagent
flag.
44. Explain the role of Phaser
in Java.
Answer:
Phaser
is a synchronization aid that allows threads to wait for others at a specific phase or step in a task. Unlike CountDownLatch
or CyclicBarrier
, it supports more flexible phase coordination and dynamic thread registration/deregistration.
45. What is java.lang.instrument
and how does it relate to the JVM?
Answer:
The java.lang.instrument
package provides APIs to modify class behavior at runtime using agents. It can redefine classes, gather profiling information, and is heavily used in instrumentation and monitoring tools.
46. Explain the concept of a Java WeakHashMap
.
Answer:
WeakHashMap
uses weak references for keys, meaning that if a key is no longer referenced elsewhere, it is eligible for garbage collection, even if it is still present in the map. It is useful in cases where you want cache-like behavior without preventing garbage collection.
These questions are designed to cover a wide array of topics, ensuring that candidates are tested on advanced aspects of Java, including concurrency, memory management, design patterns, and Java’s newer features.
Learn More: Carrer Guidance
1. Tableau interview questions and answers
2. LWC interview questions and answers
3. Nodejs interview questions and answers
4. Flutter Interview Questions and Answers
5. Active Directory Interview Questions and Answers for Fresher
6. Active directory interview questions answers for experienced